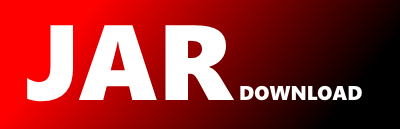
com.amazonaws.services.chimesdkmediapipelines.AmazonChimeSDKMediaPipelinesAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chimesdkmediapipelines Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chimesdkmediapipelines;
import javax.annotation.Generated;
import com.amazonaws.services.chimesdkmediapipelines.model.*;
/**
* Interface for accessing Amazon Chime SDK Media Pipelines asynchronously. Each asynchronous method will return a Java
* Future object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to
* receive notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.chimesdkmediapipelines.AbstractAmazonChimeSDKMediaPipelinesAsync} instead.
*
*
*
* The Amazon Chime SDK media pipeline APIs in this section allow software developers to create Amazon Chime SDK media
* pipelines that capture, concatenate, or stream your Amazon Chime SDK meetings. For more information about media
* pipelines, see Amazon Chime SDK media pipelines.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonChimeSDKMediaPipelinesAsync extends AmazonChimeSDKMediaPipelines {
/**
*
* Creates a media pipeline.
*
*
* @param createMediaCapturePipelineRequest
* @return A Java Future containing the result of the CreateMediaCapturePipeline operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.CreateMediaCapturePipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future createMediaCapturePipelineAsync(
CreateMediaCapturePipelineRequest createMediaCapturePipelineRequest);
/**
*
* Creates a media pipeline.
*
*
* @param createMediaCapturePipelineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMediaCapturePipeline operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.CreateMediaCapturePipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future createMediaCapturePipelineAsync(
CreateMediaCapturePipelineRequest createMediaCapturePipelineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a media concatenation pipeline.
*
*
* @param createMediaConcatenationPipelineRequest
* @return A Java Future containing the result of the CreateMediaConcatenationPipeline operation returned by the
* service.
* @sample AmazonChimeSDKMediaPipelinesAsync.CreateMediaConcatenationPipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future createMediaConcatenationPipelineAsync(
CreateMediaConcatenationPipelineRequest createMediaConcatenationPipelineRequest);
/**
*
* Creates a media concatenation pipeline.
*
*
* @param createMediaConcatenationPipelineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMediaConcatenationPipeline operation returned by the
* service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.CreateMediaConcatenationPipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future createMediaConcatenationPipelineAsync(
CreateMediaConcatenationPipelineRequest createMediaConcatenationPipelineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a media insights pipeline.
*
*
* @param createMediaInsightsPipelineRequest
* @return A Java Future containing the result of the CreateMediaInsightsPipeline operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.CreateMediaInsightsPipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future createMediaInsightsPipelineAsync(
CreateMediaInsightsPipelineRequest createMediaInsightsPipelineRequest);
/**
*
* Creates a media insights pipeline.
*
*
* @param createMediaInsightsPipelineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMediaInsightsPipeline operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.CreateMediaInsightsPipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future createMediaInsightsPipelineAsync(
CreateMediaInsightsPipelineRequest createMediaInsightsPipelineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* A structure that contains the static configurations for a media insights pipeline.
*
*
* @param createMediaInsightsPipelineConfigurationRequest
* @return A Java Future containing the result of the CreateMediaInsightsPipelineConfiguration operation returned by
* the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.CreateMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createMediaInsightsPipelineConfigurationAsync(
CreateMediaInsightsPipelineConfigurationRequest createMediaInsightsPipelineConfigurationRequest);
/**
*
* A structure that contains the static configurations for a media insights pipeline.
*
*
* @param createMediaInsightsPipelineConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMediaInsightsPipelineConfiguration operation returned by
* the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.CreateMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future createMediaInsightsPipelineConfigurationAsync(
CreateMediaInsightsPipelineConfigurationRequest createMediaInsightsPipelineConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a media live connector pipeline in an Amazon Chime SDK meeting.
*
*
* @param createMediaLiveConnectorPipelineRequest
* @return A Java Future containing the result of the CreateMediaLiveConnectorPipeline operation returned by the
* service.
* @sample AmazonChimeSDKMediaPipelinesAsync.CreateMediaLiveConnectorPipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future createMediaLiveConnectorPipelineAsync(
CreateMediaLiveConnectorPipelineRequest createMediaLiveConnectorPipelineRequest);
/**
*
* Creates a media live connector pipeline in an Amazon Chime SDK meeting.
*
*
* @param createMediaLiveConnectorPipelineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMediaLiveConnectorPipeline operation returned by the
* service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.CreateMediaLiveConnectorPipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future createMediaLiveConnectorPipelineAsync(
CreateMediaLiveConnectorPipelineRequest createMediaLiveConnectorPipelineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates an Amazon Kinesis Video Stream pool for use with media stream pipelines.
*
*
*
* If a meeting uses an opt-in Region as its MediaRegion, the KVS stream must be in that same Region. For example, if a meeting uses the
* af-south-1
Region, the KVS stream must also be in af-south-1
. However, if the meeting
* uses a Region that AWS turns on by default, the KVS stream can be in any available Region, including an opt-in
* Region. For example, if the meeting uses ca-central-1
, the KVS stream can be in
* eu-west-2
, us-east-1
, af-south-1
, or any other Region that the Amazon
* Chime SDK supports.
*
*
* To learn which AWS Region a meeting uses, call the GetMeeting
* API and use the MediaRegion parameter from the response.
*
*
* For more information about opt-in Regions, refer to Available Regions in the
* Amazon Chime SDK Developer Guide, and Specify which AWS Regions your account can use, in the AWS Account Management Reference Guide.
*
*
*
* @param createMediaPipelineKinesisVideoStreamPoolRequest
* @return A Java Future containing the result of the CreateMediaPipelineKinesisVideoStreamPool operation returned
* by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.CreateMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
java.util.concurrent.Future createMediaPipelineKinesisVideoStreamPoolAsync(
CreateMediaPipelineKinesisVideoStreamPoolRequest createMediaPipelineKinesisVideoStreamPoolRequest);
/**
*
* Creates an Amazon Kinesis Video Stream pool for use with media stream pipelines.
*
*
*
* If a meeting uses an opt-in Region as its MediaRegion, the KVS stream must be in that same Region. For example, if a meeting uses the
* af-south-1
Region, the KVS stream must also be in af-south-1
. However, if the meeting
* uses a Region that AWS turns on by default, the KVS stream can be in any available Region, including an opt-in
* Region. For example, if the meeting uses ca-central-1
, the KVS stream can be in
* eu-west-2
, us-east-1
, af-south-1
, or any other Region that the Amazon
* Chime SDK supports.
*
*
* To learn which AWS Region a meeting uses, call the GetMeeting
* API and use the MediaRegion parameter from the response.
*
*
* For more information about opt-in Regions, refer to Available Regions in the
* Amazon Chime SDK Developer Guide, and Specify which AWS Regions your account can use, in the AWS Account Management Reference Guide.
*
*
*
* @param createMediaPipelineKinesisVideoStreamPoolRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMediaPipelineKinesisVideoStreamPool operation returned
* by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.CreateMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
java.util.concurrent.Future createMediaPipelineKinesisVideoStreamPoolAsync(
CreateMediaPipelineKinesisVideoStreamPoolRequest createMediaPipelineKinesisVideoStreamPoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a streaming media pipeline.
*
*
* @param createMediaStreamPipelineRequest
* @return A Java Future containing the result of the CreateMediaStreamPipeline operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.CreateMediaStreamPipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future createMediaStreamPipelineAsync(
CreateMediaStreamPipelineRequest createMediaStreamPipelineRequest);
/**
*
* Creates a streaming media pipeline.
*
*
* @param createMediaStreamPipelineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMediaStreamPipeline operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.CreateMediaStreamPipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future createMediaStreamPipelineAsync(
CreateMediaStreamPipelineRequest createMediaStreamPipelineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the media pipeline.
*
*
* @param deleteMediaCapturePipelineRequest
* @return A Java Future containing the result of the DeleteMediaCapturePipeline operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.DeleteMediaCapturePipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMediaCapturePipelineAsync(
DeleteMediaCapturePipelineRequest deleteMediaCapturePipelineRequest);
/**
*
* Deletes the media pipeline.
*
*
* @param deleteMediaCapturePipelineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMediaCapturePipeline operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.DeleteMediaCapturePipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMediaCapturePipelineAsync(
DeleteMediaCapturePipelineRequest deleteMediaCapturePipelineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified configuration settings.
*
*
* @param deleteMediaInsightsPipelineConfigurationRequest
* @return A Java Future containing the result of the DeleteMediaInsightsPipelineConfiguration operation returned by
* the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.DeleteMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMediaInsightsPipelineConfigurationAsync(
DeleteMediaInsightsPipelineConfigurationRequest deleteMediaInsightsPipelineConfigurationRequest);
/**
*
* Deletes the specified configuration settings.
*
*
* @param deleteMediaInsightsPipelineConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMediaInsightsPipelineConfiguration operation returned by
* the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.DeleteMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMediaInsightsPipelineConfigurationAsync(
DeleteMediaInsightsPipelineConfigurationRequest deleteMediaInsightsPipelineConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the media pipeline.
*
*
* @param deleteMediaPipelineRequest
* @return A Java Future containing the result of the DeleteMediaPipeline operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.DeleteMediaPipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMediaPipelineAsync(DeleteMediaPipelineRequest deleteMediaPipelineRequest);
/**
*
* Deletes the media pipeline.
*
*
* @param deleteMediaPipelineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMediaPipeline operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.DeleteMediaPipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMediaPipelineAsync(DeleteMediaPipelineRequest deleteMediaPipelineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Amazon Kinesis Video Stream pool.
*
*
* @param deleteMediaPipelineKinesisVideoStreamPoolRequest
* @return A Java Future containing the result of the DeleteMediaPipelineKinesisVideoStreamPool operation returned
* by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.DeleteMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMediaPipelineKinesisVideoStreamPoolAsync(
DeleteMediaPipelineKinesisVideoStreamPoolRequest deleteMediaPipelineKinesisVideoStreamPoolRequest);
/**
*
* Deletes an Amazon Kinesis Video Stream pool.
*
*
* @param deleteMediaPipelineKinesisVideoStreamPoolRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMediaPipelineKinesisVideoStreamPool operation returned
* by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.DeleteMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMediaPipelineKinesisVideoStreamPoolAsync(
DeleteMediaPipelineKinesisVideoStreamPoolRequest deleteMediaPipelineKinesisVideoStreamPoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets an existing media pipeline.
*
*
* @param getMediaCapturePipelineRequest
* @return A Java Future containing the result of the GetMediaCapturePipeline operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.GetMediaCapturePipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future getMediaCapturePipelineAsync(GetMediaCapturePipelineRequest getMediaCapturePipelineRequest);
/**
*
* Gets an existing media pipeline.
*
*
* @param getMediaCapturePipelineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMediaCapturePipeline operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.GetMediaCapturePipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future getMediaCapturePipelineAsync(GetMediaCapturePipelineRequest getMediaCapturePipelineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the configuration settings for a media insights pipeline.
*
*
* @param getMediaInsightsPipelineConfigurationRequest
* @return A Java Future containing the result of the GetMediaInsightsPipelineConfiguration operation returned by
* the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.GetMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getMediaInsightsPipelineConfigurationAsync(
GetMediaInsightsPipelineConfigurationRequest getMediaInsightsPipelineConfigurationRequest);
/**
*
* Gets the configuration settings for a media insights pipeline.
*
*
* @param getMediaInsightsPipelineConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMediaInsightsPipelineConfiguration operation returned by
* the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.GetMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future getMediaInsightsPipelineConfigurationAsync(
GetMediaInsightsPipelineConfigurationRequest getMediaInsightsPipelineConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets an existing media pipeline.
*
*
* @param getMediaPipelineRequest
* @return A Java Future containing the result of the GetMediaPipeline operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.GetMediaPipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future getMediaPipelineAsync(GetMediaPipelineRequest getMediaPipelineRequest);
/**
*
* Gets an existing media pipeline.
*
*
* @param getMediaPipelineRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMediaPipeline operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.GetMediaPipeline
* @see AWS API Documentation
*/
java.util.concurrent.Future getMediaPipelineAsync(GetMediaPipelineRequest getMediaPipelineRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets an Kinesis video stream pool.
*
*
* @param getMediaPipelineKinesisVideoStreamPoolRequest
* @return A Java Future containing the result of the GetMediaPipelineKinesisVideoStreamPool operation returned by
* the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.GetMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
java.util.concurrent.Future getMediaPipelineKinesisVideoStreamPoolAsync(
GetMediaPipelineKinesisVideoStreamPoolRequest getMediaPipelineKinesisVideoStreamPoolRequest);
/**
*
* Gets an Kinesis video stream pool.
*
*
* @param getMediaPipelineKinesisVideoStreamPoolRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMediaPipelineKinesisVideoStreamPool operation returned by
* the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.GetMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
java.util.concurrent.Future getMediaPipelineKinesisVideoStreamPoolAsync(
GetMediaPipelineKinesisVideoStreamPoolRequest getMediaPipelineKinesisVideoStreamPoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the details of the specified speaker search task.
*
*
* @param getSpeakerSearchTaskRequest
* @return A Java Future containing the result of the GetSpeakerSearchTask operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.GetSpeakerSearchTask
* @see AWS API Documentation
*/
java.util.concurrent.Future getSpeakerSearchTaskAsync(GetSpeakerSearchTaskRequest getSpeakerSearchTaskRequest);
/**
*
* Retrieves the details of the specified speaker search task.
*
*
* @param getSpeakerSearchTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetSpeakerSearchTask operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.GetSpeakerSearchTask
* @see AWS API Documentation
*/
java.util.concurrent.Future getSpeakerSearchTaskAsync(GetSpeakerSearchTaskRequest getSpeakerSearchTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves the details of a voice tone analysis task.
*
*
* @param getVoiceToneAnalysisTaskRequest
* @return A Java Future containing the result of the GetVoiceToneAnalysisTask operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.GetVoiceToneAnalysisTask
* @see AWS API Documentation
*/
java.util.concurrent.Future getVoiceToneAnalysisTaskAsync(GetVoiceToneAnalysisTaskRequest getVoiceToneAnalysisTaskRequest);
/**
*
* Retrieves the details of a voice tone analysis task.
*
*
* @param getVoiceToneAnalysisTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetVoiceToneAnalysisTask operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.GetVoiceToneAnalysisTask
* @see AWS API Documentation
*/
java.util.concurrent.Future getVoiceToneAnalysisTaskAsync(GetVoiceToneAnalysisTaskRequest getVoiceToneAnalysisTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of media pipelines.
*
*
* @param listMediaCapturePipelinesRequest
* @return A Java Future containing the result of the ListMediaCapturePipelines operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.ListMediaCapturePipelines
* @see AWS API Documentation
*/
java.util.concurrent.Future listMediaCapturePipelinesAsync(
ListMediaCapturePipelinesRequest listMediaCapturePipelinesRequest);
/**
*
* Returns a list of media pipelines.
*
*
* @param listMediaCapturePipelinesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMediaCapturePipelines operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.ListMediaCapturePipelines
* @see AWS API Documentation
*/
java.util.concurrent.Future listMediaCapturePipelinesAsync(
ListMediaCapturePipelinesRequest listMediaCapturePipelinesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the available media insights pipeline configurations.
*
*
* @param listMediaInsightsPipelineConfigurationsRequest
* @return A Java Future containing the result of the ListMediaInsightsPipelineConfigurations operation returned by
* the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.ListMediaInsightsPipelineConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listMediaInsightsPipelineConfigurationsAsync(
ListMediaInsightsPipelineConfigurationsRequest listMediaInsightsPipelineConfigurationsRequest);
/**
*
* Lists the available media insights pipeline configurations.
*
*
* @param listMediaInsightsPipelineConfigurationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMediaInsightsPipelineConfigurations operation returned by
* the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.ListMediaInsightsPipelineConfigurations
* @see AWS API Documentation
*/
java.util.concurrent.Future listMediaInsightsPipelineConfigurationsAsync(
ListMediaInsightsPipelineConfigurationsRequest listMediaInsightsPipelineConfigurationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the video stream pools in the media pipeline.
*
*
* @param listMediaPipelineKinesisVideoStreamPoolsRequest
* @return A Java Future containing the result of the ListMediaPipelineKinesisVideoStreamPools operation returned by
* the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.ListMediaPipelineKinesisVideoStreamPools
* @see AWS API Documentation
*/
java.util.concurrent.Future listMediaPipelineKinesisVideoStreamPoolsAsync(
ListMediaPipelineKinesisVideoStreamPoolsRequest listMediaPipelineKinesisVideoStreamPoolsRequest);
/**
*
* Lists the video stream pools in the media pipeline.
*
*
* @param listMediaPipelineKinesisVideoStreamPoolsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMediaPipelineKinesisVideoStreamPools operation returned by
* the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.ListMediaPipelineKinesisVideoStreamPools
* @see AWS API Documentation
*/
java.util.concurrent.Future listMediaPipelineKinesisVideoStreamPoolsAsync(
ListMediaPipelineKinesisVideoStreamPoolsRequest listMediaPipelineKinesisVideoStreamPoolsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of media pipelines.
*
*
* @param listMediaPipelinesRequest
* @return A Java Future containing the result of the ListMediaPipelines operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.ListMediaPipelines
* @see AWS API Documentation
*/
java.util.concurrent.Future listMediaPipelinesAsync(ListMediaPipelinesRequest listMediaPipelinesRequest);
/**
*
* Returns a list of media pipelines.
*
*
* @param listMediaPipelinesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListMediaPipelines operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.ListMediaPipelines
* @see AWS API Documentation
*/
java.util.concurrent.Future listMediaPipelinesAsync(ListMediaPipelinesRequest listMediaPipelinesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the tags available for a media pipeline.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Lists the tags available for a media pipeline.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a speaker search task.
*
*
*
* Before starting any speaker search tasks, you must provide all notices and obtain all consents from the speaker
* as required under applicable privacy and biometrics laws, and as required under the AWS service terms for the Amazon Chime SDK.
*
*
*
* @param startSpeakerSearchTaskRequest
* @return A Java Future containing the result of the StartSpeakerSearchTask operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.StartSpeakerSearchTask
* @see AWS API Documentation
*/
java.util.concurrent.Future startSpeakerSearchTaskAsync(StartSpeakerSearchTaskRequest startSpeakerSearchTaskRequest);
/**
*
* Starts a speaker search task.
*
*
*
* Before starting any speaker search tasks, you must provide all notices and obtain all consents from the speaker
* as required under applicable privacy and biometrics laws, and as required under the AWS service terms for the Amazon Chime SDK.
*
*
*
* @param startSpeakerSearchTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartSpeakerSearchTask operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.StartSpeakerSearchTask
* @see AWS API Documentation
*/
java.util.concurrent.Future startSpeakerSearchTaskAsync(StartSpeakerSearchTaskRequest startSpeakerSearchTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts a voice tone analysis task. For more information about voice tone analysis, see Using Amazon Chime SDK voice
* analytics in the Amazon Chime SDK Developer Guide.
*
*
*
* Before starting any voice tone analysis tasks, you must provide all notices and obtain all consents from the
* speaker as required under applicable privacy and biometrics laws, and as required under the AWS service terms for the Amazon Chime SDK.
*
*
*
* @param startVoiceToneAnalysisTaskRequest
* @return A Java Future containing the result of the StartVoiceToneAnalysisTask operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.StartVoiceToneAnalysisTask
* @see AWS API Documentation
*/
java.util.concurrent.Future startVoiceToneAnalysisTaskAsync(
StartVoiceToneAnalysisTaskRequest startVoiceToneAnalysisTaskRequest);
/**
*
* Starts a voice tone analysis task. For more information about voice tone analysis, see Using Amazon Chime SDK voice
* analytics in the Amazon Chime SDK Developer Guide.
*
*
*
* Before starting any voice tone analysis tasks, you must provide all notices and obtain all consents from the
* speaker as required under applicable privacy and biometrics laws, and as required under the AWS service terms for the Amazon Chime SDK.
*
*
*
* @param startVoiceToneAnalysisTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartVoiceToneAnalysisTask operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.StartVoiceToneAnalysisTask
* @see AWS API Documentation
*/
java.util.concurrent.Future startVoiceToneAnalysisTaskAsync(
StartVoiceToneAnalysisTaskRequest startVoiceToneAnalysisTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops a speaker search task.
*
*
* @param stopSpeakerSearchTaskRequest
* @return A Java Future containing the result of the StopSpeakerSearchTask operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.StopSpeakerSearchTask
* @see AWS API Documentation
*/
java.util.concurrent.Future stopSpeakerSearchTaskAsync(StopSpeakerSearchTaskRequest stopSpeakerSearchTaskRequest);
/**
*
* Stops a speaker search task.
*
*
* @param stopSpeakerSearchTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopSpeakerSearchTask operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.StopSpeakerSearchTask
* @see AWS API Documentation
*/
java.util.concurrent.Future stopSpeakerSearchTaskAsync(StopSpeakerSearchTaskRequest stopSpeakerSearchTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops a voice tone analysis task.
*
*
* @param stopVoiceToneAnalysisTaskRequest
* @return A Java Future containing the result of the StopVoiceToneAnalysisTask operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.StopVoiceToneAnalysisTask
* @see AWS API Documentation
*/
java.util.concurrent.Future stopVoiceToneAnalysisTaskAsync(
StopVoiceToneAnalysisTaskRequest stopVoiceToneAnalysisTaskRequest);
/**
*
* Stops a voice tone analysis task.
*
*
* @param stopVoiceToneAnalysisTaskRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopVoiceToneAnalysisTask operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.StopVoiceToneAnalysisTask
* @see AWS API Documentation
*/
java.util.concurrent.Future stopVoiceToneAnalysisTaskAsync(
StopVoiceToneAnalysisTaskRequest stopVoiceToneAnalysisTaskRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The ARN of the media pipeline that you want to tag. Consists of the pipeline's endpoint region, resource ID, and
* pipeline ID.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.TagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* The ARN of the media pipeline that you want to tag. Consists of the pipeline's endpoint region, resource ID, and
* pipeline ID.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.TagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes any tags from a media pipeline.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes any tags from a media pipeline.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the media insights pipeline's configuration settings.
*
*
* @param updateMediaInsightsPipelineConfigurationRequest
* @return A Java Future containing the result of the UpdateMediaInsightsPipelineConfiguration operation returned by
* the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.UpdateMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateMediaInsightsPipelineConfigurationAsync(
UpdateMediaInsightsPipelineConfigurationRequest updateMediaInsightsPipelineConfigurationRequest);
/**
*
* Updates the media insights pipeline's configuration settings.
*
*
* @param updateMediaInsightsPipelineConfigurationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateMediaInsightsPipelineConfiguration operation returned by
* the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.UpdateMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
java.util.concurrent.Future updateMediaInsightsPipelineConfigurationAsync(
UpdateMediaInsightsPipelineConfigurationRequest updateMediaInsightsPipelineConfigurationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the status of a media insights pipeline.
*
*
* @param updateMediaInsightsPipelineStatusRequest
* @return A Java Future containing the result of the UpdateMediaInsightsPipelineStatus operation returned by the
* service.
* @sample AmazonChimeSDKMediaPipelinesAsync.UpdateMediaInsightsPipelineStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future updateMediaInsightsPipelineStatusAsync(
UpdateMediaInsightsPipelineStatusRequest updateMediaInsightsPipelineStatusRequest);
/**
*
* Updates the status of a media insights pipeline.
*
*
* @param updateMediaInsightsPipelineStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateMediaInsightsPipelineStatus operation returned by the
* service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.UpdateMediaInsightsPipelineStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future updateMediaInsightsPipelineStatusAsync(
UpdateMediaInsightsPipelineStatusRequest updateMediaInsightsPipelineStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates an Amazon Kinesis Video Stream pool in a media pipeline.
*
*
* @param updateMediaPipelineKinesisVideoStreamPoolRequest
* @return A Java Future containing the result of the UpdateMediaPipelineKinesisVideoStreamPool operation returned
* by the service.
* @sample AmazonChimeSDKMediaPipelinesAsync.UpdateMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
java.util.concurrent.Future updateMediaPipelineKinesisVideoStreamPoolAsync(
UpdateMediaPipelineKinesisVideoStreamPoolRequest updateMediaPipelineKinesisVideoStreamPoolRequest);
/**
*
* Updates an Amazon Kinesis Video Stream pool in a media pipeline.
*
*
* @param updateMediaPipelineKinesisVideoStreamPoolRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateMediaPipelineKinesisVideoStreamPool operation returned
* by the service.
* @sample AmazonChimeSDKMediaPipelinesAsyncHandler.UpdateMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
java.util.concurrent.Future updateMediaPipelineKinesisVideoStreamPoolAsync(
UpdateMediaPipelineKinesisVideoStreamPoolRequest updateMediaPipelineKinesisVideoStreamPoolRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}