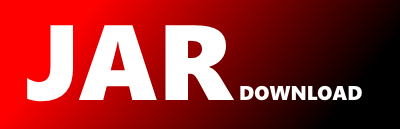
com.amazonaws.services.chimesdkidentity.AmazonChimeSDKIdentityAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chimesdkidentity Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chimesdkidentity;
import javax.annotation.Generated;
import com.amazonaws.services.chimesdkidentity.model.*;
/**
* Interface for accessing Amazon Chime SDK Identity asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.chimesdkidentity.AbstractAmazonChimeSDKIdentityAsync} instead.
*
*
*
* The Amazon Chime SDK Identity APIs in this section allow software developers to create and manage unique instances of
* their messaging applications. These APIs provide the overarching framework for creating and sending messages. For
* more information about the identity APIs, refer to Amazon
* Chime SDK identity.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonChimeSDKIdentityAsync extends AmazonChimeSDKIdentity {
/**
*
* Creates an Amazon Chime SDK messaging AppInstance
under an AWS account. Only SDK messaging customers
* use this API. CreateAppInstance
supports idempotency behavior as described in the AWS API Standard.
*
*
* identity
*
*
* @param createAppInstanceRequest
* @return A Java Future containing the result of the CreateAppInstance operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.CreateAppInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future createAppInstanceAsync(CreateAppInstanceRequest createAppInstanceRequest);
/**
*
* Creates an Amazon Chime SDK messaging AppInstance
under an AWS account. Only SDK messaging customers
* use this API. CreateAppInstance
supports idempotency behavior as described in the AWS API Standard.
*
*
* identity
*
*
* @param createAppInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAppInstance operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.CreateAppInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future createAppInstanceAsync(CreateAppInstanceRequest createAppInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Promotes an AppInstanceUser
or AppInstanceBot
to an AppInstanceAdmin
. The
* promoted entity can perform the following actions.
*
*
* -
*
* ChannelModerator
actions across all channels in the AppInstance
.
*
*
* -
*
* DeleteChannelMessage
actions.
*
*
*
*
* Only an AppInstanceUser
and AppInstanceBot
can be promoted to an
* AppInstanceAdmin
role.
*
*
* @param createAppInstanceAdminRequest
* @return A Java Future containing the result of the CreateAppInstanceAdmin operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.CreateAppInstanceAdmin
* @see AWS API Documentation
*/
java.util.concurrent.Future createAppInstanceAdminAsync(CreateAppInstanceAdminRequest createAppInstanceAdminRequest);
/**
*
* Promotes an AppInstanceUser
or AppInstanceBot
to an AppInstanceAdmin
. The
* promoted entity can perform the following actions.
*
*
* -
*
* ChannelModerator
actions across all channels in the AppInstance
.
*
*
* -
*
* DeleteChannelMessage
actions.
*
*
*
*
* Only an AppInstanceUser
and AppInstanceBot
can be promoted to an
* AppInstanceAdmin
role.
*
*
* @param createAppInstanceAdminRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAppInstanceAdmin operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.CreateAppInstanceAdmin
* @see AWS API Documentation
*/
java.util.concurrent.Future createAppInstanceAdminAsync(CreateAppInstanceAdminRequest createAppInstanceAdminRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a bot under an Amazon Chime AppInstance
. The request consists of a unique
* Configuration
and Name
for that bot.
*
*
* @param createAppInstanceBotRequest
* @return A Java Future containing the result of the CreateAppInstanceBot operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.CreateAppInstanceBot
* @see AWS API Documentation
*/
java.util.concurrent.Future createAppInstanceBotAsync(CreateAppInstanceBotRequest createAppInstanceBotRequest);
/**
*
* Creates a bot under an Amazon Chime AppInstance
. The request consists of a unique
* Configuration
and Name
for that bot.
*
*
* @param createAppInstanceBotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAppInstanceBot operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.CreateAppInstanceBot
* @see AWS API Documentation
*/
java.util.concurrent.Future createAppInstanceBotAsync(CreateAppInstanceBotRequest createAppInstanceBotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a user under an Amazon Chime AppInstance
. The request consists of a unique
* appInstanceUserId
and Name
for that user.
*
*
* @param createAppInstanceUserRequest
* @return A Java Future containing the result of the CreateAppInstanceUser operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.CreateAppInstanceUser
* @see AWS API Documentation
*/
java.util.concurrent.Future createAppInstanceUserAsync(CreateAppInstanceUserRequest createAppInstanceUserRequest);
/**
*
* Creates a user under an Amazon Chime AppInstance
. The request consists of a unique
* appInstanceUserId
and Name
for that user.
*
*
* @param createAppInstanceUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAppInstanceUser operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.CreateAppInstanceUser
* @see AWS API Documentation
*/
java.util.concurrent.Future createAppInstanceUserAsync(CreateAppInstanceUserRequest createAppInstanceUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an AppInstance
and all associated data asynchronously.
*
*
* @param deleteAppInstanceRequest
* @return A Java Future containing the result of the DeleteAppInstance operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.DeleteAppInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppInstanceAsync(DeleteAppInstanceRequest deleteAppInstanceRequest);
/**
*
* Deletes an AppInstance
and all associated data asynchronously.
*
*
* @param deleteAppInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppInstance operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.DeleteAppInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppInstanceAsync(DeleteAppInstanceRequest deleteAppInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Demotes an AppInstanceAdmin
to an AppInstanceUser
or AppInstanceBot
. This
* action does not delete the user.
*
*
* @param deleteAppInstanceAdminRequest
* @return A Java Future containing the result of the DeleteAppInstanceAdmin operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.DeleteAppInstanceAdmin
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppInstanceAdminAsync(DeleteAppInstanceAdminRequest deleteAppInstanceAdminRequest);
/**
*
* Demotes an AppInstanceAdmin
to an AppInstanceUser
or AppInstanceBot
. This
* action does not delete the user.
*
*
* @param deleteAppInstanceAdminRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppInstanceAdmin operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.DeleteAppInstanceAdmin
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppInstanceAdminAsync(DeleteAppInstanceAdminRequest deleteAppInstanceAdminRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an AppInstanceBot
.
*
*
* @param deleteAppInstanceBotRequest
* @return A Java Future containing the result of the DeleteAppInstanceBot operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.DeleteAppInstanceBot
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppInstanceBotAsync(DeleteAppInstanceBotRequest deleteAppInstanceBotRequest);
/**
*
* Deletes an AppInstanceBot
.
*
*
* @param deleteAppInstanceBotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppInstanceBot operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.DeleteAppInstanceBot
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppInstanceBotAsync(DeleteAppInstanceBotRequest deleteAppInstanceBotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an AppInstanceUser
.
*
*
* @param deleteAppInstanceUserRequest
* @return A Java Future containing the result of the DeleteAppInstanceUser operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.DeleteAppInstanceUser
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppInstanceUserAsync(DeleteAppInstanceUserRequest deleteAppInstanceUserRequest);
/**
*
* Deletes an AppInstanceUser
.
*
*
* @param deleteAppInstanceUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAppInstanceUser operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.DeleteAppInstanceUser
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAppInstanceUserAsync(DeleteAppInstanceUserRequest deleteAppInstanceUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deregisters an AppInstanceUserEndpoint
.
*
*
* @param deregisterAppInstanceUserEndpointRequest
* @return A Java Future containing the result of the DeregisterAppInstanceUserEndpoint operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsync.DeregisterAppInstanceUserEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterAppInstanceUserEndpointAsync(
DeregisterAppInstanceUserEndpointRequest deregisterAppInstanceUserEndpointRequest);
/**
*
* Deregisters an AppInstanceUserEndpoint
.
*
*
* @param deregisterAppInstanceUserEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeregisterAppInstanceUserEndpoint operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsyncHandler.DeregisterAppInstanceUserEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future deregisterAppInstanceUserEndpointAsync(
DeregisterAppInstanceUserEndpointRequest deregisterAppInstanceUserEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the full details of an AppInstance
.
*
*
* @param describeAppInstanceRequest
* @return A Java Future containing the result of the DescribeAppInstance operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.DescribeAppInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppInstanceAsync(DescribeAppInstanceRequest describeAppInstanceRequest);
/**
*
* Returns the full details of an AppInstance
.
*
*
* @param describeAppInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAppInstance operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.DescribeAppInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppInstanceAsync(DescribeAppInstanceRequest describeAppInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the full details of an AppInstanceAdmin
.
*
*
* @param describeAppInstanceAdminRequest
* @return A Java Future containing the result of the DescribeAppInstanceAdmin operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.DescribeAppInstanceAdmin
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppInstanceAdminAsync(DescribeAppInstanceAdminRequest describeAppInstanceAdminRequest);
/**
*
* Returns the full details of an AppInstanceAdmin
.
*
*
* @param describeAppInstanceAdminRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAppInstanceAdmin operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.DescribeAppInstanceAdmin
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppInstanceAdminAsync(DescribeAppInstanceAdminRequest describeAppInstanceAdminRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The AppInstanceBot's
information.
*
*
* @param describeAppInstanceBotRequest
* @return A Java Future containing the result of the DescribeAppInstanceBot operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.DescribeAppInstanceBot
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppInstanceBotAsync(DescribeAppInstanceBotRequest describeAppInstanceBotRequest);
/**
*
* The AppInstanceBot's
information.
*
*
* @param describeAppInstanceBotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAppInstanceBot operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.DescribeAppInstanceBot
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppInstanceBotAsync(DescribeAppInstanceBotRequest describeAppInstanceBotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the full details of an AppInstanceUser
.
*
*
* @param describeAppInstanceUserRequest
* @return A Java Future containing the result of the DescribeAppInstanceUser operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.DescribeAppInstanceUser
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppInstanceUserAsync(DescribeAppInstanceUserRequest describeAppInstanceUserRequest);
/**
*
* Returns the full details of an AppInstanceUser
.
*
*
* @param describeAppInstanceUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAppInstanceUser operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.DescribeAppInstanceUser
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppInstanceUserAsync(DescribeAppInstanceUserRequest describeAppInstanceUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the full details of an AppInstanceUserEndpoint
.
*
*
* @param describeAppInstanceUserEndpointRequest
* @return A Java Future containing the result of the DescribeAppInstanceUserEndpoint operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsync.DescribeAppInstanceUserEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppInstanceUserEndpointAsync(
DescribeAppInstanceUserEndpointRequest describeAppInstanceUserEndpointRequest);
/**
*
* Returns the full details of an AppInstanceUserEndpoint
.
*
*
* @param describeAppInstanceUserEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAppInstanceUserEndpoint operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsyncHandler.DescribeAppInstanceUserEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAppInstanceUserEndpointAsync(
DescribeAppInstanceUserEndpointRequest describeAppInstanceUserEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the retention settings for an AppInstance
.
*
*
* @param getAppInstanceRetentionSettingsRequest
* @return A Java Future containing the result of the GetAppInstanceRetentionSettings operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsync.GetAppInstanceRetentionSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future getAppInstanceRetentionSettingsAsync(
GetAppInstanceRetentionSettingsRequest getAppInstanceRetentionSettingsRequest);
/**
*
* Gets the retention settings for an AppInstance
.
*
*
* @param getAppInstanceRetentionSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAppInstanceRetentionSettings operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsyncHandler.GetAppInstanceRetentionSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future getAppInstanceRetentionSettingsAsync(
GetAppInstanceRetentionSettingsRequest getAppInstanceRetentionSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the administrators in the AppInstance
.
*
*
* @param listAppInstanceAdminsRequest
* @return A Java Future containing the result of the ListAppInstanceAdmins operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.ListAppInstanceAdmins
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppInstanceAdminsAsync(ListAppInstanceAdminsRequest listAppInstanceAdminsRequest);
/**
*
* Returns a list of the administrators in the AppInstance
.
*
*
* @param listAppInstanceAdminsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppInstanceAdmins operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.ListAppInstanceAdmins
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppInstanceAdminsAsync(ListAppInstanceAdminsRequest listAppInstanceAdminsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all AppInstanceBots
created under a single AppInstance
.
*
*
* @param listAppInstanceBotsRequest
* @return A Java Future containing the result of the ListAppInstanceBots operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.ListAppInstanceBots
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppInstanceBotsAsync(ListAppInstanceBotsRequest listAppInstanceBotsRequest);
/**
*
* Lists all AppInstanceBots
created under a single AppInstance
.
*
*
* @param listAppInstanceBotsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppInstanceBots operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.ListAppInstanceBots
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppInstanceBotsAsync(ListAppInstanceBotsRequest listAppInstanceBotsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all the AppInstanceUserEndpoints
created under a single AppInstanceUser
.
*
*
* @param listAppInstanceUserEndpointsRequest
* @return A Java Future containing the result of the ListAppInstanceUserEndpoints operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsync.ListAppInstanceUserEndpoints
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppInstanceUserEndpointsAsync(
ListAppInstanceUserEndpointsRequest listAppInstanceUserEndpointsRequest);
/**
*
* Lists all the AppInstanceUserEndpoints
created under a single AppInstanceUser
.
*
*
* @param listAppInstanceUserEndpointsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppInstanceUserEndpoints operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsyncHandler.ListAppInstanceUserEndpoints
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppInstanceUserEndpointsAsync(
ListAppInstanceUserEndpointsRequest listAppInstanceUserEndpointsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* List all AppInstanceUsers
created under a single AppInstance
.
*
*
* @param listAppInstanceUsersRequest
* @return A Java Future containing the result of the ListAppInstanceUsers operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.ListAppInstanceUsers
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppInstanceUsersAsync(ListAppInstanceUsersRequest listAppInstanceUsersRequest);
/**
*
* List all AppInstanceUsers
created under a single AppInstance
.
*
*
* @param listAppInstanceUsersRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppInstanceUsers operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.ListAppInstanceUsers
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppInstanceUsersAsync(ListAppInstanceUsersRequest listAppInstanceUsersRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all Amazon Chime AppInstance
s created under a single AWS account.
*
*
* @param listAppInstancesRequest
* @return A Java Future containing the result of the ListAppInstances operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.ListAppInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppInstancesAsync(ListAppInstancesRequest listAppInstancesRequest);
/**
*
* Lists all Amazon Chime AppInstance
s created under a single AWS account.
*
*
* @param listAppInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAppInstances operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.ListAppInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future listAppInstancesAsync(ListAppInstancesRequest listAppInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tags applied to an Amazon Chime SDK identity resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the tags applied to an Amazon Chime SDK identity resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets the amount of time in days that a given AppInstance
retains data.
*
*
* @param putAppInstanceRetentionSettingsRequest
* @return A Java Future containing the result of the PutAppInstanceRetentionSettings operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsync.PutAppInstanceRetentionSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future putAppInstanceRetentionSettingsAsync(
PutAppInstanceRetentionSettingsRequest putAppInstanceRetentionSettingsRequest);
/**
*
* Sets the amount of time in days that a given AppInstance
retains data.
*
*
* @param putAppInstanceRetentionSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutAppInstanceRetentionSettings operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsyncHandler.PutAppInstanceRetentionSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future putAppInstanceRetentionSettingsAsync(
PutAppInstanceRetentionSettingsRequest putAppInstanceRetentionSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets the number of days before the AppInstanceUser
is automatically deleted.
*
*
*
* A background process deletes expired AppInstanceUsers
within 6 hours of expiration. Actual deletion
* times may vary.
*
*
* Expired AppInstanceUsers
that have not yet been deleted appear as active, and you can update their
* expiration settings. The system honors the new settings.
*
*
*
* @param putAppInstanceUserExpirationSettingsRequest
* @return A Java Future containing the result of the PutAppInstanceUserExpirationSettings operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsync.PutAppInstanceUserExpirationSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future putAppInstanceUserExpirationSettingsAsync(
PutAppInstanceUserExpirationSettingsRequest putAppInstanceUserExpirationSettingsRequest);
/**
*
* Sets the number of days before the AppInstanceUser
is automatically deleted.
*
*
*
* A background process deletes expired AppInstanceUsers
within 6 hours of expiration. Actual deletion
* times may vary.
*
*
* Expired AppInstanceUsers
that have not yet been deleted appear as active, and you can update their
* expiration settings. The system honors the new settings.
*
*
*
* @param putAppInstanceUserExpirationSettingsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the PutAppInstanceUserExpirationSettings operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsyncHandler.PutAppInstanceUserExpirationSettings
* @see AWS API Documentation
*/
java.util.concurrent.Future putAppInstanceUserExpirationSettingsAsync(
PutAppInstanceUserExpirationSettingsRequest putAppInstanceUserExpirationSettingsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Registers an endpoint under an Amazon Chime AppInstanceUser
. The endpoint receives messages for a
* user. For push notifications, the endpoint is a mobile device used to receive mobile push notifications for a
* user.
*
*
* @param registerAppInstanceUserEndpointRequest
* @return A Java Future containing the result of the RegisterAppInstanceUserEndpoint operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsync.RegisterAppInstanceUserEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future registerAppInstanceUserEndpointAsync(
RegisterAppInstanceUserEndpointRequest registerAppInstanceUserEndpointRequest);
/**
*
* Registers an endpoint under an Amazon Chime AppInstanceUser
. The endpoint receives messages for a
* user. For push notifications, the endpoint is a mobile device used to receive mobile push notifications for a
* user.
*
*
* @param registerAppInstanceUserEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the RegisterAppInstanceUserEndpoint operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsyncHandler.RegisterAppInstanceUserEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future registerAppInstanceUserEndpointAsync(
RegisterAppInstanceUserEndpointRequest registerAppInstanceUserEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Applies the specified tags to the specified Amazon Chime SDK identity resource.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Applies the specified tags to the specified Amazon Chime SDK identity resource.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified tags from the specified Amazon Chime SDK identity resource.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes the specified tags from the specified Amazon Chime SDK identity resource.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates AppInstance
metadata.
*
*
* @param updateAppInstanceRequest
* @return A Java Future containing the result of the UpdateAppInstance operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.UpdateAppInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAppInstanceAsync(UpdateAppInstanceRequest updateAppInstanceRequest);
/**
*
* Updates AppInstance
metadata.
*
*
* @param updateAppInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAppInstance operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.UpdateAppInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAppInstanceAsync(UpdateAppInstanceRequest updateAppInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the name and metadata of an AppInstanceBot
.
*
*
* @param updateAppInstanceBotRequest
* @return A Java Future containing the result of the UpdateAppInstanceBot operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.UpdateAppInstanceBot
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAppInstanceBotAsync(UpdateAppInstanceBotRequest updateAppInstanceBotRequest);
/**
*
* Updates the name and metadata of an AppInstanceBot
.
*
*
* @param updateAppInstanceBotRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAppInstanceBot operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.UpdateAppInstanceBot
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAppInstanceBotAsync(UpdateAppInstanceBotRequest updateAppInstanceBotRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the details of an AppInstanceUser
. You can update names and metadata.
*
*
* @param updateAppInstanceUserRequest
* @return A Java Future containing the result of the UpdateAppInstanceUser operation returned by the service.
* @sample AmazonChimeSDKIdentityAsync.UpdateAppInstanceUser
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAppInstanceUserAsync(UpdateAppInstanceUserRequest updateAppInstanceUserRequest);
/**
*
* Updates the details of an AppInstanceUser
. You can update names and metadata.
*
*
* @param updateAppInstanceUserRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAppInstanceUser operation returned by the service.
* @sample AmazonChimeSDKIdentityAsyncHandler.UpdateAppInstanceUser
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAppInstanceUserAsync(UpdateAppInstanceUserRequest updateAppInstanceUserRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the details of an AppInstanceUserEndpoint
. You can update the name and
* AllowMessage
values.
*
*
* @param updateAppInstanceUserEndpointRequest
* @return A Java Future containing the result of the UpdateAppInstanceUserEndpoint operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsync.UpdateAppInstanceUserEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAppInstanceUserEndpointAsync(
UpdateAppInstanceUserEndpointRequest updateAppInstanceUserEndpointRequest);
/**
*
* Updates the details of an AppInstanceUserEndpoint
. You can update the name and
* AllowMessage
values.
*
*
* @param updateAppInstanceUserEndpointRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAppInstanceUserEndpoint operation returned by the
* service.
* @sample AmazonChimeSDKIdentityAsyncHandler.UpdateAppInstanceUserEndpoint
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAppInstanceUserEndpointAsync(
UpdateAppInstanceUserEndpointRequest updateAppInstanceUserEndpointRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}