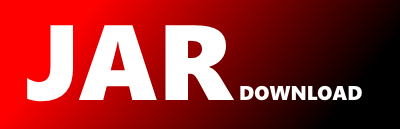
com.amazonaws.services.chime.AmazonChimeAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chime Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chime;
import javax.annotation.Generated;
import com.amazonaws.services.chime.model.*;
/**
* Interface for accessing Amazon Chime asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.chime.AbstractAmazonChimeAsync} instead.
*
*
*
*
* Most of these APIs are no longer supported and will not be updated. We recommend using the latest versions in
* the Amazon Chime SDK API
* reference, in the Amazon Chime SDK.
*
*
* Using the latest versions requires migrating to dedicated namespaces. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* The Amazon Chime application programming interface (API) is designed so administrators can perform key tasks, such as
* creating and managing Amazon Chime accounts, users, and Voice Connectors. This guide provides detailed information
* about the Amazon Chime API, including operations, types, inputs and outputs, and error codes.
*
*
* You can use an AWS SDK, the AWS Command Line Interface (AWS CLI), or the REST API to make API calls for Amazon Chime.
* We recommend using an AWS SDK or the AWS CLI. The page for each API action contains a See Also section that
* includes links to information about using the action with a language-specific AWS SDK or the AWS CLI.
*
*
* - Using an AWS SDK
* -
*
* You don't need to write code to calculate a signature for request authentication. The SDK clients authenticate your
* requests by using access keys that you provide. For more information about AWS SDKs, see the AWS Developer Center.
*
*
* - Using the AWS CLI
* -
*
* Use your access keys with the AWS CLI to make API calls. For information about setting up the AWS CLI, see Installing the AWS Command Line Interface
* in the AWS Command Line Interface User Guide. For a list of available Amazon Chime commands, see the Amazon Chime commands in the AWS CLI
* Command Reference.
*
*
* - Using REST APIs
* -
*
* If you use REST to make API calls, you must authenticate your request by providing a signature. Amazon Chime supports
* Signature Version 4. For more information, see Signature Version 4 Signing Process
* in the Amazon Web Services General Reference.
*
*
* When making REST API calls, use the service name chime
and REST endpoint
* https://service.chime.aws.amazon.com
.
*
*
*
*
* Administrative permissions are controlled using AWS Identity and Access Management (IAM). For more information, see
* Identity and Access Management for Amazon
* Chime in the Amazon Chime Administration Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonChimeAsync extends AmazonChime {
/**
*
* Associates a phone number with the specified Amazon Chime user.
*
*
* @param associatePhoneNumberWithUserRequest
* @return A Java Future containing the result of the AssociatePhoneNumberWithUser operation returned by the
* service.
* @sample AmazonChimeAsync.AssociatePhoneNumberWithUser
* @see AWS API Documentation
*/
java.util.concurrent.Future associatePhoneNumberWithUserAsync(
AssociatePhoneNumberWithUserRequest associatePhoneNumberWithUserRequest);
/**
*
* Associates a phone number with the specified Amazon Chime user.
*
*
* @param associatePhoneNumberWithUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociatePhoneNumberWithUser operation returned by the
* service.
* @sample AmazonChimeAsyncHandler.AssociatePhoneNumberWithUser
* @see AWS API Documentation
*/
java.util.concurrent.Future associatePhoneNumberWithUserAsync(
AssociatePhoneNumberWithUserRequest associatePhoneNumberWithUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates phone numbers with the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, AssociatePhoneNumbersWithVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param associatePhoneNumbersWithVoiceConnectorRequest
* @return A Java Future containing the result of the AssociatePhoneNumbersWithVoiceConnector operation returned by
* the service.
* @sample AmazonChimeAsync.AssociatePhoneNumbersWithVoiceConnector
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future associatePhoneNumbersWithVoiceConnectorAsync(
AssociatePhoneNumbersWithVoiceConnectorRequest associatePhoneNumbersWithVoiceConnectorRequest);
/**
*
* Associates phone numbers with the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, AssociatePhoneNumbersWithVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param associatePhoneNumbersWithVoiceConnectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociatePhoneNumbersWithVoiceConnector operation returned by
* the service.
* @sample AmazonChimeAsyncHandler.AssociatePhoneNumbersWithVoiceConnector
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future associatePhoneNumbersWithVoiceConnectorAsync(
AssociatePhoneNumbersWithVoiceConnectorRequest associatePhoneNumbersWithVoiceConnectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates phone numbers with the specified Amazon Chime Voice Connector group.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, AssociatePhoneNumbersWithVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param associatePhoneNumbersWithVoiceConnectorGroupRequest
* @return A Java Future containing the result of the AssociatePhoneNumbersWithVoiceConnectorGroup operation
* returned by the service.
* @sample AmazonChimeAsync.AssociatePhoneNumbersWithVoiceConnectorGroup
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future associatePhoneNumbersWithVoiceConnectorGroupAsync(
AssociatePhoneNumbersWithVoiceConnectorGroupRequest associatePhoneNumbersWithVoiceConnectorGroupRequest);
/**
*
* Associates phone numbers with the specified Amazon Chime Voice Connector group.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, AssociatePhoneNumbersWithVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param associatePhoneNumbersWithVoiceConnectorGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociatePhoneNumbersWithVoiceConnectorGroup operation
* returned by the service.
* @sample AmazonChimeAsyncHandler.AssociatePhoneNumbersWithVoiceConnectorGroup
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future associatePhoneNumbersWithVoiceConnectorGroupAsync(
AssociatePhoneNumbersWithVoiceConnectorGroupRequest associatePhoneNumbersWithVoiceConnectorGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Associates the specified sign-in delegate groups with the specified Amazon Chime account.
*
*
* @param associateSigninDelegateGroupsWithAccountRequest
* @return A Java Future containing the result of the AssociateSigninDelegateGroupsWithAccount operation returned by
* the service.
* @sample AmazonChimeAsync.AssociateSigninDelegateGroupsWithAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future associateSigninDelegateGroupsWithAccountAsync(
AssociateSigninDelegateGroupsWithAccountRequest associateSigninDelegateGroupsWithAccountRequest);
/**
*
* Associates the specified sign-in delegate groups with the specified Amazon Chime account.
*
*
* @param associateSigninDelegateGroupsWithAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the AssociateSigninDelegateGroupsWithAccount operation returned by
* the service.
* @sample AmazonChimeAsyncHandler.AssociateSigninDelegateGroupsWithAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future associateSigninDelegateGroupsWithAccountAsync(
AssociateSigninDelegateGroupsWithAccountRequest associateSigninDelegateGroupsWithAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates up to 100 new attendees for an active Amazon Chime SDK meeting.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* BatchCreateAttendee, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide.
*
*
* @param batchCreateAttendeeRequest
* @return A Java Future containing the result of the BatchCreateAttendee operation returned by the service.
* @sample AmazonChimeAsync.BatchCreateAttendee
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future batchCreateAttendeeAsync(BatchCreateAttendeeRequest batchCreateAttendeeRequest);
/**
*
* Creates up to 100 new attendees for an active Amazon Chime SDK meeting.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* BatchCreateAttendee, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide.
*
*
* @param batchCreateAttendeeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchCreateAttendee operation returned by the service.
* @sample AmazonChimeAsyncHandler.BatchCreateAttendee
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future batchCreateAttendeeAsync(BatchCreateAttendeeRequest batchCreateAttendeeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a specified number of users to a channel.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, BatchCreateChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param batchCreateChannelMembershipRequest
* @return A Java Future containing the result of the BatchCreateChannelMembership operation returned by the
* service.
* @sample AmazonChimeAsync.BatchCreateChannelMembership
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future batchCreateChannelMembershipAsync(
BatchCreateChannelMembershipRequest batchCreateChannelMembershipRequest);
/**
*
* Adds a specified number of users to a channel.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, BatchCreateChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param batchCreateChannelMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchCreateChannelMembership operation returned by the
* service.
* @sample AmazonChimeAsyncHandler.BatchCreateChannelMembership
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future batchCreateChannelMembershipAsync(
BatchCreateChannelMembershipRequest batchCreateChannelMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds up to 50 members to a chat room in an Amazon Chime Enterprise account. Members can be users or bots. The
* member role designates whether the member is a chat room administrator or a general chat room member.
*
*
* @param batchCreateRoomMembershipRequest
* @return A Java Future containing the result of the BatchCreateRoomMembership operation returned by the service.
* @sample AmazonChimeAsync.BatchCreateRoomMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future batchCreateRoomMembershipAsync(
BatchCreateRoomMembershipRequest batchCreateRoomMembershipRequest);
/**
*
* Adds up to 50 members to a chat room in an Amazon Chime Enterprise account. Members can be users or bots. The
* member role designates whether the member is a chat room administrator or a general chat room member.
*
*
* @param batchCreateRoomMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchCreateRoomMembership operation returned by the service.
* @sample AmazonChimeAsyncHandler.BatchCreateRoomMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future batchCreateRoomMembershipAsync(
BatchCreateRoomMembershipRequest batchCreateRoomMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Moves phone numbers into the Deletion queue. Phone numbers must be disassociated from any users or Amazon
* Chime Voice Connectors before they can be deleted.
*
*
* Phone numbers remain in the Deletion queue for 7 days before they are deleted permanently.
*
*
* @param batchDeletePhoneNumberRequest
* @return A Java Future containing the result of the BatchDeletePhoneNumber operation returned by the service.
* @sample AmazonChimeAsync.BatchDeletePhoneNumber
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchDeletePhoneNumberAsync(BatchDeletePhoneNumberRequest batchDeletePhoneNumberRequest);
/**
*
* Moves phone numbers into the Deletion queue. Phone numbers must be disassociated from any users or Amazon
* Chime Voice Connectors before they can be deleted.
*
*
* Phone numbers remain in the Deletion queue for 7 days before they are deleted permanently.
*
*
* @param batchDeletePhoneNumberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchDeletePhoneNumber operation returned by the service.
* @sample AmazonChimeAsyncHandler.BatchDeletePhoneNumber
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchDeletePhoneNumberAsync(BatchDeletePhoneNumberRequest batchDeletePhoneNumberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Suspends up to 50 users from a Team
or EnterpriseLWA
Amazon Chime account. For more
* information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* Users suspended from a Team
account are disassociated from the account,but they can continue to use
* Amazon Chime as free users. To remove the suspension from suspended Team
account users, invite them
* to the Team
account again. You can use the InviteUsers action to do so.
*
*
* Users suspended from an EnterpriseLWA
account are immediately signed out of Amazon Chime and can no
* longer sign in. To remove the suspension from suspended EnterpriseLWA
account users, use the
* BatchUnsuspendUser action.
*
*
* To sign out users without suspending them, use the LogoutUser action.
*
*
* @param batchSuspendUserRequest
* @return A Java Future containing the result of the BatchSuspendUser operation returned by the service.
* @sample AmazonChimeAsync.BatchSuspendUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchSuspendUserAsync(BatchSuspendUserRequest batchSuspendUserRequest);
/**
*
* Suspends up to 50 users from a Team
or EnterpriseLWA
Amazon Chime account. For more
* information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* Users suspended from a Team
account are disassociated from the account,but they can continue to use
* Amazon Chime as free users. To remove the suspension from suspended Team
account users, invite them
* to the Team
account again. You can use the InviteUsers action to do so.
*
*
* Users suspended from an EnterpriseLWA
account are immediately signed out of Amazon Chime and can no
* longer sign in. To remove the suspension from suspended EnterpriseLWA
account users, use the
* BatchUnsuspendUser action.
*
*
* To sign out users without suspending them, use the LogoutUser action.
*
*
* @param batchSuspendUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchSuspendUser operation returned by the service.
* @sample AmazonChimeAsyncHandler.BatchSuspendUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchSuspendUserAsync(BatchSuspendUserRequest batchSuspendUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the suspension from up to 50 previously suspended users for the specified Amazon Chime
* EnterpriseLWA
account. Only users on EnterpriseLWA
accounts can be unsuspended using
* this action. For more information about different account types, see Managing Your Amazon Chime Accounts
* in the account types, in the Amazon Chime Administration Guide.
*
*
* Previously suspended users who are unsuspended using this action are returned to Registered
status.
* Users who are not previously suspended are ignored.
*
*
* @param batchUnsuspendUserRequest
* @return A Java Future containing the result of the BatchUnsuspendUser operation returned by the service.
* @sample AmazonChimeAsync.BatchUnsuspendUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchUnsuspendUserAsync(BatchUnsuspendUserRequest batchUnsuspendUserRequest);
/**
*
* Removes the suspension from up to 50 previously suspended users for the specified Amazon Chime
* EnterpriseLWA
account. Only users on EnterpriseLWA
accounts can be unsuspended using
* this action. For more information about different account types, see Managing Your Amazon Chime Accounts
* in the account types, in the Amazon Chime Administration Guide.
*
*
* Previously suspended users who are unsuspended using this action are returned to Registered
status.
* Users who are not previously suspended are ignored.
*
*
* @param batchUnsuspendUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchUnsuspendUser operation returned by the service.
* @sample AmazonChimeAsyncHandler.BatchUnsuspendUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchUnsuspendUserAsync(BatchUnsuspendUserRequest batchUnsuspendUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates phone number product types or calling names. You can update one attribute at a time for each
* UpdatePhoneNumberRequestItem
. For example, you can update the product type or the calling name.
*
*
* For toll-free numbers, you cannot use the Amazon Chime Business Calling product type. For numbers outside the
* U.S., you must use the Amazon Chime SIP Media Application Dial-In product type.
*
*
* Updates to outbound calling names can take up to 72 hours to complete. Pending updates to outbound calling names
* must be complete before you can request another update.
*
*
* @param batchUpdatePhoneNumberRequest
* @return A Java Future containing the result of the BatchUpdatePhoneNumber operation returned by the service.
* @sample AmazonChimeAsync.BatchUpdatePhoneNumber
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchUpdatePhoneNumberAsync(BatchUpdatePhoneNumberRequest batchUpdatePhoneNumberRequest);
/**
*
* Updates phone number product types or calling names. You can update one attribute at a time for each
* UpdatePhoneNumberRequestItem
. For example, you can update the product type or the calling name.
*
*
* For toll-free numbers, you cannot use the Amazon Chime Business Calling product type. For numbers outside the
* U.S., you must use the Amazon Chime SIP Media Application Dial-In product type.
*
*
* Updates to outbound calling names can take up to 72 hours to complete. Pending updates to outbound calling names
* must be complete before you can request another update.
*
*
* @param batchUpdatePhoneNumberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchUpdatePhoneNumber operation returned by the service.
* @sample AmazonChimeAsyncHandler.BatchUpdatePhoneNumber
* @see AWS
* API Documentation
*/
java.util.concurrent.Future batchUpdatePhoneNumberAsync(BatchUpdatePhoneNumberRequest batchUpdatePhoneNumberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates user details within the UpdateUserRequestItem object for up to 20 users for the specified Amazon
* Chime account. Currently, only LicenseType
updates are supported for this action.
*
*
* @param batchUpdateUserRequest
* @return A Java Future containing the result of the BatchUpdateUser operation returned by the service.
* @sample AmazonChimeAsync.BatchUpdateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchUpdateUserAsync(BatchUpdateUserRequest batchUpdateUserRequest);
/**
*
* Updates user details within the UpdateUserRequestItem object for up to 20 users for the specified Amazon
* Chime account. Currently, only LicenseType
updates are supported for this action.
*
*
* @param batchUpdateUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchUpdateUser operation returned by the service.
* @sample AmazonChimeAsyncHandler.BatchUpdateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future batchUpdateUserAsync(BatchUpdateUserRequest batchUpdateUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Chime account under the administrator's AWS account. Only Team
account types are
* currently supported for this action. For more information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* @param createAccountRequest
* @return A Java Future containing the result of the CreateAccount operation returned by the service.
* @sample AmazonChimeAsync.CreateAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAccountAsync(CreateAccountRequest createAccountRequest);
/**
*
* Creates an Amazon Chime account under the administrator's AWS account. Only Team
account types are
* currently supported for this action. For more information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* @param createAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAccount operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createAccountAsync(CreateAccountRequest createAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Chime SDK messaging AppInstance
under an AWS account. Only SDK messaging customers
* use this API. CreateAppInstance
supports idempotency behavior as described in the AWS API Standard.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateAppInstance, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createAppInstanceRequest
* @return A Java Future containing the result of the CreateAppInstance operation returned by the service.
* @sample AmazonChimeAsync.CreateAppInstance
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createAppInstanceAsync(CreateAppInstanceRequest createAppInstanceRequest);
/**
*
* Creates an Amazon Chime SDK messaging AppInstance
under an AWS account. Only SDK messaging customers
* use this API. CreateAppInstance
supports idempotency behavior as described in the AWS API Standard.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateAppInstance, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createAppInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAppInstance operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateAppInstance
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createAppInstanceAsync(CreateAppInstanceRequest createAppInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Promotes an AppInstanceUser
to an AppInstanceAdmin
. The promoted user can perform the
* following actions.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateAppInstanceAdmin, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* -
*
* ChannelModerator
actions across all channels in the AppInstance
.
*
*
* -
*
* DeleteChannelMessage
actions.
*
*
*
*
* Only an AppInstanceUser
can be promoted to an AppInstanceAdmin
role.
*
*
* @param createAppInstanceAdminRequest
* @return A Java Future containing the result of the CreateAppInstanceAdmin operation returned by the service.
* @sample AmazonChimeAsync.CreateAppInstanceAdmin
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future createAppInstanceAdminAsync(CreateAppInstanceAdminRequest createAppInstanceAdminRequest);
/**
*
* Promotes an AppInstanceUser
to an AppInstanceAdmin
. The promoted user can perform the
* following actions.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateAppInstanceAdmin, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* -
*
* ChannelModerator
actions across all channels in the AppInstance
.
*
*
* -
*
* DeleteChannelMessage
actions.
*
*
*
*
* Only an AppInstanceUser
can be promoted to an AppInstanceAdmin
role.
*
*
* @param createAppInstanceAdminRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAppInstanceAdmin operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateAppInstanceAdmin
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future createAppInstanceAdminAsync(CreateAppInstanceAdminRequest createAppInstanceAdminRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a user under an Amazon Chime AppInstance
. The request consists of a unique
* appInstanceUserId
and Name
for that user.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createAppInstanceUserRequest
* @return A Java Future containing the result of the CreateAppInstanceUser operation returned by the service.
* @sample AmazonChimeAsync.CreateAppInstanceUser
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future createAppInstanceUserAsync(CreateAppInstanceUserRequest createAppInstanceUserRequest);
/**
*
* Creates a user under an Amazon Chime AppInstance
. The request consists of a unique
* appInstanceUserId
and Name
for that user.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createAppInstanceUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAppInstanceUser operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateAppInstanceUser
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future createAppInstanceUserAsync(CreateAppInstanceUserRequest createAppInstanceUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new attendee for an active Amazon Chime SDK meeting. For more information about the Amazon Chime SDK,
* see Using the Amazon Chime SDK in
* the Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateAttendee
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createAttendeeRequest
* @return A Java Future containing the result of the CreateAttendee operation returned by the service.
* @sample AmazonChimeAsync.CreateAttendee
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createAttendeeAsync(CreateAttendeeRequest createAttendeeRequest);
/**
*
* Creates a new attendee for an active Amazon Chime SDK meeting. For more information about the Amazon Chime SDK,
* see Using the Amazon Chime SDK in
* the Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateAttendee
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createAttendeeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAttendee operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateAttendee
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createAttendeeAsync(CreateAttendeeRequest createAttendeeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a bot for an Amazon Chime Enterprise account.
*
*
* @param createBotRequest
* @return A Java Future containing the result of the CreateBot operation returned by the service.
* @sample AmazonChimeAsync.CreateBot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createBotAsync(CreateBotRequest createBotRequest);
/**
*
* Creates a bot for an Amazon Chime Enterprise account.
*
*
* @param createBotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateBot operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateBot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createBotAsync(CreateBotRequest createBotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a channel to which you can add users and send messages.
*
*
* Restriction: You can't change a channel's privacy.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateChannel
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelRequest
* @return A Java Future containing the result of the CreateChannel operation returned by the service.
* @sample AmazonChimeAsync.CreateChannel
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createChannelAsync(CreateChannelRequest createChannelRequest);
/**
*
* Creates a channel to which you can add users and send messages.
*
*
* Restriction: You can't change a channel's privacy.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateChannel
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateChannel operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateChannel
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createChannelAsync(CreateChannelRequest createChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Permanently bans a member from a channel. Moderators can't add banned members to a channel. To undo a ban, you
* first have to DeleteChannelBan
, and then CreateChannelMembership
. Bans are cleaned up
* when you delete users or channels.
*
*
* If you ban a user who is already part of a channel, that user is automatically kicked from the channel.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateChannelBan, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelBanRequest
* @return A Java Future containing the result of the CreateChannelBan operation returned by the service.
* @sample AmazonChimeAsync.CreateChannelBan
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createChannelBanAsync(CreateChannelBanRequest createChannelBanRequest);
/**
*
* Permanently bans a member from a channel. Moderators can't add banned members to a channel. To undo a ban, you
* first have to DeleteChannelBan
, and then CreateChannelMembership
. Bans are cleaned up
* when you delete users or channels.
*
*
* If you ban a user who is already part of a channel, that user is automatically kicked from the channel.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateChannelBan, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelBanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateChannelBan operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateChannelBan
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createChannelBanAsync(CreateChannelBanRequest createChannelBanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a user to a channel. The InvitedBy
response field is derived from the request header. A channel
* member can:
*
*
* -
*
* List messages
*
*
* -
*
* Send messages
*
*
* -
*
* Receive messages
*
*
* -
*
* Edit their own messages
*
*
* -
*
* Leave the channel
*
*
*
*
* Privacy settings impact this action as follows:
*
*
* -
*
* Public Channels: You do not need to be a member to list messages, but you must be a member to send messages.
*
*
* -
*
* Private Channels: You must be a member to list or send messages.
*
*
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelMembershipRequest
* @return A Java Future containing the result of the CreateChannelMembership operation returned by the service.
* @sample AmazonChimeAsync.CreateChannelMembership
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future createChannelMembershipAsync(CreateChannelMembershipRequest createChannelMembershipRequest);
/**
*
* Adds a user to a channel. The InvitedBy
response field is derived from the request header. A channel
* member can:
*
*
* -
*
* List messages
*
*
* -
*
* Send messages
*
*
* -
*
* Receive messages
*
*
* -
*
* Edit their own messages
*
*
* -
*
* Leave the channel
*
*
*
*
* Privacy settings impact this action as follows:
*
*
* -
*
* Public Channels: You do not need to be a member to list messages, but you must be a member to send messages.
*
*
* -
*
* Private Channels: You must be a member to list or send messages.
*
*
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateChannelMembership operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateChannelMembership
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future createChannelMembershipAsync(CreateChannelMembershipRequest createChannelMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new ChannelModerator
. A channel moderator can:
*
*
* -
*
* Add and remove other members of the channel.
*
*
* -
*
* Add and remove other moderators of the channel.
*
*
* -
*
* Add and remove user bans for the channel.
*
*
* -
*
* Redact messages in the channel.
*
*
* -
*
* List messages in the channel.
*
*
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateChannelModerator, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelModeratorRequest
* @return A Java Future containing the result of the CreateChannelModerator operation returned by the service.
* @sample AmazonChimeAsync.CreateChannelModerator
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future createChannelModeratorAsync(CreateChannelModeratorRequest createChannelModeratorRequest);
/**
*
* Creates a new ChannelModerator
. A channel moderator can:
*
*
* -
*
* Add and remove other members of the channel.
*
*
* -
*
* Add and remove other moderators of the channel.
*
*
* -
*
* Add and remove user bans for the channel.
*
*
* -
*
* Redact messages in the channel.
*
*
* -
*
* List messages in the channel.
*
*
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateChannelModerator, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelModeratorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateChannelModerator operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateChannelModerator
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future createChannelModeratorAsync(CreateChannelModeratorRequest createChannelModeratorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a media capture pipeline.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateMediaCapturePipeline, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createMediaCapturePipelineRequest
* @return A Java Future containing the result of the CreateMediaCapturePipeline operation returned by the service.
* @sample AmazonChimeAsync.CreateMediaCapturePipeline
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future createMediaCapturePipelineAsync(
CreateMediaCapturePipelineRequest createMediaCapturePipelineRequest);
/**
*
* Creates a media capture pipeline.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateMediaCapturePipeline, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createMediaCapturePipelineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMediaCapturePipeline operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateMediaCapturePipeline
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future createMediaCapturePipelineAsync(
CreateMediaCapturePipelineRequest createMediaCapturePipelineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Amazon Chime SDK meeting in the specified media Region with no initial attendees. For more
* information about specifying media Regions, see Amazon Chime SDK Media
* Regions in the Amazon Chime SDK Developer Guide . For more information about the Amazon Chime SDK, see
* Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateMeeting
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createMeetingRequest
* @return A Java Future containing the result of the CreateMeeting operation returned by the service.
* @sample AmazonChimeAsync.CreateMeeting
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createMeetingAsync(CreateMeetingRequest createMeetingRequest);
/**
*
* Creates a new Amazon Chime SDK meeting in the specified media Region with no initial attendees. For more
* information about specifying media Regions, see Amazon Chime SDK Media
* Regions in the Amazon Chime SDK Developer Guide . For more information about the Amazon Chime SDK, see
* Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateMeeting
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createMeetingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMeeting operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateMeeting
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createMeetingAsync(CreateMeetingRequest createMeetingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Uses the join token and call metadata in a meeting request (From number, To number, and so forth) to initiate an
* outbound call to a public switched telephone network (PSTN) and join them into a Chime meeting. Also ensures that
* the From number belongs to the customer.
*
*
* To play welcome audio or implement an interactive voice response (IVR), use the
* CreateSipMediaApplicationCall
action with the corresponding SIP media application ID.
*
*
*
* This API is is not available in a dedicated namespace.
*
*
*
* @param createMeetingDialOutRequest
* @return A Java Future containing the result of the CreateMeetingDialOut operation returned by the service.
* @sample AmazonChimeAsync.CreateMeetingDialOut
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMeetingDialOutAsync(CreateMeetingDialOutRequest createMeetingDialOutRequest);
/**
*
* Uses the join token and call metadata in a meeting request (From number, To number, and so forth) to initiate an
* outbound call to a public switched telephone network (PSTN) and join them into a Chime meeting. Also ensures that
* the From number belongs to the customer.
*
*
* To play welcome audio or implement an interactive voice response (IVR), use the
* CreateSipMediaApplicationCall
action with the corresponding SIP media application ID.
*
*
*
* This API is is not available in a dedicated namespace.
*
*
*
* @param createMeetingDialOutRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMeetingDialOut operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateMeetingDialOut
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createMeetingDialOutAsync(CreateMeetingDialOutRequest createMeetingDialOutRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Amazon Chime SDK meeting in the specified media Region, with attendees. For more information about
* specifying media Regions, see Amazon Chime SDK Media
* Regions in the Amazon Chime SDK Developer Guide . For more information about the Amazon Chime SDK, see
* Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide .
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateMeetingWithAttendees, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createMeetingWithAttendeesRequest
* @return A Java Future containing the result of the CreateMeetingWithAttendees operation returned by the service.
* @sample AmazonChimeAsync.CreateMeetingWithAttendees
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future createMeetingWithAttendeesAsync(
CreateMeetingWithAttendeesRequest createMeetingWithAttendeesRequest);
/**
*
* Creates a new Amazon Chime SDK meeting in the specified media Region, with attendees. For more information about
* specifying media Regions, see Amazon Chime SDK Media
* Regions in the Amazon Chime SDK Developer Guide . For more information about the Amazon Chime SDK, see
* Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide .
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateMeetingWithAttendees, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createMeetingWithAttendeesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMeetingWithAttendees operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateMeetingWithAttendees
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future createMeetingWithAttendeesAsync(
CreateMeetingWithAttendeesRequest createMeetingWithAttendeesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an order for phone numbers to be provisioned. For toll-free numbers, you cannot use the Amazon Chime
* Business Calling product type. For numbers outside the U.S., you must use the Amazon Chime SIP Media Application
* Dial-In product type.
*
*
* @param createPhoneNumberOrderRequest
* @return A Java Future containing the result of the CreatePhoneNumberOrder operation returned by the service.
* @sample AmazonChimeAsync.CreatePhoneNumberOrder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createPhoneNumberOrderAsync(CreatePhoneNumberOrderRequest createPhoneNumberOrderRequest);
/**
*
* Creates an order for phone numbers to be provisioned. For toll-free numbers, you cannot use the Amazon Chime
* Business Calling product type. For numbers outside the U.S., you must use the Amazon Chime SIP Media Application
* Dial-In product type.
*
*
* @param createPhoneNumberOrderRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreatePhoneNumberOrder operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreatePhoneNumberOrder
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createPhoneNumberOrderAsync(CreatePhoneNumberOrderRequest createPhoneNumberOrderRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a proxy session on the specified Amazon Chime Voice Connector for the specified participant phone
* numbers.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateProxySession, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createProxySessionRequest
* @return A Java Future containing the result of the CreateProxySession operation returned by the service.
* @sample AmazonChimeAsync.CreateProxySession
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createProxySessionAsync(CreateProxySessionRequest createProxySessionRequest);
/**
*
* Creates a proxy session on the specified Amazon Chime Voice Connector for the specified participant phone
* numbers.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateProxySession, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createProxySessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateProxySession operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateProxySession
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createProxySessionAsync(CreateProxySessionRequest createProxySessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a chat room for the specified Amazon Chime Enterprise account.
*
*
* @param createRoomRequest
* @return A Java Future containing the result of the CreateRoom operation returned by the service.
* @sample AmazonChimeAsync.CreateRoom
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRoomAsync(CreateRoomRequest createRoomRequest);
/**
*
* Creates a chat room for the specified Amazon Chime Enterprise account.
*
*
* @param createRoomRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRoom operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateRoom
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRoomAsync(CreateRoomRequest createRoomRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds a member to a chat room in an Amazon Chime Enterprise account. A member can be either a user or a bot. The
* member role designates whether the member is a chat room administrator or a general chat room member.
*
*
* @param createRoomMembershipRequest
* @return A Java Future containing the result of the CreateRoomMembership operation returned by the service.
* @sample AmazonChimeAsync.CreateRoomMembership
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRoomMembershipAsync(CreateRoomMembershipRequest createRoomMembershipRequest);
/**
*
* Adds a member to a chat room in an Amazon Chime Enterprise account. A member can be either a user or a bot. The
* member role designates whether the member is a chat room administrator or a general chat room member.
*
*
* @param createRoomMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateRoomMembership operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateRoomMembership
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createRoomMembershipAsync(CreateRoomMembershipRequest createRoomMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a SIP media application.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateSipMediaApplication, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createSipMediaApplicationRequest
* @return A Java Future containing the result of the CreateSipMediaApplication operation returned by the service.
* @sample AmazonChimeAsync.CreateSipMediaApplication
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future createSipMediaApplicationAsync(
CreateSipMediaApplicationRequest createSipMediaApplicationRequest);
/**
*
* Creates a SIP media application.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateSipMediaApplication, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createSipMediaApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSipMediaApplication operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateSipMediaApplication
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future createSipMediaApplicationAsync(
CreateSipMediaApplicationRequest createSipMediaApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an outbound call to a phone number from the phone number specified in the request, and it invokes the
* endpoint of the specified sipMediaApplicationId
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateSipMediaApplicationCall, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createSipMediaApplicationCallRequest
* @return A Java Future containing the result of the CreateSipMediaApplicationCall operation returned by the
* service.
* @sample AmazonChimeAsync.CreateSipMediaApplicationCall
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future createSipMediaApplicationCallAsync(
CreateSipMediaApplicationCallRequest createSipMediaApplicationCallRequest);
/**
*
* Creates an outbound call to a phone number from the phone number specified in the request, and it invokes the
* endpoint of the specified sipMediaApplicationId
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateSipMediaApplicationCall, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createSipMediaApplicationCallRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSipMediaApplicationCall operation returned by the
* service.
* @sample AmazonChimeAsyncHandler.CreateSipMediaApplicationCall
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future createSipMediaApplicationCallAsync(
CreateSipMediaApplicationCallRequest createSipMediaApplicationCallRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a SIP rule which can be used to run a SIP media application as a target for a specific trigger type.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateSipRule,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createSipRuleRequest
* @return A Java Future containing the result of the CreateSipRule operation returned by the service.
* @sample AmazonChimeAsync.CreateSipRule
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createSipRuleAsync(CreateSipRuleRequest createSipRuleRequest);
/**
*
* Creates a SIP rule which can be used to run a SIP media application as a target for a specific trigger type.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateSipRule,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createSipRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateSipRule operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateSipRule
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createSipRuleAsync(CreateSipRuleRequest createSipRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a user under the specified Amazon Chime account.
*
*
* @param createUserRequest
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* @sample AmazonChimeAsync.CreateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createUserAsync(CreateUserRequest createUserRequest);
/**
*
* Creates a user under the specified Amazon Chime account.
*
*
* @param createUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateUser operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateUser
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createUserAsync(CreateUserRequest createUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Chime Voice Connector under the administrator's AWS account. You can choose to create an Amazon
* Chime Voice Connector in a specific AWS Region.
*
*
* Enabling CreateVoiceConnectorRequest$RequireEncryption configures your Amazon Chime Voice Connector to use
* TLS transport for SIP signaling and Secure RTP (SRTP) for media. Inbound calls use TLS transport, and unencrypted
* outbound calls are blocked.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createVoiceConnectorRequest
* @return A Java Future containing the result of the CreateVoiceConnector operation returned by the service.
* @sample AmazonChimeAsync.CreateVoiceConnector
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createVoiceConnectorAsync(CreateVoiceConnectorRequest createVoiceConnectorRequest);
/**
*
* Creates an Amazon Chime Voice Connector under the administrator's AWS account. You can choose to create an Amazon
* Chime Voice Connector in a specific AWS Region.
*
*
* Enabling CreateVoiceConnectorRequest$RequireEncryption configures your Amazon Chime Voice Connector to use
* TLS transport for SIP signaling and Secure RTP (SRTP) for media. Inbound calls use TLS transport, and unencrypted
* outbound calls are blocked.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createVoiceConnectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVoiceConnector operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateVoiceConnector
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future createVoiceConnectorAsync(CreateVoiceConnectorRequest createVoiceConnectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Chime Voice Connector group under the administrator's AWS account. You can associate Amazon
* Chime Voice Connectors with the Amazon Chime Voice Connector group by including VoiceConnectorItems
* in the request.
*
*
* You can include Amazon Chime Voice Connectors from different AWS Regions in your group. This creates a fault
* tolerant mechanism for fallback in case of availability events.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createVoiceConnectorGroupRequest
* @return A Java Future containing the result of the CreateVoiceConnectorGroup operation returned by the service.
* @sample AmazonChimeAsync.CreateVoiceConnectorGroup
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future createVoiceConnectorGroupAsync(
CreateVoiceConnectorGroupRequest createVoiceConnectorGroupRequest);
/**
*
* Creates an Amazon Chime Voice Connector group under the administrator's AWS account. You can associate Amazon
* Chime Voice Connectors with the Amazon Chime Voice Connector group by including VoiceConnectorItems
* in the request.
*
*
* You can include Amazon Chime Voice Connectors from different AWS Regions in your group. This creates a fault
* tolerant mechanism for fallback in case of availability events.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createVoiceConnectorGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateVoiceConnectorGroup operation returned by the service.
* @sample AmazonChimeAsyncHandler.CreateVoiceConnectorGroup
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future createVoiceConnectorGroupAsync(
CreateVoiceConnectorGroupRequest createVoiceConnectorGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified Amazon Chime account. You must suspend all users before deleting Team
account.
* You can use the BatchSuspendUser action to dodo.
*
*
* For EnterpriseLWA
and EnterpriseAD
accounts, you must release the claimed domains for
* your Amazon Chime account before deletion. As soon as you release the domain, all users under that account are
* suspended.
*
*
* Deleted accounts appear in your Disabled
accounts list for 90 days. To restore deleted account from
* your Disabled
accounts list, you must contact AWS Support.
*
*
* After 90 days, deleted accounts are permanently removed from your Disabled
accounts list.
*
*
* @param deleteAccountRequest
* @return A Java Future containing the result of the DeleteAccount operation returned by the service.
* @sample AmazonChimeAsync.DeleteAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAccountAsync(DeleteAccountRequest deleteAccountRequest);
/**
*
* Deletes the specified Amazon Chime account. You must suspend all users before deleting Team
account.
* You can use the BatchSuspendUser action to dodo.
*
*
* For EnterpriseLWA
and EnterpriseAD
accounts, you must release the claimed domains for
* your Amazon Chime account before deletion. As soon as you release the domain, all users under that account are
* suspended.
*
*
* Deleted accounts appear in your Disabled
accounts list for 90 days. To restore deleted account from
* your Disabled
accounts list, you must contact AWS Support.
*
*
* After 90 days, deleted accounts are permanently removed from your Disabled
accounts list.
*
*
* @param deleteAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAccount operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteAccountAsync(DeleteAccountRequest deleteAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an AppInstance
and all associated data asynchronously.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteAppInstance, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceRequest
* @return A Java Future containing the result of the DeleteAppInstance operation returned by the service.
* @sample AmazonChimeAsync.DeleteAppInstance
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteAppInstanceAsync(DeleteAppInstanceRequest deleteAppInstanceRequest);
/**
*
* Deletes an AppInstance
and all associated data asynchronously.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteAppInstance, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppInstance operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteAppInstance
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteAppInstanceAsync(DeleteAppInstanceRequest deleteAppInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Demotes an AppInstanceAdmin
to an AppInstanceUser
. This action does not delete the
* user.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteAppInstanceAdmin, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceAdminRequest
* @return A Java Future containing the result of the DeleteAppInstanceAdmin operation returned by the service.
* @sample AmazonChimeAsync.DeleteAppInstanceAdmin
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteAppInstanceAdminAsync(DeleteAppInstanceAdminRequest deleteAppInstanceAdminRequest);
/**
*
* Demotes an AppInstanceAdmin
to an AppInstanceUser
. This action does not delete the
* user.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteAppInstanceAdmin, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceAdminRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppInstanceAdmin operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteAppInstanceAdmin
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteAppInstanceAdminAsync(DeleteAppInstanceAdminRequest deleteAppInstanceAdminRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the streaming configurations of an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteAppInstanceStreamingConfigurations, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceStreamingConfigurationsRequest
* @return A Java Future containing the result of the DeleteAppInstanceStreamingConfigurations operation returned by
* the service.
* @sample AmazonChimeAsync.DeleteAppInstanceStreamingConfigurations
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteAppInstanceStreamingConfigurationsAsync(
DeleteAppInstanceStreamingConfigurationsRequest deleteAppInstanceStreamingConfigurationsRequest);
/**
*
* Deletes the streaming configurations of an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteAppInstanceStreamingConfigurations, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceStreamingConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppInstanceStreamingConfigurations operation returned by
* the service.
* @sample AmazonChimeAsyncHandler.DeleteAppInstanceStreamingConfigurations
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteAppInstanceStreamingConfigurationsAsync(
DeleteAppInstanceStreamingConfigurationsRequest deleteAppInstanceStreamingConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an AppInstanceUser
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceUserRequest
* @return A Java Future containing the result of the DeleteAppInstanceUser operation returned by the service.
* @sample AmazonChimeAsync.DeleteAppInstanceUser
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteAppInstanceUserAsync(DeleteAppInstanceUserRequest deleteAppInstanceUserRequest);
/**
*
* Deletes an AppInstanceUser
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppInstanceUser operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteAppInstanceUser
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteAppInstanceUserAsync(DeleteAppInstanceUserRequest deleteAppInstanceUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an attendee from the specified Amazon Chime SDK meeting and deletes their JoinToken
.
* Attendees are automatically deleted when a Amazon Chime SDK meeting is deleted. For more information about the
* Amazon Chime SDK, see Using the
* Amazon Chime SDK in the Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteAttendee
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAttendeeRequest
* @return A Java Future containing the result of the DeleteAttendee operation returned by the service.
* @sample AmazonChimeAsync.DeleteAttendee
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteAttendeeAsync(DeleteAttendeeRequest deleteAttendeeRequest);
/**
*
* Deletes an attendee from the specified Amazon Chime SDK meeting and deletes their JoinToken
.
* Attendees are automatically deleted when a Amazon Chime SDK meeting is deleted. For more information about the
* Amazon Chime SDK, see Using the
* Amazon Chime SDK in the Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteAttendee
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAttendeeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAttendee operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteAttendee
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteAttendeeAsync(DeleteAttendeeRequest deleteAttendeeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Immediately makes a channel and its memberships inaccessible and marks them for deletion. This is an irreversible
* process.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteChannel
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelRequest
* @return A Java Future containing the result of the DeleteChannel operation returned by the service.
* @sample AmazonChimeAsync.DeleteChannel
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteChannelAsync(DeleteChannelRequest deleteChannelRequest);
/**
*
* Immediately makes a channel and its memberships inaccessible and marks them for deletion. This is an irreversible
* process.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteChannel
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteChannel operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteChannel
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteChannelAsync(DeleteChannelRequest deleteChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a user from a channel's ban list.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelBan, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelBanRequest
* @return A Java Future containing the result of the DeleteChannelBan operation returned by the service.
* @sample AmazonChimeAsync.DeleteChannelBan
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteChannelBanAsync(DeleteChannelBanRequest deleteChannelBanRequest);
/**
*
* Removes a user from a channel's ban list.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelBan, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelBanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteChannelBan operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteChannelBan
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteChannelBanAsync(DeleteChannelBanRequest deleteChannelBanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a member from a channel.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelMembershipRequest
* @return A Java Future containing the result of the DeleteChannelMembership operation returned by the service.
* @sample AmazonChimeAsync.DeleteChannelMembership
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteChannelMembershipAsync(DeleteChannelMembershipRequest deleteChannelMembershipRequest);
/**
*
* Removes a member from a channel.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteChannelMembership operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteChannelMembership
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteChannelMembershipAsync(DeleteChannelMembershipRequest deleteChannelMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a channel message. Only admins can perform this action. Deletion makes messages inaccessible immediately.
* A background process deletes any revisions created by UpdateChannelMessage
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelMessage, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelMessageRequest
* @return A Java Future containing the result of the DeleteChannelMessage operation returned by the service.
* @sample AmazonChimeAsync.DeleteChannelMessage
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteChannelMessageAsync(DeleteChannelMessageRequest deleteChannelMessageRequest);
/**
*
* Deletes a channel message. Only admins can perform this action. Deletion makes messages inaccessible immediately.
* A background process deletes any revisions created by UpdateChannelMessage
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelMessage, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelMessageRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteChannelMessage operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteChannelMessage
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteChannelMessageAsync(DeleteChannelMessageRequest deleteChannelMessageRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a channel moderator.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelModerator, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelModeratorRequest
* @return A Java Future containing the result of the DeleteChannelModerator operation returned by the service.
* @sample AmazonChimeAsync.DeleteChannelModerator
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteChannelModeratorAsync(DeleteChannelModeratorRequest deleteChannelModeratorRequest);
/**
*
* Deletes a channel moderator.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelModerator, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelModeratorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteChannelModerator operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteChannelModerator
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteChannelModeratorAsync(DeleteChannelModeratorRequest deleteChannelModeratorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the events configuration that allows a bot to receive outgoing events.
*
*
* @param deleteEventsConfigurationRequest
* @return A Java Future containing the result of the DeleteEventsConfiguration operation returned by the service.
* @sample AmazonChimeAsync.DeleteEventsConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEventsConfigurationAsync(
DeleteEventsConfigurationRequest deleteEventsConfigurationRequest);
/**
*
* Deletes the events configuration that allows a bot to receive outgoing events.
*
*
* @param deleteEventsConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEventsConfiguration operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteEventsConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEventsConfigurationAsync(
DeleteEventsConfigurationRequest deleteEventsConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the media capture pipeline.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteMediaCapturePipeline, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteMediaCapturePipelineRequest
* @return A Java Future containing the result of the DeleteMediaCapturePipeline operation returned by the service.
* @sample AmazonChimeAsync.DeleteMediaCapturePipeline
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteMediaCapturePipelineAsync(
DeleteMediaCapturePipelineRequest deleteMediaCapturePipelineRequest);
/**
*
* Deletes the media capture pipeline.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteMediaCapturePipeline, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteMediaCapturePipelineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMediaCapturePipeline operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteMediaCapturePipeline
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteMediaCapturePipelineAsync(
DeleteMediaCapturePipelineRequest deleteMediaCapturePipelineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified Amazon Chime SDK meeting. The operation deletes all attendees, disconnects all clients, and
* prevents new clients from joining the meeting. For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteMeeting
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteMeetingRequest
* @return A Java Future containing the result of the DeleteMeeting operation returned by the service.
* @sample AmazonChimeAsync.DeleteMeeting
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteMeetingAsync(DeleteMeetingRequest deleteMeetingRequest);
/**
*
* Deletes the specified Amazon Chime SDK meeting. The operation deletes all attendees, disconnects all clients, and
* prevents new clients from joining the meeting. For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteMeeting
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteMeetingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMeeting operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteMeeting
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteMeetingAsync(DeleteMeetingRequest deleteMeetingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Moves the specified phone number into the Deletion queue. A phone number must be disassociated from any
* users or Amazon Chime Voice Connectors before it can be deleted.
*
*
* Deleted phone numbers remain in the Deletion queue for 7 days before they are deleted permanently.
*
*
* @param deletePhoneNumberRequest
* @return A Java Future containing the result of the DeletePhoneNumber operation returned by the service.
* @sample AmazonChimeAsync.DeletePhoneNumber
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deletePhoneNumberAsync(DeletePhoneNumberRequest deletePhoneNumberRequest);
/**
*
* Moves the specified phone number into the Deletion queue. A phone number must be disassociated from any
* users or Amazon Chime Voice Connectors before it can be deleted.
*
*
* Deleted phone numbers remain in the Deletion queue for 7 days before they are deleted permanently.
*
*
* @param deletePhoneNumberRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeletePhoneNumber operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeletePhoneNumber
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deletePhoneNumberAsync(DeletePhoneNumberRequest deletePhoneNumberRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified proxy session from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteProxySession, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteProxySessionRequest
* @return A Java Future containing the result of the DeleteProxySession operation returned by the service.
* @sample AmazonChimeAsync.DeleteProxySession
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteProxySessionAsync(DeleteProxySessionRequest deleteProxySessionRequest);
/**
*
* Deletes the specified proxy session from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteProxySession, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteProxySessionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteProxySession operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteProxySession
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteProxySessionAsync(DeleteProxySessionRequest deleteProxySessionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a chat room in an Amazon Chime Enterprise account.
*
*
* @param deleteRoomRequest
* @return A Java Future containing the result of the DeleteRoom operation returned by the service.
* @sample AmazonChimeAsync.DeleteRoom
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRoomAsync(DeleteRoomRequest deleteRoomRequest);
/**
*
* Deletes a chat room in an Amazon Chime Enterprise account.
*
*
* @param deleteRoomRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRoom operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteRoom
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRoomAsync(DeleteRoomRequest deleteRoomRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes a member from a chat room in an Amazon Chime Enterprise account.
*
*
* @param deleteRoomMembershipRequest
* @return A Java Future containing the result of the DeleteRoomMembership operation returned by the service.
* @sample AmazonChimeAsync.DeleteRoomMembership
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRoomMembershipAsync(DeleteRoomMembershipRequest deleteRoomMembershipRequest);
/**
*
* Removes a member from a chat room in an Amazon Chime Enterprise account.
*
*
* @param deleteRoomMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteRoomMembership operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteRoomMembership
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteRoomMembershipAsync(DeleteRoomMembershipRequest deleteRoomMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a SIP media application.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteSipMediaApplication, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteSipMediaApplicationRequest
* @return A Java Future containing the result of the DeleteSipMediaApplication operation returned by the service.
* @sample AmazonChimeAsync.DeleteSipMediaApplication
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteSipMediaApplicationAsync(
DeleteSipMediaApplicationRequest deleteSipMediaApplicationRequest);
/**
*
* Deletes a SIP media application.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteSipMediaApplication, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteSipMediaApplicationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSipMediaApplication operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteSipMediaApplication
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteSipMediaApplicationAsync(
DeleteSipMediaApplicationRequest deleteSipMediaApplicationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a SIP rule. You must disable a SIP rule before you can delete it.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteSipRule,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteSipRuleRequest
* @return A Java Future containing the result of the DeleteSipRule operation returned by the service.
* @sample AmazonChimeAsync.DeleteSipRule
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteSipRuleAsync(DeleteSipRuleRequest deleteSipRuleRequest);
/**
*
* Deletes a SIP rule. You must disable a SIP rule before you can delete it.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteSipRule,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteSipRuleRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteSipRule operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteSipRule
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteSipRuleAsync(DeleteSipRuleRequest deleteSipRuleRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified Amazon Chime Voice Connector. Any phone numbers associated with the Amazon Chime Voice
* Connector must be disassociated from it before it can be deleted.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorRequest
* @return A Java Future containing the result of the DeleteVoiceConnector operation returned by the service.
* @sample AmazonChimeAsync.DeleteVoiceConnector
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorAsync(DeleteVoiceConnectorRequest deleteVoiceConnectorRequest);
/**
*
* Deletes the specified Amazon Chime Voice Connector. Any phone numbers associated with the Amazon Chime Voice
* Connector must be disassociated from it before it can be deleted.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVoiceConnector operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteVoiceConnector
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorAsync(DeleteVoiceConnectorRequest deleteVoiceConnectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the emergency calling configuration details from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorEmergencyCallingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorEmergencyCallingConfigurationRequest
* @return A Java Future containing the result of the DeleteVoiceConnectorEmergencyCallingConfiguration operation
* returned by the service.
* @sample AmazonChimeAsync.DeleteVoiceConnectorEmergencyCallingConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorEmergencyCallingConfigurationAsync(
DeleteVoiceConnectorEmergencyCallingConfigurationRequest deleteVoiceConnectorEmergencyCallingConfigurationRequest);
/**
*
* Deletes the emergency calling configuration details from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorEmergencyCallingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorEmergencyCallingConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVoiceConnectorEmergencyCallingConfiguration operation
* returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteVoiceConnectorEmergencyCallingConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorEmergencyCallingConfigurationAsync(
DeleteVoiceConnectorEmergencyCallingConfigurationRequest deleteVoiceConnectorEmergencyCallingConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified Amazon Chime Voice Connector group. Any VoiceConnectorItems
and phone numbers
* associated with the group must be removed before it can be deleted.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorGroupRequest
* @return A Java Future containing the result of the DeleteVoiceConnectorGroup operation returned by the service.
* @sample AmazonChimeAsync.DeleteVoiceConnectorGroup
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorGroupAsync(
DeleteVoiceConnectorGroupRequest deleteVoiceConnectorGroupRequest);
/**
*
* Deletes the specified Amazon Chime Voice Connector group. Any VoiceConnectorItems
and phone numbers
* associated with the group must be removed before it can be deleted.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVoiceConnectorGroup operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteVoiceConnectorGroup
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorGroupAsync(
DeleteVoiceConnectorGroupRequest deleteVoiceConnectorGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the origination settings for the specified Amazon Chime Voice Connector.
*
*
*
* If emergency calling is configured for the Amazon Chime Voice Connector, it must be deleted prior to deleting the
* origination settings.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorOrigination, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorOriginationRequest
* @return A Java Future containing the result of the DeleteVoiceConnectorOrigination operation returned by the
* service.
* @sample AmazonChimeAsync.DeleteVoiceConnectorOrigination
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorOriginationAsync(
DeleteVoiceConnectorOriginationRequest deleteVoiceConnectorOriginationRequest);
/**
*
* Deletes the origination settings for the specified Amazon Chime Voice Connector.
*
*
*
* If emergency calling is configured for the Amazon Chime Voice Connector, it must be deleted prior to deleting the
* origination settings.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorOrigination, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorOriginationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVoiceConnectorOrigination operation returned by the
* service.
* @sample AmazonChimeAsyncHandler.DeleteVoiceConnectorOrigination
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorOriginationAsync(
DeleteVoiceConnectorOriginationRequest deleteVoiceConnectorOriginationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the proxy configuration from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteVoiceProxy, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorProxyRequest
* @return A Java Future containing the result of the DeleteVoiceConnectorProxy operation returned by the service.
* @sample AmazonChimeAsync.DeleteVoiceConnectorProxy
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorProxyAsync(
DeleteVoiceConnectorProxyRequest deleteVoiceConnectorProxyRequest);
/**
*
* Deletes the proxy configuration from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteVoiceProxy, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorProxyRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVoiceConnectorProxy operation returned by the service.
* @sample AmazonChimeAsyncHandler.DeleteVoiceConnectorProxy
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorProxyAsync(
DeleteVoiceConnectorProxyRequest deleteVoiceConnectorProxyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the streaming configuration for the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorStreamingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorStreamingConfigurationRequest
* @return A Java Future containing the result of the DeleteVoiceConnectorStreamingConfiguration operation returned
* by the service.
* @sample AmazonChimeAsync.DeleteVoiceConnectorStreamingConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorStreamingConfigurationAsync(
DeleteVoiceConnectorStreamingConfigurationRequest deleteVoiceConnectorStreamingConfigurationRequest);
/**
*
* Deletes the streaming configuration for the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorStreamingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorStreamingConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVoiceConnectorStreamingConfiguration operation returned
* by the service.
* @sample AmazonChimeAsyncHandler.DeleteVoiceConnectorStreamingConfiguration
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorStreamingConfigurationAsync(
DeleteVoiceConnectorStreamingConfigurationRequest deleteVoiceConnectorStreamingConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the termination settings for the specified Amazon Chime Voice Connector.
*
*
*
* If emergency calling is configured for the Amazon Chime Voice Connector, it must be deleted prior to deleting the
* termination settings.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorTermination, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorTerminationRequest
* @return A Java Future containing the result of the DeleteVoiceConnectorTermination operation returned by the
* service.
* @sample AmazonChimeAsync.DeleteVoiceConnectorTermination
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorTerminationAsync(
DeleteVoiceConnectorTerminationRequest deleteVoiceConnectorTerminationRequest);
/**
*
* Deletes the termination settings for the specified Amazon Chime Voice Connector.
*
*
*
* If emergency calling is configured for the Amazon Chime Voice Connector, it must be deleted prior to deleting the
* termination settings.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorTermination, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorTerminationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVoiceConnectorTermination operation returned by the
* service.
* @sample AmazonChimeAsyncHandler.DeleteVoiceConnectorTermination
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorTerminationAsync(
DeleteVoiceConnectorTerminationRequest deleteVoiceConnectorTerminationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified SIP credentials used by your equipment to authenticate during call termination.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorTerminationCredentials, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorTerminationCredentialsRequest
* @return A Java Future containing the result of the DeleteVoiceConnectorTerminationCredentials operation returned
* by the service.
* @sample AmazonChimeAsync.DeleteVoiceConnectorTerminationCredentials
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorTerminationCredentialsAsync(
DeleteVoiceConnectorTerminationCredentialsRequest deleteVoiceConnectorTerminationCredentialsRequest);
/**
*
* Deletes the specified SIP credentials used by your equipment to authenticate during call termination.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorTerminationCredentials, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorTerminationCredentialsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteVoiceConnectorTerminationCredentials operation returned
* by the service.
* @sample AmazonChimeAsyncHandler.DeleteVoiceConnectorTerminationCredentials
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future deleteVoiceConnectorTerminationCredentialsAsync(
DeleteVoiceConnectorTerminationCredentialsRequest deleteVoiceConnectorTerminationCredentialsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the full details of an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeAppInstance, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeAppInstanceRequest
* @return A Java Future containing the result of the DescribeAppInstance operation returned by the service.
* @sample AmazonChimeAsync.DescribeAppInstance
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future describeAppInstanceAsync(DescribeAppInstanceRequest describeAppInstanceRequest);
/**
*
* Returns the full details of an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeAppInstance, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeAppInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAppInstance operation returned by the service.
* @sample AmazonChimeAsyncHandler.DescribeAppInstance
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future describeAppInstanceAsync(DescribeAppInstanceRequest describeAppInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the full details of an AppInstanceAdmin
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeAppInstanceAdmin, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeAppInstanceAdminRequest
* @return A Java Future containing the result of the DescribeAppInstanceAdmin operation returned by the service.
* @sample AmazonChimeAsync.DescribeAppInstanceAdmin
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future describeAppInstanceAdminAsync(DescribeAppInstanceAdminRequest describeAppInstanceAdminRequest);
/**
*
* Returns the full details of an AppInstanceAdmin
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeAppInstanceAdmin, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeAppInstanceAdminRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAppInstanceAdmin operation returned by the service.
* @sample AmazonChimeAsyncHandler.DescribeAppInstanceAdmin
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future describeAppInstanceAdminAsync(DescribeAppInstanceAdminRequest describeAppInstanceAdminRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the full details of an AppInstanceUser
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeAppInstanceUserRequest
* @return A Java Future containing the result of the DescribeAppInstanceUser operation returned by the service.
* @sample AmazonChimeAsync.DescribeAppInstanceUser
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future describeAppInstanceUserAsync(DescribeAppInstanceUserRequest describeAppInstanceUserRequest);
/**
*
* Returns the full details of an AppInstanceUser
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeAppInstanceUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAppInstanceUser operation returned by the service.
* @sample AmazonChimeAsyncHandler.DescribeAppInstanceUser
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future describeAppInstanceUserAsync(DescribeAppInstanceUserRequest describeAppInstanceUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the full details of a channel in an Amazon Chime AppInstance
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeChannel, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelRequest
* @return A Java Future containing the result of the DescribeChannel operation returned by the service.
* @sample AmazonChimeAsync.DescribeChannel
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future describeChannelAsync(DescribeChannelRequest describeChannelRequest);
/**
*
* Returns the full details of a channel in an Amazon Chime AppInstance
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeChannel, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeChannel operation returned by the service.
* @sample AmazonChimeAsyncHandler.DescribeChannel
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future describeChannelAsync(DescribeChannelRequest describeChannelRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the full details of a channel ban.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeChannelBan, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelBanRequest
* @return A Java Future containing the result of the DescribeChannelBan operation returned by the service.
* @sample AmazonChimeAsync.DescribeChannelBan
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future describeChannelBanAsync(DescribeChannelBanRequest describeChannelBanRequest);
/**
*
* Returns the full details of a channel ban.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeChannelBan, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelBanRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeChannelBan operation returned by the service.
* @sample AmazonChimeAsyncHandler.DescribeChannelBan
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future describeChannelBanAsync(DescribeChannelBanRequest describeChannelBanRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the full details of a user's channel membership.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelMembershipRequest
* @return A Java Future containing the result of the DescribeChannelMembership operation returned by the service.
* @sample AmazonChimeAsync.DescribeChannelMembership
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeChannelMembershipAsync(
DescribeChannelMembershipRequest describeChannelMembershipRequest);
/**
*
* Returns the full details of a user's channel membership.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeChannelMembership operation returned by the service.
* @sample AmazonChimeAsyncHandler.DescribeChannelMembership
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeChannelMembershipAsync(
DescribeChannelMembershipRequest describeChannelMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the details of a channel based on the membership of the specified AppInstanceUser
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelMembershipForAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelMembershipForAppInstanceUserRequest
* @return A Java Future containing the result of the DescribeChannelMembershipForAppInstanceUser operation returned
* by the service.
* @sample AmazonChimeAsync.DescribeChannelMembershipForAppInstanceUser
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeChannelMembershipForAppInstanceUserAsync(
DescribeChannelMembershipForAppInstanceUserRequest describeChannelMembershipForAppInstanceUserRequest);
/**
*
* Returns the details of a channel based on the membership of the specified AppInstanceUser
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelMembershipForAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelMembershipForAppInstanceUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeChannelMembershipForAppInstanceUser operation returned
* by the service.
* @sample AmazonChimeAsyncHandler.DescribeChannelMembershipForAppInstanceUser
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeChannelMembershipForAppInstanceUserAsync(
DescribeChannelMembershipForAppInstanceUserRequest describeChannelMembershipForAppInstanceUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the full details of a channel moderated by the specified AppInstanceUser
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelModeratedByAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelModeratedByAppInstanceUserRequest
* @return A Java Future containing the result of the DescribeChannelModeratedByAppInstanceUser operation returned
* by the service.
* @sample AmazonChimeAsync.DescribeChannelModeratedByAppInstanceUser
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeChannelModeratedByAppInstanceUserAsync(
DescribeChannelModeratedByAppInstanceUserRequest describeChannelModeratedByAppInstanceUserRequest);
/**
*
* Returns the full details of a channel moderated by the specified AppInstanceUser
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelModeratedByAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelModeratedByAppInstanceUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeChannelModeratedByAppInstanceUser operation returned
* by the service.
* @sample AmazonChimeAsyncHandler.DescribeChannelModeratedByAppInstanceUser
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future describeChannelModeratedByAppInstanceUserAsync(
DescribeChannelModeratedByAppInstanceUserRequest describeChannelModeratedByAppInstanceUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the full details of a single ChannelModerator.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelModerator, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelModeratorRequest
* @return A Java Future containing the result of the DescribeChannelModerator operation returned by the service.
* @sample AmazonChimeAsync.DescribeChannelModerator
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future describeChannelModeratorAsync(DescribeChannelModeratorRequest describeChannelModeratorRequest);
/**
*
* Returns the full details of a single ChannelModerator.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelModerator, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelModeratorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeChannelModerator operation returned by the service.
* @sample AmazonChimeAsyncHandler.DescribeChannelModerator
* @see AWS
* API Documentation
*/
@Deprecated
java.util.concurrent.Future describeChannelModeratorAsync(DescribeChannelModeratorRequest describeChannelModeratorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates the primary provisioned phone number from the specified Amazon Chime user.
*
*
* @param disassociatePhoneNumberFromUserRequest
* @return A Java Future containing the result of the DisassociatePhoneNumberFromUser operation returned by the
* service.
* @sample AmazonChimeAsync.DisassociatePhoneNumberFromUser
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociatePhoneNumberFromUserAsync(
DisassociatePhoneNumberFromUserRequest disassociatePhoneNumberFromUserRequest);
/**
*
* Disassociates the primary provisioned phone number from the specified Amazon Chime user.
*
*
* @param disassociatePhoneNumberFromUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociatePhoneNumberFromUser operation returned by the
* service.
* @sample AmazonChimeAsyncHandler.DisassociatePhoneNumberFromUser
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociatePhoneNumberFromUserAsync(
DisassociatePhoneNumberFromUserRequest disassociatePhoneNumberFromUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates the specified phone numbers from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DisassociatePhoneNumbersFromVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param disassociatePhoneNumbersFromVoiceConnectorRequest
* @return A Java Future containing the result of the DisassociatePhoneNumbersFromVoiceConnector operation returned
* by the service.
* @sample AmazonChimeAsync.DisassociatePhoneNumbersFromVoiceConnector
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future disassociatePhoneNumbersFromVoiceConnectorAsync(
DisassociatePhoneNumbersFromVoiceConnectorRequest disassociatePhoneNumbersFromVoiceConnectorRequest);
/**
*
* Disassociates the specified phone numbers from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DisassociatePhoneNumbersFromVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param disassociatePhoneNumbersFromVoiceConnectorRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociatePhoneNumbersFromVoiceConnector operation returned
* by the service.
* @sample AmazonChimeAsyncHandler.DisassociatePhoneNumbersFromVoiceConnector
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future disassociatePhoneNumbersFromVoiceConnectorAsync(
DisassociatePhoneNumbersFromVoiceConnectorRequest disassociatePhoneNumbersFromVoiceConnectorRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates the specified phone numbers from the specified Amazon Chime Voice Connector group.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DisassociatePhoneNumbersFromVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param disassociatePhoneNumbersFromVoiceConnectorGroupRequest
* @return A Java Future containing the result of the DisassociatePhoneNumbersFromVoiceConnectorGroup operation
* returned by the service.
* @sample AmazonChimeAsync.DisassociatePhoneNumbersFromVoiceConnectorGroup
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future disassociatePhoneNumbersFromVoiceConnectorGroupAsync(
DisassociatePhoneNumbersFromVoiceConnectorGroupRequest disassociatePhoneNumbersFromVoiceConnectorGroupRequest);
/**
*
* Disassociates the specified phone numbers from the specified Amazon Chime Voice Connector group.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DisassociatePhoneNumbersFromVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param disassociatePhoneNumbersFromVoiceConnectorGroupRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociatePhoneNumbersFromVoiceConnectorGroup operation
* returned by the service.
* @sample AmazonChimeAsyncHandler.DisassociatePhoneNumbersFromVoiceConnectorGroup
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future disassociatePhoneNumbersFromVoiceConnectorGroupAsync(
DisassociatePhoneNumbersFromVoiceConnectorGroupRequest disassociatePhoneNumbersFromVoiceConnectorGroupRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Disassociates the specified sign-in delegate groups from the specified Amazon Chime account.
*
*
* @param disassociateSigninDelegateGroupsFromAccountRequest
* @return A Java Future containing the result of the DisassociateSigninDelegateGroupsFromAccount operation returned
* by the service.
* @sample AmazonChimeAsync.DisassociateSigninDelegateGroupsFromAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateSigninDelegateGroupsFromAccountAsync(
DisassociateSigninDelegateGroupsFromAccountRequest disassociateSigninDelegateGroupsFromAccountRequest);
/**
*
* Disassociates the specified sign-in delegate groups from the specified Amazon Chime account.
*
*
* @param disassociateSigninDelegateGroupsFromAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DisassociateSigninDelegateGroupsFromAccount operation returned
* by the service.
* @sample AmazonChimeAsyncHandler.DisassociateSigninDelegateGroupsFromAccount
* @see AWS API Documentation
*/
java.util.concurrent.Future disassociateSigninDelegateGroupsFromAccountAsync(
DisassociateSigninDelegateGroupsFromAccountRequest disassociateSigninDelegateGroupsFromAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves details for the specified Amazon Chime account, such as account type and supported licenses.
*
*
* @param getAccountRequest
* @return A Java Future containing the result of the GetAccount operation returned by the service.
* @sample AmazonChimeAsync.GetAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAccountAsync(GetAccountRequest getAccountRequest);
/**
*
* Retrieves details for the specified Amazon Chime account, such as account type and supported licenses.
*
*
* @param getAccountRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAccount operation returned by the service.
* @sample AmazonChimeAsyncHandler.GetAccount
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAccountAsync(GetAccountRequest getAccountRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves account settings for the specified Amazon Chime account ID, such as remote control and dialout
* settings. For more information about these settings, see Use the Policies Page in the Amazon Chime
* Administration Guide.
*
*
* @param getAccountSettingsRequest
* @return A Java Future containing the result of the GetAccountSettings operation returned by the service.
* @sample AmazonChimeAsync.GetAccountSettings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAccountSettingsAsync(GetAccountSettingsRequest getAccountSettingsRequest);
/**
*
* Retrieves account settings for the specified Amazon Chime account ID, such as remote control and dialout
* settings. For more information about these settings, see Use the Policies Page in the Amazon Chime
* Administration Guide.
*
*
* @param getAccountSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAccountSettings operation returned by the service.
* @sample AmazonChimeAsyncHandler.GetAccountSettings
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getAccountSettingsAsync(GetAccountSettingsRequest getAccountSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the retention settings for an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetMessagingRetentionSettings, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getAppInstanceRetentionSettingsRequest
* @return A Java Future containing the result of the GetAppInstanceRetentionSettings operation returned by the
* service.
* @sample AmazonChimeAsync.GetAppInstanceRetentionSettings
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future getAppInstanceRetentionSettingsAsync(
GetAppInstanceRetentionSettingsRequest getAppInstanceRetentionSettingsRequest);
/**
*
* Gets the retention settings for an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetMessagingRetentionSettings, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getAppInstanceRetentionSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAppInstanceRetentionSettings operation returned by the
* service.
* @sample AmazonChimeAsyncHandler.GetAppInstanceRetentionSettings
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future getAppInstanceRetentionSettingsAsync(
GetAppInstanceRetentionSettingsRequest getAppInstanceRetentionSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the streaming settings for an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetMessagingStreamingConfigurations, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getAppInstanceStreamingConfigurationsRequest
* @return A Java Future containing the result of the GetAppInstanceStreamingConfigurations operation returned by
* the service.
* @sample AmazonChimeAsync.GetAppInstanceStreamingConfigurations
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future getAppInstanceStreamingConfigurationsAsync(
GetAppInstanceStreamingConfigurationsRequest getAppInstanceStreamingConfigurationsRequest);
/**
*
* Gets the streaming settings for an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetMessagingStreamingConfigurations, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getAppInstanceStreamingConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAppInstanceStreamingConfigurations operation returned by
* the service.
* @sample AmazonChimeAsyncHandler.GetAppInstanceStreamingConfigurations
* @see AWS API Documentation
*/
@Deprecated
java.util.concurrent.Future getAppInstanceStreamingConfigurationsAsync(
GetAppInstanceStreamingConfigurationsRequest getAppInstanceStreamingConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the Amazon Chime SDK attendee details for a specified meeting ID and attendee ID. For more information about
* the Amazon Chime SDK, see Using the
* Amazon Chime SDK in the Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetAttendee,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getAttendeeRequest
* @return A Java Future containing the result of the GetAttendee operation returned by the service.
* @sample AmazonChimeAsync.GetAttendee
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future getAttendeeAsync(GetAttendeeRequest getAttendeeRequest);
/**
*
* Gets the Amazon Chime SDK attendee details for a specified meeting ID and attendee ID. For more information about
* the Amazon Chime SDK, see Using the
* Amazon Chime SDK in the Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetAttendee,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getAttendeeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAttendee operation returned by the service.
* @sample AmazonChimeAsyncHandler.GetAttendee
* @see AWS API
* Documentation
*/
@Deprecated
java.util.concurrent.Future getAttendeeAsync(GetAttendeeRequest getAttendeeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves details for the specified bot, such as bot email address, bot type, status, and display name.
*
*
* @param getBotRequest
* @return A Java Future containing the result of the GetBot operation returned by the service.
* @sample AmazonChimeAsync.GetBot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBotAsync(GetBotRequest getBotRequest);
/**
*
* Retrieves details for the specified bot, such as bot email address, bot type, status, and display name.
*
*
* @param getBotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetBot operation returned by the service.
* @sample AmazonChimeAsyncHandler.GetBot
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getBotAsync(GetBotRequest getBotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the full details of a channel message.
*
*
*