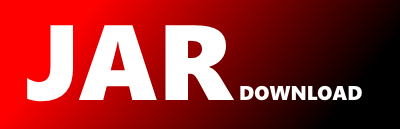
com.amazonaws.services.billingconductor.AWSBillingConductorAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-billingconductor Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.billingconductor;
import javax.annotation.Generated;
import com.amazonaws.services.billingconductor.model.*;
/**
* Interface for accessing AWSBillingConductor asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.billingconductor.AbstractAWSBillingConductorAsync} instead.
*
*
*
* Amazon Web Services Billing Conductor is a fully managed service that you can use to customize a proforma version of your billing data each month, to accurately show or chargeback your end customers. Amazon
* Web Services Billing Conductor doesn't change the way you're billed by Amazon Web Services each month by design.
* Instead, it provides you with a mechanism to configure, generate, and display rates to certain customers over a given
* billing period. You can also analyze the difference between the rates you apply to your accounting groupings relative
* to your actual rates from Amazon Web Services. As a result of your Amazon Web Services Billing Conductor
* configuration, the payer account can also see the custom rate applied on the billing details page of the Amazon Web Services Billing console, or configure a cost and usage
* report per billing group.
*
*
* This documentation shows how you can configure Amazon Web Services Billing Conductor using its API. For more
* information about using the Amazon Web Services Billing
* Conductor user interface, see the Amazon Web
* Services Billing Conductor User Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSBillingConductorAsync extends AWSBillingConductor {
/**
*
* Connects an array of account IDs in a consolidated billing family to a predefined billing group. The account IDs
* must be a part of the consolidated billing family during the current month, and not already associated with
* another billing group. The maximum number of accounts that can be associated in one call is 30.
*
*
* @param associateAccountsRequest
* @return A Java Future containing the result of the AssociateAccounts operation returned by the service.
* @sample AWSBillingConductorAsync.AssociateAccounts
* @see AWS API Documentation
*/
java.util.concurrent.Future associateAccountsAsync(AssociateAccountsRequest associateAccountsRequest);
/**
*
* Connects an array of account IDs in a consolidated billing family to a predefined billing group. The account IDs
* must be a part of the consolidated billing family during the current month, and not already associated with
* another billing group. The maximum number of accounts that can be associated in one call is 30.
*
*
* @param associateAccountsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateAccounts operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.AssociateAccounts
* @see AWS API Documentation
*/
java.util.concurrent.Future associateAccountsAsync(AssociateAccountsRequest associateAccountsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Connects an array of PricingRuleArns
to a defined PricingPlan
. The maximum number
* PricingRuleArn
that can be associated in one call is 30.
*
*
* @param associatePricingRulesRequest
* @return A Java Future containing the result of the AssociatePricingRules operation returned by the service.
* @sample AWSBillingConductorAsync.AssociatePricingRules
* @see AWS API Documentation
*/
java.util.concurrent.Future associatePricingRulesAsync(AssociatePricingRulesRequest associatePricingRulesRequest);
/**
*
* Connects an array of PricingRuleArns
to a defined PricingPlan
. The maximum number
* PricingRuleArn
that can be associated in one call is 30.
*
*
* @param associatePricingRulesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociatePricingRules operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.AssociatePricingRules
* @see AWS API Documentation
*/
java.util.concurrent.Future associatePricingRulesAsync(AssociatePricingRulesRequest associatePricingRulesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates a batch of resources to a percentage custom line item.
*
*
* @param batchAssociateResourcesToCustomLineItemRequest
* @return A Java Future containing the result of the BatchAssociateResourcesToCustomLineItem operation returned by
* the service.
* @sample AWSBillingConductorAsync.BatchAssociateResourcesToCustomLineItem
* @see AWS API Documentation
*/
java.util.concurrent.Future batchAssociateResourcesToCustomLineItemAsync(
BatchAssociateResourcesToCustomLineItemRequest batchAssociateResourcesToCustomLineItemRequest);
/**
*
* Associates a batch of resources to a percentage custom line item.
*
*
* @param batchAssociateResourcesToCustomLineItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchAssociateResourcesToCustomLineItem operation returned by
* the service.
* @sample AWSBillingConductorAsyncHandler.BatchAssociateResourcesToCustomLineItem
* @see AWS API Documentation
*/
java.util.concurrent.Future batchAssociateResourcesToCustomLineItemAsync(
BatchAssociateResourcesToCustomLineItemRequest batchAssociateResourcesToCustomLineItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a batch of resources from a percentage custom line item.
*
*
* @param batchDisassociateResourcesFromCustomLineItemRequest
* @return A Java Future containing the result of the BatchDisassociateResourcesFromCustomLineItem operation
* returned by the service.
* @sample AWSBillingConductorAsync.BatchDisassociateResourcesFromCustomLineItem
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDisassociateResourcesFromCustomLineItemAsync(
BatchDisassociateResourcesFromCustomLineItemRequest batchDisassociateResourcesFromCustomLineItemRequest);
/**
*
* Disassociates a batch of resources from a percentage custom line item.
*
*
* @param batchDisassociateResourcesFromCustomLineItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDisassociateResourcesFromCustomLineItem operation
* returned by the service.
* @sample AWSBillingConductorAsyncHandler.BatchDisassociateResourcesFromCustomLineItem
* @see AWS API Documentation
*/
java.util.concurrent.Future batchDisassociateResourcesFromCustomLineItemAsync(
BatchDisassociateResourcesFromCustomLineItemRequest batchDisassociateResourcesFromCustomLineItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a billing group that resembles a consolidated billing family that Amazon Web Services charges, based off
* of the predefined pricing plan computation.
*
*
* @param createBillingGroupRequest
* @return A Java Future containing the result of the CreateBillingGroup operation returned by the service.
* @sample AWSBillingConductorAsync.CreateBillingGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createBillingGroupAsync(CreateBillingGroupRequest createBillingGroupRequest);
/**
*
* Creates a billing group that resembles a consolidated billing family that Amazon Web Services charges, based off
* of the predefined pricing plan computation.
*
*
* @param createBillingGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateBillingGroup operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.CreateBillingGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future createBillingGroupAsync(CreateBillingGroupRequest createBillingGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a custom line item that can be used to create a one-time fixed charge that can be applied to a single
* billing group for the current or previous billing period. The one-time fixed charge is either a fee or discount.
*
*
* @param createCustomLineItemRequest
* @return A Java Future containing the result of the CreateCustomLineItem operation returned by the service.
* @sample AWSBillingConductorAsync.CreateCustomLineItem
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomLineItemAsync(CreateCustomLineItemRequest createCustomLineItemRequest);
/**
*
* Creates a custom line item that can be used to create a one-time fixed charge that can be applied to a single
* billing group for the current or previous billing period. The one-time fixed charge is either a fee or discount.
*
*
* @param createCustomLineItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateCustomLineItem operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.CreateCustomLineItem
* @see AWS API Documentation
*/
java.util.concurrent.Future createCustomLineItemAsync(CreateCustomLineItemRequest createCustomLineItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a pricing plan that is used for computing Amazon Web Services charges for billing groups.
*
*
* @param createPricingPlanRequest
* @return A Java Future containing the result of the CreatePricingPlan operation returned by the service.
* @sample AWSBillingConductorAsync.CreatePricingPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future createPricingPlanAsync(CreatePricingPlanRequest createPricingPlanRequest);
/**
*
* Creates a pricing plan that is used for computing Amazon Web Services charges for billing groups.
*
*
* @param createPricingPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePricingPlan operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.CreatePricingPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future createPricingPlanAsync(CreatePricingPlanRequest createPricingPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a pricing rule can be associated to a pricing plan, or a set of pricing plans.
*
*
* @param createPricingRuleRequest
* @return A Java Future containing the result of the CreatePricingRule operation returned by the service.
* @sample AWSBillingConductorAsync.CreatePricingRule
* @see AWS API Documentation
*/
java.util.concurrent.Future createPricingRuleAsync(CreatePricingRuleRequest createPricingRuleRequest);
/**
*
* Creates a pricing rule can be associated to a pricing plan, or a set of pricing plans.
*
*
* @param createPricingRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePricingRule operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.CreatePricingRule
* @see AWS API Documentation
*/
java.util.concurrent.Future createPricingRuleAsync(CreatePricingRuleRequest createPricingRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a billing group.
*
*
* @param deleteBillingGroupRequest
* @return A Java Future containing the result of the DeleteBillingGroup operation returned by the service.
* @sample AWSBillingConductorAsync.DeleteBillingGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBillingGroupAsync(DeleteBillingGroupRequest deleteBillingGroupRequest);
/**
*
* Deletes a billing group.
*
*
* @param deleteBillingGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteBillingGroup operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.DeleteBillingGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteBillingGroupAsync(DeleteBillingGroupRequest deleteBillingGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the custom line item identified by the given ARN in the current, or previous billing period.
*
*
* @param deleteCustomLineItemRequest
* @return A Java Future containing the result of the DeleteCustomLineItem operation returned by the service.
* @sample AWSBillingConductorAsync.DeleteCustomLineItem
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomLineItemAsync(DeleteCustomLineItemRequest deleteCustomLineItemRequest);
/**
*
* Deletes the custom line item identified by the given ARN in the current, or previous billing period.
*
*
* @param deleteCustomLineItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteCustomLineItem operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.DeleteCustomLineItem
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteCustomLineItemAsync(DeleteCustomLineItemRequest deleteCustomLineItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a pricing plan. The pricing plan must not be associated with any billing groups to delete successfully.
*
*
* @param deletePricingPlanRequest
* @return A Java Future containing the result of the DeletePricingPlan operation returned by the service.
* @sample AWSBillingConductorAsync.DeletePricingPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePricingPlanAsync(DeletePricingPlanRequest deletePricingPlanRequest);
/**
*
* Deletes a pricing plan. The pricing plan must not be associated with any billing groups to delete successfully.
*
*
* @param deletePricingPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePricingPlan operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.DeletePricingPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePricingPlanAsync(DeletePricingPlanRequest deletePricingPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the pricing rule that's identified by the input Amazon Resource Name (ARN).
*
*
* @param deletePricingRuleRequest
* @return A Java Future containing the result of the DeletePricingRule operation returned by the service.
* @sample AWSBillingConductorAsync.DeletePricingRule
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePricingRuleAsync(DeletePricingRuleRequest deletePricingRuleRequest);
/**
*
* Deletes the pricing rule that's identified by the input Amazon Resource Name (ARN).
*
*
* @param deletePricingRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePricingRule operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.DeletePricingRule
* @see AWS API Documentation
*/
java.util.concurrent.Future deletePricingRuleAsync(DeletePricingRuleRequest deletePricingRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified list of account IDs from the given billing group.
*
*
* @param disassociateAccountsRequest
* @return A Java Future containing the result of the DisassociateAccounts operation returned by the service.
* @sample AWSBillingConductorAsync.DisassociateAccounts
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateAccountsAsync(DisassociateAccountsRequest disassociateAccountsRequest);
/**
*
* Removes the specified list of account IDs from the given billing group.
*
*
* @param disassociateAccountsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateAccounts operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.DisassociateAccounts
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateAccountsAsync(DisassociateAccountsRequest disassociateAccountsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates a list of pricing rules from a pricing plan.
*
*
* @param disassociatePricingRulesRequest
* @return A Java Future containing the result of the DisassociatePricingRules operation returned by the service.
* @sample AWSBillingConductorAsync.DisassociatePricingRules
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociatePricingRulesAsync(DisassociatePricingRulesRequest disassociatePricingRulesRequest);
/**
*
* Disassociates a list of pricing rules from a pricing plan.
*
*
* @param disassociatePricingRulesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociatePricingRules operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.DisassociatePricingRules
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociatePricingRulesAsync(DisassociatePricingRulesRequest disassociatePricingRulesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the margin summary report, which includes the Amazon Web Services cost and charged amount (pro forma
* cost) by Amazon Web Service for a specific billing group.
*
*
* @param getBillingGroupCostReportRequest
* @return A Java Future containing the result of the GetBillingGroupCostReport operation returned by the service.
* @sample AWSBillingConductorAsync.GetBillingGroupCostReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getBillingGroupCostReportAsync(
GetBillingGroupCostReportRequest getBillingGroupCostReportRequest);
/**
*
* Retrieves the margin summary report, which includes the Amazon Web Services cost and charged amount (pro forma
* cost) by Amazon Web Service for a specific billing group.
*
*
* @param getBillingGroupCostReportRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBillingGroupCostReport operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.GetBillingGroupCostReport
* @see AWS API Documentation
*/
java.util.concurrent.Future getBillingGroupCostReportAsync(
GetBillingGroupCostReportRequest getBillingGroupCostReportRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This is a paginated call to list linked accounts that are linked to the payer account for the specified time
* period. If no information is provided, the current billing period is used. The response will optionally include
* the billing group that's associated with the linked account.
*
*
* @param listAccountAssociationsRequest
* @return A Java Future containing the result of the ListAccountAssociations operation returned by the service.
* @sample AWSBillingConductorAsync.ListAccountAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future listAccountAssociationsAsync(ListAccountAssociationsRequest listAccountAssociationsRequest);
/**
*
* This is a paginated call to list linked accounts that are linked to the payer account for the specified time
* period. If no information is provided, the current billing period is used. The response will optionally include
* the billing group that's associated with the linked account.
*
*
* @param listAccountAssociationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAccountAssociations operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.ListAccountAssociations
* @see AWS API Documentation
*/
java.util.concurrent.Future listAccountAssociationsAsync(ListAccountAssociationsRequest listAccountAssociationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A paginated call to retrieve a summary report of actual Amazon Web Services charges and the calculated Amazon Web
* Services charges based on the associated pricing plan of a billing group.
*
*
* @param listBillingGroupCostReportsRequest
* @return A Java Future containing the result of the ListBillingGroupCostReports operation returned by the service.
* @sample AWSBillingConductorAsync.ListBillingGroupCostReports
* @see AWS API Documentation
*/
java.util.concurrent.Future listBillingGroupCostReportsAsync(
ListBillingGroupCostReportsRequest listBillingGroupCostReportsRequest);
/**
*
* A paginated call to retrieve a summary report of actual Amazon Web Services charges and the calculated Amazon Web
* Services charges based on the associated pricing plan of a billing group.
*
*
* @param listBillingGroupCostReportsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListBillingGroupCostReports operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.ListBillingGroupCostReports
* @see AWS API Documentation
*/
java.util.concurrent.Future listBillingGroupCostReportsAsync(
ListBillingGroupCostReportsRequest listBillingGroupCostReportsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A paginated call to retrieve a list of billing groups for the given billing period. If you don't provide a
* billing group, the current billing period is used.
*
*
* @param listBillingGroupsRequest
* @return A Java Future containing the result of the ListBillingGroups operation returned by the service.
* @sample AWSBillingConductorAsync.ListBillingGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listBillingGroupsAsync(ListBillingGroupsRequest listBillingGroupsRequest);
/**
*
* A paginated call to retrieve a list of billing groups for the given billing period. If you don't provide a
* billing group, the current billing period is used.
*
*
* @param listBillingGroupsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListBillingGroups operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.ListBillingGroups
* @see AWS API Documentation
*/
java.util.concurrent.Future listBillingGroupsAsync(ListBillingGroupsRequest listBillingGroupsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A paginated call to get a list of all custom line item versions.
*
*
* @param listCustomLineItemVersionsRequest
* @return A Java Future containing the result of the ListCustomLineItemVersions operation returned by the service.
* @sample AWSBillingConductorAsync.ListCustomLineItemVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future listCustomLineItemVersionsAsync(
ListCustomLineItemVersionsRequest listCustomLineItemVersionsRequest);
/**
*
* A paginated call to get a list of all custom line item versions.
*
*
* @param listCustomLineItemVersionsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCustomLineItemVersions operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.ListCustomLineItemVersions
* @see AWS API Documentation
*/
java.util.concurrent.Future listCustomLineItemVersionsAsync(
ListCustomLineItemVersionsRequest listCustomLineItemVersionsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A paginated call to get a list of all custom line items (FFLIs) for the given billing period. If you don't
* provide a billing period, the current billing period is used.
*
*
* @param listCustomLineItemsRequest
* @return A Java Future containing the result of the ListCustomLineItems operation returned by the service.
* @sample AWSBillingConductorAsync.ListCustomLineItems
* @see AWS API Documentation
*/
java.util.concurrent.Future listCustomLineItemsAsync(ListCustomLineItemsRequest listCustomLineItemsRequest);
/**
*
* A paginated call to get a list of all custom line items (FFLIs) for the given billing period. If you don't
* provide a billing period, the current billing period is used.
*
*
* @param listCustomLineItemsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListCustomLineItems operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.ListCustomLineItems
* @see AWS API Documentation
*/
java.util.concurrent.Future listCustomLineItemsAsync(ListCustomLineItemsRequest listCustomLineItemsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A paginated call to get pricing plans for the given billing period. If you don't provide a billing period, the
* current billing period is used.
*
*
* @param listPricingPlansRequest
* @return A Java Future containing the result of the ListPricingPlans operation returned by the service.
* @sample AWSBillingConductorAsync.ListPricingPlans
* @see AWS API Documentation
*/
java.util.concurrent.Future listPricingPlansAsync(ListPricingPlansRequest listPricingPlansRequest);
/**
*
* A paginated call to get pricing plans for the given billing period. If you don't provide a billing period, the
* current billing period is used.
*
*
* @param listPricingPlansRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPricingPlans operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.ListPricingPlans
* @see AWS API Documentation
*/
java.util.concurrent.Future listPricingPlansAsync(ListPricingPlansRequest listPricingPlansRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A list of the pricing plans that are associated with a pricing rule.
*
*
* @param listPricingPlansAssociatedWithPricingRuleRequest
* @return A Java Future containing the result of the ListPricingPlansAssociatedWithPricingRule operation returned
* by the service.
* @sample AWSBillingConductorAsync.ListPricingPlansAssociatedWithPricingRule
* @see AWS API Documentation
*/
java.util.concurrent.Future listPricingPlansAssociatedWithPricingRuleAsync(
ListPricingPlansAssociatedWithPricingRuleRequest listPricingPlansAssociatedWithPricingRuleRequest);
/**
*
* A list of the pricing plans that are associated with a pricing rule.
*
*
* @param listPricingPlansAssociatedWithPricingRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPricingPlansAssociatedWithPricingRule operation returned
* by the service.
* @sample AWSBillingConductorAsyncHandler.ListPricingPlansAssociatedWithPricingRule
* @see AWS API Documentation
*/
java.util.concurrent.Future listPricingPlansAssociatedWithPricingRuleAsync(
ListPricingPlansAssociatedWithPricingRuleRequest listPricingPlansAssociatedWithPricingRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Describes a pricing rule that can be associated to a pricing plan, or set of pricing plans.
*
*
* @param listPricingRulesRequest
* @return A Java Future containing the result of the ListPricingRules operation returned by the service.
* @sample AWSBillingConductorAsync.ListPricingRules
* @see AWS API Documentation
*/
java.util.concurrent.Future listPricingRulesAsync(ListPricingRulesRequest listPricingRulesRequest);
/**
*
* Describes a pricing rule that can be associated to a pricing plan, or set of pricing plans.
*
*
* @param listPricingRulesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPricingRules operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.ListPricingRules
* @see AWS API Documentation
*/
java.util.concurrent.Future listPricingRulesAsync(ListPricingRulesRequest listPricingRulesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the pricing rules that are associated with a pricing plan.
*
*
* @param listPricingRulesAssociatedToPricingPlanRequest
* @return A Java Future containing the result of the ListPricingRulesAssociatedToPricingPlan operation returned by
* the service.
* @sample AWSBillingConductorAsync.ListPricingRulesAssociatedToPricingPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future listPricingRulesAssociatedToPricingPlanAsync(
ListPricingRulesAssociatedToPricingPlanRequest listPricingRulesAssociatedToPricingPlanRequest);
/**
*
* Lists the pricing rules that are associated with a pricing plan.
*
*
* @param listPricingRulesAssociatedToPricingPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListPricingRulesAssociatedToPricingPlan operation returned by
* the service.
* @sample AWSBillingConductorAsyncHandler.ListPricingRulesAssociatedToPricingPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future listPricingRulesAssociatedToPricingPlanAsync(
ListPricingRulesAssociatedToPricingPlanRequest listPricingRulesAssociatedToPricingPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List the resources that are associated to a custom line item.
*
*
* @param listResourcesAssociatedToCustomLineItemRequest
* @return A Java Future containing the result of the ListResourcesAssociatedToCustomLineItem operation returned by
* the service.
* @sample AWSBillingConductorAsync.ListResourcesAssociatedToCustomLineItem
* @see AWS API Documentation
*/
java.util.concurrent.Future listResourcesAssociatedToCustomLineItemAsync(
ListResourcesAssociatedToCustomLineItemRequest listResourcesAssociatedToCustomLineItemRequest);
/**
*
* List the resources that are associated to a custom line item.
*
*
* @param listResourcesAssociatedToCustomLineItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListResourcesAssociatedToCustomLineItem operation returned by
* the service.
* @sample AWSBillingConductorAsyncHandler.ListResourcesAssociatedToCustomLineItem
* @see AWS API Documentation
*/
java.util.concurrent.Future listResourcesAssociatedToCustomLineItemAsync(
ListResourcesAssociatedToCustomLineItemRequest listResourcesAssociatedToCustomLineItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A list the tags for a resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSBillingConductorAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* A list the tags for a resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified tags to a resource with the specified resourceArn
. If existing tags on a
* resource are not specified in the request parameters, they are not changed.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSBillingConductorAsync.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Associates the specified tags to a resource with the specified resourceArn
. If existing tags on a
* resource are not specified in the request parameters, they are not changed.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes specified tags from a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSBillingConductorAsync.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Deletes specified tags from a resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.UntagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This updates an existing billing group.
*
*
* @param updateBillingGroupRequest
* @return A Java Future containing the result of the UpdateBillingGroup operation returned by the service.
* @sample AWSBillingConductorAsync.UpdateBillingGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future updateBillingGroupAsync(UpdateBillingGroupRequest updateBillingGroupRequest);
/**
*
* This updates an existing billing group.
*
*
* @param updateBillingGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateBillingGroup operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.UpdateBillingGroup
* @see AWS API Documentation
*/
java.util.concurrent.Future updateBillingGroupAsync(UpdateBillingGroupRequest updateBillingGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Update an existing custom line item in the current or previous billing period.
*
*
* @param updateCustomLineItemRequest
* @return A Java Future containing the result of the UpdateCustomLineItem operation returned by the service.
* @sample AWSBillingConductorAsync.UpdateCustomLineItem
* @see AWS API Documentation
*/
java.util.concurrent.Future updateCustomLineItemAsync(UpdateCustomLineItemRequest updateCustomLineItemRequest);
/**
*
* Update an existing custom line item in the current or previous billing period.
*
*
* @param updateCustomLineItemRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateCustomLineItem operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.UpdateCustomLineItem
* @see AWS API Documentation
*/
java.util.concurrent.Future updateCustomLineItemAsync(UpdateCustomLineItemRequest updateCustomLineItemRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* This updates an existing pricing plan.
*
*
* @param updatePricingPlanRequest
* @return A Java Future containing the result of the UpdatePricingPlan operation returned by the service.
* @sample AWSBillingConductorAsync.UpdatePricingPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future updatePricingPlanAsync(UpdatePricingPlanRequest updatePricingPlanRequest);
/**
*
* This updates an existing pricing plan.
*
*
* @param updatePricingPlanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdatePricingPlan operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.UpdatePricingPlan
* @see AWS API Documentation
*/
java.util.concurrent.Future updatePricingPlanAsync(UpdatePricingPlanRequest updatePricingPlanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an existing pricing rule.
*
*
* @param updatePricingRuleRequest
* @return A Java Future containing the result of the UpdatePricingRule operation returned by the service.
* @sample AWSBillingConductorAsync.UpdatePricingRule
* @see AWS API Documentation
*/
java.util.concurrent.Future updatePricingRuleAsync(UpdatePricingRuleRequest updatePricingRuleRequest);
/**
*
* Updates an existing pricing rule.
*
*
* @param updatePricingRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdatePricingRule operation returned by the service.
* @sample AWSBillingConductorAsyncHandler.UpdatePricingRule
* @see AWS API Documentation
*/
java.util.concurrent.Future updatePricingRuleAsync(UpdatePricingRuleRequest updatePricingRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}