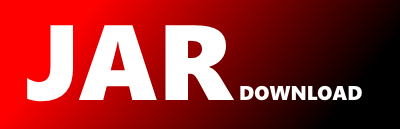
com.amazonaws.services.chimesdkmediapipelines.AmazonChimeSDKMediaPipelinesClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chimesdkmediapipelines Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chimesdkmediapipelines;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.chimesdkmediapipelines.AmazonChimeSDKMediaPipelinesClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.chimesdkmediapipelines.model.*;
import com.amazonaws.services.chimesdkmediapipelines.model.transform.*;
/**
* Client for accessing Amazon Chime SDK Media Pipelines. All service calls made using this client are blocking, and
* will not return until the service call completes.
*
*
* The Amazon Chime SDK media pipeline APIs in this section allow software developers to create Amazon Chime SDK media
* pipelines that capture, concatenate, or stream your Amazon Chime SDK meetings. For more information about media
* pipelines, see Amazon Chime SDK media pipelines.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonChimeSDKMediaPipelinesClient extends AmazonWebServiceClient implements AmazonChimeSDKMediaPipelines {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonChimeSDKMediaPipelines.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "chime";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ForbiddenException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmediapipelines.model.transform.ForbiddenExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmediapipelines.model.transform.ResourceLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnauthorizedClientException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmediapipelines.model.transform.UnauthorizedClientExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmediapipelines.model.transform.NotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceFailureException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmediapipelines.model.transform.ServiceFailureExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmediapipelines.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottledClientException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmediapipelines.model.transform.ThrottledClientExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceUnavailableException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmediapipelines.model.transform.ServiceUnavailableExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BadRequestException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmediapipelines.model.transform.BadRequestExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.chimesdkmediapipelines.model.AmazonChimeSDKMediaPipelinesException.class));
public static AmazonChimeSDKMediaPipelinesClientBuilder builder() {
return AmazonChimeSDKMediaPipelinesClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon Chime SDK Media Pipelines using the specified
* parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonChimeSDKMediaPipelinesClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon Chime SDK Media Pipelines using the specified
* parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonChimeSDKMediaPipelinesClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("media-pipelines-chime.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/chimesdkmediapipelines/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/chimesdkmediapipelines/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Creates a media pipeline.
*
*
* @param createMediaCapturePipelineRequest
* @return Result of the CreateMediaCapturePipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.CreateMediaCapturePipeline
* @see AWS API Documentation
*/
@Override
public CreateMediaCapturePipelineResult createMediaCapturePipeline(CreateMediaCapturePipelineRequest request) {
request = beforeClientExecution(request);
return executeCreateMediaCapturePipeline(request);
}
@SdkInternalApi
final CreateMediaCapturePipelineResult executeCreateMediaCapturePipeline(CreateMediaCapturePipelineRequest createMediaCapturePipelineRequest) {
ExecutionContext executionContext = createExecutionContext(createMediaCapturePipelineRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMediaCapturePipelineRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createMediaCapturePipelineRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMediaCapturePipeline");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateMediaCapturePipelineResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a media concatenation pipeline.
*
*
* @param createMediaConcatenationPipelineRequest
* @return Result of the CreateMediaConcatenationPipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.CreateMediaConcatenationPipeline
* @see AWS API Documentation
*/
@Override
public CreateMediaConcatenationPipelineResult createMediaConcatenationPipeline(CreateMediaConcatenationPipelineRequest request) {
request = beforeClientExecution(request);
return executeCreateMediaConcatenationPipeline(request);
}
@SdkInternalApi
final CreateMediaConcatenationPipelineResult executeCreateMediaConcatenationPipeline(
CreateMediaConcatenationPipelineRequest createMediaConcatenationPipelineRequest) {
ExecutionContext executionContext = createExecutionContext(createMediaConcatenationPipelineRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMediaConcatenationPipelineRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createMediaConcatenationPipelineRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMediaConcatenationPipeline");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateMediaConcatenationPipelineResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a media insights pipeline.
*
*
* @param createMediaInsightsPipelineRequest
* @return Result of the CreateMediaInsightsPipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.CreateMediaInsightsPipeline
* @see AWS API Documentation
*/
@Override
public CreateMediaInsightsPipelineResult createMediaInsightsPipeline(CreateMediaInsightsPipelineRequest request) {
request = beforeClientExecution(request);
return executeCreateMediaInsightsPipeline(request);
}
@SdkInternalApi
final CreateMediaInsightsPipelineResult executeCreateMediaInsightsPipeline(CreateMediaInsightsPipelineRequest createMediaInsightsPipelineRequest) {
ExecutionContext executionContext = createExecutionContext(createMediaInsightsPipelineRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMediaInsightsPipelineRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createMediaInsightsPipelineRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMediaInsightsPipeline");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateMediaInsightsPipelineResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* A structure that contains the static configurations for a media insights pipeline.
*
*
* @param createMediaInsightsPipelineConfigurationRequest
* @return Result of the CreateMediaInsightsPipelineConfiguration operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.CreateMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
@Override
public CreateMediaInsightsPipelineConfigurationResult createMediaInsightsPipelineConfiguration(CreateMediaInsightsPipelineConfigurationRequest request) {
request = beforeClientExecution(request);
return executeCreateMediaInsightsPipelineConfiguration(request);
}
@SdkInternalApi
final CreateMediaInsightsPipelineConfigurationResult executeCreateMediaInsightsPipelineConfiguration(
CreateMediaInsightsPipelineConfigurationRequest createMediaInsightsPipelineConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(createMediaInsightsPipelineConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMediaInsightsPipelineConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createMediaInsightsPipelineConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMediaInsightsPipelineConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateMediaInsightsPipelineConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a media live connector pipeline in an Amazon Chime SDK meeting.
*
*
* @param createMediaLiveConnectorPipelineRequest
* @return Result of the CreateMediaLiveConnectorPipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.CreateMediaLiveConnectorPipeline
* @see AWS API Documentation
*/
@Override
public CreateMediaLiveConnectorPipelineResult createMediaLiveConnectorPipeline(CreateMediaLiveConnectorPipelineRequest request) {
request = beforeClientExecution(request);
return executeCreateMediaLiveConnectorPipeline(request);
}
@SdkInternalApi
final CreateMediaLiveConnectorPipelineResult executeCreateMediaLiveConnectorPipeline(
CreateMediaLiveConnectorPipelineRequest createMediaLiveConnectorPipelineRequest) {
ExecutionContext executionContext = createExecutionContext(createMediaLiveConnectorPipelineRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMediaLiveConnectorPipelineRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createMediaLiveConnectorPipelineRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMediaLiveConnectorPipeline");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateMediaLiveConnectorPipelineResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon Kinesis Video Stream pool for use with media stream pipelines.
*
*
*
* If a meeting uses an opt-in Region as its MediaRegion, the KVS stream must be in that same Region. For example, if a meeting uses the
* af-south-1
Region, the KVS stream must also be in af-south-1
. However, if the meeting
* uses a Region that AWS turns on by default, the KVS stream can be in any available Region, including an opt-in
* Region. For example, if the meeting uses ca-central-1
, the KVS stream can be in
* eu-west-2
, us-east-1
, af-south-1
, or any other Region that the Amazon
* Chime SDK supports.
*
*
* To learn which AWS Region a meeting uses, call the GetMeeting
* API and use the MediaRegion parameter from the response.
*
*
* For more information about opt-in Regions, refer to Available Regions in the
* Amazon Chime SDK Developer Guide, and Specify which AWS Regions your account can use, in the AWS Account Management Reference Guide.
*
*
*
* @param createMediaPipelineKinesisVideoStreamPoolRequest
* @return Result of the CreateMediaPipelineKinesisVideoStreamPool operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.CreateMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
@Override
public CreateMediaPipelineKinesisVideoStreamPoolResult createMediaPipelineKinesisVideoStreamPool(CreateMediaPipelineKinesisVideoStreamPoolRequest request) {
request = beforeClientExecution(request);
return executeCreateMediaPipelineKinesisVideoStreamPool(request);
}
@SdkInternalApi
final CreateMediaPipelineKinesisVideoStreamPoolResult executeCreateMediaPipelineKinesisVideoStreamPool(
CreateMediaPipelineKinesisVideoStreamPoolRequest createMediaPipelineKinesisVideoStreamPoolRequest) {
ExecutionContext executionContext = createExecutionContext(createMediaPipelineKinesisVideoStreamPoolRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMediaPipelineKinesisVideoStreamPoolRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createMediaPipelineKinesisVideoStreamPoolRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMediaPipelineKinesisVideoStreamPool");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateMediaPipelineKinesisVideoStreamPoolResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a streaming media pipeline.
*
*
* @param createMediaStreamPipelineRequest
* @return Result of the CreateMediaStreamPipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.CreateMediaStreamPipeline
* @see AWS API Documentation
*/
@Override
public CreateMediaStreamPipelineResult createMediaStreamPipeline(CreateMediaStreamPipelineRequest request) {
request = beforeClientExecution(request);
return executeCreateMediaStreamPipeline(request);
}
@SdkInternalApi
final CreateMediaStreamPipelineResult executeCreateMediaStreamPipeline(CreateMediaStreamPipelineRequest createMediaStreamPipelineRequest) {
ExecutionContext executionContext = createExecutionContext(createMediaStreamPipelineRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMediaStreamPipelineRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createMediaStreamPipelineRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMediaStreamPipeline");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateMediaStreamPipelineResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the media pipeline.
*
*
* @param deleteMediaCapturePipelineRequest
* @return Result of the DeleteMediaCapturePipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.DeleteMediaCapturePipeline
* @see AWS API Documentation
*/
@Override
public DeleteMediaCapturePipelineResult deleteMediaCapturePipeline(DeleteMediaCapturePipelineRequest request) {
request = beforeClientExecution(request);
return executeDeleteMediaCapturePipeline(request);
}
@SdkInternalApi
final DeleteMediaCapturePipelineResult executeDeleteMediaCapturePipeline(DeleteMediaCapturePipelineRequest deleteMediaCapturePipelineRequest) {
ExecutionContext executionContext = createExecutionContext(deleteMediaCapturePipelineRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteMediaCapturePipelineRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteMediaCapturePipelineRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteMediaCapturePipeline");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteMediaCapturePipelineResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified configuration settings.
*
*
* @param deleteMediaInsightsPipelineConfigurationRequest
* @return Result of the DeleteMediaInsightsPipelineConfiguration operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.DeleteMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteMediaInsightsPipelineConfigurationResult deleteMediaInsightsPipelineConfiguration(DeleteMediaInsightsPipelineConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteMediaInsightsPipelineConfiguration(request);
}
@SdkInternalApi
final DeleteMediaInsightsPipelineConfigurationResult executeDeleteMediaInsightsPipelineConfiguration(
DeleteMediaInsightsPipelineConfigurationRequest deleteMediaInsightsPipelineConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteMediaInsightsPipelineConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteMediaInsightsPipelineConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteMediaInsightsPipelineConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteMediaInsightsPipelineConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteMediaInsightsPipelineConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the media pipeline.
*
*
* @param deleteMediaPipelineRequest
* @return Result of the DeleteMediaPipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.DeleteMediaPipeline
* @see AWS API Documentation
*/
@Override
public DeleteMediaPipelineResult deleteMediaPipeline(DeleteMediaPipelineRequest request) {
request = beforeClientExecution(request);
return executeDeleteMediaPipeline(request);
}
@SdkInternalApi
final DeleteMediaPipelineResult executeDeleteMediaPipeline(DeleteMediaPipelineRequest deleteMediaPipelineRequest) {
ExecutionContext executionContext = createExecutionContext(deleteMediaPipelineRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteMediaPipelineRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteMediaPipelineRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteMediaPipeline");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteMediaPipelineResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Amazon Kinesis Video Stream pool.
*
*
* @param deleteMediaPipelineKinesisVideoStreamPoolRequest
* @return Result of the DeleteMediaPipelineKinesisVideoStreamPool operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.DeleteMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
@Override
public DeleteMediaPipelineKinesisVideoStreamPoolResult deleteMediaPipelineKinesisVideoStreamPool(DeleteMediaPipelineKinesisVideoStreamPoolRequest request) {
request = beforeClientExecution(request);
return executeDeleteMediaPipelineKinesisVideoStreamPool(request);
}
@SdkInternalApi
final DeleteMediaPipelineKinesisVideoStreamPoolResult executeDeleteMediaPipelineKinesisVideoStreamPool(
DeleteMediaPipelineKinesisVideoStreamPoolRequest deleteMediaPipelineKinesisVideoStreamPoolRequest) {
ExecutionContext executionContext = createExecutionContext(deleteMediaPipelineKinesisVideoStreamPoolRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteMediaPipelineKinesisVideoStreamPoolRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteMediaPipelineKinesisVideoStreamPoolRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteMediaPipelineKinesisVideoStreamPool");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteMediaPipelineKinesisVideoStreamPoolResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets an existing media pipeline.
*
*
* @param getMediaCapturePipelineRequest
* @return Result of the GetMediaCapturePipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.GetMediaCapturePipeline
* @see AWS API Documentation
*/
@Override
public GetMediaCapturePipelineResult getMediaCapturePipeline(GetMediaCapturePipelineRequest request) {
request = beforeClientExecution(request);
return executeGetMediaCapturePipeline(request);
}
@SdkInternalApi
final GetMediaCapturePipelineResult executeGetMediaCapturePipeline(GetMediaCapturePipelineRequest getMediaCapturePipelineRequest) {
ExecutionContext executionContext = createExecutionContext(getMediaCapturePipelineRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMediaCapturePipelineRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getMediaCapturePipelineRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMediaCapturePipeline");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetMediaCapturePipelineResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the configuration settings for a media insights pipeline.
*
*
* @param getMediaInsightsPipelineConfigurationRequest
* @return Result of the GetMediaInsightsPipelineConfiguration operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.GetMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
@Override
public GetMediaInsightsPipelineConfigurationResult getMediaInsightsPipelineConfiguration(GetMediaInsightsPipelineConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetMediaInsightsPipelineConfiguration(request);
}
@SdkInternalApi
final GetMediaInsightsPipelineConfigurationResult executeGetMediaInsightsPipelineConfiguration(
GetMediaInsightsPipelineConfigurationRequest getMediaInsightsPipelineConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getMediaInsightsPipelineConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMediaInsightsPipelineConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getMediaInsightsPipelineConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMediaInsightsPipelineConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetMediaInsightsPipelineConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets an existing media pipeline.
*
*
* @param getMediaPipelineRequest
* @return Result of the GetMediaPipeline operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.GetMediaPipeline
* @see AWS API Documentation
*/
@Override
public GetMediaPipelineResult getMediaPipeline(GetMediaPipelineRequest request) {
request = beforeClientExecution(request);
return executeGetMediaPipeline(request);
}
@SdkInternalApi
final GetMediaPipelineResult executeGetMediaPipeline(GetMediaPipelineRequest getMediaPipelineRequest) {
ExecutionContext executionContext = createExecutionContext(getMediaPipelineRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMediaPipelineRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getMediaPipelineRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMediaPipeline");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetMediaPipelineResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets an Kinesis video stream pool.
*
*
* @param getMediaPipelineKinesisVideoStreamPoolRequest
* @return Result of the GetMediaPipelineKinesisVideoStreamPool operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.GetMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
@Override
public GetMediaPipelineKinesisVideoStreamPoolResult getMediaPipelineKinesisVideoStreamPool(GetMediaPipelineKinesisVideoStreamPoolRequest request) {
request = beforeClientExecution(request);
return executeGetMediaPipelineKinesisVideoStreamPool(request);
}
@SdkInternalApi
final GetMediaPipelineKinesisVideoStreamPoolResult executeGetMediaPipelineKinesisVideoStreamPool(
GetMediaPipelineKinesisVideoStreamPoolRequest getMediaPipelineKinesisVideoStreamPoolRequest) {
ExecutionContext executionContext = createExecutionContext(getMediaPipelineKinesisVideoStreamPoolRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMediaPipelineKinesisVideoStreamPoolRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getMediaPipelineKinesisVideoStreamPoolRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMediaPipelineKinesisVideoStreamPool");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetMediaPipelineKinesisVideoStreamPoolResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the details of the specified speaker search task.
*
*
* @param getSpeakerSearchTaskRequest
* @return Result of the GetSpeakerSearchTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.GetSpeakerSearchTask
* @see AWS API Documentation
*/
@Override
public GetSpeakerSearchTaskResult getSpeakerSearchTask(GetSpeakerSearchTaskRequest request) {
request = beforeClientExecution(request);
return executeGetSpeakerSearchTask(request);
}
@SdkInternalApi
final GetSpeakerSearchTaskResult executeGetSpeakerSearchTask(GetSpeakerSearchTaskRequest getSpeakerSearchTaskRequest) {
ExecutionContext executionContext = createExecutionContext(getSpeakerSearchTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSpeakerSearchTaskRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSpeakerSearchTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSpeakerSearchTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetSpeakerSearchTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the details of a voice tone analysis task.
*
*
* @param getVoiceToneAnalysisTaskRequest
* @return Result of the GetVoiceToneAnalysisTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.GetVoiceToneAnalysisTask
* @see AWS API Documentation
*/
@Override
public GetVoiceToneAnalysisTaskResult getVoiceToneAnalysisTask(GetVoiceToneAnalysisTaskRequest request) {
request = beforeClientExecution(request);
return executeGetVoiceToneAnalysisTask(request);
}
@SdkInternalApi
final GetVoiceToneAnalysisTaskResult executeGetVoiceToneAnalysisTask(GetVoiceToneAnalysisTaskRequest getVoiceToneAnalysisTaskRequest) {
ExecutionContext executionContext = createExecutionContext(getVoiceToneAnalysisTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetVoiceToneAnalysisTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getVoiceToneAnalysisTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetVoiceToneAnalysisTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetVoiceToneAnalysisTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of media pipelines.
*
*
* @param listMediaCapturePipelinesRequest
* @return Result of the ListMediaCapturePipelines operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.ListMediaCapturePipelines
* @see AWS API Documentation
*/
@Override
public ListMediaCapturePipelinesResult listMediaCapturePipelines(ListMediaCapturePipelinesRequest request) {
request = beforeClientExecution(request);
return executeListMediaCapturePipelines(request);
}
@SdkInternalApi
final ListMediaCapturePipelinesResult executeListMediaCapturePipelines(ListMediaCapturePipelinesRequest listMediaCapturePipelinesRequest) {
ExecutionContext executionContext = createExecutionContext(listMediaCapturePipelinesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListMediaCapturePipelinesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listMediaCapturePipelinesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListMediaCapturePipelines");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListMediaCapturePipelinesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the available media insights pipeline configurations.
*
*
* @param listMediaInsightsPipelineConfigurationsRequest
* @return Result of the ListMediaInsightsPipelineConfigurations operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.ListMediaInsightsPipelineConfigurations
* @see AWS API Documentation
*/
@Override
public ListMediaInsightsPipelineConfigurationsResult listMediaInsightsPipelineConfigurations(ListMediaInsightsPipelineConfigurationsRequest request) {
request = beforeClientExecution(request);
return executeListMediaInsightsPipelineConfigurations(request);
}
@SdkInternalApi
final ListMediaInsightsPipelineConfigurationsResult executeListMediaInsightsPipelineConfigurations(
ListMediaInsightsPipelineConfigurationsRequest listMediaInsightsPipelineConfigurationsRequest) {
ExecutionContext executionContext = createExecutionContext(listMediaInsightsPipelineConfigurationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListMediaInsightsPipelineConfigurationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listMediaInsightsPipelineConfigurationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListMediaInsightsPipelineConfigurations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListMediaInsightsPipelineConfigurationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the video stream pools in the media pipeline.
*
*
* @param listMediaPipelineKinesisVideoStreamPoolsRequest
* @return Result of the ListMediaPipelineKinesisVideoStreamPools operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.ListMediaPipelineKinesisVideoStreamPools
* @see AWS API Documentation
*/
@Override
public ListMediaPipelineKinesisVideoStreamPoolsResult listMediaPipelineKinesisVideoStreamPools(ListMediaPipelineKinesisVideoStreamPoolsRequest request) {
request = beforeClientExecution(request);
return executeListMediaPipelineKinesisVideoStreamPools(request);
}
@SdkInternalApi
final ListMediaPipelineKinesisVideoStreamPoolsResult executeListMediaPipelineKinesisVideoStreamPools(
ListMediaPipelineKinesisVideoStreamPoolsRequest listMediaPipelineKinesisVideoStreamPoolsRequest) {
ExecutionContext executionContext = createExecutionContext(listMediaPipelineKinesisVideoStreamPoolsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListMediaPipelineKinesisVideoStreamPoolsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listMediaPipelineKinesisVideoStreamPoolsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListMediaPipelineKinesisVideoStreamPools");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListMediaPipelineKinesisVideoStreamPoolsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of media pipelines.
*
*
* @param listMediaPipelinesRequest
* @return Result of the ListMediaPipelines operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.ListMediaPipelines
* @see AWS API Documentation
*/
@Override
public ListMediaPipelinesResult listMediaPipelines(ListMediaPipelinesRequest request) {
request = beforeClientExecution(request);
return executeListMediaPipelines(request);
}
@SdkInternalApi
final ListMediaPipelinesResult executeListMediaPipelines(ListMediaPipelinesRequest listMediaPipelinesRequest) {
ExecutionContext executionContext = createExecutionContext(listMediaPipelinesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListMediaPipelinesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listMediaPipelinesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListMediaPipelines");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListMediaPipelinesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the tags available for a media pipeline.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts a speaker search task.
*
*
*
* Before starting any speaker search tasks, you must provide all notices and obtain all consents from the speaker
* as required under applicable privacy and biometrics laws, and as required under the AWS service terms for the Amazon Chime SDK.
*
*
*
* @param startSpeakerSearchTaskRequest
* @return Result of the StartSpeakerSearchTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.StartSpeakerSearchTask
* @see AWS API Documentation
*/
@Override
public StartSpeakerSearchTaskResult startSpeakerSearchTask(StartSpeakerSearchTaskRequest request) {
request = beforeClientExecution(request);
return executeStartSpeakerSearchTask(request);
}
@SdkInternalApi
final StartSpeakerSearchTaskResult executeStartSpeakerSearchTask(StartSpeakerSearchTaskRequest startSpeakerSearchTaskRequest) {
ExecutionContext executionContext = createExecutionContext(startSpeakerSearchTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartSpeakerSearchTaskRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startSpeakerSearchTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartSpeakerSearchTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StartSpeakerSearchTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts a voice tone analysis task. For more information about voice tone analysis, see Using Amazon Chime SDK voice
* analytics in the Amazon Chime SDK Developer Guide.
*
*
*
* Before starting any voice tone analysis tasks, you must provide all notices and obtain all consents from the
* speaker as required under applicable privacy and biometrics laws, and as required under the AWS service terms for the Amazon Chime SDK.
*
*
*
* @param startVoiceToneAnalysisTaskRequest
* @return Result of the StartVoiceToneAnalysisTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.StartVoiceToneAnalysisTask
* @see AWS API Documentation
*/
@Override
public StartVoiceToneAnalysisTaskResult startVoiceToneAnalysisTask(StartVoiceToneAnalysisTaskRequest request) {
request = beforeClientExecution(request);
return executeStartVoiceToneAnalysisTask(request);
}
@SdkInternalApi
final StartVoiceToneAnalysisTaskResult executeStartVoiceToneAnalysisTask(StartVoiceToneAnalysisTaskRequest startVoiceToneAnalysisTaskRequest) {
ExecutionContext executionContext = createExecutionContext(startVoiceToneAnalysisTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartVoiceToneAnalysisTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(startVoiceToneAnalysisTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartVoiceToneAnalysisTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StartVoiceToneAnalysisTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops a speaker search task.
*
*
* @param stopSpeakerSearchTaskRequest
* @return Result of the StopSpeakerSearchTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.StopSpeakerSearchTask
* @see AWS API Documentation
*/
@Override
public StopSpeakerSearchTaskResult stopSpeakerSearchTask(StopSpeakerSearchTaskRequest request) {
request = beforeClientExecution(request);
return executeStopSpeakerSearchTask(request);
}
@SdkInternalApi
final StopSpeakerSearchTaskResult executeStopSpeakerSearchTask(StopSpeakerSearchTaskRequest stopSpeakerSearchTaskRequest) {
ExecutionContext executionContext = createExecutionContext(stopSpeakerSearchTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopSpeakerSearchTaskRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(stopSpeakerSearchTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopSpeakerSearchTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StopSpeakerSearchTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops a voice tone analysis task.
*
*
* @param stopVoiceToneAnalysisTaskRequest
* @return Result of the StopVoiceToneAnalysisTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.StopVoiceToneAnalysisTask
* @see AWS API Documentation
*/
@Override
public StopVoiceToneAnalysisTaskResult stopVoiceToneAnalysisTask(StopVoiceToneAnalysisTaskRequest request) {
request = beforeClientExecution(request);
return executeStopVoiceToneAnalysisTask(request);
}
@SdkInternalApi
final StopVoiceToneAnalysisTaskResult executeStopVoiceToneAnalysisTask(StopVoiceToneAnalysisTaskRequest stopVoiceToneAnalysisTaskRequest) {
ExecutionContext executionContext = createExecutionContext(stopVoiceToneAnalysisTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopVoiceToneAnalysisTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(stopVoiceToneAnalysisTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopVoiceToneAnalysisTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StopVoiceToneAnalysisTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* The ARN of the media pipeline that you want to tag. Consists of the pipeline's endpoint region, resource ID, and
* pipeline ID.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.TagResource
* @see AWS API Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes any tags from a media pipeline.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.UntagResource
* @see AWS API Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the media insights pipeline's configuration settings.
*
*
* @param updateMediaInsightsPipelineConfigurationRequest
* @return Result of the UpdateMediaInsightsPipelineConfiguration operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.UpdateMediaInsightsPipelineConfiguration
* @see AWS API Documentation
*/
@Override
public UpdateMediaInsightsPipelineConfigurationResult updateMediaInsightsPipelineConfiguration(UpdateMediaInsightsPipelineConfigurationRequest request) {
request = beforeClientExecution(request);
return executeUpdateMediaInsightsPipelineConfiguration(request);
}
@SdkInternalApi
final UpdateMediaInsightsPipelineConfigurationResult executeUpdateMediaInsightsPipelineConfiguration(
UpdateMediaInsightsPipelineConfigurationRequest updateMediaInsightsPipelineConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(updateMediaInsightsPipelineConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateMediaInsightsPipelineConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateMediaInsightsPipelineConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateMediaInsightsPipelineConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateMediaInsightsPipelineConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the status of a media insights pipeline.
*
*
* @param updateMediaInsightsPipelineStatusRequest
* @return Result of the UpdateMediaInsightsPipelineStatus operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.UpdateMediaInsightsPipelineStatus
* @see AWS API Documentation
*/
@Override
public UpdateMediaInsightsPipelineStatusResult updateMediaInsightsPipelineStatus(UpdateMediaInsightsPipelineStatusRequest request) {
request = beforeClientExecution(request);
return executeUpdateMediaInsightsPipelineStatus(request);
}
@SdkInternalApi
final UpdateMediaInsightsPipelineStatusResult executeUpdateMediaInsightsPipelineStatus(
UpdateMediaInsightsPipelineStatusRequest updateMediaInsightsPipelineStatusRequest) {
ExecutionContext executionContext = createExecutionContext(updateMediaInsightsPipelineStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateMediaInsightsPipelineStatusRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateMediaInsightsPipelineStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateMediaInsightsPipelineStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateMediaInsightsPipelineStatusResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates an Amazon Kinesis Video Stream pool in a media pipeline.
*
*
* @param updateMediaPipelineKinesisVideoStreamPoolRequest
* @return Result of the UpdateMediaPipelineKinesisVideoStreamPool operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedClientException
* The client is not currently authorized to make the request.
* @throws ThrottledClientException
* The client exceeded its request rate limit.
* @throws ConflictException
* The request could not be processed because of conflict in the current state of the resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMediaPipelines.UpdateMediaPipelineKinesisVideoStreamPool
* @see AWS API Documentation
*/
@Override
public UpdateMediaPipelineKinesisVideoStreamPoolResult updateMediaPipelineKinesisVideoStreamPool(UpdateMediaPipelineKinesisVideoStreamPoolRequest request) {
request = beforeClientExecution(request);
return executeUpdateMediaPipelineKinesisVideoStreamPool(request);
}
@SdkInternalApi
final UpdateMediaPipelineKinesisVideoStreamPoolResult executeUpdateMediaPipelineKinesisVideoStreamPool(
UpdateMediaPipelineKinesisVideoStreamPoolRequest updateMediaPipelineKinesisVideoStreamPoolRequest) {
ExecutionContext executionContext = createExecutionContext(updateMediaPipelineKinesisVideoStreamPoolRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateMediaPipelineKinesisVideoStreamPoolRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateMediaPipelineKinesisVideoStreamPoolRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Media Pipelines");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateMediaPipelineKinesisVideoStreamPool");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateMediaPipelineKinesisVideoStreamPoolResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}