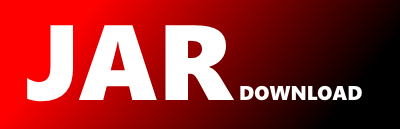
com.amazonaws.services.bedrockagent.model.GetFlowResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-bedrockagent Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.bedrockagent.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class GetFlowResult extends com.amazonaws.AmazonWebServiceResult implements Serializable, Cloneable {
/**
*
* The Amazon Resource Name (ARN) of the flow.
*
*/
private String arn;
/**
*
* The time at which the flow was created.
*
*/
private java.util.Date createdAt;
/**
*
* The Amazon Resource Name (ARN) of the KMS key that the flow is encrypted with.
*
*/
private String customerEncryptionKeyArn;
/**
*
* The definition of the nodes and connections between the nodes in the flow.
*
*/
private FlowDefinition definition;
/**
*
* The description of the flow.
*
*/
private String description;
/**
*
* The Amazon Resource Name (ARN) of the service role with permissions to create a flow. For more information, see
* Create a service row for
* flows in the Amazon Bedrock User Guide.
*
*/
private String executionRoleArn;
/**
*
* The unique identifier of the flow.
*
*/
private String id;
/**
*
* The name of the flow.
*
*/
private String name;
/**
*
* The status of the flow. The following statuses are possible:
*
*
* -
*
* NotPrepared – The flow has been created or updated, but hasn't been prepared. If you just created the flow, you
* can't test it. If you updated the flow, the DRAFT
version won't contain the latest changes for
* testing. Send a PrepareFlow request
* to package the latest changes into the DRAFT
version.
*
*
* -
*
* Preparing – The flow is being prepared so that the DRAFT
version contains the latest changes for
* testing.
*
*
* -
*
* Prepared – The flow is prepared and the DRAFT
version contains the latest changes for testing.
*
*
* -
*
* Failed – The last API operation that you invoked on the flow failed. Send a GetFlow request and
* check the error message in the validations
field.
*
*
*
*/
private String status;
/**
*
* The time at which the flow was last updated.
*
*/
private java.util.Date updatedAt;
/**
*
* A list of validation error messages related to the last failed operation on the flow.
*
*/
private java.util.List validations;
/**
*
* The version of the flow for which information was retrieved.
*
*/
private String version;
/**
*
* The Amazon Resource Name (ARN) of the flow.
*
*
* @param arn
* The Amazon Resource Name (ARN) of the flow.
*/
public void setArn(String arn) {
this.arn = arn;
}
/**
*
* The Amazon Resource Name (ARN) of the flow.
*
*
* @return The Amazon Resource Name (ARN) of the flow.
*/
public String getArn() {
return this.arn;
}
/**
*
* The Amazon Resource Name (ARN) of the flow.
*
*
* @param arn
* The Amazon Resource Name (ARN) of the flow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetFlowResult withArn(String arn) {
setArn(arn);
return this;
}
/**
*
* The time at which the flow was created.
*
*
* @param createdAt
* The time at which the flow was created.
*/
public void setCreatedAt(java.util.Date createdAt) {
this.createdAt = createdAt;
}
/**
*
* The time at which the flow was created.
*
*
* @return The time at which the flow was created.
*/
public java.util.Date getCreatedAt() {
return this.createdAt;
}
/**
*
* The time at which the flow was created.
*
*
* @param createdAt
* The time at which the flow was created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetFlowResult withCreatedAt(java.util.Date createdAt) {
setCreatedAt(createdAt);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the KMS key that the flow is encrypted with.
*
*
* @param customerEncryptionKeyArn
* The Amazon Resource Name (ARN) of the KMS key that the flow is encrypted with.
*/
public void setCustomerEncryptionKeyArn(String customerEncryptionKeyArn) {
this.customerEncryptionKeyArn = customerEncryptionKeyArn;
}
/**
*
* The Amazon Resource Name (ARN) of the KMS key that the flow is encrypted with.
*
*
* @return The Amazon Resource Name (ARN) of the KMS key that the flow is encrypted with.
*/
public String getCustomerEncryptionKeyArn() {
return this.customerEncryptionKeyArn;
}
/**
*
* The Amazon Resource Name (ARN) of the KMS key that the flow is encrypted with.
*
*
* @param customerEncryptionKeyArn
* The Amazon Resource Name (ARN) of the KMS key that the flow is encrypted with.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetFlowResult withCustomerEncryptionKeyArn(String customerEncryptionKeyArn) {
setCustomerEncryptionKeyArn(customerEncryptionKeyArn);
return this;
}
/**
*
* The definition of the nodes and connections between the nodes in the flow.
*
*
* @param definition
* The definition of the nodes and connections between the nodes in the flow.
*/
public void setDefinition(FlowDefinition definition) {
this.definition = definition;
}
/**
*
* The definition of the nodes and connections between the nodes in the flow.
*
*
* @return The definition of the nodes and connections between the nodes in the flow.
*/
public FlowDefinition getDefinition() {
return this.definition;
}
/**
*
* The definition of the nodes and connections between the nodes in the flow.
*
*
* @param definition
* The definition of the nodes and connections between the nodes in the flow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetFlowResult withDefinition(FlowDefinition definition) {
setDefinition(definition);
return this;
}
/**
*
* The description of the flow.
*
*
* @param description
* The description of the flow.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The description of the flow.
*
*
* @return The description of the flow.
*/
public String getDescription() {
return this.description;
}
/**
*
* The description of the flow.
*
*
* @param description
* The description of the flow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetFlowResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the service role with permissions to create a flow. For more information, see
* Create a service row for
* flows in the Amazon Bedrock User Guide.
*
*
* @param executionRoleArn
* The Amazon Resource Name (ARN) of the service role with permissions to create a flow. For more
* information, see Create a service row
* for flows in the Amazon Bedrock User Guide.
*/
public void setExecutionRoleArn(String executionRoleArn) {
this.executionRoleArn = executionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the service role with permissions to create a flow. For more information, see
* Create a service row for
* flows in the Amazon Bedrock User Guide.
*
*
* @return The Amazon Resource Name (ARN) of the service role with permissions to create a flow. For more
* information, see Create a service row
* for flows in the Amazon Bedrock User Guide.
*/
public String getExecutionRoleArn() {
return this.executionRoleArn;
}
/**
*
* The Amazon Resource Name (ARN) of the service role with permissions to create a flow. For more information, see
* Create a service row for
* flows in the Amazon Bedrock User Guide.
*
*
* @param executionRoleArn
* The Amazon Resource Name (ARN) of the service role with permissions to create a flow. For more
* information, see Create a service row
* for flows in the Amazon Bedrock User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetFlowResult withExecutionRoleArn(String executionRoleArn) {
setExecutionRoleArn(executionRoleArn);
return this;
}
/**
*
* The unique identifier of the flow.
*
*
* @param id
* The unique identifier of the flow.
*/
public void setId(String id) {
this.id = id;
}
/**
*
* The unique identifier of the flow.
*
*
* @return The unique identifier of the flow.
*/
public String getId() {
return this.id;
}
/**
*
* The unique identifier of the flow.
*
*
* @param id
* The unique identifier of the flow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetFlowResult withId(String id) {
setId(id);
return this;
}
/**
*
* The name of the flow.
*
*
* @param name
* The name of the flow.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* The name of the flow.
*
*
* @return The name of the flow.
*/
public String getName() {
return this.name;
}
/**
*
* The name of the flow.
*
*
* @param name
* The name of the flow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetFlowResult withName(String name) {
setName(name);
return this;
}
/**
*
* The status of the flow. The following statuses are possible:
*
*
* -
*
* NotPrepared – The flow has been created or updated, but hasn't been prepared. If you just created the flow, you
* can't test it. If you updated the flow, the DRAFT
version won't contain the latest changes for
* testing. Send a PrepareFlow request
* to package the latest changes into the DRAFT
version.
*
*
* -
*
* Preparing – The flow is being prepared so that the DRAFT
version contains the latest changes for
* testing.
*
*
* -
*
* Prepared – The flow is prepared and the DRAFT
version contains the latest changes for testing.
*
*
* -
*
* Failed – The last API operation that you invoked on the flow failed. Send a GetFlow request and
* check the error message in the validations
field.
*
*
*
*
* @param status
* The status of the flow. The following statuses are possible:
*
* -
*
* NotPrepared – The flow has been created or updated, but hasn't been prepared. If you just created the
* flow, you can't test it. If you updated the flow, the DRAFT
version won't contain the latest
* changes for testing. Send a PrepareFlow
* request to package the latest changes into the DRAFT
version.
*
*
* -
*
* Preparing – The flow is being prepared so that the DRAFT
version contains the latest changes
* for testing.
*
*
* -
*
* Prepared – The flow is prepared and the DRAFT
version contains the latest changes for
* testing.
*
*
* -
*
* Failed – The last API operation that you invoked on the flow failed. Send a GetFlow request
* and check the error message in the validations
field.
*
*
* @see FlowStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the flow. The following statuses are possible:
*
*
* -
*
* NotPrepared – The flow has been created or updated, but hasn't been prepared. If you just created the flow, you
* can't test it. If you updated the flow, the DRAFT
version won't contain the latest changes for
* testing. Send a PrepareFlow request
* to package the latest changes into the DRAFT
version.
*
*
* -
*
* Preparing – The flow is being prepared so that the DRAFT
version contains the latest changes for
* testing.
*
*
* -
*
* Prepared – The flow is prepared and the DRAFT
version contains the latest changes for testing.
*
*
* -
*
* Failed – The last API operation that you invoked on the flow failed. Send a GetFlow request and
* check the error message in the validations
field.
*
*
*
*
* @return The status of the flow. The following statuses are possible:
*
* -
*
* NotPrepared – The flow has been created or updated, but hasn't been prepared. If you just created the
* flow, you can't test it. If you updated the flow, the DRAFT
version won't contain the latest
* changes for testing. Send a PrepareFlow
* request to package the latest changes into the DRAFT
version.
*
*
* -
*
* Preparing – The flow is being prepared so that the DRAFT
version contains the latest changes
* for testing.
*
*
* -
*
* Prepared – The flow is prepared and the DRAFT
version contains the latest changes for
* testing.
*
*
* -
*
* Failed – The last API operation that you invoked on the flow failed. Send a GetFlow request
* and check the error message in the validations
field.
*
*
* @see FlowStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the flow. The following statuses are possible:
*
*
* -
*
* NotPrepared – The flow has been created or updated, but hasn't been prepared. If you just created the flow, you
* can't test it. If you updated the flow, the DRAFT
version won't contain the latest changes for
* testing. Send a PrepareFlow request
* to package the latest changes into the DRAFT
version.
*
*
* -
*
* Preparing – The flow is being prepared so that the DRAFT
version contains the latest changes for
* testing.
*
*
* -
*
* Prepared – The flow is prepared and the DRAFT
version contains the latest changes for testing.
*
*
* -
*
* Failed – The last API operation that you invoked on the flow failed. Send a GetFlow request and
* check the error message in the validations
field.
*
*
*
*
* @param status
* The status of the flow. The following statuses are possible:
*
* -
*
* NotPrepared – The flow has been created or updated, but hasn't been prepared. If you just created the
* flow, you can't test it. If you updated the flow, the DRAFT
version won't contain the latest
* changes for testing. Send a PrepareFlow
* request to package the latest changes into the DRAFT
version.
*
*
* -
*
* Preparing – The flow is being prepared so that the DRAFT
version contains the latest changes
* for testing.
*
*
* -
*
* Prepared – The flow is prepared and the DRAFT
version contains the latest changes for
* testing.
*
*
* -
*
* Failed – The last API operation that you invoked on the flow failed. Send a GetFlow request
* and check the error message in the validations
field.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see FlowStatus
*/
public GetFlowResult withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the flow. The following statuses are possible:
*
*
* -
*
* NotPrepared – The flow has been created or updated, but hasn't been prepared. If you just created the flow, you
* can't test it. If you updated the flow, the DRAFT
version won't contain the latest changes for
* testing. Send a PrepareFlow request
* to package the latest changes into the DRAFT
version.
*
*
* -
*
* Preparing – The flow is being prepared so that the DRAFT
version contains the latest changes for
* testing.
*
*
* -
*
* Prepared – The flow is prepared and the DRAFT
version contains the latest changes for testing.
*
*
* -
*
* Failed – The last API operation that you invoked on the flow failed. Send a GetFlow request and
* check the error message in the validations
field.
*
*
*
*
* @param status
* The status of the flow. The following statuses are possible:
*
* -
*
* NotPrepared – The flow has been created or updated, but hasn't been prepared. If you just created the
* flow, you can't test it. If you updated the flow, the DRAFT
version won't contain the latest
* changes for testing. Send a PrepareFlow
* request to package the latest changes into the DRAFT
version.
*
*
* -
*
* Preparing – The flow is being prepared so that the DRAFT
version contains the latest changes
* for testing.
*
*
* -
*
* Prepared – The flow is prepared and the DRAFT
version contains the latest changes for
* testing.
*
*
* -
*
* Failed – The last API operation that you invoked on the flow failed. Send a GetFlow request
* and check the error message in the validations
field.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see FlowStatus
*/
public GetFlowResult withStatus(FlowStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The time at which the flow was last updated.
*
*
* @param updatedAt
* The time at which the flow was last updated.
*/
public void setUpdatedAt(java.util.Date updatedAt) {
this.updatedAt = updatedAt;
}
/**
*
* The time at which the flow was last updated.
*
*
* @return The time at which the flow was last updated.
*/
public java.util.Date getUpdatedAt() {
return this.updatedAt;
}
/**
*
* The time at which the flow was last updated.
*
*
* @param updatedAt
* The time at which the flow was last updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetFlowResult withUpdatedAt(java.util.Date updatedAt) {
setUpdatedAt(updatedAt);
return this;
}
/**
*
* A list of validation error messages related to the last failed operation on the flow.
*
*
* @return A list of validation error messages related to the last failed operation on the flow.
*/
public java.util.List getValidations() {
return validations;
}
/**
*
* A list of validation error messages related to the last failed operation on the flow.
*
*
* @param validations
* A list of validation error messages related to the last failed operation on the flow.
*/
public void setValidations(java.util.Collection validations) {
if (validations == null) {
this.validations = null;
return;
}
this.validations = new java.util.ArrayList(validations);
}
/**
*
* A list of validation error messages related to the last failed operation on the flow.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setValidations(java.util.Collection)} or {@link #withValidations(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param validations
* A list of validation error messages related to the last failed operation on the flow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetFlowResult withValidations(FlowValidation... validations) {
if (this.validations == null) {
setValidations(new java.util.ArrayList(validations.length));
}
for (FlowValidation ele : validations) {
this.validations.add(ele);
}
return this;
}
/**
*
* A list of validation error messages related to the last failed operation on the flow.
*
*
* @param validations
* A list of validation error messages related to the last failed operation on the flow.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetFlowResult withValidations(java.util.Collection validations) {
setValidations(validations);
return this;
}
/**
*
* The version of the flow for which information was retrieved.
*
*
* @param version
* The version of the flow for which information was retrieved.
*/
public void setVersion(String version) {
this.version = version;
}
/**
*
* The version of the flow for which information was retrieved.
*
*
* @return The version of the flow for which information was retrieved.
*/
public String getVersion() {
return this.version;
}
/**
*
* The version of the flow for which information was retrieved.
*
*
* @param version
* The version of the flow for which information was retrieved.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public GetFlowResult withVersion(String version) {
setVersion(version);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getArn() != null)
sb.append("Arn: ").append(getArn()).append(",");
if (getCreatedAt() != null)
sb.append("CreatedAt: ").append(getCreatedAt()).append(",");
if (getCustomerEncryptionKeyArn() != null)
sb.append("CustomerEncryptionKeyArn: ").append(getCustomerEncryptionKeyArn()).append(",");
if (getDefinition() != null)
sb.append("Definition: ").append(getDefinition()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getExecutionRoleArn() != null)
sb.append("ExecutionRoleArn: ").append(getExecutionRoleArn()).append(",");
if (getId() != null)
sb.append("Id: ").append(getId()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getUpdatedAt() != null)
sb.append("UpdatedAt: ").append(getUpdatedAt()).append(",");
if (getValidations() != null)
sb.append("Validations: ").append(getValidations()).append(",");
if (getVersion() != null)
sb.append("Version: ").append(getVersion());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetFlowResult == false)
return false;
GetFlowResult other = (GetFlowResult) obj;
if (other.getArn() == null ^ this.getArn() == null)
return false;
if (other.getArn() != null && other.getArn().equals(this.getArn()) == false)
return false;
if (other.getCreatedAt() == null ^ this.getCreatedAt() == null)
return false;
if (other.getCreatedAt() != null && other.getCreatedAt().equals(this.getCreatedAt()) == false)
return false;
if (other.getCustomerEncryptionKeyArn() == null ^ this.getCustomerEncryptionKeyArn() == null)
return false;
if (other.getCustomerEncryptionKeyArn() != null && other.getCustomerEncryptionKeyArn().equals(this.getCustomerEncryptionKeyArn()) == false)
return false;
if (other.getDefinition() == null ^ this.getDefinition() == null)
return false;
if (other.getDefinition() != null && other.getDefinition().equals(this.getDefinition()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getExecutionRoleArn() == null ^ this.getExecutionRoleArn() == null)
return false;
if (other.getExecutionRoleArn() != null && other.getExecutionRoleArn().equals(this.getExecutionRoleArn()) == false)
return false;
if (other.getId() == null ^ this.getId() == null)
return false;
if (other.getId() != null && other.getId().equals(this.getId()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getUpdatedAt() == null ^ this.getUpdatedAt() == null)
return false;
if (other.getUpdatedAt() != null && other.getUpdatedAt().equals(this.getUpdatedAt()) == false)
return false;
if (other.getValidations() == null ^ this.getValidations() == null)
return false;
if (other.getValidations() != null && other.getValidations().equals(this.getValidations()) == false)
return false;
if (other.getVersion() == null ^ this.getVersion() == null)
return false;
if (other.getVersion() != null && other.getVersion().equals(this.getVersion()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getArn() == null) ? 0 : getArn().hashCode());
hashCode = prime * hashCode + ((getCreatedAt() == null) ? 0 : getCreatedAt().hashCode());
hashCode = prime * hashCode + ((getCustomerEncryptionKeyArn() == null) ? 0 : getCustomerEncryptionKeyArn().hashCode());
hashCode = prime * hashCode + ((getDefinition() == null) ? 0 : getDefinition().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getExecutionRoleArn() == null) ? 0 : getExecutionRoleArn().hashCode());
hashCode = prime * hashCode + ((getId() == null) ? 0 : getId().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getUpdatedAt() == null) ? 0 : getUpdatedAt().hashCode());
hashCode = prime * hashCode + ((getValidations() == null) ? 0 : getValidations().hashCode());
hashCode = prime * hashCode + ((getVersion() == null) ? 0 : getVersion().hashCode());
return hashCode;
}
@Override
public GetFlowResult clone() {
try {
return (GetFlowResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}