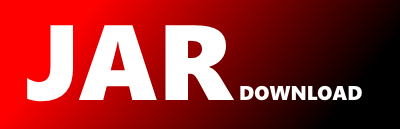
com.amazonaws.services.chimesdkvoice.AmazonChimeSDKVoiceClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-chimesdkvoice Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.chimesdkvoice;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.chimesdkvoice.AmazonChimeSDKVoiceClientBuilder;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.chimesdkvoice.model.*;
import com.amazonaws.services.chimesdkvoice.model.transform.*;
/**
* Client for accessing Amazon Chime SDK Voice. All service calls made using this client are blocking, and will not
* return until the service call completes.
*
*
* The Amazon Chime SDK telephony APIs in this section enable developers to create PSTN calling solutions that use
* Amazon Chime SDK Voice Connectors, and Amazon Chime SDK SIP media applications. Developers can also order and manage
* phone numbers, create and manage Voice Connectors and SIP media applications, and run voice analytics.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonChimeSDKVoiceClient extends AmazonWebServiceClient implements AmazonChimeSDKVoice {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonChimeSDKVoice.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "chime";
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
private static final com.amazonaws.protocol.json.SdkJsonProtocolFactory protocolFactory = new com.amazonaws.protocol.json.SdkJsonProtocolFactory(
new JsonClientMetadata()
.withProtocolVersion("1.1")
.withSupportsCbor(false)
.withSupportsIon(false)
.withContentTypeOverride("application/json")
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ForbiddenException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkvoice.model.transform.ForbiddenExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ResourceLimitExceededException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkvoice.model.transform.ResourceLimitExceededExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("GoneException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkvoice.model.transform.GoneExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnauthorizedClientException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkvoice.model.transform.UnauthorizedClientExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("NotFoundException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkvoice.model.transform.NotFoundExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceFailureException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkvoice.model.transform.ServiceFailureExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("AccessDeniedException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkvoice.model.transform.AccessDeniedExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ConflictException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkvoice.model.transform.ConflictExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("UnprocessableEntityException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkvoice.model.transform.UnprocessableEntityExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ThrottledClientException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkvoice.model.transform.ThrottledClientExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("ServiceUnavailableException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkvoice.model.transform.ServiceUnavailableExceptionUnmarshaller.getInstance()))
.addErrorMetadata(
new JsonErrorShapeMetadata().withErrorCode("BadRequestException").withExceptionUnmarshaller(
com.amazonaws.services.chimesdkvoice.model.transform.BadRequestExceptionUnmarshaller.getInstance()))
.withBaseServiceExceptionClass(com.amazonaws.services.chimesdkvoice.model.AmazonChimeSDKVoiceException.class));
public static AmazonChimeSDKVoiceClientBuilder builder() {
return AmazonChimeSDKVoiceClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on Amazon Chime SDK Voice using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonChimeSDKVoiceClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on Amazon Chime SDK Voice using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonChimeSDKVoiceClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
setEndpoint("voice-chime.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/chimesdkvoice/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/chimesdkvoice/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Associates phone numbers with the specified Amazon Chime SDK Voice Connector.
*
*
* @param associatePhoneNumbersWithVoiceConnectorRequest
* @return Result of the AssociatePhoneNumbersWithVoiceConnector operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.AssociatePhoneNumbersWithVoiceConnector
* @see AWS API Documentation
*/
@Override
public AssociatePhoneNumbersWithVoiceConnectorResult associatePhoneNumbersWithVoiceConnector(AssociatePhoneNumbersWithVoiceConnectorRequest request) {
request = beforeClientExecution(request);
return executeAssociatePhoneNumbersWithVoiceConnector(request);
}
@SdkInternalApi
final AssociatePhoneNumbersWithVoiceConnectorResult executeAssociatePhoneNumbersWithVoiceConnector(
AssociatePhoneNumbersWithVoiceConnectorRequest associatePhoneNumbersWithVoiceConnectorRequest) {
ExecutionContext executionContext = createExecutionContext(associatePhoneNumbersWithVoiceConnectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociatePhoneNumbersWithVoiceConnectorRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associatePhoneNumbersWithVoiceConnectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociatePhoneNumbersWithVoiceConnector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociatePhoneNumbersWithVoiceConnectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Associates phone numbers with the specified Amazon Chime SDK Voice Connector group.
*
*
* @param associatePhoneNumbersWithVoiceConnectorGroupRequest
* @return Result of the AssociatePhoneNumbersWithVoiceConnectorGroup operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.AssociatePhoneNumbersWithVoiceConnectorGroup
* @see AWS API Documentation
*/
@Override
public AssociatePhoneNumbersWithVoiceConnectorGroupResult associatePhoneNumbersWithVoiceConnectorGroup(
AssociatePhoneNumbersWithVoiceConnectorGroupRequest request) {
request = beforeClientExecution(request);
return executeAssociatePhoneNumbersWithVoiceConnectorGroup(request);
}
@SdkInternalApi
final AssociatePhoneNumbersWithVoiceConnectorGroupResult executeAssociatePhoneNumbersWithVoiceConnectorGroup(
AssociatePhoneNumbersWithVoiceConnectorGroupRequest associatePhoneNumbersWithVoiceConnectorGroupRequest) {
ExecutionContext executionContext = createExecutionContext(associatePhoneNumbersWithVoiceConnectorGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new AssociatePhoneNumbersWithVoiceConnectorGroupRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(associatePhoneNumbersWithVoiceConnectorGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "AssociatePhoneNumbersWithVoiceConnectorGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new AssociatePhoneNumbersWithVoiceConnectorGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Moves phone numbers into the Deletion queue. Phone numbers must be disassociated from any users or Amazon
* Chime SDK Voice Connectors before they can be deleted.
*
*
* Phone numbers remain in the Deletion queue for 7 days before they are deleted permanently.
*
*
* @param batchDeletePhoneNumberRequest
* @return Result of the BatchDeletePhoneNumber operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.BatchDeletePhoneNumber
* @see AWS API Documentation
*/
@Override
public BatchDeletePhoneNumberResult batchDeletePhoneNumber(BatchDeletePhoneNumberRequest request) {
request = beforeClientExecution(request);
return executeBatchDeletePhoneNumber(request);
}
@SdkInternalApi
final BatchDeletePhoneNumberResult executeBatchDeletePhoneNumber(BatchDeletePhoneNumberRequest batchDeletePhoneNumberRequest) {
ExecutionContext executionContext = createExecutionContext(batchDeletePhoneNumberRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchDeletePhoneNumberRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(batchDeletePhoneNumberRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchDeletePhoneNumber");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchDeletePhoneNumberResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates one or more phone numbers.
*
*
* @param batchUpdatePhoneNumberRequest
* @return Result of the BatchUpdatePhoneNumber operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.BatchUpdatePhoneNumber
* @see AWS API Documentation
*/
@Override
public BatchUpdatePhoneNumberResult batchUpdatePhoneNumber(BatchUpdatePhoneNumberRequest request) {
request = beforeClientExecution(request);
return executeBatchUpdatePhoneNumber(request);
}
@SdkInternalApi
final BatchUpdatePhoneNumberResult executeBatchUpdatePhoneNumber(BatchUpdatePhoneNumberRequest batchUpdatePhoneNumberRequest) {
ExecutionContext executionContext = createExecutionContext(batchUpdatePhoneNumberRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchUpdatePhoneNumberRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(batchUpdatePhoneNumberRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchUpdatePhoneNumber");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new BatchUpdatePhoneNumberResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an order for phone numbers to be provisioned. For numbers outside the U.S., you must use the Amazon Chime
* SDK SIP media application dial-in product type.
*
*
* @param createPhoneNumberOrderRequest
* @return Result of the CreatePhoneNumberOrder operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.CreatePhoneNumberOrder
* @see AWS API Documentation
*/
@Override
public CreatePhoneNumberOrderResult createPhoneNumberOrder(CreatePhoneNumberOrderRequest request) {
request = beforeClientExecution(request);
return executeCreatePhoneNumberOrder(request);
}
@SdkInternalApi
final CreatePhoneNumberOrderResult executeCreatePhoneNumberOrder(CreatePhoneNumberOrderRequest createPhoneNumberOrderRequest) {
ExecutionContext executionContext = createExecutionContext(createPhoneNumberOrderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreatePhoneNumberOrderRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createPhoneNumberOrderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreatePhoneNumberOrder");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreatePhoneNumberOrderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a proxy session for the specified Amazon Chime SDK Voice Connector for the specified participant phone
* numbers.
*
*
* @param createProxySessionRequest
* @return Result of the CreateProxySession operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.CreateProxySession
* @see AWS API Documentation
*/
@Override
public CreateProxySessionResult createProxySession(CreateProxySessionRequest request) {
request = beforeClientExecution(request);
return executeCreateProxySession(request);
}
@SdkInternalApi
final CreateProxySessionResult executeCreateProxySession(CreateProxySessionRequest createProxySessionRequest) {
ExecutionContext executionContext = createExecutionContext(createProxySessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateProxySessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createProxySessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateProxySession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateProxySessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a SIP media application. For more information about SIP media applications, see Managing SIP media
* applications and rules in the Amazon Chime SDK Administrator Guide.
*
*
* @param createSipMediaApplicationRequest
* @return Result of the CreateSipMediaApplication operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.CreateSipMediaApplication
* @see AWS API Documentation
*/
@Override
public CreateSipMediaApplicationResult createSipMediaApplication(CreateSipMediaApplicationRequest request) {
request = beforeClientExecution(request);
return executeCreateSipMediaApplication(request);
}
@SdkInternalApi
final CreateSipMediaApplicationResult executeCreateSipMediaApplication(CreateSipMediaApplicationRequest createSipMediaApplicationRequest) {
ExecutionContext executionContext = createExecutionContext(createSipMediaApplicationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSipMediaApplicationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createSipMediaApplicationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSipMediaApplication");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateSipMediaApplicationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an outbound call to a phone number from the phone number specified in the request, and it invokes the
* endpoint of the specified sipMediaApplicationId
.
*
*
* @param createSipMediaApplicationCallRequest
* @return Result of the CreateSipMediaApplicationCall operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.CreateSipMediaApplicationCall
* @see AWS API Documentation
*/
@Override
public CreateSipMediaApplicationCallResult createSipMediaApplicationCall(CreateSipMediaApplicationCallRequest request) {
request = beforeClientExecution(request);
return executeCreateSipMediaApplicationCall(request);
}
@SdkInternalApi
final CreateSipMediaApplicationCallResult executeCreateSipMediaApplicationCall(CreateSipMediaApplicationCallRequest createSipMediaApplicationCallRequest) {
ExecutionContext executionContext = createExecutionContext(createSipMediaApplicationCallRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSipMediaApplicationCallRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createSipMediaApplicationCallRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSipMediaApplicationCall");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateSipMediaApplicationCallResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a SIP rule, which can be used to run a SIP media application as a target for a specific trigger type. For
* more information about SIP rules, see Managing SIP media
* applications and rules in the Amazon Chime SDK Administrator Guide.
*
*
* @param createSipRuleRequest
* @return Result of the CreateSipRule operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.CreateSipRule
* @see AWS
* API Documentation
*/
@Override
public CreateSipRuleResult createSipRule(CreateSipRuleRequest request) {
request = beforeClientExecution(request);
return executeCreateSipRule(request);
}
@SdkInternalApi
final CreateSipRuleResult executeCreateSipRule(CreateSipRuleRequest createSipRuleRequest) {
ExecutionContext executionContext = createExecutionContext(createSipRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateSipRuleRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createSipRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateSipRule");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateSipRuleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon Chime SDK Voice Connector. For more information about Voice Connectors, see Managing Amazon Chime SDK
* Voice Connector groups in the Amazon Chime SDK Administrator Guide.
*
*
* @param createVoiceConnectorRequest
* @return Result of the CreateVoiceConnector operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.CreateVoiceConnector
* @see AWS API Documentation
*/
@Override
public CreateVoiceConnectorResult createVoiceConnector(CreateVoiceConnectorRequest request) {
request = beforeClientExecution(request);
return executeCreateVoiceConnector(request);
}
@SdkInternalApi
final CreateVoiceConnectorResult executeCreateVoiceConnector(CreateVoiceConnectorRequest createVoiceConnectorRequest) {
ExecutionContext executionContext = createExecutionContext(createVoiceConnectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateVoiceConnectorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createVoiceConnectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateVoiceConnector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateVoiceConnectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates an Amazon Chime SDK Voice Connector group under the administrator's AWS account. You can associate Amazon
* Chime SDK Voice Connectors with the Voice Connector group by including VoiceConnectorItems
in the
* request.
*
*
* You can include Voice Connectors from different AWS Regions in your group. This creates a fault tolerant
* mechanism for fallback in case of availability events.
*
*
* @param createVoiceConnectorGroupRequest
* @return Result of the CreateVoiceConnectorGroup operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.CreateVoiceConnectorGroup
* @see AWS API Documentation
*/
@Override
public CreateVoiceConnectorGroupResult createVoiceConnectorGroup(CreateVoiceConnectorGroupRequest request) {
request = beforeClientExecution(request);
return executeCreateVoiceConnectorGroup(request);
}
@SdkInternalApi
final CreateVoiceConnectorGroupResult executeCreateVoiceConnectorGroup(CreateVoiceConnectorGroupRequest createVoiceConnectorGroupRequest) {
ExecutionContext executionContext = createExecutionContext(createVoiceConnectorGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateVoiceConnectorGroupRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createVoiceConnectorGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateVoiceConnectorGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateVoiceConnectorGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a voice profile, which consists of an enrolled user and their latest voice print.
*
*
*
* Before creating any voice profiles, you must provide all notices and obtain all consents from the speaker as
* required under applicable privacy and biometrics laws, and as required under the AWS service terms for the Amazon Chime SDK.
*
*
*
* For more information about voice profiles and voice analytics, see Using Amazon Chime SDK Voice
* Analytics in the Amazon Chime SDK Developer Guide.
*
*
* @param createVoiceProfileRequest
* @return Result of the CreateVoiceProfile operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws GoneException
* Access to the target resource is no longer available at the origin server. This condition is likely to be
* permanent.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.CreateVoiceProfile
* @see AWS API Documentation
*/
@Override
public CreateVoiceProfileResult createVoiceProfile(CreateVoiceProfileRequest request) {
request = beforeClientExecution(request);
return executeCreateVoiceProfile(request);
}
@SdkInternalApi
final CreateVoiceProfileResult executeCreateVoiceProfile(CreateVoiceProfileRequest createVoiceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(createVoiceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateVoiceProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(createVoiceProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateVoiceProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new CreateVoiceProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a voice profile domain, a collection of voice profiles, their voice prints, and encrypted enrollment
* audio.
*
*
*
* Before creating any voice profiles, you must provide all notices and obtain all consents from the speaker as
* required under applicable privacy and biometrics laws, and as required under the AWS service terms for the Amazon Chime SDK.
*
*
*
* For more information about voice profile domains, see Using Amazon Chime SDK Voice
* Analytics in the Amazon Chime SDK Developer Guide.
*
*
* @param createVoiceProfileDomainRequest
* @return Result of the CreateVoiceProfileDomain operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.CreateVoiceProfileDomain
* @see AWS API Documentation
*/
@Override
public CreateVoiceProfileDomainResult createVoiceProfileDomain(CreateVoiceProfileDomainRequest request) {
request = beforeClientExecution(request);
return executeCreateVoiceProfileDomain(request);
}
@SdkInternalApi
final CreateVoiceProfileDomainResult executeCreateVoiceProfileDomain(CreateVoiceProfileDomainRequest createVoiceProfileDomainRequest) {
ExecutionContext executionContext = createExecutionContext(createVoiceProfileDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateVoiceProfileDomainRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(createVoiceProfileDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateVoiceProfileDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new CreateVoiceProfileDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Moves the specified phone number into the Deletion queue. A phone number must be disassociated from any
* users or Amazon Chime SDK Voice Connectors before it can be deleted.
*
*
* Deleted phone numbers remain in the Deletion queue queue for 7 days before they are deleted permanently.
*
*
* @param deletePhoneNumberRequest
* @return Result of the DeletePhoneNumber operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DeletePhoneNumber
* @see AWS API Documentation
*/
@Override
public DeletePhoneNumberResult deletePhoneNumber(DeletePhoneNumberRequest request) {
request = beforeClientExecution(request);
return executeDeletePhoneNumber(request);
}
@SdkInternalApi
final DeletePhoneNumberResult executeDeletePhoneNumber(DeletePhoneNumberRequest deletePhoneNumberRequest) {
ExecutionContext executionContext = createExecutionContext(deletePhoneNumberRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeletePhoneNumberRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deletePhoneNumberRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeletePhoneNumber");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeletePhoneNumberResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified proxy session from the specified Amazon Chime SDK Voice Connector.
*
*
* @param deleteProxySessionRequest
* @return Result of the DeleteProxySession operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DeleteProxySession
* @see AWS API Documentation
*/
@Override
public DeleteProxySessionResult deleteProxySession(DeleteProxySessionRequest request) {
request = beforeClientExecution(request);
return executeDeleteProxySession(request);
}
@SdkInternalApi
final DeleteProxySessionResult executeDeleteProxySession(DeleteProxySessionRequest deleteProxySessionRequest) {
ExecutionContext executionContext = createExecutionContext(deleteProxySessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteProxySessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteProxySessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteProxySession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteProxySessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a SIP media application.
*
*
* @param deleteSipMediaApplicationRequest
* @return Result of the DeleteSipMediaApplication operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DeleteSipMediaApplication
* @see AWS API Documentation
*/
@Override
public DeleteSipMediaApplicationResult deleteSipMediaApplication(DeleteSipMediaApplicationRequest request) {
request = beforeClientExecution(request);
return executeDeleteSipMediaApplication(request);
}
@SdkInternalApi
final DeleteSipMediaApplicationResult executeDeleteSipMediaApplication(DeleteSipMediaApplicationRequest deleteSipMediaApplicationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSipMediaApplicationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSipMediaApplicationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteSipMediaApplicationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSipMediaApplication");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteSipMediaApplicationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a SIP rule.
*
*
* @param deleteSipRuleRequest
* @return Result of the DeleteSipRule operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DeleteSipRule
* @see AWS
* API Documentation
*/
@Override
public DeleteSipRuleResult deleteSipRule(DeleteSipRuleRequest request) {
request = beforeClientExecution(request);
return executeDeleteSipRule(request);
}
@SdkInternalApi
final DeleteSipRuleResult executeDeleteSipRule(DeleteSipRuleRequest deleteSipRuleRequest) {
ExecutionContext executionContext = createExecutionContext(deleteSipRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteSipRuleRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteSipRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteSipRule");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteSipRuleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Amazon Chime SDK Voice Connector. Any phone numbers associated with the Amazon Chime SDK Voice
* Connector must be disassociated from it before it can be deleted.
*
*
* @param deleteVoiceConnectorRequest
* @return Result of the DeleteVoiceConnector operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DeleteVoiceConnector
* @see AWS API Documentation
*/
@Override
public DeleteVoiceConnectorResult deleteVoiceConnector(DeleteVoiceConnectorRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnector(request);
}
@SdkInternalApi
final DeleteVoiceConnectorResult executeDeleteVoiceConnector(DeleteVoiceConnectorRequest deleteVoiceConnectorRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteVoiceConnectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteVoiceConnectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the emergency calling details from the specified Amazon Chime SDK Voice Connector.
*
*
* @param deleteVoiceConnectorEmergencyCallingConfigurationRequest
* @return Result of the DeleteVoiceConnectorEmergencyCallingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DeleteVoiceConnectorEmergencyCallingConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteVoiceConnectorEmergencyCallingConfigurationResult deleteVoiceConnectorEmergencyCallingConfiguration(
DeleteVoiceConnectorEmergencyCallingConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnectorEmergencyCallingConfiguration(request);
}
@SdkInternalApi
final DeleteVoiceConnectorEmergencyCallingConfigurationResult executeDeleteVoiceConnectorEmergencyCallingConfiguration(
DeleteVoiceConnectorEmergencyCallingConfigurationRequest deleteVoiceConnectorEmergencyCallingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorEmergencyCallingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorEmergencyCallingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVoiceConnectorEmergencyCallingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnectorEmergencyCallingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVoiceConnectorEmergencyCallingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes an Amazon Chime SDK Voice Connector group. Any VoiceConnectorItems
and phone numbers
* associated with the group must be removed before it can be deleted.
*
*
* @param deleteVoiceConnectorGroupRequest
* @return Result of the DeleteVoiceConnectorGroup operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DeleteVoiceConnectorGroup
* @see AWS API Documentation
*/
@Override
public DeleteVoiceConnectorGroupResult deleteVoiceConnectorGroup(DeleteVoiceConnectorGroupRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnectorGroup(request);
}
@SdkInternalApi
final DeleteVoiceConnectorGroupResult executeDeleteVoiceConnectorGroup(DeleteVoiceConnectorGroupRequest deleteVoiceConnectorGroupRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorGroupRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVoiceConnectorGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnectorGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVoiceConnectorGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the origination settings for the specified Amazon Chime SDK Voice Connector.
*
*
*
* If emergency calling is configured for the Voice Connector, it must be deleted prior to deleting the origination
* settings.
*
*
*
* @param deleteVoiceConnectorOriginationRequest
* @return Result of the DeleteVoiceConnectorOrigination operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DeleteVoiceConnectorOrigination
* @see AWS API Documentation
*/
@Override
public DeleteVoiceConnectorOriginationResult deleteVoiceConnectorOrigination(DeleteVoiceConnectorOriginationRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnectorOrigination(request);
}
@SdkInternalApi
final DeleteVoiceConnectorOriginationResult executeDeleteVoiceConnectorOrigination(
DeleteVoiceConnectorOriginationRequest deleteVoiceConnectorOriginationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorOriginationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorOriginationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVoiceConnectorOriginationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnectorOrigination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVoiceConnectorOriginationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the proxy configuration from the specified Amazon Chime SDK Voice Connector.
*
*
* @param deleteVoiceConnectorProxyRequest
* @return Result of the DeleteVoiceConnectorProxy operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DeleteVoiceConnectorProxy
* @see AWS API Documentation
*/
@Override
public DeleteVoiceConnectorProxyResult deleteVoiceConnectorProxy(DeleteVoiceConnectorProxyRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnectorProxy(request);
}
@SdkInternalApi
final DeleteVoiceConnectorProxyResult executeDeleteVoiceConnectorProxy(DeleteVoiceConnectorProxyRequest deleteVoiceConnectorProxyRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorProxyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorProxyRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVoiceConnectorProxyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnectorProxy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVoiceConnectorProxyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a Voice Connector's streaming configuration.
*
*
* @param deleteVoiceConnectorStreamingConfigurationRequest
* @return Result of the DeleteVoiceConnectorStreamingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DeleteVoiceConnectorStreamingConfiguration
* @see AWS API Documentation
*/
@Override
public DeleteVoiceConnectorStreamingConfigurationResult deleteVoiceConnectorStreamingConfiguration(DeleteVoiceConnectorStreamingConfigurationRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnectorStreamingConfiguration(request);
}
@SdkInternalApi
final DeleteVoiceConnectorStreamingConfigurationResult executeDeleteVoiceConnectorStreamingConfiguration(
DeleteVoiceConnectorStreamingConfigurationRequest deleteVoiceConnectorStreamingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorStreamingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorStreamingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVoiceConnectorStreamingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnectorStreamingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVoiceConnectorStreamingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the termination settings for the specified Amazon Chime SDK Voice Connector.
*
*
*
* If emergency calling is configured for the Voice Connector, it must be deleted prior to deleting the termination
* settings.
*
*
*
* @param deleteVoiceConnectorTerminationRequest
* @return Result of the DeleteVoiceConnectorTermination operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DeleteVoiceConnectorTermination
* @see AWS API Documentation
*/
@Override
public DeleteVoiceConnectorTerminationResult deleteVoiceConnectorTermination(DeleteVoiceConnectorTerminationRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnectorTermination(request);
}
@SdkInternalApi
final DeleteVoiceConnectorTerminationResult executeDeleteVoiceConnectorTermination(
DeleteVoiceConnectorTerminationRequest deleteVoiceConnectorTerminationRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorTerminationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorTerminationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVoiceConnectorTerminationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnectorTermination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVoiceConnectorTerminationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified SIP credentials used by your equipment to authenticate during call termination.
*
*
* @param deleteVoiceConnectorTerminationCredentialsRequest
* @return Result of the DeleteVoiceConnectorTerminationCredentials operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DeleteVoiceConnectorTerminationCredentials
* @see AWS API Documentation
*/
@Override
public DeleteVoiceConnectorTerminationCredentialsResult deleteVoiceConnectorTerminationCredentials(DeleteVoiceConnectorTerminationCredentialsRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceConnectorTerminationCredentials(request);
}
@SdkInternalApi
final DeleteVoiceConnectorTerminationCredentialsResult executeDeleteVoiceConnectorTerminationCredentials(
DeleteVoiceConnectorTerminationCredentialsRequest deleteVoiceConnectorTerminationCredentialsRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceConnectorTerminationCredentialsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceConnectorTerminationCredentialsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVoiceConnectorTerminationCredentialsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceConnectorTerminationCredentials");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVoiceConnectorTerminationCredentialsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a voice profile, including its voice print and enrollment data. WARNING: This action is not reversible.
*
*
* @param deleteVoiceProfileRequest
* @return Result of the DeleteVoiceProfile operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DeleteVoiceProfile
* @see AWS API Documentation
*/
@Override
public DeleteVoiceProfileResult deleteVoiceProfile(DeleteVoiceProfileRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceProfile(request);
}
@SdkInternalApi
final DeleteVoiceProfileResult executeDeleteVoiceProfile(DeleteVoiceProfileRequest deleteVoiceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(deleteVoiceProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new DeleteVoiceProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes all voice profiles in the domain. WARNING: This action is not reversible.
*
*
* @param deleteVoiceProfileDomainRequest
* @return Result of the DeleteVoiceProfileDomain operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DeleteVoiceProfileDomain
* @see AWS API Documentation
*/
@Override
public DeleteVoiceProfileDomainResult deleteVoiceProfileDomain(DeleteVoiceProfileDomainRequest request) {
request = beforeClientExecution(request);
return executeDeleteVoiceProfileDomain(request);
}
@SdkInternalApi
final DeleteVoiceProfileDomainResult executeDeleteVoiceProfileDomain(DeleteVoiceProfileDomainRequest deleteVoiceProfileDomainRequest) {
ExecutionContext executionContext = createExecutionContext(deleteVoiceProfileDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteVoiceProfileDomainRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(deleteVoiceProfileDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteVoiceProfileDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DeleteVoiceProfileDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates the specified phone numbers from the specified Amazon Chime SDK Voice Connector.
*
*
* @param disassociatePhoneNumbersFromVoiceConnectorRequest
* @return Result of the DisassociatePhoneNumbersFromVoiceConnector operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DisassociatePhoneNumbersFromVoiceConnector
* @see AWS API Documentation
*/
@Override
public DisassociatePhoneNumbersFromVoiceConnectorResult disassociatePhoneNumbersFromVoiceConnector(DisassociatePhoneNumbersFromVoiceConnectorRequest request) {
request = beforeClientExecution(request);
return executeDisassociatePhoneNumbersFromVoiceConnector(request);
}
@SdkInternalApi
final DisassociatePhoneNumbersFromVoiceConnectorResult executeDisassociatePhoneNumbersFromVoiceConnector(
DisassociatePhoneNumbersFromVoiceConnectorRequest disassociatePhoneNumbersFromVoiceConnectorRequest) {
ExecutionContext executionContext = createExecutionContext(disassociatePhoneNumbersFromVoiceConnectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociatePhoneNumbersFromVoiceConnectorRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociatePhoneNumbersFromVoiceConnectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociatePhoneNumbersFromVoiceConnector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociatePhoneNumbersFromVoiceConnectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Disassociates the specified phone numbers from the specified Amazon Chime SDK Voice Connector group.
*
*
* @param disassociatePhoneNumbersFromVoiceConnectorGroupRequest
* @return Result of the DisassociatePhoneNumbersFromVoiceConnectorGroup operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.DisassociatePhoneNumbersFromVoiceConnectorGroup
* @see AWS API Documentation
*/
@Override
public DisassociatePhoneNumbersFromVoiceConnectorGroupResult disassociatePhoneNumbersFromVoiceConnectorGroup(
DisassociatePhoneNumbersFromVoiceConnectorGroupRequest request) {
request = beforeClientExecution(request);
return executeDisassociatePhoneNumbersFromVoiceConnectorGroup(request);
}
@SdkInternalApi
final DisassociatePhoneNumbersFromVoiceConnectorGroupResult executeDisassociatePhoneNumbersFromVoiceConnectorGroup(
DisassociatePhoneNumbersFromVoiceConnectorGroupRequest disassociatePhoneNumbersFromVoiceConnectorGroupRequest) {
ExecutionContext executionContext = createExecutionContext(disassociatePhoneNumbersFromVoiceConnectorGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DisassociatePhoneNumbersFromVoiceConnectorGroupRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(disassociatePhoneNumbersFromVoiceConnectorGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DisassociatePhoneNumbersFromVoiceConnectorGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new DisassociatePhoneNumbersFromVoiceConnectorGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the global settings for the Amazon Chime SDK Voice Connectors in an AWS account.
*
*
* @param getGlobalSettingsRequest
* @return Result of the GetGlobalSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetGlobalSettings
* @see AWS API Documentation
*/
@Override
public GetGlobalSettingsResult getGlobalSettings(GetGlobalSettingsRequest request) {
request = beforeClientExecution(request);
return executeGetGlobalSettings(request);
}
@SdkInternalApi
final GetGlobalSettingsResult executeGetGlobalSettings(GetGlobalSettingsRequest getGlobalSettingsRequest) {
ExecutionContext executionContext = createExecutionContext(getGlobalSettingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetGlobalSettingsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getGlobalSettingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetGlobalSettings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetGlobalSettingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves details for the specified phone number ID, such as associations, capabilities, and product type.
*
*
* @param getPhoneNumberRequest
* @return Result of the GetPhoneNumber operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetPhoneNumber
* @see AWS
* API Documentation
*/
@Override
public GetPhoneNumberResult getPhoneNumber(GetPhoneNumberRequest request) {
request = beforeClientExecution(request);
return executeGetPhoneNumber(request);
}
@SdkInternalApi
final GetPhoneNumberResult executeGetPhoneNumber(GetPhoneNumberRequest getPhoneNumberRequest) {
ExecutionContext executionContext = createExecutionContext(getPhoneNumberRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPhoneNumberRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getPhoneNumberRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetPhoneNumber");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetPhoneNumberResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves details for the specified phone number order, such as the order creation timestamp, phone numbers in
* E.164 format, product type, and order status.
*
*
* @param getPhoneNumberOrderRequest
* @return Result of the GetPhoneNumberOrder operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetPhoneNumberOrder
* @see AWS API Documentation
*/
@Override
public GetPhoneNumberOrderResult getPhoneNumberOrder(GetPhoneNumberOrderRequest request) {
request = beforeClientExecution(request);
return executeGetPhoneNumberOrder(request);
}
@SdkInternalApi
final GetPhoneNumberOrderResult executeGetPhoneNumberOrder(GetPhoneNumberOrderRequest getPhoneNumberOrderRequest) {
ExecutionContext executionContext = createExecutionContext(getPhoneNumberOrderRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPhoneNumberOrderRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getPhoneNumberOrderRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetPhoneNumberOrder");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetPhoneNumberOrderResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the phone number settings for the administrator's AWS account, such as the default outbound calling
* name.
*
*
* @param getPhoneNumberSettingsRequest
* @return Result of the GetPhoneNumberSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetPhoneNumberSettings
* @see AWS API Documentation
*/
@Override
public GetPhoneNumberSettingsResult getPhoneNumberSettings(GetPhoneNumberSettingsRequest request) {
request = beforeClientExecution(request);
return executeGetPhoneNumberSettings(request);
}
@SdkInternalApi
final GetPhoneNumberSettingsResult executeGetPhoneNumberSettings(GetPhoneNumberSettingsRequest getPhoneNumberSettingsRequest) {
ExecutionContext executionContext = createExecutionContext(getPhoneNumberSettingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetPhoneNumberSettingsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getPhoneNumberSettingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetPhoneNumberSettings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetPhoneNumberSettingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the specified proxy session details for the specified Amazon Chime SDK Voice Connector.
*
*
* @param getProxySessionRequest
* @return Result of the GetProxySession operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetProxySession
* @see AWS API Documentation
*/
@Override
public GetProxySessionResult getProxySession(GetProxySessionRequest request) {
request = beforeClientExecution(request);
return executeGetProxySession(request);
}
@SdkInternalApi
final GetProxySessionResult executeGetProxySession(GetProxySessionRequest getProxySessionRequest) {
ExecutionContext executionContext = createExecutionContext(getProxySessionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetProxySessionRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getProxySessionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetProxySession");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetProxySessionResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the information for a SIP media application, including name, AWS Region, and endpoints.
*
*
* @param getSipMediaApplicationRequest
* @return Result of the GetSipMediaApplication operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetSipMediaApplication
* @see AWS API Documentation
*/
@Override
public GetSipMediaApplicationResult getSipMediaApplication(GetSipMediaApplicationRequest request) {
request = beforeClientExecution(request);
return executeGetSipMediaApplication(request);
}
@SdkInternalApi
final GetSipMediaApplicationResult executeGetSipMediaApplication(GetSipMediaApplicationRequest getSipMediaApplicationRequest) {
ExecutionContext executionContext = createExecutionContext(getSipMediaApplicationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSipMediaApplicationRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSipMediaApplicationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSipMediaApplication");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetSipMediaApplicationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Gets the Alexa Skill configuration for the SIP media application.
*
*
* @param getSipMediaApplicationAlexaSkillConfigurationRequest
* @return Result of the GetSipMediaApplicationAlexaSkillConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetSipMediaApplicationAlexaSkillConfiguration
* @see AWS API Documentation
*/
@Override
public GetSipMediaApplicationAlexaSkillConfigurationResult getSipMediaApplicationAlexaSkillConfiguration(
GetSipMediaApplicationAlexaSkillConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetSipMediaApplicationAlexaSkillConfiguration(request);
}
@SdkInternalApi
final GetSipMediaApplicationAlexaSkillConfigurationResult executeGetSipMediaApplicationAlexaSkillConfiguration(
GetSipMediaApplicationAlexaSkillConfigurationRequest getSipMediaApplicationAlexaSkillConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getSipMediaApplicationAlexaSkillConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSipMediaApplicationAlexaSkillConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getSipMediaApplicationAlexaSkillConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSipMediaApplicationAlexaSkillConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetSipMediaApplicationAlexaSkillConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the logging configuration for the specified SIP media application.
*
*
* @param getSipMediaApplicationLoggingConfigurationRequest
* @return Result of the GetSipMediaApplicationLoggingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetSipMediaApplicationLoggingConfiguration
* @see AWS API Documentation
*/
@Override
public GetSipMediaApplicationLoggingConfigurationResult getSipMediaApplicationLoggingConfiguration(GetSipMediaApplicationLoggingConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetSipMediaApplicationLoggingConfiguration(request);
}
@SdkInternalApi
final GetSipMediaApplicationLoggingConfigurationResult executeGetSipMediaApplicationLoggingConfiguration(
GetSipMediaApplicationLoggingConfigurationRequest getSipMediaApplicationLoggingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getSipMediaApplicationLoggingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSipMediaApplicationLoggingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getSipMediaApplicationLoggingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSipMediaApplicationLoggingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetSipMediaApplicationLoggingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the details of a SIP rule, such as the rule ID, name, triggers, and target endpoints.
*
*
* @param getSipRuleRequest
* @return Result of the GetSipRule operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetSipRule
* @see AWS API
* Documentation
*/
@Override
public GetSipRuleResult getSipRule(GetSipRuleRequest request) {
request = beforeClientExecution(request);
return executeGetSipRule(request);
}
@SdkInternalApi
final GetSipRuleResult executeGetSipRule(GetSipRuleRequest getSipRuleRequest) {
ExecutionContext executionContext = createExecutionContext(getSipRuleRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSipRuleRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSipRuleRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSipRule");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(new JsonOperationMetadata()
.withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetSipRuleResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the details of the specified speaker search task.
*
*
* @param getSpeakerSearchTaskRequest
* @return Result of the GetSpeakerSearchTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AmazonChimeSDKVoice.GetSpeakerSearchTask
* @see AWS API Documentation
*/
@Override
public GetSpeakerSearchTaskResult getSpeakerSearchTask(GetSpeakerSearchTaskRequest request) {
request = beforeClientExecution(request);
return executeGetSpeakerSearchTask(request);
}
@SdkInternalApi
final GetSpeakerSearchTaskResult executeGetSpeakerSearchTask(GetSpeakerSearchTaskRequest getSpeakerSearchTaskRequest) {
ExecutionContext executionContext = createExecutionContext(getSpeakerSearchTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetSpeakerSearchTaskRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getSpeakerSearchTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetSpeakerSearchTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetSpeakerSearchTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves details for the specified Amazon Chime SDK Voice Connector, such as timestamps,name, outbound host, and
* encryption requirements.
*
*
* @param getVoiceConnectorRequest
* @return Result of the GetVoiceConnector operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetVoiceConnector
* @see AWS API Documentation
*/
@Override
public GetVoiceConnectorResult getVoiceConnector(GetVoiceConnectorRequest request) {
request = beforeClientExecution(request);
return executeGetVoiceConnector(request);
}
@SdkInternalApi
final GetVoiceConnectorResult executeGetVoiceConnector(GetVoiceConnectorRequest getVoiceConnectorRequest) {
ExecutionContext executionContext = createExecutionContext(getVoiceConnectorRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetVoiceConnectorRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getVoiceConnectorRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetVoiceConnector");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetVoiceConnectorResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the emergency calling configuration details for the specified Voice Connector.
*
*
* @param getVoiceConnectorEmergencyCallingConfigurationRequest
* @return Result of the GetVoiceConnectorEmergencyCallingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetVoiceConnectorEmergencyCallingConfiguration
* @see AWS API Documentation
*/
@Override
public GetVoiceConnectorEmergencyCallingConfigurationResult getVoiceConnectorEmergencyCallingConfiguration(
GetVoiceConnectorEmergencyCallingConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetVoiceConnectorEmergencyCallingConfiguration(request);
}
@SdkInternalApi
final GetVoiceConnectorEmergencyCallingConfigurationResult executeGetVoiceConnectorEmergencyCallingConfiguration(
GetVoiceConnectorEmergencyCallingConfigurationRequest getVoiceConnectorEmergencyCallingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getVoiceConnectorEmergencyCallingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetVoiceConnectorEmergencyCallingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getVoiceConnectorEmergencyCallingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetVoiceConnectorEmergencyCallingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetVoiceConnectorEmergencyCallingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves details for the specified Amazon Chime SDK Voice Connector group, such as timestamps,name, and
* associated VoiceConnectorItems
.
*
*
* @param getVoiceConnectorGroupRequest
* @return Result of the GetVoiceConnectorGroup operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetVoiceConnectorGroup
* @see AWS API Documentation
*/
@Override
public GetVoiceConnectorGroupResult getVoiceConnectorGroup(GetVoiceConnectorGroupRequest request) {
request = beforeClientExecution(request);
return executeGetVoiceConnectorGroup(request);
}
@SdkInternalApi
final GetVoiceConnectorGroupResult executeGetVoiceConnectorGroup(GetVoiceConnectorGroupRequest getVoiceConnectorGroupRequest) {
ExecutionContext executionContext = createExecutionContext(getVoiceConnectorGroupRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetVoiceConnectorGroupRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getVoiceConnectorGroupRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetVoiceConnectorGroup");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetVoiceConnectorGroupResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the logging configuration settings for the specified Voice Connector. Shows whether SIP message logs
* are enabled for sending to Amazon CloudWatch Logs.
*
*
* @param getVoiceConnectorLoggingConfigurationRequest
* @return Result of the GetVoiceConnectorLoggingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetVoiceConnectorLoggingConfiguration
* @see AWS API Documentation
*/
@Override
public GetVoiceConnectorLoggingConfigurationResult getVoiceConnectorLoggingConfiguration(GetVoiceConnectorLoggingConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetVoiceConnectorLoggingConfiguration(request);
}
@SdkInternalApi
final GetVoiceConnectorLoggingConfigurationResult executeGetVoiceConnectorLoggingConfiguration(
GetVoiceConnectorLoggingConfigurationRequest getVoiceConnectorLoggingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getVoiceConnectorLoggingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetVoiceConnectorLoggingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getVoiceConnectorLoggingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetVoiceConnectorLoggingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetVoiceConnectorLoggingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the origination settings for the specified Voice Connector.
*
*
* @param getVoiceConnectorOriginationRequest
* @return Result of the GetVoiceConnectorOrigination operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetVoiceConnectorOrigination
* @see AWS API Documentation
*/
@Override
public GetVoiceConnectorOriginationResult getVoiceConnectorOrigination(GetVoiceConnectorOriginationRequest request) {
request = beforeClientExecution(request);
return executeGetVoiceConnectorOrigination(request);
}
@SdkInternalApi
final GetVoiceConnectorOriginationResult executeGetVoiceConnectorOrigination(GetVoiceConnectorOriginationRequest getVoiceConnectorOriginationRequest) {
ExecutionContext executionContext = createExecutionContext(getVoiceConnectorOriginationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetVoiceConnectorOriginationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getVoiceConnectorOriginationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetVoiceConnectorOrigination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetVoiceConnectorOriginationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the proxy configuration details for the specified Amazon Chime SDK Voice Connector.
*
*
* @param getVoiceConnectorProxyRequest
* @return Result of the GetVoiceConnectorProxy operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetVoiceConnectorProxy
* @see AWS API Documentation
*/
@Override
public GetVoiceConnectorProxyResult getVoiceConnectorProxy(GetVoiceConnectorProxyRequest request) {
request = beforeClientExecution(request);
return executeGetVoiceConnectorProxy(request);
}
@SdkInternalApi
final GetVoiceConnectorProxyResult executeGetVoiceConnectorProxy(GetVoiceConnectorProxyRequest getVoiceConnectorProxyRequest) {
ExecutionContext executionContext = createExecutionContext(getVoiceConnectorProxyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetVoiceConnectorProxyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getVoiceConnectorProxyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetVoiceConnectorProxy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetVoiceConnectorProxyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the streaming configuration details for the specified Amazon Chime SDK Voice Connector. Shows whether
* media streaming is enabled for sending to Amazon Kinesis. It also shows the retention period, in hours, for the
* Amazon Kinesis data.
*
*
* @param getVoiceConnectorStreamingConfigurationRequest
* @return Result of the GetVoiceConnectorStreamingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetVoiceConnectorStreamingConfiguration
* @see AWS API Documentation
*/
@Override
public GetVoiceConnectorStreamingConfigurationResult getVoiceConnectorStreamingConfiguration(GetVoiceConnectorStreamingConfigurationRequest request) {
request = beforeClientExecution(request);
return executeGetVoiceConnectorStreamingConfiguration(request);
}
@SdkInternalApi
final GetVoiceConnectorStreamingConfigurationResult executeGetVoiceConnectorStreamingConfiguration(
GetVoiceConnectorStreamingConfigurationRequest getVoiceConnectorStreamingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(getVoiceConnectorStreamingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetVoiceConnectorStreamingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getVoiceConnectorStreamingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetVoiceConnectorStreamingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetVoiceConnectorStreamingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the termination setting details for the specified Voice Connector.
*
*
* @param getVoiceConnectorTerminationRequest
* @return Result of the GetVoiceConnectorTermination operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetVoiceConnectorTermination
* @see AWS API Documentation
*/
@Override
public GetVoiceConnectorTerminationResult getVoiceConnectorTermination(GetVoiceConnectorTerminationRequest request) {
request = beforeClientExecution(request);
return executeGetVoiceConnectorTermination(request);
}
@SdkInternalApi
final GetVoiceConnectorTerminationResult executeGetVoiceConnectorTermination(GetVoiceConnectorTerminationRequest getVoiceConnectorTerminationRequest) {
ExecutionContext executionContext = createExecutionContext(getVoiceConnectorTerminationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetVoiceConnectorTerminationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getVoiceConnectorTerminationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetVoiceConnectorTermination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetVoiceConnectorTerminationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the last time a SIP OPTIONS
ping was received from your SIP
* infrastructure for the specified Amazon Chime SDK Voice Connector.
*
*
* @param getVoiceConnectorTerminationHealthRequest
* @return Result of the GetVoiceConnectorTerminationHealth operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetVoiceConnectorTerminationHealth
* @see AWS API Documentation
*/
@Override
public GetVoiceConnectorTerminationHealthResult getVoiceConnectorTerminationHealth(GetVoiceConnectorTerminationHealthRequest request) {
request = beforeClientExecution(request);
return executeGetVoiceConnectorTerminationHealth(request);
}
@SdkInternalApi
final GetVoiceConnectorTerminationHealthResult executeGetVoiceConnectorTerminationHealth(
GetVoiceConnectorTerminationHealthRequest getVoiceConnectorTerminationHealthRequest) {
ExecutionContext executionContext = createExecutionContext(getVoiceConnectorTerminationHealthRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetVoiceConnectorTerminationHealthRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getVoiceConnectorTerminationHealthRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetVoiceConnectorTerminationHealth");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetVoiceConnectorTerminationHealthResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the details of the specified voice profile.
*
*
* @param getVoiceProfileRequest
* @return Result of the GetVoiceProfile operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetVoiceProfile
* @see AWS API Documentation
*/
@Override
public GetVoiceProfileResult getVoiceProfile(GetVoiceProfileRequest request) {
request = beforeClientExecution(request);
return executeGetVoiceProfile(request);
}
@SdkInternalApi
final GetVoiceProfileResult executeGetVoiceProfile(GetVoiceProfileRequest getVoiceProfileRequest) {
ExecutionContext executionContext = createExecutionContext(getVoiceProfileRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetVoiceProfileRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getVoiceProfileRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetVoiceProfile");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new GetVoiceProfileResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the details of the specified voice profile domain.
*
*
* @param getVoiceProfileDomainRequest
* @return Result of the GetVoiceProfileDomain operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.GetVoiceProfileDomain
* @see AWS API Documentation
*/
@Override
public GetVoiceProfileDomainResult getVoiceProfileDomain(GetVoiceProfileDomainRequest request) {
request = beforeClientExecution(request);
return executeGetVoiceProfileDomain(request);
}
@SdkInternalApi
final GetVoiceProfileDomainResult executeGetVoiceProfileDomain(GetVoiceProfileDomainRequest getVoiceProfileDomainRequest) {
ExecutionContext executionContext = createExecutionContext(getVoiceProfileDomainRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetVoiceProfileDomainRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(getVoiceProfileDomainRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetVoiceProfileDomain");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetVoiceProfileDomainResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves the details of a voice tone analysis task.
*
*
* @param getVoiceToneAnalysisTaskRequest
* @return Result of the GetVoiceToneAnalysisTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @sample AmazonChimeSDKVoice.GetVoiceToneAnalysisTask
* @see AWS API Documentation
*/
@Override
public GetVoiceToneAnalysisTaskResult getVoiceToneAnalysisTask(GetVoiceToneAnalysisTaskRequest request) {
request = beforeClientExecution(request);
return executeGetVoiceToneAnalysisTask(request);
}
@SdkInternalApi
final GetVoiceToneAnalysisTaskResult executeGetVoiceToneAnalysisTask(GetVoiceToneAnalysisTaskRequest getVoiceToneAnalysisTaskRequest) {
ExecutionContext executionContext = createExecutionContext(getVoiceToneAnalysisTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetVoiceToneAnalysisTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(getVoiceToneAnalysisTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetVoiceToneAnalysisTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new GetVoiceToneAnalysisTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the available AWS Regions in which you can create an Amazon Chime SDK Voice Connector.
*
*
* @param listAvailableVoiceConnectorRegionsRequest
* @return Result of the ListAvailableVoiceConnectorRegions operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.ListAvailableVoiceConnectorRegions
* @see AWS API Documentation
*/
@Override
public ListAvailableVoiceConnectorRegionsResult listAvailableVoiceConnectorRegions(ListAvailableVoiceConnectorRegionsRequest request) {
request = beforeClientExecution(request);
return executeListAvailableVoiceConnectorRegions(request);
}
@SdkInternalApi
final ListAvailableVoiceConnectorRegionsResult executeListAvailableVoiceConnectorRegions(
ListAvailableVoiceConnectorRegionsRequest listAvailableVoiceConnectorRegionsRequest) {
ExecutionContext executionContext = createExecutionContext(listAvailableVoiceConnectorRegionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListAvailableVoiceConnectorRegionsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listAvailableVoiceConnectorRegionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListAvailableVoiceConnectorRegions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListAvailableVoiceConnectorRegionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the phone numbers for an administrator's Amazon Chime SDK account.
*
*
* @param listPhoneNumberOrdersRequest
* @return Result of the ListPhoneNumberOrders operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.ListPhoneNumberOrders
* @see AWS API Documentation
*/
@Override
public ListPhoneNumberOrdersResult listPhoneNumberOrders(ListPhoneNumberOrdersRequest request) {
request = beforeClientExecution(request);
return executeListPhoneNumberOrders(request);
}
@SdkInternalApi
final ListPhoneNumberOrdersResult executeListPhoneNumberOrders(ListPhoneNumberOrdersRequest listPhoneNumberOrdersRequest) {
ExecutionContext executionContext = createExecutionContext(listPhoneNumberOrdersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPhoneNumberOrdersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listPhoneNumberOrdersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPhoneNumberOrders");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListPhoneNumberOrdersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the phone numbers for the specified Amazon Chime SDK account, Amazon Chime SDK user, Amazon Chime SDK Voice
* Connector, or Amazon Chime SDK Voice Connector group.
*
*
* @param listPhoneNumbersRequest
* @return Result of the ListPhoneNumbers operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.ListPhoneNumbers
* @see AWS API Documentation
*/
@Override
public ListPhoneNumbersResult listPhoneNumbers(ListPhoneNumbersRequest request) {
request = beforeClientExecution(request);
return executeListPhoneNumbers(request);
}
@SdkInternalApi
final ListPhoneNumbersResult executeListPhoneNumbers(ListPhoneNumbersRequest listPhoneNumbersRequest) {
ExecutionContext executionContext = createExecutionContext(listPhoneNumbersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListPhoneNumbersRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listPhoneNumbersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListPhoneNumbers");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListPhoneNumbersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the proxy sessions for the specified Amazon Chime SDK Voice Connector.
*
*
* @param listProxySessionsRequest
* @return Result of the ListProxySessions operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.ListProxySessions
* @see AWS API Documentation
*/
@Override
public ListProxySessionsResult listProxySessions(ListProxySessionsRequest request) {
request = beforeClientExecution(request);
return executeListProxySessions(request);
}
@SdkInternalApi
final ListProxySessionsResult executeListProxySessions(ListProxySessionsRequest listProxySessionsRequest) {
ExecutionContext executionContext = createExecutionContext(listProxySessionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListProxySessionsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listProxySessionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListProxySessions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListProxySessionsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the SIP media applications under the administrator's AWS account.
*
*
* @param listSipMediaApplicationsRequest
* @return Result of the ListSipMediaApplications operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.ListSipMediaApplications
* @see AWS API Documentation
*/
@Override
public ListSipMediaApplicationsResult listSipMediaApplications(ListSipMediaApplicationsRequest request) {
request = beforeClientExecution(request);
return executeListSipMediaApplications(request);
}
@SdkInternalApi
final ListSipMediaApplicationsResult executeListSipMediaApplications(ListSipMediaApplicationsRequest listSipMediaApplicationsRequest) {
ExecutionContext executionContext = createExecutionContext(listSipMediaApplicationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSipMediaApplicationsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listSipMediaApplicationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSipMediaApplications");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListSipMediaApplicationsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the SIP rules under the administrator's AWS account.
*
*
* @param listSipRulesRequest
* @return Result of the ListSipRules operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.ListSipRules
* @see AWS
* API Documentation
*/
@Override
public ListSipRulesResult listSipRules(ListSipRulesRequest request) {
request = beforeClientExecution(request);
return executeListSipRules(request);
}
@SdkInternalApi
final ListSipRulesResult executeListSipRules(ListSipRulesRequest listSipRulesRequest) {
ExecutionContext executionContext = createExecutionContext(listSipRulesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSipRulesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listSipRulesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSipRules");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListSipRulesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the countries that you can order phone numbers from.
*
*
* @param listSupportedPhoneNumberCountriesRequest
* @return Result of the ListSupportedPhoneNumberCountries operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.ListSupportedPhoneNumberCountries
* @see AWS API Documentation
*/
@Override
public ListSupportedPhoneNumberCountriesResult listSupportedPhoneNumberCountries(ListSupportedPhoneNumberCountriesRequest request) {
request = beforeClientExecution(request);
return executeListSupportedPhoneNumberCountries(request);
}
@SdkInternalApi
final ListSupportedPhoneNumberCountriesResult executeListSupportedPhoneNumberCountries(
ListSupportedPhoneNumberCountriesRequest listSupportedPhoneNumberCountriesRequest) {
ExecutionContext executionContext = createExecutionContext(listSupportedPhoneNumberCountriesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListSupportedPhoneNumberCountriesRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listSupportedPhoneNumberCountriesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListSupportedPhoneNumberCountries");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListSupportedPhoneNumberCountriesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a list of the tags in a given resource.
*
*
* @param listTagsForResourceRequest
* @return Result of the ListTagsForResource operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.ListTagsForResource
* @see AWS API Documentation
*/
@Override
public ListTagsForResourceResult listTagsForResource(ListTagsForResourceRequest request) {
request = beforeClientExecution(request);
return executeListTagsForResource(request);
}
@SdkInternalApi
final ListTagsForResourceResult executeListTagsForResource(ListTagsForResourceRequest listTagsForResourceRequest) {
ExecutionContext executionContext = createExecutionContext(listTagsForResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTagsForResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listTagsForResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTagsForResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListTagsForResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the Amazon Chime SDK Voice Connector groups in the administrator's AWS account.
*
*
* @param listVoiceConnectorGroupsRequest
* @return Result of the ListVoiceConnectorGroups operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.ListVoiceConnectorGroups
* @see AWS API Documentation
*/
@Override
public ListVoiceConnectorGroupsResult listVoiceConnectorGroups(ListVoiceConnectorGroupsRequest request) {
request = beforeClientExecution(request);
return executeListVoiceConnectorGroups(request);
}
@SdkInternalApi
final ListVoiceConnectorGroupsResult executeListVoiceConnectorGroups(ListVoiceConnectorGroupsRequest listVoiceConnectorGroupsRequest) {
ExecutionContext executionContext = createExecutionContext(listVoiceConnectorGroupsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVoiceConnectorGroupsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listVoiceConnectorGroupsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListVoiceConnectorGroups");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListVoiceConnectorGroupsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the SIP credentials for the specified Amazon Chime SDK Voice Connector.
*
*
* @param listVoiceConnectorTerminationCredentialsRequest
* @return Result of the ListVoiceConnectorTerminationCredentials operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.ListVoiceConnectorTerminationCredentials
* @see AWS API Documentation
*/
@Override
public ListVoiceConnectorTerminationCredentialsResult listVoiceConnectorTerminationCredentials(ListVoiceConnectorTerminationCredentialsRequest request) {
request = beforeClientExecution(request);
return executeListVoiceConnectorTerminationCredentials(request);
}
@SdkInternalApi
final ListVoiceConnectorTerminationCredentialsResult executeListVoiceConnectorTerminationCredentials(
ListVoiceConnectorTerminationCredentialsRequest listVoiceConnectorTerminationCredentialsRequest) {
ExecutionContext executionContext = createExecutionContext(listVoiceConnectorTerminationCredentialsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVoiceConnectorTerminationCredentialsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listVoiceConnectorTerminationCredentialsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListVoiceConnectorTerminationCredentials");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListVoiceConnectorTerminationCredentialsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the Amazon Chime SDK Voice Connectors in the administrators AWS account.
*
*
* @param listVoiceConnectorsRequest
* @return Result of the ListVoiceConnectors operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.ListVoiceConnectors
* @see AWS API Documentation
*/
@Override
public ListVoiceConnectorsResult listVoiceConnectors(ListVoiceConnectorsRequest request) {
request = beforeClientExecution(request);
return executeListVoiceConnectors(request);
}
@SdkInternalApi
final ListVoiceConnectorsResult executeListVoiceConnectors(ListVoiceConnectorsRequest listVoiceConnectorsRequest) {
ExecutionContext executionContext = createExecutionContext(listVoiceConnectorsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVoiceConnectorsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listVoiceConnectorsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListVoiceConnectors");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListVoiceConnectorsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the specified voice profile domains in the administrator's AWS account.
*
*
* @param listVoiceProfileDomainsRequest
* @return Result of the ListVoiceProfileDomains operation returned by the service.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.ListVoiceProfileDomains
* @see AWS API Documentation
*/
@Override
public ListVoiceProfileDomainsResult listVoiceProfileDomains(ListVoiceProfileDomainsRequest request) {
request = beforeClientExecution(request);
return executeListVoiceProfileDomains(request);
}
@SdkInternalApi
final ListVoiceProfileDomainsResult executeListVoiceProfileDomains(ListVoiceProfileDomainsRequest listVoiceProfileDomainsRequest) {
ExecutionContext executionContext = createExecutionContext(listVoiceProfileDomainsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVoiceProfileDomainsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(listVoiceProfileDomainsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListVoiceProfileDomains");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new ListVoiceProfileDomainsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists the voice profiles in a voice profile domain.
*
*
* @param listVoiceProfilesRequest
* @return Result of the ListVoiceProfiles operation returned by the service.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.ListVoiceProfiles
* @see AWS API Documentation
*/
@Override
public ListVoiceProfilesResult listVoiceProfiles(ListVoiceProfilesRequest request) {
request = beforeClientExecution(request);
return executeListVoiceProfiles(request);
}
@SdkInternalApi
final ListVoiceProfilesResult executeListVoiceProfiles(ListVoiceProfilesRequest listVoiceProfilesRequest) {
ExecutionContext executionContext = createExecutionContext(listVoiceProfilesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListVoiceProfilesRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(listVoiceProfilesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListVoiceProfiles");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new ListVoiceProfilesResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the Alexa Skill configuration for the SIP media application.
*
*
* @param putSipMediaApplicationAlexaSkillConfigurationRequest
* @return Result of the PutSipMediaApplicationAlexaSkillConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.PutSipMediaApplicationAlexaSkillConfiguration
* @see AWS API Documentation
*/
@Override
public PutSipMediaApplicationAlexaSkillConfigurationResult putSipMediaApplicationAlexaSkillConfiguration(
PutSipMediaApplicationAlexaSkillConfigurationRequest request) {
request = beforeClientExecution(request);
return executePutSipMediaApplicationAlexaSkillConfiguration(request);
}
@SdkInternalApi
final PutSipMediaApplicationAlexaSkillConfigurationResult executePutSipMediaApplicationAlexaSkillConfiguration(
PutSipMediaApplicationAlexaSkillConfigurationRequest putSipMediaApplicationAlexaSkillConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(putSipMediaApplicationAlexaSkillConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutSipMediaApplicationAlexaSkillConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putSipMediaApplicationAlexaSkillConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutSipMediaApplicationAlexaSkillConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutSipMediaApplicationAlexaSkillConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the logging configuration for the specified SIP media application.
*
*
* @param putSipMediaApplicationLoggingConfigurationRequest
* @return Result of the PutSipMediaApplicationLoggingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.PutSipMediaApplicationLoggingConfiguration
* @see AWS API Documentation
*/
@Override
public PutSipMediaApplicationLoggingConfigurationResult putSipMediaApplicationLoggingConfiguration(PutSipMediaApplicationLoggingConfigurationRequest request) {
request = beforeClientExecution(request);
return executePutSipMediaApplicationLoggingConfiguration(request);
}
@SdkInternalApi
final PutSipMediaApplicationLoggingConfigurationResult executePutSipMediaApplicationLoggingConfiguration(
PutSipMediaApplicationLoggingConfigurationRequest putSipMediaApplicationLoggingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(putSipMediaApplicationLoggingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutSipMediaApplicationLoggingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putSipMediaApplicationLoggingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutSipMediaApplicationLoggingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutSipMediaApplicationLoggingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a Voice Connector's emergency calling configuration.
*
*
* @param putVoiceConnectorEmergencyCallingConfigurationRequest
* @return Result of the PutVoiceConnectorEmergencyCallingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.PutVoiceConnectorEmergencyCallingConfiguration
* @see AWS API Documentation
*/
@Override
public PutVoiceConnectorEmergencyCallingConfigurationResult putVoiceConnectorEmergencyCallingConfiguration(
PutVoiceConnectorEmergencyCallingConfigurationRequest request) {
request = beforeClientExecution(request);
return executePutVoiceConnectorEmergencyCallingConfiguration(request);
}
@SdkInternalApi
final PutVoiceConnectorEmergencyCallingConfigurationResult executePutVoiceConnectorEmergencyCallingConfiguration(
PutVoiceConnectorEmergencyCallingConfigurationRequest putVoiceConnectorEmergencyCallingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(putVoiceConnectorEmergencyCallingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutVoiceConnectorEmergencyCallingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putVoiceConnectorEmergencyCallingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutVoiceConnectorEmergencyCallingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutVoiceConnectorEmergencyCallingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a Voice Connector's logging configuration.
*
*
* @param putVoiceConnectorLoggingConfigurationRequest
* @return Result of the PutVoiceConnectorLoggingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.PutVoiceConnectorLoggingConfiguration
* @see AWS API Documentation
*/
@Override
public PutVoiceConnectorLoggingConfigurationResult putVoiceConnectorLoggingConfiguration(PutVoiceConnectorLoggingConfigurationRequest request) {
request = beforeClientExecution(request);
return executePutVoiceConnectorLoggingConfiguration(request);
}
@SdkInternalApi
final PutVoiceConnectorLoggingConfigurationResult executePutVoiceConnectorLoggingConfiguration(
PutVoiceConnectorLoggingConfigurationRequest putVoiceConnectorLoggingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(putVoiceConnectorLoggingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutVoiceConnectorLoggingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putVoiceConnectorLoggingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutVoiceConnectorLoggingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutVoiceConnectorLoggingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a Voice Connector's origination settings.
*
*
* @param putVoiceConnectorOriginationRequest
* @return Result of the PutVoiceConnectorOrigination operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.PutVoiceConnectorOrigination
* @see AWS API Documentation
*/
@Override
public PutVoiceConnectorOriginationResult putVoiceConnectorOrigination(PutVoiceConnectorOriginationRequest request) {
request = beforeClientExecution(request);
return executePutVoiceConnectorOrigination(request);
}
@SdkInternalApi
final PutVoiceConnectorOriginationResult executePutVoiceConnectorOrigination(PutVoiceConnectorOriginationRequest putVoiceConnectorOriginationRequest) {
ExecutionContext executionContext = createExecutionContext(putVoiceConnectorOriginationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutVoiceConnectorOriginationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putVoiceConnectorOriginationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutVoiceConnectorOrigination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutVoiceConnectorOriginationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Puts the specified proxy configuration to the specified Amazon Chime SDK Voice Connector.
*
*
* @param putVoiceConnectorProxyRequest
* @return Result of the PutVoiceConnectorProxy operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.PutVoiceConnectorProxy
* @see AWS API Documentation
*/
@Override
public PutVoiceConnectorProxyResult putVoiceConnectorProxy(PutVoiceConnectorProxyRequest request) {
request = beforeClientExecution(request);
return executePutVoiceConnectorProxy(request);
}
@SdkInternalApi
final PutVoiceConnectorProxyResult executePutVoiceConnectorProxy(PutVoiceConnectorProxyRequest putVoiceConnectorProxyRequest) {
ExecutionContext executionContext = createExecutionContext(putVoiceConnectorProxyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutVoiceConnectorProxyRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(putVoiceConnectorProxyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutVoiceConnectorProxy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutVoiceConnectorProxyResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a Voice Connector's streaming configuration settings.
*
*
* @param putVoiceConnectorStreamingConfigurationRequest
* @return Result of the PutVoiceConnectorStreamingConfiguration operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.PutVoiceConnectorStreamingConfiguration
* @see AWS API Documentation
*/
@Override
public PutVoiceConnectorStreamingConfigurationResult putVoiceConnectorStreamingConfiguration(PutVoiceConnectorStreamingConfigurationRequest request) {
request = beforeClientExecution(request);
return executePutVoiceConnectorStreamingConfiguration(request);
}
@SdkInternalApi
final PutVoiceConnectorStreamingConfigurationResult executePutVoiceConnectorStreamingConfiguration(
PutVoiceConnectorStreamingConfigurationRequest putVoiceConnectorStreamingConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(putVoiceConnectorStreamingConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutVoiceConnectorStreamingConfigurationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putVoiceConnectorStreamingConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutVoiceConnectorStreamingConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutVoiceConnectorStreamingConfigurationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a Voice Connector's termination settings.
*
*
* @param putVoiceConnectorTerminationRequest
* @return Result of the PutVoiceConnectorTermination operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.PutVoiceConnectorTermination
* @see AWS API Documentation
*/
@Override
public PutVoiceConnectorTerminationResult putVoiceConnectorTermination(PutVoiceConnectorTerminationRequest request) {
request = beforeClientExecution(request);
return executePutVoiceConnectorTermination(request);
}
@SdkInternalApi
final PutVoiceConnectorTerminationResult executePutVoiceConnectorTermination(PutVoiceConnectorTerminationRequest putVoiceConnectorTerminationRequest) {
ExecutionContext executionContext = createExecutionContext(putVoiceConnectorTerminationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutVoiceConnectorTerminationRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putVoiceConnectorTerminationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutVoiceConnectorTermination");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutVoiceConnectorTerminationResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a Voice Connector's termination credentials.
*
*
* @param putVoiceConnectorTerminationCredentialsRequest
* @return Result of the PutVoiceConnectorTerminationCredentials operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.PutVoiceConnectorTerminationCredentials
* @see AWS API Documentation
*/
@Override
public PutVoiceConnectorTerminationCredentialsResult putVoiceConnectorTerminationCredentials(PutVoiceConnectorTerminationCredentialsRequest request) {
request = beforeClientExecution(request);
return executePutVoiceConnectorTerminationCredentials(request);
}
@SdkInternalApi
final PutVoiceConnectorTerminationCredentialsResult executePutVoiceConnectorTerminationCredentials(
PutVoiceConnectorTerminationCredentialsRequest putVoiceConnectorTerminationCredentialsRequest) {
ExecutionContext executionContext = createExecutionContext(putVoiceConnectorTerminationCredentialsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PutVoiceConnectorTerminationCredentialsRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(putVoiceConnectorTerminationCredentialsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PutVoiceConnectorTerminationCredentials");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new PutVoiceConnectorTerminationCredentialsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Restores a deleted phone number.
*
*
* @param restorePhoneNumberRequest
* @return Result of the RestorePhoneNumber operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.RestorePhoneNumber
* @see AWS API Documentation
*/
@Override
public RestorePhoneNumberResult restorePhoneNumber(RestorePhoneNumberRequest request) {
request = beforeClientExecution(request);
return executeRestorePhoneNumber(request);
}
@SdkInternalApi
final RestorePhoneNumberResult executeRestorePhoneNumber(RestorePhoneNumberRequest restorePhoneNumberRequest) {
ExecutionContext executionContext = createExecutionContext(restorePhoneNumberRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RestorePhoneNumberRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(restorePhoneNumberRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RestorePhoneNumber");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new RestorePhoneNumberResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Searches the provisioned phone numbers in an organization.
*
*
* @param searchAvailablePhoneNumbersRequest
* @return Result of the SearchAvailablePhoneNumbers operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.SearchAvailablePhoneNumbers
* @see AWS API Documentation
*/
@Override
public SearchAvailablePhoneNumbersResult searchAvailablePhoneNumbers(SearchAvailablePhoneNumbersRequest request) {
request = beforeClientExecution(request);
return executeSearchAvailablePhoneNumbers(request);
}
@SdkInternalApi
final SearchAvailablePhoneNumbersResult executeSearchAvailablePhoneNumbers(SearchAvailablePhoneNumbersRequest searchAvailablePhoneNumbersRequest) {
ExecutionContext executionContext = createExecutionContext(searchAvailablePhoneNumbersRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SearchAvailablePhoneNumbersRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(searchAvailablePhoneNumbersRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SearchAvailablePhoneNumbers");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new SearchAvailablePhoneNumbersResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts a speaker search task.
*
*
*
* Before starting any speaker search tasks, you must provide all notices and obtain all consents from the speaker
* as required under applicable privacy and biometrics laws, and as required under the AWS service terms for the Amazon Chime SDK.
*
*
*
* @param startSpeakerSearchTaskRequest
* @return Result of the StartSpeakerSearchTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws GoneException
* Access to the target resource is no longer available at the origin server. This condition is likely to be
* permanent.
* @throws UnprocessableEntityException
* A well-formed request couldn't be followed due to semantic errors.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.StartSpeakerSearchTask
* @see AWS API Documentation
*/
@Override
public StartSpeakerSearchTaskResult startSpeakerSearchTask(StartSpeakerSearchTaskRequest request) {
request = beforeClientExecution(request);
return executeStartSpeakerSearchTask(request);
}
@SdkInternalApi
final StartSpeakerSearchTaskResult executeStartSpeakerSearchTask(StartSpeakerSearchTaskRequest startSpeakerSearchTaskRequest) {
ExecutionContext executionContext = createExecutionContext(startSpeakerSearchTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartSpeakerSearchTaskRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(startSpeakerSearchTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartSpeakerSearchTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StartSpeakerSearchTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Starts a voice tone analysis task. For more information about voice tone analysis, see Using Amazon Chime SDK voice
* analytics in the Amazon Chime SDK Developer Guide.
*
*
*
* Before starting any voice tone analysis tasks, you must provide all notices and obtain all consents from the
* speaker as required under applicable privacy and biometrics laws, and as required under the AWS service terms for the Amazon Chime SDK.
*
*
*
* @param startVoiceToneAnalysisTaskRequest
* @return Result of the StartVoiceToneAnalysisTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws GoneException
* Access to the target resource is no longer available at the origin server. This condition is likely to be
* permanent.
* @throws UnprocessableEntityException
* A well-formed request couldn't be followed due to semantic errors.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.StartVoiceToneAnalysisTask
* @see AWS API Documentation
*/
@Override
public StartVoiceToneAnalysisTaskResult startVoiceToneAnalysisTask(StartVoiceToneAnalysisTaskRequest request) {
request = beforeClientExecution(request);
return executeStartVoiceToneAnalysisTask(request);
}
@SdkInternalApi
final StartVoiceToneAnalysisTaskResult executeStartVoiceToneAnalysisTask(StartVoiceToneAnalysisTaskRequest startVoiceToneAnalysisTaskRequest) {
ExecutionContext executionContext = createExecutionContext(startVoiceToneAnalysisTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StartVoiceToneAnalysisTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(startVoiceToneAnalysisTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StartVoiceToneAnalysisTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StartVoiceToneAnalysisTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops a speaker search task.
*
*
* @param stopSpeakerSearchTaskRequest
* @return Result of the StopSpeakerSearchTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws UnprocessableEntityException
* A well-formed request couldn't be followed due to semantic errors.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.StopSpeakerSearchTask
* @see AWS API Documentation
*/
@Override
public StopSpeakerSearchTaskResult stopSpeakerSearchTask(StopSpeakerSearchTaskRequest request) {
request = beforeClientExecution(request);
return executeStopSpeakerSearchTask(request);
}
@SdkInternalApi
final StopSpeakerSearchTaskResult executeStopSpeakerSearchTask(StopSpeakerSearchTaskRequest stopSpeakerSearchTaskRequest) {
ExecutionContext executionContext = createExecutionContext(stopSpeakerSearchTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopSpeakerSearchTaskRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(stopSpeakerSearchTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopSpeakerSearchTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory
.createResponseHandler(new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StopSpeakerSearchTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops a voice tone analysis task.
*
*
* @param stopVoiceToneAnalysisTaskRequest
* @return Result of the StopVoiceToneAnalysisTask operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws AccessDeniedException
* You don't have the permissions needed to run this action.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws UnprocessableEntityException
* A well-formed request couldn't be followed due to semantic errors.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.StopVoiceToneAnalysisTask
* @see AWS API Documentation
*/
@Override
public StopVoiceToneAnalysisTaskResult stopVoiceToneAnalysisTask(StopVoiceToneAnalysisTaskRequest request) {
request = beforeClientExecution(request);
return executeStopVoiceToneAnalysisTask(request);
}
@SdkInternalApi
final StopVoiceToneAnalysisTaskResult executeStopVoiceToneAnalysisTask(StopVoiceToneAnalysisTaskRequest stopVoiceToneAnalysisTaskRequest) {
ExecutionContext executionContext = createExecutionContext(stopVoiceToneAnalysisTaskRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopVoiceToneAnalysisTaskRequestProtocolMarshaller(protocolFactory).marshall(super
.beforeMarshalling(stopVoiceToneAnalysisTaskRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopVoiceToneAnalysisTask");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false),
new StopVoiceToneAnalysisTaskResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Adds a tag to the specified resource.
*
*
* @param tagResourceRequest
* @return Result of the TagResource operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ResourceLimitExceededException
* The request exceeds the resource limit.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.TagResource
* @see AWS
* API Documentation
*/
@Override
public TagResourceResult tagResource(TagResourceRequest request) {
request = beforeClientExecution(request);
return executeTagResource(request);
}
@SdkInternalApi
final TagResourceResult executeTagResource(TagResourceRequest tagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(tagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(tagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new TagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Removes tags from a resource.
*
*
* @param untagResourceRequest
* @return Result of the UntagResource operation returned by the service.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.UntagResource
* @see AWS
* API Documentation
*/
@Override
public UntagResourceResult untagResource(UntagResourceRequest request) {
request = beforeClientExecution(request);
return executeUntagResource(request);
}
@SdkInternalApi
final UntagResourceResult executeUntagResource(UntagResourceRequest untagResourceRequest) {
ExecutionContext executionContext = createExecutionContext(untagResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UntagResourceRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(untagResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UntagResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UntagResourceResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates global settings for the Amazon Chime SDK Voice Connectors in an AWS account.
*
*
* @param updateGlobalSettingsRequest
* @return Result of the UpdateGlobalSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.UpdateGlobalSettings
* @see AWS API Documentation
*/
@Override
public UpdateGlobalSettingsResult updateGlobalSettings(UpdateGlobalSettingsRequest request) {
request = beforeClientExecution(request);
return executeUpdateGlobalSettings(request);
}
@SdkInternalApi
final UpdateGlobalSettingsResult executeUpdateGlobalSettings(UpdateGlobalSettingsRequest updateGlobalSettingsRequest) {
ExecutionContext executionContext = createExecutionContext(updateGlobalSettingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateGlobalSettingsRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updateGlobalSettingsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateGlobalSettings");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdateGlobalSettingsResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates phone number details, such as product type or calling name, for the specified phone number ID. You can
* update one phone number detail at a time. For example, you can update either the product type or the calling name
* in one action.
*
*
* For numbers outside the U.S., you must use the Amazon Chime SDK SIP Media Application Dial-In product type.
*
*
* Updates to outbound calling names can take 72 hours to complete. Pending updates to outbound calling names must
* be complete before you can request another update.
*
*
* @param updatePhoneNumberRequest
* @return Result of the UpdatePhoneNumber operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws NotFoundException
* The requested resource couldn't be found.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ConflictException
* Multiple instances of the same request were made simultaneously.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.UpdatePhoneNumber
* @see AWS API Documentation
*/
@Override
public UpdatePhoneNumberResult updatePhoneNumber(UpdatePhoneNumberRequest request) {
request = beforeClientExecution(request);
return executeUpdatePhoneNumber(request);
}
@SdkInternalApi
final UpdatePhoneNumberResult executeUpdatePhoneNumber(UpdatePhoneNumberRequest updatePhoneNumberRequest) {
ExecutionContext executionContext = createExecutionContext(updatePhoneNumberRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdatePhoneNumberRequestProtocolMarshaller(protocolFactory).marshall(super.beforeMarshalling(updatePhoneNumberRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "Chime SDK Voice");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdatePhoneNumber");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
HttpResponseHandler> responseHandler = protocolFactory.createResponseHandler(
new JsonOperationMetadata().withPayloadJson(true).withHasStreamingSuccessResponse(false), new UpdatePhoneNumberResultJsonUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the phone number settings for the administrator's AWS account, such as the default outbound calling name.
* You can update the default outbound calling name once every seven days. Outbound calling names can take up to 72
* hours to update.
*
*
* @param updatePhoneNumberSettingsRequest
* @return Result of the UpdatePhoneNumberSettings operation returned by the service.
* @throws UnauthorizedClientException
* The client isn't authorized to request a resource.
* @throws ForbiddenException
* The client is permanently forbidden from making the request.
* @throws BadRequestException
* The input parameters don't match the service's restrictions.
* @throws ThrottledClientException
* The number of customer requests exceeds the request rate limit.
* @throws ServiceUnavailableException
* The service is currently unavailable.
* @throws ServiceFailureException
* The service encountered an unexpected error.
* @sample AmazonChimeSDKVoice.UpdatePhoneNumberSettings
* @see AWS API Documentation
*/
@Override
public UpdatePhoneNumberSettingsResult updatePhoneNumberSettings(UpdatePhoneNumberSettingsRequest request) {
request = beforeClientExecution(request);
return executeUpdatePhoneNumberSettings(request);
}
@SdkInternalApi
final UpdatePhoneNumberSettingsResult executeUpdatePhoneNumberSettings(UpdatePhoneNumberSettingsRequest updatePhoneNumberSettingsRequest) {
ExecutionContext executionContext = createExecutionContext(updatePhoneNumberSettingsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response