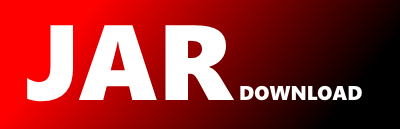
com.amazonaws.services.cloudformation.AmazonCloudFormationClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2010-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.cloudformation;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import java.util.Map.Entry;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.services.cloudformation.model.*;
import com.amazonaws.services.cloudformation.model.transform.*;
/**
* Client for accessing AWS CloudFormation. All service calls made using this
* client are blocking, and will not return until the service call completes.
*
* AWS CloudFormation
*
* AWS CloudFormation enables you to create and manage AWS infrastructure
* deployments predictably and repeatedly. AWS CloudFormation helps you leverage
* AWS products such as Amazon EC2, EBS, Amazon SNS, ELB, and Auto Scaling to
* build highly-reliable, highly scalable, cost effective applications without
* worrying about creating and configuring the underlying AWS infrastructure.
*
*
* With AWS CloudFormation, you declare all of your resources and dependencies
* in a template file. The template defines a collection of resources as a
* single unit called a stack. AWS CloudFormation creates and deletes all member
* resources of the stack together and manages all dependencies between the
* resources for you.
*
*
* For more information about this product, go to the CloudFormation Product Page.
*
*
* Amazon CloudFormation makes use of other AWS products. If you need additional
* technical information about a specific AWS product, you can find the
* product's technical documentation at http://aws.amazon.com/documentation/.
*
*/
public class AmazonCloudFormationClient extends AmazonWebServiceClient
implements AmazonCloudFormation {
/** Provider for AWS credentials. */
private AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory
.getLog(AmazonCloudFormation.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "cloudformation";
/** The region metadata service name for computing region endpoints. */
private static final String DEFAULT_ENDPOINT_PREFIX = "cloudformation";
/**
* List of exception unmarshallers for all AWS CloudFormation exceptions.
*/
protected final List> exceptionUnmarshallers = new ArrayList>();
/**
* Constructs a new client to invoke service methods on AWS CloudFormation.
* A credentials provider chain will be used that searches for credentials
* in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonCloudFormationClient() {
this(new DefaultAWSCredentialsProviderChain(),
com.amazonaws.PredefinedClientConfigurations.defaultConfig());
}
/**
* Constructs a new client to invoke service methods on AWS CloudFormation.
* A credentials provider chain will be used that searches for credentials
* in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2
* metadata service
*
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to AWS CloudFormation (ex: proxy settings, retry counts,
* etc.).
*
* @see DefaultAWSCredentialsProviderChain
*/
public AmazonCloudFormationClient(ClientConfiguration clientConfiguration) {
this(new DefaultAWSCredentialsProviderChain(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on AWS CloudFormation
* using the specified AWS account credentials.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
*/
public AmazonCloudFormationClient(AWSCredentials awsCredentials) {
this(awsCredentials, com.amazonaws.PredefinedClientConfigurations
.defaultConfig());
}
/**
* Constructs a new client to invoke service methods on AWS CloudFormation
* using the specified AWS account credentials and client configuration
* options.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when
* authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to AWS CloudFormation (ex: proxy settings, retry counts,
* etc.).
*/
public AmazonCloudFormationClient(AWSCredentials awsCredentials,
ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(
awsCredentials);
init();
}
/**
* Constructs a new client to invoke service methods on AWS CloudFormation
* using the specified AWS account credentials provider.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
*/
public AmazonCloudFormationClient(
AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider,
com.amazonaws.PredefinedClientConfigurations.defaultConfig());
}
/**
* Constructs a new client to invoke service methods on AWS CloudFormation
* using the specified AWS account credentials provider and client
* configuration options.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to AWS CloudFormation (ex: proxy settings, retry counts,
* etc.).
*/
public AmazonCloudFormationClient(
AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on AWS CloudFormation
* using the specified AWS account credentials provider, client
* configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and
* will not return until the service call completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to
* authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client
* connects to AWS CloudFormation (ex: proxy settings, retry counts,
* etc.).
* @param requestMetricCollector
* optional request metric collector
*/
public AmazonCloudFormationClient(
AWSCredentialsProvider awsCredentialsProvider,
ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
init();
}
private void init() {
exceptionUnmarshallers.add(new LimitExceededExceptionUnmarshaller());
exceptionUnmarshallers.add(new AlreadyExistsExceptionUnmarshaller());
exceptionUnmarshallers
.add(new InsufficientCapabilitiesExceptionUnmarshaller());
exceptionUnmarshallers.add(new StandardErrorUnmarshaller());
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("https://cloudformation.us-east-1.amazonaws.com");
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(DEFAULT_ENDPOINT_PREFIX);
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s
.addAll(chainFactory
.newRequestHandlerChain("/com/amazonaws/services/cloudformation/request.handlers"));
requestHandler2s
.addAll(chainFactory
.newRequestHandler2Chain("/com/amazonaws/services/cloudformation/request.handler2s"));
}
/**
*
* Cancels an update on the specified stack. If the call completes
* successfully, the stack rolls back the update and reverts to the previous
* stack configuration.
*
* You can cancel only stacks that are in the UPDATE_IN_PROGRESS
* state.
*
* @param cancelUpdateStackRequest
* The input for the CancelUpdateStack action.
*/
@Override
public void cancelUpdateStack(
CancelUpdateStackRequest cancelUpdateStackRequest) {
ExecutionContext executionContext = createExecutionContext(cancelUpdateStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelUpdateStackRequestMarshaller()
.marshall(cancelUpdateStackRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
null);
invoke(request, responseHandler, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a stack as specified in the template. After the call completes
* successfully, the stack creation starts. You can check the status of the
* stack via the DescribeStacks API.
*
*
* @param createStackRequest
* The input for CreateStack action.
* @return Result of the CreateStack operation returned by the service.
* @throws LimitExceededException
* Quota for the resource has already been reached.
* @throws AlreadyExistsException
* Resource with the name requested already exists.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that were not
* specified in the Capabilities parameter.
*/
@Override
public CreateStackResult createStack(CreateStackRequest createStackRequest) {
ExecutionContext executionContext = createExecutionContext(createStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateStackRequestMarshaller()
.marshall(createStackRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateStackResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a specified stack. Once the call completes successfully, stack
* deletion starts. Deleted stacks do not show up in the
* DescribeStacks API if the deletion has been completed
* successfully.
*
*
* @param deleteStackRequest
* The input for DeleteStack action.
*/
@Override
public void deleteStack(DeleteStackRequest deleteStackRequest) {
ExecutionContext executionContext = createExecutionContext(deleteStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteStackRequestMarshaller()
.marshall(deleteStackRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
null);
invoke(request, responseHandler, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves your account's AWS CloudFormation limits, such as the maximum
* number of stacks that you can create in your account.
*
*
* @param describeAccountLimitsRequest
* The input for the DescribeAccountLimits action.
* @return Result of the DescribeAccountLimits operation returned by the
* service.
*/
@Override
public DescribeAccountLimitsResult describeAccountLimits(
DescribeAccountLimitsRequest describeAccountLimitsRequest) {
ExecutionContext executionContext = createExecutionContext(describeAccountLimitsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAccountLimitsRequestMarshaller()
.marshall(describeAccountLimitsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeAccountLimitsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns all stack related events for a specified stack. For more
* information about a stack's event history, go to Stacks in the AWS CloudFormation User Guide.
*
* You can list events for stacks that have failed to create or have
* been deleted by specifying the unique stack identifier (stack ID).
*
* @param describeStackEventsRequest
* The input for DescribeStackEvents action.
* @return Result of the DescribeStackEvents operation returned by the
* service.
*/
@Override
public DescribeStackEventsResult describeStackEvents(
DescribeStackEventsRequest describeStackEventsRequest) {
ExecutionContext executionContext = createExecutionContext(describeStackEventsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStackEventsRequestMarshaller()
.marshall(describeStackEventsRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeStackEventsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a description of the specified resource in the specified stack.
*
*
* For deleted stacks, DescribeStackResource returns resource information
* for up to 90 days after the stack has been deleted.
*
*
* @param describeStackResourceRequest
* The input for DescribeStackResource action.
* @return Result of the DescribeStackResource operation returned by the
* service.
*/
@Override
public DescribeStackResourceResult describeStackResource(
DescribeStackResourceRequest describeStackResourceRequest) {
ExecutionContext executionContext = createExecutionContext(describeStackResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStackResourceRequestMarshaller()
.marshall(describeStackResourceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeStackResourceResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns AWS resource descriptions for running and deleted stacks. If
* StackName
is specified, all the associated resources that
* are part of the stack are returned. If PhysicalResourceId
is
* specified, the associated resources of the stack that the resource
* belongs to are returned.
*
* Only the first 100 resources will be returned. If your stack has
* more resources than this, you should use ListStackResources
* instead.
*
* For deleted stacks, DescribeStackResources
returns resource
* information for up to 90 days after the stack has been deleted.
*
*
* You must specify either StackName
or
* PhysicalResourceId
, but not both. In addition, you can
* specify LogicalResourceId
to filter the returned result. For
* more information about resources, the LogicalResourceId
and
* PhysicalResourceId
, go to the AWS
* CloudFormation User Guide.
*
* A ValidationError
is returned if you specify both
* StackName
and PhysicalResourceId
in the same
* request.
*
* @param describeStackResourcesRequest
* The input for DescribeStackResources action.
* @return Result of the DescribeStackResources operation returned by the
* service.
*/
@Override
public DescribeStackResourcesResult describeStackResources(
DescribeStackResourcesRequest describeStackResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(describeStackResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStackResourcesRequestMarshaller()
.marshall(describeStackResourcesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeStackResourcesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the description for the specified stack; if no stack name was
* specified, then it returns the description for all the stacks created.
*
*
* @param describeStacksRequest
* The input for DescribeStacks action.
* @return Result of the DescribeStacks operation returned by the service.
*/
@Override
public DescribeStacksResult describeStacks(
DescribeStacksRequest describeStacksRequest) {
ExecutionContext executionContext = createExecutionContext(describeStacksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStacksRequestMarshaller()
.marshall(describeStacksRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeStacksResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeStacksResult describeStacks() {
return describeStacks(new DescribeStacksRequest());
}
/**
*
* Returns the estimated monthly cost of a template. The return value is an
* AWS Simple Monthly Calculator URL with a query string that describes the
* resources required to run the template.
*
*
* @param estimateTemplateCostRequest
* @return Result of the EstimateTemplateCost operation returned by the
* service.
*/
@Override
public EstimateTemplateCostResult estimateTemplateCost(
EstimateTemplateCostRequest estimateTemplateCostRequest) {
ExecutionContext executionContext = createExecutionContext(estimateTemplateCostRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EstimateTemplateCostRequestMarshaller()
.marshall(estimateTemplateCostRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new EstimateTemplateCostResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public EstimateTemplateCostResult estimateTemplateCost() {
return estimateTemplateCost(new EstimateTemplateCostRequest());
}
/**
*
* Returns the stack policy for a specified stack. If a stack doesn't have a
* policy, a null value is returned.
*
*
* @param getStackPolicyRequest
* The input for the GetStackPolicy action.
* @return Result of the GetStackPolicy operation returned by the service.
*/
@Override
public GetStackPolicyResult getStackPolicy(
GetStackPolicyRequest getStackPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getStackPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetStackPolicyRequestMarshaller()
.marshall(getStackPolicyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetStackPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the template body for a specified stack. You can get the template
* for running or deleted stacks.
*
*
* For deleted stacks, GetTemplate returns the template for up to 90 days
* after the stack has been deleted.
*
* If the template does not exist, a ValidationError
is
* returned.
*
* @param getTemplateRequest
* The input for a GetTemplate action.
* @return Result of the GetTemplate operation returned by the service.
*/
@Override
public GetTemplateResult getTemplate(GetTemplateRequest getTemplateRequest) {
ExecutionContext executionContext = createExecutionContext(getTemplateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetTemplateRequestMarshaller()
.marshall(getTemplateRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetTemplateResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a new or existing template. The
* GetTemplateSummary
action is useful for viewing parameter
* information, such as default parameter values and parameter types, before
* you create or update a stack.
*
*
* You can use the GetTemplateSummary
action when you submit a
* template, or you can get template information for a running or deleted
* stack.
*
*
* For deleted stacks, GetTemplateSummary
returns the template
* information for up to 90 days after the stack has been deleted. If the
* template does not exist, a ValidationError
is returned.
*
*
* @param getTemplateSummaryRequest
* The input for the GetTemplateSummary action.
* @return Result of the GetTemplateSummary operation returned by the
* service.
*/
@Override
public GetTemplateSummaryResult getTemplateSummary(
GetTemplateSummaryRequest getTemplateSummaryRequest) {
ExecutionContext executionContext = createExecutionContext(getTemplateSummaryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetTemplateSummaryRequestMarshaller()
.marshall(getTemplateSummaryRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetTemplateSummaryResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public GetTemplateSummaryResult getTemplateSummary() {
return getTemplateSummary(new GetTemplateSummaryRequest());
}
/**
*
* Returns descriptions of all resources of the specified stack.
*
*
* For deleted stacks, ListStackResources returns resource information for
* up to 90 days after the stack has been deleted.
*
*
* @param listStackResourcesRequest
* The input for the ListStackResource action.
* @return Result of the ListStackResources operation returned by the
* service.
*/
@Override
public ListStackResourcesResult listStackResources(
ListStackResourcesRequest listStackResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(listStackResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListStackResourcesRequestMarshaller()
.marshall(listStackResourcesRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListStackResourcesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the summary information for stacks whose status matches the
* specified StackStatusFilter. Summary information for stacks that have
* been deleted is kept for 90 days after the stack is deleted. If no
* StackStatusFilter is specified, summary information for all stacks is
* returned (including existing stacks and stacks that have been deleted).
*
*
* @param listStacksRequest
* The input for ListStacks action.
* @return Result of the ListStacks operation returned by the service.
*/
@Override
public ListStacksResult listStacks(ListStacksRequest listStacksRequest) {
ExecutionContext executionContext = createExecutionContext(listStacksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListStacksRequestMarshaller()
.marshall(listStacksRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListStacksResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public ListStacksResult listStacks() {
return listStacks(new ListStacksRequest());
}
/**
*
* Sets a stack policy for a specified stack.
*
*
* @param setStackPolicyRequest
* The input for the SetStackPolicy action.
*/
@Override
public void setStackPolicy(SetStackPolicyRequest setStackPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(setStackPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetStackPolicyRequestMarshaller()
.marshall(setStackPolicyRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
null);
invoke(request, responseHandler, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Sends a signal to the specified resource with a success or failure
* status. You can use the SignalResource API in conjunction with a creation
* policy or update policy. AWS CloudFormation doesn't proceed with a stack
* creation or update until resources receive the required number of signals
* or the timeout period is exceeded. The SignalResource API is useful in
* cases where you want to send signals from anywhere other than an Amazon
* EC2 instance.
*
*
* @param signalResourceRequest
* The input for the SignalResource action.
*/
@Override
public void signalResource(SignalResourceRequest signalResourceRequest) {
ExecutionContext executionContext = createExecutionContext(signalResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SignalResourceRequestMarshaller()
.marshall(signalResourceRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
null);
invoke(request, responseHandler, executionContext);
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a stack as specified in the template. After the call completes
* successfully, the stack update starts. You can check the status of the
* stack via the DescribeStacks action.
*
*
* To get a copy of the template for an existing stack, you can use the
* GetTemplate action.
*
*
* Tags that were associated with this stack during creation time will still
* be associated with the stack after an UpdateStack
operation.
*
*
* For more information about creating an update template, updating a stack,
* and monitoring the progress of the update, see Updating a Stack.
*
*
* @param updateStackRequest
* The input for UpdateStack action.
* @return Result of the UpdateStack operation returned by the service.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that were not
* specified in the Capabilities parameter.
*/
@Override
public UpdateStackResult updateStack(UpdateStackRequest updateStackRequest) {
ExecutionContext executionContext = createExecutionContext(updateStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateStackRequestMarshaller()
.marshall(updateStackRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new UpdateStackResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Validates a specified template.
*
*
* @param validateTemplateRequest
* The input for ValidateTemplate action.
* @return Result of the ValidateTemplate operation returned by the service.
*/
@Override
public ValidateTemplateResult validateTemplate(
ValidateTemplateRequest validateTemplateRequest) {
ExecutionContext executionContext = createExecutionContext(validateTemplateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ValidateTemplateRequestMarshaller()
.marshall(validateTemplateRequest);
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ValidateTemplateResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful,
* request, typically used for debugging issues where a service isn't acting
* as expected. This data isn't considered part of the result data returned
* by an operation, so it's available through this separate, diagnostic
* interface.
*
* Response metadata is only cached for a limited period of time, so if you
* need to access this extra diagnostic information for an executed request,
* you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none
* is available.
*/
public ResponseMetadata getCachedResponseMetadata(
AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
private Response invoke(
Request request,
HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
request.setEndpoint(endpoint);
request.setTimeOffset(timeOffset);
AWSRequestMetrics awsRequestMetrics = executionContext
.getAwsRequestMetrics();
AWSCredentials credentials;
awsRequestMetrics.startEvent(Field.CredentialsRequestTime);
try {
credentials = awsCredentialsProvider.getCredentials();
} finally {
awsRequestMetrics.endEvent(Field.CredentialsRequestTime);
}
AmazonWebServiceRequest originalRequest = request.getOriginalRequest();
if (originalRequest != null
&& originalRequest.getRequestCredentials() != null) {
credentials = originalRequest.getRequestCredentials();
}
executionContext.setCredentials(credentials);
DefaultErrorResponseHandler errorResponseHandler = new DefaultErrorResponseHandler(
exceptionUnmarshallers);
return client.execute(request, responseHandler, errorResponseHandler,
executionContext);
}
}