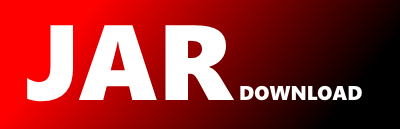
com.amazonaws.services.cloudformation.model.StackSetOperation Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudformation.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* The structure that contains information about a stack set operation.
*
*
* @see AWS
* API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StackSetOperation implements Serializable, Cloneable {
/**
*
* The unique ID of a stack set operation.
*
*/
private String operationId;
/**
*
* The ID of the stack set.
*
*/
private String stackSetId;
/**
*
* The type of stack set operation: CREATE
, UPDATE
, or DELETE
. Create and
* delete operations affect only the specified stack set instances that are associated with the specified stack set.
* Update operations affect both the stack set itself, as well as all associated stack set instances.
*
*/
private String action;
/**
*
* The status of the operation.
*
*
* -
*
* FAILED
: The operation exceeded the specified failure tolerance. The failure tolerance value that
* you've set for an operation is applied for each region during stack create and update operations. If the number
* of failed stacks within a region exceeds the failure tolerance, the status of the operation in the region is set
* to FAILED
. This in turn sets the status of the operation as a whole to FAILED
, and AWS
* CloudFormation cancels the operation in any remaining regions.
*
*
* -
*
* RUNNING
: The operation is currently being performed.
*
*
* -
*
* STOPPED
: The user has cancelled the operation.
*
*
* -
*
* STOPPING
: The operation is in the process of stopping, at user request.
*
*
* -
*
* SUCCEEDED
: The operation completed creating or updating all the specified stacks without exceeding
* the failure tolerance for the operation.
*
*
*
*/
private String status;
/**
*
* The preferences for how AWS CloudFormation performs this stack set operation.
*
*/
private StackSetOperationPreferences operationPreferences;
/**
*
* For stack set operations of action type DELETE
, specifies whether to remove the stack instances from
* the specified stack set, but doesn't delete the stacks. You can't reassociate a retained stack, or add an
* existing, saved stack to a new stack set.
*
*/
private Boolean retainStacks;
/**
*
* The Amazon Resource Number (ARN) of the IAM role used to perform this stack set operation.
*
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within the
* same administrator account. For more information, see Define Permissions
* for Multiple Administrators in the AWS CloudFormation User Guide.
*
*/
private String administrationRoleARN;
/**
*
* The name of the IAM execution role used to create or update the stack set.
*
*
* Use customized execution roles to control which stack resources users and groups can include in their stack sets.
*
*/
private String executionRoleName;
/**
*
* The time at which the operation was initiated. Note that the creation times for the stack set operation might
* differ from the creation time of the individual stacks themselves. This is because AWS CloudFormation needs to
* perform preparatory work for the operation, such as dispatching the work to the requested regions, before
* actually creating the first stacks.
*
*/
private java.util.Date creationTimestamp;
/**
*
* The time at which the stack set operation ended, across all accounts and regions specified. Note that this
* doesn't necessarily mean that the stack set operation was successful, or even attempted, in each account or
* region.
*
*/
private java.util.Date endTimestamp;
/**
*
* The unique ID of a stack set operation.
*
*
* @param operationId
* The unique ID of a stack set operation.
*/
public void setOperationId(String operationId) {
this.operationId = operationId;
}
/**
*
* The unique ID of a stack set operation.
*
*
* @return The unique ID of a stack set operation.
*/
public String getOperationId() {
return this.operationId;
}
/**
*
* The unique ID of a stack set operation.
*
*
* @param operationId
* The unique ID of a stack set operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetOperation withOperationId(String operationId) {
setOperationId(operationId);
return this;
}
/**
*
* The ID of the stack set.
*
*
* @param stackSetId
* The ID of the stack set.
*/
public void setStackSetId(String stackSetId) {
this.stackSetId = stackSetId;
}
/**
*
* The ID of the stack set.
*
*
* @return The ID of the stack set.
*/
public String getStackSetId() {
return this.stackSetId;
}
/**
*
* The ID of the stack set.
*
*
* @param stackSetId
* The ID of the stack set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetOperation withStackSetId(String stackSetId) {
setStackSetId(stackSetId);
return this;
}
/**
*
* The type of stack set operation: CREATE
, UPDATE
, or DELETE
. Create and
* delete operations affect only the specified stack set instances that are associated with the specified stack set.
* Update operations affect both the stack set itself, as well as all associated stack set instances.
*
*
* @param action
* The type of stack set operation: CREATE
, UPDATE
, or DELETE
. Create
* and delete operations affect only the specified stack set instances that are associated with the specified
* stack set. Update operations affect both the stack set itself, as well as all associated stack set
* instances.
* @see StackSetOperationAction
*/
public void setAction(String action) {
this.action = action;
}
/**
*
* The type of stack set operation: CREATE
, UPDATE
, or DELETE
. Create and
* delete operations affect only the specified stack set instances that are associated with the specified stack set.
* Update operations affect both the stack set itself, as well as all associated stack set instances.
*
*
* @return The type of stack set operation: CREATE
, UPDATE
, or DELETE
. Create
* and delete operations affect only the specified stack set instances that are associated with the
* specified stack set. Update operations affect both the stack set itself, as well as all associated
* stack set instances.
* @see StackSetOperationAction
*/
public String getAction() {
return this.action;
}
/**
*
* The type of stack set operation: CREATE
, UPDATE
, or DELETE
. Create and
* delete operations affect only the specified stack set instances that are associated with the specified stack set.
* Update operations affect both the stack set itself, as well as all associated stack set instances.
*
*
* @param action
* The type of stack set operation: CREATE
, UPDATE
, or DELETE
. Create
* and delete operations affect only the specified stack set instances that are associated with the specified
* stack set. Update operations affect both the stack set itself, as well as all associated stack set
* instances.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackSetOperationAction
*/
public StackSetOperation withAction(String action) {
setAction(action);
return this;
}
/**
*
* The type of stack set operation: CREATE
, UPDATE
, or DELETE
. Create and
* delete operations affect only the specified stack set instances that are associated with the specified stack set.
* Update operations affect both the stack set itself, as well as all associated stack set instances.
*
*
* @param action
* The type of stack set operation: CREATE
, UPDATE
, or DELETE
. Create
* and delete operations affect only the specified stack set instances that are associated with the specified
* stack set. Update operations affect both the stack set itself, as well as all associated stack set
* instances.
* @see StackSetOperationAction
*/
public void setAction(StackSetOperationAction action) {
withAction(action);
}
/**
*
* The type of stack set operation: CREATE
, UPDATE
, or DELETE
. Create and
* delete operations affect only the specified stack set instances that are associated with the specified stack set.
* Update operations affect both the stack set itself, as well as all associated stack set instances.
*
*
* @param action
* The type of stack set operation: CREATE
, UPDATE
, or DELETE
. Create
* and delete operations affect only the specified stack set instances that are associated with the specified
* stack set. Update operations affect both the stack set itself, as well as all associated stack set
* instances.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackSetOperationAction
*/
public StackSetOperation withAction(StackSetOperationAction action) {
this.action = action.toString();
return this;
}
/**
*
* The status of the operation.
*
*
* -
*
* FAILED
: The operation exceeded the specified failure tolerance. The failure tolerance value that
* you've set for an operation is applied for each region during stack create and update operations. If the number
* of failed stacks within a region exceeds the failure tolerance, the status of the operation in the region is set
* to FAILED
. This in turn sets the status of the operation as a whole to FAILED
, and AWS
* CloudFormation cancels the operation in any remaining regions.
*
*
* -
*
* RUNNING
: The operation is currently being performed.
*
*
* -
*
* STOPPED
: The user has cancelled the operation.
*
*
* -
*
* STOPPING
: The operation is in the process of stopping, at user request.
*
*
* -
*
* SUCCEEDED
: The operation completed creating or updating all the specified stacks without exceeding
* the failure tolerance for the operation.
*
*
*
*
* @param status
* The status of the operation.
*
* -
*
* FAILED
: The operation exceeded the specified failure tolerance. The failure tolerance value
* that you've set for an operation is applied for each region during stack create and update operations. If
* the number of failed stacks within a region exceeds the failure tolerance, the status of the operation in
* the region is set to FAILED
. This in turn sets the status of the operation as a whole to
* FAILED
, and AWS CloudFormation cancels the operation in any remaining regions.
*
*
* -
*
* RUNNING
: The operation is currently being performed.
*
*
* -
*
* STOPPED
: The user has cancelled the operation.
*
*
* -
*
* STOPPING
: The operation is in the process of stopping, at user request.
*
*
* -
*
* SUCCEEDED
: The operation completed creating or updating all the specified stacks without
* exceeding the failure tolerance for the operation.
*
*
* @see StackSetOperationStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the operation.
*
*
* -
*
* FAILED
: The operation exceeded the specified failure tolerance. The failure tolerance value that
* you've set for an operation is applied for each region during stack create and update operations. If the number
* of failed stacks within a region exceeds the failure tolerance, the status of the operation in the region is set
* to FAILED
. This in turn sets the status of the operation as a whole to FAILED
, and AWS
* CloudFormation cancels the operation in any remaining regions.
*
*
* -
*
* RUNNING
: The operation is currently being performed.
*
*
* -
*
* STOPPED
: The user has cancelled the operation.
*
*
* -
*
* STOPPING
: The operation is in the process of stopping, at user request.
*
*
* -
*
* SUCCEEDED
: The operation completed creating or updating all the specified stacks without exceeding
* the failure tolerance for the operation.
*
*
*
*
* @return The status of the operation.
*
* -
*
* FAILED
: The operation exceeded the specified failure tolerance. The failure tolerance value
* that you've set for an operation is applied for each region during stack create and update operations. If
* the number of failed stacks within a region exceeds the failure tolerance, the status of the operation in
* the region is set to FAILED
. This in turn sets the status of the operation as a whole to
* FAILED
, and AWS CloudFormation cancels the operation in any remaining regions.
*
*
* -
*
* RUNNING
: The operation is currently being performed.
*
*
* -
*
* STOPPED
: The user has cancelled the operation.
*
*
* -
*
* STOPPING
: The operation is in the process of stopping, at user request.
*
*
* -
*
* SUCCEEDED
: The operation completed creating or updating all the specified stacks without
* exceeding the failure tolerance for the operation.
*
*
* @see StackSetOperationStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the operation.
*
*
* -
*
* FAILED
: The operation exceeded the specified failure tolerance. The failure tolerance value that
* you've set for an operation is applied for each region during stack create and update operations. If the number
* of failed stacks within a region exceeds the failure tolerance, the status of the operation in the region is set
* to FAILED
. This in turn sets the status of the operation as a whole to FAILED
, and AWS
* CloudFormation cancels the operation in any remaining regions.
*
*
* -
*
* RUNNING
: The operation is currently being performed.
*
*
* -
*
* STOPPED
: The user has cancelled the operation.
*
*
* -
*
* STOPPING
: The operation is in the process of stopping, at user request.
*
*
* -
*
* SUCCEEDED
: The operation completed creating or updating all the specified stacks without exceeding
* the failure tolerance for the operation.
*
*
*
*
* @param status
* The status of the operation.
*
* -
*
* FAILED
: The operation exceeded the specified failure tolerance. The failure tolerance value
* that you've set for an operation is applied for each region during stack create and update operations. If
* the number of failed stacks within a region exceeds the failure tolerance, the status of the operation in
* the region is set to FAILED
. This in turn sets the status of the operation as a whole to
* FAILED
, and AWS CloudFormation cancels the operation in any remaining regions.
*
*
* -
*
* RUNNING
: The operation is currently being performed.
*
*
* -
*
* STOPPED
: The user has cancelled the operation.
*
*
* -
*
* STOPPING
: The operation is in the process of stopping, at user request.
*
*
* -
*
* SUCCEEDED
: The operation completed creating or updating all the specified stacks without
* exceeding the failure tolerance for the operation.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackSetOperationStatus
*/
public StackSetOperation withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the operation.
*
*
* -
*
* FAILED
: The operation exceeded the specified failure tolerance. The failure tolerance value that
* you've set for an operation is applied for each region during stack create and update operations. If the number
* of failed stacks within a region exceeds the failure tolerance, the status of the operation in the region is set
* to FAILED
. This in turn sets the status of the operation as a whole to FAILED
, and AWS
* CloudFormation cancels the operation in any remaining regions.
*
*
* -
*
* RUNNING
: The operation is currently being performed.
*
*
* -
*
* STOPPED
: The user has cancelled the operation.
*
*
* -
*
* STOPPING
: The operation is in the process of stopping, at user request.
*
*
* -
*
* SUCCEEDED
: The operation completed creating or updating all the specified stacks without exceeding
* the failure tolerance for the operation.
*
*
*
*
* @param status
* The status of the operation.
*
* -
*
* FAILED
: The operation exceeded the specified failure tolerance. The failure tolerance value
* that you've set for an operation is applied for each region during stack create and update operations. If
* the number of failed stacks within a region exceeds the failure tolerance, the status of the operation in
* the region is set to FAILED
. This in turn sets the status of the operation as a whole to
* FAILED
, and AWS CloudFormation cancels the operation in any remaining regions.
*
*
* -
*
* RUNNING
: The operation is currently being performed.
*
*
* -
*
* STOPPED
: The user has cancelled the operation.
*
*
* -
*
* STOPPING
: The operation is in the process of stopping, at user request.
*
*
* -
*
* SUCCEEDED
: The operation completed creating or updating all the specified stacks without
* exceeding the failure tolerance for the operation.
*
*
* @see StackSetOperationStatus
*/
public void setStatus(StackSetOperationStatus status) {
withStatus(status);
}
/**
*
* The status of the operation.
*
*
* -
*
* FAILED
: The operation exceeded the specified failure tolerance. The failure tolerance value that
* you've set for an operation is applied for each region during stack create and update operations. If the number
* of failed stacks within a region exceeds the failure tolerance, the status of the operation in the region is set
* to FAILED
. This in turn sets the status of the operation as a whole to FAILED
, and AWS
* CloudFormation cancels the operation in any remaining regions.
*
*
* -
*
* RUNNING
: The operation is currently being performed.
*
*
* -
*
* STOPPED
: The user has cancelled the operation.
*
*
* -
*
* STOPPING
: The operation is in the process of stopping, at user request.
*
*
* -
*
* SUCCEEDED
: The operation completed creating or updating all the specified stacks without exceeding
* the failure tolerance for the operation.
*
*
*
*
* @param status
* The status of the operation.
*
* -
*
* FAILED
: The operation exceeded the specified failure tolerance. The failure tolerance value
* that you've set for an operation is applied for each region during stack create and update operations. If
* the number of failed stacks within a region exceeds the failure tolerance, the status of the operation in
* the region is set to FAILED
. This in turn sets the status of the operation as a whole to
* FAILED
, and AWS CloudFormation cancels the operation in any remaining regions.
*
*
* -
*
* RUNNING
: The operation is currently being performed.
*
*
* -
*
* STOPPED
: The user has cancelled the operation.
*
*
* -
*
* STOPPING
: The operation is in the process of stopping, at user request.
*
*
* -
*
* SUCCEEDED
: The operation completed creating or updating all the specified stacks without
* exceeding the failure tolerance for the operation.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackSetOperationStatus
*/
public StackSetOperation withStatus(StackSetOperationStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The preferences for how AWS CloudFormation performs this stack set operation.
*
*
* @param operationPreferences
* The preferences for how AWS CloudFormation performs this stack set operation.
*/
public void setOperationPreferences(StackSetOperationPreferences operationPreferences) {
this.operationPreferences = operationPreferences;
}
/**
*
* The preferences for how AWS CloudFormation performs this stack set operation.
*
*
* @return The preferences for how AWS CloudFormation performs this stack set operation.
*/
public StackSetOperationPreferences getOperationPreferences() {
return this.operationPreferences;
}
/**
*
* The preferences for how AWS CloudFormation performs this stack set operation.
*
*
* @param operationPreferences
* The preferences for how AWS CloudFormation performs this stack set operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetOperation withOperationPreferences(StackSetOperationPreferences operationPreferences) {
setOperationPreferences(operationPreferences);
return this;
}
/**
*
* For stack set operations of action type DELETE
, specifies whether to remove the stack instances from
* the specified stack set, but doesn't delete the stacks. You can't reassociate a retained stack, or add an
* existing, saved stack to a new stack set.
*
*
* @param retainStacks
* For stack set operations of action type DELETE
, specifies whether to remove the stack
* instances from the specified stack set, but doesn't delete the stacks. You can't reassociate a retained
* stack, or add an existing, saved stack to a new stack set.
*/
public void setRetainStacks(Boolean retainStacks) {
this.retainStacks = retainStacks;
}
/**
*
* For stack set operations of action type DELETE
, specifies whether to remove the stack instances from
* the specified stack set, but doesn't delete the stacks. You can't reassociate a retained stack, or add an
* existing, saved stack to a new stack set.
*
*
* @return For stack set operations of action type DELETE
, specifies whether to remove the stack
* instances from the specified stack set, but doesn't delete the stacks. You can't reassociate a retained
* stack, or add an existing, saved stack to a new stack set.
*/
public Boolean getRetainStacks() {
return this.retainStacks;
}
/**
*
* For stack set operations of action type DELETE
, specifies whether to remove the stack instances from
* the specified stack set, but doesn't delete the stacks. You can't reassociate a retained stack, or add an
* existing, saved stack to a new stack set.
*
*
* @param retainStacks
* For stack set operations of action type DELETE
, specifies whether to remove the stack
* instances from the specified stack set, but doesn't delete the stacks. You can't reassociate a retained
* stack, or add an existing, saved stack to a new stack set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetOperation withRetainStacks(Boolean retainStacks) {
setRetainStacks(retainStacks);
return this;
}
/**
*
* For stack set operations of action type DELETE
, specifies whether to remove the stack instances from
* the specified stack set, but doesn't delete the stacks. You can't reassociate a retained stack, or add an
* existing, saved stack to a new stack set.
*
*
* @return For stack set operations of action type DELETE
, specifies whether to remove the stack
* instances from the specified stack set, but doesn't delete the stacks. You can't reassociate a retained
* stack, or add an existing, saved stack to a new stack set.
*/
public Boolean isRetainStacks() {
return this.retainStacks;
}
/**
*
* The Amazon Resource Number (ARN) of the IAM role used to perform this stack set operation.
*
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within the
* same administrator account. For more information, see Define Permissions
* for Multiple Administrators in the AWS CloudFormation User Guide.
*
*
* @param administrationRoleARN
* The Amazon Resource Number (ARN) of the IAM role used to perform this stack set operation.
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within
* the same administrator account. For more information, see Define
* Permissions for Multiple Administrators in the AWS CloudFormation User Guide.
*/
public void setAdministrationRoleARN(String administrationRoleARN) {
this.administrationRoleARN = administrationRoleARN;
}
/**
*
* The Amazon Resource Number (ARN) of the IAM role used to perform this stack set operation.
*
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within the
* same administrator account. For more information, see Define Permissions
* for Multiple Administrators in the AWS CloudFormation User Guide.
*
*
* @return The Amazon Resource Number (ARN) of the IAM role used to perform this stack set operation.
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within
* the same administrator account. For more information, see Define
* Permissions for Multiple Administrators in the AWS CloudFormation User Guide.
*/
public String getAdministrationRoleARN() {
return this.administrationRoleARN;
}
/**
*
* The Amazon Resource Number (ARN) of the IAM role used to perform this stack set operation.
*
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within the
* same administrator account. For more information, see Define Permissions
* for Multiple Administrators in the AWS CloudFormation User Guide.
*
*
* @param administrationRoleARN
* The Amazon Resource Number (ARN) of the IAM role used to perform this stack set operation.
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within
* the same administrator account. For more information, see Define
* Permissions for Multiple Administrators in the AWS CloudFormation User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetOperation withAdministrationRoleARN(String administrationRoleARN) {
setAdministrationRoleARN(administrationRoleARN);
return this;
}
/**
*
* The name of the IAM execution role used to create or update the stack set.
*
*
* Use customized execution roles to control which stack resources users and groups can include in their stack sets.
*
*
* @param executionRoleName
* The name of the IAM execution role used to create or update the stack set.
*
* Use customized execution roles to control which stack resources users and groups can include in their
* stack sets.
*/
public void setExecutionRoleName(String executionRoleName) {
this.executionRoleName = executionRoleName;
}
/**
*
* The name of the IAM execution role used to create or update the stack set.
*
*
* Use customized execution roles to control which stack resources users and groups can include in their stack sets.
*
*
* @return The name of the IAM execution role used to create or update the stack set.
*
* Use customized execution roles to control which stack resources users and groups can include in their
* stack sets.
*/
public String getExecutionRoleName() {
return this.executionRoleName;
}
/**
*
* The name of the IAM execution role used to create or update the stack set.
*
*
* Use customized execution roles to control which stack resources users and groups can include in their stack sets.
*
*
* @param executionRoleName
* The name of the IAM execution role used to create or update the stack set.
*
* Use customized execution roles to control which stack resources users and groups can include in their
* stack sets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetOperation withExecutionRoleName(String executionRoleName) {
setExecutionRoleName(executionRoleName);
return this;
}
/**
*
* The time at which the operation was initiated. Note that the creation times for the stack set operation might
* differ from the creation time of the individual stacks themselves. This is because AWS CloudFormation needs to
* perform preparatory work for the operation, such as dispatching the work to the requested regions, before
* actually creating the first stacks.
*
*
* @param creationTimestamp
* The time at which the operation was initiated. Note that the creation times for the stack set operation
* might differ from the creation time of the individual stacks themselves. This is because AWS
* CloudFormation needs to perform preparatory work for the operation, such as dispatching the work to the
* requested regions, before actually creating the first stacks.
*/
public void setCreationTimestamp(java.util.Date creationTimestamp) {
this.creationTimestamp = creationTimestamp;
}
/**
*
* The time at which the operation was initiated. Note that the creation times for the stack set operation might
* differ from the creation time of the individual stacks themselves. This is because AWS CloudFormation needs to
* perform preparatory work for the operation, such as dispatching the work to the requested regions, before
* actually creating the first stacks.
*
*
* @return The time at which the operation was initiated. Note that the creation times for the stack set operation
* might differ from the creation time of the individual stacks themselves. This is because AWS
* CloudFormation needs to perform preparatory work for the operation, such as dispatching the work to the
* requested regions, before actually creating the first stacks.
*/
public java.util.Date getCreationTimestamp() {
return this.creationTimestamp;
}
/**
*
* The time at which the operation was initiated. Note that the creation times for the stack set operation might
* differ from the creation time of the individual stacks themselves. This is because AWS CloudFormation needs to
* perform preparatory work for the operation, such as dispatching the work to the requested regions, before
* actually creating the first stacks.
*
*
* @param creationTimestamp
* The time at which the operation was initiated. Note that the creation times for the stack set operation
* might differ from the creation time of the individual stacks themselves. This is because AWS
* CloudFormation needs to perform preparatory work for the operation, such as dispatching the work to the
* requested regions, before actually creating the first stacks.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetOperation withCreationTimestamp(java.util.Date creationTimestamp) {
setCreationTimestamp(creationTimestamp);
return this;
}
/**
*
* The time at which the stack set operation ended, across all accounts and regions specified. Note that this
* doesn't necessarily mean that the stack set operation was successful, or even attempted, in each account or
* region.
*
*
* @param endTimestamp
* The time at which the stack set operation ended, across all accounts and regions specified. Note that this
* doesn't necessarily mean that the stack set operation was successful, or even attempted, in each account
* or region.
*/
public void setEndTimestamp(java.util.Date endTimestamp) {
this.endTimestamp = endTimestamp;
}
/**
*
* The time at which the stack set operation ended, across all accounts and regions specified. Note that this
* doesn't necessarily mean that the stack set operation was successful, or even attempted, in each account or
* region.
*
*
* @return The time at which the stack set operation ended, across all accounts and regions specified. Note that
* this doesn't necessarily mean that the stack set operation was successful, or even attempted, in each
* account or region.
*/
public java.util.Date getEndTimestamp() {
return this.endTimestamp;
}
/**
*
* The time at which the stack set operation ended, across all accounts and regions specified. Note that this
* doesn't necessarily mean that the stack set operation was successful, or even attempted, in each account or
* region.
*
*
* @param endTimestamp
* The time at which the stack set operation ended, across all accounts and regions specified. Note that this
* doesn't necessarily mean that the stack set operation was successful, or even attempted, in each account
* or region.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetOperation withEndTimestamp(java.util.Date endTimestamp) {
setEndTimestamp(endTimestamp);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getOperationId() != null)
sb.append("OperationId: ").append(getOperationId()).append(",");
if (getStackSetId() != null)
sb.append("StackSetId: ").append(getStackSetId()).append(",");
if (getAction() != null)
sb.append("Action: ").append(getAction()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getOperationPreferences() != null)
sb.append("OperationPreferences: ").append(getOperationPreferences()).append(",");
if (getRetainStacks() != null)
sb.append("RetainStacks: ").append(getRetainStacks()).append(",");
if (getAdministrationRoleARN() != null)
sb.append("AdministrationRoleARN: ").append(getAdministrationRoleARN()).append(",");
if (getExecutionRoleName() != null)
sb.append("ExecutionRoleName: ").append(getExecutionRoleName()).append(",");
if (getCreationTimestamp() != null)
sb.append("CreationTimestamp: ").append(getCreationTimestamp()).append(",");
if (getEndTimestamp() != null)
sb.append("EndTimestamp: ").append(getEndTimestamp());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StackSetOperation == false)
return false;
StackSetOperation other = (StackSetOperation) obj;
if (other.getOperationId() == null ^ this.getOperationId() == null)
return false;
if (other.getOperationId() != null && other.getOperationId().equals(this.getOperationId()) == false)
return false;
if (other.getStackSetId() == null ^ this.getStackSetId() == null)
return false;
if (other.getStackSetId() != null && other.getStackSetId().equals(this.getStackSetId()) == false)
return false;
if (other.getAction() == null ^ this.getAction() == null)
return false;
if (other.getAction() != null && other.getAction().equals(this.getAction()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getOperationPreferences() == null ^ this.getOperationPreferences() == null)
return false;
if (other.getOperationPreferences() != null && other.getOperationPreferences().equals(this.getOperationPreferences()) == false)
return false;
if (other.getRetainStacks() == null ^ this.getRetainStacks() == null)
return false;
if (other.getRetainStacks() != null && other.getRetainStacks().equals(this.getRetainStacks()) == false)
return false;
if (other.getAdministrationRoleARN() == null ^ this.getAdministrationRoleARN() == null)
return false;
if (other.getAdministrationRoleARN() != null && other.getAdministrationRoleARN().equals(this.getAdministrationRoleARN()) == false)
return false;
if (other.getExecutionRoleName() == null ^ this.getExecutionRoleName() == null)
return false;
if (other.getExecutionRoleName() != null && other.getExecutionRoleName().equals(this.getExecutionRoleName()) == false)
return false;
if (other.getCreationTimestamp() == null ^ this.getCreationTimestamp() == null)
return false;
if (other.getCreationTimestamp() != null && other.getCreationTimestamp().equals(this.getCreationTimestamp()) == false)
return false;
if (other.getEndTimestamp() == null ^ this.getEndTimestamp() == null)
return false;
if (other.getEndTimestamp() != null && other.getEndTimestamp().equals(this.getEndTimestamp()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getOperationId() == null) ? 0 : getOperationId().hashCode());
hashCode = prime * hashCode + ((getStackSetId() == null) ? 0 : getStackSetId().hashCode());
hashCode = prime * hashCode + ((getAction() == null) ? 0 : getAction().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getOperationPreferences() == null) ? 0 : getOperationPreferences().hashCode());
hashCode = prime * hashCode + ((getRetainStacks() == null) ? 0 : getRetainStacks().hashCode());
hashCode = prime * hashCode + ((getAdministrationRoleARN() == null) ? 0 : getAdministrationRoleARN().hashCode());
hashCode = prime * hashCode + ((getExecutionRoleName() == null) ? 0 : getExecutionRoleName().hashCode());
hashCode = prime * hashCode + ((getCreationTimestamp() == null) ? 0 : getCreationTimestamp().hashCode());
hashCode = prime * hashCode + ((getEndTimestamp() == null) ? 0 : getEndTimestamp().hashCode());
return hashCode;
}
@Override
public StackSetOperation clone() {
try {
return (StackSetOperation) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}