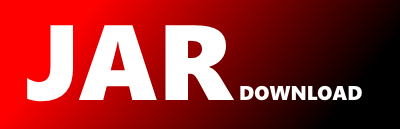
com.amazonaws.services.chime.AmazonChimeClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chime Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chime;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.chime.AmazonChimeClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.chime.model.*;
import com.amazonaws.services.chime.model.transform.*;
/**
* Client for accessing Amazon Chime. All service calls made using this client are blocking, and will not return until
* the service call completes.
*
*
*
* Most of these APIs are no longer supported and will not be updated. We recommend using the latest versions in
* the Amazon Chime SDK API
* reference, in the Amazon Chime SDK.
*
*
* Using the latest versions requires migrating to dedicated namespaces. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* The Amazon Chime application programming interface (API) is designed so administrators can perform key tasks, such as
* creating and managing Amazon Chime accounts, users, and Voice Connectors. This guide provides detailed information
* about the Amazon Chime API, including operations, types, inputs and outputs, and error codes.
*
*
* You can use an AWS SDK, the AWS Command Line Interface (AWS CLI), or the REST API to make API calls for Amazon Chime.
* We recommend using an AWS SDK or the AWS CLI. The page for each API action contains a See Also section that
* includes links to information about using the action with a language-specific AWS SDK or the AWS CLI.
*
*
* - Using an AWS SDK
* -
*
* You don't need to write code to calculate a signature for request authentication. The SDK clients authenticate your
* requests by using access keys that you provide. For more information about AWS SDKs, see the AWS Developer Center.
*
*
* - Using the AWS CLI
* -
*
* Use your access keys with the AWS CLI to make API calls. For information about setting up the AWS CLI, see Installing the AWS Command Line Interface
* in the AWS Command Line Interface User Guide. For a list of available Amazon Chime commands, see the Amazon Chime commands in the AWS CLI
* Command Reference.
*
*
* - Using REST APIs
* -
*
* If you use REST to make API calls, you must authenticate your request by providing a signature. Amazon Chime supports
* Signature Version 4. For more information, see Signature Version 4 Signing Process
* in the Amazon Web Services General Reference.
*
*
* When making REST API calls, use the service name chime
and REST endpoint
* https://service.chime.aws.amazon.com
.
*
*
*
*
* Administrative permissions are controlled using AWS Identity and Access Management (IAM). For more information, see
* Identity and Access Management for Amazon
* Chime in the Amazon Chime Administration Guide.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonChimeClient extends AmazonWebServiceClient implements AmazonChime {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonChime.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "chime";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ForbiddenException").withExceptionUnmarshaller(
com.amazonaws.services.chime.model.transform.ForbiddenExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.chime.model.transform.NotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceFailureException").withExceptionUnmarshaller(
com.amazonaws.services.chime.model.transform.ServiceFailureExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.chime.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnprocessableEntityException").withExceptionUnmarshaller(
com.amazonaws.services.chime.model.transform.UnprocessableEntityExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BadRequestException").withExceptionUnmarshaller(
com.amazonaws.services.chime.model.transform.BadRequestExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.chime.model.transform.ResourceLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnauthorizedClientException").withExceptionUnmarshaller(
com.amazonaws.services.chime.model.transform.UnauthorizedClientExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.chime.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottledClientException").withExceptionUnmarshaller(
com.amazonaws.services.chime.model.transform.ThrottledClientExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceUnavailableException").withExceptionUnmarshaller(
com.amazonaws.services.chime.model.transform.ServiceUnavailableExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.chime.model.AmazonChimeException.class));
public static AmazonChimeClientBuilder builder() {
return AmazonChimeClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon Chime using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonChimeClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon Chime using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonChimeClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("chime.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/chime/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/chime/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Associates a phone number with the specified Amazon Chime user.
*
*
* @param associatePhoneNumberWithUserRequest
* @return Result of the AssociatePhoneNumberWithUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.AssociatePhoneNumberWithUser
* @see AWS API Documentation
*/
@Override
public AssociatePhoneNumberWithUserResult associatePhoneNumberWithUser(AssociatePhoneNumberWithUserRequest request) {
request = beforeClientExecution(request);
return executeAssociatePhoneNumberWithUser(request);
}
@SdkInternalApi
final AssociatePhoneNumberWithUserResult executeAssociatePhoneNumberWithUser(AssociatePhoneNumberWithUserRequest associatePhoneNumberWithUserRequest) {
ExecutionContext executionContext = createExecutionContext(associatePhoneNumberWithUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociatePhoneNumberWithUserRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associatePhoneNumberWithUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociatePhoneNumberWithUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociatePhoneNumberWithUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates phone numbers with the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, AssociatePhoneNumbersWithVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param associatePhoneNumbersWithVoiceConnectorRequest
* @return Result of the AssociatePhoneNumbersWithVoiceConnector operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.AssociatePhoneNumbersWithVoiceConnector
* @see AWS API Documentation
*/
@Override
@Deprecated
public AssociatePhoneNumbersWithVoiceConnectorResult associatePhoneNumbersWithVoiceConnector(AssociatePhoneNumbersWithVoiceConnectorRequest request) {
request = beforeClientExecution(request);
return executeAssociatePhoneNumbersWithVoiceConnector(request);
}
@SdkInternalApi
final AssociatePhoneNumbersWithVoiceConnectorResult executeAssociatePhoneNumbersWithVoiceConnector(
AssociatePhoneNumbersWithVoiceConnectorRequest associatePhoneNumbersWithVoiceConnectorRequest) {
ExecutionContext executionContext = createExecutionContext(associatePhoneNumbersWithVoiceConnectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociatePhoneNumbersWithVoiceConnectorRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associatePhoneNumbersWithVoiceConnectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociatePhoneNumbersWithVoiceConnector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociatePhoneNumbersWithVoiceConnectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates phone numbers with the specified Amazon Chime Voice Connector group.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, AssociatePhoneNumbersWithVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param associatePhoneNumbersWithVoiceConnectorGroupRequest
* @return Result of the AssociatePhoneNumbersWithVoiceConnectorGroup operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.AssociatePhoneNumbersWithVoiceConnectorGroup
* @see AWS API Documentation
*/
@Override
@Deprecated
public AssociatePhoneNumbersWithVoiceConnectorGroupResult associatePhoneNumbersWithVoiceConnectorGroup(
AssociatePhoneNumbersWithVoiceConnectorGroupRequest request) {
request = beforeClientExecution(request);
return executeAssociatePhoneNumbersWithVoiceConnectorGroup(request);
}
@SdkInternalApi
final AssociatePhoneNumbersWithVoiceConnectorGroupResult executeAssociatePhoneNumbersWithVoiceConnectorGroup(
AssociatePhoneNumbersWithVoiceConnectorGroupRequest associatePhoneNumbersWithVoiceConnectorGroupRequest) {
ExecutionContext executionContext = createExecutionContext(associatePhoneNumbersWithVoiceConnectorGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociatePhoneNumbersWithVoiceConnectorGroupRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associatePhoneNumbersWithVoiceConnectorGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociatePhoneNumbersWithVoiceConnectorGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociatePhoneNumbersWithVoiceConnectorGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates the specified sign-in delegate groups with the specified Amazon Chime account.
*
*
* @param associateSigninDelegateGroupsWithAccountRequest
* @return Result of the AssociateSigninDelegateGroupsWithAccount operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.AssociateSigninDelegateGroupsWithAccount
* @see AWS API Documentation
*/
@Override
public AssociateSigninDelegateGroupsWithAccountResult associateSigninDelegateGroupsWithAccount(AssociateSigninDelegateGroupsWithAccountRequest request) {
request = beforeClientExecution(request);
return executeAssociateSigninDelegateGroupsWithAccount(request);
}
@SdkInternalApi
final AssociateSigninDelegateGroupsWithAccountResult executeAssociateSigninDelegateGroupsWithAccount(
AssociateSigninDelegateGroupsWithAccountRequest associateSigninDelegateGroupsWithAccountRequest) {
ExecutionContext executionContext = createExecutionContext(associateSigninDelegateGroupsWithAccountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociateSigninDelegateGroupsWithAccountRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associateSigninDelegateGroupsWithAccountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociateSigninDelegateGroupsWithAccount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociateSigninDelegateGroupsWithAccountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates up to 100 new attendees for an active Amazon Chime SDK meeting.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* BatchCreateAttendee, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide.
*
*
* @param batchCreateAttendeeRequest
* @return Result of the BatchCreateAttendee operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchCreateAttendee
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public BatchCreateAttendeeResult batchCreateAttendee(BatchCreateAttendeeRequest request) {
request = beforeClientExecution(request);
return executeBatchCreateAttendee(request);
}
@SdkInternalApi
final BatchCreateAttendeeResult executeBatchCreateAttendee(BatchCreateAttendeeRequest batchCreateAttendeeRequest) {
ExecutionContext executionContext = createExecutionContext(batchCreateAttendeeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchCreateAttendeeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(batchCreateAttendeeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchCreateAttendee");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new BatchCreateAttendeeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds a specified number of users to a channel.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, BatchCreateChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param batchCreateChannelMembershipRequest
* @return Result of the BatchCreateChannelMembership operation returned by the service.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @sample AmazonChime.BatchCreateChannelMembership
* @see AWS API Documentation
*/
@Override
@Deprecated
public BatchCreateChannelMembershipResult batchCreateChannelMembership(BatchCreateChannelMembershipRequest request) {
request = beforeClientExecution(request);
return executeBatchCreateChannelMembership(request);
}
@SdkInternalApi
final BatchCreateChannelMembershipResult executeBatchCreateChannelMembership(BatchCreateChannelMembershipRequest batchCreateChannelMembershipRequest) {
ExecutionContext executionContext = createExecutionContext(batchCreateChannelMembershipRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchCreateChannelMembershipRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(batchCreateChannelMembershipRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchCreateChannelMembership");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchCreateChannelMembershipResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds up to 50 members to a chat room in an Amazon Chime Enterprise account. Members can be users or bots. The
* member role designates whether the member is a chat room administrator or a general chat room member.
*
*
* @param batchCreateRoomMembershipRequest
* @return Result of the BatchCreateRoomMembership operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchCreateRoomMembership
* @see AWS API Documentation
*/
@Override
public BatchCreateRoomMembershipResult batchCreateRoomMembership(BatchCreateRoomMembershipRequest request) {
request = beforeClientExecution(request);
return executeBatchCreateRoomMembership(request);
}
@SdkInternalApi
final BatchCreateRoomMembershipResult executeBatchCreateRoomMembership(BatchCreateRoomMembershipRequest batchCreateRoomMembershipRequest) {
ExecutionContext executionContext = createExecutionContext(batchCreateRoomMembershipRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchCreateRoomMembershipRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(batchCreateRoomMembershipRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchCreateRoomMembership");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchCreateRoomMembershipResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Moves phone numbers into the Deletion queue. Phone numbers must be disassociated from any users or Amazon
* Chime Voice Connectors before they can be deleted.
*
*
* Phone numbers remain in the Deletion queue for 7 days before they are deleted permanently.
*
*
* @param batchDeletePhoneNumberRequest
* @return Result of the BatchDeletePhoneNumber operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchDeletePhoneNumber
* @see AWS
* API Documentation
*/
@Override
public BatchDeletePhoneNumberResult batchDeletePhoneNumber(BatchDeletePhoneNumberRequest request) {
request = beforeClientExecution(request);
return executeBatchDeletePhoneNumber(request);
}
@SdkInternalApi
final BatchDeletePhoneNumberResult executeBatchDeletePhoneNumber(BatchDeletePhoneNumberRequest batchDeletePhoneNumberRequest) {
ExecutionContext executionContext = createExecutionContext(batchDeletePhoneNumberRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchDeletePhoneNumberRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(batchDeletePhoneNumberRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchDeletePhoneNumber");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchDeletePhoneNumberResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Suspends up to 50 users from a Team
or EnterpriseLWA
Amazon Chime account. For more
* information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* Users suspended from a Team
account are disassociated from the account,but they can continue to use
* Amazon Chime as free users. To remove the suspension from suspended Team
account users, invite them
* to the Team
account again. You can use the InviteUsers action to do so.
*
*
* Users suspended from an EnterpriseLWA
account are immediately signed out of Amazon Chime and can no
* longer sign in. To remove the suspension from suspended EnterpriseLWA
account users, use the
* BatchUnsuspendUser action.
*
*
* To sign out users without suspending them, use the LogoutUser action.
*
*
* @param batchSuspendUserRequest
* @return Result of the BatchSuspendUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchSuspendUser
* @see AWS API
* Documentation
*/
@Override
public BatchSuspendUserResult batchSuspendUser(BatchSuspendUserRequest request) {
request = beforeClientExecution(request);
return executeBatchSuspendUser(request);
}
@SdkInternalApi
final BatchSuspendUserResult executeBatchSuspendUser(BatchSuspendUserRequest batchSuspendUserRequest) {
ExecutionContext executionContext = createExecutionContext(batchSuspendUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchSuspendUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(batchSuspendUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchSuspendUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new BatchSuspendUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the suspension from up to 50 previously suspended users for the specified Amazon Chime
* EnterpriseLWA
account. Only users on EnterpriseLWA
accounts can be unsuspended using
* this action. For more information about different account types, see Managing Your Amazon Chime Accounts
* in the account types, in the Amazon Chime Administration Guide.
*
*
* Previously suspended users who are unsuspended using this action are returned to Registered
status.
* Users who are not previously suspended are ignored.
*
*
* @param batchUnsuspendUserRequest
* @return Result of the BatchUnsuspendUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchUnsuspendUser
* @see AWS API
* Documentation
*/
@Override
public BatchUnsuspendUserResult batchUnsuspendUser(BatchUnsuspendUserRequest request) {
request = beforeClientExecution(request);
return executeBatchUnsuspendUser(request);
}
@SdkInternalApi
final BatchUnsuspendUserResult executeBatchUnsuspendUser(BatchUnsuspendUserRequest batchUnsuspendUserRequest) {
ExecutionContext executionContext = createExecutionContext(batchUnsuspendUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchUnsuspendUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(batchUnsuspendUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchUnsuspendUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new BatchUnsuspendUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates phone number product types or calling names. You can update one attribute at a time for each
* UpdatePhoneNumberRequestItem
. For example, you can update the product type or the calling name.
*
*
* For toll-free numbers, you cannot use the Amazon Chime Business Calling product type. For numbers outside the
* U.S., you must use the Amazon Chime SIP Media Application Dial-In product type.
*
*
* Updates to outbound calling names can take up to 72 hours to complete. Pending updates to outbound calling names
* must be complete before you can request another update.
*
*
* @param batchUpdatePhoneNumberRequest
* @return Result of the BatchUpdatePhoneNumber operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchUpdatePhoneNumber
* @see AWS
* API Documentation
*/
@Override
public BatchUpdatePhoneNumberResult batchUpdatePhoneNumber(BatchUpdatePhoneNumberRequest request) {
request = beforeClientExecution(request);
return executeBatchUpdatePhoneNumber(request);
}
@SdkInternalApi
final BatchUpdatePhoneNumberResult executeBatchUpdatePhoneNumber(BatchUpdatePhoneNumberRequest batchUpdatePhoneNumberRequest) {
ExecutionContext executionContext = createExecutionContext(batchUpdatePhoneNumberRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchUpdatePhoneNumberRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(batchUpdatePhoneNumberRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchUpdatePhoneNumber");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchUpdatePhoneNumberResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates user details within the UpdateUserRequestItem object for up to 20 users for the specified Amazon
* Chime account. Currently, only LicenseType
updates are supported for this action.
*
*
* @param batchUpdateUserRequest
* @return Result of the BatchUpdateUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.BatchUpdateUser
* @see AWS API
* Documentation
*/
@Override
public BatchUpdateUserResult batchUpdateUser(BatchUpdateUserRequest request) {
request = beforeClientExecution(request);
return executeBatchUpdateUser(request);
}
@SdkInternalApi
final BatchUpdateUserResult executeBatchUpdateUser(BatchUpdateUserRequest batchUpdateUserRequest) {
ExecutionContext executionContext = createExecutionContext(batchUpdateUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchUpdateUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(batchUpdateUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchUpdateUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new BatchUpdateUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon Chime account under the administrator's AWS account. Only Team
account types are
* currently supported for this action. For more information about different account types, see Managing Your Amazon Chime
* Accounts in the Amazon Chime Administration Guide.
*
*
* @param createAccountRequest
* @return Result of the CreateAccount operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateAccount
* @see AWS API
* Documentation
*/
@Override
public CreateAccountResult createAccount(CreateAccountRequest request) {
request = beforeClientExecution(request);
return executeCreateAccount(request);
}
@SdkInternalApi
final CreateAccountResult executeCreateAccount(CreateAccountRequest createAccountRequest) {
ExecutionContext executionContext = createExecutionContext(createAccountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAccountRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAccountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAccount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateAccountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon Chime SDK messaging AppInstance
under an AWS account. Only SDK messaging customers
* use this API. CreateAppInstance
supports idempotency behavior as described in the AWS API Standard.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateAppInstance, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createAppInstanceRequest
* @return Result of the CreateAppInstance operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateAppInstance
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public CreateAppInstanceResult createAppInstance(CreateAppInstanceRequest request) {
request = beforeClientExecution(request);
return executeCreateAppInstance(request);
}
@SdkInternalApi
final CreateAppInstanceResult executeCreateAppInstance(CreateAppInstanceRequest createAppInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(createAppInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAppInstanceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAppInstanceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAppInstance");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "identity-";
String resolvedHostPrefix = String.format("identity-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateAppInstanceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Promotes an AppInstanceUser
to an AppInstanceAdmin
. The promoted user can perform the
* following actions.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateAppInstanceAdmin, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* -
*
* ChannelModerator
actions across all channels in the AppInstance
.
*
*
* -
*
* DeleteChannelMessage
actions.
*
*
*
*
* Only an AppInstanceUser
can be promoted to an AppInstanceAdmin
role.
*
*
* @param createAppInstanceAdminRequest
* @return Result of the CreateAppInstanceAdmin operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateAppInstanceAdmin
* @see AWS
* API Documentation
*/
@Override
@Deprecated
public CreateAppInstanceAdminResult createAppInstanceAdmin(CreateAppInstanceAdminRequest request) {
request = beforeClientExecution(request);
return executeCreateAppInstanceAdmin(request);
}
@SdkInternalApi
final CreateAppInstanceAdminResult executeCreateAppInstanceAdmin(CreateAppInstanceAdminRequest createAppInstanceAdminRequest) {
ExecutionContext executionContext = createExecutionContext(createAppInstanceAdminRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAppInstanceAdminRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAppInstanceAdminRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAppInstanceAdmin");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "identity-";
String resolvedHostPrefix = String.format("identity-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateAppInstanceAdminResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a user under an Amazon Chime AppInstance
. The request consists of a unique
* appInstanceUserId
and Name
for that user.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createAppInstanceUserRequest
* @return Result of the CreateAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateAppInstanceUser
* @see AWS
* API Documentation
*/
@Override
@Deprecated
public CreateAppInstanceUserResult createAppInstanceUser(CreateAppInstanceUserRequest request) {
request = beforeClientExecution(request);
return executeCreateAppInstanceUser(request);
}
@SdkInternalApi
final CreateAppInstanceUserResult executeCreateAppInstanceUser(CreateAppInstanceUserRequest createAppInstanceUserRequest) {
ExecutionContext executionContext = createExecutionContext(createAppInstanceUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAppInstanceUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAppInstanceUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAppInstanceUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "identity-";
String resolvedHostPrefix = String.format("identity-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateAppInstanceUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new attendee for an active Amazon Chime SDK meeting. For more information about the Amazon Chime SDK,
* see Using the Amazon Chime SDK in
* the Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateAttendee
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createAttendeeRequest
* @return Result of the CreateAttendee operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateAttendee
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public CreateAttendeeResult createAttendee(CreateAttendeeRequest request) {
request = beforeClientExecution(request);
return executeCreateAttendee(request);
}
@SdkInternalApi
final CreateAttendeeResult executeCreateAttendee(CreateAttendeeRequest createAttendeeRequest) {
ExecutionContext executionContext = createExecutionContext(createAttendeeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAttendeeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAttendeeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAttendee");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateAttendeeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a bot for an Amazon Chime Enterprise account.
*
*
* @param createBotRequest
* @return Result of the CreateBot operation returned by the service.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @sample AmazonChime.CreateBot
* @see AWS API
* Documentation
*/
@Override
public CreateBotResult createBot(CreateBotRequest request) {
request = beforeClientExecution(request);
return executeCreateBot(request);
}
@SdkInternalApi
final CreateBotResult executeCreateBot(CreateBotRequest createBotRequest) {
ExecutionContext executionContext = createExecutionContext(createBotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateBotRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createBotRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateBot");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateBotResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a channel to which you can add users and send messages.
*
*
* Restriction: You can't change a channel's privacy.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateChannel
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelRequest
* @return Result of the CreateChannel operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateChannel
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public CreateChannelResult createChannel(CreateChannelRequest request) {
request = beforeClientExecution(request);
return executeCreateChannel(request);
}
@SdkInternalApi
final CreateChannelResult executeCreateChannel(CreateChannelRequest createChannelRequest) {
ExecutionContext executionContext = createExecutionContext(createChannelRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateChannelRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createChannelRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateChannel");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateChannelResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Permanently bans a member from a channel. Moderators can't add banned members to a channel. To undo a ban, you
* first have to DeleteChannelBan
, and then CreateChannelMembership
. Bans are cleaned up
* when you delete users or channels.
*
*
* If you ban a user who is already part of a channel, that user is automatically kicked from the channel.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateChannelBan, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelBanRequest
* @return Result of the CreateChannelBan operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateChannelBan
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public CreateChannelBanResult createChannelBan(CreateChannelBanRequest request) {
request = beforeClientExecution(request);
return executeCreateChannelBan(request);
}
@SdkInternalApi
final CreateChannelBanResult executeCreateChannelBan(CreateChannelBanRequest createChannelBanRequest) {
ExecutionContext executionContext = createExecutionContext(createChannelBanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateChannelBanRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createChannelBanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateChannelBan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateChannelBanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds a user to a channel. The InvitedBy
response field is derived from the request header. A channel
* member can:
*
*
* -
*
* List messages
*
*
* -
*
* Send messages
*
*
* -
*
* Receive messages
*
*
* -
*
* Edit their own messages
*
*
* -
*
* Leave the channel
*
*
*
*
* Privacy settings impact this action as follows:
*
*
* -
*
* Public Channels: You do not need to be a member to list messages, but you must be a member to send messages.
*
*
* -
*
* Private Channels: You must be a member to list or send messages.
*
*
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelMembershipRequest
* @return Result of the CreateChannelMembership operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateChannelMembership
* @see AWS
* API Documentation
*/
@Override
@Deprecated
public CreateChannelMembershipResult createChannelMembership(CreateChannelMembershipRequest request) {
request = beforeClientExecution(request);
return executeCreateChannelMembership(request);
}
@SdkInternalApi
final CreateChannelMembershipResult executeCreateChannelMembership(CreateChannelMembershipRequest createChannelMembershipRequest) {
ExecutionContext executionContext = createExecutionContext(createChannelMembershipRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateChannelMembershipRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createChannelMembershipRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateChannelMembership");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateChannelMembershipResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new ChannelModerator
. A channel moderator can:
*
*
* -
*
* Add and remove other members of the channel.
*
*
* -
*
* Add and remove other moderators of the channel.
*
*
* -
*
* Add and remove user bans for the channel.
*
*
* -
*
* Redact messages in the channel.
*
*
* -
*
* List messages in the channel.
*
*
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateChannelModerator, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createChannelModeratorRequest
* @return Result of the CreateChannelModerator operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateChannelModerator
* @see AWS
* API Documentation
*/
@Override
@Deprecated
public CreateChannelModeratorResult createChannelModerator(CreateChannelModeratorRequest request) {
request = beforeClientExecution(request);
return executeCreateChannelModerator(request);
}
@SdkInternalApi
final CreateChannelModeratorResult executeCreateChannelModerator(CreateChannelModeratorRequest createChannelModeratorRequest) {
ExecutionContext executionContext = createExecutionContext(createChannelModeratorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateChannelModeratorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createChannelModeratorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateChannelModerator");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateChannelModeratorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a media capture pipeline.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateMediaCapturePipeline, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createMediaCapturePipelineRequest
* @return Result of the CreateMediaCapturePipeline operation returned by the service.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateMediaCapturePipeline
* @see AWS API Documentation
*/
@Override
@Deprecated
public CreateMediaCapturePipelineResult createMediaCapturePipeline(CreateMediaCapturePipelineRequest request) {
request = beforeClientExecution(request);
return executeCreateMediaCapturePipeline(request);
}
@SdkInternalApi
final CreateMediaCapturePipelineResult executeCreateMediaCapturePipeline(CreateMediaCapturePipelineRequest createMediaCapturePipelineRequest) {
ExecutionContext executionContext = createExecutionContext(createMediaCapturePipelineRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMediaCapturePipelineRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createMediaCapturePipelineRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMediaCapturePipeline");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateMediaCapturePipelineResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new Amazon Chime SDK meeting in the specified media Region with no initial attendees. For more
* information about specifying media Regions, see Amazon Chime SDK Media
* Regions in the Amazon Chime SDK Developer Guide . For more information about the Amazon Chime SDK, see
* Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateMeeting
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createMeetingRequest
* @return Result of the CreateMeeting operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateMeeting
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public CreateMeetingResult createMeeting(CreateMeetingRequest request) {
request = beforeClientExecution(request);
return executeCreateMeeting(request);
}
@SdkInternalApi
final CreateMeetingResult executeCreateMeeting(CreateMeetingRequest createMeetingRequest) {
ExecutionContext executionContext = createExecutionContext(createMeetingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMeetingRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createMeetingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMeeting");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateMeetingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Uses the join token and call metadata in a meeting request (From number, To number, and so forth) to initiate an
* outbound call to a public switched telephone network (PSTN) and join them into a Chime meeting. Also ensures that
* the From number belongs to the customer.
*
*
* To play welcome audio or implement an interactive voice response (IVR), use the
* CreateSipMediaApplicationCall
action with the corresponding SIP media application ID.
*
*
*
* This API is is not available in a dedicated namespace.
*
*
*
* @param createMeetingDialOutRequest
* @return Result of the CreateMeetingDialOut operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateMeetingDialOut
* @see AWS API
* Documentation
*/
@Override
public CreateMeetingDialOutResult createMeetingDialOut(CreateMeetingDialOutRequest request) {
request = beforeClientExecution(request);
return executeCreateMeetingDialOut(request);
}
@SdkInternalApi
final CreateMeetingDialOutResult executeCreateMeetingDialOut(CreateMeetingDialOutRequest createMeetingDialOutRequest) {
ExecutionContext executionContext = createExecutionContext(createMeetingDialOutRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMeetingDialOutRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createMeetingDialOutRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMeetingDialOut");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateMeetingDialOutResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new Amazon Chime SDK meeting in the specified media Region, with attendees. For more information about
* specifying media Regions, see Amazon Chime SDK Media
* Regions in the Amazon Chime SDK Developer Guide . For more information about the Amazon Chime SDK, see
* Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide .
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateMeetingWithAttendees, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createMeetingWithAttendeesRequest
* @return Result of the CreateMeetingWithAttendees operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateMeetingWithAttendees
* @see AWS API Documentation
*/
@Override
@Deprecated
public CreateMeetingWithAttendeesResult createMeetingWithAttendees(CreateMeetingWithAttendeesRequest request) {
request = beforeClientExecution(request);
return executeCreateMeetingWithAttendees(request);
}
@SdkInternalApi
final CreateMeetingWithAttendeesResult executeCreateMeetingWithAttendees(CreateMeetingWithAttendeesRequest createMeetingWithAttendeesRequest) {
ExecutionContext executionContext = createExecutionContext(createMeetingWithAttendeesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMeetingWithAttendeesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createMeetingWithAttendeesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMeetingWithAttendees");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateMeetingWithAttendeesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an order for phone numbers to be provisioned. For toll-free numbers, you cannot use the Amazon Chime
* Business Calling product type. For numbers outside the U.S., you must use the Amazon Chime SIP Media Application
* Dial-In product type.
*
*
* @param createPhoneNumberOrderRequest
* @return Result of the CreatePhoneNumberOrder operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreatePhoneNumberOrder
* @see AWS
* API Documentation
*/
@Override
public CreatePhoneNumberOrderResult createPhoneNumberOrder(CreatePhoneNumberOrderRequest request) {
request = beforeClientExecution(request);
return executeCreatePhoneNumberOrder(request);
}
@SdkInternalApi
final CreatePhoneNumberOrderResult executeCreatePhoneNumberOrder(CreatePhoneNumberOrderRequest createPhoneNumberOrderRequest) {
ExecutionContext executionContext = createExecutionContext(createPhoneNumberOrderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreatePhoneNumberOrderRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createPhoneNumberOrderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreatePhoneNumberOrder");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreatePhoneNumberOrderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a proxy session on the specified Amazon Chime Voice Connector for the specified participant phone
* numbers.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateProxySession, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createProxySessionRequest
* @return Result of the CreateProxySession operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateProxySession
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public CreateProxySessionResult createProxySession(CreateProxySessionRequest request) {
request = beforeClientExecution(request);
return executeCreateProxySession(request);
}
@SdkInternalApi
final CreateProxySessionResult executeCreateProxySession(CreateProxySessionRequest createProxySessionRequest) {
ExecutionContext executionContext = createExecutionContext(createProxySessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateProxySessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createProxySessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateProxySession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateProxySessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a chat room for the specified Amazon Chime Enterprise account.
*
*
* @param createRoomRequest
* @return Result of the CreateRoom operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateRoom
* @see AWS API
* Documentation
*/
@Override
public CreateRoomResult createRoom(CreateRoomRequest request) {
request = beforeClientExecution(request);
return executeCreateRoom(request);
}
@SdkInternalApi
final CreateRoomResult executeCreateRoom(CreateRoomRequest createRoomRequest) {
ExecutionContext executionContext = createExecutionContext(createRoomRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateRoomRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createRoomRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateRoom");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateRoomResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds a member to a chat room in an Amazon Chime Enterprise account. A member can be either a user or a bot. The
* member role designates whether the member is a chat room administrator or a general chat room member.
*
*
* @param createRoomMembershipRequest
* @return Result of the CreateRoomMembership operation returned by the service.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateRoomMembership
* @see AWS API
* Documentation
*/
@Override
public CreateRoomMembershipResult createRoomMembership(CreateRoomMembershipRequest request) {
request = beforeClientExecution(request);
return executeCreateRoomMembership(request);
}
@SdkInternalApi
final CreateRoomMembershipResult executeCreateRoomMembership(CreateRoomMembershipRequest createRoomMembershipRequest) {
ExecutionContext executionContext = createExecutionContext(createRoomMembershipRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateRoomMembershipRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createRoomMembershipRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateRoomMembership");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateRoomMembershipResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a SIP media application.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateSipMediaApplication, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createSipMediaApplicationRequest
* @return Result of the CreateSipMediaApplication operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateSipMediaApplication
* @see AWS API Documentation
*/
@Override
@Deprecated
public CreateSipMediaApplicationResult createSipMediaApplication(CreateSipMediaApplicationRequest request) {
request = beforeClientExecution(request);
return executeCreateSipMediaApplication(request);
}
@SdkInternalApi
final CreateSipMediaApplicationResult executeCreateSipMediaApplication(CreateSipMediaApplicationRequest createSipMediaApplicationRequest) {
ExecutionContext executionContext = createExecutionContext(createSipMediaApplicationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSipMediaApplicationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createSipMediaApplicationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSipMediaApplication");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateSipMediaApplicationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an outbound call to a phone number from the phone number specified in the request, and it invokes the
* endpoint of the specified sipMediaApplicationId
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateSipMediaApplicationCall, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createSipMediaApplicationCallRequest
* @return Result of the CreateSipMediaApplicationCall operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateSipMediaApplicationCall
* @see AWS API Documentation
*/
@Override
@Deprecated
public CreateSipMediaApplicationCallResult createSipMediaApplicationCall(CreateSipMediaApplicationCallRequest request) {
request = beforeClientExecution(request);
return executeCreateSipMediaApplicationCall(request);
}
@SdkInternalApi
final CreateSipMediaApplicationCallResult executeCreateSipMediaApplicationCall(CreateSipMediaApplicationCallRequest createSipMediaApplicationCallRequest) {
ExecutionContext executionContext = createExecutionContext(createSipMediaApplicationCallRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSipMediaApplicationCallRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createSipMediaApplicationCallRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSipMediaApplicationCall");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateSipMediaApplicationCallResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a SIP rule which can be used to run a SIP media application as a target for a specific trigger type.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, CreateSipRule,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createSipRuleRequest
* @return Result of the CreateSipRule operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateSipRule
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public CreateSipRuleResult createSipRule(CreateSipRuleRequest request) {
request = beforeClientExecution(request);
return executeCreateSipRule(request);
}
@SdkInternalApi
final CreateSipRuleResult executeCreateSipRule(CreateSipRuleRequest createSipRuleRequest) {
ExecutionContext executionContext = createExecutionContext(createSipRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSipRuleRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createSipRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSipRule");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateSipRuleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a user under the specified Amazon Chime account.
*
*
* @param createUserRequest
* @return Result of the CreateUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateUser
* @see AWS API
* Documentation
*/
@Override
public CreateUserResult createUser(CreateUserRequest request) {
request = beforeClientExecution(request);
return executeCreateUser(request);
}
@SdkInternalApi
final CreateUserResult executeCreateUser(CreateUserRequest createUserRequest) {
ExecutionContext executionContext = createExecutionContext(createUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon Chime Voice Connector under the administrator's AWS account. You can choose to create an Amazon
* Chime Voice Connector in a specific AWS Region.
*
*
* Enabling CreateVoiceConnectorRequest$RequireEncryption configures your Amazon Chime Voice Connector to use
* TLS transport for SIP signaling and Secure RTP (SRTP) for media. Inbound calls use TLS transport, and unencrypted
* outbound calls are blocked.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createVoiceConnectorRequest
* @return Result of the CreateVoiceConnector operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateVoiceConnector
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public CreateVoiceConnectorResult createVoiceConnector(CreateVoiceConnectorRequest request) {
request = beforeClientExecution(request);
return executeCreateVoiceConnector(request);
}
@SdkInternalApi
final CreateVoiceConnectorResult executeCreateVoiceConnector(CreateVoiceConnectorRequest createVoiceConnectorRequest) {
ExecutionContext executionContext = createExecutionContext(createVoiceConnectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateVoiceConnectorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createVoiceConnectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateVoiceConnector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateVoiceConnectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon Chime Voice Connector group under the administrator's AWS account. You can associate Amazon
* Chime Voice Connectors with the Amazon Chime Voice Connector group by including VoiceConnectorItems
* in the request.
*
*
* You can include Amazon Chime Voice Connectors from different AWS Regions in your group. This creates a fault
* tolerant mechanism for fallback in case of availability events.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* CreateVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param createVoiceConnectorGroupRequest
* @return Result of the CreateVoiceConnectorGroup operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have permissions to perform the requested operation.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.CreateVoiceConnectorGroup
* @see AWS API Documentation
*/
@Override
@Deprecated
public CreateVoiceConnectorGroupResult createVoiceConnectorGroup(CreateVoiceConnectorGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateVoiceConnectorGroup(request);
}
@SdkInternalApi
final CreateVoiceConnectorGroupResult executeCreateVoiceConnectorGroup(CreateVoiceConnectorGroupRequest createVoiceConnectorGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createVoiceConnectorGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateVoiceConnectorGroupRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createVoiceConnectorGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateVoiceConnectorGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateVoiceConnectorGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified Amazon Chime account. You must suspend all users before deleting Team
account.
* You can use the BatchSuspendUser action to dodo.
*
*
* For EnterpriseLWA
and EnterpriseAD
accounts, you must release the claimed domains for
* your Amazon Chime account before deletion. As soon as you release the domain, all users under that account are
* suspended.
*
*
* Deleted accounts appear in your Disabled
accounts list for 90 days. To restore deleted account from
* your Disabled
accounts list, you must contact AWS Support.
*
*
* After 90 days, deleted accounts are permanently removed from your Disabled
accounts list.
*
*
* @param deleteAccountRequest
* @return Result of the DeleteAccount operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnprocessableEntityException
* The request was well-formed but was unable to be followed due to semantic errors.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteAccount
* @see AWS API
* Documentation
*/
@Override
public DeleteAccountResult deleteAccount(DeleteAccountRequest request) {
request = beforeClientExecution(request);
return executeDeleteAccount(request);
}
@SdkInternalApi
final DeleteAccountResult executeDeleteAccount(DeleteAccountRequest deleteAccountRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAccountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAccountRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAccountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAccount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAccountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an AppInstance
and all associated data asynchronously.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteAppInstance, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceRequest
* @return Result of the DeleteAppInstance operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteAppInstance
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public DeleteAppInstanceResult deleteAppInstance(DeleteAppInstanceRequest request) {
request = beforeClientExecution(request);
return executeDeleteAppInstance(request);
}
@SdkInternalApi
final DeleteAppInstanceResult executeDeleteAppInstance(DeleteAppInstanceRequest deleteAppInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAppInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAppInstanceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAppInstanceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAppInstance");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "identity-";
String resolvedHostPrefix = String.format("identity-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAppInstanceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Demotes an AppInstanceAdmin
to an AppInstanceUser
. This action does not delete the
* user.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteAppInstanceAdmin, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceAdminRequest
* @return Result of the DeleteAppInstanceAdmin operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteAppInstanceAdmin
* @see AWS
* API Documentation
*/
@Override
@Deprecated
public DeleteAppInstanceAdminResult deleteAppInstanceAdmin(DeleteAppInstanceAdminRequest request) {
request = beforeClientExecution(request);
return executeDeleteAppInstanceAdmin(request);
}
@SdkInternalApi
final DeleteAppInstanceAdminResult executeDeleteAppInstanceAdmin(DeleteAppInstanceAdminRequest deleteAppInstanceAdminRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAppInstanceAdminRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAppInstanceAdminRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAppInstanceAdminRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAppInstanceAdmin");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "identity-";
String resolvedHostPrefix = String.format("identity-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteAppInstanceAdminResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the streaming configurations of an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteAppInstanceStreamingConfigurations, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceStreamingConfigurationsRequest
* @return Result of the DeleteAppInstanceStreamingConfigurations operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteAppInstanceStreamingConfigurations
* @see AWS API Documentation
*/
@Override
@Deprecated
public DeleteAppInstanceStreamingConfigurationsResult deleteAppInstanceStreamingConfigurations(DeleteAppInstanceStreamingConfigurationsRequest request) {
request = beforeClientExecution(request);
return executeDeleteAppInstanceStreamingConfigurations(request);
}
@SdkInternalApi
final DeleteAppInstanceStreamingConfigurationsResult executeDeleteAppInstanceStreamingConfigurations(
DeleteAppInstanceStreamingConfigurationsRequest deleteAppInstanceStreamingConfigurationsRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAppInstanceStreamingConfigurationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAppInstanceStreamingConfigurationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteAppInstanceStreamingConfigurationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAppInstanceStreamingConfigurations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteAppInstanceStreamingConfigurationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an AppInstanceUser
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAppInstanceUserRequest
* @return Result of the DeleteAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteAppInstanceUser
* @see AWS
* API Documentation
*/
@Override
@Deprecated
public DeleteAppInstanceUserResult deleteAppInstanceUser(DeleteAppInstanceUserRequest request) {
request = beforeClientExecution(request);
return executeDeleteAppInstanceUser(request);
}
@SdkInternalApi
final DeleteAppInstanceUserResult executeDeleteAppInstanceUser(DeleteAppInstanceUserRequest deleteAppInstanceUserRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAppInstanceUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAppInstanceUserRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAppInstanceUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAppInstanceUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "identity-";
String resolvedHostPrefix = String.format("identity-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteAppInstanceUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an attendee from the specified Amazon Chime SDK meeting and deletes their JoinToken
.
* Attendees are automatically deleted when a Amazon Chime SDK meeting is deleted. For more information about the
* Amazon Chime SDK, see Using the
* Amazon Chime SDK in the Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteAttendee
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteAttendeeRequest
* @return Result of the DeleteAttendee operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteAttendee
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public DeleteAttendeeResult deleteAttendee(DeleteAttendeeRequest request) {
request = beforeClientExecution(request);
return executeDeleteAttendee(request);
}
@SdkInternalApi
final DeleteAttendeeResult executeDeleteAttendee(DeleteAttendeeRequest deleteAttendeeRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAttendeeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAttendeeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAttendeeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAttendee");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAttendeeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Immediately makes a channel and its memberships inaccessible and marks them for deletion. This is an irreversible
* process.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteChannel
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelRequest
* @return Result of the DeleteChannel operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteChannel
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public DeleteChannelResult deleteChannel(DeleteChannelRequest request) {
request = beforeClientExecution(request);
return executeDeleteChannel(request);
}
@SdkInternalApi
final DeleteChannelResult executeDeleteChannel(DeleteChannelRequest deleteChannelRequest) {
ExecutionContext executionContext = createExecutionContext(deleteChannelRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteChannelRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteChannelRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteChannel");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteChannelResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes a user from a channel's ban list.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelBan, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelBanRequest
* @return Result of the DeleteChannelBan operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteChannelBan
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public DeleteChannelBanResult deleteChannelBan(DeleteChannelBanRequest request) {
request = beforeClientExecution(request);
return executeDeleteChannelBan(request);
}
@SdkInternalApi
final DeleteChannelBanResult executeDeleteChannelBan(DeleteChannelBanRequest deleteChannelBanRequest) {
ExecutionContext executionContext = createExecutionContext(deleteChannelBanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteChannelBanRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteChannelBanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteChannelBan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteChannelBanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes a member from a channel.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelMembershipRequest
* @return Result of the DeleteChannelMembership operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteChannelMembership
* @see AWS
* API Documentation
*/
@Override
@Deprecated
public DeleteChannelMembershipResult deleteChannelMembership(DeleteChannelMembershipRequest request) {
request = beforeClientExecution(request);
return executeDeleteChannelMembership(request);
}
@SdkInternalApi
final DeleteChannelMembershipResult executeDeleteChannelMembership(DeleteChannelMembershipRequest deleteChannelMembershipRequest) {
ExecutionContext executionContext = createExecutionContext(deleteChannelMembershipRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteChannelMembershipRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteChannelMembershipRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteChannelMembership");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteChannelMembershipResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a channel message. Only admins can perform this action. Deletion makes messages inaccessible immediately.
* A background process deletes any revisions created by UpdateChannelMessage
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelMessage, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelMessageRequest
* @return Result of the DeleteChannelMessage operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteChannelMessage
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public DeleteChannelMessageResult deleteChannelMessage(DeleteChannelMessageRequest request) {
request = beforeClientExecution(request);
return executeDeleteChannelMessage(request);
}
@SdkInternalApi
final DeleteChannelMessageResult executeDeleteChannelMessage(DeleteChannelMessageRequest deleteChannelMessageRequest) {
ExecutionContext executionContext = createExecutionContext(deleteChannelMessageRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteChannelMessageRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteChannelMessageRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteChannelMessage");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteChannelMessageResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a channel moderator.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteChannelModerator, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteChannelModeratorRequest
* @return Result of the DeleteChannelModerator operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteChannelModerator
* @see AWS
* API Documentation
*/
@Override
@Deprecated
public DeleteChannelModeratorResult deleteChannelModerator(DeleteChannelModeratorRequest request) {
request = beforeClientExecution(request);
return executeDeleteChannelModerator(request);
}
@SdkInternalApi
final DeleteChannelModeratorResult executeDeleteChannelModerator(DeleteChannelModeratorRequest deleteChannelModeratorRequest) {
ExecutionContext executionContext = createExecutionContext(deleteChannelModeratorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteChannelModeratorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteChannelModeratorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteChannelModerator");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteChannelModeratorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the events configuration that allows a bot to receive outgoing events.
*
*
* @param deleteEventsConfigurationRequest
* @return Result of the DeleteEventsConfiguration operation returned by the service.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @sample AmazonChime.DeleteEventsConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteEventsConfigurationResult deleteEventsConfiguration(DeleteEventsConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteEventsConfiguration(request);
}
@SdkInternalApi
final DeleteEventsConfigurationResult executeDeleteEventsConfiguration(DeleteEventsConfigurationRequest deleteEventsConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteEventsConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteEventsConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteEventsConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteEventsConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteEventsConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the media capture pipeline.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteMediaCapturePipeline, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteMediaCapturePipelineRequest
* @return Result of the DeleteMediaCapturePipeline operation returned by the service.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteMediaCapturePipeline
* @see AWS API Documentation
*/
@Override
@Deprecated
public DeleteMediaCapturePipelineResult deleteMediaCapturePipeline(DeleteMediaCapturePipelineRequest request) {
request = beforeClientExecution(request);
return executeDeleteMediaCapturePipeline(request);
}
@SdkInternalApi
final DeleteMediaCapturePipelineResult executeDeleteMediaCapturePipeline(DeleteMediaCapturePipelineRequest deleteMediaCapturePipelineRequest) {
ExecutionContext executionContext = createExecutionContext(deleteMediaCapturePipelineRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteMediaCapturePipelineRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteMediaCapturePipelineRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteMediaCapturePipeline");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteMediaCapturePipelineResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified Amazon Chime SDK meeting. The operation deletes all attendees, disconnects all clients, and
* prevents new clients from joining the meeting. For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteMeeting
* , in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteMeetingRequest
* @return Result of the DeleteMeeting operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteMeeting
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public DeleteMeetingResult deleteMeeting(DeleteMeetingRequest request) {
request = beforeClientExecution(request);
return executeDeleteMeeting(request);
}
@SdkInternalApi
final DeleteMeetingResult executeDeleteMeeting(DeleteMeetingRequest deleteMeetingRequest) {
ExecutionContext executionContext = createExecutionContext(deleteMeetingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteMeetingRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteMeetingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteMeeting");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteMeetingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Moves the specified phone number into the Deletion queue. A phone number must be disassociated from any
* users or Amazon Chime Voice Connectors before it can be deleted.
*
*
* Deleted phone numbers remain in the Deletion queue for 7 days before they are deleted permanently.
*
*
* @param deletePhoneNumberRequest
* @return Result of the DeletePhoneNumber operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeletePhoneNumber
* @see AWS API
* Documentation
*/
@Override
public DeletePhoneNumberResult deletePhoneNumber(DeletePhoneNumberRequest request) {
request = beforeClientExecution(request);
return executeDeletePhoneNumber(request);
}
@SdkInternalApi
final DeletePhoneNumberResult executeDeletePhoneNumber(DeletePhoneNumberRequest deletePhoneNumberRequest) {
ExecutionContext executionContext = createExecutionContext(deletePhoneNumberRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeletePhoneNumberRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deletePhoneNumberRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeletePhoneNumber");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeletePhoneNumberResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified proxy session from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteProxySession, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteProxySessionRequest
* @return Result of the DeleteProxySession operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteProxySession
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public DeleteProxySessionResult deleteProxySession(DeleteProxySessionRequest request) {
request = beforeClientExecution(request);
return executeDeleteProxySession(request);
}
@SdkInternalApi
final DeleteProxySessionResult executeDeleteProxySession(DeleteProxySessionRequest deleteProxySessionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteProxySessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteProxySessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteProxySessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteProxySession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteProxySessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a chat room in an Amazon Chime Enterprise account.
*
*
* @param deleteRoomRequest
* @return Result of the DeleteRoom operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteRoom
* @see AWS API
* Documentation
*/
@Override
public DeleteRoomResult deleteRoom(DeleteRoomRequest request) {
request = beforeClientExecution(request);
return executeDeleteRoom(request);
}
@SdkInternalApi
final DeleteRoomResult executeDeleteRoom(DeleteRoomRequest deleteRoomRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRoomRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRoomRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteRoomRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteRoom");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteRoomResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes a member from a chat room in an Amazon Chime Enterprise account.
*
*
* @param deleteRoomMembershipRequest
* @return Result of the DeleteRoomMembership operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteRoomMembership
* @see AWS API
* Documentation
*/
@Override
public DeleteRoomMembershipResult deleteRoomMembership(DeleteRoomMembershipRequest request) {
request = beforeClientExecution(request);
return executeDeleteRoomMembership(request);
}
@SdkInternalApi
final DeleteRoomMembershipResult executeDeleteRoomMembership(DeleteRoomMembershipRequest deleteRoomMembershipRequest) {
ExecutionContext executionContext = createExecutionContext(deleteRoomMembershipRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteRoomMembershipRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteRoomMembershipRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteRoomMembership");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteRoomMembershipResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a SIP media application.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteSipMediaApplication, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteSipMediaApplicationRequest
* @return Result of the DeleteSipMediaApplication operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteSipMediaApplication
* @see AWS API Documentation
*/
@Override
@Deprecated
public DeleteSipMediaApplicationResult deleteSipMediaApplication(DeleteSipMediaApplicationRequest request) {
request = beforeClientExecution(request);
return executeDeleteSipMediaApplication(request);
}
@SdkInternalApi
final DeleteSipMediaApplicationResult executeDeleteSipMediaApplication(DeleteSipMediaApplicationRequest deleteSipMediaApplicationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSipMediaApplicationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSipMediaApplicationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteSipMediaApplicationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSipMediaApplication");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteSipMediaApplicationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a SIP rule. You must disable a SIP rule before you can delete it.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteSipRule,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteSipRuleRequest
* @return Result of the DeleteSipRule operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteSipRule
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public DeleteSipRuleResult deleteSipRule(DeleteSipRuleRequest request) {
request = beforeClientExecution(request);
return executeDeleteSipRule(request);
}
@SdkInternalApi
final DeleteSipRuleResult executeDeleteSipRule(DeleteSipRuleRequest deleteSipRuleRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSipRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSipRuleRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteSipRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSipRule");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteSipRuleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified Amazon Chime Voice Connector. Any phone numbers associated with the Amazon Chime Voice
* Connector must be disassociated from it before it can be deleted.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorRequest
* @return Result of the DeleteVoiceConnector operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnector
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public DeleteVoiceConnectorResult deleteVoiceConnector(DeleteVoiceConnectorRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnector(request);
}
@SdkInternalApi
final DeleteVoiceConnectorResult executeDeleteVoiceConnector(DeleteVoiceConnectorRequest deleteVoiceConnectorRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteVoiceConnectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteVoiceConnectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the emergency calling configuration details from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorEmergencyCallingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorEmergencyCallingConfigurationRequest
* @return Result of the DeleteVoiceConnectorEmergencyCallingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnectorEmergencyCallingConfiguration
* @see AWS API Documentation
*/
@Override
@Deprecated
public DeleteVoiceConnectorEmergencyCallingConfigurationResult deleteVoiceConnectorEmergencyCallingConfiguration(
DeleteVoiceConnectorEmergencyCallingConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnectorEmergencyCallingConfiguration(request);
}
@SdkInternalApi
final DeleteVoiceConnectorEmergencyCallingConfigurationResult executeDeleteVoiceConnectorEmergencyCallingConfiguration(
DeleteVoiceConnectorEmergencyCallingConfigurationRequest deleteVoiceConnectorEmergencyCallingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorEmergencyCallingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorEmergencyCallingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVoiceConnectorEmergencyCallingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnectorEmergencyCallingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVoiceConnectorEmergencyCallingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified Amazon Chime Voice Connector group. Any VoiceConnectorItems
and phone numbers
* associated with the group must be removed before it can be deleted.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorGroupRequest
* @return Result of the DeleteVoiceConnectorGroup operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnectorGroup
* @see AWS API Documentation
*/
@Override
@Deprecated
public DeleteVoiceConnectorGroupResult deleteVoiceConnectorGroup(DeleteVoiceConnectorGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnectorGroup(request);
}
@SdkInternalApi
final DeleteVoiceConnectorGroupResult executeDeleteVoiceConnectorGroup(DeleteVoiceConnectorGroupRequest deleteVoiceConnectorGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorGroupRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVoiceConnectorGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnectorGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVoiceConnectorGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the origination settings for the specified Amazon Chime Voice Connector.
*
*
*
* If emergency calling is configured for the Amazon Chime Voice Connector, it must be deleted prior to deleting the
* origination settings.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorOrigination, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorOriginationRequest
* @return Result of the DeleteVoiceConnectorOrigination operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnectorOrigination
* @see AWS API Documentation
*/
@Override
@Deprecated
public DeleteVoiceConnectorOriginationResult deleteVoiceConnectorOrigination(DeleteVoiceConnectorOriginationRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnectorOrigination(request);
}
@SdkInternalApi
final DeleteVoiceConnectorOriginationResult executeDeleteVoiceConnectorOrigination(
DeleteVoiceConnectorOriginationRequest deleteVoiceConnectorOriginationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorOriginationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorOriginationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVoiceConnectorOriginationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnectorOrigination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVoiceConnectorOriginationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the proxy configuration from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DeleteVoiceProxy, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorProxyRequest
* @return Result of the DeleteVoiceConnectorProxy operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnectorProxy
* @see AWS API Documentation
*/
@Override
@Deprecated
public DeleteVoiceConnectorProxyResult deleteVoiceConnectorProxy(DeleteVoiceConnectorProxyRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnectorProxy(request);
}
@SdkInternalApi
final DeleteVoiceConnectorProxyResult executeDeleteVoiceConnectorProxy(DeleteVoiceConnectorProxyRequest deleteVoiceConnectorProxyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorProxyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorProxyRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVoiceConnectorProxyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnectorProxy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVoiceConnectorProxyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the streaming configuration for the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorStreamingConfiguration, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorStreamingConfigurationRequest
* @return Result of the DeleteVoiceConnectorStreamingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnectorStreamingConfiguration
* @see AWS API Documentation
*/
@Override
@Deprecated
public DeleteVoiceConnectorStreamingConfigurationResult deleteVoiceConnectorStreamingConfiguration(DeleteVoiceConnectorStreamingConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnectorStreamingConfiguration(request);
}
@SdkInternalApi
final DeleteVoiceConnectorStreamingConfigurationResult executeDeleteVoiceConnectorStreamingConfiguration(
DeleteVoiceConnectorStreamingConfigurationRequest deleteVoiceConnectorStreamingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorStreamingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorStreamingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVoiceConnectorStreamingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnectorStreamingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVoiceConnectorStreamingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the termination settings for the specified Amazon Chime Voice Connector.
*
*
*
* If emergency calling is configured for the Amazon Chime Voice Connector, it must be deleted prior to deleting the
* termination settings.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorTermination, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorTerminationRequest
* @return Result of the DeleteVoiceConnectorTermination operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnectorTermination
* @see AWS API Documentation
*/
@Override
@Deprecated
public DeleteVoiceConnectorTerminationResult deleteVoiceConnectorTermination(DeleteVoiceConnectorTerminationRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnectorTermination(request);
}
@SdkInternalApi
final DeleteVoiceConnectorTerminationResult executeDeleteVoiceConnectorTermination(
DeleteVoiceConnectorTerminationRequest deleteVoiceConnectorTerminationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorTerminationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorTerminationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVoiceConnectorTerminationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnectorTermination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVoiceConnectorTerminationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified SIP credentials used by your equipment to authenticate during call termination.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DeleteVoiceConnectorTerminationCredentials, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param deleteVoiceConnectorTerminationCredentialsRequest
* @return Result of the DeleteVoiceConnectorTerminationCredentials operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DeleteVoiceConnectorTerminationCredentials
* @see AWS API Documentation
*/
@Override
@Deprecated
public DeleteVoiceConnectorTerminationCredentialsResult deleteVoiceConnectorTerminationCredentials(DeleteVoiceConnectorTerminationCredentialsRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnectorTerminationCredentials(request);
}
@SdkInternalApi
final DeleteVoiceConnectorTerminationCredentialsResult executeDeleteVoiceConnectorTerminationCredentials(
DeleteVoiceConnectorTerminationCredentialsRequest deleteVoiceConnectorTerminationCredentialsRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorTerminationCredentialsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorTerminationCredentialsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVoiceConnectorTerminationCredentialsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnectorTerminationCredentials");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVoiceConnectorTerminationCredentialsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the full details of an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeAppInstance, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeAppInstanceRequest
* @return Result of the DescribeAppInstance operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeAppInstance
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public DescribeAppInstanceResult describeAppInstance(DescribeAppInstanceRequest request) {
request = beforeClientExecution(request);
return executeDescribeAppInstance(request);
}
@SdkInternalApi
final DescribeAppInstanceResult executeDescribeAppInstance(DescribeAppInstanceRequest describeAppInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(describeAppInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAppInstanceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeAppInstanceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAppInstance");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "identity-";
String resolvedHostPrefix = String.format("identity-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeAppInstanceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the full details of an AppInstanceAdmin
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeAppInstanceAdmin, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeAppInstanceAdminRequest
* @return Result of the DescribeAppInstanceAdmin operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeAppInstanceAdmin
* @see AWS
* API Documentation
*/
@Override
@Deprecated
public DescribeAppInstanceAdminResult describeAppInstanceAdmin(DescribeAppInstanceAdminRequest request) {
request = beforeClientExecution(request);
return executeDescribeAppInstanceAdmin(request);
}
@SdkInternalApi
final DescribeAppInstanceAdminResult executeDescribeAppInstanceAdmin(DescribeAppInstanceAdminRequest describeAppInstanceAdminRequest) {
ExecutionContext executionContext = createExecutionContext(describeAppInstanceAdminRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAppInstanceAdminRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeAppInstanceAdminRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAppInstanceAdmin");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "identity-";
String resolvedHostPrefix = String.format("identity-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAppInstanceAdminResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the full details of an AppInstanceUser
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeAppInstanceUserRequest
* @return Result of the DescribeAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeAppInstanceUser
* @see AWS
* API Documentation
*/
@Override
@Deprecated
public DescribeAppInstanceUserResult describeAppInstanceUser(DescribeAppInstanceUserRequest request) {
request = beforeClientExecution(request);
return executeDescribeAppInstanceUser(request);
}
@SdkInternalApi
final DescribeAppInstanceUserResult executeDescribeAppInstanceUser(DescribeAppInstanceUserRequest describeAppInstanceUserRequest) {
ExecutionContext executionContext = createExecutionContext(describeAppInstanceUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAppInstanceUserRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeAppInstanceUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAppInstanceUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "identity-";
String resolvedHostPrefix = String.format("identity-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeAppInstanceUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the full details of a channel in an Amazon Chime AppInstance
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeChannel, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelRequest
* @return Result of the DescribeChannel operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeChannel
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public DescribeChannelResult describeChannel(DescribeChannelRequest request) {
request = beforeClientExecution(request);
return executeDescribeChannel(request);
}
@SdkInternalApi
final DescribeChannelResult executeDescribeChannel(DescribeChannelRequest describeChannelRequest) {
ExecutionContext executionContext = createExecutionContext(describeChannelRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeChannelRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeChannelRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeChannel");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeChannelResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the full details of a channel ban.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version,
* DescribeChannelBan, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelBanRequest
* @return Result of the DescribeChannelBan operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeChannelBan
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public DescribeChannelBanResult describeChannelBan(DescribeChannelBanRequest request) {
request = beforeClientExecution(request);
return executeDescribeChannelBan(request);
}
@SdkInternalApi
final DescribeChannelBanResult executeDescribeChannelBan(DescribeChannelBanRequest describeChannelBanRequest) {
ExecutionContext executionContext = createExecutionContext(describeChannelBanRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeChannelBanRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(describeChannelBanRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeChannelBan");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DescribeChannelBanResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the full details of a user's channel membership.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelMembership, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelMembershipRequest
* @return Result of the DescribeChannelMembership operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeChannelMembership
* @see AWS API Documentation
*/
@Override
@Deprecated
public DescribeChannelMembershipResult describeChannelMembership(DescribeChannelMembershipRequest request) {
request = beforeClientExecution(request);
return executeDescribeChannelMembership(request);
}
@SdkInternalApi
final DescribeChannelMembershipResult executeDescribeChannelMembership(DescribeChannelMembershipRequest describeChannelMembershipRequest) {
ExecutionContext executionContext = createExecutionContext(describeChannelMembershipRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeChannelMembershipRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeChannelMembershipRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeChannelMembership");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeChannelMembershipResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the details of a channel based on the membership of the specified AppInstanceUser
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelMembershipForAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelMembershipForAppInstanceUserRequest
* @return Result of the DescribeChannelMembershipForAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeChannelMembershipForAppInstanceUser
* @see AWS API Documentation
*/
@Override
@Deprecated
public DescribeChannelMembershipForAppInstanceUserResult describeChannelMembershipForAppInstanceUser(
DescribeChannelMembershipForAppInstanceUserRequest request) {
request = beforeClientExecution(request);
return executeDescribeChannelMembershipForAppInstanceUser(request);
}
@SdkInternalApi
final DescribeChannelMembershipForAppInstanceUserResult executeDescribeChannelMembershipForAppInstanceUser(
DescribeChannelMembershipForAppInstanceUserRequest describeChannelMembershipForAppInstanceUserRequest) {
ExecutionContext executionContext = createExecutionContext(describeChannelMembershipForAppInstanceUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeChannelMembershipForAppInstanceUserRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeChannelMembershipForAppInstanceUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeChannelMembershipForAppInstanceUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeChannelMembershipForAppInstanceUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the full details of a channel moderated by the specified AppInstanceUser
.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelModeratedByAppInstanceUser, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelModeratedByAppInstanceUserRequest
* @return Result of the DescribeChannelModeratedByAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeChannelModeratedByAppInstanceUser
* @see AWS API Documentation
*/
@Override
@Deprecated
public DescribeChannelModeratedByAppInstanceUserResult describeChannelModeratedByAppInstanceUser(DescribeChannelModeratedByAppInstanceUserRequest request) {
request = beforeClientExecution(request);
return executeDescribeChannelModeratedByAppInstanceUser(request);
}
@SdkInternalApi
final DescribeChannelModeratedByAppInstanceUserResult executeDescribeChannelModeratedByAppInstanceUser(
DescribeChannelModeratedByAppInstanceUserRequest describeChannelModeratedByAppInstanceUserRequest) {
ExecutionContext executionContext = createExecutionContext(describeChannelModeratedByAppInstanceUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeChannelModeratedByAppInstanceUserRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeChannelModeratedByAppInstanceUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeChannelModeratedByAppInstanceUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeChannelModeratedByAppInstanceUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the full details of a single ChannelModerator.
*
*
*
* The x-amz-chime-bearer
request header is mandatory. Use the AppInstanceUserArn
of the
* user that makes the API call as the value in the header.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DescribeChannelModerator, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param describeChannelModeratorRequest
* @return Result of the DescribeChannelModerator operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DescribeChannelModerator
* @see AWS
* API Documentation
*/
@Override
@Deprecated
public DescribeChannelModeratorResult describeChannelModerator(DescribeChannelModeratorRequest request) {
request = beforeClientExecution(request);
return executeDescribeChannelModerator(request);
}
@SdkInternalApi
final DescribeChannelModeratorResult executeDescribeChannelModerator(DescribeChannelModeratorRequest describeChannelModeratorRequest) {
ExecutionContext executionContext = createExecutionContext(describeChannelModeratorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeChannelModeratorRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(describeChannelModeratorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeChannelModerator");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "messaging-";
String resolvedHostPrefix = String.format("messaging-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DescribeChannelModeratorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates the primary provisioned phone number from the specified Amazon Chime user.
*
*
* @param disassociatePhoneNumberFromUserRequest
* @return Result of the DisassociatePhoneNumberFromUser operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DisassociatePhoneNumberFromUser
* @see AWS API Documentation
*/
@Override
public DisassociatePhoneNumberFromUserResult disassociatePhoneNumberFromUser(DisassociatePhoneNumberFromUserRequest request) {
request = beforeClientExecution(request);
return executeDisassociatePhoneNumberFromUser(request);
}
@SdkInternalApi
final DisassociatePhoneNumberFromUserResult executeDisassociatePhoneNumberFromUser(
DisassociatePhoneNumberFromUserRequest disassociatePhoneNumberFromUserRequest) {
ExecutionContext executionContext = createExecutionContext(disassociatePhoneNumberFromUserRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociatePhoneNumberFromUserRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociatePhoneNumberFromUserRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociatePhoneNumberFromUser");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociatePhoneNumberFromUserResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates the specified phone numbers from the specified Amazon Chime Voice Connector.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DisassociatePhoneNumbersFromVoiceConnector, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param disassociatePhoneNumbersFromVoiceConnectorRequest
* @return Result of the DisassociatePhoneNumbersFromVoiceConnector operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DisassociatePhoneNumbersFromVoiceConnector
* @see AWS API Documentation
*/
@Override
@Deprecated
public DisassociatePhoneNumbersFromVoiceConnectorResult disassociatePhoneNumbersFromVoiceConnector(DisassociatePhoneNumbersFromVoiceConnectorRequest request) {
request = beforeClientExecution(request);
return executeDisassociatePhoneNumbersFromVoiceConnector(request);
}
@SdkInternalApi
final DisassociatePhoneNumbersFromVoiceConnectorResult executeDisassociatePhoneNumbersFromVoiceConnector(
DisassociatePhoneNumbersFromVoiceConnectorRequest disassociatePhoneNumbersFromVoiceConnectorRequest) {
ExecutionContext executionContext = createExecutionContext(disassociatePhoneNumbersFromVoiceConnectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociatePhoneNumbersFromVoiceConnectorRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociatePhoneNumbersFromVoiceConnectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociatePhoneNumbersFromVoiceConnector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociatePhoneNumbersFromVoiceConnectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates the specified phone numbers from the specified Amazon Chime Voice Connector group.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, DisassociatePhoneNumbersFromVoiceConnectorGroup, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param disassociatePhoneNumbersFromVoiceConnectorGroupRequest
* @return Result of the DisassociatePhoneNumbersFromVoiceConnectorGroup operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DisassociatePhoneNumbersFromVoiceConnectorGroup
* @see AWS API Documentation
*/
@Override
@Deprecated
public DisassociatePhoneNumbersFromVoiceConnectorGroupResult disassociatePhoneNumbersFromVoiceConnectorGroup(
DisassociatePhoneNumbersFromVoiceConnectorGroupRequest request) {
request = beforeClientExecution(request);
return executeDisassociatePhoneNumbersFromVoiceConnectorGroup(request);
}
@SdkInternalApi
final DisassociatePhoneNumbersFromVoiceConnectorGroupResult executeDisassociatePhoneNumbersFromVoiceConnectorGroup(
DisassociatePhoneNumbersFromVoiceConnectorGroupRequest disassociatePhoneNumbersFromVoiceConnectorGroupRequest) {
ExecutionContext executionContext = createExecutionContext(disassociatePhoneNumbersFromVoiceConnectorGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociatePhoneNumbersFromVoiceConnectorGroupRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociatePhoneNumbersFromVoiceConnectorGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociatePhoneNumbersFromVoiceConnectorGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociatePhoneNumbersFromVoiceConnectorGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates the specified sign-in delegate groups from the specified Amazon Chime account.
*
*
* @param disassociateSigninDelegateGroupsFromAccountRequest
* @return Result of the DisassociateSigninDelegateGroupsFromAccount operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.DisassociateSigninDelegateGroupsFromAccount
* @see AWS API Documentation
*/
@Override
public DisassociateSigninDelegateGroupsFromAccountResult disassociateSigninDelegateGroupsFromAccount(
DisassociateSigninDelegateGroupsFromAccountRequest request) {
request = beforeClientExecution(request);
return executeDisassociateSigninDelegateGroupsFromAccount(request);
}
@SdkInternalApi
final DisassociateSigninDelegateGroupsFromAccountResult executeDisassociateSigninDelegateGroupsFromAccount(
DisassociateSigninDelegateGroupsFromAccountRequest disassociateSigninDelegateGroupsFromAccountRequest) {
ExecutionContext executionContext = createExecutionContext(disassociateSigninDelegateGroupsFromAccountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociateSigninDelegateGroupsFromAccountRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociateSigninDelegateGroupsFromAccountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociateSigninDelegateGroupsFromAccount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociateSigninDelegateGroupsFromAccountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves details for the specified Amazon Chime account, such as account type and supported licenses.
*
*
* @param getAccountRequest
* @return Result of the GetAccount operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetAccount
* @see AWS API
* Documentation
*/
@Override
public GetAccountResult getAccount(GetAccountRequest request) {
request = beforeClientExecution(request);
return executeGetAccount(request);
}
@SdkInternalApi
final GetAccountResult executeGetAccount(GetAccountRequest getAccountRequest) {
ExecutionContext executionContext = createExecutionContext(getAccountRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAccountRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getAccountRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAccount");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetAccountResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves account settings for the specified Amazon Chime account ID, such as remote control and dialout
* settings. For more information about these settings, see Use the Policies Page in the Amazon Chime
* Administration Guide.
*
*
* @param getAccountSettingsRequest
* @return Result of the GetAccountSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetAccountSettings
* @see AWS API
* Documentation
*/
@Override
public GetAccountSettingsResult getAccountSettings(GetAccountSettingsRequest request) {
request = beforeClientExecution(request);
return executeGetAccountSettings(request);
}
@SdkInternalApi
final GetAccountSettingsResult executeGetAccountSettings(GetAccountSettingsRequest getAccountSettingsRequest) {
ExecutionContext executionContext = createExecutionContext(getAccountSettingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAccountSettingsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getAccountSettingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAccountSettings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetAccountSettingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the retention settings for an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetMessagingRetentionSettings, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getAppInstanceRetentionSettingsRequest
* @return Result of the GetAppInstanceRetentionSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetAppInstanceRetentionSettings
* @see AWS API Documentation
*/
@Override
@Deprecated
public GetAppInstanceRetentionSettingsResult getAppInstanceRetentionSettings(GetAppInstanceRetentionSettingsRequest request) {
request = beforeClientExecution(request);
return executeGetAppInstanceRetentionSettings(request);
}
@SdkInternalApi
final GetAppInstanceRetentionSettingsResult executeGetAppInstanceRetentionSettings(
GetAppInstanceRetentionSettingsRequest getAppInstanceRetentionSettingsRequest) {
ExecutionContext executionContext = createExecutionContext(getAppInstanceRetentionSettingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAppInstanceRetentionSettingsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getAppInstanceRetentionSettingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAppInstanceRetentionSettings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
URI endpointTraitHost = null;
if (!clientConfiguration.isDisableHostPrefixInjection()) {
String hostPrefix = "identity-";
String resolvedHostPrefix = String.format("identity-");
endpointTraitHost = UriResourcePathUtils.updateUriHost(endpoint, resolvedHostPrefix);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetAppInstanceRetentionSettingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext, null, endpointTraitHost);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the streaming settings for an AppInstance
.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetMessagingStreamingConfigurations, in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getAppInstanceStreamingConfigurationsRequest
* @return Result of the GetAppInstanceStreamingConfigurations operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetAppInstanceStreamingConfigurations
* @see AWS API Documentation
*/
@Override
@Deprecated
public GetAppInstanceStreamingConfigurationsResult getAppInstanceStreamingConfigurations(GetAppInstanceStreamingConfigurationsRequest request) {
request = beforeClientExecution(request);
return executeGetAppInstanceStreamingConfigurations(request);
}
@SdkInternalApi
final GetAppInstanceStreamingConfigurationsResult executeGetAppInstanceStreamingConfigurations(
GetAppInstanceStreamingConfigurationsRequest getAppInstanceStreamingConfigurationsRequest) {
ExecutionContext executionContext = createExecutionContext(getAppInstanceStreamingConfigurationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAppInstanceStreamingConfigurationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getAppInstanceStreamingConfigurationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAppInstanceStreamingConfigurations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetAppInstanceStreamingConfigurationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the Amazon Chime SDK attendee details for a specified meeting ID and attendee ID. For more information about
* the Amazon Chime SDK, see Using the
* Amazon Chime SDK in the Amazon Chime SDK Developer Guide.
*
*
*
* This API is is no longer supported and will not be updated. We recommend using the latest version, GetAttendee,
* in the Amazon Chime SDK.
*
*
* Using the latest version requires migrating to a dedicated namespace. For more information, refer to Migrating from the Amazon
* Chime namespace in the Amazon Chime SDK Developer Guide.
*
*
*
* @param getAttendeeRequest
* @return Result of the GetAttendee operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChime.GetAttendee
* @see AWS API
* Documentation
*/
@Override
@Deprecated
public GetAttendeeResult getAttendee(GetAttendeeRequest request) {
request = beforeClientExecution(request);
return executeGetAttendee(request);
}
@SdkInternalApi
final GetAttendeeResult executeGetAttendee(GetAttendeeRequest getAttendeeRequest) {
ExecutionContext executionContext = createExecutionContext(getAttendeeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAttendeeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getAttendeeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAttendee");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetAttendeeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves details for the specified bot, such as bot email address, bot type, status, and display name.
*
*
* @param getBotRequest
* @return Result of the GetBot operation returned by the service.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @sample AmazonChime.GetBot
* @see AWS API
* Documentation
*/
@Override
public GetBotResult getBot(GetBotRequest request) {
request = beforeClientExecution(request);
return executeGetBot(request);
}
@SdkInternalApi
final GetBotResult executeGetBot(GetBotRequest getBotRequest) {
ExecutionContext executionContext = createExecutionContext(getBotRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetBotRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getBotRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetBot");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetBotResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the full details of a channel message.
*
*
*