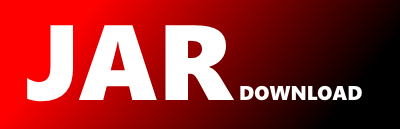
com.amazonaws.services.chimesdkmediapipelines.AmazonChimeSDKMediaPipelines Maven / Gradle / Ivy
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chimesdkmediapipelines;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.chimesdkmediapipelines.model.*;
/**
* Interface for accessing Amazon Chime SDK Media Pipelines.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.chimesdkmediapipelines.AbstractAmazonChimeSDKMediaPipelines} instead.
*
*
*
* The Amazon Chime SDK media pipeline APIs in this section allow software developers to create Amazon Chime SDK media
* pipelines that capture, concatenate, or stream your Amazon Chime SDK meetings. For more information about media
* pipelines, see Amazon Chime SDK media pipelines.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonChimeSDKMediaPipelines {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "media-pipelines-chime";
/**
*
* Creates a media pipeline.
*
*
* @param createMediaCapturePipelineRequest
* @return Result of the CreateMediaCapturePipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.CreateMediaCapturePipeline
* @see AWS API Documentation
*/
CreateMediaCapturePipelineResult createMediaCapturePipeline(CreateMediaCapturePipelineRequest createMediaCapturePipelineRequest);
/**
*
* Creates a media concatenation pipeline.
*
*
* @param createMediaConcatenationPipelineRequest
* @return Result of the CreateMediaConcatenationPipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.CreateMediaConcatenationPipeline
* @see AWS API Documentation
*/
CreateMediaConcatenationPipelineResult createMediaConcatenationPipeline(CreateMediaConcatenationPipelineRequest createMediaConcatenationPipelineRequest);
/**
*
* Creates a media insights pipeline.
*
*
* @param createMediaInsightsPipelineRequest
* @return Result of the CreateMediaInsightsPipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.CreateMediaInsightsPipeline
* @see AWS API Documentation
*/
CreateMediaInsightsPipelineResult createMediaInsightsPipeline(CreateMediaInsightsPipelineRequest createMediaInsightsPipelineRequest);
/**
*
* A structure that contains the static configurations for a media insights pipeline.
*
*
* @param createMediaInsightsPipelineConfigurationRequest
* @return Result of the CreateMediaInsightsPipelineConfiguration operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.CreateMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
CreateMediaInsightsPipelineConfigurationResult createMediaInsightsPipelineConfiguration(
CreateMediaInsightsPipelineConfigurationRequest createMediaInsightsPipelineConfigurationRequest);
/**
*
* Creates a media live connector pipeline in an Amazon Chime SDK meeting.
*
*
* @param createMediaLiveConnectorPipelineRequest
* @return Result of the CreateMediaLiveConnectorPipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.CreateMediaLiveConnectorPipeline
* @see AWS API Documentation
*/
CreateMediaLiveConnectorPipelineResult createMediaLiveConnectorPipeline(CreateMediaLiveConnectorPipelineRequest createMediaLiveConnectorPipelineRequest);
/**
*
* Creates an Amazon Kinesis Video Stream pool for use with media stream pipelines.
*
*
*
* If a meeting uses an opt-in Region as its MediaRegion, the KVS stream must be in that same Region. For example, if a meeting uses the
* af-south-1
Region, the KVS stream must also be in af-south-1
. However, if the meeting
* uses a Region that AWS turns on by default, the KVS stream can be in any available Region, including an opt-in
* Region. For example, if the meeting uses ca-central-1
, the KVS stream can be in
* eu-west-2
, us-east-1
, af-south-1
, or any other Region that the Amazon
* Chime SDK supports.
*
*
* To learn which AWS Region a meeting uses, call the GetMeeting
* API and use the MediaRegion parameter from the response.
*
*
* For more information about opt-in Regions, refer to Available Regions in the
* Amazon Chime SDK Developer Guide, and Specify which AWS Regions your account can use, in the AWS Account Management Reference Guide.
*
*
*
* @param createMediaPipelineKinesisVideoStreamPoolRequest
* @return Result of the CreateMediaPipelineKinesisVideoStreamPool operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.CreateMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
CreateMediaPipelineKinesisVideoStreamPoolResult createMediaPipelineKinesisVideoStreamPool(
CreateMediaPipelineKinesisVideoStreamPoolRequest createMediaPipelineKinesisVideoStreamPoolRequest);
/**
*
* Creates a streaming media pipeline.
*
*
* @param createMediaStreamPipelineRequest
* @return Result of the CreateMediaStreamPipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.CreateMediaStreamPipeline
* @see AWS API Documentation
*/
CreateMediaStreamPipelineResult createMediaStreamPipeline(CreateMediaStreamPipelineRequest createMediaStreamPipelineRequest);
/**
*
* Deletes the media pipeline.
*
*
* @param deleteMediaCapturePipelineRequest
* @return Result of the DeleteMediaCapturePipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.DeleteMediaCapturePipeline
* @see AWS API Documentation
*/
DeleteMediaCapturePipelineResult deleteMediaCapturePipeline(DeleteMediaCapturePipelineRequest deleteMediaCapturePipelineRequest);
/**
*
* Deletes the specified configuration settings.
*
*
* @param deleteMediaInsightsPipelineConfigurationRequest
* @return Result of the DeleteMediaInsightsPipelineConfiguration operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.DeleteMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
DeleteMediaInsightsPipelineConfigurationResult deleteMediaInsightsPipelineConfiguration(
DeleteMediaInsightsPipelineConfigurationRequest deleteMediaInsightsPipelineConfigurationRequest);
/**
*
* Deletes the media pipeline.
*
*
* @param deleteMediaPipelineRequest
* @return Result of the DeleteMediaPipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.DeleteMediaPipeline
* @see AWS API Documentation
*/
DeleteMediaPipelineResult deleteMediaPipeline(DeleteMediaPipelineRequest deleteMediaPipelineRequest);
/**
*
* Deletes an Amazon Kinesis Video Stream pool.
*
*
* @param deleteMediaPipelineKinesisVideoStreamPoolRequest
* @return Result of the DeleteMediaPipelineKinesisVideoStreamPool operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.DeleteMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
DeleteMediaPipelineKinesisVideoStreamPoolResult deleteMediaPipelineKinesisVideoStreamPool(
DeleteMediaPipelineKinesisVideoStreamPoolRequest deleteMediaPipelineKinesisVideoStreamPoolRequest);
/**
*
* Gets an existing media pipeline.
*
*
* @param getMediaCapturePipelineRequest
* @return Result of the GetMediaCapturePipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.GetMediaCapturePipeline
* @see AWS API Documentation
*/
GetMediaCapturePipelineResult getMediaCapturePipeline(GetMediaCapturePipelineRequest getMediaCapturePipelineRequest);
/**
*
* Gets the configuration settings for a media insights pipeline.
*
*
* @param getMediaInsightsPipelineConfigurationRequest
* @return Result of the GetMediaInsightsPipelineConfiguration operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.GetMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
GetMediaInsightsPipelineConfigurationResult getMediaInsightsPipelineConfiguration(
GetMediaInsightsPipelineConfigurationRequest getMediaInsightsPipelineConfigurationRequest);
/**
*
* Gets an existing media pipeline.
*
*
* @param getMediaPipelineRequest
* @return Result of the GetMediaPipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.GetMediaPipeline
* @see AWS API Documentation
*/
GetMediaPipelineResult getMediaPipeline(GetMediaPipelineRequest getMediaPipelineRequest);
/**
*
* Gets an Kinesis video stream pool.
*
*
* @param getMediaPipelineKinesisVideoStreamPoolRequest
* @return Result of the GetMediaPipelineKinesisVideoStreamPool operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.GetMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
GetMediaPipelineKinesisVideoStreamPoolResult getMediaPipelineKinesisVideoStreamPool(
GetMediaPipelineKinesisVideoStreamPoolRequest getMediaPipelineKinesisVideoStreamPoolRequest);
/**
*
* Retrieves the details of the specified speaker search task.
*
*
* @param getSpeakerSearchTaskRequest
* @return Result of the GetSpeakerSearchTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.GetSpeakerSearchTask
* @see AWS API Documentation
*/
GetSpeakerSearchTaskResult getSpeakerSearchTask(GetSpeakerSearchTaskRequest getSpeakerSearchTaskRequest);
/**
*
* Retrieves the details of a voice tone analysis task.
*
*
* @param getVoiceToneAnalysisTaskRequest
* @return Result of the GetVoiceToneAnalysisTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.GetVoiceToneAnalysisTask
* @see AWS API Documentation
*/
GetVoiceToneAnalysisTaskResult getVoiceToneAnalysisTask(GetVoiceToneAnalysisTaskRequest getVoiceToneAnalysisTaskRequest);
/**
*
* Returns a list of media pipelines.
*
*
* @param listMediaCapturePipelinesRequest
* @return Result of the ListMediaCapturePipelines operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.ListMediaCapturePipelines
* @see AWS API Documentation
*/
ListMediaCapturePipelinesResult listMediaCapturePipelines(ListMediaCapturePipelinesRequest listMediaCapturePipelinesRequest);
/**
*
* Lists the available media insights pipeline configurations.
*
*
* @param listMediaInsightsPipelineConfigurationsRequest
* @return Result of the ListMediaInsightsPipelineConfigurations operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.ListMediaInsightsPipelineConfigurations
* @see AWS API Documentation
*/
ListMediaInsightsPipelineConfigurationsResult listMediaInsightsPipelineConfigurations(
ListMediaInsightsPipelineConfigurationsRequest listMediaInsightsPipelineConfigurationsRequest);
/**
*
* Lists the video stream pools in the media pipeline.
*
*
* @param listMediaPipelineKinesisVideoStreamPoolsRequest
* @return Result of the ListMediaPipelineKinesisVideoStreamPools operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.ListMediaPipelineKinesisVideoStreamPools
* @see AWS API Documentation
*/
ListMediaPipelineKinesisVideoStreamPoolsResult listMediaPipelineKinesisVideoStreamPools(
ListMediaPipelineKinesisVideoStreamPoolsRequest listMediaPipelineKinesisVideoStreamPoolsRequest);
/**
*
* Returns a list of media pipelines.
*
*
* @param listMediaPipelinesRequest
* @return Result of the ListMediaPipelines operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.ListMediaPipelines
* @see AWS API Documentation
*/
ListMediaPipelinesResult listMediaPipelines(ListMediaPipelinesRequest listMediaPipelinesRequest);
/**
*
* Lists the tags available for a media pipeline.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.ListTagsForResource
* @see AWS API Documentation
*/
ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Starts a speaker search task.
*
*
*
* Before starting any speaker search tasks, you must provide all notices and obtain all consents from the speaker
* as required under applicable privacy and biometrics laws, and as required under the AWS service terms for the Amazon Chime SDK.
*
*
*
* @param startSpeakerSearchTaskRequest
* @return Result of the StartSpeakerSearchTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.StartSpeakerSearchTask
* @see AWS API Documentation
*/
StartSpeakerSearchTaskResult startSpeakerSearchTask(StartSpeakerSearchTaskRequest startSpeakerSearchTaskRequest);
/**
*
* Starts a voice tone analysis task. For more information about voice tone analysis, see Using Amazon Chime SDK voice
* analytics in the Amazon Chime SDK Developer Guide.
*
*
*
* Before starting any voice tone analysis tasks, you must provide all notices and obtain all consents from the
* speaker as required under applicable privacy and biometrics laws, and as required under the AWS service terms for the Amazon Chime SDK.
*
*
*
* @param startVoiceToneAnalysisTaskRequest
* @return Result of the StartVoiceToneAnalysisTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.StartVoiceToneAnalysisTask
* @see AWS API Documentation
*/
StartVoiceToneAnalysisTaskResult startVoiceToneAnalysisTask(StartVoiceToneAnalysisTaskRequest startVoiceToneAnalysisTaskRequest);
/**
*
* Stops a speaker search task.
*
*
* @param stopSpeakerSearchTaskRequest
* @return Result of the StopSpeakerSearchTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.StopSpeakerSearchTask
* @see AWS API Documentation
*/
StopSpeakerSearchTaskResult stopSpeakerSearchTask(StopSpeakerSearchTaskRequest stopSpeakerSearchTaskRequest);
/**
*
* Stops a voice tone analysis task.
*
*
* @param stopVoiceToneAnalysisTaskRequest
* @return Result of the StopVoiceToneAnalysisTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.StopVoiceToneAnalysisTask
* @see AWS API Documentation
*/
StopVoiceToneAnalysisTaskResult stopVoiceToneAnalysisTask(StopVoiceToneAnalysisTaskRequest stopVoiceToneAnalysisTaskRequest);
/**
*
* The ARN of the media pipeline that you want to tag. Consists of the pipeline's endpoint region, resource ID, and
* pipeline ID.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.TagResource
* @see AWS API Documentation
*/
TagResourceResult tagResource(TagResourceRequest tagResourceRequest);
/**
*
* Removes any tags from a media pipeline.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.UntagResource
* @see AWS API Documentation
*/
UntagResourceResult untagResource(UntagResourceRequest untagResourceRequest);
/**
*
* Updates the media insights pipeline's configuration settings.
*
*
* @param updateMediaInsightsPipelineConfigurationRequest
* @return Result of the UpdateMediaInsightsPipelineConfiguration operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.UpdateMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
UpdateMediaInsightsPipelineConfigurationResult updateMediaInsightsPipelineConfiguration(
UpdateMediaInsightsPipelineConfigurationRequest updateMediaInsightsPipelineConfigurationRequest);
/**
*
* Updates the status of a media insights pipeline.
*
*
* @param updateMediaInsightsPipelineStatusRequest
* @return Result of the UpdateMediaInsightsPipelineStatus operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.UpdateMediaInsightsPipelineStatus
* @see AWS API Documentation
*/
UpdateMediaInsightsPipelineStatusResult updateMediaInsightsPipelineStatus(UpdateMediaInsightsPipelineStatusRequest updateMediaInsightsPipelineStatusRequest);
/**
*
* Updates an Amazon Kinesis Video Stream pool in a media pipeline.
*
*
* @param updateMediaPipelineKinesisVideoStreamPoolRequest
* @return Result of the UpdateMediaPipelineKinesisVideoStreamPool operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.UpdateMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
UpdateMediaPipelineKinesisVideoStreamPoolResult updateMediaPipelineKinesisVideoStreamPool(
UpdateMediaPipelineKinesisVideoStreamPoolRequest updateMediaPipelineKinesisVideoStreamPoolRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
}