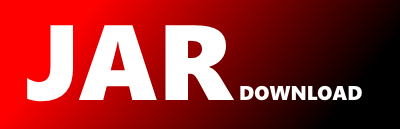
com.amazonaws.services.cloudformation.model.CreateStackSetRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2014-2019 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudformation.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateStackSetRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name to associate with the stack set. The name must be unique in the region where you create your stack set.
*
*
*
* A stack name can contain only alphanumeric characters (case-sensitive) and hyphens. It must start with an
* alphabetic character and can't be longer than 128 characters.
*
*
*/
private String stackSetName;
/**
*
* A description of the stack set. You can use the description to identify the stack set's purpose or other
* important information.
*
*/
private String description;
/**
*
* The structure that contains the template body, with a minimum length of 1 byte and a maximum length of 51,200
* bytes. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*
*/
private String templateBody;
/**
*
* The location of the file that contains the template body. The URL must point to a template (maximum size: 460,800
* bytes) that's located in an Amazon S3 bucket. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*
*/
private String templateURL;
/**
*
* The input parameters for the stack set template.
*
*/
private com.amazonaws.internal.SdkInternalList parameters;
/**
*
* In some cases, you must explicitly acknowledge that your stack set template contains certain capabilities in
* order for AWS CloudFormation to create the stack set and related stack instances.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to create a
* stack directly from the processed template, without first reviewing the resulting changes in a change set, you
* must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
*
*/
private com.amazonaws.internal.SdkInternalList capabilities;
/**
*
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation also
* propagates these tags to supported resources that are created in the stacks. A maximum number of 50 tags can be
* specified.
*
*
* If you specify tags as part of a CreateStackSet
action, AWS CloudFormation checks to see if you have
* the required IAM permission to tag resources. If you don't, the entire CreateStackSet
action fails
* with an access denied
error, and the stack set is not created.
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The Amazon Resource Number (ARN) of the IAM role to use to create this stack set.
*
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups can
* manage specific stack sets within the same administrator account. For more information, see Prerequisites:
* Granting Permissions for Stack Set Operations in the AWS CloudFormation User Guide.
*
*/
private String administrationRoleARN;
/**
*
* The name of the IAM execution role to use to create the stack set. If you do not specify an execution role, AWS
* CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack set operation.
*
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources users and
* groups can include in their stack sets.
*
*/
private String executionRoleName;
/**
*
* A unique identifier for this CreateStackSet
request. Specify this token if you plan to retry
* requests so that AWS CloudFormation knows that you're not attempting to create another stack set with the same
* name. You might retry CreateStackSet
requests to ensure that AWS CloudFormation successfully
* received them.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*/
private String clientRequestToken;
/**
*
* The name to associate with the stack set. The name must be unique in the region where you create your stack set.
*
*
*
* A stack name can contain only alphanumeric characters (case-sensitive) and hyphens. It must start with an
* alphabetic character and can't be longer than 128 characters.
*
*
*
* @param stackSetName
* The name to associate with the stack set. The name must be unique in the region where you create your
* stack set.
*
* A stack name can contain only alphanumeric characters (case-sensitive) and hyphens. It must start with an
* alphabetic character and can't be longer than 128 characters.
*
*/
public void setStackSetName(String stackSetName) {
this.stackSetName = stackSetName;
}
/**
*
* The name to associate with the stack set. The name must be unique in the region where you create your stack set.
*
*
*
* A stack name can contain only alphanumeric characters (case-sensitive) and hyphens. It must start with an
* alphabetic character and can't be longer than 128 characters.
*
*
*
* @return The name to associate with the stack set. The name must be unique in the region where you create your
* stack set.
*
* A stack name can contain only alphanumeric characters (case-sensitive) and hyphens. It must start with an
* alphabetic character and can't be longer than 128 characters.
*
*/
public String getStackSetName() {
return this.stackSetName;
}
/**
*
* The name to associate with the stack set. The name must be unique in the region where you create your stack set.
*
*
*
* A stack name can contain only alphanumeric characters (case-sensitive) and hyphens. It must start with an
* alphabetic character and can't be longer than 128 characters.
*
*
*
* @param stackSetName
* The name to associate with the stack set. The name must be unique in the region where you create your
* stack set.
*
* A stack name can contain only alphanumeric characters (case-sensitive) and hyphens. It must start with an
* alphabetic character and can't be longer than 128 characters.
*
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackSetRequest withStackSetName(String stackSetName) {
setStackSetName(stackSetName);
return this;
}
/**
*
* A description of the stack set. You can use the description to identify the stack set's purpose or other
* important information.
*
*
* @param description
* A description of the stack set. You can use the description to identify the stack set's purpose or other
* important information.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the stack set. You can use the description to identify the stack set's purpose or other
* important information.
*
*
* @return A description of the stack set. You can use the description to identify the stack set's purpose or other
* important information.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the stack set. You can use the description to identify the stack set's purpose or other
* important information.
*
*
* @param description
* A description of the stack set. You can use the description to identify the stack set's purpose or other
* important information.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackSetRequest withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The structure that contains the template body, with a minimum length of 1 byte and a maximum length of 51,200
* bytes. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*
*
* @param templateBody
* The structure that contains the template body, with a minimum length of 1 byte and a maximum length of
* 51,200 bytes. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*/
public void setTemplateBody(String templateBody) {
this.templateBody = templateBody;
}
/**
*
* The structure that contains the template body, with a minimum length of 1 byte and a maximum length of 51,200
* bytes. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*
*
* @return The structure that contains the template body, with a minimum length of 1 byte and a maximum length of
* 51,200 bytes. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*/
public String getTemplateBody() {
return this.templateBody;
}
/**
*
* The structure that contains the template body, with a minimum length of 1 byte and a maximum length of 51,200
* bytes. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*
*
* @param templateBody
* The structure that contains the template body, with a minimum length of 1 byte and a maximum length of
* 51,200 bytes. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackSetRequest withTemplateBody(String templateBody) {
setTemplateBody(templateBody);
return this;
}
/**
*
* The location of the file that contains the template body. The URL must point to a template (maximum size: 460,800
* bytes) that's located in an Amazon S3 bucket. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*
*
* @param templateURL
* The location of the file that contains the template body. The URL must point to a template (maximum size:
* 460,800 bytes) that's located in an Amazon S3 bucket. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*/
public void setTemplateURL(String templateURL) {
this.templateURL = templateURL;
}
/**
*
* The location of the file that contains the template body. The URL must point to a template (maximum size: 460,800
* bytes) that's located in an Amazon S3 bucket. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*
*
* @return The location of the file that contains the template body. The URL must point to a template (maximum size:
* 460,800 bytes) that's located in an Amazon S3 bucket. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*/
public String getTemplateURL() {
return this.templateURL;
}
/**
*
* The location of the file that contains the template body. The URL must point to a template (maximum size: 460,800
* bytes) that's located in an Amazon S3 bucket. For more information, see Template Anatomy
* in the AWS CloudFormation User Guide.
*
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
*
*
* @param templateURL
* The location of the file that contains the template body. The URL must point to a template (maximum size:
* 460,800 bytes) that's located in an Amazon S3 bucket. For more information, see Template
* Anatomy in the AWS CloudFormation User Guide.
*
* Conditional: You must specify either the TemplateBody or the TemplateURL parameter, but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackSetRequest withTemplateURL(String templateURL) {
setTemplateURL(templateURL);
return this;
}
/**
*
* The input parameters for the stack set template.
*
*
* @return The input parameters for the stack set template.
*/
public java.util.List getParameters() {
if (parameters == null) {
parameters = new com.amazonaws.internal.SdkInternalList();
}
return parameters;
}
/**
*
* The input parameters for the stack set template.
*
*
* @param parameters
* The input parameters for the stack set template.
*/
public void setParameters(java.util.Collection parameters) {
if (parameters == null) {
this.parameters = null;
return;
}
this.parameters = new com.amazonaws.internal.SdkInternalList(parameters);
}
/**
*
* The input parameters for the stack set template.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setParameters(java.util.Collection)} or {@link #withParameters(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param parameters
* The input parameters for the stack set template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackSetRequest withParameters(Parameter... parameters) {
if (this.parameters == null) {
setParameters(new com.amazonaws.internal.SdkInternalList(parameters.length));
}
for (Parameter ele : parameters) {
this.parameters.add(ele);
}
return this;
}
/**
*
* The input parameters for the stack set template.
*
*
* @param parameters
* The input parameters for the stack set template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackSetRequest withParameters(java.util.Collection parameters) {
setParameters(parameters);
return this;
}
/**
*
* In some cases, you must explicitly acknowledge that your stack set template contains certain capabilities in
* order for AWS CloudFormation to create the stack set and related stack instances.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to create a
* stack directly from the processed template, without first reviewing the resulting changes in a change set, you
* must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
*
*
* @return In some cases, you must explicitly acknowledge that your stack set template contains certain capabilities
* in order for AWS CloudFormation to create the stack set and related stack instances.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for
* example, by creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must
* explicitly acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to
* create a stack directly from the processed template, without first reviewing the resulting changes in a
* change set, you must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
* @see Capability
*/
public java.util.List getCapabilities() {
if (capabilities == null) {
capabilities = new com.amazonaws.internal.SdkInternalList();
}
return capabilities;
}
/**
*
* In some cases, you must explicitly acknowledge that your stack set template contains certain capabilities in
* order for AWS CloudFormation to create the stack set and related stack instances.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to create a
* stack directly from the processed template, without first reviewing the resulting changes in a change set, you
* must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack set template contains certain capabilities
* in order for AWS CloudFormation to create the stack set and related stack instances.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example,
* by creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to
* create a stack directly from the processed template, without first reviewing the resulting changes in a
* change set, you must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
* @see Capability
*/
public void setCapabilities(java.util.Collection capabilities) {
if (capabilities == null) {
this.capabilities = null;
return;
}
this.capabilities = new com.amazonaws.internal.SdkInternalList(capabilities);
}
/**
*
* In some cases, you must explicitly acknowledge that your stack set template contains certain capabilities in
* order for AWS CloudFormation to create the stack set and related stack instances.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to create a
* stack directly from the processed template, without first reviewing the resulting changes in a change set, you
* must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCapabilities(java.util.Collection)} or {@link #withCapabilities(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack set template contains certain capabilities
* in order for AWS CloudFormation to create the stack set and related stack instances.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example,
* by creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to
* create a stack directly from the processed template, without first reviewing the resulting changes in a
* change set, you must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Capability
*/
public CreateStackSetRequest withCapabilities(String... capabilities) {
if (this.capabilities == null) {
setCapabilities(new com.amazonaws.internal.SdkInternalList(capabilities.length));
}
for (String ele : capabilities) {
this.capabilities.add(ele);
}
return this;
}
/**
*
* In some cases, you must explicitly acknowledge that your stack set template contains certain capabilities in
* order for AWS CloudFormation to create the stack set and related stack instances.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to create a
* stack directly from the processed template, without first reviewing the resulting changes in a change set, you
* must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack set template contains certain capabilities
* in order for AWS CloudFormation to create the stack set and related stack instances.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example,
* by creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to
* create a stack directly from the processed template, without first reviewing the resulting changes in a
* change set, you must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Capability
*/
public CreateStackSetRequest withCapabilities(java.util.Collection capabilities) {
setCapabilities(capabilities);
return this;
}
/**
*
* In some cases, you must explicitly acknowledge that your stack set template contains certain capabilities in
* order for AWS CloudFormation to create the stack set and related stack instances.
*
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example, by
* creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated with
* them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to create a
* stack directly from the processed template, without first reviewing the resulting changes in a change set, you
* must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
*
*
* @param capabilities
* In some cases, you must explicitly acknowledge that your stack set template contains certain capabilities
* in order for AWS CloudFormation to create the stack set and related stack instances.
*
* -
*
* CAPABILITY_IAM
and CAPABILITY_NAMED_IAM
*
*
* Some stack templates might include resources that can affect permissions in your AWS account; for example,
* by creating new AWS Identity and Access Management (IAM) users. For those stack sets, you must explicitly
* acknowledge this by specifying one of these capabilities.
*
*
* The following IAM resources require you to specify either the CAPABILITY_IAM
or
* CAPABILITY_NAMED_IAM
capability.
*
*
* -
*
* If you have IAM resources, you can specify either capability.
*
*
* -
*
* If you have IAM resources with custom names, you must specify CAPABILITY_NAMED_IAM
.
*
*
* -
*
* If you don't specify either of these capabilities, AWS CloudFormation returns an
* InsufficientCapabilities
error.
*
*
*
*
* If your stack template contains these resources, we recommend that you review all permissions associated
* with them and edit their permissions if necessary.
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* -
*
*
* AWS::IAM::Role
*
*
* -
*
*
* AWS::IAM::User
*
*
* -
*
*
*
*
* For more information, see Acknowledging IAM Resources in AWS CloudFormation Templates.
*
*
* -
*
* CAPABILITY_AUTO_EXPAND
*
*
* Some templates contain macros. If your stack template contains one or more macros, and you choose to
* create a stack directly from the processed template, without first reviewing the resulting changes in a
* change set, you must acknowledge this capability. For more information, see Using AWS
* CloudFormation Macros to Perform Custom Processing on Templates.
*
*
*
* Stack sets do not currently support macros in stack templates. (This includes the AWS::Include and AWS::Serverless transforms, which are macros hosted by AWS CloudFormation.) Even if you specify this
* capability, if you include a macro in your template the stack set operation will fail.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see Capability
*/
public CreateStackSetRequest withCapabilities(Capability... capabilities) {
com.amazonaws.internal.SdkInternalList capabilitiesCopy = new com.amazonaws.internal.SdkInternalList(capabilities.length);
for (Capability value : capabilities) {
capabilitiesCopy.add(value.toString());
}
if (getCapabilities() == null) {
setCapabilities(capabilitiesCopy);
} else {
getCapabilities().addAll(capabilitiesCopy);
}
return this;
}
/**
*
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation also
* propagates these tags to supported resources that are created in the stacks. A maximum number of 50 tags can be
* specified.
*
*
* If you specify tags as part of a CreateStackSet
action, AWS CloudFormation checks to see if you have
* the required IAM permission to tag resources. If you don't, the entire CreateStackSet
action fails
* with an access denied
error, and the stack set is not created.
*
*
* @return The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation
* also propagates these tags to supported resources that are created in the stacks. A maximum number of 50
* tags can be specified.
*
* If you specify tags as part of a CreateStackSet
action, AWS CloudFormation checks to see if
* you have the required IAM permission to tag resources. If you don't, the entire
* CreateStackSet
action fails with an access denied
error, and the stack set is
* not created.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation also
* propagates these tags to supported resources that are created in the stacks. A maximum number of 50 tags can be
* specified.
*
*
* If you specify tags as part of a CreateStackSet
action, AWS CloudFormation checks to see if you have
* the required IAM permission to tag resources. If you don't, the entire CreateStackSet
action fails
* with an access denied
error, and the stack set is not created.
*
*
* @param tags
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation
* also propagates these tags to supported resources that are created in the stacks. A maximum number of 50
* tags can be specified.
*
* If you specify tags as part of a CreateStackSet
action, AWS CloudFormation checks to see if
* you have the required IAM permission to tag resources. If you don't, the entire
* CreateStackSet
action fails with an access denied
error, and the stack set is
* not created.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation also
* propagates these tags to supported resources that are created in the stacks. A maximum number of 50 tags can be
* specified.
*
*
* If you specify tags as part of a CreateStackSet
action, AWS CloudFormation checks to see if you have
* the required IAM permission to tag resources. If you don't, the entire CreateStackSet
action fails
* with an access denied
error, and the stack set is not created.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation
* also propagates these tags to supported resources that are created in the stacks. A maximum number of 50
* tags can be specified.
*
* If you specify tags as part of a CreateStackSet
action, AWS CloudFormation checks to see if
* you have the required IAM permission to tag resources. If you don't, the entire
* CreateStackSet
action fails with an access denied
error, and the stack set is
* not created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackSetRequest withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation also
* propagates these tags to supported resources that are created in the stacks. A maximum number of 50 tags can be
* specified.
*
*
* If you specify tags as part of a CreateStackSet
action, AWS CloudFormation checks to see if you have
* the required IAM permission to tag resources. If you don't, the entire CreateStackSet
action fails
* with an access denied
error, and the stack set is not created.
*
*
* @param tags
* The key-value pairs to associate with this stack set and the stacks created from it. AWS CloudFormation
* also propagates these tags to supported resources that are created in the stacks. A maximum number of 50
* tags can be specified.
*
* If you specify tags as part of a CreateStackSet
action, AWS CloudFormation checks to see if
* you have the required IAM permission to tag resources. If you don't, the entire
* CreateStackSet
action fails with an access denied
error, and the stack set is
* not created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackSetRequest withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The Amazon Resource Number (ARN) of the IAM role to use to create this stack set.
*
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups can
* manage specific stack sets within the same administrator account. For more information, see Prerequisites:
* Granting Permissions for Stack Set Operations in the AWS CloudFormation User Guide.
*
*
* @param administrationRoleARN
* The Amazon Resource Number (ARN) of the IAM role to use to create this stack set.
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups
* can manage specific stack sets within the same administrator account. For more information, see Prerequisites:
* Granting Permissions for Stack Set Operations in the AWS CloudFormation User Guide.
*/
public void setAdministrationRoleARN(String administrationRoleARN) {
this.administrationRoleARN = administrationRoleARN;
}
/**
*
* The Amazon Resource Number (ARN) of the IAM role to use to create this stack set.
*
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups can
* manage specific stack sets within the same administrator account. For more information, see Prerequisites:
* Granting Permissions for Stack Set Operations in the AWS CloudFormation User Guide.
*
*
* @return The Amazon Resource Number (ARN) of the IAM role to use to create this stack set.
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups
* can manage specific stack sets within the same administrator account. For more information, see Prerequisites: Granting Permissions for Stack Set Operations in the AWS CloudFormation User
* Guide.
*/
public String getAdministrationRoleARN() {
return this.administrationRoleARN;
}
/**
*
* The Amazon Resource Number (ARN) of the IAM role to use to create this stack set.
*
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups can
* manage specific stack sets within the same administrator account. For more information, see Prerequisites:
* Granting Permissions for Stack Set Operations in the AWS CloudFormation User Guide.
*
*
* @param administrationRoleARN
* The Amazon Resource Number (ARN) of the IAM role to use to create this stack set.
*
* Specify an IAM role only if you are using customized administrator roles to control which users or groups
* can manage specific stack sets within the same administrator account. For more information, see Prerequisites:
* Granting Permissions for Stack Set Operations in the AWS CloudFormation User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackSetRequest withAdministrationRoleARN(String administrationRoleARN) {
setAdministrationRoleARN(administrationRoleARN);
return this;
}
/**
*
* The name of the IAM execution role to use to create the stack set. If you do not specify an execution role, AWS
* CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack set operation.
*
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources users and
* groups can include in their stack sets.
*
*
* @param executionRoleName
* The name of the IAM execution role to use to create the stack set. If you do not specify an execution
* role, AWS CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack
* set operation.
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources
* users and groups can include in their stack sets.
*/
public void setExecutionRoleName(String executionRoleName) {
this.executionRoleName = executionRoleName;
}
/**
*
* The name of the IAM execution role to use to create the stack set. If you do not specify an execution role, AWS
* CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack set operation.
*
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources users and
* groups can include in their stack sets.
*
*
* @return The name of the IAM execution role to use to create the stack set. If you do not specify an execution
* role, AWS CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack
* set operation.
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources
* users and groups can include in their stack sets.
*/
public String getExecutionRoleName() {
return this.executionRoleName;
}
/**
*
* The name of the IAM execution role to use to create the stack set. If you do not specify an execution role, AWS
* CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack set operation.
*
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources users and
* groups can include in their stack sets.
*
*
* @param executionRoleName
* The name of the IAM execution role to use to create the stack set. If you do not specify an execution
* role, AWS CloudFormation uses the AWSCloudFormationStackSetExecutionRole
role for the stack
* set operation.
*
* Specify an IAM role only if you are using customized execution roles to control which stack resources
* users and groups can include in their stack sets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackSetRequest withExecutionRoleName(String executionRoleName) {
setExecutionRoleName(executionRoleName);
return this;
}
/**
*
* A unique identifier for this CreateStackSet
request. Specify this token if you plan to retry
* requests so that AWS CloudFormation knows that you're not attempting to create another stack set with the same
* name. You might retry CreateStackSet
requests to ensure that AWS CloudFormation successfully
* received them.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* @param clientRequestToken
* A unique identifier for this CreateStackSet
request. Specify this token if you plan to retry
* requests so that AWS CloudFormation knows that you're not attempting to create another stack set with the
* same name. You might retry CreateStackSet
requests to ensure that AWS CloudFormation
* successfully received them.
*
* If you don't specify an operation ID, the SDK generates one automatically.
*/
public void setClientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
}
/**
*
* A unique identifier for this CreateStackSet
request. Specify this token if you plan to retry
* requests so that AWS CloudFormation knows that you're not attempting to create another stack set with the same
* name. You might retry CreateStackSet
requests to ensure that AWS CloudFormation successfully
* received them.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* @return A unique identifier for this CreateStackSet
request. Specify this token if you plan to retry
* requests so that AWS CloudFormation knows that you're not attempting to create another stack set with the
* same name. You might retry CreateStackSet
requests to ensure that AWS CloudFormation
* successfully received them.
*
* If you don't specify an operation ID, the SDK generates one automatically.
*/
public String getClientRequestToken() {
return this.clientRequestToken;
}
/**
*
* A unique identifier for this CreateStackSet
request. Specify this token if you plan to retry
* requests so that AWS CloudFormation knows that you're not attempting to create another stack set with the same
* name. You might retry CreateStackSet
requests to ensure that AWS CloudFormation successfully
* received them.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* @param clientRequestToken
* A unique identifier for this CreateStackSet
request. Specify this token if you plan to retry
* requests so that AWS CloudFormation knows that you're not attempting to create another stack set with the
* same name. You might retry CreateStackSet
requests to ensure that AWS CloudFormation
* successfully received them.
*
* If you don't specify an operation ID, the SDK generates one automatically.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackSetRequest withClientRequestToken(String clientRequestToken) {
setClientRequestToken(clientRequestToken);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStackSetName() != null)
sb.append("StackSetName: ").append(getStackSetName()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getTemplateBody() != null)
sb.append("TemplateBody: ").append(getTemplateBody()).append(",");
if (getTemplateURL() != null)
sb.append("TemplateURL: ").append(getTemplateURL()).append(",");
if (getParameters() != null)
sb.append("Parameters: ").append(getParameters()).append(",");
if (getCapabilities() != null)
sb.append("Capabilities: ").append(getCapabilities()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getAdministrationRoleARN() != null)
sb.append("AdministrationRoleARN: ").append(getAdministrationRoleARN()).append(",");
if (getExecutionRoleName() != null)
sb.append("ExecutionRoleName: ").append(getExecutionRoleName()).append(",");
if (getClientRequestToken() != null)
sb.append("ClientRequestToken: ").append(getClientRequestToken());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateStackSetRequest == false)
return false;
CreateStackSetRequest other = (CreateStackSetRequest) obj;
if (other.getStackSetName() == null ^ this.getStackSetName() == null)
return false;
if (other.getStackSetName() != null && other.getStackSetName().equals(this.getStackSetName()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getTemplateBody() == null ^ this.getTemplateBody() == null)
return false;
if (other.getTemplateBody() != null && other.getTemplateBody().equals(this.getTemplateBody()) == false)
return false;
if (other.getTemplateURL() == null ^ this.getTemplateURL() == null)
return false;
if (other.getTemplateURL() != null && other.getTemplateURL().equals(this.getTemplateURL()) == false)
return false;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false)
return false;
if (other.getCapabilities() == null ^ this.getCapabilities() == null)
return false;
if (other.getCapabilities() != null && other.getCapabilities().equals(this.getCapabilities()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getAdministrationRoleARN() == null ^ this.getAdministrationRoleARN() == null)
return false;
if (other.getAdministrationRoleARN() != null && other.getAdministrationRoleARN().equals(this.getAdministrationRoleARN()) == false)
return false;
if (other.getExecutionRoleName() == null ^ this.getExecutionRoleName() == null)
return false;
if (other.getExecutionRoleName() != null && other.getExecutionRoleName().equals(this.getExecutionRoleName()) == false)
return false;
if (other.getClientRequestToken() == null ^ this.getClientRequestToken() == null)
return false;
if (other.getClientRequestToken() != null && other.getClientRequestToken().equals(this.getClientRequestToken()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStackSetName() == null) ? 0 : getStackSetName().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getTemplateBody() == null) ? 0 : getTemplateBody().hashCode());
hashCode = prime * hashCode + ((getTemplateURL() == null) ? 0 : getTemplateURL().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
hashCode = prime * hashCode + ((getCapabilities() == null) ? 0 : getCapabilities().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getAdministrationRoleARN() == null) ? 0 : getAdministrationRoleARN().hashCode());
hashCode = prime * hashCode + ((getExecutionRoleName() == null) ? 0 : getExecutionRoleName().hashCode());
hashCode = prime * hashCode + ((getClientRequestToken() == null) ? 0 : getClientRequestToken().hashCode());
return hashCode;
}
@Override
public CreateStackSetRequest clone() {
return (CreateStackSetRequest) super.clone();
}
}