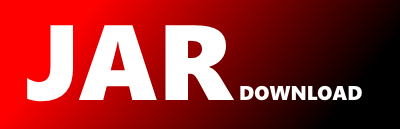
com.amazonaws.services.bedrock.model.GetEvaluationJobResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-bedrock Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.bedrock.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetEvaluationJobResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The name of the model evaluation job.
*
*/
private String jobName;
/**
*
* The status of the model evaluation job.
*
*/
private String status;
/**
*
* The Amazon Resource Name (ARN) of the model evaluation job.
*
*/
private String jobArn;
/**
*
* The description of the model evaluation job.
*
*/
private String jobDescription;
/**
*
* The Amazon Resource Name (ARN) of the IAM service role used in the model evaluation job.
*
*/
private String roleArn;
/**
*
* The Amazon Resource Name (ARN) of the customer managed key specified when the model evaluation job was created.
*
*/
private String customerEncryptionKeyId;
/**
*
* The type of model evaluation job.
*
*/
private String jobType;
/**
*
* Contains details about the type of model evaluation job, the metrics used, the task type selected, the datasets
* used, and any custom metrics you defined.
*
*/
private EvaluationConfig evaluationConfig;
/**
*
* Details about the models you specified in your model evaluation job.
*
*/
private EvaluationInferenceConfig inferenceConfig;
/**
*
* Amazon S3 location for where output data is saved.
*
*/
private EvaluationOutputDataConfig outputDataConfig;
/**
*
* When the model evaluation job was created.
*
*/
private java.util.Date creationTime;
/**
*
* When the model evaluation job was last modified.
*
*/
private java.util.Date lastModifiedTime;
/**
*
* An array of strings the specify why the model evaluation job has failed.
*
*/
private java.util.List failureMessages;
/**
*
* The name of the model evaluation job.
*
*
* @param jobName
* The name of the model evaluation job.
*/
public void setJobName(String jobName) {
this.jobName = jobName;
}
/**
*
* The name of the model evaluation job.
*
*
* @return The name of the model evaluation job.
*/
public String getJobName() {
return this.jobName;
}
/**
*
* The name of the model evaluation job.
*
*
* @param jobName
* The name of the model evaluation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEvaluationJobResult withJobName(String jobName) {
setJobName(jobName);
return this;
}
/**
*
* The status of the model evaluation job.
*
*
* @param status
* The status of the model evaluation job.
* @see EvaluationJobStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the model evaluation job.
*
*
* @return The status of the model evaluation job.
* @see EvaluationJobStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the model evaluation job.
*
*
* @param status
* The status of the model evaluation job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EvaluationJobStatus
*/
public GetEvaluationJobResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the model evaluation job.
*
*
* @param status
* The status of the model evaluation job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EvaluationJobStatus
*/
public GetEvaluationJobResult withStatus(EvaluationJobStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the model evaluation job.
*
*
* @param jobArn
* The Amazon Resource Name (ARN) of the model evaluation job.
*/
public void setJobArn(String jobArn) {
this.jobArn = jobArn;
}
/**
*
* The Amazon Resource Name (ARN) of the model evaluation job.
*
*
* @return The Amazon Resource Name (ARN) of the model evaluation job.
*/
public String getJobArn() {
return this.jobArn;
}
/**
*
* The Amazon Resource Name (ARN) of the model evaluation job.
*
*
* @param jobArn
* The Amazon Resource Name (ARN) of the model evaluation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEvaluationJobResult withJobArn(String jobArn) {
setJobArn(jobArn);
return this;
}
/**
*
* The description of the model evaluation job.
*
*
* @param jobDescription
* The description of the model evaluation job.
*/
public void setJobDescription(String jobDescription) {
this.jobDescription = jobDescription;
}
/**
*
* The description of the model evaluation job.
*
*
* @return The description of the model evaluation job.
*/
public String getJobDescription() {
return this.jobDescription;
}
/**
*
* The description of the model evaluation job.
*
*
* @param jobDescription
* The description of the model evaluation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEvaluationJobResult withJobDescription(String jobDescription) {
setJobDescription(jobDescription);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM service role used in the model evaluation job.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM service role used in the model evaluation job.
*/
public void setRoleArn(String roleArn) {
this.roleArn = roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM service role used in the model evaluation job.
*
*
* @return The Amazon Resource Name (ARN) of the IAM service role used in the model evaluation job.
*/
public String getRoleArn() {
return this.roleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM service role used in the model evaluation job.
*
*
* @param roleArn
* The Amazon Resource Name (ARN) of the IAM service role used in the model evaluation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEvaluationJobResult withRoleArn(String roleArn) {
setRoleArn(roleArn);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the customer managed key specified when the model evaluation job was created.
*
*
* @param customerEncryptionKeyId
* The Amazon Resource Name (ARN) of the customer managed key specified when the model evaluation job was
* created.
*/
public void setCustomerEncryptionKeyId(String customerEncryptionKeyId) {
this.customerEncryptionKeyId = customerEncryptionKeyId;
}
/**
*
* The Amazon Resource Name (ARN) of the customer managed key specified when the model evaluation job was created.
*
*
* @return The Amazon Resource Name (ARN) of the customer managed key specified when the model evaluation job was
* created.
*/
public String getCustomerEncryptionKeyId() {
return this.customerEncryptionKeyId;
}
/**
*
* The Amazon Resource Name (ARN) of the customer managed key specified when the model evaluation job was created.
*
*
* @param customerEncryptionKeyId
* The Amazon Resource Name (ARN) of the customer managed key specified when the model evaluation job was
* created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEvaluationJobResult withCustomerEncryptionKeyId(String customerEncryptionKeyId) {
setCustomerEncryptionKeyId(customerEncryptionKeyId);
return this;
}
/**
*
* The type of model evaluation job.
*
*
* @param jobType
* The type of model evaluation job.
* @see EvaluationJobType
*/
public void setJobType(String jobType) {
this.jobType = jobType;
}
/**
*
* The type of model evaluation job.
*
*
* @return The type of model evaluation job.
* @see EvaluationJobType
*/
public String getJobType() {
return this.jobType;
}
/**
*
* The type of model evaluation job.
*
*
* @param jobType
* The type of model evaluation job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EvaluationJobType
*/
public GetEvaluationJobResult withJobType(String jobType) {
setJobType(jobType);
return this;
}
/**
*
* The type of model evaluation job.
*
*
* @param jobType
* The type of model evaluation job.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EvaluationJobType
*/
public GetEvaluationJobResult withJobType(EvaluationJobType jobType) {
this.jobType = jobType.toString();
return this;
}
/**
*
* Contains details about the type of model evaluation job, the metrics used, the task type selected, the datasets
* used, and any custom metrics you defined.
*
*
* @param evaluationConfig
* Contains details about the type of model evaluation job, the metrics used, the task type selected, the
* datasets used, and any custom metrics you defined.
*/
public void setEvaluationConfig(EvaluationConfig evaluationConfig) {
this.evaluationConfig = evaluationConfig;
}
/**
*
* Contains details about the type of model evaluation job, the metrics used, the task type selected, the datasets
* used, and any custom metrics you defined.
*
*
* @return Contains details about the type of model evaluation job, the metrics used, the task type selected, the
* datasets used, and any custom metrics you defined.
*/
public EvaluationConfig getEvaluationConfig() {
return this.evaluationConfig;
}
/**
*
* Contains details about the type of model evaluation job, the metrics used, the task type selected, the datasets
* used, and any custom metrics you defined.
*
*
* @param evaluationConfig
* Contains details about the type of model evaluation job, the metrics used, the task type selected, the
* datasets used, and any custom metrics you defined.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEvaluationJobResult withEvaluationConfig(EvaluationConfig evaluationConfig) {
setEvaluationConfig(evaluationConfig);
return this;
}
/**
*
* Details about the models you specified in your model evaluation job.
*
*
* @param inferenceConfig
* Details about the models you specified in your model evaluation job.
*/
public void setInferenceConfig(EvaluationInferenceConfig inferenceConfig) {
this.inferenceConfig = inferenceConfig;
}
/**
*
* Details about the models you specified in your model evaluation job.
*
*
* @return Details about the models you specified in your model evaluation job.
*/
public EvaluationInferenceConfig getInferenceConfig() {
return this.inferenceConfig;
}
/**
*
* Details about the models you specified in your model evaluation job.
*
*
* @param inferenceConfig
* Details about the models you specified in your model evaluation job.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEvaluationJobResult withInferenceConfig(EvaluationInferenceConfig inferenceConfig) {
setInferenceConfig(inferenceConfig);
return this;
}
/**
*
* Amazon S3 location for where output data is saved.
*
*
* @param outputDataConfig
* Amazon S3 location for where output data is saved.
*/
public void setOutputDataConfig(EvaluationOutputDataConfig outputDataConfig) {
this.outputDataConfig = outputDataConfig;
}
/**
*
* Amazon S3 location for where output data is saved.
*
*
* @return Amazon S3 location for where output data is saved.
*/
public EvaluationOutputDataConfig getOutputDataConfig() {
return this.outputDataConfig;
}
/**
*
* Amazon S3 location for where output data is saved.
*
*
* @param outputDataConfig
* Amazon S3 location for where output data is saved.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEvaluationJobResult withOutputDataConfig(EvaluationOutputDataConfig outputDataConfig) {
setOutputDataConfig(outputDataConfig);
return this;
}
/**
*
* When the model evaluation job was created.
*
*
* @param creationTime
* When the model evaluation job was created.
*/
public void setCreationTime(java.util.Date creationTime) {
this.creationTime = creationTime;
}
/**
*
* When the model evaluation job was created.
*
*
* @return When the model evaluation job was created.
*/
public java.util.Date getCreationTime() {
return this.creationTime;
}
/**
*
* When the model evaluation job was created.
*
*
* @param creationTime
* When the model evaluation job was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEvaluationJobResult withCreationTime(java.util.Date creationTime) {
setCreationTime(creationTime);
return this;
}
/**
*
* When the model evaluation job was last modified.
*
*
* @param lastModifiedTime
* When the model evaluation job was last modified.
*/
public void setLastModifiedTime(java.util.Date lastModifiedTime) {
this.lastModifiedTime = lastModifiedTime;
}
/**
*
* When the model evaluation job was last modified.
*
*
* @return When the model evaluation job was last modified.
*/
public java.util.Date getLastModifiedTime() {
return this.lastModifiedTime;
}
/**
*
* When the model evaluation job was last modified.
*
*
* @param lastModifiedTime
* When the model evaluation job was last modified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEvaluationJobResult withLastModifiedTime(java.util.Date lastModifiedTime) {
setLastModifiedTime(lastModifiedTime);
return this;
}
/**
*
* An array of strings the specify why the model evaluation job has failed.
*
*
* @return An array of strings the specify why the model evaluation job has failed.
*/
public java.util.List getFailureMessages() {
return failureMessages;
}
/**
*
* An array of strings the specify why the model evaluation job has failed.
*
*
* @param failureMessages
* An array of strings the specify why the model evaluation job has failed.
*/
public void setFailureMessages(java.util.Collection failureMessages) {
if (failureMessages == null) {
this.failureMessages = null;
return;
}
this.failureMessages = new java.util.ArrayList(failureMessages);
}
/**
*
* An array of strings the specify why the model evaluation job has failed.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setFailureMessages(java.util.Collection)} or {@link #withFailureMessages(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param failureMessages
* An array of strings the specify why the model evaluation job has failed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEvaluationJobResult withFailureMessages(String... failureMessages) {
if (this.failureMessages == null) {
setFailureMessages(new java.util.ArrayList(failureMessages.length));
}
for (String ele : failureMessages) {
this.failureMessages.add(ele);
}
return this;
}
/**
*
* An array of strings the specify why the model evaluation job has failed.
*
*
* @param failureMessages
* An array of strings the specify why the model evaluation job has failed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetEvaluationJobResult withFailureMessages(java.util.Collection failureMessages) {
setFailureMessages(failureMessages);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getJobName() != null)
sb.append("JobName: ").append(getJobName()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getJobArn() != null)
sb.append("JobArn: ").append(getJobArn()).append(",");
if (getJobDescription() != null)
sb.append("JobDescription: ").append("***Sensitive Data Redacted***").append(",");
if (getRoleArn() != null)
sb.append("RoleArn: ").append(getRoleArn()).append(",");
if (getCustomerEncryptionKeyId() != null)
sb.append("CustomerEncryptionKeyId: ").append(getCustomerEncryptionKeyId()).append(",");
if (getJobType() != null)
sb.append("JobType: ").append(getJobType()).append(",");
if (getEvaluationConfig() != null)
sb.append("EvaluationConfig: ").append(getEvaluationConfig()).append(",");
if (getInferenceConfig() != null)
sb.append("InferenceConfig: ").append(getInferenceConfig()).append(",");
if (getOutputDataConfig() != null)
sb.append("OutputDataConfig: ").append(getOutputDataConfig()).append(",");
if (getCreationTime() != null)
sb.append("CreationTime: ").append(getCreationTime()).append(",");
if (getLastModifiedTime() != null)
sb.append("LastModifiedTime: ").append(getLastModifiedTime()).append(",");
if (getFailureMessages() != null)
sb.append("FailureMessages: ").append(getFailureMessages());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetEvaluationJobResult == false)
return false;
GetEvaluationJobResult other = (GetEvaluationJobResult) obj;
if (other.getJobName() == null ^ this.getJobName() == null)
return false;
if (other.getJobName() != null && other.getJobName().equals(this.getJobName()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getJobArn() == null ^ this.getJobArn() == null)
return false;
if (other.getJobArn() != null && other.getJobArn().equals(this.getJobArn()) == false)
return false;
if (other.getJobDescription() == null ^ this.getJobDescription() == null)
return false;
if (other.getJobDescription() != null && other.getJobDescription().equals(this.getJobDescription()) == false)
return false;
if (other.getRoleArn() == null ^ this.getRoleArn() == null)
return false;
if (other.getRoleArn() != null && other.getRoleArn().equals(this.getRoleArn()) == false)
return false;
if (other.getCustomerEncryptionKeyId() == null ^ this.getCustomerEncryptionKeyId() == null)
return false;
if (other.getCustomerEncryptionKeyId() != null && other.getCustomerEncryptionKeyId().equals(this.getCustomerEncryptionKeyId()) == false)
return false;
if (other.getJobType() == null ^ this.getJobType() == null)
return false;
if (other.getJobType() != null && other.getJobType().equals(this.getJobType()) == false)
return false;
if (other.getEvaluationConfig() == null ^ this.getEvaluationConfig() == null)
return false;
if (other.getEvaluationConfig() != null && other.getEvaluationConfig().equals(this.getEvaluationConfig()) == false)
return false;
if (other.getInferenceConfig() == null ^ this.getInferenceConfig() == null)
return false;
if (other.getInferenceConfig() != null && other.getInferenceConfig().equals(this.getInferenceConfig()) == false)
return false;
if (other.getOutputDataConfig() == null ^ this.getOutputDataConfig() == null)
return false;
if (other.getOutputDataConfig() != null && other.getOutputDataConfig().equals(this.getOutputDataConfig()) == false)
return false;
if (other.getCreationTime() == null ^ this.getCreationTime() == null)
return false;
if (other.getCreationTime() != null && other.getCreationTime().equals(this.getCreationTime()) == false)
return false;
if (other.getLastModifiedTime() == null ^ this.getLastModifiedTime() == null)
return false;
if (other.getLastModifiedTime() != null && other.getLastModifiedTime().equals(this.getLastModifiedTime()) == false)
return false;
if (other.getFailureMessages() == null ^ this.getFailureMessages() == null)
return false;
if (other.getFailureMessages() != null && other.getFailureMessages().equals(this.getFailureMessages()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getJobName() == null) ? 0 : getJobName().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getJobArn() == null) ? 0 : getJobArn().hashCode());
hashCode = prime * hashCode + ((getJobDescription() == null) ? 0 : getJobDescription().hashCode());
hashCode = prime * hashCode + ((getRoleArn() == null) ? 0 : getRoleArn().hashCode());
hashCode = prime * hashCode + ((getCustomerEncryptionKeyId() == null) ? 0 : getCustomerEncryptionKeyId().hashCode());
hashCode = prime * hashCode + ((getJobType() == null) ? 0 : getJobType().hashCode());
hashCode = prime * hashCode + ((getEvaluationConfig() == null) ? 0 : getEvaluationConfig().hashCode());
hashCode = prime * hashCode + ((getInferenceConfig() == null) ? 0 : getInferenceConfig().hashCode());
hashCode = prime * hashCode + ((getOutputDataConfig() == null) ? 0 : getOutputDataConfig().hashCode());
hashCode = prime * hashCode + ((getCreationTime() == null) ? 0 : getCreationTime().hashCode());
hashCode = prime * hashCode + ((getLastModifiedTime() == null) ? 0 : getLastModifiedTime().hashCode());
hashCode = prime * hashCode + ((getFailureMessages() == null) ? 0 : getFailureMessages().hashCode());
return hashCode;
}
@Override
public GetEvaluationJobResult clone() {
try {
return (GetEvaluationJobResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}