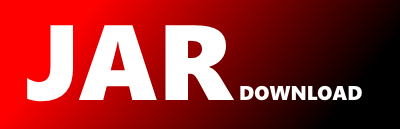
com.amazonaws.services.chime.AmazonChimeAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chime Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chime;
import javax.annotation.Generated;
import com.amazonaws.services.chime.model.*;
/**
* Interface for accessing Amazon Chime asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.chime.AbstractAmazonChimeAsync} instead.
*
*
*
* The Amazon Chime API (application programming interface) is designed for administrators to use to perform key tasks,
* such as creating and managing Amazon Chime accounts and users. This guide provides detailed information about the
* Amazon Chime API, including operations, types, inputs and outputs, and error codes.
*
*
* You can use an AWS SDK, the AWS Command Line Interface (AWS CLI), or the REST API to make API calls. We recommend
* using an AWS SDK or the AWS CLI. Each API operation includes links to information about using it with a
* language-specific AWS SDK or the AWS CLI.
*
*
* - Using an AWS SDK
* -
*
* You don't need to write code to calculate a signature for request authentication. The SDK clients authenticate your
* requests by using access keys that you provide. For more information about AWS SDKs, see the AWS Developer Center.
*
*
* - Using the AWS CLI
* -
*
* Use your access keys with the AWS CLI to make API calls. For information about setting up the AWS CLI, see Installing the AWS Command Line Interface
* in the AWS Command Line Interface User Guide. For a list of available Amazon Chime commands, see the Amazon Chime commands in the AWS CLI
* Command Reference.
*
*
* - Using REST API
* -
*
* If you use REST to make API calls, you must authenticate your request by providing a signature. Amazon Chime supports
* signature version 4. For more information, see Signature Version 4 Signing Process
* in the Amazon Web Services General Reference.
*
*
* When making REST API calls, use the service name chime
and REST endpoint
* https://service.chime.aws.amazon.com
.
*
*
*
*
* Administrative permissions are controlled using AWS Identity and Access Management (IAM). For more information, see
* Control Access to the Amazon Chime
* Console in the Amazon Chime Administration Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonChimeAsync extends AmazonChime {
/**
*
* Suspends up to 50 users from a Team
or EnterpriseLWA
Amazon Chime account. For more
* information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* Users suspended from a Team
account are dissociated from the account, but they can continue to use
* Amazon Chime as free users. To remove the suspension from suspended Team
account users, invite them
* to the Team
account again. You can use the InviteUsers action to do so.
*
*
* Users suspended from an EnterpriseLWA
account are immediately signed out of Amazon Chime and are no
* longer able to sign in. To remove the suspension from suspended EnterpriseLWA
account users, use the
* BatchUnsuspendUser action.
*
*
* To sign out users without suspending them, use the LogoutUser action.
*
*
* @param batchSuspendUserRequest
* @return A Java Future containing the result of the BatchSuspendUser operation returned by the service.
* @sample AmazonChimeAsync.BatchSuspendUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchSuspendUserAsync(BatchSuspendUserRequest batchSuspendUserRequest);
/**
*
* Suspends up to 50 users from a Team
or EnterpriseLWA
Amazon Chime account. For more
* information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* Users suspended from a Team
account are dissociated from the account, but they can continue to use
* Amazon Chime as free users. To remove the suspension from suspended Team
account users, invite them
* to the Team
account again. You can use the InviteUsers action to do so.
*
*
* Users suspended from an EnterpriseLWA
account are immediately signed out of Amazon Chime and are no
* longer able to sign in. To remove the suspension from suspended EnterpriseLWA
account users, use the
* BatchUnsuspendUser action.
*
*
* To sign out users without suspending them, use the LogoutUser action.
*
*
* @param batchSuspendUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchSuspendUser operation returned by the service.
* @sample AmazonChimeAsyncHandler.BatchSuspendUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchSuspendUserAsync(BatchSuspendUserRequest batchSuspendUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the suspension from up to 50 previously suspended users for the specified Amazon Chime
* EnterpriseLWA
account. Only users on EnterpriseLWA
accounts can be unsuspended using
* this action. For more information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* Previously suspended users who are unsuspended using this action are returned to Registered
status.
* Users who are not previously suspended are ignored.
*
*
* @param batchUnsuspendUserRequest
* @return A Java Future containing the result of the BatchUnsuspendUser operation returned by the service.
* @sample AmazonChimeAsync.BatchUnsuspendUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchUnsuspendUserAsync(BatchUnsuspendUserRequest batchUnsuspendUserRequest);
/**
*
* Removes the suspension from up to 50 previously suspended users for the specified Amazon Chime
* EnterpriseLWA
account. Only users on EnterpriseLWA
accounts can be unsuspended using
* this action. For more information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* Previously suspended users who are unsuspended using this action are returned to Registered
status.
* Users who are not previously suspended are ignored.
*
*
* @param batchUnsuspendUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchUnsuspendUser operation returned by the service.
* @sample AmazonChimeAsyncHandler.BatchUnsuspendUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchUnsuspendUserAsync(BatchUnsuspendUserRequest batchUnsuspendUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates user details within the UpdateUserRequestItem object for up to 20 users for the specified Amazon
* Chime account. Currently, only LicenseType
updates are supported for this action.
*
*
* @param batchUpdateUserRequest
* @return A Java Future containing the result of the BatchUpdateUser operation returned by the service.
* @sample AmazonChimeAsync.BatchUpdateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchUpdateUserAsync(BatchUpdateUserRequest batchUpdateUserRequest);
/**
*
* Updates user details within the UpdateUserRequestItem object for up to 20 users for the specified Amazon
* Chime account. Currently, only LicenseType
updates are supported for this action.
*
*
* @param batchUpdateUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchUpdateUser operation returned by the service.
* @sample AmazonChimeAsyncHandler.BatchUpdateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchUpdateUserAsync(BatchUpdateUserRequest batchUpdateUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Chime account under the administrator's AWS account. Only Team
account types are
* currently supported for this action. For more information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* @param createAccountRequest
* @return A Java Future containing the result of the CreateAccount operation returned by the service.
* @sample AmazonChimeAsync.CreateAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAccountAsync(CreateAccountRequest createAccountRequest);
/**
*
* Creates an Amazon Chime account under the administrator's AWS account. Only Team
account types are
* currently supported for this action. For more information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* @param createAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAccount operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAccountAsync(CreateAccountRequest createAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified Amazon Chime account. You must suspend all users before deleting a Team
* account. You can use the BatchSuspendUser action to do so.
*
*
* For EnterpriseLWA
and EnterpriseAD
accounts, you must release the claimed domains for
* your Amazon Chime account before deletion. As soon as you release the domain, all users under that account are
* suspended.
*
*
* Deleted accounts appear in your Disabled
accounts list for 90 days. To restore a deleted account
* from your Disabled
accounts list, you must contact AWS Support.
*
*
* After 90 days, deleted accounts are permanently removed from your Disabled
accounts list.
*
*
* @param deleteAccountRequest
* @return A Java Future containing the result of the DeleteAccount operation returned by the service.
* @sample AmazonChimeAsync.DeleteAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAccountAsync(DeleteAccountRequest deleteAccountRequest);
/**
*
* Deletes the specified Amazon Chime account. You must suspend all users before deleting a Team
* account. You can use the BatchSuspendUser action to do so.
*
*
* For EnterpriseLWA
and EnterpriseAD
accounts, you must release the claimed domains for
* your Amazon Chime account before deletion. As soon as you release the domain, all users under that account are
* suspended.
*
*
* Deleted accounts appear in your Disabled
accounts list for 90 days. To restore a deleted account
* from your Disabled
accounts list, you must contact AWS Support.
*
*
* After 90 days, deleted accounts are permanently removed from your Disabled
accounts list.
*
*
* @param deleteAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAccount operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAccountAsync(DeleteAccountRequest deleteAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves details for the specified Amazon Chime account, such as account type and supported licenses.
*
*
* @param getAccountRequest
* @return A Java Future containing the result of the GetAccount operation returned by the service.
* @sample AmazonChimeAsync.GetAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAccountAsync(GetAccountRequest getAccountRequest);
/**
*
* Retrieves details for the specified Amazon Chime account, such as account type and supported licenses.
*
*
* @param getAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAccount operation returned by the service.
* @sample AmazonChimeAsyncHandler.GetAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAccountAsync(GetAccountRequest getAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves account settings for the specified Amazon Chime account ID, such as remote control and dial out
* settings. For more information about these settings, see Use the Policies Page in the Amazon Chime
* Administration Guide.
*
*
* @param getAccountSettingsRequest
* @return A Java Future containing the result of the GetAccountSettings operation returned by the service.
* @sample AmazonChimeAsync.GetAccountSettings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAccountSettingsAsync(GetAccountSettingsRequest getAccountSettingsRequest);
/**
*
* Retrieves account settings for the specified Amazon Chime account ID, such as remote control and dial out
* settings. For more information about these settings, see Use the Policies Page in the Amazon Chime
* Administration Guide.
*
*
* @param getAccountSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAccountSettings operation returned by the service.
* @sample AmazonChimeAsyncHandler.GetAccountSettings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAccountSettingsAsync(GetAccountSettingsRequest getAccountSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves details for the specified user ID, such as primary email address, license type, and personal meeting
* PIN.
*
*
* To retrieve user details with an email address instead of a user ID, use the ListUsers action, and then
* filter by email address.
*
*
* @param getUserRequest
* @return A Java Future containing the result of the GetUser operation returned by the service.
* @sample AmazonChimeAsync.GetUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getUserAsync(GetUserRequest getUserRequest);
/**
*
* Retrieves details for the specified user ID, such as primary email address, license type, and personal meeting
* PIN.
*
*
* To retrieve user details with an email address instead of a user ID, use the ListUsers action, and then
* filter by email address.
*
*
* @param getUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetUser operation returned by the service.
* @sample AmazonChimeAsyncHandler.GetUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getUserAsync(GetUserRequest getUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sends email invites to as many as 50 users, inviting them to the specified Amazon Chime Team
* account. Only Team
account types are currently supported for this action.
*
*
* @param inviteUsersRequest
* @return A Java Future containing the result of the InviteUsers operation returned by the service.
* @sample AmazonChimeAsync.InviteUsers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future inviteUsersAsync(InviteUsersRequest inviteUsersRequest);
/**
*
* Sends email invites to as many as 50 users, inviting them to the specified Amazon Chime Team
* account. Only Team
account types are currently supported for this action.
*
*
* @param inviteUsersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the InviteUsers operation returned by the service.
* @sample AmazonChimeAsyncHandler.InviteUsers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future inviteUsersAsync(InviteUsersRequest inviteUsersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the Amazon Chime accounts under the administrator's AWS account. You can filter accounts by account name
* prefix. To find out which Amazon Chime account a user belongs to, you can filter by the user's email address,
* which returns one account result.
*
*
* @param listAccountsRequest
* @return A Java Future containing the result of the ListAccounts operation returned by the service.
* @sample AmazonChimeAsync.ListAccounts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAccountsAsync(ListAccountsRequest listAccountsRequest);
/**
*
* Lists the Amazon Chime accounts under the administrator's AWS account. You can filter accounts by account name
* prefix. To find out which Amazon Chime account a user belongs to, you can filter by the user's email address,
* which returns one account result.
*
*
* @param listAccountsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAccounts operation returned by the service.
* @sample AmazonChimeAsyncHandler.ListAccounts
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listAccountsAsync(ListAccountsRequest listAccountsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the users that belong to the specified Amazon Chime account. You can specify an email address to list only
* the user that the email address belongs to.
*
*
* @param listUsersRequest
* @return A Java Future containing the result of the ListUsers operation returned by the service.
* @sample AmazonChimeAsync.ListUsers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listUsersAsync(ListUsersRequest listUsersRequest);
/**
*
* Lists the users that belong to the specified Amazon Chime account. You can specify an email address to list only
* the user that the email address belongs to.
*
*
* @param listUsersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListUsers operation returned by the service.
* @sample AmazonChimeAsyncHandler.ListUsers
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listUsersAsync(ListUsersRequest listUsersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Logs out the specified user from all of the devices they are currently logged into.
*
*
* @param logoutUserRequest
* @return A Java Future containing the result of the LogoutUser operation returned by the service.
* @sample AmazonChimeAsync.LogoutUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future logoutUserAsync(LogoutUserRequest logoutUserRequest);
/**
*
* Logs out the specified user from all of the devices they are currently logged into.
*
*
* @param logoutUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the LogoutUser operation returned by the service.
* @sample AmazonChimeAsyncHandler.LogoutUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future logoutUserAsync(LogoutUserRequest logoutUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Resets the personal meeting PIN for the specified user on an Amazon Chime account. Returns the User object
* with the updated personal meeting PIN.
*
*
* @param resetPersonalPINRequest
* @return A Java Future containing the result of the ResetPersonalPIN operation returned by the service.
* @sample AmazonChimeAsync.ResetPersonalPIN
* @see AWS API
* Documentation
*/
java.util.concurrent.Future resetPersonalPINAsync(ResetPersonalPINRequest resetPersonalPINRequest);
/**
*
* Resets the personal meeting PIN for the specified user on an Amazon Chime account. Returns the User object
* with the updated personal meeting PIN.
*
*
* @param resetPersonalPINRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ResetPersonalPIN operation returned by the service.
* @sample AmazonChimeAsyncHandler.ResetPersonalPIN
* @see AWS API
* Documentation
*/
java.util.concurrent.Future resetPersonalPINAsync(ResetPersonalPINRequest resetPersonalPINRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates account details for the specified Amazon Chime account. Currently, only account name updates are
* supported for this action.
*
*
* @param updateAccountRequest
* @return A Java Future containing the result of the UpdateAccount operation returned by the service.
* @sample AmazonChimeAsync.UpdateAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAccountAsync(UpdateAccountRequest updateAccountRequest);
/**
*
* Updates account details for the specified Amazon Chime account. Currently, only account name updates are
* supported for this action.
*
*
* @param updateAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAccount operation returned by the service.
* @sample AmazonChimeAsyncHandler.UpdateAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateAccountAsync(UpdateAccountRequest updateAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the settings for the specified Amazon Chime account. You can update settings for remote control of shared
* screens, or for the dial-out option. For more information about these settings, see Use the Policies Page in the Amazon Chime
* Administration Guide.
*
*
* @param updateAccountSettingsRequest
* @return A Java Future containing the result of the UpdateAccountSettings operation returned by the service.
* @sample AmazonChimeAsync.UpdateAccountSettings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateAccountSettingsAsync(UpdateAccountSettingsRequest updateAccountSettingsRequest);
/**
*
* Updates the settings for the specified Amazon Chime account. You can update settings for remote control of shared
* screens, or for the dial-out option. For more information about these settings, see Use the Policies Page in the Amazon Chime
* Administration Guide.
*
*
* @param updateAccountSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAccountSettings operation returned by the service.
* @sample AmazonChimeAsyncHandler.UpdateAccountSettings
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateAccountSettingsAsync(UpdateAccountSettingsRequest updateAccountSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates user details for a specified user ID. Currently, only LicenseType
updates are supported for
* this action.
*
*
* @param updateUserRequest
* @return A Java Future containing the result of the UpdateUser operation returned by the service.
* @sample AmazonChimeAsync.UpdateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateUserAsync(UpdateUserRequest updateUserRequest);
/**
*
* Updates user details for a specified user ID. Currently, only LicenseType
updates are supported for
* this action.
*
*
* @param updateUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateUser operation returned by the service.
* @sample AmazonChimeAsyncHandler.UpdateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateUserAsync(UpdateUserRequest updateUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}