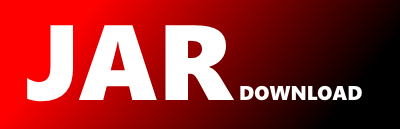
com.amazonaws.services.cloud9.AWSCloud9Async Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloud9 Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloud9;
import javax.annotation.Generated;
import com.amazonaws.services.cloud9.model.*;
/**
* Interface for accessing AWS Cloud9 asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.cloud9.AbstractAWSCloud9Async} instead.
*
*
* Cloud9
*
* Cloud9 is a collection of tools that you can use to code, build, run, test, debug, and release software in the cloud.
*
*
* For more information about Cloud9, see the Cloud9 User
* Guide.
*
*
* Cloud9 supports these operations:
*
*
* -
*
* CreateEnvironmentEC2
: Creates an Cloud9 development environment, launches an Amazon EC2 instance, and
* then connects from the instance to the environment.
*
*
* -
*
* CreateEnvironmentMembership
: Adds an environment member to an environment.
*
*
* -
*
* DeleteEnvironment
: Deletes an environment. If an Amazon EC2 instance is connected to the environment,
* also terminates the instance.
*
*
* -
*
* DeleteEnvironmentMembership
: Deletes an environment member from an environment.
*
*
* -
*
* DescribeEnvironmentMemberships
: Gets information about environment members for an environment.
*
*
* -
*
* DescribeEnvironments
: Gets information about environments.
*
*
* -
*
* DescribeEnvironmentStatus
: Gets status information for an environment.
*
*
* -
*
* ListEnvironments
: Gets a list of environment identifiers.
*
*
* -
*
* ListTagsForResource
: Gets the tags for an environment.
*
*
* -
*
* TagResource
: Adds tags to an environment.
*
*
* -
*
* UntagResource
: Removes tags from an environment.
*
*
* -
*
* UpdateEnvironment
: Changes the settings of an existing environment.
*
*
* -
*
* UpdateEnvironmentMembership
: Changes the settings of an existing environment member for an environment.
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AWSCloud9Async extends AWSCloud9 {
/**
*
* Creates an Cloud9 development environment, launches an Amazon Elastic Compute Cloud (Amazon EC2) instance, and
* then connects from the instance to the environment.
*
*
* @param createEnvironmentEC2Request
* @return A Java Future containing the result of the CreateEnvironmentEC2 operation returned by the service.
* @sample AWSCloud9Async.CreateEnvironmentEC2
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEnvironmentEC2Async(CreateEnvironmentEC2Request createEnvironmentEC2Request);
/**
*
* Creates an Cloud9 development environment, launches an Amazon Elastic Compute Cloud (Amazon EC2) instance, and
* then connects from the instance to the environment.
*
*
* @param createEnvironmentEC2Request
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEnvironmentEC2 operation returned by the service.
* @sample AWSCloud9AsyncHandler.CreateEnvironmentEC2
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createEnvironmentEC2Async(CreateEnvironmentEC2Request createEnvironmentEC2Request,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds an environment member to an Cloud9 development environment.
*
*
* @param createEnvironmentMembershipRequest
* @return A Java Future containing the result of the CreateEnvironmentMembership operation returned by the service.
* @sample AWSCloud9Async.CreateEnvironmentMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future createEnvironmentMembershipAsync(
CreateEnvironmentMembershipRequest createEnvironmentMembershipRequest);
/**
*
* Adds an environment member to an Cloud9 development environment.
*
*
* @param createEnvironmentMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateEnvironmentMembership operation returned by the service.
* @sample AWSCloud9AsyncHandler.CreateEnvironmentMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future createEnvironmentMembershipAsync(
CreateEnvironmentMembershipRequest createEnvironmentMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an Cloud9 development environment. If an Amazon EC2 instance is connected to the environment, also
* terminates the instance.
*
*
* @param deleteEnvironmentRequest
* @return A Java Future containing the result of the DeleteEnvironment operation returned by the service.
* @sample AWSCloud9Async.DeleteEnvironment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteEnvironmentAsync(DeleteEnvironmentRequest deleteEnvironmentRequest);
/**
*
* Deletes an Cloud9 development environment. If an Amazon EC2 instance is connected to the environment, also
* terminates the instance.
*
*
* @param deleteEnvironmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEnvironment operation returned by the service.
* @sample AWSCloud9AsyncHandler.DeleteEnvironment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteEnvironmentAsync(DeleteEnvironmentRequest deleteEnvironmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes an environment member from a development environment.
*
*
* @param deleteEnvironmentMembershipRequest
* @return A Java Future containing the result of the DeleteEnvironmentMembership operation returned by the service.
* @sample AWSCloud9Async.DeleteEnvironmentMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEnvironmentMembershipAsync(
DeleteEnvironmentMembershipRequest deleteEnvironmentMembershipRequest);
/**
*
* Deletes an environment member from a development environment.
*
*
* @param deleteEnvironmentMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteEnvironmentMembership operation returned by the service.
* @sample AWSCloud9AsyncHandler.DeleteEnvironmentMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteEnvironmentMembershipAsync(
DeleteEnvironmentMembershipRequest deleteEnvironmentMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about environment members for an Cloud9 development environment.
*
*
* @param describeEnvironmentMembershipsRequest
* @return A Java Future containing the result of the DescribeEnvironmentMemberships operation returned by the
* service.
* @sample AWSCloud9Async.DescribeEnvironmentMemberships
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEnvironmentMembershipsAsync(
DescribeEnvironmentMembershipsRequest describeEnvironmentMembershipsRequest);
/**
*
* Gets information about environment members for an Cloud9 development environment.
*
*
* @param describeEnvironmentMembershipsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEnvironmentMemberships operation returned by the
* service.
* @sample AWSCloud9AsyncHandler.DescribeEnvironmentMemberships
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEnvironmentMembershipsAsync(
DescribeEnvironmentMembershipsRequest describeEnvironmentMembershipsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets status information for an Cloud9 development environment.
*
*
* @param describeEnvironmentStatusRequest
* @return A Java Future containing the result of the DescribeEnvironmentStatus operation returned by the service.
* @sample AWSCloud9Async.DescribeEnvironmentStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEnvironmentStatusAsync(
DescribeEnvironmentStatusRequest describeEnvironmentStatusRequest);
/**
*
* Gets status information for an Cloud9 development environment.
*
*
* @param describeEnvironmentStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEnvironmentStatus operation returned by the service.
* @sample AWSCloud9AsyncHandler.DescribeEnvironmentStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeEnvironmentStatusAsync(
DescribeEnvironmentStatusRequest describeEnvironmentStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets information about Cloud9 development environments.
*
*
* @param describeEnvironmentsRequest
* @return A Java Future containing the result of the DescribeEnvironments operation returned by the service.
* @sample AWSCloud9Async.DescribeEnvironments
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEnvironmentsAsync(DescribeEnvironmentsRequest describeEnvironmentsRequest);
/**
*
* Gets information about Cloud9 development environments.
*
*
* @param describeEnvironmentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeEnvironments operation returned by the service.
* @sample AWSCloud9AsyncHandler.DescribeEnvironments
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeEnvironmentsAsync(DescribeEnvironmentsRequest describeEnvironmentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of Cloud9 development environment identifiers.
*
*
* @param listEnvironmentsRequest
* @return A Java Future containing the result of the ListEnvironments operation returned by the service.
* @sample AWSCloud9Async.ListEnvironments
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listEnvironmentsAsync(ListEnvironmentsRequest listEnvironmentsRequest);
/**
*
* Gets a list of Cloud9 development environment identifiers.
*
*
* @param listEnvironmentsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListEnvironments operation returned by the service.
* @sample AWSCloud9AsyncHandler.ListEnvironments
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listEnvironmentsAsync(ListEnvironmentsRequest listEnvironmentsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Gets a list of the tags associated with an Cloud9 development environment.
*
*
* @param listTagsForResourceRequest
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSCloud9Async.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest);
/**
*
* Gets a list of the tags associated with an Cloud9 development environment.
*
*
* @param listTagsForResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListTagsForResource operation returned by the service.
* @sample AWSCloud9AsyncHandler.ListTagsForResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listTagsForResourceAsync(ListTagsForResourceRequest listTagsForResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Adds tags to an Cloud9 development environment.
*
*
*
* Tags that you add to an Cloud9 environment by using this method will NOT be automatically propagated to
* underlying resources.
*
*
*
* @param tagResourceRequest
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSCloud9Async.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest);
/**
*
* Adds tags to an Cloud9 development environment.
*
*
*
* Tags that you add to an Cloud9 environment by using this method will NOT be automatically propagated to
* underlying resources.
*
*
*
* @param tagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the TagResource operation returned by the service.
* @sample AWSCloud9AsyncHandler.TagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future tagResourceAsync(TagResourceRequest tagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Removes tags from an Cloud9 development environment.
*
*
* @param untagResourceRequest
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSCloud9Async.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest);
/**
*
* Removes tags from an Cloud9 development environment.
*
*
* @param untagResourceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UntagResource operation returned by the service.
* @sample AWSCloud9AsyncHandler.UntagResource
* @see AWS API
* Documentation
*/
java.util.concurrent.Future untagResourceAsync(UntagResourceRequest untagResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Changes the settings of an existing Cloud9 development environment.
*
*
* @param updateEnvironmentRequest
* @return A Java Future containing the result of the UpdateEnvironment operation returned by the service.
* @sample AWSCloud9Async.UpdateEnvironment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateEnvironmentAsync(UpdateEnvironmentRequest updateEnvironmentRequest);
/**
*
* Changes the settings of an existing Cloud9 development environment.
*
*
* @param updateEnvironmentRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateEnvironment operation returned by the service.
* @sample AWSCloud9AsyncHandler.UpdateEnvironment
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateEnvironmentAsync(UpdateEnvironmentRequest updateEnvironmentRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Changes the settings of an existing environment member for an Cloud9 development environment.
*
*
* @param updateEnvironmentMembershipRequest
* @return A Java Future containing the result of the UpdateEnvironmentMembership operation returned by the service.
* @sample AWSCloud9Async.UpdateEnvironmentMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future updateEnvironmentMembershipAsync(
UpdateEnvironmentMembershipRequest updateEnvironmentMembershipRequest);
/**
*
* Changes the settings of an existing environment member for an Cloud9 development environment.
*
*
* @param updateEnvironmentMembershipRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateEnvironmentMembership operation returned by the service.
* @sample AWSCloud9AsyncHandler.UpdateEnvironmentMembership
* @see AWS API Documentation
*/
java.util.concurrent.Future updateEnvironmentMembershipAsync(
UpdateEnvironmentMembershipRequest updateEnvironmentMembershipRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}