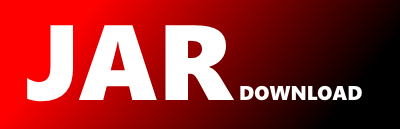
com.amazonaws.services.chime.model.CreateMeetingWithAttendeesRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chime Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chime.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateMeetingWithAttendeesRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The unique identifier for the client request. Use a different token for different meetings.
*
*/
private String clientRequestToken;
/**
*
* The external meeting ID.
*
*/
private String externalMeetingId;
/**
*
* Reserved.
*
*/
private String meetingHostId;
/**
*
* The Region in which to create the meeting. Default: us-east-1
.
*
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
, ca-central-1
,
* eu-central-1
, eu-north-1
, eu-south-1
, eu-west-1
,
* eu-west-2
, eu-west-3
, sa-east-1
, us-east-1
,
* us-east-2
, us-west-1
, us-west-2
.
*
*/
private String mediaRegion;
/**
*
* The tag key-value pairs.
*
*/
private java.util.List tags;
/**
*
* The resource target configurations for receiving Amazon Chime SDK meeting and attendee event notifications. The
* Amazon Chime SDK supports resource targets located in the US East (N. Virginia) AWS Region (us-east-1).
*
*/
private MeetingNotificationConfiguration notificationsConfiguration;
/**
*
* The request containing the attendees to create.
*
*/
private java.util.List attendees;
/**
*
* The unique identifier for the client request. Use a different token for different meetings.
*
*
* @param clientRequestToken
* The unique identifier for the client request. Use a different token for different meetings.
*/
public void setClientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
}
/**
*
* The unique identifier for the client request. Use a different token for different meetings.
*
*
* @return The unique identifier for the client request. Use a different token for different meetings.
*/
public String getClientRequestToken() {
return this.clientRequestToken;
}
/**
*
* The unique identifier for the client request. Use a different token for different meetings.
*
*
* @param clientRequestToken
* The unique identifier for the client request. Use a different token for different meetings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withClientRequestToken(String clientRequestToken) {
setClientRequestToken(clientRequestToken);
return this;
}
/**
*
* The external meeting ID.
*
*
* @param externalMeetingId
* The external meeting ID.
*/
public void setExternalMeetingId(String externalMeetingId) {
this.externalMeetingId = externalMeetingId;
}
/**
*
* The external meeting ID.
*
*
* @return The external meeting ID.
*/
public String getExternalMeetingId() {
return this.externalMeetingId;
}
/**
*
* The external meeting ID.
*
*
* @param externalMeetingId
* The external meeting ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withExternalMeetingId(String externalMeetingId) {
setExternalMeetingId(externalMeetingId);
return this;
}
/**
*
* Reserved.
*
*
* @param meetingHostId
* Reserved.
*/
public void setMeetingHostId(String meetingHostId) {
this.meetingHostId = meetingHostId;
}
/**
*
* Reserved.
*
*
* @return Reserved.
*/
public String getMeetingHostId() {
return this.meetingHostId;
}
/**
*
* Reserved.
*
*
* @param meetingHostId
* Reserved.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withMeetingHostId(String meetingHostId) {
setMeetingHostId(meetingHostId);
return this;
}
/**
*
* The Region in which to create the meeting. Default: us-east-1
.
*
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
, ca-central-1
,
* eu-central-1
, eu-north-1
, eu-south-1
, eu-west-1
,
* eu-west-2
, eu-west-3
, sa-east-1
, us-east-1
,
* us-east-2
, us-west-1
, us-west-2
.
*
*
* @param mediaRegion
* The Region in which to create the meeting. Default: us-east-1
.
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
,
* ca-central-1
, eu-central-1
, eu-north-1
, eu-south-1
* , eu-west-1
, eu-west-2
, eu-west-3
, sa-east-1
,
* us-east-1
, us-east-2
, us-west-1
, us-west-2
.
*/
public void setMediaRegion(String mediaRegion) {
this.mediaRegion = mediaRegion;
}
/**
*
* The Region in which to create the meeting. Default: us-east-1
.
*
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
, ca-central-1
,
* eu-central-1
, eu-north-1
, eu-south-1
, eu-west-1
,
* eu-west-2
, eu-west-3
, sa-east-1
, us-east-1
,
* us-east-2
, us-west-1
, us-west-2
.
*
*
* @return The Region in which to create the meeting. Default: us-east-1
.
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
,
* ca-central-1
, eu-central-1
, eu-north-1
, eu-south-1
* , eu-west-1
, eu-west-2
, eu-west-3
, sa-east-1
,
* us-east-1
, us-east-2
, us-west-1
, us-west-2
.
*/
public String getMediaRegion() {
return this.mediaRegion;
}
/**
*
* The Region in which to create the meeting. Default: us-east-1
.
*
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
, ca-central-1
,
* eu-central-1
, eu-north-1
, eu-south-1
, eu-west-1
,
* eu-west-2
, eu-west-3
, sa-east-1
, us-east-1
,
* us-east-2
, us-west-1
, us-west-2
.
*
*
* @param mediaRegion
* The Region in which to create the meeting. Default: us-east-1
.
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
,
* ca-central-1
, eu-central-1
, eu-north-1
, eu-south-1
* , eu-west-1
, eu-west-2
, eu-west-3
, sa-east-1
,
* us-east-1
, us-east-2
, us-west-1
, us-west-2
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withMediaRegion(String mediaRegion) {
setMediaRegion(mediaRegion);
return this;
}
/**
*
* The tag key-value pairs.
*
*
* @return The tag key-value pairs.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* The tag key-value pairs.
*
*
* @param tags
* The tag key-value pairs.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* The tag key-value pairs.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The tag key-value pairs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The tag key-value pairs.
*
*
* @param tags
* The tag key-value pairs.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The resource target configurations for receiving Amazon Chime SDK meeting and attendee event notifications. The
* Amazon Chime SDK supports resource targets located in the US East (N. Virginia) AWS Region (us-east-1).
*
*
* @param notificationsConfiguration
* The resource target configurations for receiving Amazon Chime SDK meeting and attendee event
* notifications. The Amazon Chime SDK supports resource targets located in the US East (N. Virginia) AWS
* Region (us-east-1).
*/
public void setNotificationsConfiguration(MeetingNotificationConfiguration notificationsConfiguration) {
this.notificationsConfiguration = notificationsConfiguration;
}
/**
*
* The resource target configurations for receiving Amazon Chime SDK meeting and attendee event notifications. The
* Amazon Chime SDK supports resource targets located in the US East (N. Virginia) AWS Region (us-east-1).
*
*
* @return The resource target configurations for receiving Amazon Chime SDK meeting and attendee event
* notifications. The Amazon Chime SDK supports resource targets located in the US East (N. Virginia) AWS
* Region (us-east-1).
*/
public MeetingNotificationConfiguration getNotificationsConfiguration() {
return this.notificationsConfiguration;
}
/**
*
* The resource target configurations for receiving Amazon Chime SDK meeting and attendee event notifications. The
* Amazon Chime SDK supports resource targets located in the US East (N. Virginia) AWS Region (us-east-1).
*
*
* @param notificationsConfiguration
* The resource target configurations for receiving Amazon Chime SDK meeting and attendee event
* notifications. The Amazon Chime SDK supports resource targets located in the US East (N. Virginia) AWS
* Region (us-east-1).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withNotificationsConfiguration(MeetingNotificationConfiguration notificationsConfiguration) {
setNotificationsConfiguration(notificationsConfiguration);
return this;
}
/**
*
* The request containing the attendees to create.
*
*
* @return The request containing the attendees to create.
*/
public java.util.List getAttendees() {
return attendees;
}
/**
*
* The request containing the attendees to create.
*
*
* @param attendees
* The request containing the attendees to create.
*/
public void setAttendees(java.util.Collection attendees) {
if (attendees == null) {
this.attendees = null;
return;
}
this.attendees = new java.util.ArrayList(attendees);
}
/**
*
* The request containing the attendees to create.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAttendees(java.util.Collection)} or {@link #withAttendees(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param attendees
* The request containing the attendees to create.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withAttendees(CreateAttendeeRequestItem... attendees) {
if (this.attendees == null) {
setAttendees(new java.util.ArrayList(attendees.length));
}
for (CreateAttendeeRequestItem ele : attendees) {
this.attendees.add(ele);
}
return this;
}
/**
*
* The request containing the attendees to create.
*
*
* @param attendees
* The request containing the attendees to create.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withAttendees(java.util.Collection attendees) {
setAttendees(attendees);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getClientRequestToken() != null)
sb.append("ClientRequestToken: ").append("***Sensitive Data Redacted***").append(",");
if (getExternalMeetingId() != null)
sb.append("ExternalMeetingId: ").append("***Sensitive Data Redacted***").append(",");
if (getMeetingHostId() != null)
sb.append("MeetingHostId: ").append("***Sensitive Data Redacted***").append(",");
if (getMediaRegion() != null)
sb.append("MediaRegion: ").append(getMediaRegion()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getNotificationsConfiguration() != null)
sb.append("NotificationsConfiguration: ").append(getNotificationsConfiguration()).append(",");
if (getAttendees() != null)
sb.append("Attendees: ").append(getAttendees());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateMeetingWithAttendeesRequest == false)
return false;
CreateMeetingWithAttendeesRequest other = (CreateMeetingWithAttendeesRequest) obj;
if (other.getClientRequestToken() == null ^ this.getClientRequestToken() == null)
return false;
if (other.getClientRequestToken() != null && other.getClientRequestToken().equals(this.getClientRequestToken()) == false)
return false;
if (other.getExternalMeetingId() == null ^ this.getExternalMeetingId() == null)
return false;
if (other.getExternalMeetingId() != null && other.getExternalMeetingId().equals(this.getExternalMeetingId()) == false)
return false;
if (other.getMeetingHostId() == null ^ this.getMeetingHostId() == null)
return false;
if (other.getMeetingHostId() != null && other.getMeetingHostId().equals(this.getMeetingHostId()) == false)
return false;
if (other.getMediaRegion() == null ^ this.getMediaRegion() == null)
return false;
if (other.getMediaRegion() != null && other.getMediaRegion().equals(this.getMediaRegion()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getNotificationsConfiguration() == null ^ this.getNotificationsConfiguration() == null)
return false;
if (other.getNotificationsConfiguration() != null && other.getNotificationsConfiguration().equals(this.getNotificationsConfiguration()) == false)
return false;
if (other.getAttendees() == null ^ this.getAttendees() == null)
return false;
if (other.getAttendees() != null && other.getAttendees().equals(this.getAttendees()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getClientRequestToken() == null) ? 0 : getClientRequestToken().hashCode());
hashCode = prime * hashCode + ((getExternalMeetingId() == null) ? 0 : getExternalMeetingId().hashCode());
hashCode = prime * hashCode + ((getMeetingHostId() == null) ? 0 : getMeetingHostId().hashCode());
hashCode = prime * hashCode + ((getMediaRegion() == null) ? 0 : getMediaRegion().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getNotificationsConfiguration() == null) ? 0 : getNotificationsConfiguration().hashCode());
hashCode = prime * hashCode + ((getAttendees() == null) ? 0 : getAttendees().hashCode());
return hashCode;
}
@Override
public CreateMeetingWithAttendeesRequest clone() {
return (CreateMeetingWithAttendeesRequest) super.clone();
}
}