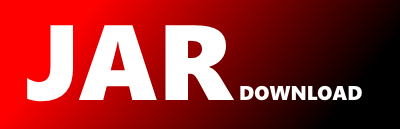
com.amazonaws.services.cloudformation.AmazonCloudFormationAsync Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudformation;
import javax.annotation.Generated;
import com.amazonaws.services.cloudformation.model.*;
/**
* Interface for accessing AWS CloudFormation asynchronously. Each asynchronous method will return a Java Future object
* representing the asynchronous operation; overloads which accept an {@code AsyncHandler} can be used to receive
* notification when an asynchronous operation completes.
*
* Note: Do not directly implement this interface, new methods are added to it regularly. Extend from
* {@link com.amazonaws.services.cloudformation.AbstractAmazonCloudFormationAsync} instead.
*
*
* AWS CloudFormation
*
* AWS CloudFormation allows you to create and manage AWS infrastructure deployments predictably and repeatedly. You can
* use AWS CloudFormation to leverage AWS products, such as Amazon Elastic Compute Cloud, Amazon Elastic Block Store,
* Amazon Simple Notification Service, Elastic Load Balancing, and Auto Scaling to build highly-reliable, highly
* scalable, cost-effective applications without creating or configuring the underlying AWS infrastructure.
*
*
* With AWS CloudFormation, you declare all of your resources and dependencies in a template file. The template defines
* a collection of resources as a single unit called a stack. AWS CloudFormation creates and deletes all member
* resources of the stack together and manages all dependencies between the resources for you.
*
*
* For more information about AWS CloudFormation, see the AWS
* CloudFormation Product Page.
*
*
* Amazon CloudFormation makes use of other AWS products. If you need additional technical information about a specific
* AWS product, you can find the product's technical documentation at docs.aws.amazon.com.
*
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public interface AmazonCloudFormationAsync extends AmazonCloudFormation {
/**
*
* Cancels an update on the specified stack. If the call completes successfully, the stack rolls back the update and
* reverts to the previous stack configuration.
*
*
*
* You can cancel only stacks that are in the UPDATE_IN_PROGRESS state.
*
*
*
* @param cancelUpdateStackRequest
* The input for the CancelUpdateStack action.
* @return A Java Future containing the result of the CancelUpdateStack operation returned by the service.
* @sample AmazonCloudFormationAsync.CancelUpdateStack
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelUpdateStackAsync(CancelUpdateStackRequest cancelUpdateStackRequest);
/**
*
* Cancels an update on the specified stack. If the call completes successfully, the stack rolls back the update and
* reverts to the previous stack configuration.
*
*
*
* You can cancel only stacks that are in the UPDATE_IN_PROGRESS state.
*
*
*
* @param cancelUpdateStackRequest
* The input for the CancelUpdateStack action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CancelUpdateStack operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.CancelUpdateStack
* @see AWS API Documentation
*/
java.util.concurrent.Future cancelUpdateStackAsync(CancelUpdateStackRequest cancelUpdateStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* For a specified stack that is in the UPDATE_ROLLBACK_FAILED
state, continues rolling it back to the
* UPDATE_ROLLBACK_COMPLETE
state. Depending on the cause of the failure, you can manually fix the error and continue the rollback. By continuing the rollback, you can return your stack to a working
* state (the UPDATE_ROLLBACK_COMPLETE
state), and then try to update the stack again.
*
*
* A stack goes into the UPDATE_ROLLBACK_FAILED
state when AWS CloudFormation cannot roll back all
* changes after a failed stack update. For example, you might have a stack that is rolling back to an old database
* instance that was deleted outside of AWS CloudFormation. Because AWS CloudFormation doesn't know the database was
* deleted, it assumes that the database instance still exists and attempts to roll back to it, causing the update
* rollback to fail.
*
*
* @param continueUpdateRollbackRequest
* The input for the ContinueUpdateRollback action.
* @return A Java Future containing the result of the ContinueUpdateRollback operation returned by the service.
* @sample AmazonCloudFormationAsync.ContinueUpdateRollback
* @see AWS API Documentation
*/
java.util.concurrent.Future continueUpdateRollbackAsync(ContinueUpdateRollbackRequest continueUpdateRollbackRequest);
/**
*
* For a specified stack that is in the UPDATE_ROLLBACK_FAILED
state, continues rolling it back to the
* UPDATE_ROLLBACK_COMPLETE
state. Depending on the cause of the failure, you can manually fix the error and continue the rollback. By continuing the rollback, you can return your stack to a working
* state (the UPDATE_ROLLBACK_COMPLETE
state), and then try to update the stack again.
*
*
* A stack goes into the UPDATE_ROLLBACK_FAILED
state when AWS CloudFormation cannot roll back all
* changes after a failed stack update. For example, you might have a stack that is rolling back to an old database
* instance that was deleted outside of AWS CloudFormation. Because AWS CloudFormation doesn't know the database was
* deleted, it assumes that the database instance still exists and attempts to roll back to it, causing the update
* rollback to fail.
*
*
* @param continueUpdateRollbackRequest
* The input for the ContinueUpdateRollback action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ContinueUpdateRollback operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.ContinueUpdateRollback
* @see AWS API Documentation
*/
java.util.concurrent.Future continueUpdateRollbackAsync(ContinueUpdateRollbackRequest continueUpdateRollbackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a list of changes that will be applied to a stack so that you can review the changes before executing
* them. You can create a change set for a stack that doesn't exist or an existing stack. If you create a change set
* for a stack that doesn't exist, the change set shows all of the resources that AWS CloudFormation will create. If
* you create a change set for an existing stack, AWS CloudFormation compares the stack's information with the
* information that you submit in the change set and lists the differences. Use change sets to understand which
* resources AWS CloudFormation will create or change, and how it will change resources in an existing stack, before
* you create or update a stack.
*
*
* To create a change set for a stack that doesn't exist, for the ChangeSetType
parameter, specify
* CREATE
. To create a change set for an existing stack, specify UPDATE
for the
* ChangeSetType
parameter. After the CreateChangeSet
call successfully completes, AWS
* CloudFormation starts creating the change set. To check the status of the change set or to review it, use the
* DescribeChangeSet action.
*
*
* When you are satisfied with the changes the change set will make, execute the change set by using the
* ExecuteChangeSet action. AWS CloudFormation doesn't make changes until you execute the change set.
*
*
* @param createChangeSetRequest
* The input for the CreateChangeSet action.
* @return A Java Future containing the result of the CreateChangeSet operation returned by the service.
* @sample AmazonCloudFormationAsync.CreateChangeSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createChangeSetAsync(CreateChangeSetRequest createChangeSetRequest);
/**
*
* Creates a list of changes that will be applied to a stack so that you can review the changes before executing
* them. You can create a change set for a stack that doesn't exist or an existing stack. If you create a change set
* for a stack that doesn't exist, the change set shows all of the resources that AWS CloudFormation will create. If
* you create a change set for an existing stack, AWS CloudFormation compares the stack's information with the
* information that you submit in the change set and lists the differences. Use change sets to understand which
* resources AWS CloudFormation will create or change, and how it will change resources in an existing stack, before
* you create or update a stack.
*
*
* To create a change set for a stack that doesn't exist, for the ChangeSetType
parameter, specify
* CREATE
. To create a change set for an existing stack, specify UPDATE
for the
* ChangeSetType
parameter. After the CreateChangeSet
call successfully completes, AWS
* CloudFormation starts creating the change set. To check the status of the change set or to review it, use the
* DescribeChangeSet action.
*
*
* When you are satisfied with the changes the change set will make, execute the change set by using the
* ExecuteChangeSet action. AWS CloudFormation doesn't make changes until you execute the change set.
*
*
* @param createChangeSetRequest
* The input for the CreateChangeSet action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateChangeSet operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.CreateChangeSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createChangeSetAsync(CreateChangeSetRequest createChangeSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a stack as specified in the template. After the call completes successfully, the stack creation starts.
* You can check the status of the stack via the DescribeStacks API.
*
*
* @param createStackRequest
* The input for CreateStack action.
* @return A Java Future containing the result of the CreateStack operation returned by the service.
* @sample AmazonCloudFormationAsync.CreateStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createStackAsync(CreateStackRequest createStackRequest);
/**
*
* Creates a stack as specified in the template. After the call completes successfully, the stack creation starts.
* You can check the status of the stack via the DescribeStacks API.
*
*
* @param createStackRequest
* The input for CreateStack action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStack operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.CreateStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future createStackAsync(CreateStackRequest createStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates stack instances for the specified accounts, within the specified regions. A stack instance refers to a
* stack in a specific account and region. Accounts
and Regions
are required
* parameters—you must specify at least one account and one region.
*
*
* @param createStackInstancesRequest
* @return A Java Future containing the result of the CreateStackInstances operation returned by the service.
* @sample AmazonCloudFormationAsync.CreateStackInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future createStackInstancesAsync(CreateStackInstancesRequest createStackInstancesRequest);
/**
*
* Creates stack instances for the specified accounts, within the specified regions. A stack instance refers to a
* stack in a specific account and region. Accounts
and Regions
are required
* parameters—you must specify at least one account and one region.
*
*
* @param createStackInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStackInstances operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.CreateStackInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future createStackInstancesAsync(CreateStackInstancesRequest createStackInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Creates a stack set.
*
*
* @param createStackSetRequest
* @return A Java Future containing the result of the CreateStackSet operation returned by the service.
* @sample AmazonCloudFormationAsync.CreateStackSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createStackSetAsync(CreateStackSetRequest createStackSetRequest);
/**
*
* Creates a stack set.
*
*
* @param createStackSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the CreateStackSet operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.CreateStackSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future createStackSetAsync(CreateStackSetRequest createStackSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes the specified change set. Deleting change sets ensures that no one executes the wrong change set.
*
*
* If the call successfully completes, AWS CloudFormation successfully deleted the change set.
*
*
* @param deleteChangeSetRequest
* The input for the DeleteChangeSet action.
* @return A Java Future containing the result of the DeleteChangeSet operation returned by the service.
* @sample AmazonCloudFormationAsync.DeleteChangeSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteChangeSetAsync(DeleteChangeSetRequest deleteChangeSetRequest);
/**
*
* Deletes the specified change set. Deleting change sets ensures that no one executes the wrong change set.
*
*
* If the call successfully completes, AWS CloudFormation successfully deleted the change set.
*
*
* @param deleteChangeSetRequest
* The input for the DeleteChangeSet action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteChangeSet operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DeleteChangeSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteChangeSetAsync(DeleteChangeSetRequest deleteChangeSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a specified stack. Once the call completes successfully, stack deletion starts. Deleted stacks do not
* show up in the DescribeStacks API if the deletion has been completed successfully.
*
*
* @param deleteStackRequest
* The input for DeleteStack action.
* @return A Java Future containing the result of the DeleteStack operation returned by the service.
* @sample AmazonCloudFormationAsync.DeleteStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteStackAsync(DeleteStackRequest deleteStackRequest);
/**
*
* Deletes a specified stack. Once the call completes successfully, stack deletion starts. Deleted stacks do not
* show up in the DescribeStacks API if the deletion has been completed successfully.
*
*
* @param deleteStackRequest
* The input for DeleteStack action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteStack operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DeleteStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future deleteStackAsync(DeleteStackRequest deleteStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes stack instances for the specified accounts, in the specified regions.
*
*
* @param deleteStackInstancesRequest
* @return A Java Future containing the result of the DeleteStackInstances operation returned by the service.
* @sample AmazonCloudFormationAsync.DeleteStackInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteStackInstancesAsync(DeleteStackInstancesRequest deleteStackInstancesRequest);
/**
*
* Deletes stack instances for the specified accounts, in the specified regions.
*
*
* @param deleteStackInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteStackInstances operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DeleteStackInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future deleteStackInstancesAsync(DeleteStackInstancesRequest deleteStackInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Deletes a stack set. Before you can delete a stack set, all of its member stack instances must be deleted. For
* more information about how to do this, see DeleteStackInstances.
*
*
* @param deleteStackSetRequest
* @return A Java Future containing the result of the DeleteStackSet operation returned by the service.
* @sample AmazonCloudFormationAsync.DeleteStackSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteStackSetAsync(DeleteStackSetRequest deleteStackSetRequest);
/**
*
* Deletes a stack set. Before you can delete a stack set, all of its member stack instances must be deleted. For
* more information about how to do this, see DeleteStackInstances.
*
*
* @param deleteStackSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DeleteStackSet operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DeleteStackSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future deleteStackSetAsync(DeleteStackSetRequest deleteStackSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Retrieves your account's AWS CloudFormation limits, such as the maximum number of stacks that you can create in
* your account. For more information about account limits, see AWS
* CloudFormation Limits in the AWS CloudFormation User Guide.
*
*
* @param describeAccountLimitsRequest
* The input for the DescribeAccountLimits action.
* @return A Java Future containing the result of the DescribeAccountLimits operation returned by the service.
* @sample AmazonCloudFormationAsync.DescribeAccountLimits
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccountLimitsAsync(DescribeAccountLimitsRequest describeAccountLimitsRequest);
/**
*
* Retrieves your account's AWS CloudFormation limits, such as the maximum number of stacks that you can create in
* your account. For more information about account limits, see AWS
* CloudFormation Limits in the AWS CloudFormation User Guide.
*
*
* @param describeAccountLimitsRequest
* The input for the DescribeAccountLimits action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeAccountLimits operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DescribeAccountLimits
* @see AWS API Documentation
*/
java.util.concurrent.Future describeAccountLimitsAsync(DescribeAccountLimitsRequest describeAccountLimitsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the inputs for the change set and a list of changes that AWS CloudFormation will make if you execute the
* change set. For more information, see Updating Stacks Using Change Sets in the AWS CloudFormation User Guide.
*
*
* @param describeChangeSetRequest
* The input for the DescribeChangeSet action.
* @return A Java Future containing the result of the DescribeChangeSet operation returned by the service.
* @sample AmazonCloudFormationAsync.DescribeChangeSet
* @see AWS API Documentation
*/
java.util.concurrent.Future describeChangeSetAsync(DescribeChangeSetRequest describeChangeSetRequest);
/**
*
* Returns the inputs for the change set and a list of changes that AWS CloudFormation will make if you execute the
* change set. For more information, see Updating Stacks Using Change Sets in the AWS CloudFormation User Guide.
*
*
* @param describeChangeSetRequest
* The input for the DescribeChangeSet action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeChangeSet operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DescribeChangeSet
* @see AWS API Documentation
*/
java.util.concurrent.Future describeChangeSetAsync(DescribeChangeSetRequest describeChangeSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a stack drift detection operation. A stack drift detection operation detects whether a
* stack's actual configuration differs, or has drifted, from it's expected configuration, as defined in the
* stack template and any values specified as template parameters. A stack is considered to have drifted if one or
* more of its resources have drifted. For more information on stack and resource drift, see Detecting
* Unregulated Configuration Changes to Stacks and Resources.
*
*
* Use DetectStackDrift to initiate a stack drift detection operation. DetectStackDrift
returns
* a StackDriftDetectionId
you can use to monitor the progress of the operation using
* DescribeStackDriftDetectionStatus
. Once the drift detection operation has completed, use
* DescribeStackResourceDrifts to return drift information about the stack and its resources.
*
*
* @param describeStackDriftDetectionStatusRequest
* @return A Java Future containing the result of the DescribeStackDriftDetectionStatus operation returned by the
* service.
* @sample AmazonCloudFormationAsync.DescribeStackDriftDetectionStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackDriftDetectionStatusAsync(
DescribeStackDriftDetectionStatusRequest describeStackDriftDetectionStatusRequest);
/**
*
* Returns information about a stack drift detection operation. A stack drift detection operation detects whether a
* stack's actual configuration differs, or has drifted, from it's expected configuration, as defined in the
* stack template and any values specified as template parameters. A stack is considered to have drifted if one or
* more of its resources have drifted. For more information on stack and resource drift, see Detecting
* Unregulated Configuration Changes to Stacks and Resources.
*
*
* Use DetectStackDrift to initiate a stack drift detection operation. DetectStackDrift
returns
* a StackDriftDetectionId
you can use to monitor the progress of the operation using
* DescribeStackDriftDetectionStatus
. Once the drift detection operation has completed, use
* DescribeStackResourceDrifts to return drift information about the stack and its resources.
*
*
* @param describeStackDriftDetectionStatusRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStackDriftDetectionStatus operation returned by the
* service.
* @sample AmazonCloudFormationAsyncHandler.DescribeStackDriftDetectionStatus
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackDriftDetectionStatusAsync(
DescribeStackDriftDetectionStatusRequest describeStackDriftDetectionStatusRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns all stack related events for a specified stack in reverse chronological order. For more information about
* a stack's event history, go to Stacks in the AWS
* CloudFormation User Guide.
*
*
*
* You can list events for stacks that have failed to create or have been deleted by specifying the unique stack
* identifier (stack ID).
*
*
*
* @param describeStackEventsRequest
* The input for DescribeStackEvents action.
* @return A Java Future containing the result of the DescribeStackEvents operation returned by the service.
* @sample AmazonCloudFormationAsync.DescribeStackEvents
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackEventsAsync(DescribeStackEventsRequest describeStackEventsRequest);
/**
*
* Returns all stack related events for a specified stack in reverse chronological order. For more information about
* a stack's event history, go to Stacks in the AWS
* CloudFormation User Guide.
*
*
*
* You can list events for stacks that have failed to create or have been deleted by specifying the unique stack
* identifier (stack ID).
*
*
*
* @param describeStackEventsRequest
* The input for DescribeStackEvents action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStackEvents operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DescribeStackEvents
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackEventsAsync(DescribeStackEventsRequest describeStackEventsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the stack instance that's associated with the specified stack set, AWS account, and region.
*
*
* For a list of stack instances that are associated with a specific stack set, use ListStackInstances.
*
*
* @param describeStackInstanceRequest
* @return A Java Future containing the result of the DescribeStackInstance operation returned by the service.
* @sample AmazonCloudFormationAsync.DescribeStackInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackInstanceAsync(DescribeStackInstanceRequest describeStackInstanceRequest);
/**
*
* Returns the stack instance that's associated with the specified stack set, AWS account, and region.
*
*
* For a list of stack instances that are associated with a specific stack set, use ListStackInstances.
*
*
* @param describeStackInstanceRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStackInstance operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DescribeStackInstance
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackInstanceAsync(DescribeStackInstanceRequest describeStackInstanceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns a description of the specified resource in the specified stack.
*
*
* For deleted stacks, DescribeStackResource returns resource information for up to 90 days after the stack has been
* deleted.
*
*
* @param describeStackResourceRequest
* The input for DescribeStackResource action.
* @return A Java Future containing the result of the DescribeStackResource operation returned by the service.
* @sample AmazonCloudFormationAsync.DescribeStackResource
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackResourceAsync(DescribeStackResourceRequest describeStackResourceRequest);
/**
*
* Returns a description of the specified resource in the specified stack.
*
*
* For deleted stacks, DescribeStackResource returns resource information for up to 90 days after the stack has been
* deleted.
*
*
* @param describeStackResourceRequest
* The input for DescribeStackResource action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStackResource operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DescribeStackResource
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackResourceAsync(DescribeStackResourceRequest describeStackResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns drift information for the resources that have been checked for drift in the specified stack. This
* includes actual and expected configuration values for resources where AWS CloudFormation detects configuration
* drift.
*
*
* For a given stack, there will be one StackResourceDrift
for each stack resource that has been
* checked for drift. Resources that have not yet been checked for drift are not included. Resources that do not
* currently support drift detection are not checked, and so not included. For a list of resources that support
* drift detection, see Resources that Support Drift Detection.
*
*
* Use DetectStackResourceDrift to detect drift on individual resources, or DetectStackDrift to detect
* drift on all supported resources for a given stack.
*
*
* @param describeStackResourceDriftsRequest
* @return A Java Future containing the result of the DescribeStackResourceDrifts operation returned by the service.
* @sample AmazonCloudFormationAsync.DescribeStackResourceDrifts
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackResourceDriftsAsync(
DescribeStackResourceDriftsRequest describeStackResourceDriftsRequest);
/**
*
* Returns drift information for the resources that have been checked for drift in the specified stack. This
* includes actual and expected configuration values for resources where AWS CloudFormation detects configuration
* drift.
*
*
* For a given stack, there will be one StackResourceDrift
for each stack resource that has been
* checked for drift. Resources that have not yet been checked for drift are not included. Resources that do not
* currently support drift detection are not checked, and so not included. For a list of resources that support
* drift detection, see Resources that Support Drift Detection.
*
*
* Use DetectStackResourceDrift to detect drift on individual resources, or DetectStackDrift to detect
* drift on all supported resources for a given stack.
*
*
* @param describeStackResourceDriftsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStackResourceDrifts operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DescribeStackResourceDrifts
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackResourceDriftsAsync(
DescribeStackResourceDriftsRequest describeStackResourceDriftsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns AWS resource descriptions for running and deleted stacks. If StackName
is specified, all the
* associated resources that are part of the stack are returned. If PhysicalResourceId
is specified,
* the associated resources of the stack that the resource belongs to are returned.
*
*
*
* Only the first 100 resources will be returned. If your stack has more resources than this, you should use
* ListStackResources
instead.
*
*
*
* For deleted stacks, DescribeStackResources
returns resource information for up to 90 days after the
* stack has been deleted.
*
*
* You must specify either StackName
or PhysicalResourceId
, but not both. In addition, you
* can specify LogicalResourceId
to filter the returned result. For more information about resources,
* the LogicalResourceId
and PhysicalResourceId
, go to the AWS CloudFormation User Guide.
*
*
*
* A ValidationError
is returned if you specify both StackName
and
* PhysicalResourceId
in the same request.
*
*
*
* @param describeStackResourcesRequest
* The input for DescribeStackResources action.
* @return A Java Future containing the result of the DescribeStackResources operation returned by the service.
* @sample AmazonCloudFormationAsync.DescribeStackResources
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackResourcesAsync(DescribeStackResourcesRequest describeStackResourcesRequest);
/**
*
* Returns AWS resource descriptions for running and deleted stacks. If StackName
is specified, all the
* associated resources that are part of the stack are returned. If PhysicalResourceId
is specified,
* the associated resources of the stack that the resource belongs to are returned.
*
*
*
* Only the first 100 resources will be returned. If your stack has more resources than this, you should use
* ListStackResources
instead.
*
*
*
* For deleted stacks, DescribeStackResources
returns resource information for up to 90 days after the
* stack has been deleted.
*
*
* You must specify either StackName
or PhysicalResourceId
, but not both. In addition, you
* can specify LogicalResourceId
to filter the returned result. For more information about resources,
* the LogicalResourceId
and PhysicalResourceId
, go to the AWS CloudFormation User Guide.
*
*
*
* A ValidationError
is returned if you specify both StackName
and
* PhysicalResourceId
in the same request.
*
*
*
* @param describeStackResourcesRequest
* The input for DescribeStackResources action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStackResources operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DescribeStackResources
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackResourcesAsync(DescribeStackResourcesRequest describeStackResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the description of the specified stack set.
*
*
* @param describeStackSetRequest
* @return A Java Future containing the result of the DescribeStackSet operation returned by the service.
* @sample AmazonCloudFormationAsync.DescribeStackSet
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackSetAsync(DescribeStackSetRequest describeStackSetRequest);
/**
*
* Returns the description of the specified stack set.
*
*
* @param describeStackSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStackSet operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DescribeStackSet
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackSetAsync(DescribeStackSetRequest describeStackSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the description of the specified stack set operation.
*
*
* @param describeStackSetOperationRequest
* @return A Java Future containing the result of the DescribeStackSetOperation operation returned by the service.
* @sample AmazonCloudFormationAsync.DescribeStackSetOperation
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackSetOperationAsync(
DescribeStackSetOperationRequest describeStackSetOperationRequest);
/**
*
* Returns the description of the specified stack set operation.
*
*
* @param describeStackSetOperationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStackSetOperation operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DescribeStackSetOperation
* @see AWS API Documentation
*/
java.util.concurrent.Future describeStackSetOperationAsync(
DescribeStackSetOperationRequest describeStackSetOperationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the description for the specified stack; if no stack name was specified, then it returns the description
* for all the stacks created.
*
*
*
* If the stack does not exist, an AmazonCloudFormationException
is returned.
*
*
*
* @param describeStacksRequest
* The input for DescribeStacks action.
* @return A Java Future containing the result of the DescribeStacks operation returned by the service.
* @sample AmazonCloudFormationAsync.DescribeStacks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeStacksAsync(DescribeStacksRequest describeStacksRequest);
/**
*
* Returns the description for the specified stack; if no stack name was specified, then it returns the description
* for all the stacks created.
*
*
*
* If the stack does not exist, an AmazonCloudFormationException
is returned.
*
*
*
* @param describeStacksRequest
* The input for DescribeStacks action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DescribeStacks operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DescribeStacks
* @see AWS
* API Documentation
*/
java.util.concurrent.Future describeStacksAsync(DescribeStacksRequest describeStacksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the DescribeStacks operation.
*
* @see #describeStacksAsync(DescribeStacksRequest)
*/
java.util.concurrent.Future describeStacksAsync();
/**
* Simplified method form for invoking the DescribeStacks operation with an AsyncHandler.
*
* @see #describeStacksAsync(DescribeStacksRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future describeStacksAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Detects whether a stack's actual configuration differs, or has drifted, from it's expected configuration,
* as defined in the stack template and any values specified as template parameters. For each resource in the stack
* that supports drift detection, AWS CloudFormation compares the actual configuration of the resource with its
* expected template configuration. Only resource properties explicitly defined in the stack template are checked
* for drift. A stack is considered to have drifted if one or more of its resources differ from their expected
* template configurations. For more information, see Detecting
* Unregulated Configuration Changes to Stacks and Resources.
*
*
* Use DetectStackDrift
to detect drift on all supported resources for a given stack, or
* DetectStackResourceDrift to detect drift on individual resources.
*
*
* For a list of stack resources that currently support drift detection, see Resources that Support Drift Detection.
*
*
* DetectStackDrift
can take up to several minutes, depending on the number of resources contained
* within the stack. Use DescribeStackDriftDetectionStatus to monitor the progress of a detect stack drift
* operation. Once the drift detection operation has completed, use DescribeStackResourceDrifts to return
* drift information about the stack and its resources.
*
*
* When detecting drift on a stack, AWS CloudFormation does not detect drift on any nested stacks belonging to that
* stack. Perform DetectStackDrift
directly on the nested stack itself.
*
*
* @param detectStackDriftRequest
* @return A Java Future containing the result of the DetectStackDrift operation returned by the service.
* @sample AmazonCloudFormationAsync.DetectStackDrift
* @see AWS API Documentation
*/
java.util.concurrent.Future detectStackDriftAsync(DetectStackDriftRequest detectStackDriftRequest);
/**
*
* Detects whether a stack's actual configuration differs, or has drifted, from it's expected configuration,
* as defined in the stack template and any values specified as template parameters. For each resource in the stack
* that supports drift detection, AWS CloudFormation compares the actual configuration of the resource with its
* expected template configuration. Only resource properties explicitly defined in the stack template are checked
* for drift. A stack is considered to have drifted if one or more of its resources differ from their expected
* template configurations. For more information, see Detecting
* Unregulated Configuration Changes to Stacks and Resources.
*
*
* Use DetectStackDrift
to detect drift on all supported resources for a given stack, or
* DetectStackResourceDrift to detect drift on individual resources.
*
*
* For a list of stack resources that currently support drift detection, see Resources that Support Drift Detection.
*
*
* DetectStackDrift
can take up to several minutes, depending on the number of resources contained
* within the stack. Use DescribeStackDriftDetectionStatus to monitor the progress of a detect stack drift
* operation. Once the drift detection operation has completed, use DescribeStackResourceDrifts to return
* drift information about the stack and its resources.
*
*
* When detecting drift on a stack, AWS CloudFormation does not detect drift on any nested stacks belonging to that
* stack. Perform DetectStackDrift
directly on the nested stack itself.
*
*
* @param detectStackDriftRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DetectStackDrift operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DetectStackDrift
* @see AWS API Documentation
*/
java.util.concurrent.Future detectStackDriftAsync(DetectStackDriftRequest detectStackDriftRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about whether a resource's actual configuration differs, or has drifted, from it's
* expected configuration, as defined in the stack template and any values specified as template parameters. This
* information includes actual and expected property values for resources in which AWS CloudFormation detects drift.
* Only resource properties explicitly defined in the stack template are checked for drift. For more information
* about stack and resource drift, see Detecting
* Unregulated Configuration Changes to Stacks and Resources.
*
*
* Use DetectStackResourceDrift
to detect drift on individual resources, or DetectStackDrift to
* detect drift on all resources in a given stack that support drift detection.
*
*
* Resources that do not currently support drift detection cannot be checked. For a list of resources that support
* drift detection, see Resources that Support Drift Detection.
*
*
* @param detectStackResourceDriftRequest
* @return A Java Future containing the result of the DetectStackResourceDrift operation returned by the service.
* @sample AmazonCloudFormationAsync.DetectStackResourceDrift
* @see AWS API Documentation
*/
java.util.concurrent.Future detectStackResourceDriftAsync(DetectStackResourceDriftRequest detectStackResourceDriftRequest);
/**
*
* Returns information about whether a resource's actual configuration differs, or has drifted, from it's
* expected configuration, as defined in the stack template and any values specified as template parameters. This
* information includes actual and expected property values for resources in which AWS CloudFormation detects drift.
* Only resource properties explicitly defined in the stack template are checked for drift. For more information
* about stack and resource drift, see Detecting
* Unregulated Configuration Changes to Stacks and Resources.
*
*
* Use DetectStackResourceDrift
to detect drift on individual resources, or DetectStackDrift to
* detect drift on all resources in a given stack that support drift detection.
*
*
* Resources that do not currently support drift detection cannot be checked. For a list of resources that support
* drift detection, see Resources that Support Drift Detection.
*
*
* @param detectStackResourceDriftRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the DetectStackResourceDrift operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.DetectStackResourceDrift
* @see AWS API Documentation
*/
java.util.concurrent.Future detectStackResourceDriftAsync(DetectStackResourceDriftRequest detectStackResourceDriftRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the estimated monthly cost of a template. The return value is an AWS Simple Monthly Calculator URL with a
* query string that describes the resources required to run the template.
*
*
* @param estimateTemplateCostRequest
* The input for an EstimateTemplateCost action.
* @return A Java Future containing the result of the EstimateTemplateCost operation returned by the service.
* @sample AmazonCloudFormationAsync.EstimateTemplateCost
* @see AWS API Documentation
*/
java.util.concurrent.Future estimateTemplateCostAsync(EstimateTemplateCostRequest estimateTemplateCostRequest);
/**
*
* Returns the estimated monthly cost of a template. The return value is an AWS Simple Monthly Calculator URL with a
* query string that describes the resources required to run the template.
*
*
* @param estimateTemplateCostRequest
* The input for an EstimateTemplateCost action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the EstimateTemplateCost operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.EstimateTemplateCost
* @see AWS API Documentation
*/
java.util.concurrent.Future estimateTemplateCostAsync(EstimateTemplateCostRequest estimateTemplateCostRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the EstimateTemplateCost operation.
*
* @see #estimateTemplateCostAsync(EstimateTemplateCostRequest)
*/
java.util.concurrent.Future estimateTemplateCostAsync();
/**
* Simplified method form for invoking the EstimateTemplateCost operation with an AsyncHandler.
*
* @see #estimateTemplateCostAsync(EstimateTemplateCostRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future estimateTemplateCostAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a stack using the input information that was provided when the specified change set was created. After
* the call successfully completes, AWS CloudFormation starts updating the stack. Use the DescribeStacks
* action to view the status of the update.
*
*
* When you execute a change set, AWS CloudFormation deletes all other change sets associated with the stack because
* they aren't valid for the updated stack.
*
*
* If a stack policy is associated with the stack, AWS CloudFormation enforces the policy during the update. You
* can't specify a temporary stack policy that overrides the current policy.
*
*
* @param executeChangeSetRequest
* The input for the ExecuteChangeSet action.
* @return A Java Future containing the result of the ExecuteChangeSet operation returned by the service.
* @sample AmazonCloudFormationAsync.ExecuteChangeSet
* @see AWS API Documentation
*/
java.util.concurrent.Future executeChangeSetAsync(ExecuteChangeSetRequest executeChangeSetRequest);
/**
*
* Updates a stack using the input information that was provided when the specified change set was created. After
* the call successfully completes, AWS CloudFormation starts updating the stack. Use the DescribeStacks
* action to view the status of the update.
*
*
* When you execute a change set, AWS CloudFormation deletes all other change sets associated with the stack because
* they aren't valid for the updated stack.
*
*
* If a stack policy is associated with the stack, AWS CloudFormation enforces the policy during the update. You
* can't specify a temporary stack policy that overrides the current policy.
*
*
* @param executeChangeSetRequest
* The input for the ExecuteChangeSet action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ExecuteChangeSet operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.ExecuteChangeSet
* @see AWS API Documentation
*/
java.util.concurrent.Future executeChangeSetAsync(ExecuteChangeSetRequest executeChangeSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the stack policy for a specified stack. If a stack doesn't have a policy, a null value is returned.
*
*
* @param getStackPolicyRequest
* The input for the GetStackPolicy action.
* @return A Java Future containing the result of the GetStackPolicy operation returned by the service.
* @sample AmazonCloudFormationAsync.GetStackPolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getStackPolicyAsync(GetStackPolicyRequest getStackPolicyRequest);
/**
*
* Returns the stack policy for a specified stack. If a stack doesn't have a policy, a null value is returned.
*
*
* @param getStackPolicyRequest
* The input for the GetStackPolicy action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetStackPolicy operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.GetStackPolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future getStackPolicyAsync(GetStackPolicyRequest getStackPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the template body for a specified stack. You can get the template for running or deleted stacks.
*
*
* For deleted stacks, GetTemplate returns the template for up to 90 days after the stack has been deleted.
*
*
*
* If the template does not exist, a ValidationError
is returned.
*
*
*
* @param getTemplateRequest
* The input for a GetTemplate action.
* @return A Java Future containing the result of the GetTemplate operation returned by the service.
* @sample AmazonCloudFormationAsync.GetTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTemplateAsync(GetTemplateRequest getTemplateRequest);
/**
*
* Returns the template body for a specified stack. You can get the template for running or deleted stacks.
*
*
* For deleted stacks, GetTemplate returns the template for up to 90 days after the stack has been deleted.
*
*
*
* If the template does not exist, a ValidationError
is returned.
*
*
*
* @param getTemplateRequest
* The input for a GetTemplate action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTemplate operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.GetTemplate
* @see AWS API
* Documentation
*/
java.util.concurrent.Future getTemplateAsync(GetTemplateRequest getTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns information about a new or existing template. The GetTemplateSummary
action is useful for
* viewing parameter information, such as default parameter values and parameter types, before you create or update
* a stack or stack set.
*
*
* You can use the GetTemplateSummary
action when you submit a template, or you can get template
* information for a stack set, or a running or deleted stack.
*
*
* For deleted stacks, GetTemplateSummary
returns the template information for up to 90 days after the
* stack has been deleted. If the template does not exist, a ValidationError
is returned.
*
*
* @param getTemplateSummaryRequest
* The input for the GetTemplateSummary action.
* @return A Java Future containing the result of the GetTemplateSummary operation returned by the service.
* @sample AmazonCloudFormationAsync.GetTemplateSummary
* @see AWS API Documentation
*/
java.util.concurrent.Future getTemplateSummaryAsync(GetTemplateSummaryRequest getTemplateSummaryRequest);
/**
*
* Returns information about a new or existing template. The GetTemplateSummary
action is useful for
* viewing parameter information, such as default parameter values and parameter types, before you create or update
* a stack or stack set.
*
*
* You can use the GetTemplateSummary
action when you submit a template, or you can get template
* information for a stack set, or a running or deleted stack.
*
*
* For deleted stacks, GetTemplateSummary
returns the template information for up to 90 days after the
* stack has been deleted. If the template does not exist, a ValidationError
is returned.
*
*
* @param getTemplateSummaryRequest
* The input for the GetTemplateSummary action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the GetTemplateSummary operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.GetTemplateSummary
* @see AWS API Documentation
*/
java.util.concurrent.Future getTemplateSummaryAsync(GetTemplateSummaryRequest getTemplateSummaryRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the GetTemplateSummary operation.
*
* @see #getTemplateSummaryAsync(GetTemplateSummaryRequest)
*/
java.util.concurrent.Future getTemplateSummaryAsync();
/**
* Simplified method form for invoking the GetTemplateSummary operation with an AsyncHandler.
*
* @see #getTemplateSummaryAsync(GetTemplateSummaryRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future getTemplateSummaryAsync(
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the ID and status of each active change set for a stack. For example, AWS CloudFormation lists change
* sets that are in the CREATE_IN_PROGRESS
or CREATE_PENDING
state.
*
*
* @param listChangeSetsRequest
* The input for the ListChangeSets action.
* @return A Java Future containing the result of the ListChangeSets operation returned by the service.
* @sample AmazonCloudFormationAsync.ListChangeSets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listChangeSetsAsync(ListChangeSetsRequest listChangeSetsRequest);
/**
*
* Returns the ID and status of each active change set for a stack. For example, AWS CloudFormation lists change
* sets that are in the CREATE_IN_PROGRESS
or CREATE_PENDING
state.
*
*
* @param listChangeSetsRequest
* The input for the ListChangeSets action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListChangeSets operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.ListChangeSets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listChangeSetsAsync(ListChangeSetsRequest listChangeSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all exported output values in the account and region in which you call this action. Use this action to see
* the exported output values that you can import into other stacks. To import values, use the
* Fn::ImportValue
function.
*
*
* For more information, see AWS
* CloudFormation Export Stack Output Values.
*
*
* @param listExportsRequest
* @return A Java Future containing the result of the ListExports operation returned by the service.
* @sample AmazonCloudFormationAsync.ListExports
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listExportsAsync(ListExportsRequest listExportsRequest);
/**
*
* Lists all exported output values in the account and region in which you call this action. Use this action to see
* the exported output values that you can import into other stacks. To import values, use the
* Fn::ImportValue
function.
*
*
* For more information, see AWS
* CloudFormation Export Stack Output Values.
*
*
* @param listExportsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListExports operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.ListExports
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listExportsAsync(ListExportsRequest listExportsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Lists all stacks that are importing an exported output value. To modify or remove an exported output value, first
* use this action to see which stacks are using it. To see the exported output values in your account, see
* ListExports.
*
*
* For more information about importing an exported output value, see the
* Fn::ImportValue
function.
*
*
* @param listImportsRequest
* @return A Java Future containing the result of the ListImports operation returned by the service.
* @sample AmazonCloudFormationAsync.ListImports
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listImportsAsync(ListImportsRequest listImportsRequest);
/**
*
* Lists all stacks that are importing an exported output value. To modify or remove an exported output value, first
* use this action to see which stacks are using it. To see the exported output values in your account, see
* ListExports.
*
*
* For more information about importing an exported output value, see the
* Fn::ImportValue
function.
*
*
* @param listImportsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListImports operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.ListImports
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listImportsAsync(ListImportsRequest listImportsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns summary information about stack instances that are associated with the specified stack set. You can
* filter for stack instances that are associated with a specific AWS account name or region.
*
*
* @param listStackInstancesRequest
* @return A Java Future containing the result of the ListStackInstances operation returned by the service.
* @sample AmazonCloudFormationAsync.ListStackInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future listStackInstancesAsync(ListStackInstancesRequest listStackInstancesRequest);
/**
*
* Returns summary information about stack instances that are associated with the specified stack set. You can
* filter for stack instances that are associated with a specific AWS account name or region.
*
*
* @param listStackInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStackInstances operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.ListStackInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future listStackInstancesAsync(ListStackInstancesRequest listStackInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns descriptions of all resources of the specified stack.
*
*
* For deleted stacks, ListStackResources returns resource information for up to 90 days after the stack has been
* deleted.
*
*
* @param listStackResourcesRequest
* The input for the ListStackResource action.
* @return A Java Future containing the result of the ListStackResources operation returned by the service.
* @sample AmazonCloudFormationAsync.ListStackResources
* @see AWS API Documentation
*/
java.util.concurrent.Future listStackResourcesAsync(ListStackResourcesRequest listStackResourcesRequest);
/**
*
* Returns descriptions of all resources of the specified stack.
*
*
* For deleted stacks, ListStackResources returns resource information for up to 90 days after the stack has been
* deleted.
*
*
* @param listStackResourcesRequest
* The input for the ListStackResource action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStackResources operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.ListStackResources
* @see AWS API Documentation
*/
java.util.concurrent.Future listStackResourcesAsync(ListStackResourcesRequest listStackResourcesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns summary information about the results of a stack set operation.
*
*
* @param listStackSetOperationResultsRequest
* @return A Java Future containing the result of the ListStackSetOperationResults operation returned by the
* service.
* @sample AmazonCloudFormationAsync.ListStackSetOperationResults
* @see AWS API Documentation
*/
java.util.concurrent.Future listStackSetOperationResultsAsync(
ListStackSetOperationResultsRequest listStackSetOperationResultsRequest);
/**
*
* Returns summary information about the results of a stack set operation.
*
*
* @param listStackSetOperationResultsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStackSetOperationResults operation returned by the
* service.
* @sample AmazonCloudFormationAsyncHandler.ListStackSetOperationResults
* @see AWS API Documentation
*/
java.util.concurrent.Future listStackSetOperationResultsAsync(
ListStackSetOperationResultsRequest listStackSetOperationResultsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns summary information about operations performed on a stack set.
*
*
* @param listStackSetOperationsRequest
* @return A Java Future containing the result of the ListStackSetOperations operation returned by the service.
* @sample AmazonCloudFormationAsync.ListStackSetOperations
* @see AWS API Documentation
*/
java.util.concurrent.Future listStackSetOperationsAsync(ListStackSetOperationsRequest listStackSetOperationsRequest);
/**
*
* Returns summary information about operations performed on a stack set.
*
*
* @param listStackSetOperationsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStackSetOperations operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.ListStackSetOperations
* @see AWS API Documentation
*/
java.util.concurrent.Future listStackSetOperationsAsync(ListStackSetOperationsRequest listStackSetOperationsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns summary information about stack sets that are associated with the user.
*
*
* @param listStackSetsRequest
* @return A Java Future containing the result of the ListStackSets operation returned by the service.
* @sample AmazonCloudFormationAsync.ListStackSets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listStackSetsAsync(ListStackSetsRequest listStackSetsRequest);
/**
*
* Returns summary information about stack sets that are associated with the user.
*
*
* @param listStackSetsRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStackSets operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.ListStackSets
* @see AWS
* API Documentation
*/
java.util.concurrent.Future listStackSetsAsync(ListStackSetsRequest listStackSetsRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Returns the summary information for stacks whose status matches the specified StackStatusFilter. Summary
* information for stacks that have been deleted is kept for 90 days after the stack is deleted. If no
* StackStatusFilter is specified, summary information for all stacks is returned (including existing stacks and
* stacks that have been deleted).
*
*
* @param listStacksRequest
* The input for ListStacks action.
* @return A Java Future containing the result of the ListStacks operation returned by the service.
* @sample AmazonCloudFormationAsync.ListStacks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listStacksAsync(ListStacksRequest listStacksRequest);
/**
*
* Returns the summary information for stacks whose status matches the specified StackStatusFilter. Summary
* information for stacks that have been deleted is kept for 90 days after the stack is deleted. If no
* StackStatusFilter is specified, summary information for all stacks is returned (including existing stacks and
* stacks that have been deleted).
*
*
* @param listStacksRequest
* The input for ListStacks action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ListStacks operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.ListStacks
* @see AWS API
* Documentation
*/
java.util.concurrent.Future listStacksAsync(ListStacksRequest listStacksRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
* Simplified method form for invoking the ListStacks operation.
*
* @see #listStacksAsync(ListStacksRequest)
*/
java.util.concurrent.Future listStacksAsync();
/**
* Simplified method form for invoking the ListStacks operation with an AsyncHandler.
*
* @see #listStacksAsync(ListStacksRequest, com.amazonaws.handlers.AsyncHandler)
*/
java.util.concurrent.Future listStacksAsync(com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sets a stack policy for a specified stack.
*
*
* @param setStackPolicyRequest
* The input for the SetStackPolicy action.
* @return A Java Future containing the result of the SetStackPolicy operation returned by the service.
* @sample AmazonCloudFormationAsync.SetStackPolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future setStackPolicyAsync(SetStackPolicyRequest setStackPolicyRequest);
/**
*
* Sets a stack policy for a specified stack.
*
*
* @param setStackPolicyRequest
* The input for the SetStackPolicy action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SetStackPolicy operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.SetStackPolicy
* @see AWS
* API Documentation
*/
java.util.concurrent.Future setStackPolicyAsync(SetStackPolicyRequest setStackPolicyRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Sends a signal to the specified resource with a success or failure status. You can use the SignalResource API in
* conjunction with a creation policy or update policy. AWS CloudFormation doesn't proceed with a stack creation or
* update until resources receive the required number of signals or the timeout period is exceeded. The
* SignalResource API is useful in cases where you want to send signals from anywhere other than an Amazon EC2
* instance.
*
*
* @param signalResourceRequest
* The input for the SignalResource action.
* @return A Java Future containing the result of the SignalResource operation returned by the service.
* @sample AmazonCloudFormationAsync.SignalResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future signalResourceAsync(SignalResourceRequest signalResourceRequest);
/**
*
* Sends a signal to the specified resource with a success or failure status. You can use the SignalResource API in
* conjunction with a creation policy or update policy. AWS CloudFormation doesn't proceed with a stack creation or
* update until resources receive the required number of signals or the timeout period is exceeded. The
* SignalResource API is useful in cases where you want to send signals from anywhere other than an Amazon EC2
* instance.
*
*
* @param signalResourceRequest
* The input for the SignalResource action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the SignalResource operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.SignalResource
* @see AWS
* API Documentation
*/
java.util.concurrent.Future signalResourceAsync(SignalResourceRequest signalResourceRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Stops an in-progress operation on a stack set and its associated stack instances.
*
*
* @param stopStackSetOperationRequest
* @return A Java Future containing the result of the StopStackSetOperation operation returned by the service.
* @sample AmazonCloudFormationAsync.StopStackSetOperation
* @see AWS API Documentation
*/
java.util.concurrent.Future stopStackSetOperationAsync(StopStackSetOperationRequest stopStackSetOperationRequest);
/**
*
* Stops an in-progress operation on a stack set and its associated stack instances.
*
*
* @param stopStackSetOperationRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the StopStackSetOperation operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.StopStackSetOperation
* @see AWS API Documentation
*/
java.util.concurrent.Future stopStackSetOperationAsync(StopStackSetOperationRequest stopStackSetOperationRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates a stack as specified in the template. After the call completes successfully, the stack update starts. You
* can check the status of the stack via the DescribeStacks action.
*
*
* To get a copy of the template for an existing stack, you can use the GetTemplate action.
*
*
* For more information about creating an update template, updating a stack, and monitoring the progress of the
* update, see Updating a
* Stack.
*
*
* @param updateStackRequest
* The input for an UpdateStack action.
* @return A Java Future containing the result of the UpdateStack operation returned by the service.
* @sample AmazonCloudFormationAsync.UpdateStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateStackAsync(UpdateStackRequest updateStackRequest);
/**
*
* Updates a stack as specified in the template. After the call completes successfully, the stack update starts. You
* can check the status of the stack via the DescribeStacks action.
*
*
* To get a copy of the template for an existing stack, you can use the GetTemplate action.
*
*
* For more information about creating an update template, updating a stack, and monitoring the progress of the
* update, see Updating a
* Stack.
*
*
* @param updateStackRequest
* The input for an UpdateStack action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateStack operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.UpdateStack
* @see AWS API
* Documentation
*/
java.util.concurrent.Future updateStackAsync(UpdateStackRequest updateStackRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the parameter values for stack instances for the specified accounts, within the specified regions. A
* stack instance refers to a stack in a specific account and region.
*
*
* You can only update stack instances in regions and accounts where they already exist; to create additional stack
* instances, use CreateStackInstances.
*
*
* During stack set updates, any parameters overridden for a stack instance are not updated, but retain their
* overridden value.
*
*
* You can only update the parameter values that are specified in the stack set; to add or delete a parameter
* itself, use UpdateStackSet
* to update the stack set template. If you add a parameter to a template, before you can override the
* parameter value specified in the stack set you must first use UpdateStackSet to update all stack instances with the updated template and parameter value specified in the
* stack set. Once a stack instance has been updated with the new parameter, you can then override the parameter
* value using UpdateStackInstances
.
*
*
* @param updateStackInstancesRequest
* @return A Java Future containing the result of the UpdateStackInstances operation returned by the service.
* @sample AmazonCloudFormationAsync.UpdateStackInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future updateStackInstancesAsync(UpdateStackInstancesRequest updateStackInstancesRequest);
/**
*
* Updates the parameter values for stack instances for the specified accounts, within the specified regions. A
* stack instance refers to a stack in a specific account and region.
*
*
* You can only update stack instances in regions and accounts where they already exist; to create additional stack
* instances, use CreateStackInstances.
*
*
* During stack set updates, any parameters overridden for a stack instance are not updated, but retain their
* overridden value.
*
*
* You can only update the parameter values that are specified in the stack set; to add or delete a parameter
* itself, use UpdateStackSet
* to update the stack set template. If you add a parameter to a template, before you can override the
* parameter value specified in the stack set you must first use UpdateStackSet to update all stack instances with the updated template and parameter value specified in the
* stack set. Once a stack instance has been updated with the new parameter, you can then override the parameter
* value using UpdateStackInstances
.
*
*
* @param updateStackInstancesRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateStackInstances operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.UpdateStackInstances
* @see AWS API Documentation
*/
java.util.concurrent.Future updateStackInstancesAsync(UpdateStackInstancesRequest updateStackInstancesRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates the stack set, and associated stack instances in the specified accounts and regions.
*
*
* Even if the stack set operation created by updating the stack set fails (completely or partially, below or above
* a specified failure tolerance), the stack set is updated with your changes. Subsequent
* CreateStackInstances calls on the specified stack set use the updated stack set.
*
*
* @param updateStackSetRequest
* @return A Java Future containing the result of the UpdateStackSet operation returned by the service.
* @sample AmazonCloudFormationAsync.UpdateStackSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateStackSetAsync(UpdateStackSetRequest updateStackSetRequest);
/**
*
* Updates the stack set, and associated stack instances in the specified accounts and regions.
*
*
* Even if the stack set operation created by updating the stack set fails (completely or partially, below or above
* a specified failure tolerance), the stack set is updated with your changes. Subsequent
* CreateStackInstances calls on the specified stack set use the updated stack set.
*
*
* @param updateStackSetRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateStackSet operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.UpdateStackSet
* @see AWS
* API Documentation
*/
java.util.concurrent.Future updateStackSetAsync(UpdateStackSetRequest updateStackSetRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Updates termination protection for the specified stack. If a user attempts to delete a stack with termination
* protection enabled, the operation fails and the stack remains unchanged. For more information, see Protecting a Stack From Being Deleted
* in the AWS CloudFormation User Guide.
*
*
* For nested stacks, termination
* protection is set on the root stack and cannot be changed directly on the nested stack.
*
*
* @param updateTerminationProtectionRequest
* @return A Java Future containing the result of the UpdateTerminationProtection operation returned by the service.
* @sample AmazonCloudFormationAsync.UpdateTerminationProtection
* @see AWS API Documentation
*/
java.util.concurrent.Future updateTerminationProtectionAsync(
UpdateTerminationProtectionRequest updateTerminationProtectionRequest);
/**
*
* Updates termination protection for the specified stack. If a user attempts to delete a stack with termination
* protection enabled, the operation fails and the stack remains unchanged. For more information, see Protecting a Stack From Being Deleted
* in the AWS CloudFormation User Guide.
*
*
* For nested stacks, termination
* protection is set on the root stack and cannot be changed directly on the nested stack.
*
*
* @param updateTerminationProtectionRequest
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the UpdateTerminationProtection operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.UpdateTerminationProtection
* @see AWS API Documentation
*/
java.util.concurrent.Future updateTerminationProtectionAsync(
UpdateTerminationProtectionRequest updateTerminationProtectionRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
/**
*
* Validates a specified template. AWS CloudFormation first checks if the template is valid JSON. If it isn't, AWS
* CloudFormation checks if the template is valid YAML. If both these checks fail, AWS CloudFormation returns a
* template validation error.
*
*
* @param validateTemplateRequest
* The input for ValidateTemplate action.
* @return A Java Future containing the result of the ValidateTemplate operation returned by the service.
* @sample AmazonCloudFormationAsync.ValidateTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future validateTemplateAsync(ValidateTemplateRequest validateTemplateRequest);
/**
*
* Validates a specified template. AWS CloudFormation first checks if the template is valid JSON. If it isn't, AWS
* CloudFormation checks if the template is valid YAML. If both these checks fail, AWS CloudFormation returns a
* template validation error.
*
*
* @param validateTemplateRequest
* The input for ValidateTemplate action.
* @param asyncHandler
* Asynchronous callback handler for events in the lifecycle of the request. Users can provide an
* implementation of the callback methods in this interface to receive notification of successful or
* unsuccessful completion of the operation.
* @return A Java Future containing the result of the ValidateTemplate operation returned by the service.
* @sample AmazonCloudFormationAsyncHandler.ValidateTemplate
* @see AWS API Documentation
*/
java.util.concurrent.Future validateTemplateAsync(ValidateTemplateRequest validateTemplateRequest,
com.amazonaws.handlers.AsyncHandler asyncHandler);
}