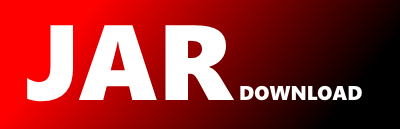
com.amazonaws.services.chime.model.Meeting Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chime Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chime.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.protocol.StructuredPojo;
import com.amazonaws.protocol.ProtocolMarshaller;
/**
*
* A meeting created using the Amazon Chime SDK.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class Meeting implements Serializable, Cloneable, StructuredPojo {
/**
*
* The Amazon Chime SDK meeting ID.
*
*/
private String meetingId;
/**
*
* The external meeting ID.
*
*/
private String externalMeetingId;
/**
*
* The media placement for the meeting.
*
*/
private MediaPlacement mediaPlacement;
/**
*
* The Region in which you create the meeting. Available values: af-south-1
,
* ap-northeast-1
, ap-northeast-2
, ap-south-1
, ap-southeast-1
,
* ap-southeast-2
, ca-central-1
, eu-central-1
, eu-north-1
,
* eu-south-1
, eu-west-1
, eu-west-2
, eu-west-3
,
* sa-east-1
, us-east-1
, us-east-2
, us-west-1
,
* us-west-2
.
*
*/
private String mediaRegion;
/**
*
* The Amazon Chime SDK meeting ID.
*
*
* @param meetingId
* The Amazon Chime SDK meeting ID.
*/
public void setMeetingId(String meetingId) {
this.meetingId = meetingId;
}
/**
*
* The Amazon Chime SDK meeting ID.
*
*
* @return The Amazon Chime SDK meeting ID.
*/
public String getMeetingId() {
return this.meetingId;
}
/**
*
* The Amazon Chime SDK meeting ID.
*
*
* @param meetingId
* The Amazon Chime SDK meeting ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Meeting withMeetingId(String meetingId) {
setMeetingId(meetingId);
return this;
}
/**
*
* The external meeting ID.
*
*
* @param externalMeetingId
* The external meeting ID.
*/
public void setExternalMeetingId(String externalMeetingId) {
this.externalMeetingId = externalMeetingId;
}
/**
*
* The external meeting ID.
*
*
* @return The external meeting ID.
*/
public String getExternalMeetingId() {
return this.externalMeetingId;
}
/**
*
* The external meeting ID.
*
*
* @param externalMeetingId
* The external meeting ID.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Meeting withExternalMeetingId(String externalMeetingId) {
setExternalMeetingId(externalMeetingId);
return this;
}
/**
*
* The media placement for the meeting.
*
*
* @param mediaPlacement
* The media placement for the meeting.
*/
public void setMediaPlacement(MediaPlacement mediaPlacement) {
this.mediaPlacement = mediaPlacement;
}
/**
*
* The media placement for the meeting.
*
*
* @return The media placement for the meeting.
*/
public MediaPlacement getMediaPlacement() {
return this.mediaPlacement;
}
/**
*
* The media placement for the meeting.
*
*
* @param mediaPlacement
* The media placement for the meeting.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Meeting withMediaPlacement(MediaPlacement mediaPlacement) {
setMediaPlacement(mediaPlacement);
return this;
}
/**
*
* The Region in which you create the meeting. Available values: af-south-1
,
* ap-northeast-1
, ap-northeast-2
, ap-south-1
, ap-southeast-1
,
* ap-southeast-2
, ca-central-1
, eu-central-1
, eu-north-1
,
* eu-south-1
, eu-west-1
, eu-west-2
, eu-west-3
,
* sa-east-1
, us-east-1
, us-east-2
, us-west-1
,
* us-west-2
.
*
*
* @param mediaRegion
* The Region in which you create the meeting. Available values: af-south-1
,
* ap-northeast-1
, ap-northeast-2
, ap-south-1
,
* ap-southeast-1
, ap-southeast-2
, ca-central-1
,
* eu-central-1
, eu-north-1
, eu-south-1
, eu-west-1
,
* eu-west-2
, eu-west-3
, sa-east-1
, us-east-1
,
* us-east-2
, us-west-1
, us-west-2
.
*/
public void setMediaRegion(String mediaRegion) {
this.mediaRegion = mediaRegion;
}
/**
*
* The Region in which you create the meeting. Available values: af-south-1
,
* ap-northeast-1
, ap-northeast-2
, ap-south-1
, ap-southeast-1
,
* ap-southeast-2
, ca-central-1
, eu-central-1
, eu-north-1
,
* eu-south-1
, eu-west-1
, eu-west-2
, eu-west-3
,
* sa-east-1
, us-east-1
, us-east-2
, us-west-1
,
* us-west-2
.
*
*
* @return The Region in which you create the meeting. Available values: af-south-1
,
* ap-northeast-1
, ap-northeast-2
, ap-south-1
,
* ap-southeast-1
, ap-southeast-2
, ca-central-1
,
* eu-central-1
, eu-north-1
, eu-south-1
, eu-west-1
,
* eu-west-2
, eu-west-3
, sa-east-1
, us-east-1
,
* us-east-2
, us-west-1
, us-west-2
.
*/
public String getMediaRegion() {
return this.mediaRegion;
}
/**
*
* The Region in which you create the meeting. Available values: af-south-1
,
* ap-northeast-1
, ap-northeast-2
, ap-south-1
, ap-southeast-1
,
* ap-southeast-2
, ca-central-1
, eu-central-1
, eu-north-1
,
* eu-south-1
, eu-west-1
, eu-west-2
, eu-west-3
,
* sa-east-1
, us-east-1
, us-east-2
, us-west-1
,
* us-west-2
.
*
*
* @param mediaRegion
* The Region in which you create the meeting. Available values: af-south-1
,
* ap-northeast-1
, ap-northeast-2
, ap-south-1
,
* ap-southeast-1
, ap-southeast-2
, ca-central-1
,
* eu-central-1
, eu-north-1
, eu-south-1
, eu-west-1
,
* eu-west-2
, eu-west-3
, sa-east-1
, us-east-1
,
* us-east-2
, us-west-1
, us-west-2
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public Meeting withMediaRegion(String mediaRegion) {
setMediaRegion(mediaRegion);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getMeetingId() != null)
sb.append("MeetingId: ").append(getMeetingId()).append(",");
if (getExternalMeetingId() != null)
sb.append("ExternalMeetingId: ").append("***Sensitive Data Redacted***").append(",");
if (getMediaPlacement() != null)
sb.append("MediaPlacement: ").append(getMediaPlacement()).append(",");
if (getMediaRegion() != null)
sb.append("MediaRegion: ").append(getMediaRegion());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof Meeting == false)
return false;
Meeting other = (Meeting) obj;
if (other.getMeetingId() == null ^ this.getMeetingId() == null)
return false;
if (other.getMeetingId() != null && other.getMeetingId().equals(this.getMeetingId()) == false)
return false;
if (other.getExternalMeetingId() == null ^ this.getExternalMeetingId() == null)
return false;
if (other.getExternalMeetingId() != null && other.getExternalMeetingId().equals(this.getExternalMeetingId()) == false)
return false;
if (other.getMediaPlacement() == null ^ this.getMediaPlacement() == null)
return false;
if (other.getMediaPlacement() != null && other.getMediaPlacement().equals(this.getMediaPlacement()) == false)
return false;
if (other.getMediaRegion() == null ^ this.getMediaRegion() == null)
return false;
if (other.getMediaRegion() != null && other.getMediaRegion().equals(this.getMediaRegion()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getMeetingId() == null) ? 0 : getMeetingId().hashCode());
hashCode = prime * hashCode + ((getExternalMeetingId() == null) ? 0 : getExternalMeetingId().hashCode());
hashCode = prime * hashCode + ((getMediaPlacement() == null) ? 0 : getMediaPlacement().hashCode());
hashCode = prime * hashCode + ((getMediaRegion() == null) ? 0 : getMediaRegion().hashCode());
return hashCode;
}
@Override
public Meeting clone() {
try {
return (Meeting) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
@com.amazonaws.annotation.SdkInternalApi
@Override
public void marshall(ProtocolMarshaller protocolMarshaller) {
com.amazonaws.services.chime.model.transform.MeetingMarshaller.getInstance().marshall(this, protocolMarshaller);
}
}