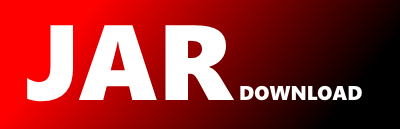
com.amazonaws.services.cloudformation.model.UpdateStackSetRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudformation.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class UpdateStackSetRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name or unique ID of the stack set that you want to update.
*
*/
private String stackSetName;
/**
*
* A brief description of updates that you are making.
*
*/
private String description;
/**
*
* The structure that contains the template body, with a minimum length of 1 byte and a maximum length of 51,200
* bytes. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*/
private String templateBody;
/**
*
* The location of the file that contains the template body. The URL must point to a template (maximum size: 460,800
* bytes) that is located in an Amazon S3 bucket. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*/
private String templateURL;
/**
*
* Use the existing template that's associated with the stack set that you're updating.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*/
private Boolean usePreviousTemplate;
/**
*
* A list of input parameters for the stack set template.
*
*/
private com.amazonaws.internal.SdkInternalList parameters;
/**
*
* A list of values that you must specify before AWS CloudFormation can create certain stack sets. Some stack set
* templates might include resources that can affect permissions in your AWS account—for example, by creating new
* AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly acknowledge their
* capabilities by specifying this parameter.
*
*
* The only valid values are CAPABILITY_IAM and CAPABILITY_NAMED_IAM. The following resources require you to specify
* this parameter:
*
*
* -
*
* AWS::IAM::AccessKey
*
*
* -
*
* AWS::IAM::Group
*
*
* -
*
* AWS::IAM::InstanceProfile
*
*
* -
*
* AWS::IAM::Policy
*
*
* -
*
* AWS::IAM::Role
*
*
* -
*
* AWS::IAM::User
*
*
* -
*
* AWS::IAM::UserToGroupAddition
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions that are associated
* with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom names, you
* must specify CAPABILITY_NAMED_IAM. If you don't specify this parameter, this action returns an
* InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*/
private com.amazonaws.internal.SdkInternalList capabilities;
/**
*
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation also
* propagates these tags to supported resources that are created in the stacks. You can specify a maximum number of
* 50 tags.
*
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently associated with
* this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you want
* associated with this stack set, even tags you've specifed before (for example, when creating the stack set or
* during a previous update of the stack set.). Any tags that you don't include in the updated list of tags are
* removed from the stack set, and therefore from the stacks and resources as well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to see if you
* have the required IAM permission to tag resources. If you omit tags that are currently associated with the stack
* set from the list of tags you specify, AWS CloudFormation assumes that you want to remove those tags from the
* stack set, and checks to see if you have permission to untag resources. If you don't have the necessary
* permission(s), the entire UpdateStackSet
action fails with an access denied
error, and
* the stack set is not updated.
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* Preferences for how AWS CloudFormation performs this stack set operation.
*
*/
private StackSetOperationPreferences operationPreferences;
/**
*
* The Amazon Resource Number (ARN) of the IAM role to use to update this stack set.
*
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups can
* manage specific stack sets within the same administrator account. For more information, see Define Permissions
* for Multiple Administrators in the AWS CloudFormation User Guide.
*
*
* If you specify a customized administrator role, AWS CloudFormation uses that role to update the stack. If you do
* not specify a customized administrator role, AWS CloudFormation performs the update using the role previously
* associated with the stack set, so long as you have permissions to perform operations on the stack set.
*
*/
private String administrationRoleARN;
/**
*
* The name of the IAM execution role to use to update the stack set. If you do not specify an execution role, AWS
* CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack set operation.
*
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources users and
* groups can include in their stack sets.
*
*
* If you specify a customized execution role, AWS CloudFormation uses that role to update the stack. If you do not
* specify a customized execution role, AWS CloudFormation performs the update using the role previously associated
* with the stack set, so long as you have permissions to perform operations on the stack set.
*
*/
private String executionRoleName;
/**
*
* The unique ID for this stack set operation.
*
*
* The operation ID also functions as an idempotency token, to ensure that AWS CloudFormation performs the stack set
* operation only once, even if you retry the request multiple times. You might retry stack set operation requests
* to ensure that AWS CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, AWS CloudFormation generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*
*/
private String operationId;
/**
*
* The accounts in which to update associated stack instances. If you specify accounts, you must also specify the
* regions in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the Accounts
* or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS CloudFormation
* marks all stack instances with a status of OUTDATED
prior to updating the stack instances in the
* specified accounts and regions. If the stack set update does not include changes to the template or parameters,
* AWS CloudFormation updates the stack instances in the specified accounts and regions, while leaving all other
* stack instances with their existing stack instance status.
*
*/
private com.amazonaws.internal.SdkInternalList accounts;
/**
*
* The regions in which to update associated stack instances. If you specify regions, you must also specify accounts
* in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the Accounts
* or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS CloudFormation
* marks all stack instances with a status of OUTDATED
prior to updating the stack instances in the
* specified accounts and regions. If the stack set update does not include changes to the template or parameters,
* AWS CloudFormation updates the stack instances in the specified accounts and regions, while leaving all other
* stack instances with their existing stack instance status.
*
*/
private com.amazonaws.internal.SdkInternalList regions;
/**
*
* The name or unique ID of the stack set that you want to update.
*
*
* @param stackSetName
* The name or unique ID of the stack set that you want to update.
*/
public void setStackSetName(String stackSetName) {
this.stackSetName = stackSetName;
}
/**
*
* The name or unique ID of the stack set that you want to update.
*
*
* @return The name or unique ID of the stack set that you want to update.
*/
public String getStackSetName() {
return this.stackSetName;
}
/**
*
* The name or unique ID of the stack set that you want to update.
*
*
* @param stackSetName
* The name or unique ID of the stack set that you want to update.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withStackSetName(String stackSetName) {
setStackSetName(stackSetName);
return this;
}
/**
*
* A brief description of updates that you are making.
*
*
* @param description
* A brief description of updates that you are making.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A brief description of updates that you are making.
*
*
* @return A brief description of updates that you are making.
*/
public String getDescription() {
return this.description;
}
/**
*
* A brief description of updates that you are making.
*
*
* @param description
* A brief description of updates that you are making.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The structure that contains the template body, with a minimum length of 1 byte and a maximum length of 51,200
* bytes. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*
* @param templateBody
* The structure that contains the template body, with a minimum length of 1 byte and a maximum length of
* 51,200 bytes. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*/
public void setTemplateBody(String templateBody) {
this.templateBody = templateBody;
}
/**
*
* The structure that contains the template body, with a minimum length of 1 byte and a maximum length of 51,200
* bytes. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*
* @return The structure that contains the template body, with a minimum length of 1 byte and a maximum length of
* 51,200 bytes. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*/
public String getTemplateBody() {
return this.templateBody;
}
/**
*
* The structure that contains the template body, with a minimum length of 1 byte and a maximum length of 51,200
* bytes. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*
* @param templateBody
* The structure that contains the template body, with a minimum length of 1 byte and a maximum length of
* 51,200 bytes. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withTemplateBody(String templateBody) {
setTemplateBody(templateBody);
return this;
}
/**
*
* The location of the file that contains the template body. The URL must point to a template (maximum size: 460,800
* bytes) that is located in an Amazon S3 bucket. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*
* @param templateURL
* The location of the file that contains the template body. The URL must point to a template (maximum size:
* 460,800 bytes) that is located in an Amazon S3 bucket. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*/
public void setTemplateURL(String templateURL) {
this.templateURL = templateURL;
}
/**
*
* The location of the file that contains the template body. The URL must point to a template (maximum size: 460,800
* bytes) that is located in an Amazon S3 bucket. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*
* @return The location of the file that contains the template body. The URL must point to a template (maximum size:
* 460,800 bytes) that is located in an Amazon S3 bucket. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*/
public String getTemplateURL() {
return this.templateURL;
}
/**
*
* The location of the file that contains the template body. The URL must point to a template (maximum size: 460,800
* bytes) that is located in an Amazon S3 bucket. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*
* @param templateURL
* The location of the file that contains the template body. The URL must point to a template (maximum size:
* 460,800 bytes) that is located in an Amazon S3 bucket. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withTemplateURL(String templateURL) {
setTemplateURL(templateURL);
return this;
}
/**
*
* Use the existing template that's associated with the stack set that you're updating.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*
* @param usePreviousTemplate
* Use the existing template that's associated with the stack set that you're updating.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*/
public void setUsePreviousTemplate(Boolean usePreviousTemplate) {
this.usePreviousTemplate = usePreviousTemplate;
}
/**
*
* Use the existing template that's associated with the stack set that you're updating.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*
* @return Use the existing template that's associated with the stack set that you're updating.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*/
public Boolean getUsePreviousTemplate() {
return this.usePreviousTemplate;
}
/**
*
* Use the existing template that's associated with the stack set that you're updating.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*
* @param usePreviousTemplate
* Use the existing template that's associated with the stack set that you're updating.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withUsePreviousTemplate(Boolean usePreviousTemplate) {
setUsePreviousTemplate(usePreviousTemplate);
return this;
}
/**
*
* Use the existing template that's associated with the stack set that you're updating.
*
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*
*
* @return Use the existing template that's associated with the stack set that you're updating.
*
* Conditional: You must specify only one of the following parameters: TemplateBody
or
* TemplateURL
—or set UsePreviousTemplate
to true.
*/
public Boolean isUsePreviousTemplate() {
return this.usePreviousTemplate;
}
/**
*
* A list of input parameters for the stack set template.
*
*
* @return A list of input parameters for the stack set template.
*/
public java.util.List getParameters() {
if (parameters == null) {
parameters = new com.amazonaws.internal.SdkInternalList();
}
return parameters;
}
/**
*
* A list of input parameters for the stack set template.
*
*
* @param parameters
* A list of input parameters for the stack set template.
*/
public void setParameters(java.util.Collection parameters) {
if (parameters == null) {
this.parameters = null;
return;
}
this.parameters = new com.amazonaws.internal.SdkInternalList(parameters);
}
/**
*
* A list of input parameters for the stack set template.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setParameters(java.util.Collection)} or {@link #withParameters(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param parameters
* A list of input parameters for the stack set template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withParameters(Parameter... parameters) {
if (this.parameters == null) {
setParameters(new com.amazonaws.internal.SdkInternalList(parameters.length));
}
for (Parameter ele : parameters) {
this.parameters.add(ele);
}
return this;
}
/**
*
* A list of input parameters for the stack set template.
*
*
* @param parameters
* A list of input parameters for the stack set template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withParameters(java.util.Collection parameters) {
setParameters(parameters);
return this;
}
/**
*
* A list of values that you must specify before AWS CloudFormation can create certain stack sets. Some stack set
* templates might include resources that can affect permissions in your AWS account—for example, by creating new
* AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly acknowledge their
* capabilities by specifying this parameter.
*
*
* The only valid values are CAPABILITY_IAM and CAPABILITY_NAMED_IAM. The following resources require you to specify
* this parameter:
*
*
* -
*
* AWS::IAM::AccessKey
*
*
* -
*
* AWS::IAM::Group
*
*
* -
*
* AWS::IAM::InstanceProfile
*
*
* -
*
* AWS::IAM::Policy
*
*
* -
*
* AWS::IAM::Role
*
*
* -
*
* AWS::IAM::User
*
*
* -
*
* AWS::IAM::UserToGroupAddition
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions that are associated
* with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom names, you
* must specify CAPABILITY_NAMED_IAM. If you don't specify this parameter, this action returns an
* InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* @return A list of values that you must specify before AWS CloudFormation can create certain stack sets. Some
* stack set templates might include resources that can affect permissions in your AWS account—for example,
* by creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge their capabilities by specifying this parameter.
*
* The only valid values are CAPABILITY_IAM and CAPABILITY_NAMED_IAM. The following resources require you to
* specify this parameter:
*
*
* -
*
* AWS::IAM::AccessKey
*
*
* -
*
* AWS::IAM::Group
*
*
* -
*
* AWS::IAM::InstanceProfile
*
*
* -
*
* AWS::IAM::Policy
*
*
* -
*
* AWS::IAM::Role
*
*
* -
*
* AWS::IAM::User
*
*
* -
*
* AWS::IAM::UserToGroupAddition
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions that are
* associated with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom
* names, you must specify CAPABILITY_NAMED_IAM. If you don't specify this parameter, this action returns an
* InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
* @see Capability
*/
public java.util.List getCapabilities() {
if (capabilities == null) {
capabilities = new com.amazonaws.internal.SdkInternalList();
}
return capabilities;
}
/**
*
* A list of values that you must specify before AWS CloudFormation can create certain stack sets. Some stack set
* templates might include resources that can affect permissions in your AWS account—for example, by creating new
* AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly acknowledge their
* capabilities by specifying this parameter.
*
*
* The only valid values are CAPABILITY_IAM and CAPABILITY_NAMED_IAM. The following resources require you to specify
* this parameter:
*
*
* -
*
* AWS::IAM::AccessKey
*
*
* -
*
* AWS::IAM::Group
*
*
* -
*
* AWS::IAM::InstanceProfile
*
*
* -
*
* AWS::IAM::Policy
*
*
* -
*
* AWS::IAM::Role
*
*
* -
*
* AWS::IAM::User
*
*
* -
*
* AWS::IAM::UserToGroupAddition
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions that are associated
* with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom names, you
* must specify CAPABILITY_NAMED_IAM. If you don't specify this parameter, this action returns an
* InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* @param capabilities
* A list of values that you must specify before AWS CloudFormation can create certain stack sets. Some stack
* set templates might include resources that can affect permissions in your AWS account—for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge their capabilities by specifying this parameter.
*
* The only valid values are CAPABILITY_IAM and CAPABILITY_NAMED_IAM. The following resources require you to
* specify this parameter:
*
*
* -
*
* AWS::IAM::AccessKey
*
*
* -
*
* AWS::IAM::Group
*
*
* -
*
* AWS::IAM::InstanceProfile
*
*
* -
*
* AWS::IAM::Policy
*
*
* -
*
* AWS::IAM::Role
*
*
* -
*
* AWS::IAM::User
*
*
* -
*
* AWS::IAM::UserToGroupAddition
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions that are
* associated with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom names,
* you must specify CAPABILITY_NAMED_IAM. If you don't specify this parameter, this action returns an
* InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
* @see Capability
*/
public void setCapabilities(java.util.Collection capabilities) {
if (capabilities == null) {
this.capabilities = null;
return;
}
this.capabilities = new com.amazonaws.internal.SdkInternalList(capabilities);
}
/**
*
* A list of values that you must specify before AWS CloudFormation can create certain stack sets. Some stack set
* templates might include resources that can affect permissions in your AWS account—for example, by creating new
* AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly acknowledge their
* capabilities by specifying this parameter.
*
*
* The only valid values are CAPABILITY_IAM and CAPABILITY_NAMED_IAM. The following resources require you to specify
* this parameter:
*
*
* -
*
* AWS::IAM::AccessKey
*
*
* -
*
* AWS::IAM::Group
*
*
* -
*
* AWS::IAM::InstanceProfile
*
*
* -
*
* AWS::IAM::Policy
*
*
* -
*
* AWS::IAM::Role
*
*
* -
*
* AWS::IAM::User
*
*
* -
*
* AWS::IAM::UserToGroupAddition
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions that are associated
* with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom names, you
* must specify CAPABILITY_NAMED_IAM. If you don't specify this parameter, this action returns an
* InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCapabilities(java.util.Collection)} or {@link #withCapabilities(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param capabilities
* A list of values that you must specify before AWS CloudFormation can create certain stack sets. Some stack
* set templates might include resources that can affect permissions in your AWS account—for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge their capabilities by specifying this parameter.
*
* The only valid values are CAPABILITY_IAM and CAPABILITY_NAMED_IAM. The following resources require you to
* specify this parameter:
*
*
* -
*
* AWS::IAM::AccessKey
*
*
* -
*
* AWS::IAM::Group
*
*
* -
*
* AWS::IAM::InstanceProfile
*
*
* -
*
* AWS::IAM::Policy
*
*
* -
*
* AWS::IAM::Role
*
*
* -
*
* AWS::IAM::User
*
*
* -
*
* AWS::IAM::UserToGroupAddition
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions that are
* associated with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom names,
* you must specify CAPABILITY_NAMED_IAM. If you don't specify this parameter, this action returns an
* InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Capability
*/
public UpdateStackSetRequest withCapabilities(String... capabilities) {
if (this.capabilities == null) {
setCapabilities(new com.amazonaws.internal.SdkInternalList(capabilities.length));
}
for (String ele : capabilities) {
this.capabilities.add(ele);
}
return this;
}
/**
*
* A list of values that you must specify before AWS CloudFormation can create certain stack sets. Some stack set
* templates might include resources that can affect permissions in your AWS account—for example, by creating new
* AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly acknowledge their
* capabilities by specifying this parameter.
*
*
* The only valid values are CAPABILITY_IAM and CAPABILITY_NAMED_IAM. The following resources require you to specify
* this parameter:
*
*
* -
*
* AWS::IAM::AccessKey
*
*
* -
*
* AWS::IAM::Group
*
*
* -
*
* AWS::IAM::InstanceProfile
*
*
* -
*
* AWS::IAM::Policy
*
*
* -
*
* AWS::IAM::Role
*
*
* -
*
* AWS::IAM::User
*
*
* -
*
* AWS::IAM::UserToGroupAddition
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions that are associated
* with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom names, you
* must specify CAPABILITY_NAMED_IAM. If you don't specify this parameter, this action returns an
* InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* @param capabilities
* A list of values that you must specify before AWS CloudFormation can create certain stack sets. Some stack
* set templates might include resources that can affect permissions in your AWS account—for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge their capabilities by specifying this parameter.
*
* The only valid values are CAPABILITY_IAM and CAPABILITY_NAMED_IAM. The following resources require you to
* specify this parameter:
*
*
* -
*
* AWS::IAM::AccessKey
*
*
* -
*
* AWS::IAM::Group
*
*
* -
*
* AWS::IAM::InstanceProfile
*
*
* -
*
* AWS::IAM::Policy
*
*
* -
*
* AWS::IAM::Role
*
*
* -
*
* AWS::IAM::User
*
*
* -
*
* AWS::IAM::UserToGroupAddition
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions that are
* associated with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom names,
* you must specify CAPABILITY_NAMED_IAM. If you don't specify this parameter, this action returns an
* InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Capability
*/
public UpdateStackSetRequest withCapabilities(java.util.Collection capabilities) {
setCapabilities(capabilities);
return this;
}
/**
*
* A list of values that you must specify before AWS CloudFormation can create certain stack sets. Some stack set
* templates might include resources that can affect permissions in your AWS account—for example, by creating new
* AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly acknowledge their
* capabilities by specifying this parameter.
*
*
* The only valid values are CAPABILITY_IAM and CAPABILITY_NAMED_IAM. The following resources require you to specify
* this parameter:
*
*
* -
*
* AWS::IAM::AccessKey
*
*
* -
*
* AWS::IAM::Group
*
*
* -
*
* AWS::IAM::InstanceProfile
*
*
* -
*
* AWS::IAM::Policy
*
*
* -
*
* AWS::IAM::Role
*
*
* -
*
* AWS::IAM::User
*
*
* -
*
* AWS::IAM::UserToGroupAddition
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions that are associated
* with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom names, you
* must specify CAPABILITY_NAMED_IAM. If you don't specify this parameter, this action returns an
* InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* @param capabilities
* A list of values that you must specify before AWS CloudFormation can create certain stack sets. Some stack
* set templates might include resources that can affect permissions in your AWS account—for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge their capabilities by specifying this parameter.
*
* The only valid values are CAPABILITY_IAM and CAPABILITY_NAMED_IAM. The following resources require you to
* specify this parameter:
*
*
* -
*
* AWS::IAM::AccessKey
*
*
* -
*
* AWS::IAM::Group
*
*
* -
*
* AWS::IAM::InstanceProfile
*
*
* -
*
* AWS::IAM::Policy
*
*
* -
*
* AWS::IAM::Role
*
*
* -
*
* AWS::IAM::User
*
*
* -
*
* AWS::IAM::UserToGroupAddition
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions that are
* associated with them and edit their permissions if necessary.
*
*
* If you have IAM resources, you can specify either capability. If you have IAM resources with custom names,
* you must specify CAPABILITY_NAMED_IAM. If you don't specify this parameter, this action returns an
* InsufficientCapabilities
error.
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Capability
*/
public UpdateStackSetRequest withCapabilities(Capability... capabilities) {
com.amazonaws.internal.SdkInternalList capabilitiesCopy = new com.amazonaws.internal.SdkInternalList(capabilities.length);
for (Capability value : capabilities) {
capabilitiesCopy.add(value.toString());
}
if (getCapabilities() == null) {
setCapabilities(capabilitiesCopy);
} else {
getCapabilities().addAll(capabilitiesCopy);
}
return this;
}
/**
*
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation also
* propagates these tags to supported resources that are created in the stacks. You can specify a maximum number of
* 50 tags.
*
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently associated with
* this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you want
* associated with this stack set, even tags you've specifed before (for example, when creating the stack set or
* during a previous update of the stack set.). Any tags that you don't include in the updated list of tags are
* removed from the stack set, and therefore from the stacks and resources as well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to see if you
* have the required IAM permission to tag resources. If you omit tags that are currently associated with the stack
* set from the list of tags you specify, AWS CloudFormation assumes that you want to remove those tags from the
* stack set, and checks to see if you have permission to untag resources. If you don't have the necessary
* permission(s), the entire UpdateStackSet
action fails with an access denied
error, and
* the stack set is not updated.
*
*
* @return The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation
* also propagates these tags to supported resources that are created in the stacks. You can specify a
* maximum number of 50 tags.
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently associated
* with this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you want
* associated with this stack set, even tags you've specifed before (for example, when creating the stack
* set or during a previous update of the stack set.). Any tags that you don't include in the updated list
* of tags are removed from the stack set, and therefore from the stacks and resources as well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to
* see if you have the required IAM permission to tag resources. If you omit tags that are currently
* associated with the stack set from the list of tags you specify, AWS CloudFormation assumes that you want
* to remove those tags from the stack set, and checks to see if you have permission to untag resources. If
* you don't have the necessary permission(s), the entire UpdateStackSet
action fails with an
* access denied
error, and the stack set is not updated.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation also
* propagates these tags to supported resources that are created in the stacks. You can specify a maximum number of
* 50 tags.
*
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently associated with
* this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you want
* associated with this stack set, even tags you've specifed before (for example, when creating the stack set or
* during a previous update of the stack set.). Any tags that you don't include in the updated list of tags are
* removed from the stack set, and therefore from the stacks and resources as well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to see if you
* have the required IAM permission to tag resources. If you omit tags that are currently associated with the stack
* set from the list of tags you specify, AWS CloudFormation assumes that you want to remove those tags from the
* stack set, and checks to see if you have permission to untag resources. If you don't have the necessary
* permission(s), the entire UpdateStackSet
action fails with an access denied
error, and
* the stack set is not updated.
*
*
* @param tags
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation
* also propagates these tags to supported resources that are created in the stacks. You can specify a
* maximum number of 50 tags.
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently associated
* with this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you want
* associated with this stack set, even tags you've specifed before (for example, when creating the stack set
* or during a previous update of the stack set.). Any tags that you don't include in the updated list of
* tags are removed from the stack set, and therefore from the stacks and resources as well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to see
* if you have the required IAM permission to tag resources. If you omit tags that are currently associated
* with the stack set from the list of tags you specify, AWS CloudFormation assumes that you want to remove
* those tags from the stack set, and checks to see if you have permission to untag resources. If you don't
* have the necessary permission(s), the entire UpdateStackSet
action fails with an
* access denied
error, and the stack set is not updated.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation also
* propagates these tags to supported resources that are created in the stacks. You can specify a maximum number of
* 50 tags.
*
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently associated with
* this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you want
* associated with this stack set, even tags you've specifed before (for example, when creating the stack set or
* during a previous update of the stack set.). Any tags that you don't include in the updated list of tags are
* removed from the stack set, and therefore from the stacks and resources as well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to see if you
* have the required IAM permission to tag resources. If you omit tags that are currently associated with the stack
* set from the list of tags you specify, AWS CloudFormation assumes that you want to remove those tags from the
* stack set, and checks to see if you have permission to untag resources. If you don't have the necessary
* permission(s), the entire UpdateStackSet
action fails with an access denied
error, and
* the stack set is not updated.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation
* also propagates these tags to supported resources that are created in the stacks. You can specify a
* maximum number of 50 tags.
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently associated
* with this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you want
* associated with this stack set, even tags you've specifed before (for example, when creating the stack set
* or during a previous update of the stack set.). Any tags that you don't include in the updated list of
* tags are removed from the stack set, and therefore from the stacks and resources as well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to see
* if you have the required IAM permission to tag resources. If you omit tags that are currently associated
* with the stack set from the list of tags you specify, AWS CloudFormation assumes that you want to remove
* those tags from the stack set, and checks to see if you have permission to untag resources. If you don't
* have the necessary permission(s), the entire UpdateStackSet
action fails with an
* access denied
error, and the stack set is not updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation also
* propagates these tags to supported resources that are created in the stacks. You can specify a maximum number of
* 50 tags.
*
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently associated with
* this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you want
* associated with this stack set, even tags you've specifed before (for example, when creating the stack set or
* during a previous update of the stack set.). Any tags that you don't include in the updated list of tags are
* removed from the stack set, and therefore from the stacks and resources as well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to see if you
* have the required IAM permission to tag resources. If you omit tags that are currently associated with the stack
* set from the list of tags you specify, AWS CloudFormation assumes that you want to remove those tags from the
* stack set, and checks to see if you have permission to untag resources. If you don't have the necessary
* permission(s), the entire UpdateStackSet
action fails with an access denied
error, and
* the stack set is not updated.
*
*
* @param tags
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation
* also propagates these tags to supported resources that are created in the stacks. You can specify a
* maximum number of 50 tags.
*
* If you specify tags for this parameter, those tags replace any list of tags that are currently associated
* with this stack set. This means:
*
*
* -
*
* If you don't specify this parameter, AWS CloudFormation doesn't modify the stack's tags.
*
*
* -
*
* If you specify any tags using this parameter, you must specify all the tags that you want
* associated with this stack set, even tags you've specifed before (for example, when creating the stack set
* or during a previous update of the stack set.). Any tags that you don't include in the updated list of
* tags are removed from the stack set, and therefore from the stacks and resources as well.
*
*
* -
*
* If you specify an empty value, AWS CloudFormation removes all currently associated tags.
*
*
*
*
* If you specify new tags as part of an UpdateStackSet
action, AWS CloudFormation checks to see
* if you have the required IAM permission to tag resources. If you omit tags that are currently associated
* with the stack set from the list of tags you specify, AWS CloudFormation assumes that you want to remove
* those tags from the stack set, and checks to see if you have permission to untag resources. If you don't
* have the necessary permission(s), the entire UpdateStackSet
action fails with an
* access denied
error, and the stack set is not updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* Preferences for how AWS CloudFormation performs this stack set operation.
*
*
* @param operationPreferences
* Preferences for how AWS CloudFormation performs this stack set operation.
*/
public void setOperationPreferences(StackSetOperationPreferences operationPreferences) {
this.operationPreferences = operationPreferences;
}
/**
*
* Preferences for how AWS CloudFormation performs this stack set operation.
*
*
* @return Preferences for how AWS CloudFormation performs this stack set operation.
*/
public StackSetOperationPreferences getOperationPreferences() {
return this.operationPreferences;
}
/**
*
* Preferences for how AWS CloudFormation performs this stack set operation.
*
*
* @param operationPreferences
* Preferences for how AWS CloudFormation performs this stack set operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withOperationPreferences(StackSetOperationPreferences operationPreferences) {
setOperationPreferences(operationPreferences);
return this;
}
/**
*
* The Amazon Resource Number (ARN) of the IAM role to use to update this stack set.
*
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups can
* manage specific stack sets within the same administrator account. For more information, see Define Permissions
* for Multiple Administrators in the AWS CloudFormation User Guide.
*
*
* If you specify a customized administrator role, AWS CloudFormation uses that role to update the stack. If you do
* not specify a customized administrator role, AWS CloudFormation performs the update using the role previously
* associated with the stack set, so long as you have permissions to perform operations on the stack set.
*
*
* @param administrationRoleARN
* The Amazon Resource Number (ARN) of the IAM role to use to update this stack set.
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups
* can manage specific stack sets within the same administrator account. For more information, see Define
* Permissions for Multiple Administrators in the AWS CloudFormation User Guide.
*
*
* If you specify a customized administrator role, AWS CloudFormation uses that role to update the stack. If
* you do not specify a customized administrator role, AWS CloudFormation performs the update using the role
* previously associated with the stack set, so long as you have permissions to perform operations on the
* stack set.
*/
public void setAdministrationRoleARN(String administrationRoleARN) {
this.administrationRoleARN = administrationRoleARN;
}
/**
*
* The Amazon Resource Number (ARN) of the IAM role to use to update this stack set.
*
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups can
* manage specific stack sets within the same administrator account. For more information, see Define Permissions
* for Multiple Administrators in the AWS CloudFormation User Guide.
*
*
* If you specify a customized administrator role, AWS CloudFormation uses that role to update the stack. If you do
* not specify a customized administrator role, AWS CloudFormation performs the update using the role previously
* associated with the stack set, so long as you have permissions to perform operations on the stack set.
*
*
* @return The Amazon Resource Number (ARN) of the IAM role to use to update this stack set.
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups
* can manage specific stack sets within the same administrator account. For more information, see Define
* Permissions for Multiple Administrators in the AWS CloudFormation User Guide.
*
*
* If you specify a customized administrator role, AWS CloudFormation uses that role to update the stack. If
* you do not specify a customized administrator role, AWS CloudFormation performs the update using the role
* previously associated with the stack set, so long as you have permissions to perform operations on the
* stack set.
*/
public String getAdministrationRoleARN() {
return this.administrationRoleARN;
}
/**
*
* The Amazon Resource Number (ARN) of the IAM role to use to update this stack set.
*
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups can
* manage specific stack sets within the same administrator account. For more information, see Define Permissions
* for Multiple Administrators in the AWS CloudFormation User Guide.
*
*
* If you specify a customized administrator role, AWS CloudFormation uses that role to update the stack. If you do
* not specify a customized administrator role, AWS CloudFormation performs the update using the role previously
* associated with the stack set, so long as you have permissions to perform operations on the stack set.
*
*
* @param administrationRoleARN
* The Amazon Resource Number (ARN) of the IAM role to use to update this stack set.
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups
* can manage specific stack sets within the same administrator account. For more information, see Define
* Permissions for Multiple Administrators in the AWS CloudFormation User Guide.
*
*
* If you specify a customized administrator role, AWS CloudFormation uses that role to update the stack. If
* you do not specify a customized administrator role, AWS CloudFormation performs the update using the role
* previously associated with the stack set, so long as you have permissions to perform operations on the
* stack set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withAdministrationRoleARN(String administrationRoleARN) {
setAdministrationRoleARN(administrationRoleARN);
return this;
}
/**
*
* The name of the IAM execution role to use to update the stack set. If you do not specify an execution role, AWS
* CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack set operation.
*
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources users and
* groups can include in their stack sets.
*
*
* If you specify a customized execution role, AWS CloudFormation uses that role to update the stack. If you do not
* specify a customized execution role, AWS CloudFormation performs the update using the role previously associated
* with the stack set, so long as you have permissions to perform operations on the stack set.
*
*
* @param executionRoleName
* The name of the IAM execution role to use to update the stack set. If you do not specify an execution
* role, AWS CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack
* set operation.
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources
* users and groups can include in their stack sets.
*
*
* If you specify a customized execution role, AWS CloudFormation uses that role to update the stack. If you
* do not specify a customized execution role, AWS CloudFormation performs the update using the role
* previously associated with the stack set, so long as you have permissions to perform operations on the
* stack set.
*/
public void setExecutionRoleName(String executionRoleName) {
this.executionRoleName = executionRoleName;
}
/**
*
* The name of the IAM execution role to use to update the stack set. If you do not specify an execution role, AWS
* CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack set operation.
*
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources users and
* groups can include in their stack sets.
*
*
* If you specify a customized execution role, AWS CloudFormation uses that role to update the stack. If you do not
* specify a customized execution role, AWS CloudFormation performs the update using the role previously associated
* with the stack set, so long as you have permissions to perform operations on the stack set.
*
*
* @return The name of the IAM execution role to use to update the stack set. If you do not specify an execution
* role, AWS CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack
* set operation.
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources
* users and groups can include in their stack sets.
*
*
* If you specify a customized execution role, AWS CloudFormation uses that role to update the stack. If you
* do not specify a customized execution role, AWS CloudFormation performs the update using the role
* previously associated with the stack set, so long as you have permissions to perform operations on the
* stack set.
*/
public String getExecutionRoleName() {
return this.executionRoleName;
}
/**
*
* The name of the IAM execution role to use to update the stack set. If you do not specify an execution role, AWS
* CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack set operation.
*
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources users and
* groups can include in their stack sets.
*
*
* If you specify a customized execution role, AWS CloudFormation uses that role to update the stack. If you do not
* specify a customized execution role, AWS CloudFormation performs the update using the role previously associated
* with the stack set, so long as you have permissions to perform operations on the stack set.
*
*
* @param executionRoleName
* The name of the IAM execution role to use to update the stack set. If you do not specify an execution
* role, AWS CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack
* set operation.
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources
* users and groups can include in their stack sets.
*
*
* If you specify a customized execution role, AWS CloudFormation uses that role to update the stack. If you
* do not specify a customized execution role, AWS CloudFormation performs the update using the role
* previously associated with the stack set, so long as you have permissions to perform operations on the
* stack set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withExecutionRoleName(String executionRoleName) {
setExecutionRoleName(executionRoleName);
return this;
}
/**
*
* The unique ID for this stack set operation.
*
*
* The operation ID also functions as an idempotency token, to ensure that AWS CloudFormation performs the stack set
* operation only once, even if you retry the request multiple times. You might retry stack set operation requests
* to ensure that AWS CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, AWS CloudFormation generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*
*
* @param operationId
* The unique ID for this stack set operation.
*
* The operation ID also functions as an idempotency token, to ensure that AWS CloudFormation performs the
* stack set operation only once, even if you retry the request multiple times. You might retry stack set
* operation requests to ensure that AWS CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, AWS CloudFormation generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*/
public void setOperationId(String operationId) {
this.operationId = operationId;
}
/**
*
* The unique ID for this stack set operation.
*
*
* The operation ID also functions as an idempotency token, to ensure that AWS CloudFormation performs the stack set
* operation only once, even if you retry the request multiple times. You might retry stack set operation requests
* to ensure that AWS CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, AWS CloudFormation generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*
*
* @return The unique ID for this stack set operation.
*
* The operation ID also functions as an idempotency token, to ensure that AWS CloudFormation performs the
* stack set operation only once, even if you retry the request multiple times. You might retry stack set
* operation requests to ensure that AWS CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, AWS CloudFormation generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*/
public String getOperationId() {
return this.operationId;
}
/**
*
* The unique ID for this stack set operation.
*
*
* The operation ID also functions as an idempotency token, to ensure that AWS CloudFormation performs the stack set
* operation only once, even if you retry the request multiple times. You might retry stack set operation requests
* to ensure that AWS CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, AWS CloudFormation generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*
*
* @param operationId
* The unique ID for this stack set operation.
*
* The operation ID also functions as an idempotency token, to ensure that AWS CloudFormation performs the
* stack set operation only once, even if you retry the request multiple times. You might retry stack set
* operation requests to ensure that AWS CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, AWS CloudFormation generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withOperationId(String operationId) {
setOperationId(operationId);
return this;
}
/**
*
* The accounts in which to update associated stack instances. If you specify accounts, you must also specify the
* regions in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the Accounts
* or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS CloudFormation
* marks all stack instances with a status of OUTDATED
prior to updating the stack instances in the
* specified accounts and regions. If the stack set update does not include changes to the template or parameters,
* AWS CloudFormation updates the stack instances in the specified accounts and regions, while leaving all other
* stack instances with their existing stack instance status.
*
*
* @return The accounts in which to update associated stack instances. If you specify accounts, you must also
* specify the regions in which to update stack set instances.
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the
* stack instances in the specified accounts and regions. If the stack set update does not include changes
* to the template or parameters, AWS CloudFormation updates the stack instances in the specified accounts
* and regions, while leaving all other stack instances with their existing stack instance status.
*/
public java.util.List getAccounts() {
if (accounts == null) {
accounts = new com.amazonaws.internal.SdkInternalList();
}
return accounts;
}
/**
*
* The accounts in which to update associated stack instances. If you specify accounts, you must also specify the
* regions in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the Accounts
* or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS CloudFormation
* marks all stack instances with a status of OUTDATED
prior to updating the stack instances in the
* specified accounts and regions. If the stack set update does not include changes to the template or parameters,
* AWS CloudFormation updates the stack instances in the specified accounts and regions, while leaving all other
* stack instances with their existing stack instance status.
*
*
* @param accounts
* The accounts in which to update associated stack instances. If you specify accounts, you must also specify
* the regions in which to update stack set instances.
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the
* stack instances in the specified accounts and regions. If the stack set update does not include changes to
* the template or parameters, AWS CloudFormation updates the stack instances in the specified accounts and
* regions, while leaving all other stack instances with their existing stack instance status.
*/
public void setAccounts(java.util.Collection accounts) {
if (accounts == null) {
this.accounts = null;
return;
}
this.accounts = new com.amazonaws.internal.SdkInternalList(accounts);
}
/**
*
* The accounts in which to update associated stack instances. If you specify accounts, you must also specify the
* regions in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the Accounts
* or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS CloudFormation
* marks all stack instances with a status of OUTDATED
prior to updating the stack instances in the
* specified accounts and regions. If the stack set update does not include changes to the template or parameters,
* AWS CloudFormation updates the stack instances in the specified accounts and regions, while leaving all other
* stack instances with their existing stack instance status.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAccounts(java.util.Collection)} or {@link #withAccounts(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param accounts
* The accounts in which to update associated stack instances. If you specify accounts, you must also specify
* the regions in which to update stack set instances.
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the
* stack instances in the specified accounts and regions. If the stack set update does not include changes to
* the template or parameters, AWS CloudFormation updates the stack instances in the specified accounts and
* regions, while leaving all other stack instances with their existing stack instance status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withAccounts(String... accounts) {
if (this.accounts == null) {
setAccounts(new com.amazonaws.internal.SdkInternalList(accounts.length));
}
for (String ele : accounts) {
this.accounts.add(ele);
}
return this;
}
/**
*
* The accounts in which to update associated stack instances. If you specify accounts, you must also specify the
* regions in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the Accounts
* or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS CloudFormation
* marks all stack instances with a status of OUTDATED
prior to updating the stack instances in the
* specified accounts and regions. If the stack set update does not include changes to the template or parameters,
* AWS CloudFormation updates the stack instances in the specified accounts and regions, while leaving all other
* stack instances with their existing stack instance status.
*
*
* @param accounts
* The accounts in which to update associated stack instances. If you specify accounts, you must also specify
* the regions in which to update stack set instances.
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the
* stack instances in the specified accounts and regions. If the stack set update does not include changes to
* the template or parameters, AWS CloudFormation updates the stack instances in the specified accounts and
* regions, while leaving all other stack instances with their existing stack instance status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withAccounts(java.util.Collection accounts) {
setAccounts(accounts);
return this;
}
/**
*
* The regions in which to update associated stack instances. If you specify regions, you must also specify accounts
* in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the Accounts
* or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS CloudFormation
* marks all stack instances with a status of OUTDATED
prior to updating the stack instances in the
* specified accounts and regions. If the stack set update does not include changes to the template or parameters,
* AWS CloudFormation updates the stack instances in the specified accounts and regions, while leaving all other
* stack instances with their existing stack instance status.
*
*
* @return The regions in which to update associated stack instances. If you specify regions, you must also specify
* accounts in which to update stack set instances.
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the
* stack instances in the specified accounts and regions. If the stack set update does not include changes
* to the template or parameters, AWS CloudFormation updates the stack instances in the specified accounts
* and regions, while leaving all other stack instances with their existing stack instance status.
*/
public java.util.List getRegions() {
if (regions == null) {
regions = new com.amazonaws.internal.SdkInternalList();
}
return regions;
}
/**
*
* The regions in which to update associated stack instances. If you specify regions, you must also specify accounts
* in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the Accounts
* or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS CloudFormation
* marks all stack instances with a status of OUTDATED
prior to updating the stack instances in the
* specified accounts and regions. If the stack set update does not include changes to the template or parameters,
* AWS CloudFormation updates the stack instances in the specified accounts and regions, while leaving all other
* stack instances with their existing stack instance status.
*
*
* @param regions
* The regions in which to update associated stack instances. If you specify regions, you must also specify
* accounts in which to update stack set instances.
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the
* stack instances in the specified accounts and regions. If the stack set update does not include changes to
* the template or parameters, AWS CloudFormation updates the stack instances in the specified accounts and
* regions, while leaving all other stack instances with their existing stack instance status.
*/
public void setRegions(java.util.Collection regions) {
if (regions == null) {
this.regions = null;
return;
}
this.regions = new com.amazonaws.internal.SdkInternalList(regions);
}
/**
*
* The regions in which to update associated stack instances. If you specify regions, you must also specify accounts
* in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the Accounts
* or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS CloudFormation
* marks all stack instances with a status of OUTDATED
prior to updating the stack instances in the
* specified accounts and regions. If the stack set update does not include changes to the template or parameters,
* AWS CloudFormation updates the stack instances in the specified accounts and regions, while leaving all other
* stack instances with their existing stack instance status.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRegions(java.util.Collection)} or {@link #withRegions(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param regions
* The regions in which to update associated stack instances. If you specify regions, you must also specify
* accounts in which to update stack set instances.
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the
* stack instances in the specified accounts and regions. If the stack set update does not include changes to
* the template or parameters, AWS CloudFormation updates the stack instances in the specified accounts and
* regions, while leaving all other stack instances with their existing stack instance status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withRegions(String... regions) {
if (this.regions == null) {
setRegions(new com.amazonaws.internal.SdkInternalList(regions.length));
}
for (String ele : regions) {
this.regions.add(ele);
}
return this;
}
/**
*
* The regions in which to update associated stack instances. If you specify regions, you must also specify accounts
* in which to update stack set instances.
*
*
* To update all the stack instances associated with this stack set, do not specify the Accounts
* or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS CloudFormation
* marks all stack instances with a status of OUTDATED
prior to updating the stack instances in the
* specified accounts and regions. If the stack set update does not include changes to the template or parameters,
* AWS CloudFormation updates the stack instances in the specified accounts and regions, while leaving all other
* stack instances with their existing stack instance status.
*
*
* @param regions
* The regions in which to update associated stack instances. If you specify regions, you must also specify
* accounts in which to update stack set instances.
*
* To update all the stack instances associated with this stack set, do not specify the
* Accounts
or Regions
properties.
*
*
* If the stack set update includes changes to the template (that is, if the TemplateBody
or
* TemplateURL
properties are specified), or the Parameters
property, AWS
* CloudFormation marks all stack instances with a status of OUTDATED
prior to updating the
* stack instances in the specified accounts and regions. If the stack set update does not include changes to
* the template or parameters, AWS CloudFormation updates the stack instances in the specified accounts and
* regions, while leaving all other stack instances with their existing stack instance status.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public UpdateStackSetRequest withRegions(java.util.Collection regions) {
setRegions(regions);
return this;
}
/**
* Returns a string representation of this object; useful for testing and debugging.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStackSetName() != null)
sb.append("StackSetName: ").append(getStackSetName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getTemplateBody() != null)
sb.append("TemplateBody: ").append(getTemplateBody()).append(",");
if (getTemplateURL() != null)
sb.append("TemplateURL: ").append(getTemplateURL()).append(",");
if (getUsePreviousTemplate() != null)
sb.append("UsePreviousTemplate: ").append(getUsePreviousTemplate()).append(",");
if (getParameters() != null)
sb.append("Parameters: ").append(getParameters()).append(",");
if (getCapabilities() != null)
sb.append("Capabilities: ").append(getCapabilities()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getOperationPreferences() != null)
sb.append("OperationPreferences: ").append(getOperationPreferences()).append(",");
if (getAdministrationRoleARN() != null)
sb.append("AdministrationRoleARN: ").append(getAdministrationRoleARN()).append(",");
if (getExecutionRoleName() != null)
sb.append("ExecutionRoleName: ").append(getExecutionRoleName()).append(",");
if (getOperationId() != null)
sb.append("OperationId: ").append(getOperationId()).append(",");
if (getAccounts() != null)
sb.append("Accounts: ").append(getAccounts()).append(",");
if (getRegions() != null)
sb.append("Regions: ").append(getRegions());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof UpdateStackSetRequest == false)
return false;
UpdateStackSetRequest other = (UpdateStackSetRequest) obj;
if (other.getStackSetName() == null ^ this.getStackSetName() == null)
return false;
if (other.getStackSetName() != null && other.getStackSetName().equals(this.getStackSetName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getTemplateBody() == null ^ this.getTemplateBody() == null)
return false;
if (other.getTemplateBody() != null && other.getTemplateBody().equals(this.getTemplateBody()) == false)
return false;
if (other.getTemplateURL() == null ^ this.getTemplateURL() == null)
return false;
if (other.getTemplateURL() != null && other.getTemplateURL().equals(this.getTemplateURL()) == false)
return false;
if (other.getUsePreviousTemplate() == null ^ this.getUsePreviousTemplate() == null)
return false;
if (other.getUsePreviousTemplate() != null && other.getUsePreviousTemplate().equals(this.getUsePreviousTemplate()) == false)
return false;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false)
return false;
if (other.getCapabilities() == null ^ this.getCapabilities() == null)
return false;
if (other.getCapabilities() != null && other.getCapabilities().equals(this.getCapabilities()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getOperationPreferences() == null ^ this.getOperationPreferences() == null)
return false;
if (other.getOperationPreferences() != null && other.getOperationPreferences().equals(this.getOperationPreferences()) == false)
return false;
if (other.getAdministrationRoleARN() == null ^ this.getAdministrationRoleARN() == null)
return false;
if (other.getAdministrationRoleARN() != null && other.getAdministrationRoleARN().equals(this.getAdministrationRoleARN()) == false)
return false;
if (other.getExecutionRoleName() == null ^ this.getExecutionRoleName() == null)
return false;
if (other.getExecutionRoleName() != null && other.getExecutionRoleName().equals(this.getExecutionRoleName()) == false)
return false;
if (other.getOperationId() == null ^ this.getOperationId() == null)
return false;
if (other.getOperationId() != null && other.getOperationId().equals(this.getOperationId()) == false)
return false;
if (other.getAccounts() == null ^ this.getAccounts() == null)
return false;
if (other.getAccounts() != null && other.getAccounts().equals(this.getAccounts()) == false)
return false;
if (other.getRegions() == null ^ this.getRegions() == null)
return false;
if (other.getRegions() != null && other.getRegions().equals(this.getRegions()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStackSetName() == null) ? 0 : getStackSetName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getTemplateBody() == null) ? 0 : getTemplateBody().hashCode());
hashCode = prime * hashCode + ((getTemplateURL() == null) ? 0 : getTemplateURL().hashCode());
hashCode = prime * hashCode + ((getUsePreviousTemplate() == null) ? 0 : getUsePreviousTemplate().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
hashCode = prime * hashCode + ((getCapabilities() == null) ? 0 : getCapabilities().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getOperationPreferences() == null) ? 0 : getOperationPreferences().hashCode());
hashCode = prime * hashCode + ((getAdministrationRoleARN() == null) ? 0 : getAdministrationRoleARN().hashCode());
hashCode = prime * hashCode + ((getExecutionRoleName() == null) ? 0 : getExecutionRoleName().hashCode());
hashCode = prime * hashCode + ((getOperationId() == null) ? 0 : getOperationId().hashCode());
hashCode = prime * hashCode + ((getAccounts() == null) ? 0 : getAccounts().hashCode());
hashCode = prime * hashCode + ((getRegions() == null) ? 0 : getRegions().hashCode());
return hashCode;
}
@Override
public UpdateStackSetRequest clone() {
return (UpdateStackSetRequest) super.clone();
}
}