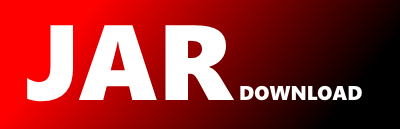
com.amazonaws.services.cloudformation.model.GetTemplateSummaryResult Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2010-2015 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License").
* You may not use this file except in compliance with the License.
* A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed
* on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either
* express or implied. See the License for the specific language governing
* permissions and limitations under the License.
*/
package com.amazonaws.services.cloudformation.model;
import java.io.Serializable;
/**
*
* The output for the GetTemplateSummary action.
*
*/
public class GetTemplateSummaryResult implements Serializable, Cloneable {
/**
*
* A list of parameter declarations that describe various properties for
* each parameter.
*
*/
private com.amazonaws.internal.SdkInternalList parameters;
/**
*
* The value that is defined in the Description
property of the
* template.
*
*/
private String description;
/**
*
* The capabilities found within the template. Currently, AWS CloudFormation
* supports only the CAPABILITY_IAM capability. If your template contains
* IAM resources, you must specify the CAPABILITY_IAM value for this
* parameter when you use the CreateStack or UpdateStack
* actions with your template; otherwise, those actions return an
* InsufficientCapabilities error.
*
*/
private com.amazonaws.internal.SdkInternalList capabilities;
/**
*
* The list of resources that generated the values in the
* Capabilities
response element.
*
*/
private String capabilitiesReason;
/**
*
* A list of all the template resource types that are defined in the
* template, such as AWS::EC2::Instance
,
* AWS::Dynamo::Table
, and
* Custom::MyCustomInstance
. Use the following syntax to
* describe template resource types: AWS::*
(for all AWS
* resources), Custom::*
(for all custom resources),
* Custom::logical_ID
(for a specific custom resource),
* AWS::service_name::*
(for all resources of a
* particular AWS service), and
* AWS::service_name::resource_logical_ID
(for a
* specific AWS resource).
*
*/
private com.amazonaws.internal.SdkInternalList resourceTypes;
/**
*
* The AWS template format version, which identifies the capabilities of the
* template.
*
*/
private String version;
/**
*
* The value that is defined for the Metadata
property of the
* template.
*
*/
private String metadata;
/**
*
* A list of parameter declarations that describe various properties for
* each parameter.
*
*
* @return A list of parameter declarations that describe various properties
* for each parameter.
*/
public java.util.List getParameters() {
if (parameters == null) {
parameters = new com.amazonaws.internal.SdkInternalList();
}
return parameters;
}
/**
*
* A list of parameter declarations that describe various properties for
* each parameter.
*
*
* @param parameters
* A list of parameter declarations that describe various properties
* for each parameter.
*/
public void setParameters(
java.util.Collection parameters) {
if (parameters == null) {
this.parameters = null;
return;
}
this.parameters = new com.amazonaws.internal.SdkInternalList(
parameters);
}
/**
*
* A list of parameter declarations that describe various properties for
* each parameter.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setParameters(java.util.Collection)} or
* {@link #withParameters(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param parameters
* A list of parameter declarations that describe various properties
* for each parameter.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public GetTemplateSummaryResult withParameters(
ParameterDeclaration... parameters) {
if (this.parameters == null) {
setParameters(new com.amazonaws.internal.SdkInternalList(
parameters.length));
}
for (ParameterDeclaration ele : parameters) {
this.parameters.add(ele);
}
return this;
}
/**
*
* A list of parameter declarations that describe various properties for
* each parameter.
*
*
* @param parameters
* A list of parameter declarations that describe various properties
* for each parameter.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public GetTemplateSummaryResult withParameters(
java.util.Collection parameters) {
setParameters(parameters);
return this;
}
/**
*
* The value that is defined in the Description
property of the
* template.
*
*
* @param description
* The value that is defined in the Description
property
* of the template.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* The value that is defined in the Description
property of the
* template.
*
*
* @return The value that is defined in the Description
* property of the template.
*/
public String getDescription() {
return this.description;
}
/**
*
* The value that is defined in the Description
property of the
* template.
*
*
* @param description
* The value that is defined in the Description
property
* of the template.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public GetTemplateSummaryResult withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The capabilities found within the template. Currently, AWS CloudFormation
* supports only the CAPABILITY_IAM capability. If your template contains
* IAM resources, you must specify the CAPABILITY_IAM value for this
* parameter when you use the CreateStack or UpdateStack
* actions with your template; otherwise, those actions return an
* InsufficientCapabilities error.
*
*
* @return The capabilities found within the template. Currently, AWS
* CloudFormation supports only the CAPABILITY_IAM capability. If
* your template contains IAM resources, you must specify the
* CAPABILITY_IAM value for this parameter when you use the
* CreateStack or UpdateStack actions with your
* template; otherwise, those actions return an
* InsufficientCapabilities error.
* @see Capability
*/
public java.util.List getCapabilities() {
if (capabilities == null) {
capabilities = new com.amazonaws.internal.SdkInternalList();
}
return capabilities;
}
/**
*
* The capabilities found within the template. Currently, AWS CloudFormation
* supports only the CAPABILITY_IAM capability. If your template contains
* IAM resources, you must specify the CAPABILITY_IAM value for this
* parameter when you use the CreateStack or UpdateStack
* actions with your template; otherwise, those actions return an
* InsufficientCapabilities error.
*
*
* @param capabilities
* The capabilities found within the template. Currently, AWS
* CloudFormation supports only the CAPABILITY_IAM capability. If
* your template contains IAM resources, you must specify the
* CAPABILITY_IAM value for this parameter when you use the
* CreateStack or UpdateStack actions with your
* template; otherwise, those actions return an
* InsufficientCapabilities error.
* @see Capability
*/
public void setCapabilities(java.util.Collection capabilities) {
if (capabilities == null) {
this.capabilities = null;
return;
}
this.capabilities = new com.amazonaws.internal.SdkInternalList(
capabilities);
}
/**
*
* The capabilities found within the template. Currently, AWS CloudFormation
* supports only the CAPABILITY_IAM capability. If your template contains
* IAM resources, you must specify the CAPABILITY_IAM value for this
* parameter when you use the CreateStack or UpdateStack
* actions with your template; otherwise, those actions return an
* InsufficientCapabilities error.
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setCapabilities(java.util.Collection)} or
* {@link #withCapabilities(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param capabilities
* The capabilities found within the template. Currently, AWS
* CloudFormation supports only the CAPABILITY_IAM capability. If
* your template contains IAM resources, you must specify the
* CAPABILITY_IAM value for this parameter when you use the
* CreateStack or UpdateStack actions with your
* template; otherwise, those actions return an
* InsufficientCapabilities error.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see Capability
*/
public GetTemplateSummaryResult withCapabilities(String... capabilities) {
if (this.capabilities == null) {
setCapabilities(new com.amazonaws.internal.SdkInternalList(
capabilities.length));
}
for (String ele : capabilities) {
this.capabilities.add(ele);
}
return this;
}
/**
*
* The capabilities found within the template. Currently, AWS CloudFormation
* supports only the CAPABILITY_IAM capability. If your template contains
* IAM resources, you must specify the CAPABILITY_IAM value for this
* parameter when you use the CreateStack or UpdateStack
* actions with your template; otherwise, those actions return an
* InsufficientCapabilities error.
*
*
* @param capabilities
* The capabilities found within the template. Currently, AWS
* CloudFormation supports only the CAPABILITY_IAM capability. If
* your template contains IAM resources, you must specify the
* CAPABILITY_IAM value for this parameter when you use the
* CreateStack or UpdateStack actions with your
* template; otherwise, those actions return an
* InsufficientCapabilities error.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see Capability
*/
public GetTemplateSummaryResult withCapabilities(
java.util.Collection capabilities) {
setCapabilities(capabilities);
return this;
}
/**
*
* The capabilities found within the template. Currently, AWS CloudFormation
* supports only the CAPABILITY_IAM capability. If your template contains
* IAM resources, you must specify the CAPABILITY_IAM value for this
* parameter when you use the CreateStack or UpdateStack
* actions with your template; otherwise, those actions return an
* InsufficientCapabilities error.
*
*
* @param capabilities
* The capabilities found within the template. Currently, AWS
* CloudFormation supports only the CAPABILITY_IAM capability. If
* your template contains IAM resources, you must specify the
* CAPABILITY_IAM value for this parameter when you use the
* CreateStack or UpdateStack actions with your
* template; otherwise, those actions return an
* InsufficientCapabilities error.
* @return Returns a reference to this object so that method calls can be
* chained together.
* @see Capability
*/
public GetTemplateSummaryResult withCapabilities(Capability... capabilities) {
com.amazonaws.internal.SdkInternalList capabilitiesCopy = new com.amazonaws.internal.SdkInternalList(
capabilities.length);
for (Capability value : capabilities) {
capabilitiesCopy.add(value.toString());
}
if (getCapabilities() == null) {
setCapabilities(capabilitiesCopy);
} else {
getCapabilities().addAll(capabilitiesCopy);
}
return this;
}
/**
*
* The list of resources that generated the values in the
* Capabilities
response element.
*
*
* @param capabilitiesReason
* The list of resources that generated the values in the
* Capabilities
response element.
*/
public void setCapabilitiesReason(String capabilitiesReason) {
this.capabilitiesReason = capabilitiesReason;
}
/**
*
* The list of resources that generated the values in the
* Capabilities
response element.
*
*
* @return The list of resources that generated the values in the
* Capabilities
response element.
*/
public String getCapabilitiesReason() {
return this.capabilitiesReason;
}
/**
*
* The list of resources that generated the values in the
* Capabilities
response element.
*
*
* @param capabilitiesReason
* The list of resources that generated the values in the
* Capabilities
response element.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public GetTemplateSummaryResult withCapabilitiesReason(
String capabilitiesReason) {
setCapabilitiesReason(capabilitiesReason);
return this;
}
/**
*
* A list of all the template resource types that are defined in the
* template, such as AWS::EC2::Instance
,
* AWS::Dynamo::Table
, and
* Custom::MyCustomInstance
. Use the following syntax to
* describe template resource types: AWS::*
(for all AWS
* resources), Custom::*
(for all custom resources),
* Custom::logical_ID
(for a specific custom resource),
* AWS::service_name::*
(for all resources of a
* particular AWS service), and
* AWS::service_name::resource_logical_ID
(for a
* specific AWS resource).
*
*
* @return A list of all the template resource types that are defined in the
* template, such as AWS::EC2::Instance
,
* AWS::Dynamo::Table
, and
* Custom::MyCustomInstance
. Use the following syntax
* to describe template resource types: AWS::*
(for all
* AWS resources), Custom::*
(for all custom
* resources), Custom::logical_ID
(for a
* specific custom resource),
* AWS::service_name::*
(for all resources of a
* particular AWS service), and
* AWS::service_name::resource_logical_ID
* (for a specific AWS resource).
*/
public java.util.List getResourceTypes() {
if (resourceTypes == null) {
resourceTypes = new com.amazonaws.internal.SdkInternalList();
}
return resourceTypes;
}
/**
*
* A list of all the template resource types that are defined in the
* template, such as AWS::EC2::Instance
,
* AWS::Dynamo::Table
, and
* Custom::MyCustomInstance
. Use the following syntax to
* describe template resource types: AWS::*
(for all AWS
* resources), Custom::*
(for all custom resources),
* Custom::logical_ID
(for a specific custom resource),
* AWS::service_name::*
(for all resources of a
* particular AWS service), and
* AWS::service_name::resource_logical_ID
(for a
* specific AWS resource).
*
*
* @param resourceTypes
* A list of all the template resource types that are defined in the
* template, such as AWS::EC2::Instance
,
* AWS::Dynamo::Table
, and
* Custom::MyCustomInstance
. Use the following syntax to
* describe template resource types: AWS::*
(for all AWS
* resources), Custom::*
(for all custom resources),
* Custom::logical_ID
(for a specific custom
* resource), AWS::service_name::*
(for all
* resources of a particular AWS service), and
* AWS::service_name::resource_logical_ID
* (for a specific AWS resource).
*/
public void setResourceTypes(java.util.Collection resourceTypes) {
if (resourceTypes == null) {
this.resourceTypes = null;
return;
}
this.resourceTypes = new com.amazonaws.internal.SdkInternalList(
resourceTypes);
}
/**
*
* A list of all the template resource types that are defined in the
* template, such as AWS::EC2::Instance
,
* AWS::Dynamo::Table
, and
* Custom::MyCustomInstance
. Use the following syntax to
* describe template resource types: AWS::*
(for all AWS
* resources), Custom::*
(for all custom resources),
* Custom::logical_ID
(for a specific custom resource),
* AWS::service_name::*
(for all resources of a
* particular AWS service), and
* AWS::service_name::resource_logical_ID
(for a
* specific AWS resource).
*
*
* NOTE: This method appends the values to the existing list (if
* any). Use {@link #setResourceTypes(java.util.Collection)} or
* {@link #withResourceTypes(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param resourceTypes
* A list of all the template resource types that are defined in the
* template, such as AWS::EC2::Instance
,
* AWS::Dynamo::Table
, and
* Custom::MyCustomInstance
. Use the following syntax to
* describe template resource types: AWS::*
(for all AWS
* resources), Custom::*
(for all custom resources),
* Custom::logical_ID
(for a specific custom
* resource), AWS::service_name::*
(for all
* resources of a particular AWS service), and
* AWS::service_name::resource_logical_ID
* (for a specific AWS resource).
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public GetTemplateSummaryResult withResourceTypes(String... resourceTypes) {
if (this.resourceTypes == null) {
setResourceTypes(new com.amazonaws.internal.SdkInternalList(
resourceTypes.length));
}
for (String ele : resourceTypes) {
this.resourceTypes.add(ele);
}
return this;
}
/**
*
* A list of all the template resource types that are defined in the
* template, such as AWS::EC2::Instance
,
* AWS::Dynamo::Table
, and
* Custom::MyCustomInstance
. Use the following syntax to
* describe template resource types: AWS::*
(for all AWS
* resources), Custom::*
(for all custom resources),
* Custom::logical_ID
(for a specific custom resource),
* AWS::service_name::*
(for all resources of a
* particular AWS service), and
* AWS::service_name::resource_logical_ID
(for a
* specific AWS resource).
*
*
* @param resourceTypes
* A list of all the template resource types that are defined in the
* template, such as AWS::EC2::Instance
,
* AWS::Dynamo::Table
, and
* Custom::MyCustomInstance
. Use the following syntax to
* describe template resource types: AWS::*
(for all AWS
* resources), Custom::*
(for all custom resources),
* Custom::logical_ID
(for a specific custom
* resource), AWS::service_name::*
(for all
* resources of a particular AWS service), and
* AWS::service_name::resource_logical_ID
* (for a specific AWS resource).
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public GetTemplateSummaryResult withResourceTypes(
java.util.Collection resourceTypes) {
setResourceTypes(resourceTypes);
return this;
}
/**
*
* The AWS template format version, which identifies the capabilities of the
* template.
*
*
* @param version
* The AWS template format version, which identifies the capabilities
* of the template.
*/
public void setVersion(String version) {
this.version = version;
}
/**
*
* The AWS template format version, which identifies the capabilities of the
* template.
*
*
* @return The AWS template format version, which identifies the
* capabilities of the template.
*/
public String getVersion() {
return this.version;
}
/**
*
* The AWS template format version, which identifies the capabilities of the
* template.
*
*
* @param version
* The AWS template format version, which identifies the capabilities
* of the template.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public GetTemplateSummaryResult withVersion(String version) {
setVersion(version);
return this;
}
/**
*
* The value that is defined for the Metadata
property of the
* template.
*
*
* @param metadata
* The value that is defined for the Metadata
property
* of the template.
*/
public void setMetadata(String metadata) {
this.metadata = metadata;
}
/**
*
* The value that is defined for the Metadata
property of the
* template.
*
*
* @return The value that is defined for the Metadata
property
* of the template.
*/
public String getMetadata() {
return this.metadata;
}
/**
*
* The value that is defined for the Metadata
property of the
* template.
*
*
* @param metadata
* The value that is defined for the Metadata
property
* of the template.
* @return Returns a reference to this object so that method calls can be
* chained together.
*/
public GetTemplateSummaryResult withMetadata(String metadata) {
setMetadata(metadata);
return this;
}
/**
* Returns a string representation of this object; useful for testing and
* debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getParameters() != null)
sb.append("Parameters: " + getParameters() + ",");
if (getDescription() != null)
sb.append("Description: " + getDescription() + ",");
if (getCapabilities() != null)
sb.append("Capabilities: " + getCapabilities() + ",");
if (getCapabilitiesReason() != null)
sb.append("CapabilitiesReason: " + getCapabilitiesReason() + ",");
if (getResourceTypes() != null)
sb.append("ResourceTypes: " + getResourceTypes() + ",");
if (getVersion() != null)
sb.append("Version: " + getVersion() + ",");
if (getMetadata() != null)
sb.append("Metadata: " + getMetadata());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof GetTemplateSummaryResult == false)
return false;
GetTemplateSummaryResult other = (GetTemplateSummaryResult) obj;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null
&& other.getParameters().equals(this.getParameters()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null
&& other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getCapabilities() == null ^ this.getCapabilities() == null)
return false;
if (other.getCapabilities() != null
&& other.getCapabilities().equals(this.getCapabilities()) == false)
return false;
if (other.getCapabilitiesReason() == null
^ this.getCapabilitiesReason() == null)
return false;
if (other.getCapabilitiesReason() != null
&& other.getCapabilitiesReason().equals(
this.getCapabilitiesReason()) == false)
return false;
if (other.getResourceTypes() == null ^ this.getResourceTypes() == null)
return false;
if (other.getResourceTypes() != null
&& other.getResourceTypes().equals(this.getResourceTypes()) == false)
return false;
if (other.getVersion() == null ^ this.getVersion() == null)
return false;
if (other.getVersion() != null
&& other.getVersion().equals(this.getVersion()) == false)
return false;
if (other.getMetadata() == null ^ this.getMetadata() == null)
return false;
if (other.getMetadata() != null
&& other.getMetadata().equals(this.getMetadata()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode
+ ((getParameters() == null) ? 0 : getParameters().hashCode());
hashCode = prime
* hashCode
+ ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime
* hashCode
+ ((getCapabilities() == null) ? 0 : getCapabilities()
.hashCode());
hashCode = prime
* hashCode
+ ((getCapabilitiesReason() == null) ? 0
: getCapabilitiesReason().hashCode());
hashCode = prime
* hashCode
+ ((getResourceTypes() == null) ? 0 : getResourceTypes()
.hashCode());
hashCode = prime * hashCode
+ ((getVersion() == null) ? 0 : getVersion().hashCode());
hashCode = prime * hashCode
+ ((getMetadata() == null) ? 0 : getMetadata().hashCode());
return hashCode;
}
@Override
public GetTemplateSummaryResult clone() {
try {
return (GetTemplateSummaryResult) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException(
"Got a CloneNotSupportedException from Object.clone() "
+ "even though we're Cloneable!", e);
}
}
}