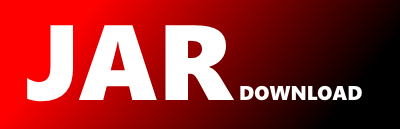
com.amazonaws.services.bedrockagent.model.CreateDataSourceRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-bedrockagent Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.bedrockagent.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateDataSourceRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If this token
* matches a previous request, Amazon Bedrock ignores the request, but does not return an error. For more
* information, see Ensuring
* idempotency.
*
*/
private String clientToken;
/**
*
* The data deletion policy for the data source.
*
*
* You can set the data deletion policy to:
*
*
* -
*
* DELETE: Deletes all underlying data belonging to the data source from the vector store upon deletion of a
* knowledge base or data source resource. Note that the vector store itself is not deleted, only the underlying
* data. This flag is ignored if an Amazon Web Services account is deleted.
*
*
* -
*
* RETAIN: Retains all underlying data in your vector store upon deletion of a knowledge base or data source
* resource.
*
*
*
*/
private String dataDeletionPolicy;
/**
*
* The connection configuration for the data source.
*
*/
private DataSourceConfiguration dataSourceConfiguration;
/**
*
* A description of the data source.
*
*/
private String description;
/**
*
* The unique identifier of the knowledge base to which to add the data source.
*
*/
private String knowledgeBaseId;
/**
*
* The name of the data source.
*
*/
private String name;
/**
*
* Contains details about the server-side encryption for the data source.
*
*/
private ServerSideEncryptionConfiguration serverSideEncryptionConfiguration;
/**
*
* Contains details about how to ingest the documents in the data source.
*
*/
private VectorIngestionConfiguration vectorIngestionConfiguration;
/**
*
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If this token
* matches a previous request, Amazon Bedrock ignores the request, but does not return an error. For more
* information, see Ensuring
* idempotency.
*
*
* @param clientToken
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If
* this token matches a previous request, Amazon Bedrock ignores the request, but does not return an error.
* For more information, see Ensuring
* idempotency.
*/
public void setClientToken(String clientToken) {
this.clientToken = clientToken;
}
/**
*
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If this token
* matches a previous request, Amazon Bedrock ignores the request, but does not return an error. For more
* information, see Ensuring
* idempotency.
*
*
* @return A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If
* this token matches a previous request, Amazon Bedrock ignores the request, but does not return an error.
* For more information, see Ensuring
* idempotency.
*/
public String getClientToken() {
return this.clientToken;
}
/**
*
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If this token
* matches a previous request, Amazon Bedrock ignores the request, but does not return an error. For more
* information, see Ensuring
* idempotency.
*
*
* @param clientToken
* A unique, case-sensitive identifier to ensure that the API request completes no more than one time. If
* this token matches a previous request, Amazon Bedrock ignores the request, but does not return an error.
* For more information, see Ensuring
* idempotency.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataSourceRequest withClientToken(String clientToken) {
setClientToken(clientToken);
return this;
}
/**
*
* The data deletion policy for the data source.
*
*
* You can set the data deletion policy to:
*
*
* -
*
* DELETE: Deletes all underlying data belonging to the data source from the vector store upon deletion of a
* knowledge base or data source resource. Note that the vector store itself is not deleted, only the underlying
* data. This flag is ignored if an Amazon Web Services account is deleted.
*
*
* -
*
* RETAIN: Retains all underlying data in your vector store upon deletion of a knowledge base or data source
* resource.
*
*
*
*
* @param dataDeletionPolicy
* The data deletion policy for the data source.
*
* You can set the data deletion policy to:
*
*
* -
*
* DELETE: Deletes all underlying data belonging to the data source from the vector store upon deletion of a
* knowledge base or data source resource. Note that the vector store itself is not deleted, only the
* underlying data. This flag is ignored if an Amazon Web Services account is deleted.
*
*
* -
*
* RETAIN: Retains all underlying data in your vector store upon deletion of a knowledge base or data source
* resource.
*
*
* @see DataDeletionPolicy
*/
public void setDataDeletionPolicy(String dataDeletionPolicy) {
this.dataDeletionPolicy = dataDeletionPolicy;
}
/**
*
* The data deletion policy for the data source.
*
*
* You can set the data deletion policy to:
*
*
* -
*
* DELETE: Deletes all underlying data belonging to the data source from the vector store upon deletion of a
* knowledge base or data source resource. Note that the vector store itself is not deleted, only the underlying
* data. This flag is ignored if an Amazon Web Services account is deleted.
*
*
* -
*
* RETAIN: Retains all underlying data in your vector store upon deletion of a knowledge base or data source
* resource.
*
*
*
*
* @return The data deletion policy for the data source.
*
* You can set the data deletion policy to:
*
*
* -
*
* DELETE: Deletes all underlying data belonging to the data source from the vector store upon deletion of a
* knowledge base or data source resource. Note that the vector store itself is not deleted, only the
* underlying data. This flag is ignored if an Amazon Web Services account is deleted.
*
*
* -
*
* RETAIN: Retains all underlying data in your vector store upon deletion of a knowledge base or data source
* resource.
*
*
* @see DataDeletionPolicy
*/
public String getDataDeletionPolicy() {
return this.dataDeletionPolicy;
}
/**
*
* The data deletion policy for the data source.
*
*
* You can set the data deletion policy to:
*
*
* -
*
* DELETE: Deletes all underlying data belonging to the data source from the vector store upon deletion of a
* knowledge base or data source resource. Note that the vector store itself is not deleted, only the underlying
* data. This flag is ignored if an Amazon Web Services account is deleted.
*
*
* -
*
* RETAIN: Retains all underlying data in your vector store upon deletion of a knowledge base or data source
* resource.
*
*
*
*
* @param dataDeletionPolicy
* The data deletion policy for the data source.
*
* You can set the data deletion policy to:
*
*
* -
*
* DELETE: Deletes all underlying data belonging to the data source from the vector store upon deletion of a
* knowledge base or data source resource. Note that the vector store itself is not deleted, only the
* underlying data. This flag is ignored if an Amazon Web Services account is deleted.
*
*
* -
*
* RETAIN: Retains all underlying data in your vector store upon deletion of a knowledge base or data source
* resource.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataDeletionPolicy
*/
public CreateDataSourceRequest withDataDeletionPolicy(String dataDeletionPolicy) {
setDataDeletionPolicy(dataDeletionPolicy);
return this;
}
/**
*
* The data deletion policy for the data source.
*
*
* You can set the data deletion policy to:
*
*
* -
*
* DELETE: Deletes all underlying data belonging to the data source from the vector store upon deletion of a
* knowledge base or data source resource. Note that the vector store itself is not deleted, only the underlying
* data. This flag is ignored if an Amazon Web Services account is deleted.
*
*
* -
*
* RETAIN: Retains all underlying data in your vector store upon deletion of a knowledge base or data source
* resource.
*
*
*
*
* @param dataDeletionPolicy
* The data deletion policy for the data source.
*
* You can set the data deletion policy to:
*
*
* -
*
* DELETE: Deletes all underlying data belonging to the data source from the vector store upon deletion of a
* knowledge base or data source resource. Note that the vector store itself is not deleted, only the
* underlying data. This flag is ignored if an Amazon Web Services account is deleted.
*
*
* -
*
* RETAIN: Retains all underlying data in your vector store upon deletion of a knowledge base or data source
* resource.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DataDeletionPolicy
*/
public CreateDataSourceRequest withDataDeletionPolicy(DataDeletionPolicy dataDeletionPolicy) {
this.dataDeletionPolicy = dataDeletionPolicy.toString();
return this;
}
/**
*
* The connection configuration for the data source.
*
*
* @param dataSourceConfiguration
* The connection configuration for the data source.
*/
public void setDataSourceConfiguration(DataSourceConfiguration dataSourceConfiguration) {
this.dataSourceConfiguration = dataSourceConfiguration;
}
/**
*
* The connection configuration for the data source.
*
*
* @return The connection configuration for the data source.
*/
public DataSourceConfiguration getDataSourceConfiguration() {
return this.dataSourceConfiguration;
}
/**
*
* The connection configuration for the data source.
*
*
* @param dataSourceConfiguration
* The connection configuration for the data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataSourceRequest withDataSourceConfiguration(DataSourceConfiguration dataSourceConfiguration) {
setDataSourceConfiguration(dataSourceConfiguration);
return this;
}
/**
*
* A description of the data source.
*
*
* @param description
* A description of the data source.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the data source.
*
*
* @return A description of the data source.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the data source.
*
*
* @param description
* A description of the data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataSourceRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The unique identifier of the knowledge base to which to add the data source.
*
*
* @param knowledgeBaseId
* The unique identifier of the knowledge base to which to add the data source.
*/
public void setKnowledgeBaseId(String knowledgeBaseId) {
this.knowledgeBaseId = knowledgeBaseId;
}
/**
*
* The unique identifier of the knowledge base to which to add the data source.
*
*
* @return The unique identifier of the knowledge base to which to add the data source.
*/
public String getKnowledgeBaseId() {
return this.knowledgeBaseId;
}
/**
*
* The unique identifier of the knowledge base to which to add the data source.
*
*
* @param knowledgeBaseId
* The unique identifier of the knowledge base to which to add the data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataSourceRequest withKnowledgeBaseId(String knowledgeBaseId) {
setKnowledgeBaseId(knowledgeBaseId);
return this;
}
/**
*
* The name of the data source.
*
*
* @param name
* The name of the data source.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the data source.
*
*
* @return The name of the data source.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the data source.
*
*
* @param name
* The name of the data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataSourceRequest withName(String name) {
setName(name);
return this;
}
/**
*
* Contains details about the server-side encryption for the data source.
*
*
* @param serverSideEncryptionConfiguration
* Contains details about the server-side encryption for the data source.
*/
public void setServerSideEncryptionConfiguration(ServerSideEncryptionConfiguration serverSideEncryptionConfiguration) {
this.serverSideEncryptionConfiguration = serverSideEncryptionConfiguration;
}
/**
*
* Contains details about the server-side encryption for the data source.
*
*
* @return Contains details about the server-side encryption for the data source.
*/
public ServerSideEncryptionConfiguration getServerSideEncryptionConfiguration() {
return this.serverSideEncryptionConfiguration;
}
/**
*
* Contains details about the server-side encryption for the data source.
*
*
* @param serverSideEncryptionConfiguration
* Contains details about the server-side encryption for the data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataSourceRequest withServerSideEncryptionConfiguration(ServerSideEncryptionConfiguration serverSideEncryptionConfiguration) {
setServerSideEncryptionConfiguration(serverSideEncryptionConfiguration);
return this;
}
/**
*
* Contains details about how to ingest the documents in the data source.
*
*
* @param vectorIngestionConfiguration
* Contains details about how to ingest the documents in the data source.
*/
public void setVectorIngestionConfiguration(VectorIngestionConfiguration vectorIngestionConfiguration) {
this.vectorIngestionConfiguration = vectorIngestionConfiguration;
}
/**
*
* Contains details about how to ingest the documents in the data source.
*
*
* @return Contains details about how to ingest the documents in the data source.
*/
public VectorIngestionConfiguration getVectorIngestionConfiguration() {
return this.vectorIngestionConfiguration;
}
/**
*
* Contains details about how to ingest the documents in the data source.
*
*
* @param vectorIngestionConfiguration
* Contains details about how to ingest the documents in the data source.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateDataSourceRequest withVectorIngestionConfiguration(VectorIngestionConfiguration vectorIngestionConfiguration) {
setVectorIngestionConfiguration(vectorIngestionConfiguration);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getClientToken() != null)
sb.append("ClientToken: ").append(getClientToken()).append(",");
if (getDataDeletionPolicy() != null)
sb.append("DataDeletionPolicy: ").append(getDataDeletionPolicy()).append(",");
if (getDataSourceConfiguration() != null)
sb.append("DataSourceConfiguration: ").append(getDataSourceConfiguration()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getKnowledgeBaseId() != null)
sb.append("KnowledgeBaseId: ").append(getKnowledgeBaseId()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getServerSideEncryptionConfiguration() != null)
sb.append("ServerSideEncryptionConfiguration: ").append(getServerSideEncryptionConfiguration()).append(",");
if (getVectorIngestionConfiguration() != null)
sb.append("VectorIngestionConfiguration: ").append(getVectorIngestionConfiguration());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateDataSourceRequest == false)
return false;
CreateDataSourceRequest other = (CreateDataSourceRequest) obj;
if (other.getClientToken() == null ^ this.getClientToken() == null)
return false;
if (other.getClientToken() != null && other.getClientToken().equals(this.getClientToken()) == false)
return false;
if (other.getDataDeletionPolicy() == null ^ this.getDataDeletionPolicy() == null)
return false;
if (other.getDataDeletionPolicy() != null && other.getDataDeletionPolicy().equals(this.getDataDeletionPolicy()) == false)
return false;
if (other.getDataSourceConfiguration() == null ^ this.getDataSourceConfiguration() == null)
return false;
if (other.getDataSourceConfiguration() != null && other.getDataSourceConfiguration().equals(this.getDataSourceConfiguration()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getKnowledgeBaseId() == null ^ this.getKnowledgeBaseId() == null)
return false;
if (other.getKnowledgeBaseId() != null && other.getKnowledgeBaseId().equals(this.getKnowledgeBaseId()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getServerSideEncryptionConfiguration() == null ^ this.getServerSideEncryptionConfiguration() == null)
return false;
if (other.getServerSideEncryptionConfiguration() != null
&& other.getServerSideEncryptionConfiguration().equals(this.getServerSideEncryptionConfiguration()) == false)
return false;
if (other.getVectorIngestionConfiguration() == null ^ this.getVectorIngestionConfiguration() == null)
return false;
if (other.getVectorIngestionConfiguration() != null && other.getVectorIngestionConfiguration().equals(this.getVectorIngestionConfiguration()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getClientToken() == null) ? 0 : getClientToken().hashCode());
hashCode = prime * hashCode + ((getDataDeletionPolicy() == null) ? 0 : getDataDeletionPolicy().hashCode());
hashCode = prime * hashCode + ((getDataSourceConfiguration() == null) ? 0 : getDataSourceConfiguration().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getKnowledgeBaseId() == null) ? 0 : getKnowledgeBaseId().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getServerSideEncryptionConfiguration() == null) ? 0 : getServerSideEncryptionConfiguration().hashCode());
hashCode = prime * hashCode + ((getVectorIngestionConfiguration() == null) ? 0 : getVectorIngestionConfiguration().hashCode());
return hashCode;
}
@Override
public CreateDataSourceRequest clone() {
return (CreateDataSourceRequest) super.clone();
}
}