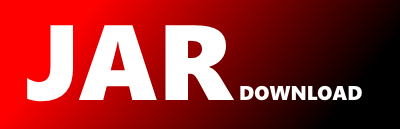
com.amazonaws.services.chatbot.AWSChatbotAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chatbot Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chatbot;
import javax.annotation.Generated;
import com.amazonaws.services.chatbot.model.*;
/**
* Interface for accessing AWS Chatbot asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.chatbot.AbstractAWSChatbotAsync} instead.
*
*
* AWS Chatbot API
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSChatbotAsync extends AWSChatbot {
/**
* Creates Chime Webhook Configuration
*
* @param createChimeWebhookConfigurationRequest
* @return A Java Future containing the result of the CreateChimeWebhookConfiguration operation returned by the
* service.
* @sample AWSChatbotAsync.CreateChimeWebhookConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createChimeWebhookConfigurationAsync(
CreateChimeWebhookConfigurationRequest createChimeWebhookConfigurationRequest);
/**
* Creates Chime Webhook Configuration
*
* @param createChimeWebhookConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateChimeWebhookConfiguration operation returned by the
* service.
* @sample AWSChatbotAsyncHandler.CreateChimeWebhookConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createChimeWebhookConfigurationAsync(
CreateChimeWebhookConfigurationRequest createChimeWebhookConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Creates MS Teams Channel Configuration
*
* @param createMicrosoftTeamsChannelConfigurationRequest
* @return A Java Future containing the result of the CreateMicrosoftTeamsChannelConfiguration operation returned by
* the service.
* @sample AWSChatbotAsync.CreateMicrosoftTeamsChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createMicrosoftTeamsChannelConfigurationAsync(
CreateMicrosoftTeamsChannelConfigurationRequest createMicrosoftTeamsChannelConfigurationRequest);
/**
* Creates MS Teams Channel Configuration
*
* @param createMicrosoftTeamsChannelConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMicrosoftTeamsChannelConfiguration operation returned by
* the service.
* @sample AWSChatbotAsyncHandler.CreateMicrosoftTeamsChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createMicrosoftTeamsChannelConfigurationAsync(
CreateMicrosoftTeamsChannelConfigurationRequest createMicrosoftTeamsChannelConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Creates Slack Channel Configuration
*
* @param createSlackChannelConfigurationRequest
* @return A Java Future containing the result of the CreateSlackChannelConfiguration operation returned by the
* service.
* @sample AWSChatbotAsync.CreateSlackChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createSlackChannelConfigurationAsync(
CreateSlackChannelConfigurationRequest createSlackChannelConfigurationRequest);
/**
* Creates Slack Channel Configuration
*
* @param createSlackChannelConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSlackChannelConfiguration operation returned by the
* service.
* @sample AWSChatbotAsyncHandler.CreateSlackChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createSlackChannelConfigurationAsync(
CreateSlackChannelConfigurationRequest createSlackChannelConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes a Chime Webhook Configuration
*
* @param deleteChimeWebhookConfigurationRequest
* @return A Java Future containing the result of the DeleteChimeWebhookConfiguration operation returned by the
* service.
* @sample AWSChatbotAsync.DeleteChimeWebhookConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteChimeWebhookConfigurationAsync(
DeleteChimeWebhookConfigurationRequest deleteChimeWebhookConfigurationRequest);
/**
* Deletes a Chime Webhook Configuration
*
* @param deleteChimeWebhookConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteChimeWebhookConfiguration operation returned by the
* service.
* @sample AWSChatbotAsyncHandler.DeleteChimeWebhookConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteChimeWebhookConfigurationAsync(
DeleteChimeWebhookConfigurationRequest deleteChimeWebhookConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes MS Teams Channel Configuration
*
* @param deleteMicrosoftTeamsChannelConfigurationRequest
* @return A Java Future containing the result of the DeleteMicrosoftTeamsChannelConfiguration operation returned by
* the service.
* @sample AWSChatbotAsync.DeleteMicrosoftTeamsChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMicrosoftTeamsChannelConfigurationAsync(
DeleteMicrosoftTeamsChannelConfigurationRequest deleteMicrosoftTeamsChannelConfigurationRequest);
/**
* Deletes MS Teams Channel Configuration
*
* @param deleteMicrosoftTeamsChannelConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMicrosoftTeamsChannelConfiguration operation returned by
* the service.
* @sample AWSChatbotAsyncHandler.DeleteMicrosoftTeamsChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMicrosoftTeamsChannelConfigurationAsync(
DeleteMicrosoftTeamsChannelConfigurationRequest deleteMicrosoftTeamsChannelConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes the Microsoft Teams team authorization allowing for channels to be configured in that Microsoft Teams
* team. Note that the Microsoft Teams team must have no channels configured to remove it.
*
* @param deleteMicrosoftTeamsConfiguredTeamRequest
* @return A Java Future containing the result of the DeleteMicrosoftTeamsConfiguredTeam operation returned by the
* service.
* @sample AWSChatbotAsync.DeleteMicrosoftTeamsConfiguredTeam
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMicrosoftTeamsConfiguredTeamAsync(
DeleteMicrosoftTeamsConfiguredTeamRequest deleteMicrosoftTeamsConfiguredTeamRequest);
/**
* Deletes the Microsoft Teams team authorization allowing for channels to be configured in that Microsoft Teams
* team. Note that the Microsoft Teams team must have no channels configured to remove it.
*
* @param deleteMicrosoftTeamsConfiguredTeamRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMicrosoftTeamsConfiguredTeam operation returned by the
* service.
* @sample AWSChatbotAsyncHandler.DeleteMicrosoftTeamsConfiguredTeam
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMicrosoftTeamsConfiguredTeamAsync(
DeleteMicrosoftTeamsConfiguredTeamRequest deleteMicrosoftTeamsConfiguredTeamRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes a Teams user identity
*
* @param deleteMicrosoftTeamsUserIdentityRequest
* @return A Java Future containing the result of the DeleteMicrosoftTeamsUserIdentity operation returned by the
* service.
* @sample AWSChatbotAsync.DeleteMicrosoftTeamsUserIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMicrosoftTeamsUserIdentityAsync(
DeleteMicrosoftTeamsUserIdentityRequest deleteMicrosoftTeamsUserIdentityRequest);
/**
* Deletes a Teams user identity
*
* @param deleteMicrosoftTeamsUserIdentityRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMicrosoftTeamsUserIdentity operation returned by the
* service.
* @sample AWSChatbotAsyncHandler.DeleteMicrosoftTeamsUserIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMicrosoftTeamsUserIdentityAsync(
DeleteMicrosoftTeamsUserIdentityRequest deleteMicrosoftTeamsUserIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes Slack Channel Configuration
*
* @param deleteSlackChannelConfigurationRequest
* @return A Java Future containing the result of the DeleteSlackChannelConfiguration operation returned by the
* service.
* @sample AWSChatbotAsync.DeleteSlackChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSlackChannelConfigurationAsync(
DeleteSlackChannelConfigurationRequest deleteSlackChannelConfigurationRequest);
/**
* Deletes Slack Channel Configuration
*
* @param deleteSlackChannelConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSlackChannelConfiguration operation returned by the
* service.
* @sample AWSChatbotAsyncHandler.DeleteSlackChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSlackChannelConfigurationAsync(
DeleteSlackChannelConfigurationRequest deleteSlackChannelConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes a Slack user identity
*
* @param deleteSlackUserIdentityRequest
* @return A Java Future containing the result of the DeleteSlackUserIdentity operation returned by the service.
* @sample AWSChatbotAsync.DeleteSlackUserIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSlackUserIdentityAsync(DeleteSlackUserIdentityRequest deleteSlackUserIdentityRequest);
/**
* Deletes a Slack user identity
*
* @param deleteSlackUserIdentityRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSlackUserIdentity operation returned by the service.
* @sample AWSChatbotAsyncHandler.DeleteSlackUserIdentity
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSlackUserIdentityAsync(DeleteSlackUserIdentityRequest deleteSlackUserIdentityRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Deletes the Slack workspace authorization that allows channels to be configured in that workspace. This requires
* all configured channels in the workspace to be deleted.
*
* @param deleteSlackWorkspaceAuthorizationRequest
* @return A Java Future containing the result of the DeleteSlackWorkspaceAuthorization operation returned by the
* service.
* @sample AWSChatbotAsync.DeleteSlackWorkspaceAuthorization
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSlackWorkspaceAuthorizationAsync(
DeleteSlackWorkspaceAuthorizationRequest deleteSlackWorkspaceAuthorizationRequest);
/**
* Deletes the Slack workspace authorization that allows channels to be configured in that workspace. This requires
* all configured channels in the workspace to be deleted.
*
* @param deleteSlackWorkspaceAuthorizationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSlackWorkspaceAuthorization operation returned by the
* service.
* @sample AWSChatbotAsyncHandler.DeleteSlackWorkspaceAuthorization
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteSlackWorkspaceAuthorizationAsync(
DeleteSlackWorkspaceAuthorizationRequest deleteSlackWorkspaceAuthorizationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Lists Chime Webhook Configurations optionally filtered by ChatConfigurationArn
*
* @param describeChimeWebhookConfigurationsRequest
* @return A Java Future containing the result of the DescribeChimeWebhookConfigurations operation returned by the
* service.
* @sample AWSChatbotAsync.DescribeChimeWebhookConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeChimeWebhookConfigurationsAsync(
DescribeChimeWebhookConfigurationsRequest describeChimeWebhookConfigurationsRequest);
/**
* Lists Chime Webhook Configurations optionally filtered by ChatConfigurationArn
*
* @param describeChimeWebhookConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeChimeWebhookConfigurations operation returned by the
* service.
* @sample AWSChatbotAsyncHandler.DescribeChimeWebhookConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeChimeWebhookConfigurationsAsync(
DescribeChimeWebhookConfigurationsRequest describeChimeWebhookConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Lists Slack Channel Configurations optionally filtered by ChatConfigurationArn
*
* @param describeSlackChannelConfigurationsRequest
* @return A Java Future containing the result of the DescribeSlackChannelConfigurations operation returned by the
* service.
* @sample AWSChatbotAsync.DescribeSlackChannelConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSlackChannelConfigurationsAsync(
DescribeSlackChannelConfigurationsRequest describeSlackChannelConfigurationsRequest);
/**
* Lists Slack Channel Configurations optionally filtered by ChatConfigurationArn
*
* @param describeSlackChannelConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSlackChannelConfigurations operation returned by the
* service.
* @sample AWSChatbotAsyncHandler.DescribeSlackChannelConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSlackChannelConfigurationsAsync(
DescribeSlackChannelConfigurationsRequest describeSlackChannelConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Lists all Slack user identities with a mapped role.
*
* @param describeSlackUserIdentitiesRequest
* @return A Java Future containing the result of the DescribeSlackUserIdentities operation returned by the service.
* @sample AWSChatbotAsync.DescribeSlackUserIdentities
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSlackUserIdentitiesAsync(
DescribeSlackUserIdentitiesRequest describeSlackUserIdentitiesRequest);
/**
* Lists all Slack user identities with a mapped role.
*
* @param describeSlackUserIdentitiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSlackUserIdentities operation returned by the service.
* @sample AWSChatbotAsyncHandler.DescribeSlackUserIdentities
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSlackUserIdentitiesAsync(
DescribeSlackUserIdentitiesRequest describeSlackUserIdentitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Lists all authorized Slack Workspaces for AWS Account
*
* @param describeSlackWorkspacesRequest
* @return A Java Future containing the result of the DescribeSlackWorkspaces operation returned by the service.
* @sample AWSChatbotAsync.DescribeSlackWorkspaces
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSlackWorkspacesAsync(DescribeSlackWorkspacesRequest describeSlackWorkspacesRequest);
/**
* Lists all authorized Slack Workspaces for AWS Account
*
* @param describeSlackWorkspacesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeSlackWorkspaces operation returned by the service.
* @sample AWSChatbotAsyncHandler.DescribeSlackWorkspaces
* @see AWS API Documentation
*/
java.util.concurrent.Future describeSlackWorkspacesAsync(DescribeSlackWorkspacesRequest describeSlackWorkspacesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Get Chatbot account level preferences
*
* @param getAccountPreferencesRequest
* @return A Java Future containing the result of the GetAccountPreferences operation returned by the service.
* @sample AWSChatbotAsync.GetAccountPreferences
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAccountPreferencesAsync(GetAccountPreferencesRequest getAccountPreferencesRequest);
/**
* Get Chatbot account level preferences
*
* @param getAccountPreferencesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAccountPreferences operation returned by the service.
* @sample AWSChatbotAsyncHandler.GetAccountPreferences
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAccountPreferencesAsync(GetAccountPreferencesRequest getAccountPreferencesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Get a single MS Teams Channel Configurations
*
* @param getMicrosoftTeamsChannelConfigurationRequest
* @return A Java Future containing the result of the GetMicrosoftTeamsChannelConfiguration operation returned by
* the service.
* @sample AWSChatbotAsync.GetMicrosoftTeamsChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getMicrosoftTeamsChannelConfigurationAsync(
GetMicrosoftTeamsChannelConfigurationRequest getMicrosoftTeamsChannelConfigurationRequest);
/**
* Get a single MS Teams Channel Configurations
*
* @param getMicrosoftTeamsChannelConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMicrosoftTeamsChannelConfiguration operation returned by
* the service.
* @sample AWSChatbotAsyncHandler.GetMicrosoftTeamsChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getMicrosoftTeamsChannelConfigurationAsync(
GetMicrosoftTeamsChannelConfigurationRequest getMicrosoftTeamsChannelConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Lists MS Teams Channel Configurations optionally filtered by TeamId
*
* @param listMicrosoftTeamsChannelConfigurationsRequest
* @return A Java Future containing the result of the ListMicrosoftTeamsChannelConfigurations operation returned by
* the service.
* @sample AWSChatbotAsync.ListMicrosoftTeamsChannelConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listMicrosoftTeamsChannelConfigurationsAsync(
ListMicrosoftTeamsChannelConfigurationsRequest listMicrosoftTeamsChannelConfigurationsRequest);
/**
* Lists MS Teams Channel Configurations optionally filtered by TeamId
*
* @param listMicrosoftTeamsChannelConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMicrosoftTeamsChannelConfigurations operation returned by
* the service.
* @sample AWSChatbotAsyncHandler.ListMicrosoftTeamsChannelConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listMicrosoftTeamsChannelConfigurationsAsync(
ListMicrosoftTeamsChannelConfigurationsRequest listMicrosoftTeamsChannelConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Lists all authorized MS teams for AWS Account
*
* @param listMicrosoftTeamsConfiguredTeamsRequest
* @return A Java Future containing the result of the ListMicrosoftTeamsConfiguredTeams operation returned by the
* service.
* @sample AWSChatbotAsync.ListMicrosoftTeamsConfiguredTeams
* @see AWS API Documentation
*/
java.util.concurrent.Future listMicrosoftTeamsConfiguredTeamsAsync(
ListMicrosoftTeamsConfiguredTeamsRequest listMicrosoftTeamsConfiguredTeamsRequest);
/**
* Lists all authorized MS teams for AWS Account
*
* @param listMicrosoftTeamsConfiguredTeamsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMicrosoftTeamsConfiguredTeams operation returned by the
* service.
* @sample AWSChatbotAsyncHandler.ListMicrosoftTeamsConfiguredTeams
* @see AWS API Documentation
*/
java.util.concurrent.Future listMicrosoftTeamsConfiguredTeamsAsync(
ListMicrosoftTeamsConfiguredTeamsRequest listMicrosoftTeamsConfiguredTeamsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Lists all Microsoft Teams user identities with a mapped role.
*
* @param listMicrosoftTeamsUserIdentitiesRequest
* @return A Java Future containing the result of the ListMicrosoftTeamsUserIdentities operation returned by the
* service.
* @sample AWSChatbotAsync.ListMicrosoftTeamsUserIdentities
* @see AWS API Documentation
*/
java.util.concurrent.Future listMicrosoftTeamsUserIdentitiesAsync(
ListMicrosoftTeamsUserIdentitiesRequest listMicrosoftTeamsUserIdentitiesRequest);
/**
* Lists all Microsoft Teams user identities with a mapped role.
*
* @param listMicrosoftTeamsUserIdentitiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMicrosoftTeamsUserIdentities operation returned by the
* service.
* @sample AWSChatbotAsyncHandler.ListMicrosoftTeamsUserIdentities
* @see AWS API Documentation
*/
java.util.concurrent.Future listMicrosoftTeamsUserIdentitiesAsync(
ListMicrosoftTeamsUserIdentitiesRequest listMicrosoftTeamsUserIdentitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Retrieves the list of tags applied to a configuration.
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSChatbotAsync.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
* Retrieves the list of tags applied to a configuration.
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSChatbotAsyncHandler.ListTagsForResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Applies the supplied tags to a configuration.
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSChatbotAsync.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
* Applies the supplied tags to a configuration.
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSChatbotAsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Removes the supplied tags from a configuration
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSChatbotAsync.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
* Removes the supplied tags from a configuration
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSChatbotAsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Update Chatbot account level preferences
*
* @param updateAccountPreferencesRequest
* @return A Java Future containing the result of the UpdateAccountPreferences operation returned by the service.
* @sample AWSChatbotAsync.UpdateAccountPreferences
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAccountPreferencesAsync(UpdateAccountPreferencesRequest updateAccountPreferencesRequest);
/**
* Update Chatbot account level preferences
*
* @param updateAccountPreferencesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAccountPreferences operation returned by the service.
* @sample AWSChatbotAsyncHandler.UpdateAccountPreferences
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAccountPreferencesAsync(UpdateAccountPreferencesRequest updateAccountPreferencesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates a Chime Webhook Configuration
*
* @param updateChimeWebhookConfigurationRequest
* @return A Java Future containing the result of the UpdateChimeWebhookConfiguration operation returned by the
* service.
* @sample AWSChatbotAsync.UpdateChimeWebhookConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateChimeWebhookConfigurationAsync(
UpdateChimeWebhookConfigurationRequest updateChimeWebhookConfigurationRequest);
/**
* Updates a Chime Webhook Configuration
*
* @param updateChimeWebhookConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateChimeWebhookConfiguration operation returned by the
* service.
* @sample AWSChatbotAsyncHandler.UpdateChimeWebhookConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateChimeWebhookConfigurationAsync(
UpdateChimeWebhookConfigurationRequest updateChimeWebhookConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates MS Teams Channel Configuration
*
* @param updateMicrosoftTeamsChannelConfigurationRequest
* @return A Java Future containing the result of the UpdateMicrosoftTeamsChannelConfiguration operation returned by
* the service.
* @sample AWSChatbotAsync.UpdateMicrosoftTeamsChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateMicrosoftTeamsChannelConfigurationAsync(
UpdateMicrosoftTeamsChannelConfigurationRequest updateMicrosoftTeamsChannelConfigurationRequest);
/**
* Updates MS Teams Channel Configuration
*
* @param updateMicrosoftTeamsChannelConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateMicrosoftTeamsChannelConfiguration operation returned by
* the service.
* @sample AWSChatbotAsyncHandler.UpdateMicrosoftTeamsChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateMicrosoftTeamsChannelConfigurationAsync(
UpdateMicrosoftTeamsChannelConfigurationRequest updateMicrosoftTeamsChannelConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Updates Slack Channel Configuration
*
* @param updateSlackChannelConfigurationRequest
* @return A Java Future containing the result of the UpdateSlackChannelConfiguration operation returned by the
* service.
* @sample AWSChatbotAsync.UpdateSlackChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSlackChannelConfigurationAsync(
UpdateSlackChannelConfigurationRequest updateSlackChannelConfigurationRequest);
/**
* Updates Slack Channel Configuration
*
* @param updateSlackChannelConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateSlackChannelConfiguration operation returned by the
* service.
* @sample AWSChatbotAsyncHandler.UpdateSlackChannelConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateSlackChannelConfigurationAsync(
UpdateSlackChannelConfigurationRequest updateSlackChannelConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}