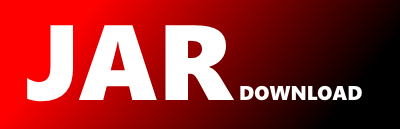
com.amazonaws.services.cloudformation.AmazonCloudFormation Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2012-2017 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudformation;
import javax.annotation.Generated;
import com.amazonaws.*;
import com.amazonaws.regions.*;
import com.amazonaws.services.cloudformation.model.*;
import com.amazonaws.services.cloudformation.waiters.AmazonCloudFormationWaiters;
/**
* Interface for accessing AWS CloudFormation.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.cloudformation.AbstractAmazonCloudFormation} instead.
*
*
* AWS CloudFormation
*
* AWS CloudFormation allows you to create and manage AWS infrastructure deployments predictably and repeatedly. You can
* use AWS CloudFormation to leverage AWS products, such as Amazon Elastic Compute Cloud, Amazon Elastic Block Store,
* Amazon Simple Notification Service, Elastic Load Balancing, and Auto Scaling to build highly-reliable, highly
* scalable, cost-effective applications without creating or configuring the underlying AWS infrastructure.
*
*
* With AWS CloudFormation, you declare all of your resources and dependencies in a template file. The template defines
* a collection of resources as a single unit called a stack. AWS CloudFormation creates and deletes all member
* resources of the stack together and manages all dependencies between the resources for you.
*
*
* For more information about AWS CloudFormation, see the AWS
* CloudFormation Product Page.
*
*
* Amazon CloudFormation makes use of other AWS products. If you need additional technical information about a specific
* AWS product, you can find the product's technical documentation at docs.aws.amazon.com.
*
*
* APIs for stacks
*
*
* When you use AWS CloudFormation, you manage related resources as a single unit called a stack. You create, update,
* and delete a collection of resources by creating, updating, and deleting stacks. All the resources in a stack are
* defined by the stack's AWS CloudFormation template.
*
*
* Actions
*
*
* -
*
*
* -
*
*
* -
*
* CreateStack
*
*
* -
*
* DeleteStack
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
* DescribeStacks
*
*
* -
*
*
* -
*
* GetStackPolicy
*
*
* -
*
* GetTemplate
*
*
* -
*
*
* -
*
* ListExports
*
*
* -
*
* ListImports
*
*
* -
*
*
* -
*
* ListStacks
*
*
* -
*
* SetStackPolicy
*
*
* -
*
* UpdateStack
*
*
* -
*
*
*
*
* Data Types
*
*
* -
*
* Export
*
*
* -
*
* Parameter
*
*
* -
*
*
* -
*
*
* -
*
* Stack
*
*
* -
*
* StackEvent
*
*
* -
*
* StackResource
*
*
* -
*
*
* -
*
*
* -
*
* StackSummary
*
*
* -
*
* Tag
*
*
* -
*
*
*
*
* APIs for change sets
*
*
* If you need to make changes to the running resources in a stack, you update the stack. Before making changes to your
* resources, you can generate a change set, which is summary of your proposed changes. Change sets allow you to see how
* your changes might impact your running resources, especially for critical resources, before implementing them.
*
*
* Actions
*
*
* -
*
* CreateChangeSet
*
*
* -
*
* DeleteChangeSet
*
*
* -
*
*
* -
*
*
* -
*
* ListChangeSets
*
*
*
*
* Data Types
*
*
* -
*
* Change
*
*
* -
*
*
* -
*
* ResourceChange
*
*
* -
*
*
* -
*
*
*
*
* APIs for stack sets
*
*
* AWS CloudFormation StackSets lets you create a collection, or stack set, of stacks that can automatically and safely
* provision a common set of AWS resources across multiple AWS accounts and multiple AWS regions from a single AWS
* CloudFormation template. When you create a stack set, AWS CloudFormation provisions a stack in each of the specified
* accounts and regions by using the supplied AWS CloudFormation template and parameters. Stack sets let you manage a
* common set of AWS resources in a selection of accounts and regions in a single operation.
*
*
* Actions
*
*
* -
*
*
* -
*
* CreateStackSet
*
*
* -
*
*
* -
*
* DeleteStackSet
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
* ListStackSets
*
*
* -
*
*
* -
*
* UpdateStackSet
*
*
*
*
* Data Types
*
*
* -
*
* Parameter
*
*
* -
*
* StackInstance
*
*
* -
*
*
* -
*
* StackSet
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
* StackSetSummary
*
*
* -
*
* Tag
*
*
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonCloudFormation {
/**
* The region metadata service name for computing region endpoints. You can use this value to retrieve metadata
* (such as supported regions) of the service.
*
* @see RegionUtils#getRegionsForService(String)
*/
String ENDPOINT_PREFIX = "cloudformation";
/**
* Overrides the default endpoint for this client ("https://cloudformation.us-east-1.amazonaws.com"). Callers can
* use this method to control which AWS region they want to work with.
*
* Callers can pass in just the endpoint (ex: "cloudformation.us-east-1.amazonaws.com") or a full URL, including the
* protocol (ex: "https://cloudformation.us-east-1.amazonaws.com"). If the protocol is not specified here, the
* default protocol from this client's {@link ClientConfiguration} will be used, which by default is HTTPS.
*
* For more information on using AWS regions with the AWS SDK for Java, and a complete list of all available
* endpoints for all AWS services, see:
* http://developer.amazonwebservices.com/connect/entry.jspa?externalID=3912
*
* This method is not threadsafe. An endpoint should be configured when the client is created and before any
* service requests are made. Changing it afterwards creates inevitable race conditions for any service requests in
* transit or retrying.
*
* @param endpoint
* The endpoint (ex: "cloudformation.us-east-1.amazonaws.com") or a full URL, including the protocol (ex:
* "https://cloudformation.us-east-1.amazonaws.com") of the region specific AWS endpoint this client will
* communicate with.
* @deprecated use {@link AwsClientBuilder#setEndpointConfiguration(AwsClientBuilder.EndpointConfiguration)} for
* example:
* {@code builder.setEndpointConfiguration(new EndpointConfiguration(endpoint, signingRegion));}
*/
@Deprecated
void setEndpoint(String endpoint);
/**
* An alternative to {@link AmazonCloudFormation#setEndpoint(String)}, sets the regional endpoint for this client's
* service calls. Callers can use this method to control which AWS region they want to work with.
*
* By default, all service endpoints in all regions use the https protocol. To use http instead, specify it in the
* {@link ClientConfiguration} supplied at construction.
*
* This method is not threadsafe. A region should be configured when the client is created and before any service
* requests are made. Changing it afterwards creates inevitable race conditions for any service requests in transit
* or retrying.
*
* @param region
* The region this client will communicate with. See {@link Region#getRegion(com.amazonaws.regions.Regions)}
* for accessing a given region. Must not be null and must be a region where the service is available.
*
* @see Region#getRegion(com.amazonaws.regions.Regions)
* @see Region#createClient(Class, com.amazonaws.auth.AWSCredentialsProvider, ClientConfiguration)
* @see Region#isServiceSupported(String)
* @deprecated use {@link AwsClientBuilder#setRegion(String)}
*/
@Deprecated
void setRegion(Region region);
/**
*
* Cancels an update on the specified stack. If the call completes successfully, the stack rolls back the update and
* reverts to the previous stack configuration.
*
*
*
* You can cancel only stacks that are in the UPDATE_IN_PROGRESS state.
*
*
*
* @param cancelUpdateStackRequest
* The input for the CancelUpdateStack action.
* @return Result of the CancelUpdateStack operation returned by the service.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @sample AmazonCloudFormation.CancelUpdateStack
* @see AWS API Documentation
*/
CancelUpdateStackResult cancelUpdateStack(CancelUpdateStackRequest cancelUpdateStackRequest);
/**
*
* For a specified stack that is in the UPDATE_ROLLBACK_FAILED
state, continues rolling it back to the
* UPDATE_ROLLBACK_COMPLETE
state. Depending on the cause of the failure, you can manually fix the error and continue the rollback. By continuing the rollback, you can return your stack to a working
* state (the UPDATE_ROLLBACK_COMPLETE
state), and then try to update the stack again.
*
*
* A stack goes into the UPDATE_ROLLBACK_FAILED
state when AWS CloudFormation cannot roll back all
* changes after a failed stack update. For example, you might have a stack that is rolling back to an old database
* instance that was deleted outside of AWS CloudFormation. Because AWS CloudFormation doesn't know the database was
* deleted, it assumes that the database instance still exists and attempts to roll back to it, causing the update
* rollback to fail.
*
*
* @param continueUpdateRollbackRequest
* The input for the ContinueUpdateRollback action.
* @return Result of the ContinueUpdateRollback operation returned by the service.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @sample AmazonCloudFormation.ContinueUpdateRollback
* @see AWS API Documentation
*/
ContinueUpdateRollbackResult continueUpdateRollback(ContinueUpdateRollbackRequest continueUpdateRollbackRequest);
/**
*
* Creates a list of changes that will be applied to a stack so that you can review the changes before executing
* them. You can create a change set for a stack that doesn't exist or an existing stack. If you create a change set
* for a stack that doesn't exist, the change set shows all of the resources that AWS CloudFormation will create. If
* you create a change set for an existing stack, AWS CloudFormation compares the stack's information with the
* information that you submit in the change set and lists the differences. Use change sets to understand which
* resources AWS CloudFormation will create or change, and how it will change resources in an existing stack, before
* you create or update a stack.
*
*
* To create a change set for a stack that doesn't exist, for the ChangeSetType
parameter, specify
* CREATE
. To create a change set for an existing stack, specify UPDATE
for the
* ChangeSetType
parameter. After the CreateChangeSet
call successfully completes, AWS
* CloudFormation starts creating the change set. To check the status of the change set or to review it, use the
* DescribeChangeSet action.
*
*
* When you are satisfied with the changes the change set will make, execute the change set by using the
* ExecuteChangeSet action. AWS CloudFormation doesn't make changes until you execute the change set.
*
*
* @param createChangeSetRequest
* The input for the CreateChangeSet action.
* @return Result of the CreateChangeSet operation returned by the service.
* @throws AlreadyExistsException
* The resource with the name requested already exists.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @throws LimitExceededException
* The quota for the resource has already been reached.
* @sample AmazonCloudFormation.CreateChangeSet
* @see AWS
* API Documentation
*/
CreateChangeSetResult createChangeSet(CreateChangeSetRequest createChangeSetRequest);
/**
*
* Creates a stack as specified in the template. After the call completes successfully, the stack creation starts.
* You can check the status of the stack via the DescribeStacks API.
*
*
* @param createStackRequest
* The input for CreateStack action.
* @return Result of the CreateStack operation returned by the service.
* @throws LimitExceededException
* The quota for the resource has already been reached.
* @throws AlreadyExistsException
* The resource with the name requested already exists.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @sample AmazonCloudFormation.CreateStack
* @see AWS API
* Documentation
*/
CreateStackResult createStack(CreateStackRequest createStackRequest);
/**
*
* Creates stack instances for the specified accounts, within the specified regions. A stack instance refers to a
* stack in a specific account and region. Accounts
and Regions
are required
* parameters—you must specify at least one account and one region.
*
*
* @param createStackInstancesRequest
* @return Result of the CreateStackInstances operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws LimitExceededException
* The quota for the resource has already been reached.
* @sample AmazonCloudFormation.CreateStackInstances
* @see AWS API Documentation
*/
CreateStackInstancesResult createStackInstances(CreateStackInstancesRequest createStackInstancesRequest);
/**
*
* Creates a stack set.
*
*
* @param createStackSetRequest
* @return Result of the CreateStackSet operation returned by the service.
* @throws NameAlreadyExistsException
* The specified name is already in use.
* @throws CreatedButModifiedException
* The specified resource exists, but has been changed.
* @throws LimitExceededException
* The quota for the resource has already been reached.
* @sample AmazonCloudFormation.CreateStackSet
* @see AWS
* API Documentation
*/
CreateStackSetResult createStackSet(CreateStackSetRequest createStackSetRequest);
/**
*
* Deletes the specified change set. Deleting change sets ensures that no one executes the wrong change set.
*
*
* If the call successfully completes, AWS CloudFormation successfully deleted the change set.
*
*
* @param deleteChangeSetRequest
* The input for the DeleteChangeSet action.
* @return Result of the DeleteChangeSet operation returned by the service.
* @throws InvalidChangeSetStatusException
* The specified change set can't be used to update the stack. For example, the change set status might be
* CREATE_IN_PROGRESS
, or the stack status might be UPDATE_IN_PROGRESS
.
* @sample AmazonCloudFormation.DeleteChangeSet
* @see AWS
* API Documentation
*/
DeleteChangeSetResult deleteChangeSet(DeleteChangeSetRequest deleteChangeSetRequest);
/**
*
* Deletes a specified stack. Once the call completes successfully, stack deletion starts. Deleted stacks do not
* show up in the DescribeStacks API if the deletion has been completed successfully.
*
*
* @param deleteStackRequest
* The input for DeleteStack action.
* @return Result of the DeleteStack operation returned by the service.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @sample AmazonCloudFormation.DeleteStack
* @see AWS API
* Documentation
*/
DeleteStackResult deleteStack(DeleteStackRequest deleteStackRequest);
/**
*
* Deletes stack instances for the specified accounts, in the specified regions.
*
*
* @param deleteStackInstancesRequest
* @return Result of the DeleteStackInstances operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @sample AmazonCloudFormation.DeleteStackInstances
* @see AWS API Documentation
*/
DeleteStackInstancesResult deleteStackInstances(DeleteStackInstancesRequest deleteStackInstancesRequest);
/**
*
* Deletes a stack set. Before you can delete a stack set, all of its member stack instances must be deleted. For
* more information about how to do this, see DeleteStackInstances.
*
*
* @param deleteStackSetRequest
* @return Result of the DeleteStackSet operation returned by the service.
* @throws StackSetNotEmptyException
* You can't yet delete this stack set, because it still contains one or more stack instances. Delete all
* stack instances from the stack set before deleting the stack set.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @sample AmazonCloudFormation.DeleteStackSet
* @see AWS
* API Documentation
*/
DeleteStackSetResult deleteStackSet(DeleteStackSetRequest deleteStackSetRequest);
/**
*
* Retrieves your account's AWS CloudFormation limits, such as the maximum number of stacks that you can create in
* your account.
*
*
* @param describeAccountLimitsRequest
* The input for the DescribeAccountLimits action.
* @return Result of the DescribeAccountLimits operation returned by the service.
* @sample AmazonCloudFormation.DescribeAccountLimits
* @see AWS API Documentation
*/
DescribeAccountLimitsResult describeAccountLimits(DescribeAccountLimitsRequest describeAccountLimitsRequest);
/**
*
* Returns the inputs for the change set and a list of changes that AWS CloudFormation will make if you execute the
* change set. For more information, see Updating Stacks Using Change Sets in the AWS CloudFormation User Guide.
*
*
* @param describeChangeSetRequest
* The input for the DescribeChangeSet action.
* @return Result of the DescribeChangeSet operation returned by the service.
* @throws ChangeSetNotFoundException
* The specified change set name or ID doesn't exit. To view valid change sets for a stack, use the
* ListChangeSets
action.
* @sample AmazonCloudFormation.DescribeChangeSet
* @see AWS API Documentation
*/
DescribeChangeSetResult describeChangeSet(DescribeChangeSetRequest describeChangeSetRequest);
/**
*
* Returns all stack related events for a specified stack in reverse chronological order. For more information about
* a stack's event history, go to Stacks in the AWS
* CloudFormation User Guide.
*
*
*
* You can list events for stacks that have failed to create or have been deleted by specifying the unique stack
* identifier (stack ID).
*
*
*
* @param describeStackEventsRequest
* The input for DescribeStackEvents action.
* @return Result of the DescribeStackEvents operation returned by the service.
* @sample AmazonCloudFormation.DescribeStackEvents
* @see AWS API Documentation
*/
DescribeStackEventsResult describeStackEvents(DescribeStackEventsRequest describeStackEventsRequest);
/**
*
* Returns the stack instance that's associated with the specified stack set, AWS account, and region.
*
*
* For a list of stack instances that are associated with a specific stack set, use ListStackInstances.
*
*
* @param describeStackInstanceRequest
* @return Result of the DescribeStackInstance operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws StackInstanceNotFoundException
* The specified stack instance doesn't exist.
* @sample AmazonCloudFormation.DescribeStackInstance
* @see AWS API Documentation
*/
DescribeStackInstanceResult describeStackInstance(DescribeStackInstanceRequest describeStackInstanceRequest);
/**
*
* Returns a description of the specified resource in the specified stack.
*
*
* For deleted stacks, DescribeStackResource returns resource information for up to 90 days after the stack has been
* deleted.
*
*
* @param describeStackResourceRequest
* The input for DescribeStackResource action.
* @return Result of the DescribeStackResource operation returned by the service.
* @sample AmazonCloudFormation.DescribeStackResource
* @see AWS API Documentation
*/
DescribeStackResourceResult describeStackResource(DescribeStackResourceRequest describeStackResourceRequest);
/**
*
* Returns AWS resource descriptions for running and deleted stacks. If StackName
is specified, all the
* associated resources that are part of the stack are returned. If PhysicalResourceId
is specified,
* the associated resources of the stack that the resource belongs to are returned.
*
*
*
* Only the first 100 resources will be returned. If your stack has more resources than this, you should use
* ListStackResources
instead.
*
*
*
* For deleted stacks, DescribeStackResources
returns resource information for up to 90 days after the
* stack has been deleted.
*
*
* You must specify either StackName
or PhysicalResourceId
, but not both. In addition, you
* can specify LogicalResourceId
to filter the returned result. For more information about resources,
* the LogicalResourceId
and PhysicalResourceId
, go to the AWS CloudFormation User Guide.
*
*
*
* A ValidationError
is returned if you specify both StackName
and
* PhysicalResourceId
in the same request.
*
*
*
* @param describeStackResourcesRequest
* The input for DescribeStackResources action.
* @return Result of the DescribeStackResources operation returned by the service.
* @sample AmazonCloudFormation.DescribeStackResources
* @see AWS API Documentation
*/
DescribeStackResourcesResult describeStackResources(DescribeStackResourcesRequest describeStackResourcesRequest);
/**
*
* Returns the description of the specified stack set.
*
*
* @param describeStackSetRequest
* @return Result of the DescribeStackSet operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @sample AmazonCloudFormation.DescribeStackSet
* @see AWS API Documentation
*/
DescribeStackSetResult describeStackSet(DescribeStackSetRequest describeStackSetRequest);
/**
*
* Returns the description of the specified stack set operation.
*
*
* @param describeStackSetOperationRequest
* @return Result of the DescribeStackSetOperation operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @sample AmazonCloudFormation.DescribeStackSetOperation
* @see AWS API Documentation
*/
DescribeStackSetOperationResult describeStackSetOperation(DescribeStackSetOperationRequest describeStackSetOperationRequest);
/**
*
* Returns the description for the specified stack; if no stack name was specified, then it returns the description
* for all the stacks created.
*
*
*
* If the stack does not exist, an AmazonCloudFormationException
is returned.
*
*
*
* @param describeStacksRequest
* The input for DescribeStacks action.
* @return Result of the DescribeStacks operation returned by the service.
* @sample AmazonCloudFormation.DescribeStacks
* @see AWS
* API Documentation
*/
DescribeStacksResult describeStacks(DescribeStacksRequest describeStacksRequest);
/**
* Simplified method form for invoking the DescribeStacks operation.
*
* @see #describeStacks(DescribeStacksRequest)
*/
DescribeStacksResult describeStacks();
/**
*
* Returns the estimated monthly cost of a template. The return value is an AWS Simple Monthly Calculator URL with a
* query string that describes the resources required to run the template.
*
*
* @param estimateTemplateCostRequest
* The input for an EstimateTemplateCost action.
* @return Result of the EstimateTemplateCost operation returned by the service.
* @sample AmazonCloudFormation.EstimateTemplateCost
* @see AWS API Documentation
*/
EstimateTemplateCostResult estimateTemplateCost(EstimateTemplateCostRequest estimateTemplateCostRequest);
/**
* Simplified method form for invoking the EstimateTemplateCost operation.
*
* @see #estimateTemplateCost(EstimateTemplateCostRequest)
*/
EstimateTemplateCostResult estimateTemplateCost();
/**
*
* Updates a stack using the input information that was provided when the specified change set was created. After
* the call successfully completes, AWS CloudFormation starts updating the stack. Use the DescribeStacks
* action to view the status of the update.
*
*
* When you execute a change set, AWS CloudFormation deletes all other change sets associated with the stack because
* they aren't valid for the updated stack.
*
*
* If a stack policy is associated with the stack, AWS CloudFormation enforces the policy during the update. You
* can't specify a temporary stack policy that overrides the current policy.
*
*
* @param executeChangeSetRequest
* The input for the ExecuteChangeSet action.
* @return Result of the ExecuteChangeSet operation returned by the service.
* @throws InvalidChangeSetStatusException
* The specified change set can't be used to update the stack. For example, the change set status might be
* CREATE_IN_PROGRESS
, or the stack status might be UPDATE_IN_PROGRESS
.
* @throws ChangeSetNotFoundException
* The specified change set name or ID doesn't exit. To view valid change sets for a stack, use the
* ListChangeSets
action.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @sample AmazonCloudFormation.ExecuteChangeSet
* @see AWS API Documentation
*/
ExecuteChangeSetResult executeChangeSet(ExecuteChangeSetRequest executeChangeSetRequest);
/**
*
* Returns the stack policy for a specified stack. If a stack doesn't have a policy, a null value is returned.
*
*
* @param getStackPolicyRequest
* The input for the GetStackPolicy action.
* @return Result of the GetStackPolicy operation returned by the service.
* @sample AmazonCloudFormation.GetStackPolicy
* @see AWS
* API Documentation
*/
GetStackPolicyResult getStackPolicy(GetStackPolicyRequest getStackPolicyRequest);
/**
*
* Returns the template body for a specified stack. You can get the template for running or deleted stacks.
*
*
* For deleted stacks, GetTemplate returns the template for up to 90 days after the stack has been deleted.
*
*
*
* If the template does not exist, a ValidationError
is returned.
*
*
*
* @param getTemplateRequest
* The input for a GetTemplate action.
* @return Result of the GetTemplate operation returned by the service.
* @throws ChangeSetNotFoundException
* The specified change set name or ID doesn't exit. To view valid change sets for a stack, use the
* ListChangeSets
action.
* @sample AmazonCloudFormation.GetTemplate
* @see AWS API
* Documentation
*/
GetTemplateResult getTemplate(GetTemplateRequest getTemplateRequest);
/**
*
* Returns information about a new or existing template. The GetTemplateSummary
action is useful for
* viewing parameter information, such as default parameter values and parameter types, before you create or update
* a stack.
*
*
* You can use the GetTemplateSummary
action when you submit a template, or you can get template
* information for a running or deleted stack.
*
*
* For deleted stacks, GetTemplateSummary
returns the template information for up to 90 days after the
* stack has been deleted. If the template does not exist, a ValidationError
is returned.
*
*
* @param getTemplateSummaryRequest
* The input for the GetTemplateSummary action.
* @return Result of the GetTemplateSummary operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @sample AmazonCloudFormation.GetTemplateSummary
* @see AWS API Documentation
*/
GetTemplateSummaryResult getTemplateSummary(GetTemplateSummaryRequest getTemplateSummaryRequest);
/**
* Simplified method form for invoking the GetTemplateSummary operation.
*
* @see #getTemplateSummary(GetTemplateSummaryRequest)
*/
GetTemplateSummaryResult getTemplateSummary();
/**
*
* Returns the ID and status of each active change set for a stack. For example, AWS CloudFormation lists change
* sets that are in the CREATE_IN_PROGRESS
or CREATE_PENDING
state.
*
*
* @param listChangeSetsRequest
* The input for the ListChangeSets action.
* @return Result of the ListChangeSets operation returned by the service.
* @sample AmazonCloudFormation.ListChangeSets
* @see AWS
* API Documentation
*/
ListChangeSetsResult listChangeSets(ListChangeSetsRequest listChangeSetsRequest);
/**
*
* Lists all exported output values in the account and region in which you call this action. Use this action to see
* the exported output values that you can import into other stacks. To import values, use the
* Fn::ImportValue
function.
*
*
* For more information, see AWS
* CloudFormation Export Stack Output Values.
*
*
* @param listExportsRequest
* @return Result of the ListExports operation returned by the service.
* @sample AmazonCloudFormation.ListExports
* @see AWS API
* Documentation
*/
ListExportsResult listExports(ListExportsRequest listExportsRequest);
/**
*
* Lists all stacks that are importing an exported output value. To modify or remove an exported output value, first
* use this action to see which stacks are using it. To see the exported output values in your account, see
* ListExports.
*
*
* For more information about importing an exported output value, see the
* Fn::ImportValue
function.
*
*
* @param listImportsRequest
* @return Result of the ListImports operation returned by the service.
* @sample AmazonCloudFormation.ListImports
* @see AWS API
* Documentation
*/
ListImportsResult listImports(ListImportsRequest listImportsRequest);
/**
*
* Returns summary information about stack instances that are associated with the specified stack set. You can
* filter for stack instances that are associated with a specific AWS account name or region.
*
*
* @param listStackInstancesRequest
* @return Result of the ListStackInstances operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @sample AmazonCloudFormation.ListStackInstances
* @see AWS API Documentation
*/
ListStackInstancesResult listStackInstances(ListStackInstancesRequest listStackInstancesRequest);
/**
*
* Returns descriptions of all resources of the specified stack.
*
*
* For deleted stacks, ListStackResources returns resource information for up to 90 days after the stack has been
* deleted.
*
*
* @param listStackResourcesRequest
* The input for the ListStackResource action.
* @return Result of the ListStackResources operation returned by the service.
* @sample AmazonCloudFormation.ListStackResources
* @see AWS API Documentation
*/
ListStackResourcesResult listStackResources(ListStackResourcesRequest listStackResourcesRequest);
/**
*
* Returns summary information about the results of a stack set operation.
*
*
* @param listStackSetOperationResultsRequest
* @return Result of the ListStackSetOperationResults operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @sample AmazonCloudFormation.ListStackSetOperationResults
* @see AWS API Documentation
*/
ListStackSetOperationResultsResult listStackSetOperationResults(ListStackSetOperationResultsRequest listStackSetOperationResultsRequest);
/**
*
* Returns summary information about operations performed on a stack set.
*
*
* @param listStackSetOperationsRequest
* @return Result of the ListStackSetOperations operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @sample AmazonCloudFormation.ListStackSetOperations
* @see AWS API Documentation
*/
ListStackSetOperationsResult listStackSetOperations(ListStackSetOperationsRequest listStackSetOperationsRequest);
/**
*
* Returns summary information about stack sets that are associated with the user.
*
*
* @param listStackSetsRequest
* @return Result of the ListStackSets operation returned by the service.
* @sample AmazonCloudFormation.ListStackSets
* @see AWS
* API Documentation
*/
ListStackSetsResult listStackSets(ListStackSetsRequest listStackSetsRequest);
/**
*
* Returns the summary information for stacks whose status matches the specified StackStatusFilter. Summary
* information for stacks that have been deleted is kept for 90 days after the stack is deleted. If no
* StackStatusFilter is specified, summary information for all stacks is returned (including existing stacks and
* stacks that have been deleted).
*
*
* @param listStacksRequest
* The input for ListStacks action.
* @return Result of the ListStacks operation returned by the service.
* @sample AmazonCloudFormation.ListStacks
* @see AWS API
* Documentation
*/
ListStacksResult listStacks(ListStacksRequest listStacksRequest);
/**
* Simplified method form for invoking the ListStacks operation.
*
* @see #listStacks(ListStacksRequest)
*/
ListStacksResult listStacks();
/**
*
* Sets a stack policy for a specified stack.
*
*
* @param setStackPolicyRequest
* The input for the SetStackPolicy action.
* @return Result of the SetStackPolicy operation returned by the service.
* @sample AmazonCloudFormation.SetStackPolicy
* @see AWS
* API Documentation
*/
SetStackPolicyResult setStackPolicy(SetStackPolicyRequest setStackPolicyRequest);
/**
*
* Sends a signal to the specified resource with a success or failure status. You can use the SignalResource API in
* conjunction with a creation policy or update policy. AWS CloudFormation doesn't proceed with a stack creation or
* update until resources receive the required number of signals or the timeout period is exceeded. The
* SignalResource API is useful in cases where you want to send signals from anywhere other than an Amazon EC2
* instance.
*
*
* @param signalResourceRequest
* The input for the SignalResource action.
* @return Result of the SignalResource operation returned by the service.
* @sample AmazonCloudFormation.SignalResource
* @see AWS
* API Documentation
*/
SignalResourceResult signalResource(SignalResourceRequest signalResourceRequest);
/**
*
* Stops an in-progress operation on a stack set and its associated stack instances.
*
*
* @param stopStackSetOperationRequest
* @return Result of the StopStackSetOperation operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @sample AmazonCloudFormation.StopStackSetOperation
* @see AWS API Documentation
*/
StopStackSetOperationResult stopStackSetOperation(StopStackSetOperationRequest stopStackSetOperationRequest);
/**
*
* Updates a stack as specified in the template. After the call completes successfully, the stack update starts. You
* can check the status of the stack via the DescribeStacks action.
*
*
* To get a copy of the template for an existing stack, you can use the GetTemplate action.
*
*
* For more information about creating an update template, updating a stack, and monitoring the progress of the
* update, see Updating a
* Stack.
*
*
* @param updateStackRequest
* The input for an UpdateStack action.
* @return Result of the UpdateStack operation returned by the service.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @sample AmazonCloudFormation.UpdateStack
* @see AWS API
* Documentation
*/
UpdateStackResult updateStack(UpdateStackRequest updateStackRequest);
/**
*
* Updates the stack set and all associated stack instances.
*
*
* Even if the stack set operation created by updating the stack set fails (completely or partially, below or above
* a specified failure tolerance), the stack set is updated with your changes. Subsequent
* CreateStackInstances calls on the specified stack set use the updated stack set.
*
*
* @param updateStackSetRequest
* @return Result of the UpdateStackSet operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @sample AmazonCloudFormation.UpdateStackSet
* @see AWS
* API Documentation
*/
UpdateStackSetResult updateStackSet(UpdateStackSetRequest updateStackSetRequest);
/**
*
* Validates a specified template. AWS CloudFormation first checks if the template is valid JSON. If it isn't, AWS
* CloudFormation checks if the template is valid YAML. If both these checks fail, AWS CloudFormation returns a
* template validation error.
*
*
* @param validateTemplateRequest
* The input for ValidateTemplate action.
* @return Result of the ValidateTemplate operation returned by the service.
* @sample AmazonCloudFormation.ValidateTemplate
* @see AWS API Documentation
*/
ValidateTemplateResult validateTemplate(ValidateTemplateRequest validateTemplateRequest);
/**
* Shuts down this client object, releasing any resources that might be held open. This is an optional method, and
* callers are not expected to call it, but can if they want to explicitly release any open resources. Once a client
* has been shutdown, it should not be used to make any more requests.
*/
void shutdown();
/**
* Returns additional metadata for a previously executed successful request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing a request.
*
* @param request
* The originally executed request.
*
* @return The response metadata for the specified request, or null if none is available.
*/
ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request);
AmazonCloudFormationWaiters waiters();
}