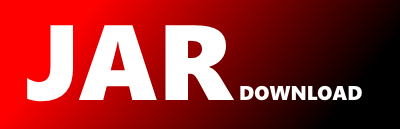
com.amazonaws.services.chimesdkmeetings.model.CreateMeetingRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chimesdkmeetings Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chimesdkmeetings.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateMeetingRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The unique identifier for the client request. Use a different token for different meetings.
*
*/
private String clientRequestToken;
/**
*
* The Region in which to create the meeting.
*
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
, ca-central-1
,
* eu-central-1
, eu-north-1
, eu-south-1
, eu-west-1
,
* eu-west-2
, eu-west-3
, sa-east-1
, us-east-1
,
* us-east-2
, us-west-1
, us-west-2
.
*
*
* Available values in Amazon Web Services GovCloud (US) Regions: us-gov-east-1
,
* us-gov-west-1
.
*
*/
private String mediaRegion;
/**
*
* Reserved.
*
*/
private String meetingHostId;
/**
*
* The external meeting ID.
*
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix. Case
* insensitive.
*
*/
private String externalMeetingId;
/**
*
* The configuration for resource targets to receive notifications when meeting and attendee events occur.
*
*/
private NotificationsConfiguration notificationsConfiguration;
/**
*
* Lists the audio and video features enabled for a meeting, such as echo reduction.
*
*/
private MeetingFeaturesConfiguration meetingFeatures;
/**
*
* When specified, replicates the media from the primary meeting to the new meeting.
*
*/
private String primaryMeetingId;
/**
*
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their users.
*
*/
private java.util.List tenantIds;
/**
*
* Applies one or more tags to an Amazon Chime SDK meeting. Note the following:
*
*
* -
*
* Not all resources have tags. For a list of services with resources that support tagging using this operation, see
* Services
* that support the Resource Groups Tagging API. If the resource doesn't yet support this operation, the
* resource's service might support tagging using its own API operations. For more information, refer to the
* documentation for that service.
*
*
* -
*
* Each resource can have up to 50 tags. For other limits, see Tag Naming and Usage
* Conventions in the AWS General Reference.
*
*
* -
*
* You can only tag resources that are located in the specified Amazon Web Services Region for the Amazon Web
* Services account.
*
*
* -
*
* To add tags to a resource, you need the necessary permissions for the service that the resource belongs to as
* well as permissions for adding tags. For more information, see the documentation for each service.
*
*
*
*
*
* Do not store personally identifiable information (PII) or other confidential or sensitive information in tags. We
* use tags to provide you with billing and administration services. Tags are not intended to be used for private or
* sensitive data.
*
*
*
* Minimum permissions
*
*
* In addition to the tag:TagResources
permission required by this operation, you must also have the
* tagging permission defined by the service that created the resource. For example, to tag a
* ChimeSDKMeetings
instance using the TagResources
operation, you must have both of the
* following permissions:
*
*
* tag:TagResources
*
*
* ChimeSDKMeetings:CreateTags
*
*
*
* Some services might have specific requirements for tagging some resources. For example, to tag an Amazon S3
* bucket, you must also have the s3:GetBucketTagging
permission. If the expected minimum permissions
* don't work, check the documentation for that service's tagging APIs for more information.
*
*
*/
private java.util.List tags;
/**
*
* The unique identifier for the client request. Use a different token for different meetings.
*
*
* @param clientRequestToken
* The unique identifier for the client request. Use a different token for different meetings.
*/
public void setClientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
}
/**
*
* The unique identifier for the client request. Use a different token for different meetings.
*
*
* @return The unique identifier for the client request. Use a different token for different meetings.
*/
public String getClientRequestToken() {
return this.clientRequestToken;
}
/**
*
* The unique identifier for the client request. Use a different token for different meetings.
*
*
* @param clientRequestToken
* The unique identifier for the client request. Use a different token for different meetings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingRequest withClientRequestToken(String clientRequestToken) {
setClientRequestToken(clientRequestToken);
return this;
}
/**
*
* The Region in which to create the meeting.
*
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
, ca-central-1
,
* eu-central-1
, eu-north-1
, eu-south-1
, eu-west-1
,
* eu-west-2
, eu-west-3
, sa-east-1
, us-east-1
,
* us-east-2
, us-west-1
, us-west-2
.
*
*
* Available values in Amazon Web Services GovCloud (US) Regions: us-gov-east-1
,
* us-gov-west-1
.
*
*
* @param mediaRegion
* The Region in which to create the meeting.
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
,
* ca-central-1
, eu-central-1
, eu-north-1
, eu-south-1
,
* eu-west-1
, eu-west-2
, eu-west-3
, sa-east-1
,
* us-east-1
, us-east-2
, us-west-1
, us-west-2
.
*
*
* Available values in Amazon Web Services GovCloud (US) Regions: us-gov-east-1
,
* us-gov-west-1
.
*/
public void setMediaRegion(String mediaRegion) {
this.mediaRegion = mediaRegion;
}
/**
*
* The Region in which to create the meeting.
*
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
, ca-central-1
,
* eu-central-1
, eu-north-1
, eu-south-1
, eu-west-1
,
* eu-west-2
, eu-west-3
, sa-east-1
, us-east-1
,
* us-east-2
, us-west-1
, us-west-2
.
*
*
* Available values in Amazon Web Services GovCloud (US) Regions: us-gov-east-1
,
* us-gov-west-1
.
*
*
* @return The Region in which to create the meeting.
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
,
* ca-central-1
, eu-central-1
, eu-north-1
, eu-south-1
,
* eu-west-1
, eu-west-2
, eu-west-3
, sa-east-1
,
* us-east-1
, us-east-2
, us-west-1
, us-west-2
.
*
*
* Available values in Amazon Web Services GovCloud (US) Regions: us-gov-east-1
,
* us-gov-west-1
.
*/
public String getMediaRegion() {
return this.mediaRegion;
}
/**
*
* The Region in which to create the meeting.
*
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
, ca-central-1
,
* eu-central-1
, eu-north-1
, eu-south-1
, eu-west-1
,
* eu-west-2
, eu-west-3
, sa-east-1
, us-east-1
,
* us-east-2
, us-west-1
, us-west-2
.
*
*
* Available values in Amazon Web Services GovCloud (US) Regions: us-gov-east-1
,
* us-gov-west-1
.
*
*
* @param mediaRegion
* The Region in which to create the meeting.
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
,
* ca-central-1
, eu-central-1
, eu-north-1
, eu-south-1
,
* eu-west-1
, eu-west-2
, eu-west-3
, sa-east-1
,
* us-east-1
, us-east-2
, us-west-1
, us-west-2
.
*
*
* Available values in Amazon Web Services GovCloud (US) Regions: us-gov-east-1
,
* us-gov-west-1
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingRequest withMediaRegion(String mediaRegion) {
setMediaRegion(mediaRegion);
return this;
}
/**
*
* Reserved.
*
*
* @param meetingHostId
* Reserved.
*/
public void setMeetingHostId(String meetingHostId) {
this.meetingHostId = meetingHostId;
}
/**
*
* Reserved.
*
*
* @return Reserved.
*/
public String getMeetingHostId() {
return this.meetingHostId;
}
/**
*
* Reserved.
*
*
* @param meetingHostId
* Reserved.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingRequest withMeetingHostId(String meetingHostId) {
setMeetingHostId(meetingHostId);
return this;
}
/**
*
* The external meeting ID.
*
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix. Case
* insensitive.
*
*
* @param externalMeetingId
* The external meeting ID.
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix.
* Case insensitive.
*/
public void setExternalMeetingId(String externalMeetingId) {
this.externalMeetingId = externalMeetingId;
}
/**
*
* The external meeting ID.
*
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix. Case
* insensitive.
*
*
* @return The external meeting ID.
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix.
* Case insensitive.
*/
public String getExternalMeetingId() {
return this.externalMeetingId;
}
/**
*
* The external meeting ID.
*
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix. Case
* insensitive.
*
*
* @param externalMeetingId
* The external meeting ID.
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix.
* Case insensitive.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingRequest withExternalMeetingId(String externalMeetingId) {
setExternalMeetingId(externalMeetingId);
return this;
}
/**
*
* The configuration for resource targets to receive notifications when meeting and attendee events occur.
*
*
* @param notificationsConfiguration
* The configuration for resource targets to receive notifications when meeting and attendee events occur.
*/
public void setNotificationsConfiguration(NotificationsConfiguration notificationsConfiguration) {
this.notificationsConfiguration = notificationsConfiguration;
}
/**
*
* The configuration for resource targets to receive notifications when meeting and attendee events occur.
*
*
* @return The configuration for resource targets to receive notifications when meeting and attendee events occur.
*/
public NotificationsConfiguration getNotificationsConfiguration() {
return this.notificationsConfiguration;
}
/**
*
* The configuration for resource targets to receive notifications when meeting and attendee events occur.
*
*
* @param notificationsConfiguration
* The configuration for resource targets to receive notifications when meeting and attendee events occur.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingRequest withNotificationsConfiguration(NotificationsConfiguration notificationsConfiguration) {
setNotificationsConfiguration(notificationsConfiguration);
return this;
}
/**
*
* Lists the audio and video features enabled for a meeting, such as echo reduction.
*
*
* @param meetingFeatures
* Lists the audio and video features enabled for a meeting, such as echo reduction.
*/
public void setMeetingFeatures(MeetingFeaturesConfiguration meetingFeatures) {
this.meetingFeatures = meetingFeatures;
}
/**
*
* Lists the audio and video features enabled for a meeting, such as echo reduction.
*
*
* @return Lists the audio and video features enabled for a meeting, such as echo reduction.
*/
public MeetingFeaturesConfiguration getMeetingFeatures() {
return this.meetingFeatures;
}
/**
*
* Lists the audio and video features enabled for a meeting, such as echo reduction.
*
*
* @param meetingFeatures
* Lists the audio and video features enabled for a meeting, such as echo reduction.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingRequest withMeetingFeatures(MeetingFeaturesConfiguration meetingFeatures) {
setMeetingFeatures(meetingFeatures);
return this;
}
/**
*
* When specified, replicates the media from the primary meeting to the new meeting.
*
*
* @param primaryMeetingId
* When specified, replicates the media from the primary meeting to the new meeting.
*/
public void setPrimaryMeetingId(String primaryMeetingId) {
this.primaryMeetingId = primaryMeetingId;
}
/**
*
* When specified, replicates the media from the primary meeting to the new meeting.
*
*
* @return When specified, replicates the media from the primary meeting to the new meeting.
*/
public String getPrimaryMeetingId() {
return this.primaryMeetingId;
}
/**
*
* When specified, replicates the media from the primary meeting to the new meeting.
*
*
* @param primaryMeetingId
* When specified, replicates the media from the primary meeting to the new meeting.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingRequest withPrimaryMeetingId(String primaryMeetingId) {
setPrimaryMeetingId(primaryMeetingId);
return this;
}
/**
*
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their users.
*
*
* @return A consistent and opaque identifier, created and maintained by the builder to represent a segment of their
* users.
*/
public java.util.List getTenantIds() {
return tenantIds;
}
/**
*
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their users.
*
*
* @param tenantIds
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their
* users.
*/
public void setTenantIds(java.util.Collection tenantIds) {
if (tenantIds == null) {
this.tenantIds = null;
return;
}
this.tenantIds = new java.util.ArrayList(tenantIds);
}
/**
*
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their users.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTenantIds(java.util.Collection)} or {@link #withTenantIds(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param tenantIds
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their
* users.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingRequest withTenantIds(String... tenantIds) {
if (this.tenantIds == null) {
setTenantIds(new java.util.ArrayList(tenantIds.length));
}
for (String ele : tenantIds) {
this.tenantIds.add(ele);
}
return this;
}
/**
*
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their users.
*
*
* @param tenantIds
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their
* users.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingRequest withTenantIds(java.util.Collection tenantIds) {
setTenantIds(tenantIds);
return this;
}
/**
*
* Applies one or more tags to an Amazon Chime SDK meeting. Note the following:
*
*
* -
*
* Not all resources have tags. For a list of services with resources that support tagging using this operation, see
* Services
* that support the Resource Groups Tagging API. If the resource doesn't yet support this operation, the
* resource's service might support tagging using its own API operations. For more information, refer to the
* documentation for that service.
*
*
* -
*
* Each resource can have up to 50 tags. For other limits, see Tag Naming and Usage
* Conventions in the AWS General Reference.
*
*
* -
*
* You can only tag resources that are located in the specified Amazon Web Services Region for the Amazon Web
* Services account.
*
*
* -
*
* To add tags to a resource, you need the necessary permissions for the service that the resource belongs to as
* well as permissions for adding tags. For more information, see the documentation for each service.
*
*
*
*
*
* Do not store personally identifiable information (PII) or other confidential or sensitive information in tags. We
* use tags to provide you with billing and administration services. Tags are not intended to be used for private or
* sensitive data.
*
*
*
* Minimum permissions
*
*
* In addition to the tag:TagResources
permission required by this operation, you must also have the
* tagging permission defined by the service that created the resource. For example, to tag a
* ChimeSDKMeetings
instance using the TagResources
operation, you must have both of the
* following permissions:
*
*
* tag:TagResources
*
*
* ChimeSDKMeetings:CreateTags
*
*
*
* Some services might have specific requirements for tagging some resources. For example, to tag an Amazon S3
* bucket, you must also have the s3:GetBucketTagging
permission. If the expected minimum permissions
* don't work, check the documentation for that service's tagging APIs for more information.
*
*
*
* @return Applies one or more tags to an Amazon Chime SDK meeting. Note the following:
*
* -
*
* Not all resources have tags. For a list of services with resources that support tagging using this
* operation, see Services that support the Resource Groups Tagging API. If the resource doesn't yet support this
* operation, the resource's service might support tagging using its own API operations. For more
* information, refer to the documentation for that service.
*
*
* -
*
* Each resource can have up to 50 tags. For other limits, see Tag Naming and
* Usage Conventions in the AWS General Reference.
*
*
* -
*
* You can only tag resources that are located in the specified Amazon Web Services Region for the Amazon
* Web Services account.
*
*
* -
*
* To add tags to a resource, you need the necessary permissions for the service that the resource belongs
* to as well as permissions for adding tags. For more information, see the documentation for each service.
*
*
*
*
*
* Do not store personally identifiable information (PII) or other confidential or sensitive information in
* tags. We use tags to provide you with billing and administration services. Tags are not intended to be
* used for private or sensitive data.
*
*
*
* Minimum permissions
*
*
* In addition to the tag:TagResources
permission required by this operation, you must also
* have the tagging permission defined by the service that created the resource. For example, to tag a
* ChimeSDKMeetings
instance using the TagResources
operation, you must have both
* of the following permissions:
*
*
* tag:TagResources
*
*
* ChimeSDKMeetings:CreateTags
*
*
*
* Some services might have specific requirements for tagging some resources. For example, to tag an Amazon
* S3 bucket, you must also have the s3:GetBucketTagging
permission. If the expected minimum
* permissions don't work, check the documentation for that service's tagging APIs for more information.
*
*/
public java.util.List getTags() {
return tags;
}
/**
*
* Applies one or more tags to an Amazon Chime SDK meeting. Note the following:
*
*
* -
*
* Not all resources have tags. For a list of services with resources that support tagging using this operation, see
* Services
* that support the Resource Groups Tagging API. If the resource doesn't yet support this operation, the
* resource's service might support tagging using its own API operations. For more information, refer to the
* documentation for that service.
*
*
* -
*
* Each resource can have up to 50 tags. For other limits, see Tag Naming and Usage
* Conventions in the AWS General Reference.
*
*
* -
*
* You can only tag resources that are located in the specified Amazon Web Services Region for the Amazon Web
* Services account.
*
*
* -
*
* To add tags to a resource, you need the necessary permissions for the service that the resource belongs to as
* well as permissions for adding tags. For more information, see the documentation for each service.
*
*
*
*
*
* Do not store personally identifiable information (PII) or other confidential or sensitive information in tags. We
* use tags to provide you with billing and administration services. Tags are not intended to be used for private or
* sensitive data.
*
*
*
* Minimum permissions
*
*
* In addition to the tag:TagResources
permission required by this operation, you must also have the
* tagging permission defined by the service that created the resource. For example, to tag a
* ChimeSDKMeetings
instance using the TagResources
operation, you must have both of the
* following permissions:
*
*
* tag:TagResources
*
*
* ChimeSDKMeetings:CreateTags
*
*
*
* Some services might have specific requirements for tagging some resources. For example, to tag an Amazon S3
* bucket, you must also have the s3:GetBucketTagging
permission. If the expected minimum permissions
* don't work, check the documentation for that service's tagging APIs for more information.
*
*
*
* @param tags
* Applies one or more tags to an Amazon Chime SDK meeting. Note the following:
*
* -
*
* Not all resources have tags. For a list of services with resources that support tagging using this
* operation, see Services that support the Resource Groups Tagging API. If the resource doesn't yet support this
* operation, the resource's service might support tagging using its own API operations. For more
* information, refer to the documentation for that service.
*
*
* -
*
* Each resource can have up to 50 tags. For other limits, see Tag Naming and Usage
* Conventions in the AWS General Reference.
*
*
* -
*
* You can only tag resources that are located in the specified Amazon Web Services Region for the Amazon Web
* Services account.
*
*
* -
*
* To add tags to a resource, you need the necessary permissions for the service that the resource belongs to
* as well as permissions for adding tags. For more information, see the documentation for each service.
*
*
*
*
*
* Do not store personally identifiable information (PII) or other confidential or sensitive information in
* tags. We use tags to provide you with billing and administration services. Tags are not intended to be
* used for private or sensitive data.
*
*
*
* Minimum permissions
*
*
* In addition to the tag:TagResources
permission required by this operation, you must also have
* the tagging permission defined by the service that created the resource. For example, to tag a
* ChimeSDKMeetings
instance using the TagResources
operation, you must have both
* of the following permissions:
*
*
* tag:TagResources
*
*
* ChimeSDKMeetings:CreateTags
*
*
*
* Some services might have specific requirements for tagging some resources. For example, to tag an Amazon
* S3 bucket, you must also have the s3:GetBucketTagging
permission. If the expected minimum
* permissions don't work, check the documentation for that service's tagging APIs for more information.
*
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* Applies one or more tags to an Amazon Chime SDK meeting. Note the following:
*
*
* -
*
* Not all resources have tags. For a list of services with resources that support tagging using this operation, see
* Services
* that support the Resource Groups Tagging API. If the resource doesn't yet support this operation, the
* resource's service might support tagging using its own API operations. For more information, refer to the
* documentation for that service.
*
*
* -
*
* Each resource can have up to 50 tags. For other limits, see Tag Naming and Usage
* Conventions in the AWS General Reference.
*
*
* -
*
* You can only tag resources that are located in the specified Amazon Web Services Region for the Amazon Web
* Services account.
*
*
* -
*
* To add tags to a resource, you need the necessary permissions for the service that the resource belongs to as
* well as permissions for adding tags. For more information, see the documentation for each service.
*
*
*
*
*
* Do not store personally identifiable information (PII) or other confidential or sensitive information in tags. We
* use tags to provide you with billing and administration services. Tags are not intended to be used for private or
* sensitive data.
*
*
*
* Minimum permissions
*
*
* In addition to the tag:TagResources
permission required by this operation, you must also have the
* tagging permission defined by the service that created the resource. For example, to tag a
* ChimeSDKMeetings
instance using the TagResources
operation, you must have both of the
* following permissions:
*
*
* tag:TagResources
*
*
* ChimeSDKMeetings:CreateTags
*
*
*
* Some services might have specific requirements for tagging some resources. For example, to tag an Amazon S3
* bucket, you must also have the s3:GetBucketTagging
permission. If the expected minimum permissions
* don't work, check the documentation for that service's tagging APIs for more information.
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* Applies one or more tags to an Amazon Chime SDK meeting. Note the following:
*
* -
*
* Not all resources have tags. For a list of services with resources that support tagging using this
* operation, see Services that support the Resource Groups Tagging API. If the resource doesn't yet support this
* operation, the resource's service might support tagging using its own API operations. For more
* information, refer to the documentation for that service.
*
*
* -
*
* Each resource can have up to 50 tags. For other limits, see Tag Naming and Usage
* Conventions in the AWS General Reference.
*
*
* -
*
* You can only tag resources that are located in the specified Amazon Web Services Region for the Amazon Web
* Services account.
*
*
* -
*
* To add tags to a resource, you need the necessary permissions for the service that the resource belongs to
* as well as permissions for adding tags. For more information, see the documentation for each service.
*
*
*
*
*
* Do not store personally identifiable information (PII) or other confidential or sensitive information in
* tags. We use tags to provide you with billing and administration services. Tags are not intended to be
* used for private or sensitive data.
*
*
*
* Minimum permissions
*
*
* In addition to the tag:TagResources
permission required by this operation, you must also have
* the tagging permission defined by the service that created the resource. For example, to tag a
* ChimeSDKMeetings
instance using the TagResources
operation, you must have both
* of the following permissions:
*
*
* tag:TagResources
*
*
* ChimeSDKMeetings:CreateTags
*
*
*
* Some services might have specific requirements for tagging some resources. For example, to tag an Amazon
* S3 bucket, you must also have the s3:GetBucketTagging
permission. If the expected minimum
* permissions don't work, check the documentation for that service's tagging APIs for more information.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* Applies one or more tags to an Amazon Chime SDK meeting. Note the following:
*
*
* -
*
* Not all resources have tags. For a list of services with resources that support tagging using this operation, see
* Services
* that support the Resource Groups Tagging API. If the resource doesn't yet support this operation, the
* resource's service might support tagging using its own API operations. For more information, refer to the
* documentation for that service.
*
*
* -
*
* Each resource can have up to 50 tags. For other limits, see Tag Naming and Usage
* Conventions in the AWS General Reference.
*
*
* -
*
* You can only tag resources that are located in the specified Amazon Web Services Region for the Amazon Web
* Services account.
*
*
* -
*
* To add tags to a resource, you need the necessary permissions for the service that the resource belongs to as
* well as permissions for adding tags. For more information, see the documentation for each service.
*
*
*
*
*
* Do not store personally identifiable information (PII) or other confidential or sensitive information in tags. We
* use tags to provide you with billing and administration services. Tags are not intended to be used for private or
* sensitive data.
*
*
*
* Minimum permissions
*
*
* In addition to the tag:TagResources
permission required by this operation, you must also have the
* tagging permission defined by the service that created the resource. For example, to tag a
* ChimeSDKMeetings
instance using the TagResources
operation, you must have both of the
* following permissions:
*
*
* tag:TagResources
*
*
* ChimeSDKMeetings:CreateTags
*
*
*
* Some services might have specific requirements for tagging some resources. For example, to tag an Amazon S3
* bucket, you must also have the s3:GetBucketTagging
permission. If the expected minimum permissions
* don't work, check the documentation for that service's tagging APIs for more information.
*
*
*
* @param tags
* Applies one or more tags to an Amazon Chime SDK meeting. Note the following:
*
* -
*
* Not all resources have tags. For a list of services with resources that support tagging using this
* operation, see Services that support the Resource Groups Tagging API. If the resource doesn't yet support this
* operation, the resource's service might support tagging using its own API operations. For more
* information, refer to the documentation for that service.
*
*
* -
*
* Each resource can have up to 50 tags. For other limits, see Tag Naming and Usage
* Conventions in the AWS General Reference.
*
*
* -
*
* You can only tag resources that are located in the specified Amazon Web Services Region for the Amazon Web
* Services account.
*
*
* -
*
* To add tags to a resource, you need the necessary permissions for the service that the resource belongs to
* as well as permissions for adding tags. For more information, see the documentation for each service.
*
*
*
*
*
* Do not store personally identifiable information (PII) or other confidential or sensitive information in
* tags. We use tags to provide you with billing and administration services. Tags are not intended to be
* used for private or sensitive data.
*
*
*
* Minimum permissions
*
*
* In addition to the tag:TagResources
permission required by this operation, you must also have
* the tagging permission defined by the service that created the resource. For example, to tag a
* ChimeSDKMeetings
instance using the TagResources
operation, you must have both
* of the following permissions:
*
*
* tag:TagResources
*
*
* ChimeSDKMeetings:CreateTags
*
*
*
* Some services might have specific requirements for tagging some resources. For example, to tag an Amazon
* S3 bucket, you must also have the s3:GetBucketTagging
permission. If the expected minimum
* permissions don't work, check the documentation for that service's tagging APIs for more information.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getClientRequestToken() != null)
sb.append("ClientRequestToken: ").append("***Sensitive Data Redacted***").append(",");
if (getMediaRegion() != null)
sb.append("MediaRegion: ").append(getMediaRegion()).append(",");
if (getMeetingHostId() != null)
sb.append("MeetingHostId: ").append("***Sensitive Data Redacted***").append(",");
if (getExternalMeetingId() != null)
sb.append("ExternalMeetingId: ").append("***Sensitive Data Redacted***").append(",");
if (getNotificationsConfiguration() != null)
sb.append("NotificationsConfiguration: ").append(getNotificationsConfiguration()).append(",");
if (getMeetingFeatures() != null)
sb.append("MeetingFeatures: ").append(getMeetingFeatures()).append(",");
if (getPrimaryMeetingId() != null)
sb.append("PrimaryMeetingId: ").append(getPrimaryMeetingId()).append(",");
if (getTenantIds() != null)
sb.append("TenantIds: ").append(getTenantIds()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateMeetingRequest == false)
return false;
CreateMeetingRequest other = (CreateMeetingRequest) obj;
if (other.getClientRequestToken() == null ^ this.getClientRequestToken() == null)
return false;
if (other.getClientRequestToken() != null && other.getClientRequestToken().equals(this.getClientRequestToken()) == false)
return false;
if (other.getMediaRegion() == null ^ this.getMediaRegion() == null)
return false;
if (other.getMediaRegion() != null && other.getMediaRegion().equals(this.getMediaRegion()) == false)
return false;
if (other.getMeetingHostId() == null ^ this.getMeetingHostId() == null)
return false;
if (other.getMeetingHostId() != null && other.getMeetingHostId().equals(this.getMeetingHostId()) == false)
return false;
if (other.getExternalMeetingId() == null ^ this.getExternalMeetingId() == null)
return false;
if (other.getExternalMeetingId() != null && other.getExternalMeetingId().equals(this.getExternalMeetingId()) == false)
return false;
if (other.getNotificationsConfiguration() == null ^ this.getNotificationsConfiguration() == null)
return false;
if (other.getNotificationsConfiguration() != null && other.getNotificationsConfiguration().equals(this.getNotificationsConfiguration()) == false)
return false;
if (other.getMeetingFeatures() == null ^ this.getMeetingFeatures() == null)
return false;
if (other.getMeetingFeatures() != null && other.getMeetingFeatures().equals(this.getMeetingFeatures()) == false)
return false;
if (other.getPrimaryMeetingId() == null ^ this.getPrimaryMeetingId() == null)
return false;
if (other.getPrimaryMeetingId() != null && other.getPrimaryMeetingId().equals(this.getPrimaryMeetingId()) == false)
return false;
if (other.getTenantIds() == null ^ this.getTenantIds() == null)
return false;
if (other.getTenantIds() != null && other.getTenantIds().equals(this.getTenantIds()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getClientRequestToken() == null) ? 0 : getClientRequestToken().hashCode());
hashCode = prime * hashCode + ((getMediaRegion() == null) ? 0 : getMediaRegion().hashCode());
hashCode = prime * hashCode + ((getMeetingHostId() == null) ? 0 : getMeetingHostId().hashCode());
hashCode = prime * hashCode + ((getExternalMeetingId() == null) ? 0 : getExternalMeetingId().hashCode());
hashCode = prime * hashCode + ((getNotificationsConfiguration() == null) ? 0 : getNotificationsConfiguration().hashCode());
hashCode = prime * hashCode + ((getMeetingFeatures() == null) ? 0 : getMeetingFeatures().hashCode());
hashCode = prime * hashCode + ((getPrimaryMeetingId() == null) ? 0 : getPrimaryMeetingId().hashCode());
hashCode = prime * hashCode + ((getTenantIds() == null) ? 0 : getTenantIds().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateMeetingRequest clone() {
return (CreateMeetingRequest) super.clone();
}
}