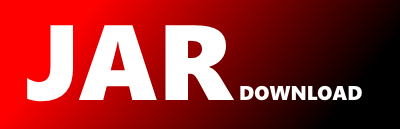
com.amazonaws.services.bedrockagent.model.CreatePromptResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-bedrockagent Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.bedrockagent.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreatePromptResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) of the prompt.
*
*/
private String arn;
/**
*
* The time at which the prompt was created.
*
*/
private java.util.Date createdAt;
/**
*
* The Amazon Resource Name (ARN) of the KMS key that you encrypted the prompt with.
*
*/
private String customerEncryptionKeyArn;
/**
*
* The name of the default variant for your prompt.
*
*/
private String defaultVariant;
/**
*
* The description of the prompt.
*
*/
private String description;
/**
*
* The unique identifier of the prompt.
*
*/
private String id;
/**
*
* The name of the prompt.
*
*/
private String name;
/**
*
* The time at which the prompt was last updated.
*
*/
private java.util.Date updatedAt;
/**
*
* A list of objects, each containing details about a variant of the prompt.
*
*/
private java.util.List variants;
/**
*
* The version of the prompt. When you create a prompt, the version created is the DRAFT
version.
*
*/
private String version;
/**
*
* The Amazon Resource Name (ARN) of the prompt.
*
*
* @param arn
* The Amazon Resource Name (ARN) of the prompt.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The Amazon Resource Name (ARN) of the prompt.
*
*
* @return The Amazon Resource Name (ARN) of the prompt.
*/
public String getArn() {
return this.arn;
}
/**
*
* The Amazon Resource Name (ARN) of the prompt.
*
*
* @param arn
* The Amazon Resource Name (ARN) of the prompt.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePromptResult withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* The time at which the prompt was created.
*
*
* @param createdAt
* The time at which the prompt was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The time at which the prompt was created.
*
*
* @return The time at which the prompt was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The time at which the prompt was created.
*
*
* @param createdAt
* The time at which the prompt was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePromptResult withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the KMS key that you encrypted the prompt with.
*
*
* @param customerEncryptionKeyArn
* The Amazon Resource Name (ARN) of the KMS key that you encrypted the prompt with.
*/
public void setCustomerEncryptionKeyArn(String customerEncryptionKeyArn) {
this.customerEncryptionKeyArn = customerEncryptionKeyArn;
}
/**
*
* The Amazon Resource Name (ARN) of the KMS key that you encrypted the prompt with.
*
*
* @return The Amazon Resource Name (ARN) of the KMS key that you encrypted the prompt with.
*/
public String getCustomerEncryptionKeyArn() {
return this.customerEncryptionKeyArn;
}
/**
*
* The Amazon Resource Name (ARN) of the KMS key that you encrypted the prompt with.
*
*
* @param customerEncryptionKeyArn
* The Amazon Resource Name (ARN) of the KMS key that you encrypted the prompt with.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePromptResult withCustomerEncryptionKeyArn(String customerEncryptionKeyArn) {
setCustomerEncryptionKeyArn(customerEncryptionKeyArn);
return this;
}
/**
*
* The name of the default variant for your prompt.
*
*
* @param defaultVariant
* The name of the default variant for your prompt.
*/
public void setDefaultVariant(String defaultVariant) {
this.defaultVariant = defaultVariant;
}
/**
*
* The name of the default variant for your prompt.
*
*
* @return The name of the default variant for your prompt.
*/
public String getDefaultVariant() {
return this.defaultVariant;
}
/**
*
* The name of the default variant for your prompt.
*
*
* @param defaultVariant
* The name of the default variant for your prompt.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePromptResult withDefaultVariant(String defaultVariant) {
setDefaultVariant(defaultVariant);
return this;
}
/**
*
* The description of the prompt.
*
*
* @param description
* The description of the prompt.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description of the prompt.
*
*
* @return The description of the prompt.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description of the prompt.
*
*
* @param description
* The description of the prompt.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePromptResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The unique identifier of the prompt.
*
*
* @param id
* The unique identifier of the prompt.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The unique identifier of the prompt.
*
*
* @return The unique identifier of the prompt.
*/
public String getId() {
return this.id;
}
/**
*
* The unique identifier of the prompt.
*
*
* @param id
* The unique identifier of the prompt.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePromptResult withId(String id) {
setId(id);
return this;
}
/**
*
* The name of the prompt.
*
*
* @param name
* The name of the prompt.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the prompt.
*
*
* @return The name of the prompt.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the prompt.
*
*
* @param name
* The name of the prompt.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePromptResult withName(String name) {
setName(name);
return this;
}
/**
*
* The time at which the prompt was last updated.
*
*
* @param updatedAt
* The time at which the prompt was last updated.
*/
public void setUpdatedAt(java.util.Date updatedAt) {
this.updatedAt = updatedAt;
}
/**
*
* The time at which the prompt was last updated.
*
*
* @return The time at which the prompt was last updated.
*/
public java.util.Date getUpdatedAt() {
return this.updatedAt;
}
/**
*
* The time at which the prompt was last updated.
*
*
* @param updatedAt
* The time at which the prompt was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePromptResult withUpdatedAt(java.util.Date updatedAt) {
setUpdatedAt(updatedAt);
return this;
}
/**
*
* A list of objects, each containing details about a variant of the prompt.
*
*
* @return A list of objects, each containing details about a variant of the prompt.
*/
public java.util.List getVariants() {
return variants;
}
/**
*
* A list of objects, each containing details about a variant of the prompt.
*
*
* @param variants
* A list of objects, each containing details about a variant of the prompt.
*/
public void setVariants(java.util.Collection variants) {
if (variants == null) {
this.variants = null;
return;
}
this.variants = new java.util.ArrayList(variants);
}
/**
*
* A list of objects, each containing details about a variant of the prompt.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setVariants(java.util.Collection)} or {@link #withVariants(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param variants
* A list of objects, each containing details about a variant of the prompt.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePromptResult withVariants(PromptVariant... variants) {
if (this.variants == null) {
setVariants(new java.util.ArrayList(variants.length));
}
for (PromptVariant ele : variants) {
this.variants.add(ele);
}
return this;
}
/**
*
* A list of objects, each containing details about a variant of the prompt.
*
*
* @param variants
* A list of objects, each containing details about a variant of the prompt.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePromptResult withVariants(java.util.Collection variants) {
setVariants(variants);
return this;
}
/**
*
* The version of the prompt. When you create a prompt, the version created is the DRAFT
version.
*
*
* @param version
* The version of the prompt. When you create a prompt, the version created is the DRAFT
* version.
*/
public void setVersion(String version) {
this.version = version;
}
/**
*
* The version of the prompt. When you create a prompt, the version created is the DRAFT
version.
*
*
* @return The version of the prompt. When you create a prompt, the version created is the DRAFT
* version.
*/
public String getVersion() {
return this.version;
}
/**
*
* The version of the prompt. When you create a prompt, the version created is the DRAFT
version.
*
*
* @param version
* The version of the prompt. When you create a prompt, the version created is the DRAFT
* version.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreatePromptResult withVersion(String version) {
setVersion(version);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getCustomerEncryptionKeyArn() != null)
sb.append("CustomerEncryptionKeyArn: ").append(getCustomerEncryptionKeyArn()).append(",");
if (getDefaultVariant() != null)
sb.append("DefaultVariant: ").append(getDefaultVariant()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getUpdatedAt() != null)
sb.append("UpdatedAt: ").append(getUpdatedAt()).append(",");
if (getVariants() != null)
sb.append("Variants: ").append("***Sensitive Data Redacted***").append(",");
if (getVersion() != null)
sb.append("Version: ").append(getVersion());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreatePromptResult == false)
return false;
CreatePromptResult other = (CreatePromptResult) obj;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getCustomerEncryptionKeyArn() == null ^ this.getCustomerEncryptionKeyArn() == null)
return false;
if (other.getCustomerEncryptionKeyArn() != null && other.getCustomerEncryptionKeyArn().equals(this.getCustomerEncryptionKeyArn()) == false)
return false;
if (other.getDefaultVariant() == null ^ this.getDefaultVariant() == null)
return false;
if (other.getDefaultVariant() != null && other.getDefaultVariant().equals(this.getDefaultVariant()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getUpdatedAt() == null ^ this.getUpdatedAt() == null)
return false;
if (other.getUpdatedAt() != null && other.getUpdatedAt().equals(this.getUpdatedAt()) == false)
return false;
if (other.getVariants() == null ^ this.getVariants() == null)
return false;
if (other.getVariants() != null && other.getVariants().equals(this.getVariants()) == false)
return false;
if (other.getVersion() == null ^ this.getVersion() == null)
return false;
if (other.getVersion() != null && other.getVersion().equals(this.getVersion()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getCustomerEncryptionKeyArn() == null) ? 0 : getCustomerEncryptionKeyArn().hashCode());
hashCode = prime * hashCode + ((getDefaultVariant() == null) ? 0 : getDefaultVariant().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getUpdatedAt() == null) ? 0 : getUpdatedAt().hashCode());
hashCode = prime * hashCode + ((getVariants() == null) ? 0 : getVariants().hashCode());
hashCode = prime * hashCode + ((getVersion() == null) ? 0 : getVersion().hashCode());
return hashCode;
}
@Override
public CreatePromptResult clone() {
try {
return (CreatePromptResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}