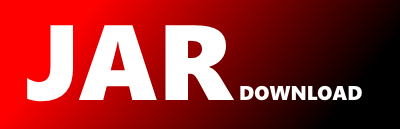
com.amazonaws.services.cloudformation.AmazonCloudFormationAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2010-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.cloudformation;
import com.amazonaws.services.cloudformation.model.*;
/**
* Interface for accessing AWS CloudFormation asynchronously. Each asynchronous
* method will return a Java Future object representing the asynchronous
* operation; overloads which accept an {@code AsyncHandler} can be used to
* receive notification when an asynchronous operation completes.
*
* AWS CloudFormation
*
* AWS CloudFormation enables you to create and manage AWS infrastructure
* deployments predictably and repeatedly. AWS CloudFormation helps you leverage
* AWS products such as Amazon EC2, EBS, Amazon SNS, ELB, and Auto Scaling to
* build highly-reliable, highly scalable, cost effective applications without
* worrying about creating and configuring the underlying AWS infrastructure.
*
*
* With AWS CloudFormation, you declare all of your resources and dependencies
* in a template file. The template defines a collection of resources as a
* single unit called a stack. AWS CloudFormation creates and deletes all member
* resources of the stack together and manages all dependencies between the
* resources for you.
*
*
* For more information about this product, go to the CloudFormation Product Page.
*
*
* Amazon CloudFormation makes use of other AWS products. If you need additional
* technical information about a specific AWS product, you can find the
* product's technical documentation at http://aws.amazon.com/documentation/.
*
*/
public interface AmazonCloudFormationAsync extends AmazonCloudFormation {
/**
*
* Cancels an update on the specified stack. If the call completes
* successfully, the stack rolls back the update and reverts to the previous
* stack configuration.
*
* You can cancel only stacks that are in the UPDATE_IN_PROGRESS
* state.
*
* @param cancelUpdateStackRequest
* The input for the CancelUpdateStack action.
*/
java.util.concurrent.Future cancelUpdateStackAsync(
CancelUpdateStackRequest cancelUpdateStackRequest);
/**
*
* Cancels an update on the specified stack. If the call completes
* successfully, the stack rolls back the update and reverts to the previous
* stack configuration.
*
* You can cancel only stacks that are in the UPDATE_IN_PROGRESS
* state.
*
* @param cancelUpdateStackRequest
* The input for the CancelUpdateStack action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
*/
java.util.concurrent.Future cancelUpdateStackAsync(
CancelUpdateStackRequest cancelUpdateStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a stack as specified in the template. After the call completes
* successfully, the stack creation starts. You can check the status of the
* stack via the DescribeStacks API.
*
*
* @param createStackRequest
* The input for CreateStack action.
* @return A Java Future containing the result of the CreateStack operation
* returned by the service.
*/
java.util.concurrent.Future createStackAsync(
CreateStackRequest createStackRequest);
/**
*
* Creates a stack as specified in the template. After the call completes
* successfully, the stack creation starts. You can check the status of the
* stack via the DescribeStacks API.
*
*
* @param createStackRequest
* The input for CreateStack action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStack operation
* returned by the service.
*/
java.util.concurrent.Future createStackAsync(
CreateStackRequest createStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a specified stack. Once the call completes successfully, stack
* deletion starts. Deleted stacks do not show up in the
* DescribeStacks API if the deletion has been completed
* successfully.
*
*
* @param deleteStackRequest
* The input for DeleteStack action.
*/
java.util.concurrent.Future deleteStackAsync(
DeleteStackRequest deleteStackRequest);
/**
*
* Deletes a specified stack. Once the call completes successfully, stack
* deletion starts. Deleted stacks do not show up in the
* DescribeStacks API if the deletion has been completed
* successfully.
*
*
* @param deleteStackRequest
* The input for DeleteStack action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
*/
java.util.concurrent.Future deleteStackAsync(
DeleteStackRequest deleteStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves your account's AWS CloudFormation limits, such as the maximum
* number of stacks that you can create in your account.
*
*
* @param describeAccountLimitsRequest
* The input for the DescribeAccountLimits action.
* @return A Java Future containing the result of the DescribeAccountLimits
* operation returned by the service.
*/
java.util.concurrent.Future describeAccountLimitsAsync(
DescribeAccountLimitsRequest describeAccountLimitsRequest);
/**
*
* Retrieves your account's AWS CloudFormation limits, such as the maximum
* number of stacks that you can create in your account.
*
*
* @param describeAccountLimitsRequest
* The input for the DescribeAccountLimits action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAccountLimits
* operation returned by the service.
*/
java.util.concurrent.Future describeAccountLimitsAsync(
DescribeAccountLimitsRequest describeAccountLimitsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns all stack related events for a specified stack. For more
* information about a stack's event history, go to Stacks in the AWS CloudFormation User Guide.
*
* You can list events for stacks that have failed to create or have
* been deleted by specifying the unique stack identifier (stack ID).
*
* @param describeStackEventsRequest
* The input for DescribeStackEvents action.
* @return A Java Future containing the result of the DescribeStackEvents
* operation returned by the service.
*/
java.util.concurrent.Future describeStackEventsAsync(
DescribeStackEventsRequest describeStackEventsRequest);
/**
*
* Returns all stack related events for a specified stack. For more
* information about a stack's event history, go to Stacks in the AWS CloudFormation User Guide.
*
* You can list events for stacks that have failed to create or have
* been deleted by specifying the unique stack identifier (stack ID).
*
* @param describeStackEventsRequest
* The input for DescribeStackEvents action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStackEvents
* operation returned by the service.
*/
java.util.concurrent.Future describeStackEventsAsync(
DescribeStackEventsRequest describeStackEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a description of the specified resource in the specified stack.
*
*
* For deleted stacks, DescribeStackResource returns resource information
* for up to 90 days after the stack has been deleted.
*
*
* @param describeStackResourceRequest
* The input for DescribeStackResource action.
* @return A Java Future containing the result of the DescribeStackResource
* operation returned by the service.
*/
java.util.concurrent.Future describeStackResourceAsync(
DescribeStackResourceRequest describeStackResourceRequest);
/**
*
* Returns a description of the specified resource in the specified stack.
*
*
* For deleted stacks, DescribeStackResource returns resource information
* for up to 90 days after the stack has been deleted.
*
*
* @param describeStackResourceRequest
* The input for DescribeStackResource action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStackResource
* operation returned by the service.
*/
java.util.concurrent.Future describeStackResourceAsync(
DescribeStackResourceRequest describeStackResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns AWS resource descriptions for running and deleted stacks. If
* StackName
is specified, all the associated resources that
* are part of the stack are returned. If PhysicalResourceId
is
* specified, the associated resources of the stack that the resource
* belongs to are returned.
*
* Only the first 100 resources will be returned. If your stack has
* more resources than this, you should use ListStackResources
* instead.
*
* For deleted stacks, DescribeStackResources
returns resource
* information for up to 90 days after the stack has been deleted.
*
*
* You must specify either StackName
or
* PhysicalResourceId
, but not both. In addition, you can
* specify LogicalResourceId
to filter the returned result. For
* more information about resources, the LogicalResourceId
and
* PhysicalResourceId
, go to the AWS
* CloudFormation User Guide.
*
* A ValidationError
is returned if you specify both
* StackName
and PhysicalResourceId
in the same
* request.
*
* @param describeStackResourcesRequest
* The input for DescribeStackResources action.
* @return A Java Future containing the result of the DescribeStackResources
* operation returned by the service.
*/
java.util.concurrent.Future describeStackResourcesAsync(
DescribeStackResourcesRequest describeStackResourcesRequest);
/**
*
* Returns AWS resource descriptions for running and deleted stacks. If
* StackName
is specified, all the associated resources that
* are part of the stack are returned. If PhysicalResourceId
is
* specified, the associated resources of the stack that the resource
* belongs to are returned.
*
* Only the first 100 resources will be returned. If your stack has
* more resources than this, you should use ListStackResources
* instead.
*
* For deleted stacks, DescribeStackResources
returns resource
* information for up to 90 days after the stack has been deleted.
*
*
* You must specify either StackName
or
* PhysicalResourceId
, but not both. In addition, you can
* specify LogicalResourceId
to filter the returned result. For
* more information about resources, the LogicalResourceId
and
* PhysicalResourceId
, go to the AWS
* CloudFormation User Guide.
*
* A ValidationError
is returned if you specify both
* StackName
and PhysicalResourceId
in the same
* request.
*
* @param describeStackResourcesRequest
* The input for DescribeStackResources action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStackResources
* operation returned by the service.
*/
java.util.concurrent.Future describeStackResourcesAsync(
DescribeStackResourcesRequest describeStackResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the description for the specified stack; if no stack name was
* specified, then it returns the description for all the stacks created.
*
*
* @param describeStacksRequest
* The input for DescribeStacks action.
* @return A Java Future containing the result of the DescribeStacks
* operation returned by the service.
*/
java.util.concurrent.Future describeStacksAsync(
DescribeStacksRequest describeStacksRequest);
/**
*
* Returns the description for the specified stack; if no stack name was
* specified, then it returns the description for all the stacks created.
*
*
* @param describeStacksRequest
* The input for DescribeStacks action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStacks
* operation returned by the service.
*/
java.util.concurrent.Future describeStacksAsync(
DescribeStacksRequest describeStacksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeStacks operation.
*
* @see #describeStacksAsync(DescribeStacksRequest)
*/
java.util.concurrent.Future describeStacksAsync();
/**
* Simplified method form for invoking the DescribeStacks operation with an
* AsyncHandler.
*
* @see #describeStacksAsync(DescribeStacksRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeStacksAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the estimated monthly cost of a template. The return value is an
* AWS Simple Monthly Calculator URL with a query string that describes the
* resources required to run the template.
*
*
* @param estimateTemplateCostRequest
* @return A Java Future containing the result of the EstimateTemplateCost
* operation returned by the service.
*/
java.util.concurrent.Future estimateTemplateCostAsync(
EstimateTemplateCostRequest estimateTemplateCostRequest);
/**
*
* Returns the estimated monthly cost of a template. The return value is an
* AWS Simple Monthly Calculator URL with a query string that describes the
* resources required to run the template.
*
*
* @param estimateTemplateCostRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EstimateTemplateCost
* operation returned by the service.
*/
java.util.concurrent.Future estimateTemplateCostAsync(
EstimateTemplateCostRequest estimateTemplateCostRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the EstimateTemplateCost operation.
*
* @see #estimateTemplateCostAsync(EstimateTemplateCostRequest)
*/
java.util.concurrent.Future estimateTemplateCostAsync();
/**
* Simplified method form for invoking the EstimateTemplateCost operation
* with an AsyncHandler.
*
* @see #estimateTemplateCostAsync(EstimateTemplateCostRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future estimateTemplateCostAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the stack policy for a specified stack. If a stack doesn't have a
* policy, a null value is returned.
*
*
* @param getStackPolicyRequest
* The input for the GetStackPolicy action.
* @return A Java Future containing the result of the GetStackPolicy
* operation returned by the service.
*/
java.util.concurrent.Future getStackPolicyAsync(
GetStackPolicyRequest getStackPolicyRequest);
/**
*
* Returns the stack policy for a specified stack. If a stack doesn't have a
* policy, a null value is returned.
*
*
* @param getStackPolicyRequest
* The input for the GetStackPolicy action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetStackPolicy
* operation returned by the service.
*/
java.util.concurrent.Future getStackPolicyAsync(
GetStackPolicyRequest getStackPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the template body for a specified stack. You can get the template
* for running or deleted stacks.
*
*
* For deleted stacks, GetTemplate returns the template for up to 90 days
* after the stack has been deleted.
*
* If the template does not exist, a ValidationError
is
* returned.
*
* @param getTemplateRequest
* The input for a GetTemplate action.
* @return A Java Future containing the result of the GetTemplate operation
* returned by the service.
*/
java.util.concurrent.Future getTemplateAsync(
GetTemplateRequest getTemplateRequest);
/**
*
* Returns the template body for a specified stack. You can get the template
* for running or deleted stacks.
*
*
* For deleted stacks, GetTemplate returns the template for up to 90 days
* after the stack has been deleted.
*
* If the template does not exist, a ValidationError
is
* returned.
*
* @param getTemplateRequest
* The input for a GetTemplate action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTemplate operation
* returned by the service.
*/
java.util.concurrent.Future getTemplateAsync(
GetTemplateRequest getTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a new or existing template. The
* GetTemplateSummary
action is useful for viewing parameter
* information, such as default parameter values and parameter types, before
* you create or update a stack.
*
*
* You can use the GetTemplateSummary
action when you submit a
* template, or you can get template information for a running or deleted
* stack.
*
*
* For deleted stacks, GetTemplateSummary
returns the template
* information for up to 90 days after the stack has been deleted. If the
* template does not exist, a ValidationError
is returned.
*
*
* @param getTemplateSummaryRequest
* The input for the GetTemplateSummary action.
* @return A Java Future containing the result of the GetTemplateSummary
* operation returned by the service.
*/
java.util.concurrent.Future getTemplateSummaryAsync(
GetTemplateSummaryRequest getTemplateSummaryRequest);
/**
*
* Returns information about a new or existing template. The
* GetTemplateSummary
action is useful for viewing parameter
* information, such as default parameter values and parameter types, before
* you create or update a stack.
*
*
* You can use the GetTemplateSummary
action when you submit a
* template, or you can get template information for a running or deleted
* stack.
*
*
* For deleted stacks, GetTemplateSummary
returns the template
* information for up to 90 days after the stack has been deleted. If the
* template does not exist, a ValidationError
is returned.
*
*
* @param getTemplateSummaryRequest
* The input for the GetTemplateSummary action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTemplateSummary
* operation returned by the service.
*/
java.util.concurrent.Future getTemplateSummaryAsync(
GetTemplateSummaryRequest getTemplateSummaryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the GetTemplateSummary operation.
*
* @see #getTemplateSummaryAsync(GetTemplateSummaryRequest)
*/
java.util.concurrent.Future getTemplateSummaryAsync();
/**
* Simplified method form for invoking the GetTemplateSummary operation with
* an AsyncHandler.
*
* @see #getTemplateSummaryAsync(GetTemplateSummaryRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future getTemplateSummaryAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns descriptions of all resources of the specified stack.
*
*
* For deleted stacks, ListStackResources returns resource information for
* up to 90 days after the stack has been deleted.
*
*
* @param listStackResourcesRequest
* The input for the ListStackResource action.
* @return A Java Future containing the result of the ListStackResources
* operation returned by the service.
*/
java.util.concurrent.Future listStackResourcesAsync(
ListStackResourcesRequest listStackResourcesRequest);
/**
*
* Returns descriptions of all resources of the specified stack.
*
*
* For deleted stacks, ListStackResources returns resource information for
* up to 90 days after the stack has been deleted.
*
*
* @param listStackResourcesRequest
* The input for the ListStackResource action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStackResources
* operation returned by the service.
*/
java.util.concurrent.Future listStackResourcesAsync(
ListStackResourcesRequest listStackResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the summary information for stacks whose status matches the
* specified StackStatusFilter. Summary information for stacks that have
* been deleted is kept for 90 days after the stack is deleted. If no
* StackStatusFilter is specified, summary information for all stacks is
* returned (including existing stacks and stacks that have been deleted).
*
*
* @param listStacksRequest
* The input for ListStacks action.
* @return A Java Future containing the result of the ListStacks operation
* returned by the service.
*/
java.util.concurrent.Future listStacksAsync(
ListStacksRequest listStacksRequest);
/**
*
* Returns the summary information for stacks whose status matches the
* specified StackStatusFilter. Summary information for stacks that have
* been deleted is kept for 90 days after the stack is deleted. If no
* StackStatusFilter is specified, summary information for all stacks is
* returned (including existing stacks and stacks that have been deleted).
*
*
* @param listStacksRequest
* The input for ListStacks action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStacks operation
* returned by the service.
*/
java.util.concurrent.Future listStacksAsync(
ListStacksRequest listStacksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListStacks operation.
*
* @see #listStacksAsync(ListStacksRequest)
*/
java.util.concurrent.Future listStacksAsync();
/**
* Simplified method form for invoking the ListStacks operation with an
* AsyncHandler.
*
* @see #listStacksAsync(ListStacksRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listStacksAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets a stack policy for a specified stack.
*
*
* @param setStackPolicyRequest
* The input for the SetStackPolicy action.
*/
java.util.concurrent.Future setStackPolicyAsync(
SetStackPolicyRequest setStackPolicyRequest);
/**
*
* Sets a stack policy for a specified stack.
*
*
* @param setStackPolicyRequest
* The input for the SetStackPolicy action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
*/
java.util.concurrent.Future setStackPolicyAsync(
SetStackPolicyRequest setStackPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sends a signal to the specified resource with a success or failure
* status. You can use the SignalResource API in conjunction with a creation
* policy or update policy. AWS CloudFormation doesn't proceed with a stack
* creation or update until resources receive the required number of signals
* or the timeout period is exceeded. The SignalResource API is useful in
* cases where you want to send signals from anywhere other than an Amazon
* EC2 instance.
*
*
* @param signalResourceRequest
* The input for the SignalResource action.
*/
java.util.concurrent.Future signalResourceAsync(
SignalResourceRequest signalResourceRequest);
/**
*
* Sends a signal to the specified resource with a success or failure
* status. You can use the SignalResource API in conjunction with a creation
* policy or update policy. AWS CloudFormation doesn't proceed with a stack
* creation or update until resources receive the required number of signals
* or the timeout period is exceeded. The SignalResource API is useful in
* cases where you want to send signals from anywhere other than an Amazon
* EC2 instance.
*
*
* @param signalResourceRequest
* The input for the SignalResource action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
*/
java.util.concurrent.Future signalResourceAsync(
SignalResourceRequest signalResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a stack as specified in the template. After the call completes
* successfully, the stack update starts. You can check the status of the
* stack via the DescribeStacks action.
*
*
* To get a copy of the template for an existing stack, you can use the
* GetTemplate action.
*
*
* Tags that were associated with this stack during creation time will still
* be associated with the stack after an UpdateStack
operation.
*
*
* For more information about creating an update template, updating a stack,
* and monitoring the progress of the update, see Updating a Stack.
*
*
* @param updateStackRequest
* The input for UpdateStack action.
* @return A Java Future containing the result of the UpdateStack operation
* returned by the service.
*/
java.util.concurrent.Future updateStackAsync(
UpdateStackRequest updateStackRequest);
/**
*
* Updates a stack as specified in the template. After the call completes
* successfully, the stack update starts. You can check the status of the
* stack via the DescribeStacks action.
*
*
* To get a copy of the template for an existing stack, you can use the
* GetTemplate action.
*
*
* Tags that were associated with this stack during creation time will still
* be associated with the stack after an UpdateStack
operation.
*
*
* For more information about creating an update template, updating a stack,
* and monitoring the progress of the update, see Updating a Stack.
*
*
* @param updateStackRequest
* The input for UpdateStack action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateStack operation
* returned by the service.
*/
java.util.concurrent.Future updateStackAsync(
UpdateStackRequest updateStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Validates a specified template.
*
*
* @param validateTemplateRequest
* The input for ValidateTemplate action.
* @return A Java Future containing the result of the ValidateTemplate
* operation returned by the service.
*/
java.util.concurrent.Future validateTemplateAsync(
ValidateTemplateRequest validateTemplateRequest);
/**
*
* Validates a specified template.
*
*
* @param validateTemplateRequest
* The input for ValidateTemplate action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the
* request. Users can provide an implementation of the callback
* methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ValidateTemplate
* operation returned by the service.
*/
java.util.concurrent.Future validateTemplateAsync(
ValidateTemplateRequest validateTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}