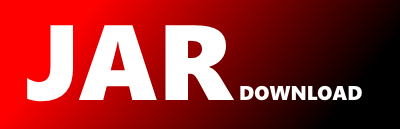
com.amazonaws.services.chime.AmazonChimeAsyncClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chime Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chime;
import javax.annotation.Generated;
import com.amazonaws.services.chime.model.*;
import com.amazonaws.client.AwsAsyncClientParams;
import com.amazonaws.annotation.ThreadSafe;
import java.util.concurrent.ExecutorService;
/**
* Client for accessing Amazon Chime asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
*
* The Amazon Chime API (application programming interface) is designed for administrators to use to perform key tasks,
* such as creating and managing Amazon Chime accounts and users. This guide provides detailed information about the
* Amazon Chime API, including operations, types, inputs and outputs, and error codes.
*
*
* You can use an AWS SDK, the AWS Command Line Interface (AWS CLI), or the REST API to make API calls. We recommend
* using an AWS SDK or the AWS CLI. Each API operation includes links to information about using it with a
* language-specific AWS SDK or the AWS CLI.
*
*
* - Using an AWS SDK
* -
*
* You don't need to write code to calculate a signature for request authentication. The SDK clients authenticate your
* requests by using access keys that you provide. For more information about AWS SDKs, see the AWS Developer Center.
*
*
* - Using the AWS CLI
* -
*
* Use your access keys with the AWS CLI to make API calls. For information about setting up the AWS CLI, see Installing the AWS Command Line Interface
* in the AWS Command Line Interface User Guide. For a list of available Amazon Chime commands, see the Amazon Chime commands in the AWS CLI
* Command Reference.
*
*
* - Using REST API
* -
*
* If you use REST to make API calls, you must authenticate your request by providing a signature. Amazon Chime supports
* signature version 4. For more information, see Signature Version 4 Signing Process
* in the Amazon Web Services General Reference.
*
*
* When making REST API calls, use the service name chime
and REST endpoint
* https://service.chime.aws.amazon.com
.
*
*
*
*
* Administrative permissions are controlled using AWS Identity and Access Management (IAM). For more information, see
* Control Access to the Amazon Chime
* Console in the Amazon Chime Administration Guide.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonChimeAsyncClient extends AmazonChimeClient implements AmazonChimeAsync {
private static final int DEFAULT_THREAD_POOL_SIZE = 50;
private final java.util.concurrent.ExecutorService executorService;
public static AmazonChimeAsyncClientBuilder asyncBuilder() {
return AmazonChimeAsyncClientBuilder.standard();
}
/**
* Constructs a new asynchronous client to invoke service methods on Amazon Chime using the specified parameters.
*
* @param asyncClientParams
* Object providing client parameters.
*/
AmazonChimeAsyncClient(AwsAsyncClientParams asyncClientParams) {
super(asyncClientParams);
this.executorService = asyncClientParams.getExecutor();
}
/**
* Returns the executor service used by this client to execute async requests.
*
* @return The executor service used by this client to execute async requests.
*/
public ExecutorService getExecutorService() {
return executorService;
}
@Override
public java.util.concurrent.Future batchSuspendUserAsync(BatchSuspendUserRequest request) {
return batchSuspendUserAsync(request, null);
}
@Override
public java.util.concurrent.Future batchSuspendUserAsync(final BatchSuspendUserRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final BatchSuspendUserRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public BatchSuspendUserResult call() throws Exception {
BatchSuspendUserResult result = null;
try {
result = executeBatchSuspendUser(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future batchUnsuspendUserAsync(BatchUnsuspendUserRequest request) {
return batchUnsuspendUserAsync(request, null);
}
@Override
public java.util.concurrent.Future batchUnsuspendUserAsync(final BatchUnsuspendUserRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final BatchUnsuspendUserRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public BatchUnsuspendUserResult call() throws Exception {
BatchUnsuspendUserResult result = null;
try {
result = executeBatchUnsuspendUser(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future batchUpdateUserAsync(BatchUpdateUserRequest request) {
return batchUpdateUserAsync(request, null);
}
@Override
public java.util.concurrent.Future batchUpdateUserAsync(final BatchUpdateUserRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final BatchUpdateUserRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public BatchUpdateUserResult call() throws Exception {
BatchUpdateUserResult result = null;
try {
result = executeBatchUpdateUser(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future createAccountAsync(CreateAccountRequest request) {
return createAccountAsync(request, null);
}
@Override
public java.util.concurrent.Future createAccountAsync(final CreateAccountRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final CreateAccountRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public CreateAccountResult call() throws Exception {
CreateAccountResult result = null;
try {
result = executeCreateAccount(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future deleteAccountAsync(DeleteAccountRequest request) {
return deleteAccountAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteAccountAsync(final DeleteAccountRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final DeleteAccountRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public DeleteAccountResult call() throws Exception {
DeleteAccountResult result = null;
try {
result = executeDeleteAccount(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getAccountAsync(GetAccountRequest request) {
return getAccountAsync(request, null);
}
@Override
public java.util.concurrent.Future getAccountAsync(final GetAccountRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final GetAccountRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public GetAccountResult call() throws Exception {
GetAccountResult result = null;
try {
result = executeGetAccount(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getAccountSettingsAsync(GetAccountSettingsRequest request) {
return getAccountSettingsAsync(request, null);
}
@Override
public java.util.concurrent.Future getAccountSettingsAsync(final GetAccountSettingsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final GetAccountSettingsRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public GetAccountSettingsResult call() throws Exception {
GetAccountSettingsResult result = null;
try {
result = executeGetAccountSettings(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getUserAsync(GetUserRequest request) {
return getUserAsync(request, null);
}
@Override
public java.util.concurrent.Future getUserAsync(final GetUserRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final GetUserRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public GetUserResult call() throws Exception {
GetUserResult result = null;
try {
result = executeGetUser(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future inviteUsersAsync(InviteUsersRequest request) {
return inviteUsersAsync(request, null);
}
@Override
public java.util.concurrent.Future inviteUsersAsync(final InviteUsersRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final InviteUsersRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public InviteUsersResult call() throws Exception {
InviteUsersResult result = null;
try {
result = executeInviteUsers(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future listAccountsAsync(ListAccountsRequest request) {
return listAccountsAsync(request, null);
}
@Override
public java.util.concurrent.Future listAccountsAsync(final ListAccountsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final ListAccountsRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public ListAccountsResult call() throws Exception {
ListAccountsResult result = null;
try {
result = executeListAccounts(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future listUsersAsync(ListUsersRequest request) {
return listUsersAsync(request, null);
}
@Override
public java.util.concurrent.Future listUsersAsync(final ListUsersRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final ListUsersRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public ListUsersResult call() throws Exception {
ListUsersResult result = null;
try {
result = executeListUsers(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future logoutUserAsync(LogoutUserRequest request) {
return logoutUserAsync(request, null);
}
@Override
public java.util.concurrent.Future logoutUserAsync(final LogoutUserRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final LogoutUserRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public LogoutUserResult call() throws Exception {
LogoutUserResult result = null;
try {
result = executeLogoutUser(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future resetPersonalPINAsync(ResetPersonalPINRequest request) {
return resetPersonalPINAsync(request, null);
}
@Override
public java.util.concurrent.Future resetPersonalPINAsync(final ResetPersonalPINRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final ResetPersonalPINRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public ResetPersonalPINResult call() throws Exception {
ResetPersonalPINResult result = null;
try {
result = executeResetPersonalPIN(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future updateAccountAsync(UpdateAccountRequest request) {
return updateAccountAsync(request, null);
}
@Override
public java.util.concurrent.Future updateAccountAsync(final UpdateAccountRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final UpdateAccountRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public UpdateAccountResult call() throws Exception {
UpdateAccountResult result = null;
try {
result = executeUpdateAccount(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future updateAccountSettingsAsync(UpdateAccountSettingsRequest request) {
return updateAccountSettingsAsync(request, null);
}
@Override
public java.util.concurrent.Future updateAccountSettingsAsync(final UpdateAccountSettingsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final UpdateAccountSettingsRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public UpdateAccountSettingsResult call() throws Exception {
UpdateAccountSettingsResult result = null;
try {
result = executeUpdateAccountSettings(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future updateUserAsync(UpdateUserRequest request) {
return updateUserAsync(request, null);
}
@Override
public java.util.concurrent.Future updateUserAsync(final UpdateUserRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
final UpdateUserRequest finalRequest = beforeClientExecution(request);
return executorService.submit(new java.util.concurrent.Callable() {
@Override
public UpdateUserResult call() throws Exception {
UpdateUserResult result = null;
try {
result = executeUpdateUser(finalRequest);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(finalRequest, result);
}
return result;
}
});
}
/**
* Shuts down the client, releasing all managed resources. This includes forcibly terminating all pending
* asynchronous service calls. Clients who wish to give pending asynchronous service calls time to complete should
* call {@code getExecutorService().shutdown()} followed by {@code getExecutorService().awaitTermination()} prior to
* calling this method.
*/
@Override
public void shutdown() {
super.shutdown();
executorService.shutdownNow();
}
}