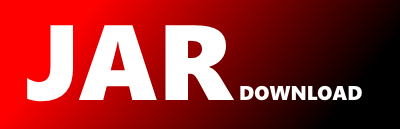
com.amazonaws.services.chimesdkmeetings.AmazonChimeSDKMeetingsAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chimesdkmeetings Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chimesdkmeetings;
import javax.annotation.Generated;
import com.amazonaws.services.chimesdkmeetings.model.*;
/**
* Interface for accessing Amazon Chime SDK Meetings asynchronously. Each asynchronous method will return a Java Future
* object representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.chimesdkmeetings.AbstractAmazonChimeSDKMeetingsAsync} instead.
*
*
*
* The Amazon Chime SDK meetings APIs in this section allow software developers to create Amazon Chime SDK meetings, set
* the Amazon Web Services Regions for meetings, create and manage users, and send and receive meeting notifications.
* For more information about the meeting APIs, see Amazon
* Chime SDK meetings.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonChimeSDKMeetingsAsync extends AmazonChimeSDKMeetings {
/**
*
* Creates up to 100 attendees for an active Amazon Chime SDK meeting. For more information about the Amazon Chime
* SDK, see Using the Amazon Chime SDK
* in the Amazon Chime Developer Guide.
*
*
* @param batchCreateAttendeeRequest
* @return A Java Future containing the result of the BatchCreateAttendee operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsync.BatchCreateAttendee
* @see AWS API Documentation
*/
java.util.concurrent.Future batchCreateAttendeeAsync(BatchCreateAttendeeRequest batchCreateAttendeeRequest);
/**
*
* Creates up to 100 attendees for an active Amazon Chime SDK meeting. For more information about the Amazon Chime
* SDK, see Using the Amazon Chime SDK
* in the Amazon Chime Developer Guide.
*
*
* @param batchCreateAttendeeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchCreateAttendee operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.BatchCreateAttendee
* @see AWS API Documentation
*/
java.util.concurrent.Future batchCreateAttendeeAsync(BatchCreateAttendeeRequest batchCreateAttendeeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates AttendeeCapabilities
except the capabilities listed in an ExcludedAttendeeIds
* table.
*
*
*
* You use the capabilities with a set of values that control what the capabilities can do, such as
* SendReceive
data. For more information about those values, see .
*
*
*
* When using capabilities, be aware of these corner cases:
*
*
* -
*
* If you specify MeetingFeatures:Video:MaxResolution:None
when you create a meeting, all API requests
* that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Video
will be rejected with ValidationError 400
.
*
*
* -
*
* If you specify MeetingFeatures:Content:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Content
will be rejected with ValidationError 400
.
*
*
* -
*
* You can't set content
capabilities to SendReceive
or Receive
unless you
* also set video
capabilities to SendReceive
or Receive
. If you don't set
* the video
capability to receive, the response will contain an HTTP 400 Bad Request status code.
* However, you can set your video
capability to receive and you set your content
* capability to not receive.
*
*
* -
*
* When you change an audio
capability from None
or Receive
to
* Send
or SendReceive
, and if the attendee left their microphone unmuted, audio will
* flow from the attendee to the other meeting participants.
*
*
* -
*
* When you change a video
or content
capability from None
or
* Receive
to Send
or SendReceive
, and if the attendee turned on their video
* or content streams, remote attendees can receive those streams, but only after media renegotiation between the
* client and the Amazon Chime back-end server.
*
*
*
*
* @param batchUpdateAttendeeCapabilitiesExceptRequest
* @return A Java Future containing the result of the BatchUpdateAttendeeCapabilitiesExcept operation returned by
* the service.
* @sample AmazonChimeSDKMeetingsAsync.BatchUpdateAttendeeCapabilitiesExcept
* @see AWS API Documentation
*/
java.util.concurrent.Future batchUpdateAttendeeCapabilitiesExceptAsync(
BatchUpdateAttendeeCapabilitiesExceptRequest batchUpdateAttendeeCapabilitiesExceptRequest);
/**
*
* Updates AttendeeCapabilities
except the capabilities listed in an ExcludedAttendeeIds
* table.
*
*
*
* You use the capabilities with a set of values that control what the capabilities can do, such as
* SendReceive
data. For more information about those values, see .
*
*
*
* When using capabilities, be aware of these corner cases:
*
*
* -
*
* If you specify MeetingFeatures:Video:MaxResolution:None
when you create a meeting, all API requests
* that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Video
will be rejected with ValidationError 400
.
*
*
* -
*
* If you specify MeetingFeatures:Content:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Content
will be rejected with ValidationError 400
.
*
*
* -
*
* You can't set content
capabilities to SendReceive
or Receive
unless you
* also set video
capabilities to SendReceive
or Receive
. If you don't set
* the video
capability to receive, the response will contain an HTTP 400 Bad Request status code.
* However, you can set your video
capability to receive and you set your content
* capability to not receive.
*
*
* -
*
* When you change an audio
capability from None
or Receive
to
* Send
or SendReceive
, and if the attendee left their microphone unmuted, audio will
* flow from the attendee to the other meeting participants.
*
*
* -
*
* When you change a video
or content
capability from None
or
* Receive
to Send
or SendReceive
, and if the attendee turned on their video
* or content streams, remote attendees can receive those streams, but only after media renegotiation between the
* client and the Amazon Chime back-end server.
*
*
*
*
* @param batchUpdateAttendeeCapabilitiesExceptRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the BatchUpdateAttendeeCapabilitiesExcept operation returned by
* the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.BatchUpdateAttendeeCapabilitiesExcept
* @see AWS API Documentation
*/
java.util.concurrent.Future batchUpdateAttendeeCapabilitiesExceptAsync(
BatchUpdateAttendeeCapabilitiesExceptRequest batchUpdateAttendeeCapabilitiesExceptRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new attendee for an active Amazon Chime SDK meeting. For more information about the Amazon Chime SDK,
* see Using the Amazon Chime SDK in the
* Amazon Chime Developer Guide.
*
*
* @param createAttendeeRequest
* @return A Java Future containing the result of the CreateAttendee operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsync.CreateAttendee
* @see AWS API Documentation
*/
java.util.concurrent.Future createAttendeeAsync(CreateAttendeeRequest createAttendeeRequest);
/**
*
* Creates a new attendee for an active Amazon Chime SDK meeting. For more information about the Amazon Chime SDK,
* see Using the Amazon Chime SDK in the
* Amazon Chime Developer Guide.
*
*
* @param createAttendeeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateAttendee operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.CreateAttendee
* @see AWS API Documentation
*/
java.util.concurrent.Future createAttendeeAsync(CreateAttendeeRequest createAttendeeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Amazon Chime SDK meeting in the specified media Region with no initial attendees. For more
* information about specifying media Regions, see Amazon Chime SDK Media
* Regions in the Amazon Chime Developer Guide. For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime Developer Guide.
*
*
* @param createMeetingRequest
* @return A Java Future containing the result of the CreateMeeting operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsync.CreateMeeting
* @see AWS API Documentation
*/
java.util.concurrent.Future createMeetingAsync(CreateMeetingRequest createMeetingRequest);
/**
*
* Creates a new Amazon Chime SDK meeting in the specified media Region with no initial attendees. For more
* information about specifying media Regions, see Amazon Chime SDK Media
* Regions in the Amazon Chime Developer Guide. For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime Developer Guide.
*
*
* @param createMeetingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMeeting operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.CreateMeeting
* @see AWS API Documentation
*/
java.util.concurrent.Future createMeetingAsync(CreateMeetingRequest createMeetingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a new Amazon Chime SDK meeting in the specified media Region, with attendees. For more information about
* specifying media Regions, see Amazon Chime SDK Media
* Regions in the Amazon Chime Developer Guide. For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime Developer Guide.
*
*
* @param createMeetingWithAttendeesRequest
* @return A Java Future containing the result of the CreateMeetingWithAttendees operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsync.CreateMeetingWithAttendees
* @see AWS API Documentation
*/
java.util.concurrent.Future createMeetingWithAttendeesAsync(
CreateMeetingWithAttendeesRequest createMeetingWithAttendeesRequest);
/**
*
* Creates a new Amazon Chime SDK meeting in the specified media Region, with attendees. For more information about
* specifying media Regions, see Amazon Chime SDK Media
* Regions in the Amazon Chime Developer Guide. For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime Developer Guide.
*
*
* @param createMeetingWithAttendeesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateMeetingWithAttendees operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.CreateMeetingWithAttendees
* @see AWS API Documentation
*/
java.util.concurrent.Future createMeetingWithAttendeesAsync(
CreateMeetingWithAttendeesRequest createMeetingWithAttendeesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an attendee from the specified Amazon Chime SDK meeting and deletes their JoinToken
.
* Attendees are automatically deleted when a Amazon Chime SDK meeting is deleted. For more information about the
* Amazon Chime SDK, see Using the Amazon
* Chime SDK in the Amazon Chime Developer Guide.
*
*
* @param deleteAttendeeRequest
* @return A Java Future containing the result of the DeleteAttendee operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsync.DeleteAttendee
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAttendeeAsync(DeleteAttendeeRequest deleteAttendeeRequest);
/**
*
* Deletes an attendee from the specified Amazon Chime SDK meeting and deletes their JoinToken
.
* Attendees are automatically deleted when a Amazon Chime SDK meeting is deleted. For more information about the
* Amazon Chime SDK, see Using the Amazon
* Chime SDK in the Amazon Chime Developer Guide.
*
*
* @param deleteAttendeeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteAttendee operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.DeleteAttendee
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteAttendeeAsync(DeleteAttendeeRequest deleteAttendeeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified Amazon Chime SDK meeting. The operation deletes all attendees, disconnects all clients, and
* prevents new clients from joining the meeting. For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime Developer Guide.
*
*
* @param deleteMeetingRequest
* @return A Java Future containing the result of the DeleteMeeting operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsync.DeleteMeeting
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMeetingAsync(DeleteMeetingRequest deleteMeetingRequest);
/**
*
* Deletes the specified Amazon Chime SDK meeting. The operation deletes all attendees, disconnects all clients, and
* prevents new clients from joining the meeting. For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime Developer Guide.
*
*
* @param deleteMeetingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteMeeting operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.DeleteMeeting
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteMeetingAsync(DeleteMeetingRequest deleteMeetingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the Amazon Chime SDK attendee details for a specified meeting ID and attendee ID. For more information about
* the Amazon Chime SDK, see Using the
* Amazon Chime SDK in the Amazon Chime Developer Guide.
*
*
* @param getAttendeeRequest
* @return A Java Future containing the result of the GetAttendee operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsync.GetAttendee
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAttendeeAsync(GetAttendeeRequest getAttendeeRequest);
/**
*
* Gets the Amazon Chime SDK attendee details for a specified meeting ID and attendee ID. For more information about
* the Amazon Chime SDK, see Using the
* Amazon Chime SDK in the Amazon Chime Developer Guide.
*
*
* @param getAttendeeRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetAttendee operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.GetAttendee
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getAttendeeAsync(GetAttendeeRequest getAttendeeRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets the Amazon Chime SDK meeting details for the specified meeting ID. For more information about the Amazon
* Chime SDK, see Using the Amazon Chime
* SDK in the Amazon Chime Developer Guide.
*
*
* @param getMeetingRequest
* @return A Java Future containing the result of the GetMeeting operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsync.GetMeeting
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMeetingAsync(GetMeetingRequest getMeetingRequest);
/**
*
* Gets the Amazon Chime SDK meeting details for the specified meeting ID. For more information about the Amazon
* Chime SDK, see Using the Amazon Chime
* SDK in the Amazon Chime Developer Guide.
*
*
* @param getMeetingRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetMeeting operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.GetMeeting
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getMeetingAsync(GetMeetingRequest getMeetingRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists the attendees for the specified Amazon Chime SDK meeting. For more information about the Amazon Chime SDK,
* see Using the Amazon Chime SDK in the
* Amazon Chime Developer Guide.
*
*
* @param listAttendeesRequest
* @return A Java Future containing the result of the ListAttendees operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsync.ListAttendees
* @see AWS API Documentation
*/
java.util.concurrent.Future listAttendeesAsync(ListAttendeesRequest listAttendeesRequest);
/**
*
* Lists the attendees for the specified Amazon Chime SDK meeting. For more information about the Amazon Chime SDK,
* see Using the Amazon Chime SDK in the
* Amazon Chime Developer Guide.
*
*
* @param listAttendeesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListAttendees operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.ListAttendees
* @see AWS API Documentation
*/
java.util.concurrent.Future listAttendeesAsync(ListAttendeesRequest listAttendeesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a list of the tags available for the specified resource.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsync.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Returns a list of the tags available for the specified resource.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.ListTagsForResource
* @see AWS API Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Starts transcription for the specified meetingId
. For more information, refer to Using Amazon Chime SDK live
* transcription in the Amazon Chime SDK Developer Guide.
*
*
* If you specify an invalid configuration, a TranscriptFailed
event will be sent with the contents of
* the BadRequestException
generated by Amazon Transcribe. For more information on each parameter and
* which combinations are valid, refer to the StartStreamTranscription API in the Amazon Transcribe Developer Guide.
*
*
*
* By default, Amazon Transcribe may use and store audio content processed by the service to develop and improve
* Amazon Web Services AI/ML services as further described in section 50 of the Amazon Web Services Service Terms. Using Amazon Transcribe may
* be subject to federal and state laws or regulations regarding the recording or interception of electronic
* communications. It is your and your end users’ responsibility to comply with all applicable laws regarding the
* recording, including properly notifying all participants in a recorded session or communication that the session
* or communication is being recorded, and obtaining all necessary consents. You can opt out from Amazon Web
* Services using audio content to develop and improve AWS AI/ML services by configuring an AI services opt out
* policy using Amazon Web Services Organizations.
*
*
*
* @param startMeetingTranscriptionRequest
* @return A Java Future containing the result of the StartMeetingTranscription operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsync.StartMeetingTranscription
* @see AWS API Documentation
*/
java.util.concurrent.Future startMeetingTranscriptionAsync(
StartMeetingTranscriptionRequest startMeetingTranscriptionRequest);
/**
*
* Starts transcription for the specified meetingId
. For more information, refer to Using Amazon Chime SDK live
* transcription in the Amazon Chime SDK Developer Guide.
*
*
* If you specify an invalid configuration, a TranscriptFailed
event will be sent with the contents of
* the BadRequestException
generated by Amazon Transcribe. For more information on each parameter and
* which combinations are valid, refer to the StartStreamTranscription API in the Amazon Transcribe Developer Guide.
*
*
*
* By default, Amazon Transcribe may use and store audio content processed by the service to develop and improve
* Amazon Web Services AI/ML services as further described in section 50 of the Amazon Web Services Service Terms. Using Amazon Transcribe may
* be subject to federal and state laws or regulations regarding the recording or interception of electronic
* communications. It is your and your end users’ responsibility to comply with all applicable laws regarding the
* recording, including properly notifying all participants in a recorded session or communication that the session
* or communication is being recorded, and obtaining all necessary consents. You can opt out from Amazon Web
* Services using audio content to develop and improve AWS AI/ML services by configuring an AI services opt out
* policy using Amazon Web Services Organizations.
*
*
*
* @param startMeetingTranscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StartMeetingTranscription operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.StartMeetingTranscription
* @see AWS API Documentation
*/
java.util.concurrent.Future startMeetingTranscriptionAsync(
StartMeetingTranscriptionRequest startMeetingTranscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops transcription for the specified meetingId
. For more information, refer to Using Amazon Chime SDK live
* transcription in the Amazon Chime SDK Developer Guide.
*
*
*
* By default, Amazon Transcribe may use and store audio content processed by the service to develop and improve
* Amazon Web Services AI/ML services as further described in section 50 of the Amazon Web Services Service Terms. Using Amazon Transcribe may
* be subject to federal and state laws or regulations regarding the recording or interception of electronic
* communications. It is your and your end users’ responsibility to comply with all applicable laws regarding the
* recording, including properly notifying all participants in a recorded session or communication that the session
* or communication is being recorded, and obtaining all necessary consents. You can opt out from Amazon Web
* Services using audio content to develop and improve Amazon Web Services AI/ML services by configuring an AI
* services opt out policy using Amazon Web Services Organizations.
*
*
*
* @param stopMeetingTranscriptionRequest
* @return A Java Future containing the result of the StopMeetingTranscription operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsync.StopMeetingTranscription
* @see AWS API Documentation
*/
java.util.concurrent.Future stopMeetingTranscriptionAsync(StopMeetingTranscriptionRequest stopMeetingTranscriptionRequest);
/**
*
* Stops transcription for the specified meetingId
. For more information, refer to Using Amazon Chime SDK live
* transcription in the Amazon Chime SDK Developer Guide.
*
*
*
* By default, Amazon Transcribe may use and store audio content processed by the service to develop and improve
* Amazon Web Services AI/ML services as further described in section 50 of the Amazon Web Services Service Terms. Using Amazon Transcribe may
* be subject to federal and state laws or regulations regarding the recording or interception of electronic
* communications. It is your and your end users’ responsibility to comply with all applicable laws regarding the
* recording, including properly notifying all participants in a recorded session or communication that the session
* or communication is being recorded, and obtaining all necessary consents. You can opt out from Amazon Web
* Services using audio content to develop and improve Amazon Web Services AI/ML services by configuring an AI
* services opt out policy using Amazon Web Services Organizations.
*
*
*
* @param stopMeetingTranscriptionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopMeetingTranscription operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.StopMeetingTranscription
* @see AWS API Documentation
*/
java.util.concurrent.Future stopMeetingTranscriptionAsync(StopMeetingTranscriptionRequest stopMeetingTranscriptionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The resource that supports tags.
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsync.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* The resource that supports tags.
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.TagResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes the specified tags from the specified resources. When you specify a tag key, the action removes both that
* key and its associated value. The operation succeeds even if you attempt to remove tags from a resource that were
* already removed. Note the following:
*
*
* -
*
* To remove tags from a resource, you need the necessary permissions for the service that the resource belongs to
* as well as permissions for removing tags. For more information, see the documentation for the service whose
* resource you want to untag.
*
*
* -
*
* You can only tag resources that are located in the specified Amazon Web Services Region for the calling Amazon
* Web Services account.
*
*
*
*
* Minimum permissions
*
*
* In addition to the tag:UntagResources
permission required by this operation, you must also have the
* remove tags permission defined by the service that created the resource. For example, to remove the tags from an
* Amazon EC2 instance using the UntagResources
operation, you must have both of the following
* permissions:
*
*
* tag:UntagResource
*
*
* ChimeSDKMeetings:DeleteTags
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsync.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes the specified tags from the specified resources. When you specify a tag key, the action removes both that
* key and its associated value. The operation succeeds even if you attempt to remove tags from a resource that were
* already removed. Note the following:
*
*
* -
*
* To remove tags from a resource, you need the necessary permissions for the service that the resource belongs to
* as well as permissions for removing tags. For more information, see the documentation for the service whose
* resource you want to untag.
*
*
* -
*
* You can only tag resources that are located in the specified Amazon Web Services Region for the calling Amazon
* Web Services account.
*
*
*
*
* Minimum permissions
*
*
* In addition to the tag:UntagResources
permission required by this operation, you must also have the
* remove tags permission defined by the service that created the resource. For example, to remove the tags from an
* Amazon EC2 instance using the UntagResources
operation, you must have both of the following
* permissions:
*
*
* tag:UntagResource
*
*
* ChimeSDKMeetings:DeleteTags
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.UntagResource
* @see AWS API Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* The capabilities that you want to update.
*
*
*
* You use the capabilities with a set of values that control what the capabilities can do, such as
* SendReceive
data. For more information about those values, see .
*
*
*
* When using capabilities, be aware of these corner cases:
*
*
* -
*
* If you specify MeetingFeatures:Video:MaxResolution:None
when you create a meeting, all API requests
* that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Video
will be rejected with ValidationError 400
.
*
*
* -
*
* If you specify MeetingFeatures:Content:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Content
will be rejected with ValidationError 400
.
*
*
* -
*
* You can't set content
capabilities to SendReceive
or Receive
unless you
* also set video
capabilities to SendReceive
or Receive
. If you don't set
* the video
capability to receive, the response will contain an HTTP 400 Bad Request status code.
* However, you can set your video
capability to receive and you set your content
* capability to not receive.
*
*
* -
*
* When you change an audio
capability from None
or Receive
to
* Send
or SendReceive
, and if the attendee left their microphone unmuted, audio will
* flow from the attendee to the other meeting participants.
*
*
* -
*
* When you change a video
or content
capability from None
or
* Receive
to Send
or SendReceive
, and if the attendee turned on their video
* or content streams, remote attendees can receive those streams, but only after media renegotiation between the
* client and the Amazon Chime back-end server.
*
*
*
*
* @param updateAttendeeCapabilitiesRequest
* @return A Java Future containing the result of the UpdateAttendeeCapabilities operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsync.UpdateAttendeeCapabilities
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAttendeeCapabilitiesAsync(
UpdateAttendeeCapabilitiesRequest updateAttendeeCapabilitiesRequest);
/**
*
* The capabilities that you want to update.
*
*
*
* You use the capabilities with a set of values that control what the capabilities can do, such as
* SendReceive
data. For more information about those values, see .
*
*
*
* When using capabilities, be aware of these corner cases:
*
*
* -
*
* If you specify MeetingFeatures:Video:MaxResolution:None
when you create a meeting, all API requests
* that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Video
will be rejected with ValidationError 400
.
*
*
* -
*
* If you specify MeetingFeatures:Content:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Content
will be rejected with ValidationError 400
.
*
*
* -
*
* You can't set content
capabilities to SendReceive
or Receive
unless you
* also set video
capabilities to SendReceive
or Receive
. If you don't set
* the video
capability to receive, the response will contain an HTTP 400 Bad Request status code.
* However, you can set your video
capability to receive and you set your content
* capability to not receive.
*
*
* -
*
* When you change an audio
capability from None
or Receive
to
* Send
or SendReceive
, and if the attendee left their microphone unmuted, audio will
* flow from the attendee to the other meeting participants.
*
*
* -
*
* When you change a video
or content
capability from None
or
* Receive
to Send
or SendReceive
, and if the attendee turned on their video
* or content streams, remote attendees can receive those streams, but only after media renegotiation between the
* client and the Amazon Chime back-end server.
*
*
*
*
* @param updateAttendeeCapabilitiesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateAttendeeCapabilities operation returned by the service.
* @sample AmazonChimeSDKMeetingsAsyncHandler.UpdateAttendeeCapabilities
* @see AWS API Documentation
*/
java.util.concurrent.Future updateAttendeeCapabilitiesAsync(
UpdateAttendeeCapabilitiesRequest updateAttendeeCapabilitiesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}