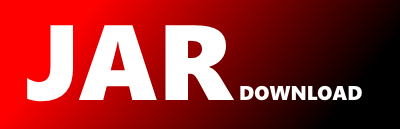
com.amazonaws.services.chime.AmazonChime Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chime Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chime;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.chime.model.*;
/**
* Interface for accessing Amazon Chime.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.chime.AbstractAmazonChime} instead.
*
*
*
*
* Most of these APIs are no longer supported and will not be updated. We recommend using the latest versions in
* the Amazon Chime SDK API
* reference, in the Amazon Chime SDK.
*
*
* Using the latest versions requires migrating to dedicated namespaces. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* The Amazon Chime application programming interface (API) is designed so administrators can perform key tasks, such as
* creating and managing Amazon Chime accounts, users, and Voice Connectors. This guide provides detailed information
* about the Amazon Chime API, including operations, types, inputs and outputs, and error codes.
*
*
* You can use an AWS SDK, the AWS Command Line Interface (AWS CLI), or the REST API to make API calls for Amazon Chime.
* We recommend using an AWS SDK or the AWS CLI. The page for each API action contains a See Also section that
* includes links to information about using the action with a language-specific AWS SDK or the AWS CLI.
*
*
* - Using an AWS SDK
* -
*
* You don't need to write code to calculate a signature for request authentication. The SDK clients authenticate your
* requests by using access keys that you provide. For more information about AWS SDKs, see the AWS Developer Center.
*
*
* - Using the AWS CLI
* -
*
* Use your access keys with the AWS CLI to make API calls. For information about setting up the AWS CLI, see Installing the AWS Command Line Interface
* in the AWS Command Line Interface User Guide. For a list of available Amazon Chime commands, see the Amazon Chime commands in the AWS CLI
* Command Reference.
*
*
* - Using REST APIs
* -
*
* If you use REST to make API calls, you must authenticate your request by providing a signature. Amazon Chime supports
* Signature Version 4. For more information, see Signature Version 4 Signing Process
* in the Amazon Web Services General Reference.
*
*
* When making REST API calls, use the service name chime
and REST endpoint
* https://service.chime.aws.amazon.com
.
*
*
*
*
* Administrative permissions are controlled using AWS Identity and Access Management (IAM). For more information, see
* Identity and Access Management for Amazon
* Chime in the Amazon Chime Administration Guide.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonChime {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "chime";
/**
*
* Associates a phone number with the specified Amazon Chime user.
*
*
* @param associatePhoneNumberWithUserRequest
* @return Result of the AssociatePhoneNumberWithUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.AssociatePhoneNumberWithUser
* @see AWS API Documentation
*/
AssociatePhoneNumberWithUserResult associatePhoneNumberWithUser(AssociatePhoneNumberWithUserRequest associatePhoneNumberWithUserRequest);
/**
*
* Associates phone numbers with the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, AssociatePhoneNumbersWithVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param associatePhoneNumbersWithVoiceConnectorRequest
* @return Result of the AssociatePhoneNumbersWithVoiceConnector operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.AssociatePhoneNumbersWithVoiceConnector
* @see AWS API Documentation
*/
@Deprecated
AssociatePhoneNumbersWithVoiceConnectorResult associatePhoneNumbersWithVoiceConnector(
AssociatePhoneNumbersWithVoiceConnectorRequest associatePhoneNumbersWithVoiceConnectorRequest);
/**
*
* Associates phone numbers with the specified Amazon Chime Voice Connector group.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, AssociatePhoneNumbersWithVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param associatePhoneNumbersWithVoiceConnectorGroupRequest
* @return Result of the AssociatePhoneNumbersWithVoiceConnectorGroup operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.AssociatePhoneNumbersWithVoiceConnectorGroup
* @see AWS API Documentation
*/
@Deprecated
AssociatePhoneNumbersWithVoiceConnectorGroupResult associatePhoneNumbersWithVoiceConnectorGroup(
AssociatePhoneNumbersWithVoiceConnectorGroupRequest associatePhoneNumbersWithVoiceConnectorGroupRequest);
/**
*
* Associates the specified sign-in delegate groups with the specified Amazon Chime account.
*
*
* @param associateSigninDelegateGroupsWithAccountRequest
* @return Result of the AssociateSigninDelegateGroupsWithAccount operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.AssociateSigninDelegateGroupsWithAccount
* @see AWS API Documentation
*/
AssociateSigninDelegateGroupsWithAccountResult associateSigninDelegateGroupsWithAccount(
AssociateSigninDelegateGroupsWithAccountRequest associateSigninDelegateGroupsWithAccountRequest);
/**
*
* Creates up to 100 new attendees for an active Amazon Chime SDK meeting.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* BatchCreateAttendee, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide.
*
*
* @param batchCreateAttendeeRequest
* @return Result of the BatchCreateAttendee operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchCreateAttendee
* @see AWS API
* Documentation
*/
@Deprecated
BatchCreateAttendeeResult batchCreateAttendee(BatchCreateAttendeeRequest batchCreateAttendeeRequest);
/**
*
* Adds a specified number of users to a channel.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, BatchCreateChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param batchCreateChannelMembershipRequest
* @return Result of the BatchCreateChannelMembership operation returned by the service.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @sample AmazonChime.BatchCreateChannelMembership
* @see AWS API Documentation
*/
@Deprecated
BatchCreateChannelMembershipResult batchCreateChannelMembership(BatchCreateChannelMembershipRequest batchCreateChannelMembershipRequest);
/**
*
* Adds up to 50 members to a chat room in an Amazon Chime Enterprise account. Members can be users or bots. The
* member role designates whether the member is a chat room administrator or a general chat room member.
*
*
* @param batchCreateRoomMembershipRequest
* @return Result of the BatchCreateRoomMembership operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchCreateRoomMembership
* @see AWS API Documentation
*/
BatchCreateRoomMembershipResult batchCreateRoomMembership(BatchCreateRoomMembershipRequest batchCreateRoomMembershipRequest);
/**
*
* Moves phone numbers into the Deletion queue. Phone numbers must be disassociated from any users or Amazon
* Chime Voice Connectors before they can be deleted.
*
*
* Phone numbers remain in the Deletion queue for 7 days before they are deleted permanently.
*
*
* @param batchDeletePhoneNumberRequest
* @return Result of the BatchDeletePhoneNumber operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchDeletePhoneNumber
* @see AWS
* API Documentation
*/
BatchDeletePhoneNumberResult batchDeletePhoneNumber(BatchDeletePhoneNumberRequest batchDeletePhoneNumberRequest);
/**
*
* Suspends up to 50 users from a Team
or EnterpriseLWA
Amazon Chime account. For more
* information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* Users suspended from a Team
account are disassociated from the account,but they can continue to use
* Amazon Chime as free users. To remove the suspension from suspended Team
account users, invite them
* to the Team
account again. You can use the InviteUsers action to do so.
*
*
* Users suspended from an EnterpriseLWA
account are immediately signed out of Amazon Chime and can no
* longer sign in. To remove the suspension from suspended EnterpriseLWA
account users, use the
* BatchUnsuspendUser action.
*
*
* To sign out users without suspending them, use the LogoutUser action.
*
*
* @param batchSuspendUserRequest
* @return Result of the BatchSuspendUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchSuspendUser
* @see AWS API
* Documentation
*/
BatchSuspendUserResult batchSuspendUser(BatchSuspendUserRequest batchSuspendUserRequest);
/**
*
* Removes the suspension from up to 50 previously suspended users for the specified Amazon Chime
* EnterpriseLWA
account. Only users on EnterpriseLWA
accounts can be unsuspended using
* this action. For more information about different account types, see Managing Your Amazon Chime Accounts
* in the account types, in the Amazon Chime Administration Guide.
*
*
* Previously suspended users who are unsuspended using this action are returned to Registered
status.
* Users who are not previously suspended are ignored.
*
*
* @param batchUnsuspendUserRequest
* @return Result of the BatchUnsuspendUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchUnsuspendUser
* @see AWS API
* Documentation
*/
BatchUnsuspendUserResult batchUnsuspendUser(BatchUnsuspendUserRequest batchUnsuspendUserRequest);
/**
*
* Updates phone number product types or calling names. You can update one attribute at a time for each
* UpdatePhoneNumberRequestItem
. For example, you can update the product type or the calling name.
*
*
* For toll-free numbers, you cannot use the Amazon Chime Business Calling product type. For numbers outside the
* U.S., you must use the Amazon Chime SIP Media Application Dial-In product type.
*
*
* Updates to outbound calling names can take up to 72 hours to complete. Pending updates to outbound calling names
* must be complete before you can request another update.
*
*
* @param batchUpdatePhoneNumberRequest
* @return Result of the BatchUpdatePhoneNumber operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchUpdatePhoneNumber
* @see AWS
* API Documentation
*/
BatchUpdatePhoneNumberResult batchUpdatePhoneNumber(BatchUpdatePhoneNumberRequest batchUpdatePhoneNumberRequest);
/**
*
* Updates user details within the UpdateUserRequestItem object for up to 20 users for the specified Amazon
* Chime account. Currently, only LicenseType
updates are supported for this action.
*
*
* @param batchUpdateUserRequest
* @return Result of the BatchUpdateUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchUpdateUser
* @see AWS API
* Documentation
*/
BatchUpdateUserResult batchUpdateUser(BatchUpdateUserRequest batchUpdateUserRequest);
/**
*
* Creates an Amazon Chime account under the administrator's AWS account. Only Team
account types are
* currently supported for this action. For more information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* @param createAccountRequest
* @return Result of the CreateAccount operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateAccount
* @see AWS API
* Documentation
*/
CreateAccountResult createAccount(CreateAccountRequest createAccountRequest);
/**
*
* Creates an Amazon Chime SDK messaging AppInstance
under an AWS account. Only SDK messaging customers
* use this API. CreateAppInstance
supports idempotency behavior as described in the AWS API Standard.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateAppInstance, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createAppInstanceRequest
* @return Result of the CreateAppInstance operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateAppInstance
* @see AWS API
* Documentation
*/
@Deprecated
CreateAppInstanceResult createAppInstance(CreateAppInstanceRequest createAppInstanceRequest);
/**
*
* Promotes an AppInstanceUser
to an AppInstanceAdmin
. The promoted user can perform the
* following actions.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateAppInstanceAdmin, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* -
*
* ChannelModerator
actions across all channels in the AppInstance
.
*
*
* -
*
* DeleteChannelMessage
actions.
*
*
*
*
* Only an AppInstanceUser
can be promoted to an AppInstanceAdmin
role.
*
*
* @param createAppInstanceAdminRequest
* @return Result of the CreateAppInstanceAdmin operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateAppInstanceAdmin
* @see AWS
* API Documentation
*/
@Deprecated
CreateAppInstanceAdminResult createAppInstanceAdmin(CreateAppInstanceAdminRequest createAppInstanceAdminRequest);
/**
*
* Creates a user under an Amazon Chime AppInstance
. The request consists of a unique
* appInstanceUserId
and Name
for that user.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createAppInstanceUserRequest
* @return Result of the CreateAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateAppInstanceUser
* @see AWS
* API Documentation
*/
@Deprecated
CreateAppInstanceUserResult createAppInstanceUser(CreateAppInstanceUserRequest createAppInstanceUserRequest);
/**
*
* Creates a new attendee for an active Amazon Chime SDK meeting. For more information about the Amazon Chime SDK,
* see Using the Amazon Chime SDK in
* the Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateAttendee
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createAttendeeRequest
* @return Result of the CreateAttendee operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateAttendee
* @see AWS API
* Documentation
*/
@Deprecated
CreateAttendeeResult createAttendee(CreateAttendeeRequest createAttendeeRequest);
/**
*
* Creates a bot for an Amazon Chime Enterprise account.
*
*
* @param createBotRequest
* @return Result of the CreateBot operation returned by the service.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @sample AmazonChime.CreateBot
* @see AWS API
* Documentation
*/
CreateBotResult createBot(CreateBotRequest createBotRequest);
/**
*
* Creates a channel to which you can add users and send messages.
*
*
* Restriction: You can't change a channel's privacy.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateChannel
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelRequest
* @return Result of the CreateChannel operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateChannel
* @see AWS API
* Documentation
*/
@Deprecated
CreateChannelResult createChannel(CreateChannelRequest createChannelRequest);
/**
*
* Permanently bans a member from a channel. Moderators can't add banned members to a channel. To undo a ban, you
* first have to DeleteChannelBan
, and then CreateChannelMembership
. Bans are cleaned up
* when you delete users or channels.
*
*
* If you ban a user who is already part of a channel, that user is automatically kicked from the channel.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateChannelBan, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelBanRequest
* @return Result of the CreateChannelBan operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateChannelBan
* @see AWS API
* Documentation
*/
@Deprecated
CreateChannelBanResult createChannelBan(CreateChannelBanRequest createChannelBanRequest);
/**
*
* Adds a user to a channel. The InvitedBy
response field is derived from the request header. A channel
* member can:
*
*
* -
*
* List messages
*
*
* -
*
* Send messages
*
*
* -
*
* Receive messages
*
*
* -
*
* Edit their own messages
*
*
* -
*
* Leave the channel
*
*
*
*
* Privacy settings impact this action as follows:
*
*
* -
*
* Public Channels: You do not need to be a member to list messages, but you must be a member to send messages.
*
*
* -
*
* Private Channels: You must be a member to list or send messages.
*
*
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelMembershipRequest
* @return Result of the CreateChannelMembership operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateChannelMembership
* @see AWS
* API Documentation
*/
@Deprecated
CreateChannelMembershipResult createChannelMembership(CreateChannelMembershipRequest createChannelMembershipRequest);
/**
*
* Creates a new ChannelModerator
. A channel moderator can:
*
*
* -
*
* Add and remove other members of the channel.
*
*
* -
*
* Add and remove other moderators of the channel.
*
*
* -
*
* Add and remove user bans for the channel.
*
*
* -
*
* Redact messages in the channel.
*
*
* -
*
* List messages in the channel.
*
*
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateChannelModerator, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelModeratorRequest
* @return Result of the CreateChannelModerator operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateChannelModerator
* @see AWS
* API Documentation
*/
@Deprecated
CreateChannelModeratorResult createChannelModerator(CreateChannelModeratorRequest createChannelModeratorRequest);
/**
*
* Creates a media capture pipeline.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateMediaCapturePipeline, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createMediaCapturePipelineRequest
* @return Result of the CreateMediaCapturePipeline operation returned by the service.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateMediaCapturePipeline
* @see AWS API Documentation
*/
@Deprecated
CreateMediaCapturePipelineResult createMediaCapturePipeline(CreateMediaCapturePipelineRequest createMediaCapturePipelineRequest);
/**
*
* Creates a new Amazon Chime SDK meeting in the specified media Region with no initial attendees. For more
* information about specifying media Regions, see Amazon Chime SDK Media
* Regions in the Amazon Chime SDK Developer Guide . For more information about the Amazon Chime SDK, see
* Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateMeeting
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createMeetingRequest
* @return Result of the CreateMeeting operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateMeeting
* @see AWS API
* Documentation
*/
@Deprecated
CreateMeetingResult createMeeting(CreateMeetingRequest createMeetingRequest);
/**
*
* Uses the join token and call metadata in a meeting request (From number, To number, and so forth) to initiate an
* outbound call to a public switched telephone network (PSTN) and join them into a Chime meeting. Also ensures that
* the From number belongs to the customer.
*
*
* To play welcome audio or implement an interactive voice response (IVR), use the
* CreateSipMediaApplicationCall
action with the corresponding SIP media application ID.
*
*
*
* This API is is not available in a dedicated namespace.
*
*
*
* @param createMeetingDialOutRequest
* @return Result of the CreateMeetingDialOut operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateMeetingDialOut
* @see AWS API
* Documentation
*/
CreateMeetingDialOutResult createMeetingDialOut(CreateMeetingDialOutRequest createMeetingDialOutRequest);
/**
*
* Creates a new Amazon Chime SDK meeting in the specified media Region, with attendees. For more information about
* specifying media Regions, see Amazon Chime SDK Media
* Regions in the Amazon Chime SDK Developer Guide . For more information about the Amazon Chime SDK, see
* Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide .
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateMeetingWithAttendees, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createMeetingWithAttendeesRequest
* @return Result of the CreateMeetingWithAttendees operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateMeetingWithAttendees
* @see AWS API Documentation
*/
@Deprecated
CreateMeetingWithAttendeesResult createMeetingWithAttendees(CreateMeetingWithAttendeesRequest createMeetingWithAttendeesRequest);
/**
*
* Creates an order for phone numbers to be provisioned. For toll-free numbers, you cannot use the Amazon Chime
* Business Calling product type. For numbers outside the U.S., you must use the Amazon Chime SIP Media Application
* Dial-In product type.
*
*
* @param createPhoneNumberOrderRequest
* @return Result of the CreatePhoneNumberOrder operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreatePhoneNumberOrder
* @see AWS
* API Documentation
*/
CreatePhoneNumberOrderResult createPhoneNumberOrder(CreatePhoneNumberOrderRequest createPhoneNumberOrderRequest);
/**
*
* Creates a proxy session on the specified Amazon Chime Voice Connector for the specified participant phone
* numbers.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateProxySession, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createProxySessionRequest
* @return Result of the CreateProxySession operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateProxySession
* @see AWS API
* Documentation
*/
@Deprecated
CreateProxySessionResult createProxySession(CreateProxySessionRequest createProxySessionRequest);
/**
*
* Creates a chat room for the specified Amazon Chime Enterprise account.
*
*
* @param createRoomRequest
* @return Result of the CreateRoom operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateRoom
* @see AWS API
* Documentation
*/
CreateRoomResult createRoom(CreateRoomRequest createRoomRequest);
/**
*
* Adds a member to a chat room in an Amazon Chime Enterprise account. A member can be either a user or a bot. The
* member role designates whether the member is a chat room administrator or a general chat room member.
*
*
* @param createRoomMembershipRequest
* @return Result of the CreateRoomMembership operation returned by the service.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateRoomMembership
* @see AWS API
* Documentation
*/
CreateRoomMembershipResult createRoomMembership(CreateRoomMembershipRequest createRoomMembershipRequest);
/**
*
* Creates a SIP media application.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateSipMediaApplication, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createSipMediaApplicationRequest
* @return Result of the CreateSipMediaApplication operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateSipMediaApplication
* @see AWS API Documentation
*/
@Deprecated
CreateSipMediaApplicationResult createSipMediaApplication(CreateSipMediaApplicationRequest createSipMediaApplicationRequest);
/**
*
* Creates an outbound call to a phone number from the phone number specified in the request, and it invokes the
* endpoint of the specified sipMediaApplicationId
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateSipMediaApplicationCall, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createSipMediaApplicationCallRequest
* @return Result of the CreateSipMediaApplicationCall operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateSipMediaApplicationCall
* @see AWS API Documentation
*/
@Deprecated
CreateSipMediaApplicationCallResult createSipMediaApplicationCall(CreateSipMediaApplicationCallRequest createSipMediaApplicationCallRequest);
/**
*
* Creates a SIP rule which can be used to run a SIP media application as a target for a specific trigger type.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateSipRule,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createSipRuleRequest
* @return Result of the CreateSipRule operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateSipRule
* @see AWS API
* Documentation
*/
@Deprecated
CreateSipRuleResult createSipRule(CreateSipRuleRequest createSipRuleRequest);
/**
*
* Creates a user under the specified Amazon Chime account.
*
*
* @param createUserRequest
* @return Result of the CreateUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateUser
* @see AWS API
* Documentation
*/
CreateUserResult createUser(CreateUserRequest createUserRequest);
/**
*
* Creates an Amazon Chime Voice Connector under the administrator's AWS account. You can choose to create an Amazon
* Chime Voice Connector in a specific AWS Region.
*
*
* Enabling CreateVoiceConnectorRequest$RequireEncryption configures your Amazon Chime Voice Connector to use
* TLS transport for SIP signaling and Secure RTP (SRTP) for media. Inbound calls use TLS transport, and unencrypted
* outbound calls are blocked.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createVoiceConnectorRequest
* @return Result of the CreateVoiceConnector operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateVoiceConnector
* @see AWS API
* Documentation
*/
@Deprecated
CreateVoiceConnectorResult createVoiceConnector(CreateVoiceConnectorRequest createVoiceConnectorRequest);
/**
*
* Creates an Amazon Chime Voice Connector group under the administrator's AWS account. You can associate Amazon
* Chime Voice Connectors with the Amazon Chime Voice Connector group by including VoiceConnectorItems
* in the request.
*
*
* You can include Amazon Chime Voice Connectors from different AWS Regions in your group. This creates a fault
* tolerant mechanism for fallback in case of availability events.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createVoiceConnectorGroupRequest
* @return Result of the CreateVoiceConnectorGroup operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateVoiceConnectorGroup
* @see AWS API Documentation
*/
@Deprecated
CreateVoiceConnectorGroupResult createVoiceConnectorGroup(CreateVoiceConnectorGroupRequest createVoiceConnectorGroupRequest);
/**
*
* Deletes the specified Amazon Chime account. You must suspend all users before deleting Team
account.
* You can use the BatchSuspendUser action to dodo.
*
*
* For EnterpriseLWA
and EnterpriseAD
accounts, you must release the claimed domains for
* your Amazon Chime account before deletion. As soon as you release the domain, all users under that account are
* suspended.
*
*
* Deleted accounts appear in your Disabled
accounts list for 90 days. To restore deleted account from
* your Disabled
accounts list, you must contact AWS Support.
*
*
* After 90 days, deleted accounts are permanently removed from your Disabled
accounts list.
*
*
* @param deleteAccountRequest
* @return Result of the DeleteAccount operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnprocessableEntityException
* The request was well-formed but was unable to be followed due to semantic errors.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteAccount
* @see AWS API
* Documentation
*/
DeleteAccountResult deleteAccount(DeleteAccountRequest deleteAccountRequest);
/**
*
* Deletes an AppInstance
and all associated data asynchronously.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteAppInstance, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceRequest
* @return Result of the DeleteAppInstance operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteAppInstance
* @see AWS API
* Documentation
*/
@Deprecated
DeleteAppInstanceResult deleteAppInstance(DeleteAppInstanceRequest deleteAppInstanceRequest);
/**
*
* Demotes an AppInstanceAdmin
to an AppInstanceUser
. This action does not delete the
* user.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteAppInstanceAdmin, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceAdminRequest
* @return Result of the DeleteAppInstanceAdmin operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteAppInstanceAdmin
* @see AWS
* API Documentation
*/
@Deprecated
DeleteAppInstanceAdminResult deleteAppInstanceAdmin(DeleteAppInstanceAdminRequest deleteAppInstanceAdminRequest);
/**
*
* Deletes the streaming configurations of an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteAppInstanceStreamingConfigurations, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceStreamingConfigurationsRequest
* @return Result of the DeleteAppInstanceStreamingConfigurations operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteAppInstanceStreamingConfigurations
* @see AWS API Documentation
*/
@Deprecated
DeleteAppInstanceStreamingConfigurationsResult deleteAppInstanceStreamingConfigurations(
DeleteAppInstanceStreamingConfigurationsRequest deleteAppInstanceStreamingConfigurationsRequest);
/**
*
* Deletes an AppInstanceUser
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceUserRequest
* @return Result of the DeleteAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteAppInstanceUser
* @see AWS
* API Documentation
*/
@Deprecated
DeleteAppInstanceUserResult deleteAppInstanceUser(DeleteAppInstanceUserRequest deleteAppInstanceUserRequest);
/**
*
* Deletes an attendee from the specified Amazon Chime SDK meeting and deletes their JoinToken
.
* Attendees are automatically deleted when a Amazon Chime SDK meeting is deleted. For more information about the
* Amazon Chime SDK, see Using the
* Amazon Chime SDK in the Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteAttendee
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAttendeeRequest
* @return Result of the DeleteAttendee operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteAttendee
* @see AWS API
* Documentation
*/
@Deprecated
DeleteAttendeeResult deleteAttendee(DeleteAttendeeRequest deleteAttendeeRequest);
/**
*
* Immediately makes a channel and its memberships inaccessible and marks them for deletion. This is an irreversible
* process.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteChannel
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelRequest
* @return Result of the DeleteChannel operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteChannel
* @see AWS API
* Documentation
*/
@Deprecated
DeleteChannelResult deleteChannel(DeleteChannelRequest deleteChannelRequest);
/**
*
* Removes a user from a channel's ban list.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelBan, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelBanRequest
* @return Result of the DeleteChannelBan operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteChannelBan
* @see AWS API
* Documentation
*/
@Deprecated
DeleteChannelBanResult deleteChannelBan(DeleteChannelBanRequest deleteChannelBanRequest);
/**
*
* Removes a member from a channel.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelMembershipRequest
* @return Result of the DeleteChannelMembership operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteChannelMembership
* @see AWS
* API Documentation
*/
@Deprecated
DeleteChannelMembershipResult deleteChannelMembership(DeleteChannelMembershipRequest deleteChannelMembershipRequest);
/**
*
* Deletes a channel message. Only admins can perform this action. Deletion makes messages inaccessible immediately.
* A background process deletes any revisions created by UpdateChannelMessage
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelMessage, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelMessageRequest
* @return Result of the DeleteChannelMessage operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteChannelMessage
* @see AWS API
* Documentation
*/
@Deprecated
DeleteChannelMessageResult deleteChannelMessage(DeleteChannelMessageRequest deleteChannelMessageRequest);
/**
*
* Deletes a channel moderator.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelModerator, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelModeratorRequest
* @return Result of the DeleteChannelModerator operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteChannelModerator
* @see AWS
* API Documentation
*/
@Deprecated
DeleteChannelModeratorResult deleteChannelModerator(DeleteChannelModeratorRequest deleteChannelModeratorRequest);
/**
*
* Deletes the events configuration that allows a bot to receive outgoing events.
*
*
* @param deleteEventsConfigurationRequest
* @return Result of the DeleteEventsConfiguration operation returned by the service.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @sample AmazonChime.DeleteEventsConfiguration
* @see AWS API Documentation
*/
DeleteEventsConfigurationResult deleteEventsConfiguration(DeleteEventsConfigurationRequest deleteEventsConfigurationRequest);
/**
*
* Deletes the media capture pipeline.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteMediaCapturePipeline, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteMediaCapturePipelineRequest
* @return Result of the DeleteMediaCapturePipeline operation returned by the service.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteMediaCapturePipeline
* @see AWS API Documentation
*/
@Deprecated
DeleteMediaCapturePipelineResult deleteMediaCapturePipeline(DeleteMediaCapturePipelineRequest deleteMediaCapturePipelineRequest);
/**
*
* Deletes the specified Amazon Chime SDK meeting. The operation deletes all attendees, disconnects all clients, and
* prevents new clients from joining the meeting. For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteMeeting
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteMeetingRequest
* @return Result of the DeleteMeeting operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteMeeting
* @see AWS API
* Documentation
*/
@Deprecated
DeleteMeetingResult deleteMeeting(DeleteMeetingRequest deleteMeetingRequest);
/**
*
* Moves the specified phone number into the Deletion queue. A phone number must be disassociated from any
* users or Amazon Chime Voice Connectors before it can be deleted.
*
*
* Deleted phone numbers remain in the Deletion queue for 7 days before they are deleted permanently.
*
*
* @param deletePhoneNumberRequest
* @return Result of the DeletePhoneNumber operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeletePhoneNumber
* @see AWS API
* Documentation
*/
DeletePhoneNumberResult deletePhoneNumber(DeletePhoneNumberRequest deletePhoneNumberRequest);
/**
*
* Deletes the specified proxy session from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteProxySession, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteProxySessionRequest
* @return Result of the DeleteProxySession operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteProxySession
* @see AWS API
* Documentation
*/
@Deprecated
DeleteProxySessionResult deleteProxySession(DeleteProxySessionRequest deleteProxySessionRequest);
/**
*
* Deletes a chat room in an Amazon Chime Enterprise account.
*
*
* @param deleteRoomRequest
* @return Result of the DeleteRoom operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteRoom
* @see AWS API
* Documentation
*/
DeleteRoomResult deleteRoom(DeleteRoomRequest deleteRoomRequest);
/**
*
* Removes a member from a chat room in an Amazon Chime Enterprise account.
*
*
* @param deleteRoomMembershipRequest
* @return Result of the DeleteRoomMembership operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteRoomMembership
* @see AWS API
* Documentation
*/
DeleteRoomMembershipResult deleteRoomMembership(DeleteRoomMembershipRequest deleteRoomMembershipRequest);
/**
*
* Deletes a SIP media application.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteSipMediaApplication, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteSipMediaApplicationRequest
* @return Result of the DeleteSipMediaApplication operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteSipMediaApplication
* @see AWS API Documentation
*/
@Deprecated
DeleteSipMediaApplicationResult deleteSipMediaApplication(DeleteSipMediaApplicationRequest deleteSipMediaApplicationRequest);
/**
*
* Deletes a SIP rule. You must disable a SIP rule before you can delete it.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteSipRule,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteSipRuleRequest
* @return Result of the DeleteSipRule operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteSipRule
* @see AWS API
* Documentation
*/
@Deprecated
DeleteSipRuleResult deleteSipRule(DeleteSipRuleRequest deleteSipRuleRequest);
/**
*
* Deletes the specified Amazon Chime Voice Connector. Any phone numbers associated with the Amazon Chime Voice
* Connector must be disassociated from it before it can be deleted.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorRequest
* @return Result of the DeleteVoiceConnector operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnector
* @see AWS API
* Documentation
*/
@Deprecated
DeleteVoiceConnectorResult deleteVoiceConnector(DeleteVoiceConnectorRequest deleteVoiceConnectorRequest);
/**
*
* Deletes the emergency calling configuration details from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorEmergencyCallingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorEmergencyCallingConfigurationRequest
* @return Result of the DeleteVoiceConnectorEmergencyCallingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnectorEmergencyCallingConfiguration
* @see AWS API Documentation
*/
@Deprecated
DeleteVoiceConnectorEmergencyCallingConfigurationResult deleteVoiceConnectorEmergencyCallingConfiguration(
DeleteVoiceConnectorEmergencyCallingConfigurationRequest deleteVoiceConnectorEmergencyCallingConfigurationRequest);
/**
*
* Deletes the specified Amazon Chime Voice Connector group. Any VoiceConnectorItems
and phone numbers
* associated with the group must be removed before it can be deleted.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorGroupRequest
* @return Result of the DeleteVoiceConnectorGroup operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnectorGroup
* @see AWS API Documentation
*/
@Deprecated
DeleteVoiceConnectorGroupResult deleteVoiceConnectorGroup(DeleteVoiceConnectorGroupRequest deleteVoiceConnectorGroupRequest);
/**
*
* Deletes the origination settings for the specified Amazon Chime Voice Connector.
*
*
*
* If emergency calling is configured for the Amazon Chime Voice Connector, it must be deleted prior to deleting the
* origination settings.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorOrigination, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorOriginationRequest
* @return Result of the DeleteVoiceConnectorOrigination operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnectorOrigination
* @see AWS API Documentation
*/
@Deprecated
DeleteVoiceConnectorOriginationResult deleteVoiceConnectorOrigination(DeleteVoiceConnectorOriginationRequest deleteVoiceConnectorOriginationRequest);
/**
*
* Deletes the proxy configuration from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteVoiceProxy, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorProxyRequest
* @return Result of the DeleteVoiceConnectorProxy operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnectorProxy
* @see AWS API Documentation
*/
@Deprecated
DeleteVoiceConnectorProxyResult deleteVoiceConnectorProxy(DeleteVoiceConnectorProxyRequest deleteVoiceConnectorProxyRequest);
/**
*
* Deletes the streaming configuration for the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorStreamingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorStreamingConfigurationRequest
* @return Result of the DeleteVoiceConnectorStreamingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnectorStreamingConfiguration
* @see AWS API Documentation
*/
@Deprecated
DeleteVoiceConnectorStreamingConfigurationResult deleteVoiceConnectorStreamingConfiguration(
DeleteVoiceConnectorStreamingConfigurationRequest deleteVoiceConnectorStreamingConfigurationRequest);
/**
*
* Deletes the termination settings for the specified Amazon Chime Voice Connector.
*
*
*
* If emergency calling is configured for the Amazon Chime Voice Connector, it must be deleted prior to deleting the
* termination settings.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorTermination, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorTerminationRequest
* @return Result of the DeleteVoiceConnectorTermination operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnectorTermination
* @see AWS API Documentation
*/
@Deprecated
DeleteVoiceConnectorTerminationResult deleteVoiceConnectorTermination(DeleteVoiceConnectorTerminationRequest deleteVoiceConnectorTerminationRequest);
/**
*
* Deletes the specified SIP credentials used by your equipment to authenticate during call termination.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorTerminationCredentials, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorTerminationCredentialsRequest
* @return Result of the DeleteVoiceConnectorTerminationCredentials operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnectorTerminationCredentials
* @see AWS API Documentation
*/
@Deprecated
DeleteVoiceConnectorTerminationCredentialsResult deleteVoiceConnectorTerminationCredentials(
DeleteVoiceConnectorTerminationCredentialsRequest deleteVoiceConnectorTerminationCredentialsRequest);
/**
*
* Returns the full details of an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeAppInstance, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeAppInstanceRequest
* @return Result of the DescribeAppInstance operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeAppInstance
* @see AWS API
* Documentation
*/
@Deprecated
DescribeAppInstanceResult describeAppInstance(DescribeAppInstanceRequest describeAppInstanceRequest);
/**
*
* Returns the full details of an AppInstanceAdmin
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeAppInstanceAdmin, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeAppInstanceAdminRequest
* @return Result of the DescribeAppInstanceAdmin operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeAppInstanceAdmin
* @see AWS
* API Documentation
*/
@Deprecated
DescribeAppInstanceAdminResult describeAppInstanceAdmin(DescribeAppInstanceAdminRequest describeAppInstanceAdminRequest);
/**
*
* Returns the full details of an AppInstanceUser
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeAppInstanceUserRequest
* @return Result of the DescribeAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeAppInstanceUser
* @see AWS
* API Documentation
*/
@Deprecated
DescribeAppInstanceUserResult describeAppInstanceUser(DescribeAppInstanceUserRequest describeAppInstanceUserRequest);
/**
*
* Returns the full details of a channel in an Amazon Chime AppInstance
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeChannel, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelRequest
* @return Result of the DescribeChannel operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeChannel
* @see AWS API
* Documentation
*/
@Deprecated
DescribeChannelResult describeChannel(DescribeChannelRequest describeChannelRequest);
/**
*
* Returns the full details of a channel ban.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeChannelBan, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelBanRequest
* @return Result of the DescribeChannelBan operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeChannelBan
* @see AWS API
* Documentation
*/
@Deprecated
DescribeChannelBanResult describeChannelBan(DescribeChannelBanRequest describeChannelBanRequest);
/**
*
* Returns the full details of a user's channel membership.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelMembershipRequest
* @return Result of the DescribeChannelMembership operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeChannelMembership
* @see AWS API Documentation
*/
@Deprecated
DescribeChannelMembershipResult describeChannelMembership(DescribeChannelMembershipRequest describeChannelMembershipRequest);
/**
*
* Returns the details of a channel based on the membership of the specified AppInstanceUser
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelMembershipForAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelMembershipForAppInstanceUserRequest
* @return Result of the DescribeChannelMembershipForAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeChannelMembershipForAppInstanceUser
* @see AWS API Documentation
*/
@Deprecated
DescribeChannelMembershipForAppInstanceUserResult describeChannelMembershipForAppInstanceUser(
DescribeChannelMembershipForAppInstanceUserRequest describeChannelMembershipForAppInstanceUserRequest);
/**
*
* Returns the full details of a channel moderated by the specified AppInstanceUser
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelModeratedByAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelModeratedByAppInstanceUserRequest
* @return Result of the DescribeChannelModeratedByAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeChannelModeratedByAppInstanceUser
* @see AWS API Documentation
*/
@Deprecated
DescribeChannelModeratedByAppInstanceUserResult describeChannelModeratedByAppInstanceUser(
DescribeChannelModeratedByAppInstanceUserRequest describeChannelModeratedByAppInstanceUserRequest);
/**
*
* Returns the full details of a single ChannelModerator.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelModerator, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelModeratorRequest
* @return Result of the DescribeChannelModerator operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeChannelModerator
* @see AWS
* API Documentation
*/
@Deprecated
DescribeChannelModeratorResult describeChannelModerator(DescribeChannelModeratorRequest describeChannelModeratorRequest);
/**
*
* Disassociates the primary provisioned phone number from the specified Amazon Chime user.
*
*
* @param disassociatePhoneNumberFromUserRequest
* @return Result of the DisassociatePhoneNumberFromUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DisassociatePhoneNumberFromUser
* @see AWS API Documentation
*/
DisassociatePhoneNumberFromUserResult disassociatePhoneNumberFromUser(DisassociatePhoneNumberFromUserRequest disassociatePhoneNumberFromUserRequest);
/**
*
* Disassociates the specified phone numbers from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DisassociatePhoneNumbersFromVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param disassociatePhoneNumbersFromVoiceConnectorRequest
* @return Result of the DisassociatePhoneNumbersFromVoiceConnector operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DisassociatePhoneNumbersFromVoiceConnector
* @see AWS API Documentation
*/
@Deprecated
DisassociatePhoneNumbersFromVoiceConnectorResult disassociatePhoneNumbersFromVoiceConnector(
DisassociatePhoneNumbersFromVoiceConnectorRequest disassociatePhoneNumbersFromVoiceConnectorRequest);
/**
*
* Disassociates the specified phone numbers from the specified Amazon Chime Voice Connector group.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DisassociatePhoneNumbersFromVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param disassociatePhoneNumbersFromVoiceConnectorGroupRequest
* @return Result of the DisassociatePhoneNumbersFromVoiceConnectorGroup operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DisassociatePhoneNumbersFromVoiceConnectorGroup
* @see AWS API Documentation
*/
@Deprecated
DisassociatePhoneNumbersFromVoiceConnectorGroupResult disassociatePhoneNumbersFromVoiceConnectorGroup(
DisassociatePhoneNumbersFromVoiceConnectorGroupRequest disassociatePhoneNumbersFromVoiceConnectorGroupRequest);
/**
*
* Disassociates the specified sign-in delegate groups from the specified Amazon Chime account.
*
*
* @param disassociateSigninDelegateGroupsFromAccountRequest
* @return Result of the DisassociateSigninDelegateGroupsFromAccount operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DisassociateSigninDelegateGroupsFromAccount
* @see AWS API Documentation
*/
DisassociateSigninDelegateGroupsFromAccountResult disassociateSigninDelegateGroupsFromAccount(
DisassociateSigninDelegateGroupsFromAccountRequest disassociateSigninDelegateGroupsFromAccountRequest);
/**
*
* Retrieves details for the specified Amazon Chime account, such as account type and supported licenses.
*
*
* @param getAccountRequest
* @return Result of the GetAccount operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetAccount
* @see AWS API
* Documentation
*/
GetAccountResult getAccount(GetAccountRequest getAccountRequest);
/**
*
* Retrieves account settings for the specified Amazon Chime account ID, such as remote control and dialout
* settings. For more information about these settings, see Use the Policies Page in the Amazon Chime
* Administration Guide.
*
*
* @param getAccountSettingsRequest
* @return Result of the GetAccountSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetAccountSettings
* @see AWS API
* Documentation
*/
GetAccountSettingsResult getAccountSettings(GetAccountSettingsRequest getAccountSettingsRequest);
/**
*
* Gets the retention settings for an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetMessagingRetentionSettings, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getAppInstanceRetentionSettingsRequest
* @return Result of the GetAppInstanceRetentionSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetAppInstanceRetentionSettings
* @see AWS API Documentation
*/
@Deprecated
GetAppInstanceRetentionSettingsResult getAppInstanceRetentionSettings(GetAppInstanceRetentionSettingsRequest getAppInstanceRetentionSettingsRequest);
/**
*
* Gets the streaming settings for an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetMessagingStreamingConfigurations, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getAppInstanceStreamingConfigurationsRequest
* @return Result of the GetAppInstanceStreamingConfigurations operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetAppInstanceStreamingConfigurations
* @see AWS API Documentation
*/
@Deprecated
GetAppInstanceStreamingConfigurationsResult getAppInstanceStreamingConfigurations(
GetAppInstanceStreamingConfigurationsRequest getAppInstanceStreamingConfigurationsRequest);
/**
*
* Gets the Amazon Chime SDK attendee details for a specified meeting ID and attendee ID. For more information about
* the Amazon Chime SDK, see Using the
* Amazon Chime SDK in the Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetAttendee,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getAttendeeRequest
* @return Result of the GetAttendee operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetAttendee
* @see AWS API
* Documentation
*/
@Deprecated
GetAttendeeResult getAttendee(GetAttendeeRequest getAttendeeRequest);
/**
*
* Retrieves details for the specified bot, such as bot email address, bot type, status, and display name.
*
*
* @param getBotRequest
* @return Result of the GetBot operation returned by the service.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @sample AmazonChime.GetBot
* @see AWS API
* Documentation
*/
GetBotResult getBot(GetBotRequest getBotRequest);
/**
*
* Gets the full details of a channel message.
*
*
*
* The x-amz-chime-bearer request header is mandatory. Use the AppInstanceUserArn
of the user that
* makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* GetChannelMessage, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getChannelMessageRequest
* @return Result of the GetChannelMessage operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetChannelMessage
* @see AWS API
* Documentation
*/
@Deprecated
GetChannelMessageResult getChannelMessage(GetChannelMessageRequest getChannelMessageRequest);
/**
*
* Gets details for an events configuration that allows a bot to receive outgoing events, such as an HTTPS endpoint
* or Lambda function ARN.
*
*
* @param getEventsConfigurationRequest
* @return Result of the GetEventsConfiguration operation returned by the service.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @sample AmazonChime.GetEventsConfiguration
* @see AWS
* API Documentation
*/
GetEventsConfigurationResult getEventsConfiguration(GetEventsConfigurationRequest getEventsConfigurationRequest);
/**
*
* Retrieves global settings for the administrator's AWS account, such as Amazon Chime Business Calling and Amazon
* Chime Voice Connector settings.
*
*
* @param getGlobalSettingsRequest
* @return Result of the GetGlobalSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetGlobalSettings
* @see AWS API
* Documentation
*/
GetGlobalSettingsResult getGlobalSettings(GetGlobalSettingsRequest getGlobalSettingsRequest);
/**
*
* Gets an existing media capture pipeline.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetMediaCapturePipeline, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getMediaCapturePipelineRequest
* @return Result of the GetMediaCapturePipeline operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetMediaCapturePipeline
* @see AWS
* API Documentation
*/
@Deprecated
GetMediaCapturePipelineResult getMediaCapturePipeline(GetMediaCapturePipelineRequest getMediaCapturePipelineRequest);
/**
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetMeeting, in
* the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* Gets the Amazon Chime SDK meeting details for the specified meeting ID. For more information about the Amazon
* Chime SDK, see Using the Amazon Chime
* SDK in the Amazon Chime SDK Developer Guide .
*
*
* @param getMeetingRequest
* @return Result of the GetMeeting operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetMeeting
* @see AWS API
* Documentation
*/
@Deprecated
GetMeetingResult getMeeting(GetMeetingRequest getMeetingRequest);
/**
*
* The details of the endpoint for the messaging session.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetMessagingSessionEndpoint, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getMessagingSessionEndpointRequest
* @return Result of the GetMessagingSessionEndpoint operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetMessagingSessionEndpoint
* @see AWS API Documentation
*/
@Deprecated
GetMessagingSessionEndpointResult getMessagingSessionEndpoint(GetMessagingSessionEndpointRequest getMessagingSessionEndpointRequest);
/**
*
* Retrieves details for the specified phone number ID, such as associations, capabilities, and product type.
*
*
* @param getPhoneNumberRequest
* @return Result of the GetPhoneNumber operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetPhoneNumber
* @see AWS API
* Documentation
*/
GetPhoneNumberResult getPhoneNumber(GetPhoneNumberRequest getPhoneNumberRequest);
/**
*
* Retrieves details for the specified phone number order, such as the order creation timestamp, phone numbers in
* E.164 format, product type, and order status.
*
*
* @param getPhoneNumberOrderRequest
* @return Result of the GetPhoneNumberOrder operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetPhoneNumberOrder
* @see AWS API
* Documentation
*/
GetPhoneNumberOrderResult getPhoneNumberOrder(GetPhoneNumberOrderRequest getPhoneNumberOrderRequest);
/**
*
* Retrieves the phone number settings for the administrator's AWS account, such as the default outbound calling
* name.
*
*
* @param getPhoneNumberSettingsRequest
* @return Result of the GetPhoneNumberSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetPhoneNumberSettings
* @see AWS
* API Documentation
*/
GetPhoneNumberSettingsResult getPhoneNumberSettings(GetPhoneNumberSettingsRequest getPhoneNumberSettingsRequest);
/**
*
* Gets the specified proxy session details for the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetProxySession
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getProxySessionRequest
* @return Result of the GetProxySession operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetProxySession
* @see AWS API
* Documentation
*/
@Deprecated
GetProxySessionResult getProxySession(GetProxySessionRequest getProxySessionRequest);
/**
*
* Gets the retention settings for the specified Amazon Chime Enterprise account. For more information about
* retention settings, see Managing Chat
* Retention Policies in the Amazon Chime Administration Guide.
*
*
* @param getRetentionSettingsRequest
* @return Result of the GetRetentionSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetRetentionSettings
* @see AWS API
* Documentation
*/
GetRetentionSettingsResult getRetentionSettings(GetRetentionSettingsRequest getRetentionSettingsRequest);
/**
*
* Retrieves room details, such as the room name, for a room in an Amazon Chime Enterprise account.
*
*
* @param getRoomRequest
* @return Result of the GetRoom operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetRoom
* @see AWS API
* Documentation
*/
GetRoomResult getRoom(GetRoomRequest getRoomRequest);
/**
*
* Retrieves the information for a SIP media application, including name, AWS Region, and endpoints.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* GetSipMediaApplication, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getSipMediaApplicationRequest
* @return Result of the GetSipMediaApplication operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetSipMediaApplication
* @see AWS
* API Documentation
*/
@Deprecated
GetSipMediaApplicationResult getSipMediaApplication(GetSipMediaApplicationRequest getSipMediaApplicationRequest);
/**
*
* Returns the logging configuration for the specified SIP media application.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetSipMediaApplicationLoggingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getSipMediaApplicationLoggingConfigurationRequest
* @return Result of the GetSipMediaApplicationLoggingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetSipMediaApplicationLoggingConfiguration
* @see AWS API Documentation
*/
@Deprecated
GetSipMediaApplicationLoggingConfigurationResult getSipMediaApplicationLoggingConfiguration(
GetSipMediaApplicationLoggingConfigurationRequest getSipMediaApplicationLoggingConfigurationRequest);
/**
*
* Retrieves the details of a SIP rule, such as the rule ID, name, triggers, and target endpoints.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetSipRule,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getSipRuleRequest
* @return Result of the GetSipRule operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetSipRule
* @see AWS API
* Documentation
*/
@Deprecated
GetSipRuleResult getSipRule(GetSipRuleRequest getSipRuleRequest);
/**
*
* Retrieves details for the specified user ID, such as primary email address, license type,and personal meeting
* PIN.
*
*
* To retrieve user details with an email address instead of a user ID, use the ListUsers action, and then
* filter by email address.
*
*
* @param getUserRequest
* @return Result of the GetUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetUser
* @see AWS API
* Documentation
*/
GetUserResult getUser(GetUserRequest getUserRequest);
/**
*
* Retrieves settings for the specified user ID, such as any associated phone number settings.
*
*
* @param getUserSettingsRequest
* @return Result of the GetUserSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetUserSettings
* @see AWS API
* Documentation
*/
GetUserSettingsResult getUserSettings(GetUserSettingsRequest getUserSettingsRequest);
/**
*
* Retrieves details for the specified Amazon Chime Voice Connector, such as timestamps,name, outbound host, and
* encryption requirements.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* GetVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getVoiceConnectorRequest
* @return Result of the GetVoiceConnector operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetVoiceConnector
* @see AWS API
* Documentation
*/
@Deprecated
GetVoiceConnectorResult getVoiceConnector(GetVoiceConnectorRequest getVoiceConnectorRequest);
/**
*
* Gets the emergency calling configuration details for the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetVoiceConnectorEmergencyCallingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getVoiceConnectorEmergencyCallingConfigurationRequest
* @return Result of the GetVoiceConnectorEmergencyCallingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetVoiceConnectorEmergencyCallingConfiguration
* @see AWS API Documentation
*/
@Deprecated
GetVoiceConnectorEmergencyCallingConfigurationResult getVoiceConnectorEmergencyCallingConfiguration(
GetVoiceConnectorEmergencyCallingConfigurationRequest getVoiceConnectorEmergencyCallingConfigurationRequest);
/**
*
* Retrieves details for the specified Amazon Chime Voice Connector group, such as timestamps,name, and associated
* VoiceConnectorItems
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* GetVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getVoiceConnectorGroupRequest
* @return Result of the GetVoiceConnectorGroup operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetVoiceConnectorGroup
* @see AWS
* API Documentation
*/
@Deprecated
GetVoiceConnectorGroupResult getVoiceConnectorGroup(GetVoiceConnectorGroupRequest getVoiceConnectorGroupRequest);
/**
*
* Retrieves the logging configuration details for the specified Amazon Chime Voice Connector. Shows whether SIP
* message logs are enabled for sending to Amazon CloudWatch Logs.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetVoiceConnectorLoggingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getVoiceConnectorLoggingConfigurationRequest
* @return Result of the GetVoiceConnectorLoggingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetVoiceConnectorLoggingConfiguration
* @see AWS API Documentation
*/
@Deprecated
GetVoiceConnectorLoggingConfigurationResult getVoiceConnectorLoggingConfiguration(
GetVoiceConnectorLoggingConfigurationRequest getVoiceConnectorLoggingConfigurationRequest);
/**
*
* Retrieves origination setting details for the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetVoiceConnectorOrigination, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getVoiceConnectorOriginationRequest
* @return Result of the GetVoiceConnectorOrigination operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetVoiceConnectorOrigination
* @see AWS API Documentation
*/
@Deprecated
GetVoiceConnectorOriginationResult getVoiceConnectorOrigination(GetVoiceConnectorOriginationRequest getVoiceConnectorOriginationRequest);
/**
*
* Gets the proxy configuration details for the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* GetVoiceConnectorProxy, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getVoiceConnectorProxyRequest
* @return Result of the GetVoiceConnectorProxy operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetVoiceConnectorProxy
* @see AWS
* API Documentation
*/
@Deprecated
GetVoiceConnectorProxyResult getVoiceConnectorProxy(GetVoiceConnectorProxyRequest getVoiceConnectorProxyRequest);
/**
*
* Retrieves the streaming configuration details for the specified Amazon Chime Voice Connector. Shows whether media
* streaming is enabled for sending to Amazon Kinesis. It also shows the retention period, in hours, for the Amazon
* Kinesis data.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetVoiceConnectorStreamingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getVoiceConnectorStreamingConfigurationRequest
* @return Result of the GetVoiceConnectorStreamingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetVoiceConnectorStreamingConfiguration
* @see AWS API Documentation
*/
@Deprecated
GetVoiceConnectorStreamingConfigurationResult getVoiceConnectorStreamingConfiguration(
GetVoiceConnectorStreamingConfigurationRequest getVoiceConnectorStreamingConfigurationRequest);
/**
*
* Retrieves termination setting details for the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetVoiceConnectorTermination, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getVoiceConnectorTerminationRequest
* @return Result of the GetVoiceConnectorTermination operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetVoiceConnectorTermination
* @see AWS API Documentation
*/
@Deprecated
GetVoiceConnectorTerminationResult getVoiceConnectorTermination(GetVoiceConnectorTerminationRequest getVoiceConnectorTerminationRequest);
/**
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetVoiceConnectorTerminationHealth, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* Retrieves information about the last time a SIP OPTIONS
ping was received from your SIP
* infrastructure for the specified Amazon Chime Voice Connector.
*
*
* @param getVoiceConnectorTerminationHealthRequest
* @return Result of the GetVoiceConnectorTerminationHealth operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetVoiceConnectorTerminationHealth
* @see AWS API Documentation
*/
@Deprecated
GetVoiceConnectorTerminationHealthResult getVoiceConnectorTerminationHealth(
GetVoiceConnectorTerminationHealthRequest getVoiceConnectorTerminationHealthRequest);
/**
*
* Sends email to a maximum of 50 users, inviting them to the specified Amazon Chime Team
account. Only
* Team
account types are currently supported for this action.
*
*
* @param inviteUsersRequest
* @return Result of the InviteUsers operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.InviteUsers
* @see AWS API
* Documentation
*/
InviteUsersResult inviteUsers(InviteUsersRequest inviteUsersRequest);
/**
*
* Lists the Amazon Chime accounts under the administrator's AWS account. You can filter accounts by account name
* prefix. To find out which Amazon Chime account a user belongs to, you can filter by the user's email address,
* which returns one account result.
*
*
* @param listAccountsRequest
* @return Result of the ListAccounts operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListAccounts
* @see AWS API
* Documentation
*/
ListAccountsResult listAccounts(ListAccountsRequest listAccountsRequest);
/**
*
* Returns a list of the administrators in the AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* ListAppInstanceAdmins, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listAppInstanceAdminsRequest
* @return Result of the ListAppInstanceAdmins operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListAppInstanceAdmins
* @see AWS
* API Documentation
*/
@Deprecated
ListAppInstanceAdminsResult listAppInstanceAdmins(ListAppInstanceAdminsRequest listAppInstanceAdminsRequest);
/**
*
* List all AppInstanceUsers
created under a single AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* ListAppInstanceUsers, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listAppInstanceUsersRequest
* @return Result of the ListAppInstanceUsers operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListAppInstanceUsers
* @see AWS API
* Documentation
*/
@Deprecated
ListAppInstanceUsersResult listAppInstanceUsers(ListAppInstanceUsersRequest listAppInstanceUsersRequest);
/**
*
* Lists all Amazon Chime AppInstance
s created under a single AWS account.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* ListAppInstances, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listAppInstancesRequest
* @return Result of the ListAppInstances operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListAppInstances
* @see AWS API
* Documentation
*/
@Deprecated
ListAppInstancesResult listAppInstances(ListAppInstancesRequest listAppInstancesRequest);
/**
*
* Lists the tags applied to an Amazon Chime SDK attendee resource.
*
*
*
* ListAttendeeTags is not supported in the Amazon Chime SDK Meetings Namespace. Update your application to remove
* calls to this API.
*
*
*
* @param listAttendeeTagsRequest
* @return Result of the ListAttendeeTags operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListAttendeeTags
* @see AWS API
* Documentation
*/
@Deprecated
ListAttendeeTagsResult listAttendeeTags(ListAttendeeTagsRequest listAttendeeTagsRequest);
/**
*
* Lists the attendees for the specified Amazon Chime SDK meeting. For more information about the Amazon Chime SDK,
* see Using the Amazon Chime SDK in
* the Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, ListAttendees
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listAttendeesRequest
* @return Result of the ListAttendees operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListAttendees
* @see AWS API
* Documentation
*/
@Deprecated
ListAttendeesResult listAttendees(ListAttendeesRequest listAttendeesRequest);
/**
*
* Lists the bots associated with the administrator's Amazon Chime Enterprise account ID.
*
*
* @param listBotsRequest
* @return Result of the ListBots operation returned by the service.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @sample AmazonChime.ListBots
* @see AWS API
* Documentation
*/
ListBotsResult listBots(ListBotsRequest listBotsRequest);
/**
*
* Lists all the users banned from a particular channel.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* ListChannelBans, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listChannelBansRequest
* @return Result of the ListChannelBans operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListChannelBans
* @see AWS API
* Documentation
*/
@Deprecated
ListChannelBansResult listChannelBans(ListChannelBansRequest listChannelBansRequest);
/**
*
* Lists all channel memberships in a channel.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* ListChannelMemberships, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listChannelMembershipsRequest
* @return Result of the ListChannelMemberships operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListChannelMemberships
* @see AWS
* API Documentation
*/
@Deprecated
ListChannelMembershipsResult listChannelMemberships(ListChannelMembershipsRequest listChannelMembershipsRequest);
/**
*
* Lists all channels that a particular AppInstanceUser
is a part of. Only an
* AppInstanceAdmin
can call the API with a user ARN that is not their own.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, ListChannelMembershipsForAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listChannelMembershipsForAppInstanceUserRequest
* @return Result of the ListChannelMembershipsForAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListChannelMembershipsForAppInstanceUser
* @see AWS API Documentation
*/
@Deprecated
ListChannelMembershipsForAppInstanceUserResult listChannelMembershipsForAppInstanceUser(
ListChannelMembershipsForAppInstanceUserRequest listChannelMembershipsForAppInstanceUserRequest);
/**
*
* List all the messages in a channel. Returns a paginated list of ChannelMessages
. By default, sorted
* by creation timestamp in descending order.
*
*
*
* Redacted messages appear in the results as empty, since they are only redacted, not deleted. Deleted messages do
* not appear in the results. This action always returns the latest version of an edited message.
*
*
* Also, the x-amz-chime-bearer request header is mandatory. Use the AppInstanceUserArn
of the user
* that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* ListChannelMessages, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listChannelMessagesRequest
* @return Result of the ListChannelMessages operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListChannelMessages
* @see AWS API
* Documentation
*/
@Deprecated
ListChannelMessagesResult listChannelMessages(ListChannelMessagesRequest listChannelMessagesRequest);
/**
*
* Lists all the moderators for a channel.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* ListChannelModerators, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listChannelModeratorsRequest
* @return Result of the ListChannelModerators operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListChannelModerators
* @see AWS
* API Documentation
*/
@Deprecated
ListChannelModeratorsResult listChannelModerators(ListChannelModeratorsRequest listChannelModeratorsRequest);
/**
*
* Lists all Channels created under a single Chime App as a paginated list. You can specify filters to narrow
* results.
*
*
* Functionality & restrictions
*
*
* -
*
* Use privacy = PUBLIC
to retrieve all public channels in the account.
*
*
* -
*
* Only an AppInstanceAdmin
can set privacy = PRIVATE
to list the private channels in an
* account.
*
*
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, ListChannels
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listChannelsRequest
* @return Result of the ListChannels operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListChannels
* @see AWS API
* Documentation
*/
@Deprecated
ListChannelsResult listChannels(ListChannelsRequest listChannelsRequest);
/**
*
* A list of the channels moderated by an AppInstanceUser
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, ListChannelsModeratedByAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listChannelsModeratedByAppInstanceUserRequest
* @return Result of the ListChannelsModeratedByAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListChannelsModeratedByAppInstanceUser
* @see AWS API Documentation
*/
@Deprecated
ListChannelsModeratedByAppInstanceUserResult listChannelsModeratedByAppInstanceUser(
ListChannelsModeratedByAppInstanceUserRequest listChannelsModeratedByAppInstanceUserRequest);
/**
*
* Returns a list of media capture pipelines.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, ListMediaCapturePipelines, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listMediaCapturePipelinesRequest
* @return Result of the ListMediaCapturePipelines operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListMediaCapturePipelines
* @see AWS API Documentation
*/
@Deprecated
ListMediaCapturePipelinesResult listMediaCapturePipelines(ListMediaCapturePipelinesRequest listMediaCapturePipelinesRequest);
/**
*
* Lists the tags applied to an Amazon Chime SDK meeting resource.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* ListTagsForResource, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listMeetingTagsRequest
* @return Result of the ListMeetingTags operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListMeetingTags
* @see AWS API
* Documentation
*/
@Deprecated
ListMeetingTagsResult listMeetingTags(ListMeetingTagsRequest listMeetingTagsRequest);
/**
*
* Lists up to 100 active Amazon Chime SDK meetings.
*
*
*
* ListMeetings is not supported in the Amazon Chime SDK Meetings Namespace. Update your application to remove calls
* to this API.
*
*
*
* For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide.
*
*
* @param listMeetingsRequest
* @return Result of the ListMeetings operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListMeetings
* @see AWS API
* Documentation
*/
@Deprecated
ListMeetingsResult listMeetings(ListMeetingsRequest listMeetingsRequest);
/**
*
* Lists the phone number orders for the administrator's Amazon Chime account.
*
*
* @param listPhoneNumberOrdersRequest
* @return Result of the ListPhoneNumberOrders operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListPhoneNumberOrders
* @see AWS
* API Documentation
*/
ListPhoneNumberOrdersResult listPhoneNumberOrders(ListPhoneNumberOrdersRequest listPhoneNumberOrdersRequest);
/**
*
* Lists the phone numbers for the specified Amazon Chime account, Amazon Chime user, Amazon Chime Voice Connector,
* or Amazon Chime Voice Connector group.
*
*
* @param listPhoneNumbersRequest
* @return Result of the ListPhoneNumbers operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListPhoneNumbers
* @see AWS API
* Documentation
*/
ListPhoneNumbersResult listPhoneNumbers(ListPhoneNumbersRequest listPhoneNumbersRequest);
/**
*
* Lists the proxy sessions for the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* ListProxySessions, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listProxySessionsRequest
* @return Result of the ListProxySessions operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListProxySessions
* @see AWS API
* Documentation
*/
@Deprecated
ListProxySessionsResult listProxySessions(ListProxySessionsRequest listProxySessionsRequest);
/**
*
* Lists the membership details for the specified room in an Amazon Chime Enterprise account, such as the members'
* IDs, email addresses, and names.
*
*
* @param listRoomMembershipsRequest
* @return Result of the ListRoomMemberships operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListRoomMemberships
* @see AWS API
* Documentation
*/
ListRoomMembershipsResult listRoomMemberships(ListRoomMembershipsRequest listRoomMembershipsRequest);
/**
*
* Lists the room details for the specified Amazon Chime Enterprise account. Optionally, filter the results by a
* member ID (user ID or bot ID) to see a list of rooms that the member belongs to.
*
*
* @param listRoomsRequest
* @return Result of the ListRooms operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListRooms
* @see AWS API
* Documentation
*/
ListRoomsResult listRooms(ListRoomsRequest listRoomsRequest);
/**
*
* Lists the SIP media applications under the administrator's AWS account.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* ListSipMediaApplications, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listSipMediaApplicationsRequest
* @return Result of the ListSipMediaApplications operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListSipMediaApplications
* @see AWS
* API Documentation
*/
@Deprecated
ListSipMediaApplicationsResult listSipMediaApplications(ListSipMediaApplicationsRequest listSipMediaApplicationsRequest);
/**
*
* Lists the SIP rules under the administrator's AWS account.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, ListSipRules,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listSipRulesRequest
* @return Result of the ListSipRules operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListSipRules
* @see AWS API
* Documentation
*/
@Deprecated
ListSipRulesResult listSipRules(ListSipRulesRequest listSipRulesRequest);
/**
*
* Lists supported phone number countries.
*
*
* @param listSupportedPhoneNumberCountriesRequest
* @return Result of the ListSupportedPhoneNumberCountries operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListSupportedPhoneNumberCountries
* @see AWS API Documentation
*/
ListSupportedPhoneNumberCountriesResult listSupportedPhoneNumberCountries(ListSupportedPhoneNumberCountriesRequest listSupportedPhoneNumberCountriesRequest);
/**
*
* Lists the tags applied to an Amazon Chime SDK meeting and messaging resources.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the applicable latest
* version in the Amazon Chime SDK.
*
*
* -
*
* For meetings: ListTagsForResource.
*
*
* -
*
* For messaging: ListTagsForResource.
*
*
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListTagsForResource
* @see AWS API
* Documentation
*/
@Deprecated
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the users that belong to the specified Amazon Chime account. You can specify an email address to list only
* the user that the email address belongs to.
*
*
* @param listUsersRequest
* @return Result of the ListUsers operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListUsers
* @see AWS API
* Documentation
*/
ListUsersResult listUsers(ListUsersRequest listUsersRequest);
/**
*
* Lists the Amazon Chime Voice Connector groups for the administrator's AWS account.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* ListVoiceConnectorGroups, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listVoiceConnectorGroupsRequest
* @return Result of the ListVoiceConnectorGroups operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListVoiceConnectorGroups
* @see AWS
* API Documentation
*/
@Deprecated
ListVoiceConnectorGroupsResult listVoiceConnectorGroups(ListVoiceConnectorGroupsRequest listVoiceConnectorGroupsRequest);
/**
*
* Lists the SIP credentials for the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, ListVoiceConnectorTerminationCredentials, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listVoiceConnectorTerminationCredentialsRequest
* @return Result of the ListVoiceConnectorTerminationCredentials operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListVoiceConnectorTerminationCredentials
* @see AWS API Documentation
*/
@Deprecated
ListVoiceConnectorTerminationCredentialsResult listVoiceConnectorTerminationCredentials(
ListVoiceConnectorTerminationCredentialsRequest listVoiceConnectorTerminationCredentialsRequest);
/**
*
* Lists the Amazon Chime Voice Connectors for the administrator's AWS account.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* ListVoiceConnectors, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param listVoiceConnectorsRequest
* @return Result of the ListVoiceConnectors operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ListVoiceConnectors
* @see AWS API
* Documentation
*/
@Deprecated
ListVoiceConnectorsResult listVoiceConnectors(ListVoiceConnectorsRequest listVoiceConnectorsRequest);
/**
*
* Logs out the specified user from all of the devices they are currently logged into.
*
*
* @param logoutUserRequest
* @return Result of the LogoutUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.LogoutUser
* @see AWS API
* Documentation
*/
LogoutUserResult logoutUser(LogoutUserRequest logoutUserRequest);
/**
*
* Sets the amount of time in days that a given AppInstance
retains data.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, PutAppInstanceRetentionSettings, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param putAppInstanceRetentionSettingsRequest
* @return Result of the PutAppInstanceRetentionSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.PutAppInstanceRetentionSettings
* @see AWS API Documentation
*/
@Deprecated
PutAppInstanceRetentionSettingsResult putAppInstanceRetentionSettings(PutAppInstanceRetentionSettingsRequest putAppInstanceRetentionSettingsRequest);
/**
*
* The data streaming configurations of an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, PutMessagingStreamingConfigurations, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param putAppInstanceStreamingConfigurationsRequest
* @return Result of the PutAppInstanceStreamingConfigurations operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.PutAppInstanceStreamingConfigurations
* @see AWS API Documentation
*/
@Deprecated
PutAppInstanceStreamingConfigurationsResult putAppInstanceStreamingConfigurations(
PutAppInstanceStreamingConfigurationsRequest putAppInstanceStreamingConfigurationsRequest);
/**
*
* Creates an events configuration that allows a bot to receive outgoing events sent by Amazon Chime. Choose either
* an HTTPS endpoint or a Lambda function ARN. For more information, see Bot.
*
*
* @param putEventsConfigurationRequest
* @return Result of the PutEventsConfiguration operation returned by the service.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @sample AmazonChime.PutEventsConfiguration
* @see AWS
* API Documentation
*/
PutEventsConfigurationResult putEventsConfiguration(PutEventsConfigurationRequest putEventsConfigurationRequest);
/**
*
* Puts retention settings for the specified Amazon Chime Enterprise account. We recommend using AWS CloudTrail to
* monitor usage of this API for your account. For more information, see Logging Amazon Chime API Calls with AWS
* CloudTrail in the Amazon Chime Administration Guide.
*
*
* To turn off existing retention settings, remove the number of days from the corresponding RetentionDays
* field in the RetentionSettings object. For more information about retention settings, see Managing Chat Retention Policies in
* the Amazon Chime Administration Guide.
*
*
* @param putRetentionSettingsRequest
* @return Result of the PutRetentionSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.PutRetentionSettings
* @see AWS API
* Documentation
*/
PutRetentionSettingsResult putRetentionSettings(PutRetentionSettingsRequest putRetentionSettingsRequest);
/**
*
* Updates the logging configuration for the specified SIP media application.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, PutSipMediaApplicationLoggingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param putSipMediaApplicationLoggingConfigurationRequest
* @return Result of the PutSipMediaApplicationLoggingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.PutSipMediaApplicationLoggingConfiguration
* @see AWS API Documentation
*/
@Deprecated
PutSipMediaApplicationLoggingConfigurationResult putSipMediaApplicationLoggingConfiguration(
PutSipMediaApplicationLoggingConfigurationRequest putSipMediaApplicationLoggingConfigurationRequest);
/**
*
* Puts emergency calling configuration details to the specified Amazon Chime Voice Connector, such as emergency
* phone numbers and calling countries. Origination and termination settings must be enabled for the Amazon Chime
* Voice Connector before emergency calling can be configured.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, PutVoiceConnectorEmergencyCallingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param putVoiceConnectorEmergencyCallingConfigurationRequest
* @return Result of the PutVoiceConnectorEmergencyCallingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.PutVoiceConnectorEmergencyCallingConfiguration
* @see AWS API Documentation
*/
@Deprecated
PutVoiceConnectorEmergencyCallingConfigurationResult putVoiceConnectorEmergencyCallingConfiguration(
PutVoiceConnectorEmergencyCallingConfigurationRequest putVoiceConnectorEmergencyCallingConfigurationRequest);
/**
*
* Adds a logging configuration for the specified Amazon Chime Voice Connector. The logging configuration specifies
* whether SIP message logs are enabled for sending to Amazon CloudWatch Logs.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, PutVoiceConnectorLoggingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param putVoiceConnectorLoggingConfigurationRequest
* @return Result of the PutVoiceConnectorLoggingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.PutVoiceConnectorLoggingConfiguration
* @see AWS API Documentation
*/
@Deprecated
PutVoiceConnectorLoggingConfigurationResult putVoiceConnectorLoggingConfiguration(
PutVoiceConnectorLoggingConfigurationRequest putVoiceConnectorLoggingConfigurationRequest);
/**
*
* Adds origination settings for the specified Amazon Chime Voice Connector.
*
*
*
* If emergency calling is configured for the Amazon Chime Voice Connector, it must be deleted prior to turning off
* origination settings.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, PutVoiceConnectorOrigination, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param putVoiceConnectorOriginationRequest
* @return Result of the PutVoiceConnectorOrigination operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.PutVoiceConnectorOrigination
* @see AWS API Documentation
*/
@Deprecated
PutVoiceConnectorOriginationResult putVoiceConnectorOrigination(PutVoiceConnectorOriginationRequest putVoiceConnectorOriginationRequest);
/**
*
* Puts the specified proxy configuration to the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* PutVoiceConnectorProxy, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param putVoiceConnectorProxyRequest
* @return Result of the PutVoiceConnectorProxy operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.PutVoiceConnectorProxy
* @see AWS
* API Documentation
*/
@Deprecated
PutVoiceConnectorProxyResult putVoiceConnectorProxy(PutVoiceConnectorProxyRequest putVoiceConnectorProxyRequest);
/**
*
* Adds a streaming configuration for the specified Amazon Chime Voice Connector. The streaming configuration
* specifies whether media streaming is enabled for sending to Kinesis. It also sets the retention period, in hours,
* for the Amazon Kinesis data.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, PutVoiceConnectorStreamingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param putVoiceConnectorStreamingConfigurationRequest
* @return Result of the PutVoiceConnectorStreamingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.PutVoiceConnectorStreamingConfiguration
* @see AWS API Documentation
*/
@Deprecated
PutVoiceConnectorStreamingConfigurationResult putVoiceConnectorStreamingConfiguration(
PutVoiceConnectorStreamingConfigurationRequest putVoiceConnectorStreamingConfigurationRequest);
/**
*
* Adds termination settings for the specified Amazon Chime Voice Connector.
*
*
*
* If emergency calling is configured for the Amazon Chime Voice Connector, it must be deleted prior to turning off
* termination settings.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, PutVoiceConnectorTermination, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param putVoiceConnectorTerminationRequest
* @return Result of the PutVoiceConnectorTermination operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.PutVoiceConnectorTermination
* @see AWS API Documentation
*/
@Deprecated
PutVoiceConnectorTerminationResult putVoiceConnectorTermination(PutVoiceConnectorTerminationRequest putVoiceConnectorTerminationRequest);
/**
*
* Adds termination SIP credentials for the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, PutVoiceConnectorTerminationCredentials, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param putVoiceConnectorTerminationCredentialsRequest
* @return Result of the PutVoiceConnectorTerminationCredentials operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.PutVoiceConnectorTerminationCredentials
* @see AWS API Documentation
*/
@Deprecated
PutVoiceConnectorTerminationCredentialsResult putVoiceConnectorTerminationCredentials(
PutVoiceConnectorTerminationCredentialsRequest putVoiceConnectorTerminationCredentialsRequest);
/**
*
* Redacts message content, but not metadata. The message exists in the back end, but the action returns null
* content, and the state shows as redacted.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* RedactChannelMessage, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param redactChannelMessageRequest
* @return Result of the RedactChannelMessage operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.RedactChannelMessage
* @see AWS API
* Documentation
*/
@Deprecated
RedactChannelMessageResult redactChannelMessage(RedactChannelMessageRequest redactChannelMessageRequest);
/**
*
* Redacts the specified message from the specified Amazon Chime conversation.
*
*
* @param redactConversationMessageRequest
* @return Result of the RedactConversationMessage operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.RedactConversationMessage
* @see AWS API Documentation
*/
RedactConversationMessageResult redactConversationMessage(RedactConversationMessageRequest redactConversationMessageRequest);
/**
*
* Redacts the specified message from the specified Amazon Chime channel.
*
*
* @param redactRoomMessageRequest
* @return Result of the RedactRoomMessage operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.RedactRoomMessage
* @see AWS API
* Documentation
*/
RedactRoomMessageResult redactRoomMessage(RedactRoomMessageRequest redactRoomMessageRequest);
/**
*
* Regenerates the security token for a bot.
*
*
* @param regenerateSecurityTokenRequest
* @return Result of the RegenerateSecurityToken operation returned by the service.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @sample AmazonChime.RegenerateSecurityToken
* @see AWS
* API Documentation
*/
RegenerateSecurityTokenResult regenerateSecurityToken(RegenerateSecurityTokenRequest regenerateSecurityTokenRequest);
/**
*
* Resets the personal meeting PIN for the specified user on an Amazon Chime account. Returns the User object
* with the updated personal meeting PIN.
*
*
* @param resetPersonalPINRequest
* @return Result of the ResetPersonalPIN operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ResetPersonalPIN
* @see AWS API
* Documentation
*/
ResetPersonalPINResult resetPersonalPIN(ResetPersonalPINRequest resetPersonalPINRequest);
/**
*
* Moves a phone number from the Deletion queue back into the phone number Inventory.
*
*
* @param restorePhoneNumberRequest
* @return Result of the RestorePhoneNumber operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.RestorePhoneNumber
* @see AWS API
* Documentation
*/
RestorePhoneNumberResult restorePhoneNumber(RestorePhoneNumberRequest restorePhoneNumberRequest);
/**
*
* Searches for phone numbers that can be ordered. For US numbers, provide at least one of the following search
* filters: AreaCode
, City
, State
, or TollFreePrefix
. If you
* provide City
, you must also provide State
. Numbers outside the US only support the
* PhoneNumberType
filter, which you must use.
*
*
* @param searchAvailablePhoneNumbersRequest
* @return Result of the SearchAvailablePhoneNumbers operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.SearchAvailablePhoneNumbers
* @see AWS API Documentation
*/
SearchAvailablePhoneNumbersResult searchAvailablePhoneNumbers(SearchAvailablePhoneNumbersRequest searchAvailablePhoneNumbersRequest);
/**
*
* Sends a message to a particular channel that the member is a part of.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
* Also, STANDARD
messages can contain 4KB of data and the 1KB of metadata. CONTROL
* messages can contain 30 bytes of data and no metadata.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* SendChannelMessage, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param sendChannelMessageRequest
* @return Result of the SendChannelMessage operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.SendChannelMessage
* @see AWS API
* Documentation
*/
@Deprecated
SendChannelMessageResult sendChannelMessage(SendChannelMessageRequest sendChannelMessageRequest);
/**
*
* Starts transcription for the specified meetingId
. For more information, refer to Using Amazon Chime SDK live
* transcription in the Amazon Chime SDK Developer Guide.
*
*
* If you specify an invalid configuration, a TranscriptFailed
event will be sent with the contents of
* the BadRequestException
generated by Amazon Transcribe. For more information on each parameter and
* which combinations are valid, refer to the StartStreamTranscription API in the Amazon Transcribe Developer Guide.
*
*
*
* Amazon Chime SDK live transcription is powered by Amazon Transcribe. Use of Amazon Transcribe is subject to the
* AWS Service Terms, including the terms specific to the AWS
* Machine Learning and Artificial Intelligence Services.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* StartMeetingTranscription, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param startMeetingTranscriptionRequest
* @return Result of the StartMeetingTranscription operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws UnprocessableEntityException
* The request was well-formed but was unable to be followed due to semantic errors.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.StartMeetingTranscription
* @see AWS API Documentation
*/
@Deprecated
StartMeetingTranscriptionResult startMeetingTranscription(StartMeetingTranscriptionRequest startMeetingTranscriptionRequest);
/**
*
* Stops transcription for the specified meetingId
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* StopMeetingTranscription, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param stopMeetingTranscriptionRequest
* @return Result of the StopMeetingTranscription operation returned by the service.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws UnprocessableEntityException
* The request was well-formed but was unable to be followed due to semantic errors.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.StopMeetingTranscription
* @see AWS
* API Documentation
*/
@Deprecated
StopMeetingTranscriptionResult stopMeetingTranscription(StopMeetingTranscriptionRequest stopMeetingTranscriptionRequest);
/**
*
* Applies the specified tags to the specified Amazon Chime attendee.
*
*
*
* TagAttendee is not supported in the Amazon Chime SDK Meetings Namespace. Update your application to remove calls
* to this API.
*
*
*
* @param tagAttendeeRequest
* @return Result of the TagAttendee operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.TagAttendee
* @see AWS API
* Documentation
*/
@Deprecated
TagAttendeeResult tagAttendee(TagAttendeeRequest tagAttendeeRequest);
/**
*
* Applies the specified tags to the specified Amazon Chime SDK meeting.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, TagResource,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param tagMeetingRequest
* @return Result of the TagMeeting operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.TagMeeting
* @see AWS API
* Documentation
*/
@Deprecated
TagMeetingResult tagMeeting(TagMeetingRequest tagMeetingRequest);
/**
*
* Applies the specified tags to the specified Amazon Chime SDK meeting resource.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, TagResource,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.TagResource
* @see AWS API
* Documentation
*/
@Deprecated
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Untags the specified tags from the specified Amazon Chime SDK attendee.
*
*
*
* UntagAttendee is not supported in the Amazon Chime SDK Meetings Namespace. Update your application to remove
* calls to this API.
*
*
*
* @param untagAttendeeRequest
* @return Result of the UntagAttendee operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UntagAttendee
* @see AWS API
* Documentation
*/
@Deprecated
UntagAttendeeResult untagAttendee(UntagAttendeeRequest untagAttendeeRequest);
/**
*
* Untags the specified tags from the specified Amazon Chime SDK meeting.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, UntagResource
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param untagMeetingRequest
* @return Result of the UntagMeeting operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UntagMeeting
* @see AWS API
* Documentation
*/
@Deprecated
UntagMeetingResult untagMeeting(UntagMeetingRequest untagMeetingRequest);
/**
*
* Untags the specified tags from the specified Amazon Chime SDK meeting resource.
*
*
* Applies the specified tags to the specified Amazon Chime SDK meeting resource.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, UntagResource
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UntagResource
* @see AWS API
* Documentation
*/
@Deprecated
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates account details for the specified Amazon Chime account. Currently, only account name and default license
* updates are supported for this action.
*
*
* @param updateAccountRequest
* @return Result of the UpdateAccount operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateAccount
* @see AWS API
* Documentation
*/
UpdateAccountResult updateAccount(UpdateAccountRequest updateAccountRequest);
/**
*
* Updates the settings for the specified Amazon Chime account. You can update settings for remote control of shared
* screens, or for the dial-out option. For more information about these settings, see Use the Policies Page in the Amazon Chime
* Administration Guide.
*
*
* @param updateAccountSettingsRequest
* @return Result of the UpdateAccountSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateAccountSettings
* @see AWS
* API Documentation
*/
UpdateAccountSettingsResult updateAccountSettings(UpdateAccountSettingsRequest updateAccountSettingsRequest);
/**
*
* Updates AppInstance
metadata.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* UpdateAppInstance, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param updateAppInstanceRequest
* @return Result of the UpdateAppInstance operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateAppInstance
* @see AWS API
* Documentation
*/
@Deprecated
UpdateAppInstanceResult updateAppInstance(UpdateAppInstanceRequest updateAppInstanceRequest);
/**
*
* Updates the details of an AppInstanceUser
. You can update names and metadata.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* UpdateAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param updateAppInstanceUserRequest
* @return Result of the UpdateAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateAppInstanceUser
* @see AWS
* API Documentation
*/
@Deprecated
UpdateAppInstanceUserResult updateAppInstanceUser(UpdateAppInstanceUserRequest updateAppInstanceUserRequest);
/**
*
* Updates the status of the specified bot, such as starting or stopping the bot from running in your Amazon Chime
* Enterprise account.
*
*
* @param updateBotRequest
* @return Result of the UpdateBot operation returned by the service.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @sample AmazonChime.UpdateBot
* @see AWS API
* Documentation
*/
UpdateBotResult updateBot(UpdateBotRequest updateBotRequest);
/**
*
* Update a channel's attributes.
*
*
* Restriction: You can't change a channel's privacy.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, UpdateChannel
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param updateChannelRequest
* @return Result of the UpdateChannel operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateChannel
* @see AWS API
* Documentation
*/
@Deprecated
UpdateChannelResult updateChannel(UpdateChannelRequest updateChannelRequest);
/**
*
* Updates the content of a message.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* UpdateChannelMessage, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param updateChannelMessageRequest
* @return Result of the UpdateChannelMessage operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateChannelMessage
* @see AWS API
* Documentation
*/
@Deprecated
UpdateChannelMessageResult updateChannelMessage(UpdateChannelMessageRequest updateChannelMessageRequest);
/**
*
* The details of the time when a user last read messages in a channel.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* UpdateChannelReadMarker, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param updateChannelReadMarkerRequest
* @return Result of the UpdateChannelReadMarker operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateChannelReadMarker
* @see AWS
* API Documentation
*/
@Deprecated
UpdateChannelReadMarkerResult updateChannelReadMarker(UpdateChannelReadMarkerRequest updateChannelReadMarkerRequest);
/**
*
* Updates global settings for the administrator's AWS account, such as Amazon Chime Business Calling and Amazon
* Chime Voice Connector settings.
*
*
* @param updateGlobalSettingsRequest
* @return Result of the UpdateGlobalSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateGlobalSettings
* @see AWS API
* Documentation
*/
UpdateGlobalSettingsResult updateGlobalSettings(UpdateGlobalSettingsRequest updateGlobalSettingsRequest);
/**
*
* Updates phone number details, such as product type or calling name, for the specified phone number ID. You can
* update one phone number detail at a time. For example, you can update either the product type or the calling name
* in one action.
*
*
* For toll-free numbers, you cannot use the Amazon Chime Business Calling product type. For numbers outside the
* U.S., you must use the Amazon Chime SIP Media Application Dial-In product type.
*
*
* Updates to outbound calling names can take 72 hours to complete. Pending updates to outbound calling names must
* be complete before you can request another update.
*
*
* @param updatePhoneNumberRequest
* @return Result of the UpdatePhoneNumber operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdatePhoneNumber
* @see AWS API
* Documentation
*/
UpdatePhoneNumberResult updatePhoneNumber(UpdatePhoneNumberRequest updatePhoneNumberRequest);
/**
*
* Updates the phone number settings for the administrator's AWS account, such as the default outbound calling name.
* You can update the default outbound calling name once every seven days. Outbound calling names can take up to 72
* hours to update.
*
*
* @param updatePhoneNumberSettingsRequest
* @return Result of the UpdatePhoneNumberSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdatePhoneNumberSettings
* @see AWS API Documentation
*/
UpdatePhoneNumberSettingsResult updatePhoneNumberSettings(UpdatePhoneNumberSettingsRequest updatePhoneNumberSettingsRequest);
/**
*
* Updates the specified proxy session details, such as voice or SMS capabilities.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* UpdateProxySession, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param updateProxySessionRequest
* @return Result of the UpdateProxySession operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateProxySession
* @see AWS API
* Documentation
*/
@Deprecated
UpdateProxySessionResult updateProxySession(UpdateProxySessionRequest updateProxySessionRequest);
/**
*
* Updates room details, such as the room name, for a room in an Amazon Chime Enterprise account.
*
*
* @param updateRoomRequest
* @return Result of the UpdateRoom operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateRoom
* @see AWS API
* Documentation
*/
UpdateRoomResult updateRoom(UpdateRoomRequest updateRoomRequest);
/**
*
* Updates room membership details, such as the member role, for a room in an Amazon Chime Enterprise account. The
* member role designates whether the member is a chat room administrator or a general chat room member. The member
* role can be updated only for user IDs.
*
*
* @param updateRoomMembershipRequest
* @return Result of the UpdateRoomMembership operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateRoomMembership
* @see AWS API
* Documentation
*/
UpdateRoomMembershipResult updateRoomMembership(UpdateRoomMembershipRequest updateRoomMembershipRequest);
/**
*
* Updates the details of the specified SIP media application.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* UpdateSipMediaApplication, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param updateSipMediaApplicationRequest
* @return Result of the UpdateSipMediaApplication operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateSipMediaApplication
* @see AWS API Documentation
*/
@Deprecated
UpdateSipMediaApplicationResult updateSipMediaApplication(UpdateSipMediaApplicationRequest updateSipMediaApplicationRequest);
/**
*
* Invokes the AWS Lambda function associated with the SIP media application and transaction ID in an update
* request. The Lambda function can then return a new set of actions.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, UpdateSipMediaApplicationCall, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param updateSipMediaApplicationCallRequest
* @return Result of the UpdateSipMediaApplicationCall operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateSipMediaApplicationCall
* @see AWS API Documentation
*/
@Deprecated
UpdateSipMediaApplicationCallResult updateSipMediaApplicationCall(UpdateSipMediaApplicationCallRequest updateSipMediaApplicationCallRequest);
/**
*
* Updates the details of the specified SIP rule.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, UpdateSipRule,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param updateSipRuleRequest
* @return Result of the UpdateSipRule operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateSipRule
* @see AWS API
* Documentation
*/
@Deprecated
UpdateSipRuleResult updateSipRule(UpdateSipRuleRequest updateSipRuleRequest);
/**
*
* Updates user details for a specified user ID. Currently, only LicenseType
updates are supported for
* this action.
*
*
* @param updateUserRequest
* @return Result of the UpdateUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateUser
* @see AWS API
* Documentation
*/
UpdateUserResult updateUser(UpdateUserRequest updateUserRequest);
/**
*
* Updates the settings for the specified user, such as phone number settings.
*
*
* @param updateUserSettingsRequest
* @return Result of the UpdateUserSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateUserSettings
* @see AWS API
* Documentation
*/
UpdateUserSettingsResult updateUserSettings(UpdateUserSettingsRequest updateUserSettingsRequest);
/**
*
* Updates details for the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* UpdateVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param updateVoiceConnectorRequest
* @return Result of the UpdateVoiceConnector operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateVoiceConnector
* @see AWS API
* Documentation
*/
@Deprecated
UpdateVoiceConnectorResult updateVoiceConnector(UpdateVoiceConnectorRequest updateVoiceConnectorRequest);
/**
*
* Updates details of the specified Amazon Chime Voice Connector group, such as the name and Amazon Chime Voice
* Connector priority ranking.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* UpdateVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param updateVoiceConnectorGroupRequest
* @return Result of the UpdateVoiceConnectorGroup operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.UpdateVoiceConnectorGroup
* @see AWS API Documentation
*/
@Deprecated
UpdateVoiceConnectorGroupResult updateVoiceConnectorGroup(UpdateVoiceConnectorGroupRequest updateVoiceConnectorGroupRequest);
/**
*
* Validates an address to be used for 911 calls made with Amazon Chime Voice Connectors. You can use validated
* addresses in a Presence Information Data Format Location Object file that you include in SIP requests. That helps
* ensure that addresses are routed to the appropriate Public Safety Answering Point.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* ValidateE911Address, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param validateE911AddressRequest
* @return Result of the ValidateE911Address operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.ValidateE911Address
* @see AWS API
* Documentation
*/
@Deprecated
ValidateE911AddressResult validateE911Address(ValidateE911AddressRequest validateE911AddressRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}