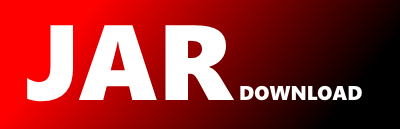
com.amazonaws.services.bedrockagent.model.AgentActionGroup Maven / Gradle / Ivy
Show all versions of aws-java-sdk-bedrockagent Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.bedrockagent.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Contains details about an action group.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AgentActionGroup implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Resource Name (ARN) of the Lambda function containing the business logic that is carried out upon
* invoking the action or the custom control method for handling the information elicited from the user.
*
*/
private ActionGroupExecutor actionGroupExecutor;
/**
*
* The unique identifier of the action group.
*
*/
private String actionGroupId;
/**
*
* The name of the action group.
*
*/
private String actionGroupName;
/**
*
* Specifies whether the action group is available for the agent to invoke or not when sending an InvokeAgent
* request.
*
*/
private String actionGroupState;
/**
*
* The unique identifier of the agent to which the action group belongs.
*
*/
private String agentId;
/**
*
* The version of the agent to which the action group belongs.
*
*/
private String agentVersion;
/**
*
* Contains either details about the S3 object containing the OpenAPI schema for the action group or the JSON or
* YAML-formatted payload defining the schema. For more information, see Action group OpenAPI
* schemas.
*
*/
private APISchema apiSchema;
/**
*
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If this token
* matches a previous request, Amazon Bedrock ignores the request, but does not return an error. For more
* information, see Ensuring
* idempotency.
*
*/
private String clientToken;
/**
*
* The time at which the action group was created.
*
*/
private java.util.Date createdAt;
/**
*
* The description of the action group.
*
*/
private String description;
/**
*
* Defines functions that each define parameters that the agent needs to invoke from the user. Each function
* represents an action in an action group.
*
*/
private FunctionSchema functionSchema;
/**
*
* If this field is set as AMAZON.UserInput
, the agent can request the user for additional information
* when trying to complete a task. The description
, apiSchema
, and
* actionGroupExecutor
fields must be blank for this action group.
*
*
* During orchestration, if the agent determines that it needs to invoke an API in an action group, but doesn't have
* enough information to complete the API request, it will invoke this action group instead and return an Observation
* reprompting the user for more information.
*
*/
private String parentActionSignature;
/**
*
* The time at which the action group was last updated.
*
*/
private java.util.Date updatedAt;
/**
*
* The Amazon Resource Name (ARN) of the Lambda function containing the business logic that is carried out upon
* invoking the action or the custom control method for handling the information elicited from the user.
*
*
* @param actionGroupExecutor
* The Amazon Resource Name (ARN) of the Lambda function containing the business logic that is carried out
* upon invoking the action or the custom control method for handling the information elicited from the user.
*/
public void setActionGroupExecutor(ActionGroupExecutor actionGroupExecutor) {
this.actionGroupExecutor = actionGroupExecutor;
}
/**
*
* The Amazon Resource Name (ARN) of the Lambda function containing the business logic that is carried out upon
* invoking the action or the custom control method for handling the information elicited from the user.
*
*
* @return The Amazon Resource Name (ARN) of the Lambda function containing the business logic that is carried out
* upon invoking the action or the custom control method for handling the information elicited from the
* user.
*/
public ActionGroupExecutor getActionGroupExecutor() {
return this.actionGroupExecutor;
}
/**
*
* The Amazon Resource Name (ARN) of the Lambda function containing the business logic that is carried out upon
* invoking the action or the custom control method for handling the information elicited from the user.
*
*
* @param actionGroupExecutor
* The Amazon Resource Name (ARN) of the Lambda function containing the business logic that is carried out
* upon invoking the action or the custom control method for handling the information elicited from the user.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AgentActionGroup withActionGroupExecutor(ActionGroupExecutor actionGroupExecutor) {
setActionGroupExecutor(actionGroupExecutor);
return this;
}
/**
*
* The unique identifier of the action group.
*
*
* @param actionGroupId
* The unique identifier of the action group.
*/
public void setActionGroupId(String actionGroupId) {
this.actionGroupId = actionGroupId;
}
/**
*
* The unique identifier of the action group.
*
*
* @return The unique identifier of the action group.
*/
public String getActionGroupId() {
return this.actionGroupId;
}
/**
*
* The unique identifier of the action group.
*
*
* @param actionGroupId
* The unique identifier of the action group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AgentActionGroup withActionGroupId(String actionGroupId) {
setActionGroupId(actionGroupId);
return this;
}
/**
*
* The name of the action group.
*
*
* @param actionGroupName
* The name of the action group.
*/
public void setActionGroupName(String actionGroupName) {
this.actionGroupName = actionGroupName;
}
/**
*
* The name of the action group.
*
*
* @return The name of the action group.
*/
public String getActionGroupName() {
return this.actionGroupName;
}
/**
*
* The name of the action group.
*
*
* @param actionGroupName
* The name of the action group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AgentActionGroup withActionGroupName(String actionGroupName) {
setActionGroupName(actionGroupName);
return this;
}
/**
*
* Specifies whether the action group is available for the agent to invoke or not when sending an InvokeAgent
* request.
*
*
* @param actionGroupState
* Specifies whether the action group is available for the agent to invoke or not when sending an InvokeAgent request.
* @see ActionGroupState
*/
public void setActionGroupState(String actionGroupState) {
this.actionGroupState = actionGroupState;
}
/**
*
* Specifies whether the action group is available for the agent to invoke or not when sending an InvokeAgent
* request.
*
*
* @return Specifies whether the action group is available for the agent to invoke or not when sending an InvokeAgent request.
* @see ActionGroupState
*/
public String getActionGroupState() {
return this.actionGroupState;
}
/**
*
* Specifies whether the action group is available for the agent to invoke or not when sending an InvokeAgent
* request.
*
*
* @param actionGroupState
* Specifies whether the action group is available for the agent to invoke or not when sending an InvokeAgent request.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ActionGroupState
*/
public AgentActionGroup withActionGroupState(String actionGroupState) {
setActionGroupState(actionGroupState);
return this;
}
/**
*
* Specifies whether the action group is available for the agent to invoke or not when sending an InvokeAgent
* request.
*
*
* @param actionGroupState
* Specifies whether the action group is available for the agent to invoke or not when sending an InvokeAgent request.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ActionGroupState
*/
public AgentActionGroup withActionGroupState(ActionGroupState actionGroupState) {
this.actionGroupState = actionGroupState.toString();
return this;
}
/**
*
* The unique identifier of the agent to which the action group belongs.
*
*
* @param agentId
* The unique identifier of the agent to which the action group belongs.
*/
public void setAgentId(String agentId) {
this.agentId = agentId;
}
/**
*
* The unique identifier of the agent to which the action group belongs.
*
*
* @return The unique identifier of the agent to which the action group belongs.
*/
public String getAgentId() {
return this.agentId;
}
/**
*
* The unique identifier of the agent to which the action group belongs.
*
*
* @param agentId
* The unique identifier of the agent to which the action group belongs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AgentActionGroup withAgentId(String agentId) {
setAgentId(agentId);
return this;
}
/**
*
* The version of the agent to which the action group belongs.
*
*
* @param agentVersion
* The version of the agent to which the action group belongs.
*/
public void setAgentVersion(String agentVersion) {
this.agentVersion = agentVersion;
}
/**
*
* The version of the agent to which the action group belongs.
*
*
* @return The version of the agent to which the action group belongs.
*/
public String getAgentVersion() {
return this.agentVersion;
}
/**
*
* The version of the agent to which the action group belongs.
*
*
* @param agentVersion
* The version of the agent to which the action group belongs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AgentActionGroup withAgentVersion(String agentVersion) {
setAgentVersion(agentVersion);
return this;
}
/**
*
* Contains either details about the S3 object containing the OpenAPI schema for the action group or the JSON or
* YAML-formatted payload defining the schema. For more information, see Action group OpenAPI
* schemas.
*
*
* @param apiSchema
* Contains either details about the S3 object containing the OpenAPI schema for the action group or the JSON
* or YAML-formatted payload defining the schema. For more information, see Action group OpenAPI
* schemas.
*/
public void setApiSchema(APISchema apiSchema) {
this.apiSchema = apiSchema;
}
/**
*
* Contains either details about the S3 object containing the OpenAPI schema for the action group or the JSON or
* YAML-formatted payload defining the schema. For more information, see Action group OpenAPI
* schemas.
*
*
* @return Contains either details about the S3 object containing the OpenAPI schema for the action group or the
* JSON or YAML-formatted payload defining the schema. For more information, see Action group OpenAPI
* schemas.
*/
public APISchema getApiSchema() {
return this.apiSchema;
}
/**
*
* Contains either details about the S3 object containing the OpenAPI schema for the action group or the JSON or
* YAML-formatted payload defining the schema. For more information, see Action group OpenAPI
* schemas.
*
*
* @param apiSchema
* Contains either details about the S3 object containing the OpenAPI schema for the action group or the JSON
* or YAML-formatted payload defining the schema. For more information, see Action group OpenAPI
* schemas.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AgentActionGroup withApiSchema(APISchema apiSchema) {
setApiSchema(apiSchema);
return this;
}
/**
*
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If this token
* matches a previous request, Amazon Bedrock ignores the request, but does not return an error. For more
* information, see Ensuring
* idempotency.
*
*
* @param clientToken
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If
* this token matches a previous request, Amazon Bedrock ignores the request, but does not return an error.
* For more information, see Ensuring
* idempotency.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If this token
* matches a previous request, Amazon Bedrock ignores the request, but does not return an error. For more
* information, see Ensuring
* idempotency.
*
*
* @return A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If
* this token matches a previous request, Amazon Bedrock ignores the request, but does not return an error.
* For more information, see Ensuring
* idempotency.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If this token
* matches a previous request, Amazon Bedrock ignores the request, but does not return an error. For more
* information, see Ensuring
* idempotency.
*
*
* @param clientToken
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If
* this token matches a previous request, Amazon Bedrock ignores the request, but does not return an error.
* For more information, see Ensuring
* idempotency.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AgentActionGroup withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* The time at which the action group was created.
*
*
* @param createdAt
* The time at which the action group was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The time at which the action group was created.
*
*
* @return The time at which the action group was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The time at which the action group was created.
*
*
* @param createdAt
* The time at which the action group was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AgentActionGroup withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The description of the action group.
*
*
* @param description
* The description of the action group.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description of the action group.
*
*
* @return The description of the action group.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description of the action group.
*
*
* @param description
* The description of the action group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AgentActionGroup withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* Defines functions that each define parameters that the agent needs to invoke from the user. Each function
* represents an action in an action group.
*
*
* @param functionSchema
* Defines functions that each define parameters that the agent needs to invoke from the user. Each function
* represents an action in an action group.
*/
public void setFunctionSchema(FunctionSchema functionSchema) {
this.functionSchema = functionSchema;
}
/**
*
* Defines functions that each define parameters that the agent needs to invoke from the user. Each function
* represents an action in an action group.
*
*
* @return Defines functions that each define parameters that the agent needs to invoke from the user. Each function
* represents an action in an action group.
*/
public FunctionSchema getFunctionSchema() {
return this.functionSchema;
}
/**
*
* Defines functions that each define parameters that the agent needs to invoke from the user. Each function
* represents an action in an action group.
*
*
* @param functionSchema
* Defines functions that each define parameters that the agent needs to invoke from the user. Each function
* represents an action in an action group.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AgentActionGroup withFunctionSchema(FunctionSchema functionSchema) {
setFunctionSchema(functionSchema);
return this;
}
/**
*
* If this field is set as AMAZON.UserInput
, the agent can request the user for additional information
* when trying to complete a task. The description
, apiSchema
, and
* actionGroupExecutor
fields must be blank for this action group.
*
*
* During orchestration, if the agent determines that it needs to invoke an API in an action group, but doesn't have
* enough information to complete the API request, it will invoke this action group instead and return an Observation
* reprompting the user for more information.
*
*
* @param parentActionSignature
* If this field is set as AMAZON.UserInput
, the agent can request the user for additional
* information when trying to complete a task. The description
, apiSchema
, and
* actionGroupExecutor
fields must be blank for this action group.
*
* During orchestration, if the agent determines that it needs to invoke an API in an action group, but
* doesn't have enough information to complete the API request, it will invoke this action group instead and
* return an Observation reprompting the user for more information.
* @see ActionGroupSignature
*/
public void setParentActionSignature(String parentActionSignature) {
this.parentActionSignature = parentActionSignature;
}
/**
*
* If this field is set as AMAZON.UserInput
, the agent can request the user for additional information
* when trying to complete a task. The description
, apiSchema
, and
* actionGroupExecutor
fields must be blank for this action group.
*
*
* During orchestration, if the agent determines that it needs to invoke an API in an action group, but doesn't have
* enough information to complete the API request, it will invoke this action group instead and return an Observation
* reprompting the user for more information.
*
*
* @return If this field is set as AMAZON.UserInput
, the agent can request the user for additional
* information when trying to complete a task. The description
, apiSchema
, and
* actionGroupExecutor
fields must be blank for this action group.
*
* During orchestration, if the agent determines that it needs to invoke an API in an action group, but
* doesn't have enough information to complete the API request, it will invoke this action group instead and
* return an Observation reprompting the user for more information.
* @see ActionGroupSignature
*/
public String getParentActionSignature() {
return this.parentActionSignature;
}
/**
*
* If this field is set as AMAZON.UserInput
, the agent can request the user for additional information
* when trying to complete a task. The description
, apiSchema
, and
* actionGroupExecutor
fields must be blank for this action group.
*
*
* During orchestration, if the agent determines that it needs to invoke an API in an action group, but doesn't have
* enough information to complete the API request, it will invoke this action group instead and return an Observation
* reprompting the user for more information.
*
*
* @param parentActionSignature
* If this field is set as AMAZON.UserInput
, the agent can request the user for additional
* information when trying to complete a task. The description
, apiSchema
, and
* actionGroupExecutor
fields must be blank for this action group.
*
* During orchestration, if the agent determines that it needs to invoke an API in an action group, but
* doesn't have enough information to complete the API request, it will invoke this action group instead and
* return an Observation reprompting the user for more information.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ActionGroupSignature
*/
public AgentActionGroup withParentActionSignature(String parentActionSignature) {
setParentActionSignature(parentActionSignature);
return this;
}
/**
*
* If this field is set as AMAZON.UserInput
, the agent can request the user for additional information
* when trying to complete a task. The description
, apiSchema
, and
* actionGroupExecutor
fields must be blank for this action group.
*
*
* During orchestration, if the agent determines that it needs to invoke an API in an action group, but doesn't have
* enough information to complete the API request, it will invoke this action group instead and return an Observation
* reprompting the user for more information.
*
*
* @param parentActionSignature
* If this field is set as AMAZON.UserInput
, the agent can request the user for additional
* information when trying to complete a task. The description
, apiSchema
, and
* actionGroupExecutor
fields must be blank for this action group.
*
* During orchestration, if the agent determines that it needs to invoke an API in an action group, but
* doesn't have enough information to complete the API request, it will invoke this action group instead and
* return an Observation reprompting the user for more information.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ActionGroupSignature
*/
public AgentActionGroup withParentActionSignature(ActionGroupSignature parentActionSignature) {
this.parentActionSignature = parentActionSignature.toString();
return this;
}
/**
*
* The time at which the action group was last updated.
*
*
* @param updatedAt
* The time at which the action group was last updated.
*/
public void setUpdatedAt(java.util.Date updatedAt) {
this.updatedAt = updatedAt;
}
/**
*
* The time at which the action group was last updated.
*
*
* @return The time at which the action group was last updated.
*/
public java.util.Date getUpdatedAt() {
return this.updatedAt;
}
/**
*
* The time at which the action group was last updated.
*
*
* @param updatedAt
* The time at which the action group was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public AgentActionGroup withUpdatedAt(java.util.Date updatedAt) {
setUpdatedAt(updatedAt);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getActionGroupExecutor() != null)
sb.append("ActionGroupExecutor: ").append(getActionGroupExecutor()).append(",");
if (getActionGroupId() != null)
sb.append("ActionGroupId: ").append(getActionGroupId()).append(",");
if (getActionGroupName() != null)
sb.append("ActionGroupName: ").append(getActionGroupName()).append(",");
if (getActionGroupState() != null)
sb.append("ActionGroupState: ").append(getActionGroupState()).append(",");
if (getAgentId() != null)
sb.append("AgentId: ").append(getAgentId()).append(",");
if (getAgentVersion() != null)
sb.append("AgentVersion: ").append(getAgentVersion()).append(",");
if (getApiSchema() != null)
sb.append("ApiSchema: ").append(getApiSchema()).append(",");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getFunctionSchema() != null)
sb.append("FunctionSchema: ").append(getFunctionSchema()).append(",");
if (getParentActionSignature() != null)
sb.append("ParentActionSignature: ").append(getParentActionSignature()).append(",");
if (getUpdatedAt() != null)
sb.append("UpdatedAt: ").append(getUpdatedAt());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof AgentActionGroup == false)
return false;
AgentActionGroup other = (AgentActionGroup) obj;
if (other.getActionGroupExecutor() == null ^ this.getActionGroupExecutor() == null)
return false;
if (other.getActionGroupExecutor() != null && other.getActionGroupExecutor().equals(this.getActionGroupExecutor()) == false)
return false;
if (other.getActionGroupId() == null ^ this.getActionGroupId() == null)
return false;
if (other.getActionGroupId() != null && other.getActionGroupId().equals(this.getActionGroupId()) == false)
return false;
if (other.getActionGroupName() == null ^ this.getActionGroupName() == null)
return false;
if (other.getActionGroupName() != null && other.getActionGroupName().equals(this.getActionGroupName()) == false)
return false;
if (other.getActionGroupState() == null ^ this.getActionGroupState() == null)
return false;
if (other.getActionGroupState() != null && other.getActionGroupState().equals(this.getActionGroupState()) == false)
return false;
if (other.getAgentId() == null ^ this.getAgentId() == null)
return false;
if (other.getAgentId() != null && other.getAgentId().equals(this.getAgentId()) == false)
return false;
if (other.getAgentVersion() == null ^ this.getAgentVersion() == null)
return false;
if (other.getAgentVersion() != null && other.getAgentVersion().equals(this.getAgentVersion()) == false)
return false;
if (other.getApiSchema() == null ^ this.getApiSchema() == null)
return false;
if (other.getApiSchema() != null && other.getApiSchema().equals(this.getApiSchema()) == false)
return false;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getFunctionSchema() == null ^ this.getFunctionSchema() == null)
return false;
if (other.getFunctionSchema() != null && other.getFunctionSchema().equals(this.getFunctionSchema()) == false)
return false;
if (other.getParentActionSignature() == null ^ this.getParentActionSignature() == null)
return false;
if (other.getParentActionSignature() != null && other.getParentActionSignature().equals(this.getParentActionSignature()) == false)
return false;
if (other.getUpdatedAt() == null ^ this.getUpdatedAt() == null)
return false;
if (other.getUpdatedAt() != null && other.getUpdatedAt().equals(this.getUpdatedAt()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getActionGroupExecutor() == null) ? 0 : getActionGroupExecutor().hashCode());
hashCode = prime * hashCode + ((getActionGroupId() == null) ? 0 : getActionGroupId().hashCode());
hashCode = prime * hashCode + ((getActionGroupName() == null) ? 0 : getActionGroupName().hashCode());
hashCode = prime * hashCode + ((getActionGroupState() == null) ? 0 : getActionGroupState().hashCode());
hashCode = prime * hashCode + ((getAgentId() == null) ? 0 : getAgentId().hashCode());
hashCode = prime * hashCode + ((getAgentVersion() == null) ? 0 : getAgentVersion().hashCode());
hashCode = prime * hashCode + ((getApiSchema() == null) ? 0 : getApiSchema().hashCode());
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getFunctionSchema() == null) ? 0 : getFunctionSchema().hashCode());
hashCode = prime * hashCode + ((getParentActionSignature() == null) ? 0 : getParentActionSignature().hashCode());
hashCode = prime * hashCode + ((getUpdatedAt() == null) ? 0 : getUpdatedAt().hashCode());
return hashCode;
}
@Override
public AgentActionGroup clone() {
try {
return (AgentActionGroup) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.bedrockagent.model.transform.AgentActionGroupMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}