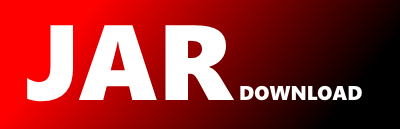
com.amazonaws.services.chime.model.EngineTranscribeSettings Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chime Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chime.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* Settings specific for Amazon Transcribe as the live transcription engine.
*
*
* If you specify an invalid combination of parameters, a TranscriptFailed
event will be sent with the
* contents of the BadRequestException
generated by Amazon Transcribe. For more information on each
* parameter and which combinations are valid, refer to the StartStreamTranscription API in the Amazon Transcribe Developer Guide.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class EngineTranscribeSettings implements Serializable, Cloneable, StructuredPojo {
/**
*
* Specify the language code that represents the language spoken.
*
*
* If you're unsure of the language spoken in your audio, consider using IdentifyLanguage
to enable
* automatic language identification.
*
*/
private String languageCode;
/**
*
* Specify how you want your vocabulary filter applied to your transcript.
*
*
* To replace words with ***
, choose mask
.
*
*
* To delete words, choose remove
.
*
*
* To flag words without changing them, choose tag
.
*
*/
private String vocabularyFilterMethod;
/**
*
* Specify the name of the custom vocabulary filter that you want to use when processing your transcription. Note
* that vocabulary filter names are case sensitive.
*
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary filter must be available in Amazon Transcribe in
* each Region.
*
*
* If you include IdentifyLanguage
and want to use one or more vocabulary filters with your
* transcription, use the VocabularyFilterNames
parameter instead.
*
*/
private String vocabularyFilterName;
/**
*
* Specify the name of the custom vocabulary that you want to use when processing your transcription. Note that
* vocabulary names are case sensitive.
*
*
* If you use Amazon Transcribe multiple Regions, the vocabulary must be available in Amazon Transcribe in each
* Region.
*
*
* If you include IdentifyLanguage
and want to use one or more custom vocabularies with your
* transcription, use the VocabularyNames
parameter instead.
*
*/
private String vocabularyName;
/**
*
* The AWS Region in which to use Amazon Transcribe.
*
*
* If you don't specify a Region, then the MediaRegion
parameter of the CreateMeeting.html API will be used. However, if Amazon Transcribe is not available in the
* MediaRegion
, then a TranscriptFailed event is sent.
*
*
* Use auto
to use Amazon Transcribe in a Region near the meeting’s MediaRegion
. For more
* information, refer to Choosing a
* transcription Region in the Amazon Chime SDK Developer Guide.
*
*/
private String region;
/**
*
* Enables partial result stabilization for your transcription. Partial result stabilization can reduce latency in
* your output, but may impact accuracy.
*
*/
private Boolean enablePartialResultsStabilization;
/**
*
* Specify the level of stability to use when you enable partial results stabilization (
* EnablePartialResultsStabilization
).
*
*
* Low stability provides the highest accuracy. High stability transcribes faster, but with slightly lower accuracy.
*
*/
private String partialResultsStability;
/**
*
* Labels all personally identifiable information (PII) identified in your transcript. If you don't include
* PiiEntityTypes
, all PII is identified.
*
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
*
*/
private String contentIdentificationType;
/**
*
* Content redaction is performed at the segment level. If you don't include PiiEntityTypes
, all PII is
* redacted.
*
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
*
*/
private String contentRedactionType;
/**
*
* Specify which types of personally identifiable information (PII) you want to redact in your transcript. You can
* include as many types as you'd like, or you can select ALL
.
*
*
* Values must be comma-separated and can include: ADDRESS
, BANK_ACCOUNT_NUMBER
,
* BANK_ROUTING
, CREDIT_DEBIT_CVV
, CREDIT_DEBIT_EXPIRY
* CREDIT_DEBIT_NUMBER
, EMAIL
,NAME
, PHONE
, PIN
,
* SSN
, or ALL
.
*
*
* Note that if you include PiiEntityTypes
, you must also include
* ContentIdentificationType
or ContentRedactionType
.
*
*
* If you include ContentRedactionType
or ContentIdentificationType
, but do not include
* PiiEntityTypes
, all PII is redacted or identified.
*
*/
private String piiEntityTypes;
/**
*
* Specify the name of the custom language model that you want to use when processing your transcription. Note that
* language model names are case sensitive.
*
*
* The language of the specified language model must match the language code. If the languages don't match, the
* custom language model isn't applied. There are no errors or warnings associated with a language mismatch.
*
*
* If you use Amazon Transcribe in multiple Regions, the custom language model must be available in Amazon
* Transcribe in each Region.
*
*/
private String languageModelName;
/**
*
* Enables automatic language identification for your transcription.
*
*
* If you include IdentifyLanguage
, you can optionally use LanguageOptions
to include a
* list of language codes that you think may be present in your audio stream. Including language options can improve
* transcription accuracy.
*
*
* You can also use PreferredLanguage
to include a preferred language. Doing so can help Amazon
* Transcribe identify the language faster.
*
*
* You must include either LanguageCode
or IdentifyLanguage
.
*
*
* Language identification can't be combined with custom language models or redaction.
*
*/
private Boolean identifyLanguage;
/**
*
* Specify two or more language codes that represent the languages you think may be present in your media; including
* more than five is not recommended. If you're unsure what languages are present, do not include this parameter.
*
*
* Including language options can improve the accuracy of language identification.
*
*
* If you include LanguageOptions
, you must also include IdentifyLanguage
.
*
*
*
* You can only include one language dialect per language. For example, you cannot include en-US
and
* en-AU
.
*
*
*/
private String languageOptions;
/**
*
* Specify a preferred language from the subset of languages codes you specified in LanguageOptions
.
*
*
* You can only use this parameter if you include IdentifyLanguage
and LanguageOptions
.
*
*/
private String preferredLanguage;
/**
*
* Specify the names of the custom vocabularies that you want to use when processing your transcription. Note that
* vocabulary names are case sensitive.
*
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary must be available in Amazon Transcribe in each
* Region.
*
*
* If you don't include IdentifyLanguage
and want to use a custom vocabulary with your transcription,
* use the VocabularyName
parameter instead.
*
*/
private String vocabularyNames;
/**
*
* Specify the names of the custom vocabulary filters that you want to use when processing your transcription. Note
* that vocabulary filter names are case sensitive.
*
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary filter must be available in Amazon Transcribe in
* each Region.
*
*
* If you're not including IdentifyLanguage
and want to use a custom vocabulary filter with your
* transcription, use the VocabularyFilterName
parameter instead.
*
*/
private String vocabularyFilterNames;
/**
*
* Specify the language code that represents the language spoken.
*
*
* If you're unsure of the language spoken in your audio, consider using IdentifyLanguage
to enable
* automatic language identification.
*
*
* @param languageCode
* Specify the language code that represents the language spoken.
*
* If you're unsure of the language spoken in your audio, consider using IdentifyLanguage
to
* enable automatic language identification.
* @see TranscribeLanguageCode
*/
public void setLanguageCode(String languageCode) {
this.languageCode = languageCode;
}
/**
*
* Specify the language code that represents the language spoken.
*
*
* If you're unsure of the language spoken in your audio, consider using IdentifyLanguage
to enable
* automatic language identification.
*
*
* @return Specify the language code that represents the language spoken.
*
* If you're unsure of the language spoken in your audio, consider using IdentifyLanguage
to
* enable automatic language identification.
* @see TranscribeLanguageCode
*/
public String getLanguageCode() {
return this.languageCode;
}
/**
*
* Specify the language code that represents the language spoken.
*
*
* If you're unsure of the language spoken in your audio, consider using IdentifyLanguage
to enable
* automatic language identification.
*
*
* @param languageCode
* Specify the language code that represents the language spoken.
*
* If you're unsure of the language spoken in your audio, consider using IdentifyLanguage
to
* enable automatic language identification.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TranscribeLanguageCode
*/
public EngineTranscribeSettings withLanguageCode(String languageCode) {
setLanguageCode(languageCode);
return this;
}
/**
*
* Specify the language code that represents the language spoken.
*
*
* If you're unsure of the language spoken in your audio, consider using IdentifyLanguage
to enable
* automatic language identification.
*
*
* @param languageCode
* Specify the language code that represents the language spoken.
*
* If you're unsure of the language spoken in your audio, consider using IdentifyLanguage
to
* enable automatic language identification.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TranscribeLanguageCode
*/
public EngineTranscribeSettings withLanguageCode(TranscribeLanguageCode languageCode) {
this.languageCode = languageCode.toString();
return this;
}
/**
*
* Specify how you want your vocabulary filter applied to your transcript.
*
*
* To replace words with ***
, choose mask
.
*
*
* To delete words, choose remove
.
*
*
* To flag words without changing them, choose tag
.
*
*
* @param vocabularyFilterMethod
* Specify how you want your vocabulary filter applied to your transcript.
*
* To replace words with ***
, choose mask
.
*
*
* To delete words, choose remove
.
*
*
* To flag words without changing them, choose tag
.
* @see TranscribeVocabularyFilterMethod
*/
public void setVocabularyFilterMethod(String vocabularyFilterMethod) {
this.vocabularyFilterMethod = vocabularyFilterMethod;
}
/**
*
* Specify how you want your vocabulary filter applied to your transcript.
*
*
* To replace words with ***
, choose mask
.
*
*
* To delete words, choose remove
.
*
*
* To flag words without changing them, choose tag
.
*
*
* @return Specify how you want your vocabulary filter applied to your transcript.
*
* To replace words with ***
, choose mask
.
*
*
* To delete words, choose remove
.
*
*
* To flag words without changing them, choose tag
.
* @see TranscribeVocabularyFilterMethod
*/
public String getVocabularyFilterMethod() {
return this.vocabularyFilterMethod;
}
/**
*
* Specify how you want your vocabulary filter applied to your transcript.
*
*
* To replace words with ***
, choose mask
.
*
*
* To delete words, choose remove
.
*
*
* To flag words without changing them, choose tag
.
*
*
* @param vocabularyFilterMethod
* Specify how you want your vocabulary filter applied to your transcript.
*
* To replace words with ***
, choose mask
.
*
*
* To delete words, choose remove
.
*
*
* To flag words without changing them, choose tag
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TranscribeVocabularyFilterMethod
*/
public EngineTranscribeSettings withVocabularyFilterMethod(String vocabularyFilterMethod) {
setVocabularyFilterMethod(vocabularyFilterMethod);
return this;
}
/**
*
* Specify how you want your vocabulary filter applied to your transcript.
*
*
* To replace words with ***
, choose mask
.
*
*
* To delete words, choose remove
.
*
*
* To flag words without changing them, choose tag
.
*
*
* @param vocabularyFilterMethod
* Specify how you want your vocabulary filter applied to your transcript.
*
* To replace words with ***
, choose mask
.
*
*
* To delete words, choose remove
.
*
*
* To flag words without changing them, choose tag
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TranscribeVocabularyFilterMethod
*/
public EngineTranscribeSettings withVocabularyFilterMethod(TranscribeVocabularyFilterMethod vocabularyFilterMethod) {
this.vocabularyFilterMethod = vocabularyFilterMethod.toString();
return this;
}
/**
*
* Specify the name of the custom vocabulary filter that you want to use when processing your transcription. Note
* that vocabulary filter names are case sensitive.
*
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary filter must be available in Amazon Transcribe in
* each Region.
*
*
* If you include IdentifyLanguage
and want to use one or more vocabulary filters with your
* transcription, use the VocabularyFilterNames
parameter instead.
*
*
* @param vocabularyFilterName
* Specify the name of the custom vocabulary filter that you want to use when processing your transcription.
* Note that vocabulary filter names are case sensitive.
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary filter must be available in Amazon
* Transcribe in each Region.
*
*
* If you include IdentifyLanguage
and want to use one or more vocabulary filters with your
* transcription, use the VocabularyFilterNames
parameter instead.
*/
public void setVocabularyFilterName(String vocabularyFilterName) {
this.vocabularyFilterName = vocabularyFilterName;
}
/**
*
* Specify the name of the custom vocabulary filter that you want to use when processing your transcription. Note
* that vocabulary filter names are case sensitive.
*
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary filter must be available in Amazon Transcribe in
* each Region.
*
*
* If you include IdentifyLanguage
and want to use one or more vocabulary filters with your
* transcription, use the VocabularyFilterNames
parameter instead.
*
*
* @return Specify the name of the custom vocabulary filter that you want to use when processing your transcription.
* Note that vocabulary filter names are case sensitive.
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary filter must be available in Amazon
* Transcribe in each Region.
*
*
* If you include IdentifyLanguage
and want to use one or more vocabulary filters with your
* transcription, use the VocabularyFilterNames
parameter instead.
*/
public String getVocabularyFilterName() {
return this.vocabularyFilterName;
}
/**
*
* Specify the name of the custom vocabulary filter that you want to use when processing your transcription. Note
* that vocabulary filter names are case sensitive.
*
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary filter must be available in Amazon Transcribe in
* each Region.
*
*
* If you include IdentifyLanguage
and want to use one or more vocabulary filters with your
* transcription, use the VocabularyFilterNames
parameter instead.
*
*
* @param vocabularyFilterName
* Specify the name of the custom vocabulary filter that you want to use when processing your transcription.
* Note that vocabulary filter names are case sensitive.
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary filter must be available in Amazon
* Transcribe in each Region.
*
*
* If you include IdentifyLanguage
and want to use one or more vocabulary filters with your
* transcription, use the VocabularyFilterNames
parameter instead.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EngineTranscribeSettings withVocabularyFilterName(String vocabularyFilterName) {
setVocabularyFilterName(vocabularyFilterName);
return this;
}
/**
*
* Specify the name of the custom vocabulary that you want to use when processing your transcription. Note that
* vocabulary names are case sensitive.
*
*
* If you use Amazon Transcribe multiple Regions, the vocabulary must be available in Amazon Transcribe in each
* Region.
*
*
* If you include IdentifyLanguage
and want to use one or more custom vocabularies with your
* transcription, use the VocabularyNames
parameter instead.
*
*
* @param vocabularyName
* Specify the name of the custom vocabulary that you want to use when processing your transcription. Note
* that vocabulary names are case sensitive.
*
* If you use Amazon Transcribe multiple Regions, the vocabulary must be available in Amazon Transcribe in
* each Region.
*
*
* If you include IdentifyLanguage
and want to use one or more custom vocabularies with your
* transcription, use the VocabularyNames
parameter instead.
*/
public void setVocabularyName(String vocabularyName) {
this.vocabularyName = vocabularyName;
}
/**
*
* Specify the name of the custom vocabulary that you want to use when processing your transcription. Note that
* vocabulary names are case sensitive.
*
*
* If you use Amazon Transcribe multiple Regions, the vocabulary must be available in Amazon Transcribe in each
* Region.
*
*
* If you include IdentifyLanguage
and want to use one or more custom vocabularies with your
* transcription, use the VocabularyNames
parameter instead.
*
*
* @return Specify the name of the custom vocabulary that you want to use when processing your transcription. Note
* that vocabulary names are case sensitive.
*
* If you use Amazon Transcribe multiple Regions, the vocabulary must be available in Amazon Transcribe in
* each Region.
*
*
* If you include IdentifyLanguage
and want to use one or more custom vocabularies with your
* transcription, use the VocabularyNames
parameter instead.
*/
public String getVocabularyName() {
return this.vocabularyName;
}
/**
*
* Specify the name of the custom vocabulary that you want to use when processing your transcription. Note that
* vocabulary names are case sensitive.
*
*
* If you use Amazon Transcribe multiple Regions, the vocabulary must be available in Amazon Transcribe in each
* Region.
*
*
* If you include IdentifyLanguage
and want to use one or more custom vocabularies with your
* transcription, use the VocabularyNames
parameter instead.
*
*
* @param vocabularyName
* Specify the name of the custom vocabulary that you want to use when processing your transcription. Note
* that vocabulary names are case sensitive.
*
* If you use Amazon Transcribe multiple Regions, the vocabulary must be available in Amazon Transcribe in
* each Region.
*
*
* If you include IdentifyLanguage
and want to use one or more custom vocabularies with your
* transcription, use the VocabularyNames
parameter instead.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EngineTranscribeSettings withVocabularyName(String vocabularyName) {
setVocabularyName(vocabularyName);
return this;
}
/**
*
* The AWS Region in which to use Amazon Transcribe.
*
*
* If you don't specify a Region, then the MediaRegion
parameter of the CreateMeeting.html API will be used. However, if Amazon Transcribe is not available in the
* MediaRegion
, then a TranscriptFailed event is sent.
*
*
* Use auto
to use Amazon Transcribe in a Region near the meeting’s MediaRegion
. For more
* information, refer to Choosing a
* transcription Region in the Amazon Chime SDK Developer Guide.
*
*
* @param region
* The AWS Region in which to use Amazon Transcribe.
*
* If you don't specify a Region, then the MediaRegion
parameter of the CreateMeeting.html API will be used. However, if Amazon Transcribe is not available in the
* MediaRegion
, then a TranscriptFailed event is sent.
*
*
* Use auto
to use Amazon Transcribe in a Region near the meeting’s MediaRegion
.
* For more information, refer to Choosing a
* transcription Region in the Amazon Chime SDK Developer Guide.
* @see TranscribeRegion
*/
public void setRegion(String region) {
this.region = region;
}
/**
*
* The AWS Region in which to use Amazon Transcribe.
*
*
* If you don't specify a Region, then the MediaRegion
parameter of the CreateMeeting.html API will be used. However, if Amazon Transcribe is not available in the
* MediaRegion
, then a TranscriptFailed event is sent.
*
*
* Use auto
to use Amazon Transcribe in a Region near the meeting’s MediaRegion
. For more
* information, refer to Choosing a
* transcription Region in the Amazon Chime SDK Developer Guide.
*
*
* @return The AWS Region in which to use Amazon Transcribe.
*
* If you don't specify a Region, then the MediaRegion
parameter of the CreateMeeting.html API will be used. However, if Amazon Transcribe is not available in the
* MediaRegion
, then a TranscriptFailed event is sent.
*
*
* Use auto
to use Amazon Transcribe in a Region near the meeting’s MediaRegion
.
* For more information, refer to Choosing
* a transcription Region in the Amazon Chime SDK Developer Guide.
* @see TranscribeRegion
*/
public String getRegion() {
return this.region;
}
/**
*
* The AWS Region in which to use Amazon Transcribe.
*
*
* If you don't specify a Region, then the MediaRegion
parameter of the CreateMeeting.html API will be used. However, if Amazon Transcribe is not available in the
* MediaRegion
, then a TranscriptFailed event is sent.
*
*
* Use auto
to use Amazon Transcribe in a Region near the meeting’s MediaRegion
. For more
* information, refer to Choosing a
* transcription Region in the Amazon Chime SDK Developer Guide.
*
*
* @param region
* The AWS Region in which to use Amazon Transcribe.
*
* If you don't specify a Region, then the MediaRegion
parameter of the CreateMeeting.html API will be used. However, if Amazon Transcribe is not available in the
* MediaRegion
, then a TranscriptFailed event is sent.
*
*
* Use auto
to use Amazon Transcribe in a Region near the meeting’s MediaRegion
.
* For more information, refer to Choosing a
* transcription Region in the Amazon Chime SDK Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TranscribeRegion
*/
public EngineTranscribeSettings withRegion(String region) {
setRegion(region);
return this;
}
/**
*
* The AWS Region in which to use Amazon Transcribe.
*
*
* If you don't specify a Region, then the MediaRegion
parameter of the CreateMeeting.html API will be used. However, if Amazon Transcribe is not available in the
* MediaRegion
, then a TranscriptFailed event is sent.
*
*
* Use auto
to use Amazon Transcribe in a Region near the meeting’s MediaRegion
. For more
* information, refer to Choosing a
* transcription Region in the Amazon Chime SDK Developer Guide.
*
*
* @param region
* The AWS Region in which to use Amazon Transcribe.
*
* If you don't specify a Region, then the MediaRegion
parameter of the CreateMeeting.html API will be used. However, if Amazon Transcribe is not available in the
* MediaRegion
, then a TranscriptFailed event is sent.
*
*
* Use auto
to use Amazon Transcribe in a Region near the meeting’s MediaRegion
.
* For more information, refer to Choosing a
* transcription Region in the Amazon Chime SDK Developer Guide.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TranscribeRegion
*/
public EngineTranscribeSettings withRegion(TranscribeRegion region) {
this.region = region.toString();
return this;
}
/**
*
* Enables partial result stabilization for your transcription. Partial result stabilization can reduce latency in
* your output, but may impact accuracy.
*
*
* @param enablePartialResultsStabilization
* Enables partial result stabilization for your transcription. Partial result stabilization can reduce
* latency in your output, but may impact accuracy.
*/
public void setEnablePartialResultsStabilization(Boolean enablePartialResultsStabilization) {
this.enablePartialResultsStabilization = enablePartialResultsStabilization;
}
/**
*
* Enables partial result stabilization for your transcription. Partial result stabilization can reduce latency in
* your output, but may impact accuracy.
*
*
* @return Enables partial result stabilization for your transcription. Partial result stabilization can reduce
* latency in your output, but may impact accuracy.
*/
public Boolean getEnablePartialResultsStabilization() {
return this.enablePartialResultsStabilization;
}
/**
*
* Enables partial result stabilization for your transcription. Partial result stabilization can reduce latency in
* your output, but may impact accuracy.
*
*
* @param enablePartialResultsStabilization
* Enables partial result stabilization for your transcription. Partial result stabilization can reduce
* latency in your output, but may impact accuracy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EngineTranscribeSettings withEnablePartialResultsStabilization(Boolean enablePartialResultsStabilization) {
setEnablePartialResultsStabilization(enablePartialResultsStabilization);
return this;
}
/**
*
* Enables partial result stabilization for your transcription. Partial result stabilization can reduce latency in
* your output, but may impact accuracy.
*
*
* @return Enables partial result stabilization for your transcription. Partial result stabilization can reduce
* latency in your output, but may impact accuracy.
*/
public Boolean isEnablePartialResultsStabilization() {
return this.enablePartialResultsStabilization;
}
/**
*
* Specify the level of stability to use when you enable partial results stabilization (
* EnablePartialResultsStabilization
).
*
*
* Low stability provides the highest accuracy. High stability transcribes faster, but with slightly lower accuracy.
*
*
* @param partialResultsStability
* Specify the level of stability to use when you enable partial results stabilization (
* EnablePartialResultsStabilization
).
*
* Low stability provides the highest accuracy. High stability transcribes faster, but with slightly lower
* accuracy.
* @see TranscribePartialResultsStability
*/
public void setPartialResultsStability(String partialResultsStability) {
this.partialResultsStability = partialResultsStability;
}
/**
*
* Specify the level of stability to use when you enable partial results stabilization (
* EnablePartialResultsStabilization
).
*
*
* Low stability provides the highest accuracy. High stability transcribes faster, but with slightly lower accuracy.
*
*
* @return Specify the level of stability to use when you enable partial results stabilization (
* EnablePartialResultsStabilization
).
*
* Low stability provides the highest accuracy. High stability transcribes faster, but with slightly lower
* accuracy.
* @see TranscribePartialResultsStability
*/
public String getPartialResultsStability() {
return this.partialResultsStability;
}
/**
*
* Specify the level of stability to use when you enable partial results stabilization (
* EnablePartialResultsStabilization
).
*
*
* Low stability provides the highest accuracy. High stability transcribes faster, but with slightly lower accuracy.
*
*
* @param partialResultsStability
* Specify the level of stability to use when you enable partial results stabilization (
* EnablePartialResultsStabilization
).
*
* Low stability provides the highest accuracy. High stability transcribes faster, but with slightly lower
* accuracy.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TranscribePartialResultsStability
*/
public EngineTranscribeSettings withPartialResultsStability(String partialResultsStability) {
setPartialResultsStability(partialResultsStability);
return this;
}
/**
*
* Specify the level of stability to use when you enable partial results stabilization (
* EnablePartialResultsStabilization
).
*
*
* Low stability provides the highest accuracy. High stability transcribes faster, but with slightly lower accuracy.
*
*
* @param partialResultsStability
* Specify the level of stability to use when you enable partial results stabilization (
* EnablePartialResultsStabilization
).
*
* Low stability provides the highest accuracy. High stability transcribes faster, but with slightly lower
* accuracy.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TranscribePartialResultsStability
*/
public EngineTranscribeSettings withPartialResultsStability(TranscribePartialResultsStability partialResultsStability) {
this.partialResultsStability = partialResultsStability.toString();
return this;
}
/**
*
* Labels all personally identifiable information (PII) identified in your transcript. If you don't include
* PiiEntityTypes
, all PII is identified.
*
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
*
*
* @param contentIdentificationType
* Labels all personally identifiable information (PII) identified in your transcript. If you don't include
* PiiEntityTypes
, all PII is identified.
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
* @see TranscribeContentIdentificationType
*/
public void setContentIdentificationType(String contentIdentificationType) {
this.contentIdentificationType = contentIdentificationType;
}
/**
*
* Labels all personally identifiable information (PII) identified in your transcript. If you don't include
* PiiEntityTypes
, all PII is identified.
*
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
*
*
* @return Labels all personally identifiable information (PII) identified in your transcript. If you don't include
* PiiEntityTypes
, all PII is identified.
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
* @see TranscribeContentIdentificationType
*/
public String getContentIdentificationType() {
return this.contentIdentificationType;
}
/**
*
* Labels all personally identifiable information (PII) identified in your transcript. If you don't include
* PiiEntityTypes
, all PII is identified.
*
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
*
*
* @param contentIdentificationType
* Labels all personally identifiable information (PII) identified in your transcript. If you don't include
* PiiEntityTypes
, all PII is identified.
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TranscribeContentIdentificationType
*/
public EngineTranscribeSettings withContentIdentificationType(String contentIdentificationType) {
setContentIdentificationType(contentIdentificationType);
return this;
}
/**
*
* Labels all personally identifiable information (PII) identified in your transcript. If you don't include
* PiiEntityTypes
, all PII is identified.
*
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
*
*
* @param contentIdentificationType
* Labels all personally identifiable information (PII) identified in your transcript. If you don't include
* PiiEntityTypes
, all PII is identified.
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TranscribeContentIdentificationType
*/
public EngineTranscribeSettings withContentIdentificationType(TranscribeContentIdentificationType contentIdentificationType) {
this.contentIdentificationType = contentIdentificationType.toString();
return this;
}
/**
*
* Content redaction is performed at the segment level. If you don't include PiiEntityTypes
, all PII is
* redacted.
*
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
*
*
* @param contentRedactionType
* Content redaction is performed at the segment level. If you don't include PiiEntityTypes
, all
* PII is redacted.
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
* @see TranscribeContentRedactionType
*/
public void setContentRedactionType(String contentRedactionType) {
this.contentRedactionType = contentRedactionType;
}
/**
*
* Content redaction is performed at the segment level. If you don't include PiiEntityTypes
, all PII is
* redacted.
*
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
*
*
* @return Content redaction is performed at the segment level. If you don't include PiiEntityTypes
,
* all PII is redacted.
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
* @see TranscribeContentRedactionType
*/
public String getContentRedactionType() {
return this.contentRedactionType;
}
/**
*
* Content redaction is performed at the segment level. If you don't include PiiEntityTypes
, all PII is
* redacted.
*
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
*
*
* @param contentRedactionType
* Content redaction is performed at the segment level. If you don't include PiiEntityTypes
, all
* PII is redacted.
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TranscribeContentRedactionType
*/
public EngineTranscribeSettings withContentRedactionType(String contentRedactionType) {
setContentRedactionType(contentRedactionType);
return this;
}
/**
*
* Content redaction is performed at the segment level. If you don't include PiiEntityTypes
, all PII is
* redacted.
*
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
*
*
* @param contentRedactionType
* Content redaction is performed at the segment level. If you don't include PiiEntityTypes
, all
* PII is redacted.
*
* You can’t set ContentIdentificationType
and ContentRedactionType
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TranscribeContentRedactionType
*/
public EngineTranscribeSettings withContentRedactionType(TranscribeContentRedactionType contentRedactionType) {
this.contentRedactionType = contentRedactionType.toString();
return this;
}
/**
*
* Specify which types of personally identifiable information (PII) you want to redact in your transcript. You can
* include as many types as you'd like, or you can select ALL
.
*
*
* Values must be comma-separated and can include: ADDRESS
, BANK_ACCOUNT_NUMBER
,
* BANK_ROUTING
, CREDIT_DEBIT_CVV
, CREDIT_DEBIT_EXPIRY
* CREDIT_DEBIT_NUMBER
, EMAIL
,NAME
, PHONE
, PIN
,
* SSN
, or ALL
.
*
*
* Note that if you include PiiEntityTypes
, you must also include
* ContentIdentificationType
or ContentRedactionType
.
*
*
* If you include ContentRedactionType
or ContentIdentificationType
, but do not include
* PiiEntityTypes
, all PII is redacted or identified.
*
*
* @param piiEntityTypes
* Specify which types of personally identifiable information (PII) you want to redact in your transcript.
* You can include as many types as you'd like, or you can select ALL
.
*
* Values must be comma-separated and can include: ADDRESS
, BANK_ACCOUNT_NUMBER
,
* BANK_ROUTING
, CREDIT_DEBIT_CVV
, CREDIT_DEBIT_EXPIRY
* CREDIT_DEBIT_NUMBER
, EMAIL
,NAME
, PHONE
,
* PIN
, SSN
, or ALL
.
*
*
* Note that if you include PiiEntityTypes
, you must also include
* ContentIdentificationType
or ContentRedactionType
.
*
*
* If you include ContentRedactionType
or ContentIdentificationType
, but do not
* include PiiEntityTypes
, all PII is redacted or identified.
*/
public void setPiiEntityTypes(String piiEntityTypes) {
this.piiEntityTypes = piiEntityTypes;
}
/**
*
* Specify which types of personally identifiable information (PII) you want to redact in your transcript. You can
* include as many types as you'd like, or you can select ALL
.
*
*
* Values must be comma-separated and can include: ADDRESS
, BANK_ACCOUNT_NUMBER
,
* BANK_ROUTING
, CREDIT_DEBIT_CVV
, CREDIT_DEBIT_EXPIRY
* CREDIT_DEBIT_NUMBER
, EMAIL
,NAME
, PHONE
, PIN
,
* SSN
, or ALL
.
*
*
* Note that if you include PiiEntityTypes
, you must also include
* ContentIdentificationType
or ContentRedactionType
.
*
*
* If you include ContentRedactionType
or ContentIdentificationType
, but do not include
* PiiEntityTypes
, all PII is redacted or identified.
*
*
* @return Specify which types of personally identifiable information (PII) you want to redact in your transcript.
* You can include as many types as you'd like, or you can select ALL
.
*
* Values must be comma-separated and can include: ADDRESS
, BANK_ACCOUNT_NUMBER
,
* BANK_ROUTING
, CREDIT_DEBIT_CVV
, CREDIT_DEBIT_EXPIRY
* CREDIT_DEBIT_NUMBER
, EMAIL
,NAME
, PHONE
,
* PIN
, SSN
, or ALL
.
*
*
* Note that if you include PiiEntityTypes
, you must also include
* ContentIdentificationType
or ContentRedactionType
.
*
*
* If you include ContentRedactionType
or ContentIdentificationType
, but do not
* include PiiEntityTypes
, all PII is redacted or identified.
*/
public String getPiiEntityTypes() {
return this.piiEntityTypes;
}
/**
*
* Specify which types of personally identifiable information (PII) you want to redact in your transcript. You can
* include as many types as you'd like, or you can select ALL
.
*
*
* Values must be comma-separated and can include: ADDRESS
, BANK_ACCOUNT_NUMBER
,
* BANK_ROUTING
, CREDIT_DEBIT_CVV
, CREDIT_DEBIT_EXPIRY
* CREDIT_DEBIT_NUMBER
, EMAIL
,NAME
, PHONE
, PIN
,
* SSN
, or ALL
.
*
*
* Note that if you include PiiEntityTypes
, you must also include
* ContentIdentificationType
or ContentRedactionType
.
*
*
* If you include ContentRedactionType
or ContentIdentificationType
, but do not include
* PiiEntityTypes
, all PII is redacted or identified.
*
*
* @param piiEntityTypes
* Specify which types of personally identifiable information (PII) you want to redact in your transcript.
* You can include as many types as you'd like, or you can select ALL
.
*
* Values must be comma-separated and can include: ADDRESS
, BANK_ACCOUNT_NUMBER
,
* BANK_ROUTING
, CREDIT_DEBIT_CVV
, CREDIT_DEBIT_EXPIRY
* CREDIT_DEBIT_NUMBER
, EMAIL
,NAME
, PHONE
,
* PIN
, SSN
, or ALL
.
*
*
* Note that if you include PiiEntityTypes
, you must also include
* ContentIdentificationType
or ContentRedactionType
.
*
*
* If you include ContentRedactionType
or ContentIdentificationType
, but do not
* include PiiEntityTypes
, all PII is redacted or identified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EngineTranscribeSettings withPiiEntityTypes(String piiEntityTypes) {
setPiiEntityTypes(piiEntityTypes);
return this;
}
/**
*
* Specify the name of the custom language model that you want to use when processing your transcription. Note that
* language model names are case sensitive.
*
*
* The language of the specified language model must match the language code. If the languages don't match, the
* custom language model isn't applied. There are no errors or warnings associated with a language mismatch.
*
*
* If you use Amazon Transcribe in multiple Regions, the custom language model must be available in Amazon
* Transcribe in each Region.
*
*
* @param languageModelName
* Specify the name of the custom language model that you want to use when processing your transcription.
* Note that language model names are case sensitive.
*
* The language of the specified language model must match the language code. If the languages don't match,
* the custom language model isn't applied. There are no errors or warnings associated with a language
* mismatch.
*
*
* If you use Amazon Transcribe in multiple Regions, the custom language model must be available in Amazon
* Transcribe in each Region.
*/
public void setLanguageModelName(String languageModelName) {
this.languageModelName = languageModelName;
}
/**
*
* Specify the name of the custom language model that you want to use when processing your transcription. Note that
* language model names are case sensitive.
*
*
* The language of the specified language model must match the language code. If the languages don't match, the
* custom language model isn't applied. There are no errors or warnings associated with a language mismatch.
*
*
* If you use Amazon Transcribe in multiple Regions, the custom language model must be available in Amazon
* Transcribe in each Region.
*
*
* @return Specify the name of the custom language model that you want to use when processing your transcription.
* Note that language model names are case sensitive.
*
* The language of the specified language model must match the language code. If the languages don't match,
* the custom language model isn't applied. There are no errors or warnings associated with a language
* mismatch.
*
*
* If you use Amazon Transcribe in multiple Regions, the custom language model must be available in Amazon
* Transcribe in each Region.
*/
public String getLanguageModelName() {
return this.languageModelName;
}
/**
*
* Specify the name of the custom language model that you want to use when processing your transcription. Note that
* language model names are case sensitive.
*
*
* The language of the specified language model must match the language code. If the languages don't match, the
* custom language model isn't applied. There are no errors or warnings associated with a language mismatch.
*
*
* If you use Amazon Transcribe in multiple Regions, the custom language model must be available in Amazon
* Transcribe in each Region.
*
*
* @param languageModelName
* Specify the name of the custom language model that you want to use when processing your transcription.
* Note that language model names are case sensitive.
*
* The language of the specified language model must match the language code. If the languages don't match,
* the custom language model isn't applied. There are no errors or warnings associated with a language
* mismatch.
*
*
* If you use Amazon Transcribe in multiple Regions, the custom language model must be available in Amazon
* Transcribe in each Region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EngineTranscribeSettings withLanguageModelName(String languageModelName) {
setLanguageModelName(languageModelName);
return this;
}
/**
*
* Enables automatic language identification for your transcription.
*
*
* If you include IdentifyLanguage
, you can optionally use LanguageOptions
to include a
* list of language codes that you think may be present in your audio stream. Including language options can improve
* transcription accuracy.
*
*
* You can also use PreferredLanguage
to include a preferred language. Doing so can help Amazon
* Transcribe identify the language faster.
*
*
* You must include either LanguageCode
or IdentifyLanguage
.
*
*
* Language identification can't be combined with custom language models or redaction.
*
*
* @param identifyLanguage
* Enables automatic language identification for your transcription.
*
* If you include IdentifyLanguage
, you can optionally use LanguageOptions
to
* include a list of language codes that you think may be present in your audio stream. Including language
* options can improve transcription accuracy.
*
*
* You can also use PreferredLanguage
to include a preferred language. Doing so can help Amazon
* Transcribe identify the language faster.
*
*
* You must include either LanguageCode
or IdentifyLanguage
.
*
*
* Language identification can't be combined with custom language models or redaction.
*/
public void setIdentifyLanguage(Boolean identifyLanguage) {
this.identifyLanguage = identifyLanguage;
}
/**
*
* Enables automatic language identification for your transcription.
*
*
* If you include IdentifyLanguage
, you can optionally use LanguageOptions
to include a
* list of language codes that you think may be present in your audio stream. Including language options can improve
* transcription accuracy.
*
*
* You can also use PreferredLanguage
to include a preferred language. Doing so can help Amazon
* Transcribe identify the language faster.
*
*
* You must include either LanguageCode
or IdentifyLanguage
.
*
*
* Language identification can't be combined with custom language models or redaction.
*
*
* @return Enables automatic language identification for your transcription.
*
* If you include IdentifyLanguage
, you can optionally use LanguageOptions
to
* include a list of language codes that you think may be present in your audio stream. Including language
* options can improve transcription accuracy.
*
*
* You can also use PreferredLanguage
to include a preferred language. Doing so can help Amazon
* Transcribe identify the language faster.
*
*
* You must include either LanguageCode
or IdentifyLanguage
.
*
*
* Language identification can't be combined with custom language models or redaction.
*/
public Boolean getIdentifyLanguage() {
return this.identifyLanguage;
}
/**
*
* Enables automatic language identification for your transcription.
*
*
* If you include IdentifyLanguage
, you can optionally use LanguageOptions
to include a
* list of language codes that you think may be present in your audio stream. Including language options can improve
* transcription accuracy.
*
*
* You can also use PreferredLanguage
to include a preferred language. Doing so can help Amazon
* Transcribe identify the language faster.
*
*
* You must include either LanguageCode
or IdentifyLanguage
.
*
*
* Language identification can't be combined with custom language models or redaction.
*
*
* @param identifyLanguage
* Enables automatic language identification for your transcription.
*
* If you include IdentifyLanguage
, you can optionally use LanguageOptions
to
* include a list of language codes that you think may be present in your audio stream. Including language
* options can improve transcription accuracy.
*
*
* You can also use PreferredLanguage
to include a preferred language. Doing so can help Amazon
* Transcribe identify the language faster.
*
*
* You must include either LanguageCode
or IdentifyLanguage
.
*
*
* Language identification can't be combined with custom language models or redaction.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EngineTranscribeSettings withIdentifyLanguage(Boolean identifyLanguage) {
setIdentifyLanguage(identifyLanguage);
return this;
}
/**
*
* Enables automatic language identification for your transcription.
*
*
* If you include IdentifyLanguage
, you can optionally use LanguageOptions
to include a
* list of language codes that you think may be present in your audio stream. Including language options can improve
* transcription accuracy.
*
*
* You can also use PreferredLanguage
to include a preferred language. Doing so can help Amazon
* Transcribe identify the language faster.
*
*
* You must include either LanguageCode
or IdentifyLanguage
.
*
*
* Language identification can't be combined with custom language models or redaction.
*
*
* @return Enables automatic language identification for your transcription.
*
* If you include IdentifyLanguage
, you can optionally use LanguageOptions
to
* include a list of language codes that you think may be present in your audio stream. Including language
* options can improve transcription accuracy.
*
*
* You can also use PreferredLanguage
to include a preferred language. Doing so can help Amazon
* Transcribe identify the language faster.
*
*
* You must include either LanguageCode
or IdentifyLanguage
.
*
*
* Language identification can't be combined with custom language models or redaction.
*/
public Boolean isIdentifyLanguage() {
return this.identifyLanguage;
}
/**
*
* Specify two or more language codes that represent the languages you think may be present in your media; including
* more than five is not recommended. If you're unsure what languages are present, do not include this parameter.
*
*
* Including language options can improve the accuracy of language identification.
*
*
* If you include LanguageOptions
, you must also include IdentifyLanguage
.
*
*
*
* You can only include one language dialect per language. For example, you cannot include en-US
and
* en-AU
.
*
*
*
* @param languageOptions
* Specify two or more language codes that represent the languages you think may be present in your media;
* including more than five is not recommended. If you're unsure what languages are present, do not include
* this parameter.
*
* Including language options can improve the accuracy of language identification.
*
*
* If you include LanguageOptions
, you must also include IdentifyLanguage
.
*
*
*
* You can only include one language dialect per language. For example, you cannot include en-US
* and en-AU
.
*
*/
public void setLanguageOptions(String languageOptions) {
this.languageOptions = languageOptions;
}
/**
*
* Specify two or more language codes that represent the languages you think may be present in your media; including
* more than five is not recommended. If you're unsure what languages are present, do not include this parameter.
*
*
* Including language options can improve the accuracy of language identification.
*
*
* If you include LanguageOptions
, you must also include IdentifyLanguage
.
*
*
*
* You can only include one language dialect per language. For example, you cannot include en-US
and
* en-AU
.
*
*
*
* @return Specify two or more language codes that represent the languages you think may be present in your media;
* including more than five is not recommended. If you're unsure what languages are present, do not include
* this parameter.
*
* Including language options can improve the accuracy of language identification.
*
*
* If you include LanguageOptions
, you must also include IdentifyLanguage
.
*
*
*
* You can only include one language dialect per language. For example, you cannot include
* en-US
and en-AU
.
*
*/
public String getLanguageOptions() {
return this.languageOptions;
}
/**
*
* Specify two or more language codes that represent the languages you think may be present in your media; including
* more than five is not recommended. If you're unsure what languages are present, do not include this parameter.
*
*
* Including language options can improve the accuracy of language identification.
*
*
* If you include LanguageOptions
, you must also include IdentifyLanguage
.
*
*
*
* You can only include one language dialect per language. For example, you cannot include en-US
and
* en-AU
.
*
*
*
* @param languageOptions
* Specify two or more language codes that represent the languages you think may be present in your media;
* including more than five is not recommended. If you're unsure what languages are present, do not include
* this parameter.
*
* Including language options can improve the accuracy of language identification.
*
*
* If you include LanguageOptions
, you must also include IdentifyLanguage
.
*
*
*
* You can only include one language dialect per language. For example, you cannot include en-US
* and en-AU
.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EngineTranscribeSettings withLanguageOptions(String languageOptions) {
setLanguageOptions(languageOptions);
return this;
}
/**
*
* Specify a preferred language from the subset of languages codes you specified in LanguageOptions
.
*
*
* You can only use this parameter if you include IdentifyLanguage
and LanguageOptions
.
*
*
* @param preferredLanguage
* Specify a preferred language from the subset of languages codes you specified in
* LanguageOptions
.
*
* You can only use this parameter if you include IdentifyLanguage
and
* LanguageOptions
.
* @see TranscribeLanguageCode
*/
public void setPreferredLanguage(String preferredLanguage) {
this.preferredLanguage = preferredLanguage;
}
/**
*
* Specify a preferred language from the subset of languages codes you specified in LanguageOptions
.
*
*
* You can only use this parameter if you include IdentifyLanguage
and LanguageOptions
.
*
*
* @return Specify a preferred language from the subset of languages codes you specified in
* LanguageOptions
.
*
* You can only use this parameter if you include IdentifyLanguage
and
* LanguageOptions
.
* @see TranscribeLanguageCode
*/
public String getPreferredLanguage() {
return this.preferredLanguage;
}
/**
*
* Specify a preferred language from the subset of languages codes you specified in LanguageOptions
.
*
*
* You can only use this parameter if you include IdentifyLanguage
and LanguageOptions
.
*
*
* @param preferredLanguage
* Specify a preferred language from the subset of languages codes you specified in
* LanguageOptions
.
*
* You can only use this parameter if you include IdentifyLanguage
and
* LanguageOptions
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TranscribeLanguageCode
*/
public EngineTranscribeSettings withPreferredLanguage(String preferredLanguage) {
setPreferredLanguage(preferredLanguage);
return this;
}
/**
*
* Specify a preferred language from the subset of languages codes you specified in LanguageOptions
.
*
*
* You can only use this parameter if you include IdentifyLanguage
and LanguageOptions
.
*
*
* @param preferredLanguage
* Specify a preferred language from the subset of languages codes you specified in
* LanguageOptions
.
*
* You can only use this parameter if you include IdentifyLanguage
and
* LanguageOptions
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see TranscribeLanguageCode
*/
public EngineTranscribeSettings withPreferredLanguage(TranscribeLanguageCode preferredLanguage) {
this.preferredLanguage = preferredLanguage.toString();
return this;
}
/**
*
* Specify the names of the custom vocabularies that you want to use when processing your transcription. Note that
* vocabulary names are case sensitive.
*
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary must be available in Amazon Transcribe in each
* Region.
*
*
* If you don't include IdentifyLanguage
and want to use a custom vocabulary with your transcription,
* use the VocabularyName
parameter instead.
*
*
* @param vocabularyNames
* Specify the names of the custom vocabularies that you want to use when processing your transcription. Note
* that vocabulary names are case sensitive.
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary must be available in Amazon Transcribe in
* each Region.
*
*
* If you don't include IdentifyLanguage
and want to use a custom vocabulary with your
* transcription, use the VocabularyName
parameter instead.
*/
public void setVocabularyNames(String vocabularyNames) {
this.vocabularyNames = vocabularyNames;
}
/**
*
* Specify the names of the custom vocabularies that you want to use when processing your transcription. Note that
* vocabulary names are case sensitive.
*
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary must be available in Amazon Transcribe in each
* Region.
*
*
* If you don't include IdentifyLanguage
and want to use a custom vocabulary with your transcription,
* use the VocabularyName
parameter instead.
*
*
* @return Specify the names of the custom vocabularies that you want to use when processing your transcription.
* Note that vocabulary names are case sensitive.
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary must be available in Amazon Transcribe
* in each Region.
*
*
* If you don't include IdentifyLanguage
and want to use a custom vocabulary with your
* transcription, use the VocabularyName
parameter instead.
*/
public String getVocabularyNames() {
return this.vocabularyNames;
}
/**
*
* Specify the names of the custom vocabularies that you want to use when processing your transcription. Note that
* vocabulary names are case sensitive.
*
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary must be available in Amazon Transcribe in each
* Region.
*
*
* If you don't include IdentifyLanguage
and want to use a custom vocabulary with your transcription,
* use the VocabularyName
parameter instead.
*
*
* @param vocabularyNames
* Specify the names of the custom vocabularies that you want to use when processing your transcription. Note
* that vocabulary names are case sensitive.
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary must be available in Amazon Transcribe in
* each Region.
*
*
* If you don't include IdentifyLanguage
and want to use a custom vocabulary with your
* transcription, use the VocabularyName
parameter instead.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EngineTranscribeSettings withVocabularyNames(String vocabularyNames) {
setVocabularyNames(vocabularyNames);
return this;
}
/**
*
* Specify the names of the custom vocabulary filters that you want to use when processing your transcription. Note
* that vocabulary filter names are case sensitive.
*
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary filter must be available in Amazon Transcribe in
* each Region.
*
*
* If you're not including IdentifyLanguage
and want to use a custom vocabulary filter with your
* transcription, use the VocabularyFilterName
parameter instead.
*
*
* @param vocabularyFilterNames
* Specify the names of the custom vocabulary filters that you want to use when processing your
* transcription. Note that vocabulary filter names are case sensitive.
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary filter must be available in Amazon
* Transcribe in each Region.
*
*
* If you're not including IdentifyLanguage
and want to use a custom vocabulary filter
* with your transcription, use the VocabularyFilterName
parameter instead.
*/
public void setVocabularyFilterNames(String vocabularyFilterNames) {
this.vocabularyFilterNames = vocabularyFilterNames;
}
/**
*
* Specify the names of the custom vocabulary filters that you want to use when processing your transcription. Note
* that vocabulary filter names are case sensitive.
*
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary filter must be available in Amazon Transcribe in
* each Region.
*
*
* If you're not including IdentifyLanguage
and want to use a custom vocabulary filter with your
* transcription, use the VocabularyFilterName
parameter instead.
*
*
* @return Specify the names of the custom vocabulary filters that you want to use when processing your
* transcription. Note that vocabulary filter names are case sensitive.
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary filter must be available in Amazon
* Transcribe in each Region.
*
*
* If you're not including IdentifyLanguage
and want to use a custom vocabulary filter
* with your transcription, use the VocabularyFilterName
parameter instead.
*/
public String getVocabularyFilterNames() {
return this.vocabularyFilterNames;
}
/**
*
* Specify the names of the custom vocabulary filters that you want to use when processing your transcription. Note
* that vocabulary filter names are case sensitive.
*
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary filter must be available in Amazon Transcribe in
* each Region.
*
*
* If you're not including IdentifyLanguage
and want to use a custom vocabulary filter with your
* transcription, use the VocabularyFilterName
parameter instead.
*
*
* @param vocabularyFilterNames
* Specify the names of the custom vocabulary filters that you want to use when processing your
* transcription. Note that vocabulary filter names are case sensitive.
*
* If you use Amazon Transcribe in multiple Regions, the vocabulary filter must be available in Amazon
* Transcribe in each Region.
*
*
* If you're not including IdentifyLanguage
and want to use a custom vocabulary filter
* with your transcription, use the VocabularyFilterName
parameter instead.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public EngineTranscribeSettings withVocabularyFilterNames(String vocabularyFilterNames) {
setVocabularyFilterNames(vocabularyFilterNames);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getLanguageCode() != null)
sb.append("LanguageCode: ").append(getLanguageCode()).append(",");
if (getVocabularyFilterMethod() != null)
sb.append("VocabularyFilterMethod: ").append(getVocabularyFilterMethod()).append(",");
if (getVocabularyFilterName() != null)
sb.append("VocabularyFilterName: ").append(getVocabularyFilterName()).append(",");
if (getVocabularyName() != null)
sb.append("VocabularyName: ").append(getVocabularyName()).append(",");
if (getRegion() != null)
sb.append("Region: ").append(getRegion()).append(",");
if (getEnablePartialResultsStabilization() != null)
sb.append("EnablePartialResultsStabilization: ").append(getEnablePartialResultsStabilization()).append(",");
if (getPartialResultsStability() != null)
sb.append("PartialResultsStability: ").append(getPartialResultsStability()).append(",");
if (getContentIdentificationType() != null)
sb.append("ContentIdentificationType: ").append(getContentIdentificationType()).append(",");
if (getContentRedactionType() != null)
sb.append("ContentRedactionType: ").append(getContentRedactionType()).append(",");
if (getPiiEntityTypes() != null)
sb.append("PiiEntityTypes: ").append(getPiiEntityTypes()).append(",");
if (getLanguageModelName() != null)
sb.append("LanguageModelName: ").append(getLanguageModelName()).append(",");
if (getIdentifyLanguage() != null)
sb.append("IdentifyLanguage: ").append(getIdentifyLanguage()).append(",");
if (getLanguageOptions() != null)
sb.append("LanguageOptions: ").append(getLanguageOptions()).append(",");
if (getPreferredLanguage() != null)
sb.append("PreferredLanguage: ").append(getPreferredLanguage()).append(",");
if (getVocabularyNames() != null)
sb.append("VocabularyNames: ").append(getVocabularyNames()).append(",");
if (getVocabularyFilterNames() != null)
sb.append("VocabularyFilterNames: ").append(getVocabularyFilterNames());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof EngineTranscribeSettings == false)
return false;
EngineTranscribeSettings other = (EngineTranscribeSettings) obj;
if (other.getLanguageCode() == null ^ this.getLanguageCode() == null)
return false;
if (other.getLanguageCode() != null && other.getLanguageCode().equals(this.getLanguageCode()) == false)
return false;
if (other.getVocabularyFilterMethod() == null ^ this.getVocabularyFilterMethod() == null)
return false;
if (other.getVocabularyFilterMethod() != null && other.getVocabularyFilterMethod().equals(this.getVocabularyFilterMethod()) == false)
return false;
if (other.getVocabularyFilterName() == null ^ this.getVocabularyFilterName() == null)
return false;
if (other.getVocabularyFilterName() != null && other.getVocabularyFilterName().equals(this.getVocabularyFilterName()) == false)
return false;
if (other.getVocabularyName() == null ^ this.getVocabularyName() == null)
return false;
if (other.getVocabularyName() != null && other.getVocabularyName().equals(this.getVocabularyName()) == false)
return false;
if (other.getRegion() == null ^ this.getRegion() == null)
return false;
if (other.getRegion() != null && other.getRegion().equals(this.getRegion()) == false)
return false;
if (other.getEnablePartialResultsStabilization() == null ^ this.getEnablePartialResultsStabilization() == null)
return false;
if (other.getEnablePartialResultsStabilization() != null
&& other.getEnablePartialResultsStabilization().equals(this.getEnablePartialResultsStabilization()) == false)
return false;
if (other.getPartialResultsStability() == null ^ this.getPartialResultsStability() == null)
return false;
if (other.getPartialResultsStability() != null && other.getPartialResultsStability().equals(this.getPartialResultsStability()) == false)
return false;
if (other.getContentIdentificationType() == null ^ this.getContentIdentificationType() == null)
return false;
if (other.getContentIdentificationType() != null && other.getContentIdentificationType().equals(this.getContentIdentificationType()) == false)
return false;
if (other.getContentRedactionType() == null ^ this.getContentRedactionType() == null)
return false;
if (other.getContentRedactionType() != null && other.getContentRedactionType().equals(this.getContentRedactionType()) == false)
return false;
if (other.getPiiEntityTypes() == null ^ this.getPiiEntityTypes() == null)
return false;
if (other.getPiiEntityTypes() != null && other.getPiiEntityTypes().equals(this.getPiiEntityTypes()) == false)
return false;
if (other.getLanguageModelName() == null ^ this.getLanguageModelName() == null)
return false;
if (other.getLanguageModelName() != null && other.getLanguageModelName().equals(this.getLanguageModelName()) == false)
return false;
if (other.getIdentifyLanguage() == null ^ this.getIdentifyLanguage() == null)
return false;
if (other.getIdentifyLanguage() != null && other.getIdentifyLanguage().equals(this.getIdentifyLanguage()) == false)
return false;
if (other.getLanguageOptions() == null ^ this.getLanguageOptions() == null)
return false;
if (other.getLanguageOptions() != null && other.getLanguageOptions().equals(this.getLanguageOptions()) == false)
return false;
if (other.getPreferredLanguage() == null ^ this.getPreferredLanguage() == null)
return false;
if (other.getPreferredLanguage() != null && other.getPreferredLanguage().equals(this.getPreferredLanguage()) == false)
return false;
if (other.getVocabularyNames() == null ^ this.getVocabularyNames() == null)
return false;
if (other.getVocabularyNames() != null && other.getVocabularyNames().equals(this.getVocabularyNames()) == false)
return false;
if (other.getVocabularyFilterNames() == null ^ this.getVocabularyFilterNames() == null)
return false;
if (other.getVocabularyFilterNames() != null && other.getVocabularyFilterNames().equals(this.getVocabularyFilterNames()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getLanguageCode() == null) ? 0 : getLanguageCode().hashCode());
hashCode = prime * hashCode + ((getVocabularyFilterMethod() == null) ? 0 : getVocabularyFilterMethod().hashCode());
hashCode = prime * hashCode + ((getVocabularyFilterName() == null) ? 0 : getVocabularyFilterName().hashCode());
hashCode = prime * hashCode + ((getVocabularyName() == null) ? 0 : getVocabularyName().hashCode());
hashCode = prime * hashCode + ((getRegion() == null) ? 0 : getRegion().hashCode());
hashCode = prime * hashCode + ((getEnablePartialResultsStabilization() == null) ? 0 : getEnablePartialResultsStabilization().hashCode());
hashCode = prime * hashCode + ((getPartialResultsStability() == null) ? 0 : getPartialResultsStability().hashCode());
hashCode = prime * hashCode + ((getContentIdentificationType() == null) ? 0 : getContentIdentificationType().hashCode());
hashCode = prime * hashCode + ((getContentRedactionType() == null) ? 0 : getContentRedactionType().hashCode());
hashCode = prime * hashCode + ((getPiiEntityTypes() == null) ? 0 : getPiiEntityTypes().hashCode());
hashCode = prime * hashCode + ((getLanguageModelName() == null) ? 0 : getLanguageModelName().hashCode());
hashCode = prime * hashCode + ((getIdentifyLanguage() == null) ? 0 : getIdentifyLanguage().hashCode());
hashCode = prime * hashCode + ((getLanguageOptions() == null) ? 0 : getLanguageOptions().hashCode());
hashCode = prime * hashCode + ((getPreferredLanguage() == null) ? 0 : getPreferredLanguage().hashCode());
hashCode = prime * hashCode + ((getVocabularyNames() == null) ? 0 : getVocabularyNames().hashCode());
hashCode = prime * hashCode + ((getVocabularyFilterNames() == null) ? 0 : getVocabularyFilterNames().hashCode());
return hashCode;
}
@Override
public EngineTranscribeSettings clone() {
try {
return (EngineTranscribeSettings) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.chime.model.transform.EngineTranscribeSettingsMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}