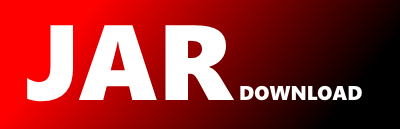
com.amazonaws.services.chimesdkidentity.AmazonChimeSDKIdentity Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chimesdkidentity Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chimesdkidentity;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.chimesdkidentity.model.*;
/**
* Interface for accessing Amazon Chime SDK Identity.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.chimesdkidentity.AbstractAmazonChimeSDKIdentity} instead.
*
*
*
* The Amazon Chime SDK Identity APIs in this section allow software developers to create and manage unique instances of
* their messaging applications. These APIs provide the overarching framework for creating and sending messages. For
* more information about the identity APIs, refer to Amazon
* Chime SDK identity.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonChimeSDKIdentity {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "identity-chime";
/**
*
* Creates an Amazon Chime SDK messaging AppInstance
under an AWS account. Only SDK messaging customers
* use this API. CreateAppInstance
supports idempotency behavior as described in the AWS API Standard.
*
*
* identity
*
*
* @param createAppInstanceRequest
* @return Result of the CreateAppInstance operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.CreateAppInstance
* @see AWS API Documentation
*/
CreateAppInstanceResult createAppInstance(CreateAppInstanceRequest createAppInstanceRequest);
/**
*
* Promotes an AppInstanceUser
or AppInstanceBot
to an AppInstanceAdmin
. The
* promoted entity can perform the following actions.
*
*
* -
*
* ChannelModerator
actions across all channels in the AppInstance
.
*
*
* -
*
* DeleteChannelMessage
actions.
*
*
*
*
* Only an AppInstanceUser
and AppInstanceBot
can be promoted to an
* AppInstanceAdmin
role.
*
*
* @param createAppInstanceAdminRequest
* @return Result of the CreateAppInstanceAdmin operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.CreateAppInstanceAdmin
* @see AWS API Documentation
*/
CreateAppInstanceAdminResult createAppInstanceAdmin(CreateAppInstanceAdminRequest createAppInstanceAdminRequest);
/**
*
* Creates a bot under an Amazon Chime AppInstance
. The request consists of a unique
* Configuration
and Name
for that bot.
*
*
* @param createAppInstanceBotRequest
* @return Result of the CreateAppInstanceBot operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.CreateAppInstanceBot
* @see AWS API Documentation
*/
CreateAppInstanceBotResult createAppInstanceBot(CreateAppInstanceBotRequest createAppInstanceBotRequest);
/**
*
* Creates a user under an Amazon Chime AppInstance
. The request consists of a unique
* appInstanceUserId
and Name
for that user.
*
*
* @param createAppInstanceUserRequest
* @return Result of the CreateAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.CreateAppInstanceUser
* @see AWS API Documentation
*/
CreateAppInstanceUserResult createAppInstanceUser(CreateAppInstanceUserRequest createAppInstanceUserRequest);
/**
*
* Deletes an AppInstance
and all associated data asynchronously.
*
*
* @param deleteAppInstanceRequest
* @return Result of the DeleteAppInstance operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.DeleteAppInstance
* @see AWS API Documentation
*/
DeleteAppInstanceResult deleteAppInstance(DeleteAppInstanceRequest deleteAppInstanceRequest);
/**
*
* Demotes an AppInstanceAdmin
to an AppInstanceUser
or AppInstanceBot
. This
* action does not delete the user.
*
*
* @param deleteAppInstanceAdminRequest
* @return Result of the DeleteAppInstanceAdmin operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.DeleteAppInstanceAdmin
* @see AWS API Documentation
*/
DeleteAppInstanceAdminResult deleteAppInstanceAdmin(DeleteAppInstanceAdminRequest deleteAppInstanceAdminRequest);
/**
*
* Deletes an AppInstanceBot
.
*
*
* @param deleteAppInstanceBotRequest
* @return Result of the DeleteAppInstanceBot operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.DeleteAppInstanceBot
* @see AWS API Documentation
*/
DeleteAppInstanceBotResult deleteAppInstanceBot(DeleteAppInstanceBotRequest deleteAppInstanceBotRequest);
/**
*
* Deletes an AppInstanceUser
.
*
*
* @param deleteAppInstanceUserRequest
* @return Result of the DeleteAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.DeleteAppInstanceUser
* @see AWS API Documentation
*/
DeleteAppInstanceUserResult deleteAppInstanceUser(DeleteAppInstanceUserRequest deleteAppInstanceUserRequest);
/**
*
* Deregisters an AppInstanceUserEndpoint
.
*
*
* @param deregisterAppInstanceUserEndpointRequest
* @return Result of the DeregisterAppInstanceUserEndpoint operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.DeregisterAppInstanceUserEndpoint
* @see AWS API Documentation
*/
DeregisterAppInstanceUserEndpointResult deregisterAppInstanceUserEndpoint(DeregisterAppInstanceUserEndpointRequest deregisterAppInstanceUserEndpointRequest);
/**
*
* Returns the full details of an AppInstance
.
*
*
* @param describeAppInstanceRequest
* @return Result of the DescribeAppInstance operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.DescribeAppInstance
* @see AWS API Documentation
*/
DescribeAppInstanceResult describeAppInstance(DescribeAppInstanceRequest describeAppInstanceRequest);
/**
*
* Returns the full details of an AppInstanceAdmin
.
*
*
* @param describeAppInstanceAdminRequest
* @return Result of the DescribeAppInstanceAdmin operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.DescribeAppInstanceAdmin
* @see AWS API Documentation
*/
DescribeAppInstanceAdminResult describeAppInstanceAdmin(DescribeAppInstanceAdminRequest describeAppInstanceAdminRequest);
/**
*
* The AppInstanceBot's
information.
*
*
* @param describeAppInstanceBotRequest
* @return Result of the DescribeAppInstanceBot operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.DescribeAppInstanceBot
* @see AWS API Documentation
*/
DescribeAppInstanceBotResult describeAppInstanceBot(DescribeAppInstanceBotRequest describeAppInstanceBotRequest);
/**
*
* Returns the full details of an AppInstanceUser
.
*
*
* @param describeAppInstanceUserRequest
* @return Result of the DescribeAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.DescribeAppInstanceUser
* @see AWS API Documentation
*/
DescribeAppInstanceUserResult describeAppInstanceUser(DescribeAppInstanceUserRequest describeAppInstanceUserRequest);
/**
*
* Returns the full details of an AppInstanceUserEndpoint
.
*
*
* @param describeAppInstanceUserEndpointRequest
* @return Result of the DescribeAppInstanceUserEndpoint operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.DescribeAppInstanceUserEndpoint
* @see AWS API Documentation
*/
DescribeAppInstanceUserEndpointResult describeAppInstanceUserEndpoint(DescribeAppInstanceUserEndpointRequest describeAppInstanceUserEndpointRequest);
/**
*
* Gets the retention settings for an AppInstance
.
*
*
* @param getAppInstanceRetentionSettingsRequest
* @return Result of the GetAppInstanceRetentionSettings operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.GetAppInstanceRetentionSettings
* @see AWS API Documentation
*/
GetAppInstanceRetentionSettingsResult getAppInstanceRetentionSettings(GetAppInstanceRetentionSettingsRequest getAppInstanceRetentionSettingsRequest);
/**
*
* Returns a list of the administrators in the AppInstance
.
*
*
* @param listAppInstanceAdminsRequest
* @return Result of the ListAppInstanceAdmins operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.ListAppInstanceAdmins
* @see AWS API Documentation
*/
ListAppInstanceAdminsResult listAppInstanceAdmins(ListAppInstanceAdminsRequest listAppInstanceAdminsRequest);
/**
*
* Lists all AppInstanceBots
created under a single AppInstance
.
*
*
* @param listAppInstanceBotsRequest
* @return Result of the ListAppInstanceBots operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.ListAppInstanceBots
* @see AWS API Documentation
*/
ListAppInstanceBotsResult listAppInstanceBots(ListAppInstanceBotsRequest listAppInstanceBotsRequest);
/**
*
* Lists all the AppInstanceUserEndpoints
created under a single AppInstanceUser
.
*
*
* @param listAppInstanceUserEndpointsRequest
* @return Result of the ListAppInstanceUserEndpoints operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.ListAppInstanceUserEndpoints
* @see AWS API Documentation
*/
ListAppInstanceUserEndpointsResult listAppInstanceUserEndpoints(ListAppInstanceUserEndpointsRequest listAppInstanceUserEndpointsRequest);
/**
*
* List all AppInstanceUsers
created under a single AppInstance
.
*
*
* @param listAppInstanceUsersRequest
* @return Result of the ListAppInstanceUsers operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.ListAppInstanceUsers
* @see AWS API Documentation
*/
ListAppInstanceUsersResult listAppInstanceUsers(ListAppInstanceUsersRequest listAppInstanceUsersRequest);
/**
*
* Lists all Amazon Chime AppInstance
s created under a single AWS account.
*
*
* @param listAppInstancesRequest
* @return Result of the ListAppInstances operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.ListAppInstances
* @see AWS API Documentation
*/
ListAppInstancesResult listAppInstances(ListAppInstancesRequest listAppInstancesRequest);
/**
*
* Lists the tags applied to an Amazon Chime SDK identity resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Sets the amount of time in days that a given AppInstance
retains data.
*
*
* @param putAppInstanceRetentionSettingsRequest
* @return Result of the PutAppInstanceRetentionSettings operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.PutAppInstanceRetentionSettings
* @see AWS API Documentation
*/
PutAppInstanceRetentionSettingsResult putAppInstanceRetentionSettings(PutAppInstanceRetentionSettingsRequest putAppInstanceRetentionSettingsRequest);
/**
*
* Sets the number of days before the AppInstanceUser
is automatically deleted.
*
*
*
* A background process deletes expired AppInstanceUsers
within 6 hours of expiration. Actual deletion
* times may vary.
*
*
* Expired AppInstanceUsers
that have not yet been deleted appear as active, and you can update their
* expiration settings. The system honors the new settings.
*
*
*
* @param putAppInstanceUserExpirationSettingsRequest
* @return Result of the PutAppInstanceUserExpirationSettings operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.PutAppInstanceUserExpirationSettings
* @see AWS API Documentation
*/
PutAppInstanceUserExpirationSettingsResult putAppInstanceUserExpirationSettings(
PutAppInstanceUserExpirationSettingsRequest putAppInstanceUserExpirationSettingsRequest);
/**
*
* Registers an endpoint under an Amazon Chime AppInstanceUser
. The endpoint receives messages for a
* user. For push notifications, the endpoint is a mobile device used to receive mobile push notifications for a
* user.
*
*
* @param registerAppInstanceUserEndpointRequest
* @return Result of the RegisterAppInstanceUserEndpoint operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.RegisterAppInstanceUserEndpoint
* @see AWS API Documentation
*/
RegisterAppInstanceUserEndpointResult registerAppInstanceUserEndpoint(RegisterAppInstanceUserEndpointRequest registerAppInstanceUserEndpointRequest);
/**
*
* Applies the specified tags to the specified Amazon Chime SDK identity resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.TagResource
* @see AWS
* API Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes the specified tags from the specified Amazon Chime SDK identity resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.UntagResource
* @see AWS API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates AppInstance
metadata.
*
*
* @param updateAppInstanceRequest
* @return Result of the UpdateAppInstance operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.UpdateAppInstance
* @see AWS API Documentation
*/
UpdateAppInstanceResult updateAppInstance(UpdateAppInstanceRequest updateAppInstanceRequest);
/**
*
* Updates the name and metadata of an AppInstanceBot
.
*
*
* @param updateAppInstanceBotRequest
* @return Result of the UpdateAppInstanceBot operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.UpdateAppInstanceBot
* @see AWS API Documentation
*/
UpdateAppInstanceBotResult updateAppInstanceBot(UpdateAppInstanceBotRequest updateAppInstanceBotRequest);
/**
*
* Updates the details of an AppInstanceUser
. You can update names and metadata.
*
*
* @param updateAppInstanceUserRequest
* @return Result of the UpdateAppInstanceUser operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.UpdateAppInstanceUser
* @see AWS API Documentation
*/
UpdateAppInstanceUserResult updateAppInstanceUser(UpdateAppInstanceUserRequest updateAppInstanceUserRequest);
/**
*
* Updates the details of an AppInstanceUserEndpoint
. You can update the name and
* AllowMessage
values.
*
*
* @param updateAppInstanceUserEndpointRequest
* @return Result of the UpdateAppInstanceUserEndpoint operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKIdentity.UpdateAppInstanceUserEndpoint
* @see AWS API Documentation
*/
UpdateAppInstanceUserEndpointResult updateAppInstanceUserEndpoint(UpdateAppInstanceUserEndpointRequest updateAppInstanceUserEndpointRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}