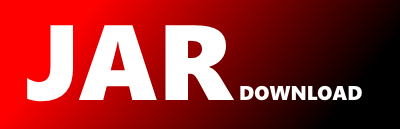
com.amazonaws.services.bedrockagent.model.PromptConfiguration Maven / Gradle / Ivy
Show all versions of aws-java-sdk-bedrockagent Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.bedrockagent.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Contains configurations to override a prompt template in one part of an agent sequence. For more information, see Advanced prompts.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class PromptConfiguration implements Serializable, Cloneable, StructuredPojo {
/**
*
* Defines the prompt template with which to replace the default prompt template. You can use placeholder variables
* in the base prompt template to customize the prompt. For more information, see Prompt template placeholder
* variables. For more information, see Configure the prompt
* templates.
*
*/
private String basePromptTemplate;
/**
*
* Contains inference parameters to use when the agent invokes a foundation model in the part of the agent sequence
* defined by the promptType
. For more information, see Inference parameters for
* foundation models.
*
*/
private InferenceConfiguration inferenceConfiguration;
/**
*
* Specifies whether to override the default parser Lambda function when parsing the raw foundation model output in
* the part of the agent sequence defined by the promptType
. If you set the field as
* OVERRIDEN
, the overrideLambda
field in the PromptOverrideConfiguration must be specified with the ARN of a Lambda function.
*
*/
private String parserMode;
/**
*
* Specifies whether to override the default prompt template for this promptType
. Set this value to
* OVERRIDDEN
to use the prompt that you provide in the basePromptTemplate
. If you leave
* it as DEFAULT
, the agent uses a default prompt template.
*
*/
private String promptCreationMode;
/**
*
* Specifies whether to allow the agent to carry out the step specified in the promptType
. If you set
* this value to DISABLED
, the agent skips that step. The default state for each
* promptType
is as follows.
*
*
* -
*
* PRE_PROCESSING
– ENABLED
*
*
* -
*
* ORCHESTRATION
– ENABLED
*
*
* -
*
* KNOWLEDGE_BASE_RESPONSE_GENERATION
– ENABLED
*
*
* -
*
* POST_PROCESSING
– DISABLED
*
*
*
*/
private String promptState;
/**
*
* The step in the agent sequence that this prompt configuration applies to.
*
*/
private String promptType;
/**
*
* Defines the prompt template with which to replace the default prompt template. You can use placeholder variables
* in the base prompt template to customize the prompt. For more information, see Prompt template placeholder
* variables. For more information, see Configure the prompt
* templates.
*
*
* @param basePromptTemplate
* Defines the prompt template with which to replace the default prompt template. You can use placeholder
* variables in the base prompt template to customize the prompt. For more information, see Prompt template
* placeholder variables. For more information, see Configure the
* prompt templates.
*/
public void setBasePromptTemplate(String basePromptTemplate) {
this.basePromptTemplate = basePromptTemplate;
}
/**
*
* Defines the prompt template with which to replace the default prompt template. You can use placeholder variables
* in the base prompt template to customize the prompt. For more information, see Prompt template placeholder
* variables. For more information, see Configure the prompt
* templates.
*
*
* @return Defines the prompt template with which to replace the default prompt template. You can use placeholder
* variables in the base prompt template to customize the prompt. For more information, see Prompt template
* placeholder variables. For more information, see Configure the
* prompt templates.
*/
public String getBasePromptTemplate() {
return this.basePromptTemplate;
}
/**
*
* Defines the prompt template with which to replace the default prompt template. You can use placeholder variables
* in the base prompt template to customize the prompt. For more information, see Prompt template placeholder
* variables. For more information, see Configure the prompt
* templates.
*
*
* @param basePromptTemplate
* Defines the prompt template with which to replace the default prompt template. You can use placeholder
* variables in the base prompt template to customize the prompt. For more information, see Prompt template
* placeholder variables. For more information, see Configure the
* prompt templates.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PromptConfiguration withBasePromptTemplate(String basePromptTemplate) {
setBasePromptTemplate(basePromptTemplate);
return this;
}
/**
*
* Contains inference parameters to use when the agent invokes a foundation model in the part of the agent sequence
* defined by the promptType
. For more information, see Inference parameters for
* foundation models.
*
*
* @param inferenceConfiguration
* Contains inference parameters to use when the agent invokes a foundation model in the part of the agent
* sequence defined by the promptType
. For more information, see Inference parameters for
* foundation models.
*/
public void setInferenceConfiguration(InferenceConfiguration inferenceConfiguration) {
this.inferenceConfiguration = inferenceConfiguration;
}
/**
*
* Contains inference parameters to use when the agent invokes a foundation model in the part of the agent sequence
* defined by the promptType
. For more information, see Inference parameters for
* foundation models.
*
*
* @return Contains inference parameters to use when the agent invokes a foundation model in the part of the agent
* sequence defined by the promptType
. For more information, see Inference parameters
* for foundation models.
*/
public InferenceConfiguration getInferenceConfiguration() {
return this.inferenceConfiguration;
}
/**
*
* Contains inference parameters to use when the agent invokes a foundation model in the part of the agent sequence
* defined by the promptType
. For more information, see Inference parameters for
* foundation models.
*
*
* @param inferenceConfiguration
* Contains inference parameters to use when the agent invokes a foundation model in the part of the agent
* sequence defined by the promptType
. For more information, see Inference parameters for
* foundation models.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public PromptConfiguration withInferenceConfiguration(InferenceConfiguration inferenceConfiguration) {
setInferenceConfiguration(inferenceConfiguration);
return this;
}
/**
*
* Specifies whether to override the default parser Lambda function when parsing the raw foundation model output in
* the part of the agent sequence defined by the promptType
. If you set the field as
* OVERRIDEN
, the overrideLambda
field in the PromptOverrideConfiguration must be specified with the ARN of a Lambda function.
*
*
* @param parserMode
* Specifies whether to override the default parser Lambda function when parsing the raw foundation model
* output in the part of the agent sequence defined by the promptType
. If you set the field as
* OVERRIDEN
, the overrideLambda
field in the PromptOverrideConfiguration must be specified with the ARN of a Lambda function.
* @see CreationMode
*/
public void setParserMode(String parserMode) {
this.parserMode = parserMode;
}
/**
*
* Specifies whether to override the default parser Lambda function when parsing the raw foundation model output in
* the part of the agent sequence defined by the promptType
. If you set the field as
* OVERRIDEN
, the overrideLambda
field in the PromptOverrideConfiguration must be specified with the ARN of a Lambda function.
*
*
* @return Specifies whether to override the default parser Lambda function when parsing the raw foundation model
* output in the part of the agent sequence defined by the promptType
. If you set the field as
* OVERRIDEN
, the overrideLambda
field in the PromptOverrideConfiguration must be specified with the ARN of a Lambda function.
* @see CreationMode
*/
public String getParserMode() {
return this.parserMode;
}
/**
*
* Specifies whether to override the default parser Lambda function when parsing the raw foundation model output in
* the part of the agent sequence defined by the promptType
. If you set the field as
* OVERRIDEN
, the overrideLambda
field in the PromptOverrideConfiguration must be specified with the ARN of a Lambda function.
*
*
* @param parserMode
* Specifies whether to override the default parser Lambda function when parsing the raw foundation model
* output in the part of the agent sequence defined by the promptType
. If you set the field as
* OVERRIDEN
, the overrideLambda
field in the PromptOverrideConfiguration must be specified with the ARN of a Lambda function.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CreationMode
*/
public PromptConfiguration withParserMode(String parserMode) {
setParserMode(parserMode);
return this;
}
/**
*
* Specifies whether to override the default parser Lambda function when parsing the raw foundation model output in
* the part of the agent sequence defined by the promptType
. If you set the field as
* OVERRIDEN
, the overrideLambda
field in the PromptOverrideConfiguration must be specified with the ARN of a Lambda function.
*
*
* @param parserMode
* Specifies whether to override the default parser Lambda function when parsing the raw foundation model
* output in the part of the agent sequence defined by the promptType
. If you set the field as
* OVERRIDEN
, the overrideLambda
field in the PromptOverrideConfiguration must be specified with the ARN of a Lambda function.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CreationMode
*/
public PromptConfiguration withParserMode(CreationMode parserMode) {
this.parserMode = parserMode.toString();
return this;
}
/**
*
* Specifies whether to override the default prompt template for this promptType
. Set this value to
* OVERRIDDEN
to use the prompt that you provide in the basePromptTemplate
. If you leave
* it as DEFAULT
, the agent uses a default prompt template.
*
*
* @param promptCreationMode
* Specifies whether to override the default prompt template for this promptType
. Set this value
* to OVERRIDDEN
to use the prompt that you provide in the basePromptTemplate
. If
* you leave it as DEFAULT
, the agent uses a default prompt template.
* @see CreationMode
*/
public void setPromptCreationMode(String promptCreationMode) {
this.promptCreationMode = promptCreationMode;
}
/**
*
* Specifies whether to override the default prompt template for this promptType
. Set this value to
* OVERRIDDEN
to use the prompt that you provide in the basePromptTemplate
. If you leave
* it as DEFAULT
, the agent uses a default prompt template.
*
*
* @return Specifies whether to override the default prompt template for this promptType
. Set this
* value to OVERRIDDEN
to use the prompt that you provide in the
* basePromptTemplate
. If you leave it as DEFAULT
, the agent uses a default prompt
* template.
* @see CreationMode
*/
public String getPromptCreationMode() {
return this.promptCreationMode;
}
/**
*
* Specifies whether to override the default prompt template for this promptType
. Set this value to
* OVERRIDDEN
to use the prompt that you provide in the basePromptTemplate
. If you leave
* it as DEFAULT
, the agent uses a default prompt template.
*
*
* @param promptCreationMode
* Specifies whether to override the default prompt template for this promptType
. Set this value
* to OVERRIDDEN
to use the prompt that you provide in the basePromptTemplate
. If
* you leave it as DEFAULT
, the agent uses a default prompt template.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CreationMode
*/
public PromptConfiguration withPromptCreationMode(String promptCreationMode) {
setPromptCreationMode(promptCreationMode);
return this;
}
/**
*
* Specifies whether to override the default prompt template for this promptType
. Set this value to
* OVERRIDDEN
to use the prompt that you provide in the basePromptTemplate
. If you leave
* it as DEFAULT
, the agent uses a default prompt template.
*
*
* @param promptCreationMode
* Specifies whether to override the default prompt template for this promptType
. Set this value
* to OVERRIDDEN
to use the prompt that you provide in the basePromptTemplate
. If
* you leave it as DEFAULT
, the agent uses a default prompt template.
* @return Returns a reference to this object so that method calls can be chained together.
* @see CreationMode
*/
public PromptConfiguration withPromptCreationMode(CreationMode promptCreationMode) {
this.promptCreationMode = promptCreationMode.toString();
return this;
}
/**
*
* Specifies whether to allow the agent to carry out the step specified in the promptType
. If you set
* this value to DISABLED
, the agent skips that step. The default state for each
* promptType
is as follows.
*
*
* -
*
* PRE_PROCESSING
– ENABLED
*
*
* -
*
* ORCHESTRATION
– ENABLED
*
*
* -
*
* KNOWLEDGE_BASE_RESPONSE_GENERATION
– ENABLED
*
*
* -
*
* POST_PROCESSING
– DISABLED
*
*
*
*
* @param promptState
* Specifies whether to allow the agent to carry out the step specified in the promptType
. If
* you set this value to DISABLED
, the agent skips that step. The default state for each
* promptType
is as follows.
*
* -
*
* PRE_PROCESSING
– ENABLED
*
*
* -
*
* ORCHESTRATION
– ENABLED
*
*
* -
*
* KNOWLEDGE_BASE_RESPONSE_GENERATION
– ENABLED
*
*
* -
*
* POST_PROCESSING
– DISABLED
*
*
* @see PromptState
*/
public void setPromptState(String promptState) {
this.promptState = promptState;
}
/**
*
* Specifies whether to allow the agent to carry out the step specified in the promptType
. If you set
* this value to DISABLED
, the agent skips that step. The default state for each
* promptType
is as follows.
*
*
* -
*
* PRE_PROCESSING
– ENABLED
*
*
* -
*
* ORCHESTRATION
– ENABLED
*
*
* -
*
* KNOWLEDGE_BASE_RESPONSE_GENERATION
– ENABLED
*
*
* -
*
* POST_PROCESSING
– DISABLED
*
*
*
*
* @return Specifies whether to allow the agent to carry out the step specified in the promptType
. If
* you set this value to DISABLED
, the agent skips that step. The default state for each
* promptType
is as follows.
*
* -
*
* PRE_PROCESSING
– ENABLED
*
*
* -
*
* ORCHESTRATION
– ENABLED
*
*
* -
*
* KNOWLEDGE_BASE_RESPONSE_GENERATION
– ENABLED
*
*
* -
*
* POST_PROCESSING
– DISABLED
*
*
* @see PromptState
*/
public String getPromptState() {
return this.promptState;
}
/**
*
* Specifies whether to allow the agent to carry out the step specified in the promptType
. If you set
* this value to DISABLED
, the agent skips that step. The default state for each
* promptType
is as follows.
*
*
* -
*
* PRE_PROCESSING
– ENABLED
*
*
* -
*
* ORCHESTRATION
– ENABLED
*
*
* -
*
* KNOWLEDGE_BASE_RESPONSE_GENERATION
– ENABLED
*
*
* -
*
* POST_PROCESSING
– DISABLED
*
*
*
*
* @param promptState
* Specifies whether to allow the agent to carry out the step specified in the promptType
. If
* you set this value to DISABLED
, the agent skips that step. The default state for each
* promptType
is as follows.
*
* -
*
* PRE_PROCESSING
– ENABLED
*
*
* -
*
* ORCHESTRATION
– ENABLED
*
*
* -
*
* KNOWLEDGE_BASE_RESPONSE_GENERATION
– ENABLED
*
*
* -
*
* POST_PROCESSING
– DISABLED
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PromptState
*/
public PromptConfiguration withPromptState(String promptState) {
setPromptState(promptState);
return this;
}
/**
*
* Specifies whether to allow the agent to carry out the step specified in the promptType
. If you set
* this value to DISABLED
, the agent skips that step. The default state for each
* promptType
is as follows.
*
*
* -
*
* PRE_PROCESSING
– ENABLED
*
*
* -
*
* ORCHESTRATION
– ENABLED
*
*
* -
*
* KNOWLEDGE_BASE_RESPONSE_GENERATION
– ENABLED
*
*
* -
*
* POST_PROCESSING
– DISABLED
*
*
*
*
* @param promptState
* Specifies whether to allow the agent to carry out the step specified in the promptType
. If
* you set this value to DISABLED
, the agent skips that step. The default state for each
* promptType
is as follows.
*
* -
*
* PRE_PROCESSING
– ENABLED
*
*
* -
*
* ORCHESTRATION
– ENABLED
*
*
* -
*
* KNOWLEDGE_BASE_RESPONSE_GENERATION
– ENABLED
*
*
* -
*
* POST_PROCESSING
– DISABLED
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PromptState
*/
public PromptConfiguration withPromptState(PromptState promptState) {
this.promptState = promptState.toString();
return this;
}
/**
*
* The step in the agent sequence that this prompt configuration applies to.
*
*
* @param promptType
* The step in the agent sequence that this prompt configuration applies to.
* @see PromptType
*/
public void setPromptType(String promptType) {
this.promptType = promptType;
}
/**
*
* The step in the agent sequence that this prompt configuration applies to.
*
*
* @return The step in the agent sequence that this prompt configuration applies to.
* @see PromptType
*/
public String getPromptType() {
return this.promptType;
}
/**
*
* The step in the agent sequence that this prompt configuration applies to.
*
*
* @param promptType
* The step in the agent sequence that this prompt configuration applies to.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PromptType
*/
public PromptConfiguration withPromptType(String promptType) {
setPromptType(promptType);
return this;
}
/**
*
* The step in the agent sequence that this prompt configuration applies to.
*
*
* @param promptType
* The step in the agent sequence that this prompt configuration applies to.
* @return Returns a reference to this object so that method calls can be chained together.
* @see PromptType
*/
public PromptConfiguration withPromptType(PromptType promptType) {
this.promptType = promptType.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getBasePromptTemplate() != null)
sb.append("BasePromptTemplate: ").append("***Sensitive Data Redacted***").append(",");
if (getInferenceConfiguration() != null)
sb.append("InferenceConfiguration: ").append(getInferenceConfiguration()).append(",");
if (getParserMode() != null)
sb.append("ParserMode: ").append(getParserMode()).append(",");
if (getPromptCreationMode() != null)
sb.append("PromptCreationMode: ").append(getPromptCreationMode()).append(",");
if (getPromptState() != null)
sb.append("PromptState: ").append(getPromptState()).append(",");
if (getPromptType() != null)
sb.append("PromptType: ").append(getPromptType());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof PromptConfiguration == false)
return false;
PromptConfiguration other = (PromptConfiguration) obj;
if (other.getBasePromptTemplate() == null ^ this.getBasePromptTemplate() == null)
return false;
if (other.getBasePromptTemplate() != null && other.getBasePromptTemplate().equals(this.getBasePromptTemplate()) == false)
return false;
if (other.getInferenceConfiguration() == null ^ this.getInferenceConfiguration() == null)
return false;
if (other.getInferenceConfiguration() != null && other.getInferenceConfiguration().equals(this.getInferenceConfiguration()) == false)
return false;
if (other.getParserMode() == null ^ this.getParserMode() == null)
return false;
if (other.getParserMode() != null && other.getParserMode().equals(this.getParserMode()) == false)
return false;
if (other.getPromptCreationMode() == null ^ this.getPromptCreationMode() == null)
return false;
if (other.getPromptCreationMode() != null && other.getPromptCreationMode().equals(this.getPromptCreationMode()) == false)
return false;
if (other.getPromptState() == null ^ this.getPromptState() == null)
return false;
if (other.getPromptState() != null && other.getPromptState().equals(this.getPromptState()) == false)
return false;
if (other.getPromptType() == null ^ this.getPromptType() == null)
return false;
if (other.getPromptType() != null && other.getPromptType().equals(this.getPromptType()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getBasePromptTemplate() == null) ? 0 : getBasePromptTemplate().hashCode());
hashCode = prime * hashCode + ((getInferenceConfiguration() == null) ? 0 : getInferenceConfiguration().hashCode());
hashCode = prime * hashCode + ((getParserMode() == null) ? 0 : getParserMode().hashCode());
hashCode = prime * hashCode + ((getPromptCreationMode() == null) ? 0 : getPromptCreationMode().hashCode());
hashCode = prime * hashCode + ((getPromptState() == null) ? 0 : getPromptState().hashCode());
hashCode = prime * hashCode + ((getPromptType() == null) ? 0 : getPromptType().hashCode());
return hashCode;
}
@Override
public PromptConfiguration clone() {
try {
return (PromptConfiguration) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.bedrockagent.model.transform.PromptConfigurationMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}