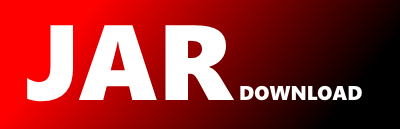
com.amazonaws.services.chimesdkmeetings.model.CreateMeetingWithAttendeesRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chimesdkmeetings Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chimesdkmeetings.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateMeetingWithAttendeesRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The unique identifier for the client request. Use a different token for different meetings.
*
*/
private String clientRequestToken;
/**
*
* The Region in which to create the meeting.
*
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
, ca-central-1
,
* eu-central-1
, eu-north-1
, eu-south-1
, eu-west-1
,
* eu-west-2
, eu-west-3
, sa-east-1
, us-east-1
,
* us-east-2
, us-west-1
, us-west-2
.
*
*
* Available values in Amazon Web Services GovCloud (US) Regions: us-gov-east-1
,
* us-gov-west-1
.
*
*/
private String mediaRegion;
/**
*
* Reserved.
*
*/
private String meetingHostId;
/**
*
* The external meeting ID.
*
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix. Case
* insensitive.
*
*/
private String externalMeetingId;
/**
*
* Lists the audio and video features enabled for a meeting, such as echo reduction.
*
*/
private MeetingFeaturesConfiguration meetingFeatures;
/**
*
* The configuration for resource targets to receive notifications when meeting and attendee events occur.
*
*/
private NotificationsConfiguration notificationsConfiguration;
/**
*
* The attendee information, including attendees' IDs and join tokens.
*
*/
private java.util.List attendees;
/**
*
* When specified, replicates the media from the primary meeting to the new meeting.
*
*/
private String primaryMeetingId;
/**
*
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their users.
*
*/
private java.util.List tenantIds;
/**
*
* The tags in the request.
*
*/
private java.util.List tags;
/**
*
* The unique identifier for the client request. Use a different token for different meetings.
*
*
* @param clientRequestToken
* The unique identifier for the client request. Use a different token for different meetings.
*/
public void setClientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
}
/**
*
* The unique identifier for the client request. Use a different token for different meetings.
*
*
* @return The unique identifier for the client request. Use a different token for different meetings.
*/
public String getClientRequestToken() {
return this.clientRequestToken;
}
/**
*
* The unique identifier for the client request. Use a different token for different meetings.
*
*
* @param clientRequestToken
* The unique identifier for the client request. Use a different token for different meetings.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withClientRequestToken(String clientRequestToken) {
setClientRequestToken(clientRequestToken);
return this;
}
/**
*
* The Region in which to create the meeting.
*
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
, ca-central-1
,
* eu-central-1
, eu-north-1
, eu-south-1
, eu-west-1
,
* eu-west-2
, eu-west-3
, sa-east-1
, us-east-1
,
* us-east-2
, us-west-1
, us-west-2
.
*
*
* Available values in Amazon Web Services GovCloud (US) Regions: us-gov-east-1
,
* us-gov-west-1
.
*
*
* @param mediaRegion
* The Region in which to create the meeting.
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
,
* ca-central-1
, eu-central-1
, eu-north-1
, eu-south-1
,
* eu-west-1
, eu-west-2
, eu-west-3
, sa-east-1
,
* us-east-1
, us-east-2
, us-west-1
, us-west-2
.
*
*
* Available values in Amazon Web Services GovCloud (US) Regions: us-gov-east-1
,
* us-gov-west-1
.
*/
public void setMediaRegion(String mediaRegion) {
this.mediaRegion = mediaRegion;
}
/**
*
* The Region in which to create the meeting.
*
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
, ca-central-1
,
* eu-central-1
, eu-north-1
, eu-south-1
, eu-west-1
,
* eu-west-2
, eu-west-3
, sa-east-1
, us-east-1
,
* us-east-2
, us-west-1
, us-west-2
.
*
*
* Available values in Amazon Web Services GovCloud (US) Regions: us-gov-east-1
,
* us-gov-west-1
.
*
*
* @return The Region in which to create the meeting.
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
,
* ca-central-1
, eu-central-1
, eu-north-1
, eu-south-1
,
* eu-west-1
, eu-west-2
, eu-west-3
, sa-east-1
,
* us-east-1
, us-east-2
, us-west-1
, us-west-2
.
*
*
* Available values in Amazon Web Services GovCloud (US) Regions: us-gov-east-1
,
* us-gov-west-1
.
*/
public String getMediaRegion() {
return this.mediaRegion;
}
/**
*
* The Region in which to create the meeting.
*
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
, ca-central-1
,
* eu-central-1
, eu-north-1
, eu-south-1
, eu-west-1
,
* eu-west-2
, eu-west-3
, sa-east-1
, us-east-1
,
* us-east-2
, us-west-1
, us-west-2
.
*
*
* Available values in Amazon Web Services GovCloud (US) Regions: us-gov-east-1
,
* us-gov-west-1
.
*
*
* @param mediaRegion
* The Region in which to create the meeting.
*
* Available values: af-south-1
, ap-northeast-1
, ap-northeast-2
,
* ap-south-1
, ap-southeast-1
, ap-southeast-2
,
* ca-central-1
, eu-central-1
, eu-north-1
, eu-south-1
,
* eu-west-1
, eu-west-2
, eu-west-3
, sa-east-1
,
* us-east-1
, us-east-2
, us-west-1
, us-west-2
.
*
*
* Available values in Amazon Web Services GovCloud (US) Regions: us-gov-east-1
,
* us-gov-west-1
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withMediaRegion(String mediaRegion) {
setMediaRegion(mediaRegion);
return this;
}
/**
*
* Reserved.
*
*
* @param meetingHostId
* Reserved.
*/
public void setMeetingHostId(String meetingHostId) {
this.meetingHostId = meetingHostId;
}
/**
*
* Reserved.
*
*
* @return Reserved.
*/
public String getMeetingHostId() {
return this.meetingHostId;
}
/**
*
* Reserved.
*
*
* @param meetingHostId
* Reserved.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withMeetingHostId(String meetingHostId) {
setMeetingHostId(meetingHostId);
return this;
}
/**
*
* The external meeting ID.
*
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix. Case
* insensitive.
*
*
* @param externalMeetingId
* The external meeting ID.
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix.
* Case insensitive.
*/
public void setExternalMeetingId(String externalMeetingId) {
this.externalMeetingId = externalMeetingId;
}
/**
*
* The external meeting ID.
*
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix. Case
* insensitive.
*
*
* @return The external meeting ID.
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix.
* Case insensitive.
*/
public String getExternalMeetingId() {
return this.externalMeetingId;
}
/**
*
* The external meeting ID.
*
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix. Case
* insensitive.
*
*
* @param externalMeetingId
* The external meeting ID.
*
* Pattern: [-_&@+=,(){}\[\]\/«».:|'"#a-zA-Z0-9À-ÿ\s]*
*
*
* Values that begin with aws:
are reserved. You can't configure a value that uses this prefix.
* Case insensitive.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withExternalMeetingId(String externalMeetingId) {
setExternalMeetingId(externalMeetingId);
return this;
}
/**
*
* Lists the audio and video features enabled for a meeting, such as echo reduction.
*
*
* @param meetingFeatures
* Lists the audio and video features enabled for a meeting, such as echo reduction.
*/
public void setMeetingFeatures(MeetingFeaturesConfiguration meetingFeatures) {
this.meetingFeatures = meetingFeatures;
}
/**
*
* Lists the audio and video features enabled for a meeting, such as echo reduction.
*
*
* @return Lists the audio and video features enabled for a meeting, such as echo reduction.
*/
public MeetingFeaturesConfiguration getMeetingFeatures() {
return this.meetingFeatures;
}
/**
*
* Lists the audio and video features enabled for a meeting, such as echo reduction.
*
*
* @param meetingFeatures
* Lists the audio and video features enabled for a meeting, such as echo reduction.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withMeetingFeatures(MeetingFeaturesConfiguration meetingFeatures) {
setMeetingFeatures(meetingFeatures);
return this;
}
/**
*
* The configuration for resource targets to receive notifications when meeting and attendee events occur.
*
*
* @param notificationsConfiguration
* The configuration for resource targets to receive notifications when meeting and attendee events occur.
*/
public void setNotificationsConfiguration(NotificationsConfiguration notificationsConfiguration) {
this.notificationsConfiguration = notificationsConfiguration;
}
/**
*
* The configuration for resource targets to receive notifications when meeting and attendee events occur.
*
*
* @return The configuration for resource targets to receive notifications when meeting and attendee events occur.
*/
public NotificationsConfiguration getNotificationsConfiguration() {
return this.notificationsConfiguration;
}
/**
*
* The configuration for resource targets to receive notifications when meeting and attendee events occur.
*
*
* @param notificationsConfiguration
* The configuration for resource targets to receive notifications when meeting and attendee events occur.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withNotificationsConfiguration(NotificationsConfiguration notificationsConfiguration) {
setNotificationsConfiguration(notificationsConfiguration);
return this;
}
/**
*
* The attendee information, including attendees' IDs and join tokens.
*
*
* @return The attendee information, including attendees' IDs and join tokens.
*/
public java.util.List getAttendees() {
return attendees;
}
/**
*
* The attendee information, including attendees' IDs and join tokens.
*
*
* @param attendees
* The attendee information, including attendees' IDs and join tokens.
*/
public void setAttendees(java.util.Collection attendees) {
if (attendees == null) {
this.attendees = null;
return;
}
this.attendees = new java.util.ArrayList(attendees);
}
/**
*
* The attendee information, including attendees' IDs and join tokens.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAttendees(java.util.Collection)} or {@link #withAttendees(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param attendees
* The attendee information, including attendees' IDs and join tokens.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withAttendees(CreateAttendeeRequestItem... attendees) {
if (this.attendees == null) {
setAttendees(new java.util.ArrayList(attendees.length));
}
for (CreateAttendeeRequestItem ele : attendees) {
this.attendees.add(ele);
}
return this;
}
/**
*
* The attendee information, including attendees' IDs and join tokens.
*
*
* @param attendees
* The attendee information, including attendees' IDs and join tokens.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withAttendees(java.util.Collection attendees) {
setAttendees(attendees);
return this;
}
/**
*
* When specified, replicates the media from the primary meeting to the new meeting.
*
*
* @param primaryMeetingId
* When specified, replicates the media from the primary meeting to the new meeting.
*/
public void setPrimaryMeetingId(String primaryMeetingId) {
this.primaryMeetingId = primaryMeetingId;
}
/**
*
* When specified, replicates the media from the primary meeting to the new meeting.
*
*
* @return When specified, replicates the media from the primary meeting to the new meeting.
*/
public String getPrimaryMeetingId() {
return this.primaryMeetingId;
}
/**
*
* When specified, replicates the media from the primary meeting to the new meeting.
*
*
* @param primaryMeetingId
* When specified, replicates the media from the primary meeting to the new meeting.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withPrimaryMeetingId(String primaryMeetingId) {
setPrimaryMeetingId(primaryMeetingId);
return this;
}
/**
*
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their users.
*
*
* @return A consistent and opaque identifier, created and maintained by the builder to represent a segment of their
* users.
*/
public java.util.List getTenantIds() {
return tenantIds;
}
/**
*
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their users.
*
*
* @param tenantIds
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their
* users.
*/
public void setTenantIds(java.util.Collection tenantIds) {
if (tenantIds == null) {
this.tenantIds = null;
return;
}
this.tenantIds = new java.util.ArrayList(tenantIds);
}
/**
*
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their users.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTenantIds(java.util.Collection)} or {@link #withTenantIds(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param tenantIds
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their
* users.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withTenantIds(String... tenantIds) {
if (this.tenantIds == null) {
setTenantIds(new java.util.ArrayList(tenantIds.length));
}
for (String ele : tenantIds) {
this.tenantIds.add(ele);
}
return this;
}
/**
*
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their users.
*
*
* @param tenantIds
* A consistent and opaque identifier, created and maintained by the builder to represent a segment of their
* users.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withTenantIds(java.util.Collection tenantIds) {
setTenantIds(tenantIds);
return this;
}
/**
*
* The tags in the request.
*
*
* @return The tags in the request.
*/
public java.util.List getTags() {
return tags;
}
/**
*
* The tags in the request.
*
*
* @param tags
* The tags in the request.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new java.util.ArrayList(tags);
}
/**
*
* The tags in the request.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The tags in the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new java.util.ArrayList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The tags in the request.
*
*
* @param tags
* The tags in the request.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateMeetingWithAttendeesRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getClientRequestToken() != null)
sb.append("ClientRequestToken: ").append("***Sensitive Data Redacted***").append(",");
if (getMediaRegion() != null)
sb.append("MediaRegion: ").append(getMediaRegion()).append(",");
if (getMeetingHostId() != null)
sb.append("MeetingHostId: ").append("***Sensitive Data Redacted***").append(",");
if (getExternalMeetingId() != null)
sb.append("ExternalMeetingId: ").append("***Sensitive Data Redacted***").append(",");
if (getMeetingFeatures() != null)
sb.append("MeetingFeatures: ").append(getMeetingFeatures()).append(",");
if (getNotificationsConfiguration() != null)
sb.append("NotificationsConfiguration: ").append(getNotificationsConfiguration()).append(",");
if (getAttendees() != null)
sb.append("Attendees: ").append(getAttendees()).append(",");
if (getPrimaryMeetingId() != null)
sb.append("PrimaryMeetingId: ").append(getPrimaryMeetingId()).append(",");
if (getTenantIds() != null)
sb.append("TenantIds: ").append(getTenantIds()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateMeetingWithAttendeesRequest == false)
return false;
CreateMeetingWithAttendeesRequest other = (CreateMeetingWithAttendeesRequest) obj;
if (other.getClientRequestToken() == null ^ this.getClientRequestToken() == null)
return false;
if (other.getClientRequestToken() != null && other.getClientRequestToken().equals(this.getClientRequestToken()) == false)
return false;
if (other.getMediaRegion() == null ^ this.getMediaRegion() == null)
return false;
if (other.getMediaRegion() != null && other.getMediaRegion().equals(this.getMediaRegion()) == false)
return false;
if (other.getMeetingHostId() == null ^ this.getMeetingHostId() == null)
return false;
if (other.getMeetingHostId() != null && other.getMeetingHostId().equals(this.getMeetingHostId()) == false)
return false;
if (other.getExternalMeetingId() == null ^ this.getExternalMeetingId() == null)
return false;
if (other.getExternalMeetingId() != null && other.getExternalMeetingId().equals(this.getExternalMeetingId()) == false)
return false;
if (other.getMeetingFeatures() == null ^ this.getMeetingFeatures() == null)
return false;
if (other.getMeetingFeatures() != null && other.getMeetingFeatures().equals(this.getMeetingFeatures()) == false)
return false;
if (other.getNotificationsConfiguration() == null ^ this.getNotificationsConfiguration() == null)
return false;
if (other.getNotificationsConfiguration() != null && other.getNotificationsConfiguration().equals(this.getNotificationsConfiguration()) == false)
return false;
if (other.getAttendees() == null ^ this.getAttendees() == null)
return false;
if (other.getAttendees() != null && other.getAttendees().equals(this.getAttendees()) == false)
return false;
if (other.getPrimaryMeetingId() == null ^ this.getPrimaryMeetingId() == null)
return false;
if (other.getPrimaryMeetingId() != null && other.getPrimaryMeetingId().equals(this.getPrimaryMeetingId()) == false)
return false;
if (other.getTenantIds() == null ^ this.getTenantIds() == null)
return false;
if (other.getTenantIds() != null && other.getTenantIds().equals(this.getTenantIds()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getClientRequestToken() == null) ? 0 : getClientRequestToken().hashCode());
hashCode = prime * hashCode + ((getMediaRegion() == null) ? 0 : getMediaRegion().hashCode());
hashCode = prime * hashCode + ((getMeetingHostId() == null) ? 0 : getMeetingHostId().hashCode());
hashCode = prime * hashCode + ((getExternalMeetingId() == null) ? 0 : getExternalMeetingId().hashCode());
hashCode = prime * hashCode + ((getMeetingFeatures() == null) ? 0 : getMeetingFeatures().hashCode());
hashCode = prime * hashCode + ((getNotificationsConfiguration() == null) ? 0 : getNotificationsConfiguration().hashCode());
hashCode = prime * hashCode + ((getAttendees() == null) ? 0 : getAttendees().hashCode());
hashCode = prime * hashCode + ((getPrimaryMeetingId() == null) ? 0 : getPrimaryMeetingId().hashCode());
hashCode = prime * hashCode + ((getTenantIds() == null) ? 0 : getTenantIds().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
return hashCode;
}
@Override
public CreateMeetingWithAttendeesRequest clone() {
return (CreateMeetingWithAttendeesRequest) super.clone();
}
}