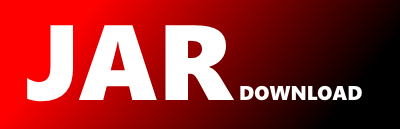
com.amazonaws.services.chimesdkmeetings.model.CreateAttendeeRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chimesdkmeetings Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chimesdkmeetings.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateAttendeeRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The unique ID of the meeting.
*
*/
private String meetingId;
/**
*
* The Amazon Chime SDK external user ID. An idempotency token. Links the attendee to an identity managed by a
* builder application.
*
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix.
*
*/
private String externalUserId;
/**
*
* The capabilities (audio
, video
, or content
) that you want to grant an
* attendee. If you don't specify capabilities, all users have send and receive capabilities on all media channels
* by default.
*
*
*
* You use the capabilities with a set of values that control what the capabilities can do, such as
* SendReceive
data. For more information about those values, see .
*
*
*
* When using capabilities, be aware of these corner cases:
*
*
* -
*
* If you specify MeetingFeatures:Video:MaxResolution:None
when you create a meeting, all API requests
* that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Video
will be rejected with ValidationError 400
.
*
*
* -
*
* If you specify MeetingFeatures:Content:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Content
will be rejected with ValidationError 400
.
*
*
* -
*
* You can't set content
capabilities to SendReceive
or Receive
unless you
* also set video
capabilities to SendReceive
or Receive
. If you don't set
* the video
capability to receive, the response will contain an HTTP 400 Bad Request status code.
* However, you can set your video
capability to receive and you set your content
* capability to not receive.
*
*
* -
*
* When you change an audio
capability from None
or Receive
to
* Send
or SendReceive
, and if the attendee left their microphone unmuted, audio will
* flow from the attendee to the other meeting participants.
*
*
* -
*
* When you change a video
or content
capability from None
or
* Receive
to Send
or SendReceive
, and if the attendee turned on their video
* or content streams, remote attendees can receive those streams, but only after media renegotiation between the
* client and the Amazon Chime back-end server.
*
*
*
*/
private AttendeeCapabilities capabilities;
/**
*
* The unique ID of the meeting.
*
*
* @param meetingId
* The unique ID of the meeting.
*/
public void setMeetingId(String meetingId) {
this.meetingId = meetingId;
}
/**
*
* The unique ID of the meeting.
*
*
* @return The unique ID of the meeting.
*/
public String getMeetingId() {
return this.meetingId;
}
/**
*
* The unique ID of the meeting.
*
*
* @param meetingId
* The unique ID of the meeting.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAttendeeRequest withMeetingId(String meetingId) {
setMeetingId(meetingId);
return this;
}
/**
*
* The Amazon Chime SDK external user ID. An idempotency token. Links the attendee to an identity managed by a
* builder application.
*
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix.
*
*
* @param externalUserId
* The Amazon Chime SDK external user ID. An idempotency token. Links the attendee to an identity managed by
* a builder application.
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix.
*/
public void setExternalUserId(String externalUserId) {
this.externalUserId = externalUserId;
}
/**
*
* The Amazon Chime SDK external user ID. An idempotency token. Links the attendee to an identity managed by a
* builder application.
*
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix.
*
*
* @return The Amazon Chime SDK external user ID. An idempotency token. Links the attendee to an identity managed by
* a builder application.
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix.
*/
public String getExternalUserId() {
return this.externalUserId;
}
/**
*
* The Amazon Chime SDK external user ID. An idempotency token. Links the attendee to an identity managed by a
* builder application.
*
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix.
*
*
* @param externalUserId
* The Amazon Chime SDK external user ID. An idempotency token. Links the attendee to an identity managed by
* a builder application.
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAttendeeRequest withExternalUserId(String externalUserId) {
setExternalUserId(externalUserId);
return this;
}
/**
*
* The capabilities (audio
, video
, or content
) that you want to grant an
* attendee. If you don't specify capabilities, all users have send and receive capabilities on all media channels
* by default.
*
*
*
* You use the capabilities with a set of values that control what the capabilities can do, such as
* SendReceive
data. For more information about those values, see .
*
*
*
* When using capabilities, be aware of these corner cases:
*
*
* -
*
* If you specify MeetingFeatures:Video:MaxResolution:None
when you create a meeting, all API requests
* that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Video
will be rejected with ValidationError 400
.
*
*
* -
*
* If you specify MeetingFeatures:Content:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Content
will be rejected with ValidationError 400
.
*
*
* -
*
* You can't set content
capabilities to SendReceive
or Receive
unless you
* also set video
capabilities to SendReceive
or Receive
. If you don't set
* the video
capability to receive, the response will contain an HTTP 400 Bad Request status code.
* However, you can set your video
capability to receive and you set your content
* capability to not receive.
*
*
* -
*
* When you change an audio
capability from None
or Receive
to
* Send
or SendReceive
, and if the attendee left their microphone unmuted, audio will
* flow from the attendee to the other meeting participants.
*
*
* -
*
* When you change a video
or content
capability from None
or
* Receive
to Send
or SendReceive
, and if the attendee turned on their video
* or content streams, remote attendees can receive those streams, but only after media renegotiation between the
* client and the Amazon Chime back-end server.
*
*
*
*
* @param capabilities
* The capabilities (audio
, video
, or content
) that you want to grant
* an attendee. If you don't specify capabilities, all users have send and receive capabilities on all media
* channels by default.
*
* You use the capabilities with a set of values that control what the capabilities can do, such as
* SendReceive
data. For more information about those values, see .
*
*
*
* When using capabilities, be aware of these corner cases:
*
*
* -
*
* If you specify MeetingFeatures:Video:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Video
will be rejected with ValidationError 400
.
*
*
* -
*
* If you specify MeetingFeatures:Content:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Content
will be rejected with ValidationError 400
.
*
*
* -
*
* You can't set content
capabilities to SendReceive
or Receive
unless
* you also set video
capabilities to SendReceive
or Receive
. If you
* don't set the video
capability to receive, the response will contain an HTTP 400 Bad Request
* status code. However, you can set your video
capability to receive and you set your
* content
capability to not receive.
*
*
* -
*
* When you change an audio
capability from None
or Receive
to
* Send
or SendReceive
, and if the attendee left their microphone unmuted, audio
* will flow from the attendee to the other meeting participants.
*
*
* -
*
* When you change a video
or content
capability from None
or
* Receive
to Send
or SendReceive
, and if the attendee turned on
* their video or content streams, remote attendees can receive those streams, but only after media
* renegotiation between the client and the Amazon Chime back-end server.
*
*
*/
public void setCapabilities(AttendeeCapabilities capabilities) {
this.capabilities = capabilities;
}
/**
*
* The capabilities (audio
, video
, or content
) that you want to grant an
* attendee. If you don't specify capabilities, all users have send and receive capabilities on all media channels
* by default.
*
*
*
* You use the capabilities with a set of values that control what the capabilities can do, such as
* SendReceive
data. For more information about those values, see .
*
*
*
* When using capabilities, be aware of these corner cases:
*
*
* -
*
* If you specify MeetingFeatures:Video:MaxResolution:None
when you create a meeting, all API requests
* that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Video
will be rejected with ValidationError 400
.
*
*
* -
*
* If you specify MeetingFeatures:Content:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Content
will be rejected with ValidationError 400
.
*
*
* -
*
* You can't set content
capabilities to SendReceive
or Receive
unless you
* also set video
capabilities to SendReceive
or Receive
. If you don't set
* the video
capability to receive, the response will contain an HTTP 400 Bad Request status code.
* However, you can set your video
capability to receive and you set your content
* capability to not receive.
*
*
* -
*
* When you change an audio
capability from None
or Receive
to
* Send
or SendReceive
, and if the attendee left their microphone unmuted, audio will
* flow from the attendee to the other meeting participants.
*
*
* -
*
* When you change a video
or content
capability from None
or
* Receive
to Send
or SendReceive
, and if the attendee turned on their video
* or content streams, remote attendees can receive those streams, but only after media renegotiation between the
* client and the Amazon Chime back-end server.
*
*
*
*
* @return The capabilities (audio
, video
, or content
) that you want to grant
* an attendee. If you don't specify capabilities, all users have send and receive capabilities on all media
* channels by default.
*
* You use the capabilities with a set of values that control what the capabilities can do, such as
* SendReceive
data. For more information about those values, see .
*
*
*
* When using capabilities, be aware of these corner cases:
*
*
* -
*
* If you specify MeetingFeatures:Video:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Video
will be rejected with ValidationError 400
.
*
*
* -
*
* If you specify MeetingFeatures:Content:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Content
will be rejected with ValidationError 400
.
*
*
* -
*
* You can't set content
capabilities to SendReceive
or Receive
* unless you also set video
capabilities to SendReceive
or Receive
.
* If you don't set the video
capability to receive, the response will contain an HTTP 400 Bad
* Request status code. However, you can set your video
capability to receive and you set your
* content
capability to not receive.
*
*
* -
*
* When you change an audio
capability from None
or Receive
to
* Send
or SendReceive
, and if the attendee left their microphone unmuted, audio
* will flow from the attendee to the other meeting participants.
*
*
* -
*
* When you change a video
or content
capability from None
or
* Receive
to Send
or SendReceive
, and if the attendee turned on
* their video or content streams, remote attendees can receive those streams, but only after media
* renegotiation between the client and the Amazon Chime back-end server.
*
*
*/
public AttendeeCapabilities getCapabilities() {
return this.capabilities;
}
/**
*
* The capabilities (audio
, video
, or content
) that you want to grant an
* attendee. If you don't specify capabilities, all users have send and receive capabilities on all media channels
* by default.
*
*
*
* You use the capabilities with a set of values that control what the capabilities can do, such as
* SendReceive
data. For more information about those values, see .
*
*
*
* When using capabilities, be aware of these corner cases:
*
*
* -
*
* If you specify MeetingFeatures:Video:MaxResolution:None
when you create a meeting, all API requests
* that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Video
will be rejected with ValidationError 400
.
*
*
* -
*
* If you specify MeetingFeatures:Content:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Content
will be rejected with ValidationError 400
.
*
*
* -
*
* You can't set content
capabilities to SendReceive
or Receive
unless you
* also set video
capabilities to SendReceive
or Receive
. If you don't set
* the video
capability to receive, the response will contain an HTTP 400 Bad Request status code.
* However, you can set your video
capability to receive and you set your content
* capability to not receive.
*
*
* -
*
* When you change an audio
capability from None
or Receive
to
* Send
or SendReceive
, and if the attendee left their microphone unmuted, audio will
* flow from the attendee to the other meeting participants.
*
*
* -
*
* When you change a video
or content
capability from None
or
* Receive
to Send
or SendReceive
, and if the attendee turned on their video
* or content streams, remote attendees can receive those streams, but only after media renegotiation between the
* client and the Amazon Chime back-end server.
*
*
*
*
* @param capabilities
* The capabilities (audio
, video
, or content
) that you want to grant
* an attendee. If you don't specify capabilities, all users have send and receive capabilities on all media
* channels by default.
*
* You use the capabilities with a set of values that control what the capabilities can do, such as
* SendReceive
data. For more information about those values, see .
*
*
*
* When using capabilities, be aware of these corner cases:
*
*
* -
*
* If you specify MeetingFeatures:Video:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Video
will be rejected with ValidationError 400
.
*
*
* -
*
* If you specify MeetingFeatures:Content:MaxResolution:None
when you create a meeting, all API
* requests that include SendReceive
, Send
, or Receive
for
* AttendeeCapabilities:Content
will be rejected with ValidationError 400
.
*
*
* -
*
* You can't set content
capabilities to SendReceive
or Receive
unless
* you also set video
capabilities to SendReceive
or Receive
. If you
* don't set the video
capability to receive, the response will contain an HTTP 400 Bad Request
* status code. However, you can set your video
capability to receive and you set your
* content
capability to not receive.
*
*
* -
*
* When you change an audio
capability from None
or Receive
to
* Send
or SendReceive
, and if the attendee left their microphone unmuted, audio
* will flow from the attendee to the other meeting participants.
*
*
* -
*
* When you change a video
or content
capability from None
or
* Receive
to Send
or SendReceive
, and if the attendee turned on
* their video or content streams, remote attendees can receive those streams, but only after media
* renegotiation between the client and the Amazon Chime back-end server.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateAttendeeRequest withCapabilities(AttendeeCapabilities capabilities) {
setCapabilities(capabilities);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getMeetingId() != null)
sb.append("MeetingId: ").append(getMeetingId()).append(",");
if (getExternalUserId() != null)
sb.append("ExternalUserId: ").append("***Sensitive Data Redacted***").append(",");
if (getCapabilities() != null)
sb.append("Capabilities: ").append(getCapabilities());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateAttendeeRequest == false)
return false;
CreateAttendeeRequest other = (CreateAttendeeRequest) obj;
if (other.getMeetingId() == null ^ this.getMeetingId() == null)
return false;
if (other.getMeetingId() != null && other.getMeetingId().equals(this.getMeetingId()) == false)
return false;
if (other.getExternalUserId() == null ^ this.getExternalUserId() == null)
return false;
if (other.getExternalUserId() != null && other.getExternalUserId().equals(this.getExternalUserId()) == false)
return false;
if (other.getCapabilities() == null ^ this.getCapabilities() == null)
return false;
if (other.getCapabilities() != null && other.getCapabilities().equals(this.getCapabilities()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getMeetingId() == null) ? 0 : getMeetingId().hashCode());
hashCode = prime * hashCode + ((getExternalUserId() == null) ? 0 : getExternalUserId().hashCode());
hashCode = prime * hashCode + ((getCapabilities() == null) ? 0 : getCapabilities().hashCode());
return hashCode;
}
@Override
public CreateAttendeeRequest clone() {
return (CreateAttendeeRequest) super.clone();
}
}