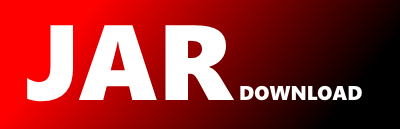
com.amazonaws.services.cloudformation.AmazonCloudFormationAsyncClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2010-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.cloudformation;
import com.amazonaws.services.cloudformation.model.*;
/**
* Interface for accessing AWS CloudFormation asynchronously. Each asynchronous
* method will return a Java Future object representing the asynchronous
* operation; overloads which accept an {@code AsyncHandler} can be used to
* receive notification when an asynchronous operation completes.
*
* AWS CloudFormation
*
* AWS CloudFormation enables you to create and manage AWS infrastructure
* deployments predictably and repeatedly. AWS CloudFormation helps you leverage
* AWS products such as Amazon EC2, EBS, Amazon SNS, ELB, and Auto Scaling to
* build highly-reliable, highly scalable, cost effective applications without
* worrying about creating and configuring the underlying AWS infrastructure.
*
*
* With AWS CloudFormation, you declare all of your resources and dependencies
* in a template file. The template defines a collection of resources as a
* single unit called a stack. AWS CloudFormation creates and deletes all member
* resources of the stack together and manages all dependencies between the
* resources for you.
*
*
* For more information about this product, go to the CloudFormation Product Page.
*
*
* Amazon CloudFormation makes use of other AWS products. If you need additional
* technical information about a specific AWS product, you can find the
* product's technical documentation at http://aws.amazon.com/documentation/.
*
*/
public class AmazonCloudFormationAsyncClient extends AmazonCloudFormationClient
implements AmazonCloudFormationAsync {
private static final int DEFAULT_THREAD_POOL_SIZE = 50;
private final java.util.concurrent.ExecutorService executorService;
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* CloudFormation. A credentials provider chain will be used that searches
* for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Credential profiles file at the default location (~/.aws/credentials)
* shared by all AWS SDKs and the AWS CLI
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* 50 threads (to match the default maximum number of concurrent connections
* to the service).
*
* @see com.amazonaws.auth.DefaultAWSCredentialsProviderChain
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AmazonCloudFormationAsyncClient() {
this(new com.amazonaws.auth.DefaultAWSCredentialsProviderChain());
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* CloudFormation. A credentials provider chain will be used that searches
* for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Credential profiles file at the default location (~/.aws/credentials)
* shared by all AWS SDKs and the AWS CLI
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* a number of threads equal to the maximum number of concurrent connections
* configured via {@code ClientConfiguration.getMaxConnections()}.
*
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to AWS CloudFormation (ex: proxy settings, retry counts,
* etc).
*
* @see com.amazonaws.auth.DefaultAWSCredentialsProviderChain
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AmazonCloudFormationAsyncClient(
com.amazonaws.ClientConfiguration clientConfiguration) {
this(new com.amazonaws.auth.DefaultAWSCredentialsProviderChain(),
clientConfiguration, java.util.concurrent.Executors
.newFixedThreadPool(clientConfiguration
.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* CloudFormation using the specified AWS account credentials.
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* 50 threads (to match the default maximum number of concurrent connections
* to the service).
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AmazonCloudFormationAsyncClient(
com.amazonaws.auth.AWSCredentials awsCredentials) {
this(awsCredentials, java.util.concurrent.Executors
.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* CloudFormation using the specified AWS account credentials and executor
* service. Default client settings will be used.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AmazonCloudFormationAsyncClient(
com.amazonaws.auth.AWSCredentials awsCredentials,
java.util.concurrent.ExecutorService executorService) {
this(awsCredentials, new com.amazonaws.ClientConfiguration(),
executorService);
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* CloudFormation using the specified AWS account credentials, executor
* service, and client configuration options.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy settings,
* etc).
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AmazonCloudFormationAsyncClient(
com.amazonaws.auth.AWSCredentials awsCredentials,
com.amazonaws.ClientConfiguration clientConfiguration,
java.util.concurrent.ExecutorService executorService) {
super(awsCredentials, clientConfiguration);
this.executorService = executorService;
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* CloudFormation using the specified AWS account credentials provider.
* Default client settings will be used.
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* 50 threads (to match the default maximum number of concurrent connections
* to the service).
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AmazonCloudFormationAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, java.util.concurrent.Executors
.newFixedThreadPool(DEFAULT_THREAD_POOL_SIZE));
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* CloudFormation using the provided AWS account credentials provider and
* client configuration options.
*
* Asynchronous methods are delegated to a fixed-size thread pool containing
* a number of threads equal to the maximum number of concurrent connections
* configured via {@code ClientConfiguration.getMaxConnections()}.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy settings,
* etc).
*
* @see com.amazonaws.auth.DefaultAWSCredentialsProviderChain
* @see java.util.concurrent.Executors#newFixedThreadPool(int)
*/
public AmazonCloudFormationAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider,
com.amazonaws.ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration,
java.util.concurrent.Executors
.newFixedThreadPool(clientConfiguration
.getMaxConnections()));
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* CloudFormation using the specified AWS account credentials provider and
* executor service. Default client settings will be used.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AmazonCloudFormationAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider,
java.util.concurrent.ExecutorService executorService) {
this(awsCredentialsProvider, new com.amazonaws.ClientConfiguration(),
executorService);
}
/**
* Constructs a new asynchronous client to invoke service methods on AWS
* CloudFormation using the specified AWS account credentials provider,
* executor service, and client configuration options.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* Client configuration options (ex: max retry limit, proxy settings,
* etc).
* @param executorService
* The executor service by which all asynchronous requests will be
* executed.
*/
public AmazonCloudFormationAsyncClient(
com.amazonaws.auth.AWSCredentialsProvider awsCredentialsProvider,
com.amazonaws.ClientConfiguration clientConfiguration,
java.util.concurrent.ExecutorService executorService) {
super(awsCredentialsProvider, clientConfiguration);
this.executorService = executorService;
}
/**
* Returns the executor service used by this client to execute async
* requests.
*
* @return The executor service used by this client to execute async
* requests.
*/
public java.util.concurrent.ExecutorService getExecutorService() {
return executorService;
}
@Override
public java.util.concurrent.Future cancelUpdateStackAsync(
CancelUpdateStackRequest request) {
return cancelUpdateStackAsync(request, null);
}
@Override
public java.util.concurrent.Future cancelUpdateStackAsync(
final CancelUpdateStackRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public Void call() throws Exception {
Void result;
try {
cancelUpdateStack(request);
result = null;
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future createStackAsync(
CreateStackRequest request) {
return createStackAsync(request, null);
}
@Override
public java.util.concurrent.Future createStackAsync(
final CreateStackRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public CreateStackResult call() throws Exception {
CreateStackResult result;
try {
result = createStack(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future deleteStackAsync(
DeleteStackRequest request) {
return deleteStackAsync(request, null);
}
@Override
public java.util.concurrent.Future deleteStackAsync(
final DeleteStackRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public Void call() throws Exception {
Void result;
try {
deleteStack(request);
result = null;
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future describeAccountLimitsAsync(
DescribeAccountLimitsRequest request) {
return describeAccountLimitsAsync(request, null);
}
@Override
public java.util.concurrent.Future describeAccountLimitsAsync(
final DescribeAccountLimitsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DescribeAccountLimitsResult call() throws Exception {
DescribeAccountLimitsResult result;
try {
result = describeAccountLimits(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future describeStackEventsAsync(
DescribeStackEventsRequest request) {
return describeStackEventsAsync(request, null);
}
@Override
public java.util.concurrent.Future describeStackEventsAsync(
final DescribeStackEventsRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DescribeStackEventsResult call() throws Exception {
DescribeStackEventsResult result;
try {
result = describeStackEvents(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future describeStackResourceAsync(
DescribeStackResourceRequest request) {
return describeStackResourceAsync(request, null);
}
@Override
public java.util.concurrent.Future describeStackResourceAsync(
final DescribeStackResourceRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DescribeStackResourceResult call() throws Exception {
DescribeStackResourceResult result;
try {
result = describeStackResource(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future describeStackResourcesAsync(
DescribeStackResourcesRequest request) {
return describeStackResourcesAsync(request, null);
}
@Override
public java.util.concurrent.Future describeStackResourcesAsync(
final DescribeStackResourcesRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DescribeStackResourcesResult call() throws Exception {
DescribeStackResourcesResult result;
try {
result = describeStackResources(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future describeStacksAsync(
DescribeStacksRequest request) {
return describeStacksAsync(request, null);
}
@Override
public java.util.concurrent.Future describeStacksAsync(
final DescribeStacksRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public DescribeStacksResult call() throws Exception {
DescribeStacksResult result;
try {
result = describeStacks(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the DescribeStacks operation.
*
* @see #describeStacksAsync(DescribeStacksRequest)
*/
@Override
public java.util.concurrent.Future describeStacksAsync() {
return describeStacksAsync(new DescribeStacksRequest());
}
/**
* Simplified method form for invoking the DescribeStacks operation with an
* AsyncHandler.
*
* @see #describeStacksAsync(DescribeStacksRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future describeStacksAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return describeStacksAsync(new DescribeStacksRequest(), asyncHandler);
}
@Override
public java.util.concurrent.Future estimateTemplateCostAsync(
EstimateTemplateCostRequest request) {
return estimateTemplateCostAsync(request, null);
}
@Override
public java.util.concurrent.Future estimateTemplateCostAsync(
final EstimateTemplateCostRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public EstimateTemplateCostResult call() throws Exception {
EstimateTemplateCostResult result;
try {
result = estimateTemplateCost(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the EstimateTemplateCost operation.
*
* @see #estimateTemplateCostAsync(EstimateTemplateCostRequest)
*/
@Override
public java.util.concurrent.Future estimateTemplateCostAsync() {
return estimateTemplateCostAsync(new EstimateTemplateCostRequest());
}
/**
* Simplified method form for invoking the EstimateTemplateCost operation
* with an AsyncHandler.
*
* @see #estimateTemplateCostAsync(EstimateTemplateCostRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future estimateTemplateCostAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return estimateTemplateCostAsync(new EstimateTemplateCostRequest(),
asyncHandler);
}
@Override
public java.util.concurrent.Future getStackPolicyAsync(
GetStackPolicyRequest request) {
return getStackPolicyAsync(request, null);
}
@Override
public java.util.concurrent.Future getStackPolicyAsync(
final GetStackPolicyRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetStackPolicyResult call() throws Exception {
GetStackPolicyResult result;
try {
result = getStackPolicy(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getTemplateAsync(
GetTemplateRequest request) {
return getTemplateAsync(request, null);
}
@Override
public java.util.concurrent.Future getTemplateAsync(
final GetTemplateRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetTemplateResult call() throws Exception {
GetTemplateResult result;
try {
result = getTemplate(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future getTemplateSummaryAsync(
GetTemplateSummaryRequest request) {
return getTemplateSummaryAsync(request, null);
}
@Override
public java.util.concurrent.Future getTemplateSummaryAsync(
final GetTemplateSummaryRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public GetTemplateSummaryResult call() throws Exception {
GetTemplateSummaryResult result;
try {
result = getTemplateSummary(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the GetTemplateSummary operation.
*
* @see #getTemplateSummaryAsync(GetTemplateSummaryRequest)
*/
@Override
public java.util.concurrent.Future getTemplateSummaryAsync() {
return getTemplateSummaryAsync(new GetTemplateSummaryRequest());
}
/**
* Simplified method form for invoking the GetTemplateSummary operation with
* an AsyncHandler.
*
* @see #getTemplateSummaryAsync(GetTemplateSummaryRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future getTemplateSummaryAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return getTemplateSummaryAsync(new GetTemplateSummaryRequest(),
asyncHandler);
}
@Override
public java.util.concurrent.Future listStackResourcesAsync(
ListStackResourcesRequest request) {
return listStackResourcesAsync(request, null);
}
@Override
public java.util.concurrent.Future listStackResourcesAsync(
final ListStackResourcesRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListStackResourcesResult call() throws Exception {
ListStackResourcesResult result;
try {
result = listStackResources(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future listStacksAsync(
ListStacksRequest request) {
return listStacksAsync(request, null);
}
@Override
public java.util.concurrent.Future listStacksAsync(
final ListStacksRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ListStacksResult call() throws Exception {
ListStacksResult result;
try {
result = listStacks(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Simplified method form for invoking the ListStacks operation.
*
* @see #listStacksAsync(ListStacksRequest)
*/
@Override
public java.util.concurrent.Future listStacksAsync() {
return listStacksAsync(new ListStacksRequest());
}
/**
* Simplified method form for invoking the ListStacks operation with an
* AsyncHandler.
*
* @see #listStacksAsync(ListStacksRequest,
* com.amazonaws.handlers.AsyncHandler)
*/
public java.util.concurrent.Future listStacksAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler) {
return listStacksAsync(new ListStacksRequest(), asyncHandler);
}
@Override
public java.util.concurrent.Future setStackPolicyAsync(
SetStackPolicyRequest request) {
return setStackPolicyAsync(request, null);
}
@Override
public java.util.concurrent.Future setStackPolicyAsync(
final SetStackPolicyRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public Void call() throws Exception {
Void result;
try {
setStackPolicy(request);
result = null;
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future signalResourceAsync(
SignalResourceRequest request) {
return signalResourceAsync(request, null);
}
@Override
public java.util.concurrent.Future signalResourceAsync(
final SignalResourceRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public Void call() throws Exception {
Void result;
try {
signalResource(request);
result = null;
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future updateStackAsync(
UpdateStackRequest request) {
return updateStackAsync(request, null);
}
@Override
public java.util.concurrent.Future updateStackAsync(
final UpdateStackRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public UpdateStackResult call() throws Exception {
UpdateStackResult result;
try {
result = updateStack(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
@Override
public java.util.concurrent.Future validateTemplateAsync(
ValidateTemplateRequest request) {
return validateTemplateAsync(request, null);
}
@Override
public java.util.concurrent.Future validateTemplateAsync(
final ValidateTemplateRequest request,
final com.amazonaws.handlers.AsyncHandler asyncHandler) {
return executorService
.submit(new java.util.concurrent.Callable() {
@Override
public ValidateTemplateResult call() throws Exception {
ValidateTemplateResult result;
try {
result = validateTemplate(request);
} catch (Exception ex) {
if (asyncHandler != null) {
asyncHandler.onError(ex);
}
throw ex;
}
if (asyncHandler != null) {
asyncHandler.onSuccess(request, result);
}
return result;
}
});
}
/**
* Shuts down the client, releasing all managed resources. This includes
* forcibly terminating all pending asynchronous service calls. Clients who
* wish to give pending asynchronous service calls time to complete should
* call {@code getExecutorService().shutdown()} followed by
* {@code getExecutorService().awaitTermination()} prior to calling this
* method.
*/
@Override
public void shutdown() {
super.shutdown();
executorService.shutdownNow();
}
}