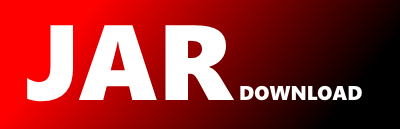
com.amazonaws.services.braket.AWSBraketAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-braket Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.braket;
import javax.annotation.Generated;
import com.amazonaws.services.braket.model.*;
/**
* Interface for accessing Braket asynchronously. Each asynchronous method will return a Java Future object representing
* the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive notification when
* an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.braket.AbstractAWSBraketAsync} instead.
*
*
*
* The Amazon Braket API Reference provides information about the operations and structures supported in Amazon Braket.
*
*
* Additional Resources:
*
*
* -
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSBraketAsync extends AWSBraket {
/**
*
* Cancels an Amazon Braket job.
*
*
* @param cancelJobRequest
* @return A Java Future containing the result of the CancelJob operation returned by the service.
* @sample AWSBraketAsync.CancelJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelJobAsync(CancelJobRequest cancelJobRequest);
/**
*
* Cancels an Amazon Braket job.
*
*
* @param cancelJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelJob operation returned by the service.
* @sample AWSBraketAsyncHandler.CancelJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelJobAsync(CancelJobRequest cancelJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Cancels the specified task.
*
*
* @param cancelQuantumTaskRequest
* @return A Java Future containing the result of the CancelQuantumTask operation returned by the service.
* @sample AWSBraketAsync.CancelQuantumTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelQuantumTaskAsync(CancelQuantumTaskRequest cancelQuantumTaskRequest);
/**
*
* Cancels the specified task.
*
*
* @param cancelQuantumTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelQuantumTask operation returned by the service.
* @sample AWSBraketAsyncHandler.CancelQuantumTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future cancelQuantumTaskAsync(CancelQuantumTaskRequest cancelQuantumTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Braket job.
*
*
* @param createJobRequest
* @return A Java Future containing the result of the CreateJob operation returned by the service.
* @sample AWSBraketAsync.CreateJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createJobAsync(CreateJobRequest createJobRequest);
/**
*
* Creates an Amazon Braket job.
*
*
* @param createJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateJob operation returned by the service.
* @sample AWSBraketAsyncHandler.CreateJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createJobAsync(CreateJobRequest createJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a quantum task.
*
*
* @param createQuantumTaskRequest
* @return A Java Future containing the result of the CreateQuantumTask operation returned by the service.
* @sample AWSBraketAsync.CreateQuantumTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createQuantumTaskAsync(CreateQuantumTaskRequest createQuantumTaskRequest);
/**
*
* Creates a quantum task.
*
*
* @param createQuantumTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateQuantumTask operation returned by the service.
* @sample AWSBraketAsyncHandler.CreateQuantumTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createQuantumTaskAsync(CreateQuantumTaskRequest createQuantumTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the devices available in Amazon Braket.
*
*
*
* For backwards compatibility with older versions of BraketSchemas, OpenQASM information is omitted from GetDevice
* API calls. To get this information the user-agent needs to present a recent version of the BraketSchemas (1.8.0
* or later). The Braket SDK automatically reports this for you. If you do not see OpenQASM results in the GetDevice
* response when using a Braket SDK, you may need to set AWS_EXECUTION_ENV environment variable to configure
* user-agent. See the code examples provided below for how to do this for the AWS CLI, Boto3, and the Go, Java, and
* JavaScript/TypeScript SDKs.
*
*
*
* @param getDeviceRequest
* @return A Java Future containing the result of the GetDevice operation returned by the service.
* @sample AWSBraketAsync.GetDevice
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDeviceAsync(GetDeviceRequest getDeviceRequest);
/**
*
* Retrieves the devices available in Amazon Braket.
*
*
*
* For backwards compatibility with older versions of BraketSchemas, OpenQASM information is omitted from GetDevice
* API calls. To get this information the user-agent needs to present a recent version of the BraketSchemas (1.8.0
* or later). The Braket SDK automatically reports this for you. If you do not see OpenQASM results in the GetDevice
* response when using a Braket SDK, you may need to set AWS_EXECUTION_ENV environment variable to configure
* user-agent. See the code examples provided below for how to do this for the AWS CLI, Boto3, and the Go, Java, and
* JavaScript/TypeScript SDKs.
*
*
*
* @param getDeviceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetDevice operation returned by the service.
* @sample AWSBraketAsyncHandler.GetDevice
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getDeviceAsync(GetDeviceRequest getDeviceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified Amazon Braket job.
*
*
* @param getJobRequest
* @return A Java Future containing the result of the GetJob operation returned by the service.
* @sample AWSBraketAsync.GetJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getJobAsync(GetJobRequest getJobRequest);
/**
*
* Retrieves the specified Amazon Braket job.
*
*
* @param getJobRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetJob operation returned by the service.
* @sample AWSBraketAsyncHandler.GetJob
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getJobAsync(GetJobRequest getJobRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the specified quantum task.
*
*
* @param getQuantumTaskRequest
* @return A Java Future containing the result of the GetQuantumTask operation returned by the service.
* @sample AWSBraketAsync.GetQuantumTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getQuantumTaskAsync(GetQuantumTaskRequest getQuantumTaskRequest);
/**
*
* Retrieves the specified quantum task.
*
*
* @param getQuantumTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetQuantumTask operation returned by the service.
* @sample AWSBraketAsyncHandler.GetQuantumTask
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getQuantumTaskAsync(GetQuantumTaskRequest getQuantumTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Shows the tags associated with this resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSBraketAsync.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Shows the tags associated with this resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSBraketAsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches for devices using the specified filters.
*
*
* @param searchDevicesRequest
* @return A Java Future containing the result of the SearchDevices operation returned by the service.
* @sample AWSBraketAsync.SearchDevices
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchDevicesAsync(SearchDevicesRequest searchDevicesRequest);
/**
*
* Searches for devices using the specified filters.
*
*
* @param searchDevicesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchDevices operation returned by the service.
* @sample AWSBraketAsyncHandler.SearchDevices
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchDevicesAsync(SearchDevicesRequest searchDevicesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches for Amazon Braket jobs that match the specified filter values.
*
*
* @param searchJobsRequest
* @return A Java Future containing the result of the SearchJobs operation returned by the service.
* @sample AWSBraketAsync.SearchJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchJobsAsync(SearchJobsRequest searchJobsRequest);
/**
*
* Searches for Amazon Braket jobs that match the specified filter values.
*
*
* @param searchJobsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchJobs operation returned by the service.
* @sample AWSBraketAsyncHandler.SearchJobs
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchJobsAsync(SearchJobsRequest searchJobsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Searches for tasks that match the specified filter values.
*
*
* @param searchQuantumTasksRequest
* @return A Java Future containing the result of the SearchQuantumTasks operation returned by the service.
* @sample AWSBraketAsync.SearchQuantumTasks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchQuantumTasksAsync(SearchQuantumTasksRequest searchQuantumTasksRequest);
/**
*
* Searches for tasks that match the specified filter values.
*
*
* @param searchQuantumTasksRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SearchQuantumTasks operation returned by the service.
* @sample AWSBraketAsyncHandler.SearchQuantumTasks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future searchQuantumTasksAsync(SearchQuantumTasksRequest searchQuantumTasksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Add a tag to the specified resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSBraketAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Add a tag to the specified resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSBraketAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Remove tags from a resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSBraketAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Remove tags from a resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSBraketAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}