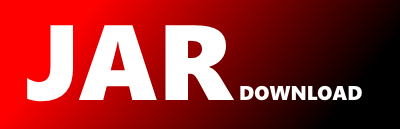
com.amazonaws.services.cloudformation.model.StackResourceDrift Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2013-2018 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudformation.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* Contains the drift information for a resource that has been checked for drift. This includes actual and expected
* property values for resources in which AWS CloudFormation has detected drift. Only resource properties explicitly
* defined in the stack template are checked for drift. For more information, see Detecting Unregulated
* Configuration Changes to Stacks and Resources.
*
*
* Resources that do not currently support drift detection cannot be checked. For a list of resources that support drift
* detection, see Resources that Support Drift Detection.
*
*
* Use DetectStackResourceDrift to detect drift on individual resources, or DetectStackDrift to detect
* drift on all resources in a given stack that support drift detection.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StackResourceDrift implements Serializable, Cloneable {
/**
*
* The ID of the stack.
*
*/
private String stackId;
/**
*
* The logical name of the resource specified in the template.
*
*/
private String logicalResourceId;
/**
*
* The name or unique identifier that corresponds to a physical instance ID of a resource supported by AWS
* CloudFormation.
*
*/
private String physicalResourceId;
/**
*
* Context information that enables AWS CloudFormation to uniquely identify a resource. AWS CloudFormation uses
* context key-value pairs in cases where a resource's logical and physical IDs are not enough to uniquely identify
* that resource. Each context key-value pair specifies a unique resource that contains the targeted resource.
*
*/
private com.amazonaws.internal.SdkInternalList physicalResourceIdContext;
/**
*
* The type of the resource.
*
*/
private String resourceType;
/**
*
* A JSON structure containing the expected property values of the stack resource, as defined in the stack template
* and any values specified as template parameters.
*
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will not be
* present.
*
*/
private String expectedProperties;
/**
*
* A JSON structure containing the actual property values of the stack resource.
*
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will not be
* present.
*
*/
private String actualProperties;
/**
*
* A collection of the resource properties whose actual values differ from their expected values. These will be
* present only for resources whose StackResourceDriftStatus
is MODIFIED
.
*
*/
private com.amazonaws.internal.SdkInternalList propertyDifferences;
/**
*
* Status of the resource's actual configuration compared to its expected configuration
*
*
* -
*
* DELETED
: The resource differs from its expected template configuration because the resource has been
* deleted.
*
*
* -
*
* MODIFIED
: One or more resource properties differ from their expected values (as defined in the stack
* template and any values specified as template parameters).
*
*
* -
*
* IN_SYNC
: The resources's actual configuration matches its expected template configuration.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation does not currently return this value.
*
*
*
*/
private String stackResourceDriftStatus;
/**
*
* Time at which AWS CloudFormation performed drift detection on the stack resource.
*
*/
private java.util.Date timestamp;
/**
*
* The ID of the stack.
*
*
* @param stackId
* The ID of the stack.
*/
public void setStackId(String stackId) {
this.stackId = stackId;
}
/**
*
* The ID of the stack.
*
*
* @return The ID of the stack.
*/
public String getStackId() {
return this.stackId;
}
/**
*
* The ID of the stack.
*
*
* @param stackId
* The ID of the stack.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackResourceDrift withStackId(String stackId) {
setStackId(stackId);
return this;
}
/**
*
* The logical name of the resource specified in the template.
*
*
* @param logicalResourceId
* The logical name of the resource specified in the template.
*/
public void setLogicalResourceId(String logicalResourceId) {
this.logicalResourceId = logicalResourceId;
}
/**
*
* The logical name of the resource specified in the template.
*
*
* @return The logical name of the resource specified in the template.
*/
public String getLogicalResourceId() {
return this.logicalResourceId;
}
/**
*
* The logical name of the resource specified in the template.
*
*
* @param logicalResourceId
* The logical name of the resource specified in the template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackResourceDrift withLogicalResourceId(String logicalResourceId) {
setLogicalResourceId(logicalResourceId);
return this;
}
/**
*
* The name or unique identifier that corresponds to a physical instance ID of a resource supported by AWS
* CloudFormation.
*
*
* @param physicalResourceId
* The name or unique identifier that corresponds to a physical instance ID of a resource supported by AWS
* CloudFormation.
*/
public void setPhysicalResourceId(String physicalResourceId) {
this.physicalResourceId = physicalResourceId;
}
/**
*
* The name or unique identifier that corresponds to a physical instance ID of a resource supported by AWS
* CloudFormation.
*
*
* @return The name or unique identifier that corresponds to a physical instance ID of a resource supported by AWS
* CloudFormation.
*/
public String getPhysicalResourceId() {
return this.physicalResourceId;
}
/**
*
* The name or unique identifier that corresponds to a physical instance ID of a resource supported by AWS
* CloudFormation.
*
*
* @param physicalResourceId
* The name or unique identifier that corresponds to a physical instance ID of a resource supported by AWS
* CloudFormation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackResourceDrift withPhysicalResourceId(String physicalResourceId) {
setPhysicalResourceId(physicalResourceId);
return this;
}
/**
*
* Context information that enables AWS CloudFormation to uniquely identify a resource. AWS CloudFormation uses
* context key-value pairs in cases where a resource's logical and physical IDs are not enough to uniquely identify
* that resource. Each context key-value pair specifies a unique resource that contains the targeted resource.
*
*
* @return Context information that enables AWS CloudFormation to uniquely identify a resource. AWS CloudFormation
* uses context key-value pairs in cases where a resource's logical and physical IDs are not enough to
* uniquely identify that resource. Each context key-value pair specifies a unique resource that contains
* the targeted resource.
*/
public java.util.List getPhysicalResourceIdContext() {
if (physicalResourceIdContext == null) {
physicalResourceIdContext = new com.amazonaws.internal.SdkInternalList();
}
return physicalResourceIdContext;
}
/**
*
* Context information that enables AWS CloudFormation to uniquely identify a resource. AWS CloudFormation uses
* context key-value pairs in cases where a resource's logical and physical IDs are not enough to uniquely identify
* that resource. Each context key-value pair specifies a unique resource that contains the targeted resource.
*
*
* @param physicalResourceIdContext
* Context information that enables AWS CloudFormation to uniquely identify a resource. AWS CloudFormation
* uses context key-value pairs in cases where a resource's logical and physical IDs are not enough to
* uniquely identify that resource. Each context key-value pair specifies a unique resource that contains the
* targeted resource.
*/
public void setPhysicalResourceIdContext(java.util.Collection physicalResourceIdContext) {
if (physicalResourceIdContext == null) {
this.physicalResourceIdContext = null;
return;
}
this.physicalResourceIdContext = new com.amazonaws.internal.SdkInternalList(physicalResourceIdContext);
}
/**
*
* Context information that enables AWS CloudFormation to uniquely identify a resource. AWS CloudFormation uses
* context key-value pairs in cases where a resource's logical and physical IDs are not enough to uniquely identify
* that resource. Each context key-value pair specifies a unique resource that contains the targeted resource.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPhysicalResourceIdContext(java.util.Collection)} or
* {@link #withPhysicalResourceIdContext(java.util.Collection)} if you want to override the existing values.
*
*
* @param physicalResourceIdContext
* Context information that enables AWS CloudFormation to uniquely identify a resource. AWS CloudFormation
* uses context key-value pairs in cases where a resource's logical and physical IDs are not enough to
* uniquely identify that resource. Each context key-value pair specifies a unique resource that contains the
* targeted resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackResourceDrift withPhysicalResourceIdContext(PhysicalResourceIdContextKeyValuePair... physicalResourceIdContext) {
if (this.physicalResourceIdContext == null) {
setPhysicalResourceIdContext(new com.amazonaws.internal.SdkInternalList(physicalResourceIdContext.length));
}
for (PhysicalResourceIdContextKeyValuePair ele : physicalResourceIdContext) {
this.physicalResourceIdContext.add(ele);
}
return this;
}
/**
*
* Context information that enables AWS CloudFormation to uniquely identify a resource. AWS CloudFormation uses
* context key-value pairs in cases where a resource's logical and physical IDs are not enough to uniquely identify
* that resource. Each context key-value pair specifies a unique resource that contains the targeted resource.
*
*
* @param physicalResourceIdContext
* Context information that enables AWS CloudFormation to uniquely identify a resource. AWS CloudFormation
* uses context key-value pairs in cases where a resource's logical and physical IDs are not enough to
* uniquely identify that resource. Each context key-value pair specifies a unique resource that contains the
* targeted resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackResourceDrift withPhysicalResourceIdContext(java.util.Collection physicalResourceIdContext) {
setPhysicalResourceIdContext(physicalResourceIdContext);
return this;
}
/**
*
* The type of the resource.
*
*
* @param resourceType
* The type of the resource.
*/
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
*
* The type of the resource.
*
*
* @return The type of the resource.
*/
public String getResourceType() {
return this.resourceType;
}
/**
*
* The type of the resource.
*
*
* @param resourceType
* The type of the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackResourceDrift withResourceType(String resourceType) {
setResourceType(resourceType);
return this;
}
/**
*
* A JSON structure containing the expected property values of the stack resource, as defined in the stack template
* and any values specified as template parameters.
*
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will not be
* present.
*
*
* @param expectedProperties
* A JSON structure containing the expected property values of the stack resource, as defined in the stack
* template and any values specified as template parameters.
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will not
* be present.
*/
public void setExpectedProperties(String expectedProperties) {
this.expectedProperties = expectedProperties;
}
/**
*
* A JSON structure containing the expected property values of the stack resource, as defined in the stack template
* and any values specified as template parameters.
*
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will not be
* present.
*
*
* @return A JSON structure containing the expected property values of the stack resource, as defined in the stack
* template and any values specified as template parameters.
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will
* not be present.
*/
public String getExpectedProperties() {
return this.expectedProperties;
}
/**
*
* A JSON structure containing the expected property values of the stack resource, as defined in the stack template
* and any values specified as template parameters.
*
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will not be
* present.
*
*
* @param expectedProperties
* A JSON structure containing the expected property values of the stack resource, as defined in the stack
* template and any values specified as template parameters.
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will not
* be present.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackResourceDrift withExpectedProperties(String expectedProperties) {
setExpectedProperties(expectedProperties);
return this;
}
/**
*
* A JSON structure containing the actual property values of the stack resource.
*
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will not be
* present.
*
*
* @param actualProperties
* A JSON structure containing the actual property values of the stack resource.
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will not
* be present.
*/
public void setActualProperties(String actualProperties) {
this.actualProperties = actualProperties;
}
/**
*
* A JSON structure containing the actual property values of the stack resource.
*
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will not be
* present.
*
*
* @return A JSON structure containing the actual property values of the stack resource.
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will
* not be present.
*/
public String getActualProperties() {
return this.actualProperties;
}
/**
*
* A JSON structure containing the actual property values of the stack resource.
*
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will not be
* present.
*
*
* @param actualProperties
* A JSON structure containing the actual property values of the stack resource.
*
* For resources whose StackResourceDriftStatus
is DELETED
, this structure will not
* be present.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackResourceDrift withActualProperties(String actualProperties) {
setActualProperties(actualProperties);
return this;
}
/**
*
* A collection of the resource properties whose actual values differ from their expected values. These will be
* present only for resources whose StackResourceDriftStatus
is MODIFIED
.
*
*
* @return A collection of the resource properties whose actual values differ from their expected values. These will
* be present only for resources whose StackResourceDriftStatus
is MODIFIED
.
*/
public java.util.List getPropertyDifferences() {
if (propertyDifferences == null) {
propertyDifferences = new com.amazonaws.internal.SdkInternalList();
}
return propertyDifferences;
}
/**
*
* A collection of the resource properties whose actual values differ from their expected values. These will be
* present only for resources whose StackResourceDriftStatus
is MODIFIED
.
*
*
* @param propertyDifferences
* A collection of the resource properties whose actual values differ from their expected values. These will
* be present only for resources whose StackResourceDriftStatus
is MODIFIED
.
*/
public void setPropertyDifferences(java.util.Collection propertyDifferences) {
if (propertyDifferences == null) {
this.propertyDifferences = null;
return;
}
this.propertyDifferences = new com.amazonaws.internal.SdkInternalList(propertyDifferences);
}
/**
*
* A collection of the resource properties whose actual values differ from their expected values. These will be
* present only for resources whose StackResourceDriftStatus
is MODIFIED
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setPropertyDifferences(java.util.Collection)} or {@link #withPropertyDifferences(java.util.Collection)}
* if you want to override the existing values.
*
*
* @param propertyDifferences
* A collection of the resource properties whose actual values differ from their expected values. These will
* be present only for resources whose StackResourceDriftStatus
is MODIFIED
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackResourceDrift withPropertyDifferences(PropertyDifference... propertyDifferences) {
if (this.propertyDifferences == null) {
setPropertyDifferences(new com.amazonaws.internal.SdkInternalList(propertyDifferences.length));
}
for (PropertyDifference ele : propertyDifferences) {
this.propertyDifferences.add(ele);
}
return this;
}
/**
*
* A collection of the resource properties whose actual values differ from their expected values. These will be
* present only for resources whose StackResourceDriftStatus
is MODIFIED
.
*
*
* @param propertyDifferences
* A collection of the resource properties whose actual values differ from their expected values. These will
* be present only for resources whose StackResourceDriftStatus
is MODIFIED
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackResourceDrift withPropertyDifferences(java.util.Collection propertyDifferences) {
setPropertyDifferences(propertyDifferences);
return this;
}
/**
*
* Status of the resource's actual configuration compared to its expected configuration
*
*
* -
*
* DELETED
: The resource differs from its expected template configuration because the resource has been
* deleted.
*
*
* -
*
* MODIFIED
: One or more resource properties differ from their expected values (as defined in the stack
* template and any values specified as template parameters).
*
*
* -
*
* IN_SYNC
: The resources's actual configuration matches its expected template configuration.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation does not currently return this value.
*
*
*
*
* @param stackResourceDriftStatus
* Status of the resource's actual configuration compared to its expected configuration
*
* -
*
* DELETED
: The resource differs from its expected template configuration because the resource
* has been deleted.
*
*
* -
*
* MODIFIED
: One or more resource properties differ from their expected values (as defined in
* the stack template and any values specified as template parameters).
*
*
* -
*
* IN_SYNC
: The resources's actual configuration matches its expected template configuration.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation does not currently return this value.
*
*
* @see StackResourceDriftStatus
*/
public void setStackResourceDriftStatus(String stackResourceDriftStatus) {
this.stackResourceDriftStatus = stackResourceDriftStatus;
}
/**
*
* Status of the resource's actual configuration compared to its expected configuration
*
*
* -
*
* DELETED
: The resource differs from its expected template configuration because the resource has been
* deleted.
*
*
* -
*
* MODIFIED
: One or more resource properties differ from their expected values (as defined in the stack
* template and any values specified as template parameters).
*
*
* -
*
* IN_SYNC
: The resources's actual configuration matches its expected template configuration.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation does not currently return this value.
*
*
*
*
* @return Status of the resource's actual configuration compared to its expected configuration
*
* -
*
* DELETED
: The resource differs from its expected template configuration because the resource
* has been deleted.
*
*
* -
*
* MODIFIED
: One or more resource properties differ from their expected values (as defined in
* the stack template and any values specified as template parameters).
*
*
* -
*
* IN_SYNC
: The resources's actual configuration matches its expected template configuration.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation does not currently return this value.
*
*
* @see StackResourceDriftStatus
*/
public String getStackResourceDriftStatus() {
return this.stackResourceDriftStatus;
}
/**
*
* Status of the resource's actual configuration compared to its expected configuration
*
*
* -
*
* DELETED
: The resource differs from its expected template configuration because the resource has been
* deleted.
*
*
* -
*
* MODIFIED
: One or more resource properties differ from their expected values (as defined in the stack
* template and any values specified as template parameters).
*
*
* -
*
* IN_SYNC
: The resources's actual configuration matches its expected template configuration.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation does not currently return this value.
*
*
*
*
* @param stackResourceDriftStatus
* Status of the resource's actual configuration compared to its expected configuration
*
* -
*
* DELETED
: The resource differs from its expected template configuration because the resource
* has been deleted.
*
*
* -
*
* MODIFIED
: One or more resource properties differ from their expected values (as defined in
* the stack template and any values specified as template parameters).
*
*
* -
*
* IN_SYNC
: The resources's actual configuration matches its expected template configuration.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation does not currently return this value.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackResourceDriftStatus
*/
public StackResourceDrift withStackResourceDriftStatus(String stackResourceDriftStatus) {
setStackResourceDriftStatus(stackResourceDriftStatus);
return this;
}
/**
*
* Status of the resource's actual configuration compared to its expected configuration
*
*
* -
*
* DELETED
: The resource differs from its expected template configuration because the resource has been
* deleted.
*
*
* -
*
* MODIFIED
: One or more resource properties differ from their expected values (as defined in the stack
* template and any values specified as template parameters).
*
*
* -
*
* IN_SYNC
: The resources's actual configuration matches its expected template configuration.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation does not currently return this value.
*
*
*
*
* @param stackResourceDriftStatus
* Status of the resource's actual configuration compared to its expected configuration
*
* -
*
* DELETED
: The resource differs from its expected template configuration because the resource
* has been deleted.
*
*
* -
*
* MODIFIED
: One or more resource properties differ from their expected values (as defined in
* the stack template and any values specified as template parameters).
*
*
* -
*
* IN_SYNC
: The resources's actual configuration matches its expected template configuration.
*
*
* -
*
* NOT_CHECKED
: AWS CloudFormation does not currently return this value.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackResourceDriftStatus
*/
public StackResourceDrift withStackResourceDriftStatus(StackResourceDriftStatus stackResourceDriftStatus) {
this.stackResourceDriftStatus = stackResourceDriftStatus.toString();
return this;
}
/**
*
* Time at which AWS CloudFormation performed drift detection on the stack resource.
*
*
* @param timestamp
* Time at which AWS CloudFormation performed drift detection on the stack resource.
*/
public void setTimestamp(java.util.Date timestamp) {
this.timestamp = timestamp;
}
/**
*
* Time at which AWS CloudFormation performed drift detection on the stack resource.
*
*
* @return Time at which AWS CloudFormation performed drift detection on the stack resource.
*/
public java.util.Date getTimestamp() {
return this.timestamp;
}
/**
*
* Time at which AWS CloudFormation performed drift detection on the stack resource.
*
*
* @param timestamp
* Time at which AWS CloudFormation performed drift detection on the stack resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackResourceDrift withTimestamp(java.util.Date timestamp) {
setTimestamp(timestamp);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStackId() != null)
sb.append("StackId: ").append(getStackId()).append(",");
if (getLogicalResourceId() != null)
sb.append("LogicalResourceId: ").append(getLogicalResourceId()).append(",");
if (getPhysicalResourceId() != null)
sb.append("PhysicalResourceId: ").append(getPhysicalResourceId()).append(",");
if (getPhysicalResourceIdContext() != null)
sb.append("PhysicalResourceIdContext: ").append(getPhysicalResourceIdContext()).append(",");
if (getResourceType() != null)
sb.append("ResourceType: ").append(getResourceType()).append(",");
if (getExpectedProperties() != null)
sb.append("ExpectedProperties: ").append(getExpectedProperties()).append(",");
if (getActualProperties() != null)
sb.append("ActualProperties: ").append(getActualProperties()).append(",");
if (getPropertyDifferences() != null)
sb.append("PropertyDifferences: ").append(getPropertyDifferences()).append(",");
if (getStackResourceDriftStatus() != null)
sb.append("StackResourceDriftStatus: ").append(getStackResourceDriftStatus()).append(",");
if (getTimestamp() != null)
sb.append("Timestamp: ").append(getTimestamp());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StackResourceDrift == false)
return false;
StackResourceDrift other = (StackResourceDrift) obj;
if (other.getStackId() == null ^ this.getStackId() == null)
return false;
if (other.getStackId() != null && other.getStackId().equals(this.getStackId()) == false)
return false;
if (other.getLogicalResourceId() == null ^ this.getLogicalResourceId() == null)
return false;
if (other.getLogicalResourceId() != null && other.getLogicalResourceId().equals(this.getLogicalResourceId()) == false)
return false;
if (other.getPhysicalResourceId() == null ^ this.getPhysicalResourceId() == null)
return false;
if (other.getPhysicalResourceId() != null && other.getPhysicalResourceId().equals(this.getPhysicalResourceId()) == false)
return false;
if (other.getPhysicalResourceIdContext() == null ^ this.getPhysicalResourceIdContext() == null)
return false;
if (other.getPhysicalResourceIdContext() != null && other.getPhysicalResourceIdContext().equals(this.getPhysicalResourceIdContext()) == false)
return false;
if (other.getResourceType() == null ^ this.getResourceType() == null)
return false;
if (other.getResourceType() != null && other.getResourceType().equals(this.getResourceType()) == false)
return false;
if (other.getExpectedProperties() == null ^ this.getExpectedProperties() == null)
return false;
if (other.getExpectedProperties() != null && other.getExpectedProperties().equals(this.getExpectedProperties()) == false)
return false;
if (other.getActualProperties() == null ^ this.getActualProperties() == null)
return false;
if (other.getActualProperties() != null && other.getActualProperties().equals(this.getActualProperties()) == false)
return false;
if (other.getPropertyDifferences() == null ^ this.getPropertyDifferences() == null)
return false;
if (other.getPropertyDifferences() != null && other.getPropertyDifferences().equals(this.getPropertyDifferences()) == false)
return false;
if (other.getStackResourceDriftStatus() == null ^ this.getStackResourceDriftStatus() == null)
return false;
if (other.getStackResourceDriftStatus() != null && other.getStackResourceDriftStatus().equals(this.getStackResourceDriftStatus()) == false)
return false;
if (other.getTimestamp() == null ^ this.getTimestamp() == null)
return false;
if (other.getTimestamp() != null && other.getTimestamp().equals(this.getTimestamp()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStackId() == null) ? 0 : getStackId().hashCode());
hashCode = prime * hashCode + ((getLogicalResourceId() == null) ? 0 : getLogicalResourceId().hashCode());
hashCode = prime * hashCode + ((getPhysicalResourceId() == null) ? 0 : getPhysicalResourceId().hashCode());
hashCode = prime * hashCode + ((getPhysicalResourceIdContext() == null) ? 0 : getPhysicalResourceIdContext().hashCode());
hashCode = prime * hashCode + ((getResourceType() == null) ? 0 : getResourceType().hashCode());
hashCode = prime * hashCode + ((getExpectedProperties() == null) ? 0 : getExpectedProperties().hashCode());
hashCode = prime * hashCode + ((getActualProperties() == null) ? 0 : getActualProperties().hashCode());
hashCode = prime * hashCode + ((getPropertyDifferences() == null) ? 0 : getPropertyDifferences().hashCode());
hashCode = prime * hashCode + ((getStackResourceDriftStatus() == null) ? 0 : getStackResourceDriftStatus().hashCode());
hashCode = prime * hashCode + ((getTimestamp() == null) ? 0 : getTimestamp().hashCode());
return hashCode;
}
@Override
public StackResourceDrift clone() {
try {
return (StackResourceDrift) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}