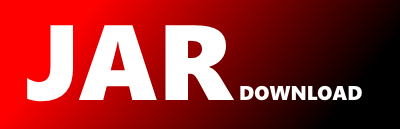
com.amazonaws.services.cloudformation.model.ResourceChangeDetail Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudformation.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* For a resource with Modify
as the action, the ResourceChange
structure describes the
* changes AWS CloudFormation will make to that resource.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ResourceChangeDetail implements Serializable, Cloneable {
/**
*
* A ResourceTargetDefinition
structure that describes the field that AWS CloudFormation will change
* and whether the resource will be recreated.
*
*/
private ResourceTargetDefinition target;
/**
*
* Indicates whether AWS CloudFormation can determine the target value, and whether the target value will change
* before you execute a change set.
*
*
* For Static
evaluations, AWS CloudFormation can determine that the target value will change, and its
* value. For example, if you directly modify the InstanceType
property of an EC2 instance, AWS
* CloudFormation knows that this property value will change, and its value, so this is a Static
* evaluation.
*
*
* For Dynamic
evaluations, cannot determine the target value because it depends on the result of an
* intrinsic function, such as a Ref
or Fn::GetAtt
intrinsic function, when the stack is
* updated. For example, if your template includes a reference to a resource that is conditionally recreated, the
* value of the reference (the physical ID of the resource) might change, depending on if the resource is recreated.
* If the resource is recreated, it will have a new physical ID, so all references to that resource will also be
* updated.
*
*/
private String evaluation;
/**
*
* The group to which the CausingEntity
value belongs. There are five entity groups:
*
*
* -
*
* ResourceReference
entities are Ref
intrinsic functions that refer to resources in the
* template, such as { "Ref" : "MyEC2InstanceResource" }
.
*
*
* -
*
* ParameterReference
entities are Ref
intrinsic functions that get template parameter
* values, such as { "Ref" : "MyPasswordParameter" }
.
*
*
* -
*
* ResourceAttribute
entities are Fn::GetAtt
intrinsic functions that get resource
* attribute values, such as { "Fn::GetAtt" : [ "MyEC2InstanceResource", "PublicDnsName" ] }
.
*
*
* -
*
* DirectModification
entities are changes that are made directly to the template.
*
*
* -
*
* Automatic
entities are AWS::CloudFormation::Stack
resource types, which are also known
* as nested stacks. If you made no changes to the AWS::CloudFormation::Stack
resource, AWS
* CloudFormation sets the ChangeSource
to Automatic
because the nested stack's template
* might have changed. Changes to a nested stack's template aren't visible to AWS CloudFormation until you run an
* update on the parent stack.
*
*
*
*/
private String changeSource;
/**
*
* The identity of the entity that triggered this change. This entity is a member of the group that is specified by
* the ChangeSource
field. For example, if you modified the value of the KeyPairName
* parameter, the CausingEntity
is the name of the parameter (KeyPairName
).
*
*
* If the ChangeSource
value is DirectModification
, no value is given for
* CausingEntity
.
*
*/
private String causingEntity;
/**
*
* A ResourceTargetDefinition
structure that describes the field that AWS CloudFormation will change
* and whether the resource will be recreated.
*
*
* @param target
* A ResourceTargetDefinition
structure that describes the field that AWS CloudFormation will
* change and whether the resource will be recreated.
*/
public void setTarget(ResourceTargetDefinition target) {
this.target = target;
}
/**
*
* A ResourceTargetDefinition
structure that describes the field that AWS CloudFormation will change
* and whether the resource will be recreated.
*
*
* @return A ResourceTargetDefinition
structure that describes the field that AWS CloudFormation will
* change and whether the resource will be recreated.
*/
public ResourceTargetDefinition getTarget() {
return this.target;
}
/**
*
* A ResourceTargetDefinition
structure that describes the field that AWS CloudFormation will change
* and whether the resource will be recreated.
*
*
* @param target
* A ResourceTargetDefinition
structure that describes the field that AWS CloudFormation will
* change and whether the resource will be recreated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceChangeDetail withTarget(ResourceTargetDefinition target) {
setTarget(target);
return this;
}
/**
*
* Indicates whether AWS CloudFormation can determine the target value, and whether the target value will change
* before you execute a change set.
*
*
* For Static
evaluations, AWS CloudFormation can determine that the target value will change, and its
* value. For example, if you directly modify the InstanceType
property of an EC2 instance, AWS
* CloudFormation knows that this property value will change, and its value, so this is a Static
* evaluation.
*
*
* For Dynamic
evaluations, cannot determine the target value because it depends on the result of an
* intrinsic function, such as a Ref
or Fn::GetAtt
intrinsic function, when the stack is
* updated. For example, if your template includes a reference to a resource that is conditionally recreated, the
* value of the reference (the physical ID of the resource) might change, depending on if the resource is recreated.
* If the resource is recreated, it will have a new physical ID, so all references to that resource will also be
* updated.
*
*
* @param evaluation
* Indicates whether AWS CloudFormation can determine the target value, and whether the target value will
* change before you execute a change set.
*
* For Static
evaluations, AWS CloudFormation can determine that the target value will change,
* and its value. For example, if you directly modify the InstanceType
property of an EC2
* instance, AWS CloudFormation knows that this property value will change, and its value, so this is a
* Static
evaluation.
*
*
* For Dynamic
evaluations, cannot determine the target value because it depends on the result
* of an intrinsic function, such as a Ref
or Fn::GetAtt
intrinsic function, when
* the stack is updated. For example, if your template includes a reference to a resource that is
* conditionally recreated, the value of the reference (the physical ID of the resource) might change,
* depending on if the resource is recreated. If the resource is recreated, it will have a new physical ID,
* so all references to that resource will also be updated.
* @see EvaluationType
*/
public void setEvaluation(String evaluation) {
this.evaluation = evaluation;
}
/**
*
* Indicates whether AWS CloudFormation can determine the target value, and whether the target value will change
* before you execute a change set.
*
*
* For Static
evaluations, AWS CloudFormation can determine that the target value will change, and its
* value. For example, if you directly modify the InstanceType
property of an EC2 instance, AWS
* CloudFormation knows that this property value will change, and its value, so this is a Static
* evaluation.
*
*
* For Dynamic
evaluations, cannot determine the target value because it depends on the result of an
* intrinsic function, such as a Ref
or Fn::GetAtt
intrinsic function, when the stack is
* updated. For example, if your template includes a reference to a resource that is conditionally recreated, the
* value of the reference (the physical ID of the resource) might change, depending on if the resource is recreated.
* If the resource is recreated, it will have a new physical ID, so all references to that resource will also be
* updated.
*
*
* @return Indicates whether AWS CloudFormation can determine the target value, and whether the target value will
* change before you execute a change set.
*
* For Static
evaluations, AWS CloudFormation can determine that the target value will change,
* and its value. For example, if you directly modify the InstanceType
property of an EC2
* instance, AWS CloudFormation knows that this property value will change, and its value, so this is a
* Static
evaluation.
*
*
* For Dynamic
evaluations, cannot determine the target value because it depends on the result
* of an intrinsic function, such as a Ref
or Fn::GetAtt
intrinsic function, when
* the stack is updated. For example, if your template includes a reference to a resource that is
* conditionally recreated, the value of the reference (the physical ID of the resource) might change,
* depending on if the resource is recreated. If the resource is recreated, it will have a new physical ID,
* so all references to that resource will also be updated.
* @see EvaluationType
*/
public String getEvaluation() {
return this.evaluation;
}
/**
*
* Indicates whether AWS CloudFormation can determine the target value, and whether the target value will change
* before you execute a change set.
*
*
* For Static
evaluations, AWS CloudFormation can determine that the target value will change, and its
* value. For example, if you directly modify the InstanceType
property of an EC2 instance, AWS
* CloudFormation knows that this property value will change, and its value, so this is a Static
* evaluation.
*
*
* For Dynamic
evaluations, cannot determine the target value because it depends on the result of an
* intrinsic function, such as a Ref
or Fn::GetAtt
intrinsic function, when the stack is
* updated. For example, if your template includes a reference to a resource that is conditionally recreated, the
* value of the reference (the physical ID of the resource) might change, depending on if the resource is recreated.
* If the resource is recreated, it will have a new physical ID, so all references to that resource will also be
* updated.
*
*
* @param evaluation
* Indicates whether AWS CloudFormation can determine the target value, and whether the target value will
* change before you execute a change set.
*
* For Static
evaluations, AWS CloudFormation can determine that the target value will change,
* and its value. For example, if you directly modify the InstanceType
property of an EC2
* instance, AWS CloudFormation knows that this property value will change, and its value, so this is a
* Static
evaluation.
*
*
* For Dynamic
evaluations, cannot determine the target value because it depends on the result
* of an intrinsic function, such as a Ref
or Fn::GetAtt
intrinsic function, when
* the stack is updated. For example, if your template includes a reference to a resource that is
* conditionally recreated, the value of the reference (the physical ID of the resource) might change,
* depending on if the resource is recreated. If the resource is recreated, it will have a new physical ID,
* so all references to that resource will also be updated.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EvaluationType
*/
public ResourceChangeDetail withEvaluation(String evaluation) {
setEvaluation(evaluation);
return this;
}
/**
*
* Indicates whether AWS CloudFormation can determine the target value, and whether the target value will change
* before you execute a change set.
*
*
* For Static
evaluations, AWS CloudFormation can determine that the target value will change, and its
* value. For example, if you directly modify the InstanceType
property of an EC2 instance, AWS
* CloudFormation knows that this property value will change, and its value, so this is a Static
* evaluation.
*
*
* For Dynamic
evaluations, cannot determine the target value because it depends on the result of an
* intrinsic function, such as a Ref
or Fn::GetAtt
intrinsic function, when the stack is
* updated. For example, if your template includes a reference to a resource that is conditionally recreated, the
* value of the reference (the physical ID of the resource) might change, depending on if the resource is recreated.
* If the resource is recreated, it will have a new physical ID, so all references to that resource will also be
* updated.
*
*
* @param evaluation
* Indicates whether AWS CloudFormation can determine the target value, and whether the target value will
* change before you execute a change set.
*
* For Static
evaluations, AWS CloudFormation can determine that the target value will change,
* and its value. For example, if you directly modify the InstanceType
property of an EC2
* instance, AWS CloudFormation knows that this property value will change, and its value, so this is a
* Static
evaluation.
*
*
* For Dynamic
evaluations, cannot determine the target value because it depends on the result
* of an intrinsic function, such as a Ref
or Fn::GetAtt
intrinsic function, when
* the stack is updated. For example, if your template includes a reference to a resource that is
* conditionally recreated, the value of the reference (the physical ID of the resource) might change,
* depending on if the resource is recreated. If the resource is recreated, it will have a new physical ID,
* so all references to that resource will also be updated.
* @see EvaluationType
*/
public void setEvaluation(EvaluationType evaluation) {
withEvaluation(evaluation);
}
/**
*
* Indicates whether AWS CloudFormation can determine the target value, and whether the target value will change
* before you execute a change set.
*
*
* For Static
evaluations, AWS CloudFormation can determine that the target value will change, and its
* value. For example, if you directly modify the InstanceType
property of an EC2 instance, AWS
* CloudFormation knows that this property value will change, and its value, so this is a Static
* evaluation.
*
*
* For Dynamic
evaluations, cannot determine the target value because it depends on the result of an
* intrinsic function, such as a Ref
or Fn::GetAtt
intrinsic function, when the stack is
* updated. For example, if your template includes a reference to a resource that is conditionally recreated, the
* value of the reference (the physical ID of the resource) might change, depending on if the resource is recreated.
* If the resource is recreated, it will have a new physical ID, so all references to that resource will also be
* updated.
*
*
* @param evaluation
* Indicates whether AWS CloudFormation can determine the target value, and whether the target value will
* change before you execute a change set.
*
* For Static
evaluations, AWS CloudFormation can determine that the target value will change,
* and its value. For example, if you directly modify the InstanceType
property of an EC2
* instance, AWS CloudFormation knows that this property value will change, and its value, so this is a
* Static
evaluation.
*
*
* For Dynamic
evaluations, cannot determine the target value because it depends on the result
* of an intrinsic function, such as a Ref
or Fn::GetAtt
intrinsic function, when
* the stack is updated. For example, if your template includes a reference to a resource that is
* conditionally recreated, the value of the reference (the physical ID of the resource) might change,
* depending on if the resource is recreated. If the resource is recreated, it will have a new physical ID,
* so all references to that resource will also be updated.
* @return Returns a reference to this object so that method calls can be chained together.
* @see EvaluationType
*/
public ResourceChangeDetail withEvaluation(EvaluationType evaluation) {
this.evaluation = evaluation.toString();
return this;
}
/**
*
* The group to which the CausingEntity
value belongs. There are five entity groups:
*
*
* -
*
* ResourceReference
entities are Ref
intrinsic functions that refer to resources in the
* template, such as { "Ref" : "MyEC2InstanceResource" }
.
*
*
* -
*
* ParameterReference
entities are Ref
intrinsic functions that get template parameter
* values, such as { "Ref" : "MyPasswordParameter" }
.
*
*
* -
*
* ResourceAttribute
entities are Fn::GetAtt
intrinsic functions that get resource
* attribute values, such as { "Fn::GetAtt" : [ "MyEC2InstanceResource", "PublicDnsName" ] }
.
*
*
* -
*
* DirectModification
entities are changes that are made directly to the template.
*
*
* -
*
* Automatic
entities are AWS::CloudFormation::Stack
resource types, which are also known
* as nested stacks. If you made no changes to the AWS::CloudFormation::Stack
resource, AWS
* CloudFormation sets the ChangeSource
to Automatic
because the nested stack's template
* might have changed. Changes to a nested stack's template aren't visible to AWS CloudFormation until you run an
* update on the parent stack.
*
*
*
*
* @param changeSource
* The group to which the CausingEntity
value belongs. There are five entity groups:
*
* -
*
* ResourceReference
entities are Ref
intrinsic functions that refer to resources
* in the template, such as { "Ref" : "MyEC2InstanceResource" }
.
*
*
* -
*
* ParameterReference
entities are Ref
intrinsic functions that get template
* parameter values, such as { "Ref" : "MyPasswordParameter" }
.
*
*
* -
*
* ResourceAttribute
entities are Fn::GetAtt
intrinsic functions that get resource
* attribute values, such as { "Fn::GetAtt" : [ "MyEC2InstanceResource", "PublicDnsName" ] }
.
*
*
* -
*
* DirectModification
entities are changes that are made directly to the template.
*
*
* -
*
* Automatic
entities are AWS::CloudFormation::Stack
resource types, which are also
* known as nested stacks. If you made no changes to the AWS::CloudFormation::Stack
resource,
* AWS CloudFormation sets the ChangeSource
to Automatic
because the nested stack's
* template might have changed. Changes to a nested stack's template aren't visible to AWS CloudFormation
* until you run an update on the parent stack.
*
*
* @see ChangeSource
*/
public void setChangeSource(String changeSource) {
this.changeSource = changeSource;
}
/**
*
* The group to which the CausingEntity
value belongs. There are five entity groups:
*
*
* -
*
* ResourceReference
entities are Ref
intrinsic functions that refer to resources in the
* template, such as { "Ref" : "MyEC2InstanceResource" }
.
*
*
* -
*
* ParameterReference
entities are Ref
intrinsic functions that get template parameter
* values, such as { "Ref" : "MyPasswordParameter" }
.
*
*
* -
*
* ResourceAttribute
entities are Fn::GetAtt
intrinsic functions that get resource
* attribute values, such as { "Fn::GetAtt" : [ "MyEC2InstanceResource", "PublicDnsName" ] }
.
*
*
* -
*
* DirectModification
entities are changes that are made directly to the template.
*
*
* -
*
* Automatic
entities are AWS::CloudFormation::Stack
resource types, which are also known
* as nested stacks. If you made no changes to the AWS::CloudFormation::Stack
resource, AWS
* CloudFormation sets the ChangeSource
to Automatic
because the nested stack's template
* might have changed. Changes to a nested stack's template aren't visible to AWS CloudFormation until you run an
* update on the parent stack.
*
*
*
*
* @return The group to which the CausingEntity
value belongs. There are five entity groups:
*
* -
*
* ResourceReference
entities are Ref
intrinsic functions that refer to resources
* in the template, such as { "Ref" : "MyEC2InstanceResource" }
.
*
*
* -
*
* ParameterReference
entities are Ref
intrinsic functions that get template
* parameter values, such as { "Ref" : "MyPasswordParameter" }
.
*
*
* -
*
* ResourceAttribute
entities are Fn::GetAtt
intrinsic functions that get resource
* attribute values, such as { "Fn::GetAtt" : [ "MyEC2InstanceResource", "PublicDnsName" ] }
.
*
*
* -
*
* DirectModification
entities are changes that are made directly to the template.
*
*
* -
*
* Automatic
entities are AWS::CloudFormation::Stack
resource types, which are
* also known as nested stacks. If you made no changes to the AWS::CloudFormation::Stack
* resource, AWS CloudFormation sets the ChangeSource
to Automatic
because the
* nested stack's template might have changed. Changes to a nested stack's template aren't visible to AWS
* CloudFormation until you run an update on the parent stack.
*
*
* @see ChangeSource
*/
public String getChangeSource() {
return this.changeSource;
}
/**
*
* The group to which the CausingEntity
value belongs. There are five entity groups:
*
*
* -
*
* ResourceReference
entities are Ref
intrinsic functions that refer to resources in the
* template, such as { "Ref" : "MyEC2InstanceResource" }
.
*
*
* -
*
* ParameterReference
entities are Ref
intrinsic functions that get template parameter
* values, such as { "Ref" : "MyPasswordParameter" }
.
*
*
* -
*
* ResourceAttribute
entities are Fn::GetAtt
intrinsic functions that get resource
* attribute values, such as { "Fn::GetAtt" : [ "MyEC2InstanceResource", "PublicDnsName" ] }
.
*
*
* -
*
* DirectModification
entities are changes that are made directly to the template.
*
*
* -
*
* Automatic
entities are AWS::CloudFormation::Stack
resource types, which are also known
* as nested stacks. If you made no changes to the AWS::CloudFormation::Stack
resource, AWS
* CloudFormation sets the ChangeSource
to Automatic
because the nested stack's template
* might have changed. Changes to a nested stack's template aren't visible to AWS CloudFormation until you run an
* update on the parent stack.
*
*
*
*
* @param changeSource
* The group to which the CausingEntity
value belongs. There are five entity groups:
*
* -
*
* ResourceReference
entities are Ref
intrinsic functions that refer to resources
* in the template, such as { "Ref" : "MyEC2InstanceResource" }
.
*
*
* -
*
* ParameterReference
entities are Ref
intrinsic functions that get template
* parameter values, such as { "Ref" : "MyPasswordParameter" }
.
*
*
* -
*
* ResourceAttribute
entities are Fn::GetAtt
intrinsic functions that get resource
* attribute values, such as { "Fn::GetAtt" : [ "MyEC2InstanceResource", "PublicDnsName" ] }
.
*
*
* -
*
* DirectModification
entities are changes that are made directly to the template.
*
*
* -
*
* Automatic
entities are AWS::CloudFormation::Stack
resource types, which are also
* known as nested stacks. If you made no changes to the AWS::CloudFormation::Stack
resource,
* AWS CloudFormation sets the ChangeSource
to Automatic
because the nested stack's
* template might have changed. Changes to a nested stack's template aren't visible to AWS CloudFormation
* until you run an update on the parent stack.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChangeSource
*/
public ResourceChangeDetail withChangeSource(String changeSource) {
setChangeSource(changeSource);
return this;
}
/**
*
* The group to which the CausingEntity
value belongs. There are five entity groups:
*
*
* -
*
* ResourceReference
entities are Ref
intrinsic functions that refer to resources in the
* template, such as { "Ref" : "MyEC2InstanceResource" }
.
*
*
* -
*
* ParameterReference
entities are Ref
intrinsic functions that get template parameter
* values, such as { "Ref" : "MyPasswordParameter" }
.
*
*
* -
*
* ResourceAttribute
entities are Fn::GetAtt
intrinsic functions that get resource
* attribute values, such as { "Fn::GetAtt" : [ "MyEC2InstanceResource", "PublicDnsName" ] }
.
*
*
* -
*
* DirectModification
entities are changes that are made directly to the template.
*
*
* -
*
* Automatic
entities are AWS::CloudFormation::Stack
resource types, which are also known
* as nested stacks. If you made no changes to the AWS::CloudFormation::Stack
resource, AWS
* CloudFormation sets the ChangeSource
to Automatic
because the nested stack's template
* might have changed. Changes to a nested stack's template aren't visible to AWS CloudFormation until you run an
* update on the parent stack.
*
*
*
*
* @param changeSource
* The group to which the CausingEntity
value belongs. There are five entity groups:
*
* -
*
* ResourceReference
entities are Ref
intrinsic functions that refer to resources
* in the template, such as { "Ref" : "MyEC2InstanceResource" }
.
*
*
* -
*
* ParameterReference
entities are Ref
intrinsic functions that get template
* parameter values, such as { "Ref" : "MyPasswordParameter" }
.
*
*
* -
*
* ResourceAttribute
entities are Fn::GetAtt
intrinsic functions that get resource
* attribute values, such as { "Fn::GetAtt" : [ "MyEC2InstanceResource", "PublicDnsName" ] }
.
*
*
* -
*
* DirectModification
entities are changes that are made directly to the template.
*
*
* -
*
* Automatic
entities are AWS::CloudFormation::Stack
resource types, which are also
* known as nested stacks. If you made no changes to the AWS::CloudFormation::Stack
resource,
* AWS CloudFormation sets the ChangeSource
to Automatic
because the nested stack's
* template might have changed. Changes to a nested stack's template aren't visible to AWS CloudFormation
* until you run an update on the parent stack.
*
*
* @see ChangeSource
*/
public void setChangeSource(ChangeSource changeSource) {
withChangeSource(changeSource);
}
/**
*
* The group to which the CausingEntity
value belongs. There are five entity groups:
*
*
* -
*
* ResourceReference
entities are Ref
intrinsic functions that refer to resources in the
* template, such as { "Ref" : "MyEC2InstanceResource" }
.
*
*
* -
*
* ParameterReference
entities are Ref
intrinsic functions that get template parameter
* values, such as { "Ref" : "MyPasswordParameter" }
.
*
*
* -
*
* ResourceAttribute
entities are Fn::GetAtt
intrinsic functions that get resource
* attribute values, such as { "Fn::GetAtt" : [ "MyEC2InstanceResource", "PublicDnsName" ] }
.
*
*
* -
*
* DirectModification
entities are changes that are made directly to the template.
*
*
* -
*
* Automatic
entities are AWS::CloudFormation::Stack
resource types, which are also known
* as nested stacks. If you made no changes to the AWS::CloudFormation::Stack
resource, AWS
* CloudFormation sets the ChangeSource
to Automatic
because the nested stack's template
* might have changed. Changes to a nested stack's template aren't visible to AWS CloudFormation until you run an
* update on the parent stack.
*
*
*
*
* @param changeSource
* The group to which the CausingEntity
value belongs. There are five entity groups:
*
* -
*
* ResourceReference
entities are Ref
intrinsic functions that refer to resources
* in the template, such as { "Ref" : "MyEC2InstanceResource" }
.
*
*
* -
*
* ParameterReference
entities are Ref
intrinsic functions that get template
* parameter values, such as { "Ref" : "MyPasswordParameter" }
.
*
*
* -
*
* ResourceAttribute
entities are Fn::GetAtt
intrinsic functions that get resource
* attribute values, such as { "Fn::GetAtt" : [ "MyEC2InstanceResource", "PublicDnsName" ] }
.
*
*
* -
*
* DirectModification
entities are changes that are made directly to the template.
*
*
* -
*
* Automatic
entities are AWS::CloudFormation::Stack
resource types, which are also
* known as nested stacks. If you made no changes to the AWS::CloudFormation::Stack
resource,
* AWS CloudFormation sets the ChangeSource
to Automatic
because the nested stack's
* template might have changed. Changes to a nested stack's template aren't visible to AWS CloudFormation
* until you run an update on the parent stack.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChangeSource
*/
public ResourceChangeDetail withChangeSource(ChangeSource changeSource) {
this.changeSource = changeSource.toString();
return this;
}
/**
*
* The identity of the entity that triggered this change. This entity is a member of the group that is specified by
* the ChangeSource
field. For example, if you modified the value of the KeyPairName
* parameter, the CausingEntity
is the name of the parameter (KeyPairName
).
*
*
* If the ChangeSource
value is DirectModification
, no value is given for
* CausingEntity
.
*
*
* @param causingEntity
* The identity of the entity that triggered this change. This entity is a member of the group that is
* specified by the ChangeSource
field. For example, if you modified the value of the
* KeyPairName
parameter, the CausingEntity
is the name of the parameter (
* KeyPairName
).
*
* If the ChangeSource
value is DirectModification
, no value is given for
* CausingEntity
.
*/
public void setCausingEntity(String causingEntity) {
this.causingEntity = causingEntity;
}
/**
*
* The identity of the entity that triggered this change. This entity is a member of the group that is specified by
* the ChangeSource
field. For example, if you modified the value of the KeyPairName
* parameter, the CausingEntity
is the name of the parameter (KeyPairName
).
*
*
* If the ChangeSource
value is DirectModification
, no value is given for
* CausingEntity
.
*
*
* @return The identity of the entity that triggered this change. This entity is a member of the group that is
* specified by the ChangeSource
field. For example, if you modified the value of the
* KeyPairName
parameter, the CausingEntity
is the name of the parameter (
* KeyPairName
).
*
* If the ChangeSource
value is DirectModification
, no value is given for
* CausingEntity
.
*/
public String getCausingEntity() {
return this.causingEntity;
}
/**
*
* The identity of the entity that triggered this change. This entity is a member of the group that is specified by
* the ChangeSource
field. For example, if you modified the value of the KeyPairName
* parameter, the CausingEntity
is the name of the parameter (KeyPairName
).
*
*
* If the ChangeSource
value is DirectModification
, no value is given for
* CausingEntity
.
*
*
* @param causingEntity
* The identity of the entity that triggered this change. This entity is a member of the group that is
* specified by the ChangeSource
field. For example, if you modified the value of the
* KeyPairName
parameter, the CausingEntity
is the name of the parameter (
* KeyPairName
).
*
* If the ChangeSource
value is DirectModification
, no value is given for
* CausingEntity
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceChangeDetail withCausingEntity(String causingEntity) {
setCausingEntity(causingEntity);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getTarget() != null)
sb.append("Target: ").append(getTarget()).append(",");
if (getEvaluation() != null)
sb.append("Evaluation: ").append(getEvaluation()).append(",");
if (getChangeSource() != null)
sb.append("ChangeSource: ").append(getChangeSource()).append(",");
if (getCausingEntity() != null)
sb.append("CausingEntity: ").append(getCausingEntity());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ResourceChangeDetail == false)
return false;
ResourceChangeDetail other = (ResourceChangeDetail) obj;
if (other.getTarget() == null ^ this.getTarget() == null)
return false;
if (other.getTarget() != null && other.getTarget().equals(this.getTarget()) == false)
return false;
if (other.getEvaluation() == null ^ this.getEvaluation() == null)
return false;
if (other.getEvaluation() != null && other.getEvaluation().equals(this.getEvaluation()) == false)
return false;
if (other.getChangeSource() == null ^ this.getChangeSource() == null)
return false;
if (other.getChangeSource() != null && other.getChangeSource().equals(this.getChangeSource()) == false)
return false;
if (other.getCausingEntity() == null ^ this.getCausingEntity() == null)
return false;
if (other.getCausingEntity() != null && other.getCausingEntity().equals(this.getCausingEntity()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getTarget() == null) ? 0 : getTarget().hashCode());
hashCode = prime * hashCode + ((getEvaluation() == null) ? 0 : getEvaluation().hashCode());
hashCode = prime * hashCode + ((getChangeSource() == null) ? 0 : getChangeSource().hashCode());
hashCode = prime * hashCode + ((getCausingEntity() == null) ? 0 : getCausingEntity().hashCode());
return hashCode;
}
@Override
public ResourceChangeDetail clone() {
try {
return (ResourceChangeDetail) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}