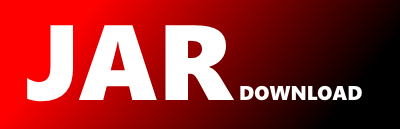
com.amazonaws.services.chime.AmazonChime Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chime Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chime;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.chime.model.*;
/**
* Interface for accessing Amazon Chime.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.chime.AbstractAmazonChime} instead.
*
*
*
* The Amazon Chime API (application programming interface) is designed for administrators to use to perform key tasks,
* such as creating and managing Amazon Chime accounts and users. This guide provides detailed information about the
* Amazon Chime API, including operations, types, inputs and outputs, and error codes.
*
*
* You can use an AWS SDK, the AWS Command Line Interface (AWS CLI), or the REST API to make API calls. We recommend
* using an AWS SDK or the AWS CLI. Each API operation includes links to information about using it with a
* language-specific AWS SDK or the AWS CLI.
*
*
* - Using an AWS SDK
* -
*
* You don't need to write code to calculate a signature for request authentication. The SDK clients authenticate your
* requests by using access keys that you provide. For more information about AWS SDKs, see the AWS Developer Center.
*
*
* - Using the AWS CLI
* -
*
* Use your access keys with the AWS CLI to make API calls. For information about setting up the AWS CLI, see Installing the AWS Command Line Interface
* in the AWS Command Line Interface User Guide. For a list of available Amazon Chime commands, see the Amazon Chime commands in the AWS CLI
* Command Reference.
*
*
* - Using REST API
* -
*
* If you use REST to make API calls, you must authenticate your request by providing a signature. Amazon Chime supports
* signature version 4. For more information, see Signature Version 4 Signing Process
* in the Amazon Web Services General Reference.
*
*
* When making REST API calls, use the service name chime
and REST endpoint
* https://service.chime.aws.amazon.com
.
*
*
*
*
* Administrative permissions are controlled using AWS Identity and Access Management (IAM). For more information, see
* Control Access to the Amazon Chime
* Console in the Amazon Chime Administration Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonChime {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "chime";
/**
*
* Suspends up to 50 users from a Team
or EnterpriseLWA
Amazon Chime account. For more
* information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* Users suspended from a Team
account are dissociated from the account, but they can continue to use
* Amazon Chime as free users. To remove the suspension from suspended Team
account users, invite them
* to the Team
account again. You can use the InviteUsers action to do so.
*
*
* Users suspended from an EnterpriseLWA
account are immediately signed out of Amazon Chime and are no
* longer able to sign in. To remove the suspension from suspended EnterpriseLWA
account users, use the
* BatchUnsuspendUser action.
*
*
* To sign out users without suspending them, use the LogoutUser action.
*
*
* @param batchSuspendUserRequest
* @return Result of the BatchSuspendUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchSuspendUser
* @see AWS API
* Documentation
*/
BatchSuspendUserResult batchSuspendUser(BatchSuspendUserRequest batchSuspendUserRequest);
/**
*
* Removes the suspension from up to 50 previously suspended users for the specified Amazon Chime
* EnterpriseLWA
account. Only users on EnterpriseLWA
accounts can be unsuspended using
* this action. For more information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* Previously suspended users who are unsuspended using this action are returned to Registered
status.
* Users who are not previously suspended are ignored.
*
*
* @param batchUnsuspendUserRequest
* @return Result of the BatchUnsuspendUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchUnsuspendUser
* @see AWS API
* Documentation
*/
BatchUnsuspendUserResult batchUnsuspendUser(BatchUnsuspendUserRequest batchUnsuspendUserRequest);
/**
*
* Updates user details within the UpdateUserRequestItem object for up to 20 users for the specified Amazon
* Chime account. Currently, only LicenseType
updates are supported for this action.
*
*
* @param batchUpdateUserRequest
* @return Result of the BatchUpdateUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchUpdateUser
* @see AWS API
* Documentation
*/
BatchUpdateUserResult batchUpdateUser(BatchUpdateUserRequest batchUpdateUserRequest);
/**
*
* Creates an Amazon Chime account under the administrator's AWS account. Only Team
account types are
* currently supported for this action. For more information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* @param createAccountRequest
* @return Result of the CreateAccount operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateAccount
* @see AWS API
* Documentation
*/
CreateAccountResult createAccount(CreateAccountRequest createAccountRequest);
/**
*
* Deletes the specified Amazon Chime account. You must suspend all users before deleting a Team
* account. You can use the BatchSuspendUser action to do so.
*
*
* For EnterpriseLWA
and EnterpriseAD
accounts, you must release the claimed domains for
* your Amazon Chime account before deletion. As soon as you release the domain, all users under that account are
* suspended.
*
*
* Deleted accounts appear in your Disabled
accounts list for 90 days. To restore a deleted account
* from your Disabled
accounts list, you must contact AWS Support.
*
*
* After 90 days, deleted accounts are permanently removed from your Disabled
accounts list.
*
*
* @param deleteAccountRequest
* @return Result of the DeleteAccount operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnprocessableEntityException
* The request was well-formed but was unable to be followed due to semantic errors.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteAccount
* @see AWS API
* Documentation
*/
DeleteAccountResult deleteAccount(DeleteAccountRequest deleteAccountRequest);
/**
*
* Retrieves details for the specified Amazon Chime account, such as account type and supported licenses.
*
*
* @param getAccountRequest
* @return Result of the GetAccount operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetAccount
* @see AWS API
* Documentation
*/
GetAccountResult getAccount(GetAccountRequest getAccountRequest);
/**
*
* Retrieves account settings for the specified Amazon Chime account ID, such as remote control and dial out
* settings. For more information about these settings, see Use the Policies Page in the Amazon Chime
* Administration Guide.
*
*
* @param getAccountSettingsRequest
* @return Result of the GetAccountSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetAccountSettings
* @see AWS API
* Documentation
*/
GetAccountSettingsResult getAccountSettings(GetAccountSettingsRequest getAccountSettingsRequest);
/**
*
* Retrieves details for the specified user ID, such as primary email address, license type, and personal meeting
* PIN.
*
*
* To retrieve user details with an email address instead of a user ID, use the ListUsers action, and then
* filter by email address.
*
*
* @param getUserRequest
* @return Result of the GetUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetUser
* @see AWS API
* Documentation
*/
GetUserResult getUser(GetUserRequest getUserRequest);
/**
*
* Sends email invites to as many as 50 users, inviting them to the specified Amazon Chime Team
* account. Only Team
account types are currently supported for this action.
*
*
* @param inviteUsersRequest
* @return Result of the InviteUsers operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.InviteUsers
* @see AWS API
* Documentation
*/
InviteUsersResult inviteUsers(InviteUsersRequest inviteUsersRequest);
/**
*
* Lists the Amazon Chime accounts under the administrator's AWS account. You can filter accounts by account name
* prefix. To find out which Amazon Chime account a user belongs to, you can filter by the user's email address,
* which returns one account result.
*
*
* @param listAccountsRequest
* @return Result of the ListAccounts operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListAccounts
* @see AWS API
* Documentation
*/
ListAccountsResult listAccounts(ListAccountsRequest listAccountsRequest);
/**
*
* Lists the users that belong to the specified Amazon Chime account. You can specify an email address to list only
* the user that the email address belongs to.
*
*
* @param listUsersRequest
* @return Result of the ListUsers operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListUsers
* @see AWS API
* Documentation
*/
ListUsersResult listUsers(ListUsersRequest listUsersRequest);
/**
*
* Logs out the specified user from all of the devices they are currently logged into.
*
*
* @param logoutUserRequest
* @return Result of the LogoutUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.LogoutUser
* @see AWS API
* Documentation
*/
LogoutUserResult logoutUser(LogoutUserRequest logoutUserRequest);
/**
*
* Resets the personal meeting PIN for the specified user on an Amazon Chime account. Returns the User object
* with the updated personal meeting PIN.
*
*
* @param resetPersonalPINRequest
* @return Result of the ResetPersonalPIN operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ResetPersonalPIN
* @see AWS API
* Documentation
*/
ResetPersonalPINResult resetPersonalPIN(ResetPersonalPINRequest resetPersonalPINRequest);
/**
*
* Updates account details for the specified Amazon Chime account. Currently, only account name updates are
* supported for this action.
*
*
* @param updateAccountRequest
* @return Result of the UpdateAccount operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateAccount
* @see AWS API
* Documentation
*/
UpdateAccountResult updateAccount(UpdateAccountRequest updateAccountRequest);
/**
*
* Updates the settings for the specified Amazon Chime account. You can update settings for remote control of shared
* screens, or for the dial-out option. For more information about these settings, see Use the Policies Page in the Amazon Chime
* Administration Guide.
*
*
* @param updateAccountSettingsRequest
* @return Result of the UpdateAccountSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateAccountSettings
* @see AWS
* API Documentation
*/
UpdateAccountSettingsResult updateAccountSettings(UpdateAccountSettingsRequest updateAccountSettingsRequest);
/**
*
* Updates user details for a specified user ID. Currently, only LicenseType
updates are supported for
* this action.
*
*
* @param updateUserRequest
* @return Result of the UpdateUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request. For example, when a user tries to create an
* account from an unsupported region.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateUser
* @see AWS API
* Documentation
*/
UpdateUserResult updateUser(UpdateUserRequest updateUserRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}