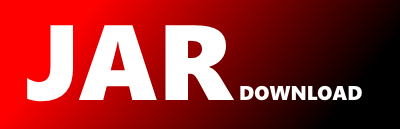
com.amazonaws.services.chimesdkmeetings.AmazonChimeSDKMeetingsClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chimesdkmeetings Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chimesdkmeetings;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.chimesdkmeetings.AmazonChimeSDKMeetingsClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.chimesdkmeetings.model.*;
import com.amazonaws.services.chimesdkmeetings.model.transform.*;
/**
* Client for accessing Amazon Chime SDK Meetings. All service calls made using this client are blocking, and will not
* return until the service call completes.
*
*
* The Amazon Chime SDK meetings APIs in this section allow software developers to create Amazon Chime SDK meetings, set
* the Amazon Web Services Regions for meetings, create and manage users, and send and receive meeting notifications.
* For more information about the meeting APIs, see Amazon
* Chime SDK meetings.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonChimeSDKMeetingsClient extends AmazonWebServiceClient implements AmazonChimeSDKMeetings {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonChimeSDKMeetings.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "chime";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmeetings.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("TooManyTagsException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmeetings.model.transform.TooManyTagsExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceNotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmeetings.model.transform.ResourceNotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnprocessableEntityException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmeetings.model.transform.UnprocessableEntityExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottlingException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmeetings.model.transform.ThrottlingExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmeetings.model.transform.NotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceUnavailableException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmeetings.model.transform.ServiceUnavailableExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnauthorizedException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmeetings.model.transform.UnauthorizedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ForbiddenException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmeetings.model.transform.ForbiddenExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BadRequestException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmeetings.model.transform.BadRequestExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("LimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmeetings.model.transform.LimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceFailureException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkmeetings.model.transform.ServiceFailureExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.chimesdkmeetings.model.AmazonChimeSDKMeetingsException.class));
public static AmazonChimeSDKMeetingsClientBuilder builder() {
return AmazonChimeSDKMeetingsClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon Chime SDK Meetings using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonChimeSDKMeetingsClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon Chime SDK Meetings using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonChimeSDKMeetingsClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("meetings-chime.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/chimesdkmeetings/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/chimesdkmeetings/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Creates up to 100 attendees for an active Amazon Chime SDK meeting. For more information about the Amazon Chime
* SDK, see Using the Amazon Chime SDK
* in the Amazon Chime Developer Guide.
*
*
* @param batchCreateAttendeeRequest
* @return Result of the BatchCreateAttendee operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws UnprocessableEntityException
* The request was well-formed but was unable to be followed due to semantic errors.
* @throws LimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @sample AmazonChimeSDKMeetings.BatchCreateAttendee
* @see AWS API Documentation
*/
@Override
public BatchCreateAttendeeResult batchCreateAttendee(BatchCreateAttendeeRequest request) {
request = beforeClientExecution(request);
return executeBatchCreateAttendee(request);
}
@SdkInternalApi
final BatchCreateAttendeeResult executeBatchCreateAttendee(BatchCreateAttendeeRequest batchCreateAttendeeRequest) {
ExecutionContext executionContext = createExecutionContext(batchCreateAttendeeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchCreateAttendeeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(batchCreateAttendeeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchCreateAttendee");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new BatchCreateAttendeeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates AttendeeCapabilities
except the capabilities listed in an ExcludedAttendeeIds
* table.
*
*
*
* You use the capabilities with a set of values that control what the capabilities can do, such as
* SendReceive
data. For more information about those values, see .
*
*
*
* When using capabilities, be aware of these corner cases:
*
*
* -
*
* If you specify MeetingFeatures:Video:MaxResolution:None
when you create a meeting, all API requests
* that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Video
will be rejected with ValidationError 400
.
*
*
* -
*
* If you specify MeetingFeatures:Content:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Content
will be rejected with ValidationError 400
.
*
*
* -
*
* You can't set content
capabilities to SendReceive
or Receive
unless you
* also set video
capabilities to SendReceive
or Receive
. If you don't set
* the video
capability to receive, the response will contain an HTTP 400 Bad Request status code.
* However, you can set your video
capability to receive and you set your content
* capability to not receive.
*
*
* -
*
* When you change an audio
capability from None
or Receive
to
* Send
or SendReceive
, and if the attendee left their microphone unmuted, audio will
* flow from the attendee to the other meeting participants.
*
*
* -
*
* When you change a video
or content
capability from None
or
* Receive
to Send
or SendReceive
, and if the attendee turned on their video
* or content streams, remote attendees can receive those streams, but only after media renegotiation between the
* client and the Amazon Chime back-end server.
*
*
*
*
* @param batchUpdateAttendeeCapabilitiesExceptRequest
* @return Result of the BatchUpdateAttendeeCapabilitiesExcept operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* Multiple instances of the same request have been made simultaneously.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @sample AmazonChimeSDKMeetings.BatchUpdateAttendeeCapabilitiesExcept
* @see AWS API Documentation
*/
@Override
public BatchUpdateAttendeeCapabilitiesExceptResult batchUpdateAttendeeCapabilitiesExcept(BatchUpdateAttendeeCapabilitiesExceptRequest request) {
request = beforeClientExecution(request);
return executeBatchUpdateAttendeeCapabilitiesExcept(request);
}
@SdkInternalApi
final BatchUpdateAttendeeCapabilitiesExceptResult executeBatchUpdateAttendeeCapabilitiesExcept(
BatchUpdateAttendeeCapabilitiesExceptRequest batchUpdateAttendeeCapabilitiesExceptRequest) {
ExecutionContext executionContext = createExecutionContext(batchUpdateAttendeeCapabilitiesExceptRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchUpdateAttendeeCapabilitiesExceptRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(batchUpdateAttendeeCapabilitiesExceptRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchUpdateAttendeeCapabilitiesExcept");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchUpdateAttendeeCapabilitiesExceptResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new attendee for an active Amazon Chime SDK meeting. For more information about the Amazon Chime SDK,
* see Using the Amazon Chime SDK in the
* Amazon Chime Developer Guide.
*
*
* @param createAttendeeRequest
* @return Result of the CreateAttendee operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws UnprocessableEntityException
* The request was well-formed but was unable to be followed due to semantic errors.
* @throws LimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @sample AmazonChimeSDKMeetings.CreateAttendee
* @see AWS API Documentation
*/
@Override
public CreateAttendeeResult createAttendee(CreateAttendeeRequest request) {
request = beforeClientExecution(request);
return executeCreateAttendee(request);
}
@SdkInternalApi
final CreateAttendeeResult executeCreateAttendee(CreateAttendeeRequest createAttendeeRequest) {
ExecutionContext executionContext = createExecutionContext(createAttendeeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateAttendeeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createAttendeeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateAttendee");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateAttendeeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new Amazon Chime SDK meeting in the specified media Region with no initial attendees. For more
* information about specifying media Regions, see Amazon Chime SDK Media
* Regions in the Amazon Chime Developer Guide. For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime Developer Guide.
*
*
* @param createMeetingRequest
* @return Result of the CreateMeeting operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* Multiple instances of the same request have been made simultaneously.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws LimitExceededException
* The request exceeds the resource limit.
* @sample AmazonChimeSDKMeetings.CreateMeeting
* @see AWS API Documentation
*/
@Override
public CreateMeetingResult createMeeting(CreateMeetingRequest request) {
request = beforeClientExecution(request);
return executeCreateMeeting(request);
}
@SdkInternalApi
final CreateMeetingResult executeCreateMeeting(CreateMeetingRequest createMeetingRequest) {
ExecutionContext executionContext = createExecutionContext(createMeetingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMeetingRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createMeetingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMeeting");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateMeetingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a new Amazon Chime SDK meeting in the specified media Region, with attendees. For more information about
* specifying media Regions, see Amazon Chime SDK Media
* Regions in the Amazon Chime Developer Guide. For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime Developer Guide.
*
*
* @param createMeetingWithAttendeesRequest
* @return Result of the CreateMeetingWithAttendees operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* Multiple instances of the same request have been made simultaneously.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws LimitExceededException
* The request exceeds the resource limit.
* @sample AmazonChimeSDKMeetings.CreateMeetingWithAttendees
* @see AWS API Documentation
*/
@Override
public CreateMeetingWithAttendeesResult createMeetingWithAttendees(CreateMeetingWithAttendeesRequest request) {
request = beforeClientExecution(request);
return executeCreateMeetingWithAttendees(request);
}
@SdkInternalApi
final CreateMeetingWithAttendeesResult executeCreateMeetingWithAttendees(CreateMeetingWithAttendeesRequest createMeetingWithAttendeesRequest) {
ExecutionContext executionContext = createExecutionContext(createMeetingWithAttendeesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateMeetingWithAttendeesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createMeetingWithAttendeesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateMeetingWithAttendees");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateMeetingWithAttendeesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an attendee from the specified Amazon Chime SDK meeting and deletes their JoinToken
.
* Attendees are automatically deleted when a Amazon Chime SDK meeting is deleted. For more information about the
* Amazon Chime SDK, see Using the Amazon
* Chime SDK in the Amazon Chime Developer Guide.
*
*
* @param deleteAttendeeRequest
* @return Result of the DeleteAttendee operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @sample AmazonChimeSDKMeetings.DeleteAttendee
* @see AWS API Documentation
*/
@Override
public DeleteAttendeeResult deleteAttendee(DeleteAttendeeRequest request) {
request = beforeClientExecution(request);
return executeDeleteAttendee(request);
}
@SdkInternalApi
final DeleteAttendeeResult executeDeleteAttendee(DeleteAttendeeRequest deleteAttendeeRequest) {
ExecutionContext executionContext = createExecutionContext(deleteAttendeeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteAttendeeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteAttendeeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteAttendee");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteAttendeeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified Amazon Chime SDK meeting. The operation deletes all attendees, disconnects all clients, and
* prevents new clients from joining the meeting. For more information about the Amazon Chime SDK, see Using the Amazon Chime SDK in the
* Amazon Chime Developer Guide.
*
*
* @param deleteMeetingRequest
* @return Result of the DeleteMeeting operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @sample AmazonChimeSDKMeetings.DeleteMeeting
* @see AWS API Documentation
*/
@Override
public DeleteMeetingResult deleteMeeting(DeleteMeetingRequest request) {
request = beforeClientExecution(request);
return executeDeleteMeeting(request);
}
@SdkInternalApi
final DeleteMeetingResult executeDeleteMeeting(DeleteMeetingRequest deleteMeetingRequest) {
ExecutionContext executionContext = createExecutionContext(deleteMeetingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteMeetingRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteMeetingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteMeeting");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteMeetingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the Amazon Chime SDK attendee details for a specified meeting ID and attendee ID. For more information about
* the Amazon Chime SDK, see Using the
* Amazon Chime SDK in the Amazon Chime Developer Guide.
*
*
* @param getAttendeeRequest
* @return Result of the GetAttendee operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @sample AmazonChimeSDKMeetings.GetAttendee
* @see AWS
* API Documentation
*/
@Override
public GetAttendeeResult getAttendee(GetAttendeeRequest request) {
request = beforeClientExecution(request);
return executeGetAttendee(request);
}
@SdkInternalApi
final GetAttendeeResult executeGetAttendee(GetAttendeeRequest getAttendeeRequest) {
ExecutionContext executionContext = createExecutionContext(getAttendeeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetAttendeeRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getAttendeeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetAttendee");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetAttendeeResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the Amazon Chime SDK meeting details for the specified meeting ID. For more information about the Amazon
* Chime SDK, see Using the Amazon Chime
* SDK in the Amazon Chime Developer Guide.
*
*
* @param getMeetingRequest
* @return Result of the GetMeeting operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @sample AmazonChimeSDKMeetings.GetMeeting
* @see AWS
* API Documentation
*/
@Override
public GetMeetingResult getMeeting(GetMeetingRequest request) {
request = beforeClientExecution(request);
return executeGetMeeting(request);
}
@SdkInternalApi
final GetMeetingResult executeGetMeeting(GetMeetingRequest getMeetingRequest) {
ExecutionContext executionContext = createExecutionContext(getMeetingRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetMeetingRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getMeetingRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetMeeting");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetMeetingResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the attendees for the specified Amazon Chime SDK meeting. For more information about the Amazon Chime SDK,
* see Using the Amazon Chime SDK in the
* Amazon Chime Developer Guide.
*
*
* @param listAttendeesRequest
* @return Result of the ListAttendees operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @sample AmazonChimeSDKMeetings.ListAttendees
* @see AWS API Documentation
*/
@Override
public ListAttendeesResult listAttendees(ListAttendeesRequest request) {
request = beforeClientExecution(request);
return executeListAttendees(request);
}
@SdkInternalApi
final ListAttendeesResult executeListAttendees(ListAttendeesRequest listAttendeesRequest) {
ExecutionContext executionContext = createExecutionContext(listAttendeesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAttendeesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listAttendeesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAttendees");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListAttendeesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of the tags available for the specified resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws LimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @throws ResourceNotFoundException
* The resource that you want to tag couldn't be found.
* @sample AmazonChimeSDKMeetings.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts transcription for the specified meetingId
. For more information, refer to Using Amazon Chime SDK live
* transcription in the Amazon Chime SDK Developer Guide.
*
*
* If you specify an invalid configuration, a TranscriptFailed
event will be sent with the contents of
* the BadRequestException
generated by Amazon Transcribe. For more information on each parameter and
* which combinations are valid, refer to the StartStreamTranscription API in the Amazon Transcribe Developer Guide.
*
*
*
* By default, Amazon Transcribe may use and store audio content processed by the service to develop and improve
* Amazon Web Services AI/ML services as further described in section 50 of the Amazon Web Services Service Terms. Using Amazon Transcribe may
* be subject to federal and state laws or regulations regarding the recording or interception of electronic
* communications. It is your and your end users’ responsibility to comply with all applicable laws regarding the
* recording, including properly notifying all participants in a recorded session or communication that the session
* or communication is being recorded, and obtaining all necessary consents. You can opt out from Amazon Web
* Services using audio content to develop and improve AWS AI/ML services by configuring an AI services opt out
* policy using Amazon Web Services Organizations.
*
*
*
* @param startMeetingTranscriptionRequest
* @return Result of the StartMeetingTranscription operation returned by the service.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws LimitExceededException
* The request exceeds the resource limit.
* @throws UnprocessableEntityException
* The request was well-formed but was unable to be followed due to semantic errors.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMeetings.StartMeetingTranscription
* @see AWS API Documentation
*/
@Override
public StartMeetingTranscriptionResult startMeetingTranscription(StartMeetingTranscriptionRequest request) {
request = beforeClientExecution(request);
return executeStartMeetingTranscription(request);
}
@SdkInternalApi
final StartMeetingTranscriptionResult executeStartMeetingTranscription(StartMeetingTranscriptionRequest startMeetingTranscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(startMeetingTranscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartMeetingTranscriptionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(startMeetingTranscriptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartMeetingTranscription");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StartMeetingTranscriptionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops transcription for the specified meetingId
. For more information, refer to Using Amazon Chime SDK live
* transcription in the Amazon Chime SDK Developer Guide.
*
*
*
* By default, Amazon Transcribe may use and store audio content processed by the service to develop and improve
* Amazon Web Services AI/ML services as further described in section 50 of the Amazon Web Services Service Terms. Using Amazon Transcribe may
* be subject to federal and state laws or regulations regarding the recording or interception of electronic
* communications. It is your and your end users’ responsibility to comply with all applicable laws regarding the
* recording, including properly notifying all participants in a recorded session or communication that the session
* or communication is being recorded, and obtaining all necessary consents. You can opt out from Amazon Web
* Services using audio content to develop and improve Amazon Web Services AI/ML services by configuring an AI
* services opt out policy using Amazon Web Services Organizations.
*
*
*
* @param stopMeetingTranscriptionRequest
* @return Result of the StopMeetingTranscription operation returned by the service.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws UnprocessableEntityException
* The request was well-formed but was unable to be followed due to semantic errors.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKMeetings.StopMeetingTranscription
* @see AWS API Documentation
*/
@Override
public StopMeetingTranscriptionResult stopMeetingTranscription(StopMeetingTranscriptionRequest request) {
request = beforeClientExecution(request);
return executeStopMeetingTranscription(request);
}
@SdkInternalApi
final StopMeetingTranscriptionResult executeStopMeetingTranscription(StopMeetingTranscriptionRequest stopMeetingTranscriptionRequest) {
ExecutionContext executionContext = createExecutionContext(stopMeetingTranscriptionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopMeetingTranscriptionRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(stopMeetingTranscriptionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopMeetingTranscription");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StopMeetingTranscriptionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* The resource that supports tags.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws LimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @throws ResourceNotFoundException
* The resource that you want to tag couldn't be found.
* @throws TooManyTagsException
* Too many tags were added to the specified resource.
* @sample AmazonChimeSDKMeetings.TagResource
* @see AWS
* API Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes the specified tags from the specified resources. When you specify a tag key, the action removes both that
* key and its associated value. The operation succeeds even if you attempt to remove tags from a resource that were
* already removed. Note the following:
*
*
* -
*
* To remove tags from a resource, you need the necessary permissions for the service that the resource belongs to
* as well as permissions for removing tags. For more information, see the documentation for the service whose
* resource you want to untag.
*
*
* -
*
* You can only tag resources that are located in the specified Amazon Web Services Region for the calling Amazon
* Web Services account.
*
*
*
*
* Minimum permissions
*
*
* In addition to the tag:UntagResources
permission required by this operation, you must also have the
* remove tags permission defined by the service that created the resource. For example, to remove the tags from an
* Amazon EC2 instance using the UntagResources
operation, you must have both of the following
* permissions:
*
*
* tag:UntagResource
*
*
* ChimeSDKMeetings:DeleteTags
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws LimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @throws ResourceNotFoundException
* The resource that you want to tag couldn't be found.
* @sample AmazonChimeSDKMeetings.UntagResource
* @see AWS API Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* The capabilities that you want to update.
*
*
*
* You use the capabilities with a set of values that control what the capabilities can do, such as
* SendReceive
data. For more information about those values, see .
*
*
*
* When using capabilities, be aware of these corner cases:
*
*
* -
*
* If you specify MeetingFeatures:Video:MaxResolution:None
when you create a meeting, all API requests
* that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Video
will be rejected with ValidationError 400
.
*
*
* -
*
* If you specify MeetingFeatures:Content:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Content
will be rejected with ValidationError 400
.
*
*
* -
*
* You can't set content
capabilities to SendReceive
or Receive
unless you
* also set video
capabilities to SendReceive
or Receive
. If you don't set
* the video
capability to receive, the response will contain an HTTP 400 Bad Request status code.
* However, you can set your video
capability to receive and you set your content
* capability to not receive.
*
*
* -
*
* When you change an audio
capability from None
or Receive
to
* Send
or SendReceive
, and if the attendee left their microphone unmuted, audio will
* flow from the attendee to the other meeting participants.
*
*
* -
*
* When you change a video
or content
capability from None
or
* Receive
to Send
or SendReceive
, and if the attendee turned on their video
* or content streams, remote attendees can receive those streams, but only after media renegotiation between the
* client and the Amazon Chime back-end server.
*
*
*
*
* @param updateAttendeeCapabilitiesRequest
* @return Result of the UpdateAttendeeCapabilities operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* Multiple instances of the same request have been made simultaneously.
* @throws UnauthorizedException
* The user isn't authorized to request a resource.
* @throws NotFoundException
* One or more of the resources in the request does not exist in the system.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ThrottlingException
* The number of customer requests exceeds the request rate limit.
* @sample AmazonChimeSDKMeetings.UpdateAttendeeCapabilities
* @see AWS API Documentation
*/
@Override
public UpdateAttendeeCapabilitiesResult updateAttendeeCapabilities(UpdateAttendeeCapabilitiesRequest request) {
request = beforeClientExecution(request);
return executeUpdateAttendeeCapabilities(request);
}
@SdkInternalApi
final UpdateAttendeeCapabilitiesResult executeUpdateAttendeeCapabilities(UpdateAttendeeCapabilitiesRequest updateAttendeeCapabilitiesRequest) {
ExecutionContext executionContext = createExecutionContext(updateAttendeeCapabilitiesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateAttendeeCapabilitiesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(updateAttendeeCapabilitiesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Meetings");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateAttendeeCapabilities");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new UpdateAttendeeCapabilitiesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
HttpResponseHandler errorResponseHandler = protocolFactory.createErrorResponseHandler(new JsonErrorResponseMetadata());
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@com.amazonaws.annotation.SdkInternalApi
static com.amazonaws.protocol.json.SdkJsonProtocolFactory getProtocolFactory() {
return protocolFactory;
}
@Override
public void shutdown() {
super.shutdown();
}
}