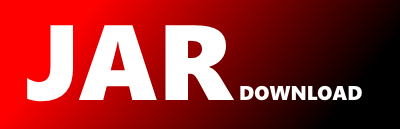
com.amazonaws.services.cloudformation.model.StackInstanceSummary Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudformation.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* The structure that contains summary information about a stack instance.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StackInstanceSummary implements Serializable, Cloneable {
/**
*
* The name or unique ID of the stack set that the stack instance is associated with.
*
*/
private String stackSetId;
/**
*
* The name of the Amazon Web Services Region that the stack instance is associated with.
*
*/
private String region;
/**
*
* [Self-managed permissions] The name of the Amazon Web Services account that the stack instance is associated
* with.
*
*/
private String account;
/**
*
* The ID of the stack instance.
*
*/
private String stackId;
/**
*
* The status of the stack instance, in terms of its synchronization with its associated stack set.
*
*
* -
*
* INOPERABLE
: A DeleteStackInstances
operation has failed and left the stack in an
* unstable state. Stacks in this state are excluded from further UpdateStackSet
operations. You might
* need to perform a DeleteStackInstances
operation, with RetainStacks
set to
* true
, to delete the stack instance, and then delete the stack manually.
*
*
* -
*
* OUTDATED
: The stack isn't currently up to date with the stack set because:
*
*
* -
*
* The associated stack failed during a CreateStackSet
or UpdateStackSet
operation.
*
*
* -
*
* The stack was part of a CreateStackSet
or UpdateStackSet
operation that failed or was
* stopped before the stack was created or updated.
*
*
*
*
* -
*
* CURRENT
: The stack is currently up to date with the stack set.
*
*
*
*/
private String status;
/**
*
* The explanation for the specific status code assigned to this stack instance.
*
*/
private String statusReason;
/**
*
* The detailed status of the stack instance.
*
*/
private StackInstanceComprehensiveStatus stackInstanceStatus;
/**
*
* [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified for
* DeploymentTargets.
*
*/
private String organizationalUnitId;
/**
*
* Status of the stack instance's actual configuration compared to the expected template and parameter configuration
* of the stack set to which it belongs.
*
*
* -
*
* DRIFTED
: The stack differs from the expected template and parameter configuration of the stack set
* to which it belongs. A stack instance is considered to have drifted if one or more of the resources in the
* associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation has not checked if the stack instance differs from its expected stack
* set configuration.
*
*
* -
*
* IN_SYNC
: The stack instance's actual configuration matches its expected stack set configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*/
private String driftStatus;
/**
*
* Most recent time when CloudFormation performed a drift detection operation on the stack instance. This value will
* be NULL
for any stack instance on which drift detection has not yet been performed.
*
*/
private java.util.Date lastDriftCheckTimestamp;
/**
*
* The name or unique ID of the stack set that the stack instance is associated with.
*
*
* @param stackSetId
* The name or unique ID of the stack set that the stack instance is associated with.
*/
public void setStackSetId(String stackSetId) {
this.stackSetId = stackSetId;
}
/**
*
* The name or unique ID of the stack set that the stack instance is associated with.
*
*
* @return The name or unique ID of the stack set that the stack instance is associated with.
*/
public String getStackSetId() {
return this.stackSetId;
}
/**
*
* The name or unique ID of the stack set that the stack instance is associated with.
*
*
* @param stackSetId
* The name or unique ID of the stack set that the stack instance is associated with.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackInstanceSummary withStackSetId(String stackSetId) {
setStackSetId(stackSetId);
return this;
}
/**
*
* The name of the Amazon Web Services Region that the stack instance is associated with.
*
*
* @param region
* The name of the Amazon Web Services Region that the stack instance is associated with.
*/
public void setRegion(String region) {
this.region = region;
}
/**
*
* The name of the Amazon Web Services Region that the stack instance is associated with.
*
*
* @return The name of the Amazon Web Services Region that the stack instance is associated with.
*/
public String getRegion() {
return this.region;
}
/**
*
* The name of the Amazon Web Services Region that the stack instance is associated with.
*
*
* @param region
* The name of the Amazon Web Services Region that the stack instance is associated with.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackInstanceSummary withRegion(String region) {
setRegion(region);
return this;
}
/**
*
* [Self-managed permissions] The name of the Amazon Web Services account that the stack instance is associated
* with.
*
*
* @param account
* [Self-managed permissions] The name of the Amazon Web Services account that the stack instance is
* associated with.
*/
public void setAccount(String account) {
this.account = account;
}
/**
*
* [Self-managed permissions] The name of the Amazon Web Services account that the stack instance is associated
* with.
*
*
* @return [Self-managed permissions] The name of the Amazon Web Services account that the stack instance is
* associated with.
*/
public String getAccount() {
return this.account;
}
/**
*
* [Self-managed permissions] The name of the Amazon Web Services account that the stack instance is associated
* with.
*
*
* @param account
* [Self-managed permissions] The name of the Amazon Web Services account that the stack instance is
* associated with.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackInstanceSummary withAccount(String account) {
setAccount(account);
return this;
}
/**
*
* The ID of the stack instance.
*
*
* @param stackId
* The ID of the stack instance.
*/
public void setStackId(String stackId) {
this.stackId = stackId;
}
/**
*
* The ID of the stack instance.
*
*
* @return The ID of the stack instance.
*/
public String getStackId() {
return this.stackId;
}
/**
*
* The ID of the stack instance.
*
*
* @param stackId
* The ID of the stack instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackInstanceSummary withStackId(String stackId) {
setStackId(stackId);
return this;
}
/**
*
* The status of the stack instance, in terms of its synchronization with its associated stack set.
*
*
* -
*
* INOPERABLE
: A DeleteStackInstances
operation has failed and left the stack in an
* unstable state. Stacks in this state are excluded from further UpdateStackSet
operations. You might
* need to perform a DeleteStackInstances
operation, with RetainStacks
set to
* true
, to delete the stack instance, and then delete the stack manually.
*
*
* -
*
* OUTDATED
: The stack isn't currently up to date with the stack set because:
*
*
* -
*
* The associated stack failed during a CreateStackSet
or UpdateStackSet
operation.
*
*
* -
*
* The stack was part of a CreateStackSet
or UpdateStackSet
operation that failed or was
* stopped before the stack was created or updated.
*
*
*
*
* -
*
* CURRENT
: The stack is currently up to date with the stack set.
*
*
*
*
* @param status
* The status of the stack instance, in terms of its synchronization with its associated stack set.
*
* -
*
* INOPERABLE
: A DeleteStackInstances
operation has failed and left the stack in an
* unstable state. Stacks in this state are excluded from further UpdateStackSet
operations. You
* might need to perform a DeleteStackInstances
operation, with RetainStacks
set to
* true
, to delete the stack instance, and then delete the stack manually.
*
*
* -
*
* OUTDATED
: The stack isn't currently up to date with the stack set because:
*
*
* -
*
* The associated stack failed during a CreateStackSet
or UpdateStackSet
operation.
*
*
* -
*
* The stack was part of a CreateStackSet
or UpdateStackSet
operation that failed
* or was stopped before the stack was created or updated.
*
*
*
*
* -
*
* CURRENT
: The stack is currently up to date with the stack set.
*
*
* @see StackInstanceStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the stack instance, in terms of its synchronization with its associated stack set.
*
*
* -
*
* INOPERABLE
: A DeleteStackInstances
operation has failed and left the stack in an
* unstable state. Stacks in this state are excluded from further UpdateStackSet
operations. You might
* need to perform a DeleteStackInstances
operation, with RetainStacks
set to
* true
, to delete the stack instance, and then delete the stack manually.
*
*
* -
*
* OUTDATED
: The stack isn't currently up to date with the stack set because:
*
*
* -
*
* The associated stack failed during a CreateStackSet
or UpdateStackSet
operation.
*
*
* -
*
* The stack was part of a CreateStackSet
or UpdateStackSet
operation that failed or was
* stopped before the stack was created or updated.
*
*
*
*
* -
*
* CURRENT
: The stack is currently up to date with the stack set.
*
*
*
*
* @return The status of the stack instance, in terms of its synchronization with its associated stack set.
*
* -
*
* INOPERABLE
: A DeleteStackInstances
operation has failed and left the stack in
* an unstable state. Stacks in this state are excluded from further UpdateStackSet
operations.
* You might need to perform a DeleteStackInstances
operation, with RetainStacks
* set to true
, to delete the stack instance, and then delete the stack manually.
*
*
* -
*
* OUTDATED
: The stack isn't currently up to date with the stack set because:
*
*
* -
*
* The associated stack failed during a CreateStackSet
or UpdateStackSet
* operation.
*
*
* -
*
* The stack was part of a CreateStackSet
or UpdateStackSet
operation that failed
* or was stopped before the stack was created or updated.
*
*
*
*
* -
*
* CURRENT
: The stack is currently up to date with the stack set.
*
*
* @see StackInstanceStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the stack instance, in terms of its synchronization with its associated stack set.
*
*
* -
*
* INOPERABLE
: A DeleteStackInstances
operation has failed and left the stack in an
* unstable state. Stacks in this state are excluded from further UpdateStackSet
operations. You might
* need to perform a DeleteStackInstances
operation, with RetainStacks
set to
* true
, to delete the stack instance, and then delete the stack manually.
*
*
* -
*
* OUTDATED
: The stack isn't currently up to date with the stack set because:
*
*
* -
*
* The associated stack failed during a CreateStackSet
or UpdateStackSet
operation.
*
*
* -
*
* The stack was part of a CreateStackSet
or UpdateStackSet
operation that failed or was
* stopped before the stack was created or updated.
*
*
*
*
* -
*
* CURRENT
: The stack is currently up to date with the stack set.
*
*
*
*
* @param status
* The status of the stack instance, in terms of its synchronization with its associated stack set.
*
* -
*
* INOPERABLE
: A DeleteStackInstances
operation has failed and left the stack in an
* unstable state. Stacks in this state are excluded from further UpdateStackSet
operations. You
* might need to perform a DeleteStackInstances
operation, with RetainStacks
set to
* true
, to delete the stack instance, and then delete the stack manually.
*
*
* -
*
* OUTDATED
: The stack isn't currently up to date with the stack set because:
*
*
* -
*
* The associated stack failed during a CreateStackSet
or UpdateStackSet
operation.
*
*
* -
*
* The stack was part of a CreateStackSet
or UpdateStackSet
operation that failed
* or was stopped before the stack was created or updated.
*
*
*
*
* -
*
* CURRENT
: The stack is currently up to date with the stack set.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackInstanceStatus
*/
public StackInstanceSummary withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the stack instance, in terms of its synchronization with its associated stack set.
*
*
* -
*
* INOPERABLE
: A DeleteStackInstances
operation has failed and left the stack in an
* unstable state. Stacks in this state are excluded from further UpdateStackSet
operations. You might
* need to perform a DeleteStackInstances
operation, with RetainStacks
set to
* true
, to delete the stack instance, and then delete the stack manually.
*
*
* -
*
* OUTDATED
: The stack isn't currently up to date with the stack set because:
*
*
* -
*
* The associated stack failed during a CreateStackSet
or UpdateStackSet
operation.
*
*
* -
*
* The stack was part of a CreateStackSet
or UpdateStackSet
operation that failed or was
* stopped before the stack was created or updated.
*
*
*
*
* -
*
* CURRENT
: The stack is currently up to date with the stack set.
*
*
*
*
* @param status
* The status of the stack instance, in terms of its synchronization with its associated stack set.
*
* -
*
* INOPERABLE
: A DeleteStackInstances
operation has failed and left the stack in an
* unstable state. Stacks in this state are excluded from further UpdateStackSet
operations. You
* might need to perform a DeleteStackInstances
operation, with RetainStacks
set to
* true
, to delete the stack instance, and then delete the stack manually.
*
*
* -
*
* OUTDATED
: The stack isn't currently up to date with the stack set because:
*
*
* -
*
* The associated stack failed during a CreateStackSet
or UpdateStackSet
operation.
*
*
* -
*
* The stack was part of a CreateStackSet
or UpdateStackSet
operation that failed
* or was stopped before the stack was created or updated.
*
*
*
*
* -
*
* CURRENT
: The stack is currently up to date with the stack set.
*
*
* @see StackInstanceStatus
*/
public void setStatus(StackInstanceStatus status) {
withStatus(status);
}
/**
*
* The status of the stack instance, in terms of its synchronization with its associated stack set.
*
*
* -
*
* INOPERABLE
: A DeleteStackInstances
operation has failed and left the stack in an
* unstable state. Stacks in this state are excluded from further UpdateStackSet
operations. You might
* need to perform a DeleteStackInstances
operation, with RetainStacks
set to
* true
, to delete the stack instance, and then delete the stack manually.
*
*
* -
*
* OUTDATED
: The stack isn't currently up to date with the stack set because:
*
*
* -
*
* The associated stack failed during a CreateStackSet
or UpdateStackSet
operation.
*
*
* -
*
* The stack was part of a CreateStackSet
or UpdateStackSet
operation that failed or was
* stopped before the stack was created or updated.
*
*
*
*
* -
*
* CURRENT
: The stack is currently up to date with the stack set.
*
*
*
*
* @param status
* The status of the stack instance, in terms of its synchronization with its associated stack set.
*
* -
*
* INOPERABLE
: A DeleteStackInstances
operation has failed and left the stack in an
* unstable state. Stacks in this state are excluded from further UpdateStackSet
operations. You
* might need to perform a DeleteStackInstances
operation, with RetainStacks
set to
* true
, to delete the stack instance, and then delete the stack manually.
*
*
* -
*
* OUTDATED
: The stack isn't currently up to date with the stack set because:
*
*
* -
*
* The associated stack failed during a CreateStackSet
or UpdateStackSet
operation.
*
*
* -
*
* The stack was part of a CreateStackSet
or UpdateStackSet
operation that failed
* or was stopped before the stack was created or updated.
*
*
*
*
* -
*
* CURRENT
: The stack is currently up to date with the stack set.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackInstanceStatus
*/
public StackInstanceSummary withStatus(StackInstanceStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The explanation for the specific status code assigned to this stack instance.
*
*
* @param statusReason
* The explanation for the specific status code assigned to this stack instance.
*/
public void setStatusReason(String statusReason) {
this.statusReason = statusReason;
}
/**
*
* The explanation for the specific status code assigned to this stack instance.
*
*
* @return The explanation for the specific status code assigned to this stack instance.
*/
public String getStatusReason() {
return this.statusReason;
}
/**
*
* The explanation for the specific status code assigned to this stack instance.
*
*
* @param statusReason
* The explanation for the specific status code assigned to this stack instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackInstanceSummary withStatusReason(String statusReason) {
setStatusReason(statusReason);
return this;
}
/**
*
* The detailed status of the stack instance.
*
*
* @param stackInstanceStatus
* The detailed status of the stack instance.
*/
public void setStackInstanceStatus(StackInstanceComprehensiveStatus stackInstanceStatus) {
this.stackInstanceStatus = stackInstanceStatus;
}
/**
*
* The detailed status of the stack instance.
*
*
* @return The detailed status of the stack instance.
*/
public StackInstanceComprehensiveStatus getStackInstanceStatus() {
return this.stackInstanceStatus;
}
/**
*
* The detailed status of the stack instance.
*
*
* @param stackInstanceStatus
* The detailed status of the stack instance.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackInstanceSummary withStackInstanceStatus(StackInstanceComprehensiveStatus stackInstanceStatus) {
setStackInstanceStatus(stackInstanceStatus);
return this;
}
/**
*
* [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified for
* DeploymentTargets.
*
*
* @param organizationalUnitId
* [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified
* for
* DeploymentTargets.
*/
public void setOrganizationalUnitId(String organizationalUnitId) {
this.organizationalUnitId = organizationalUnitId;
}
/**
*
* [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified for
* DeploymentTargets.
*
*
* @return [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified
* for DeploymentTargets.
*/
public String getOrganizationalUnitId() {
return this.organizationalUnitId;
}
/**
*
* [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified for
* DeploymentTargets.
*
*
* @param organizationalUnitId
* [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified
* for
* DeploymentTargets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackInstanceSummary withOrganizationalUnitId(String organizationalUnitId) {
setOrganizationalUnitId(organizationalUnitId);
return this;
}
/**
*
* Status of the stack instance's actual configuration compared to the expected template and parameter configuration
* of the stack set to which it belongs.
*
*
* -
*
* DRIFTED
: The stack differs from the expected template and parameter configuration of the stack set
* to which it belongs. A stack instance is considered to have drifted if one or more of the resources in the
* associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation has not checked if the stack instance differs from its expected stack
* set configuration.
*
*
* -
*
* IN_SYNC
: The stack instance's actual configuration matches its expected stack set configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*
* @param driftStatus
* Status of the stack instance's actual configuration compared to the expected template and parameter
* configuration of the stack set to which it belongs.
*
* -
*
* DRIFTED
: The stack differs from the expected template and parameter configuration of the
* stack set to which it belongs. A stack instance is considered to have drifted if one or more of the
* resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation has not checked if the stack instance differs from its expected
* stack set configuration.
*
*
* -
*
* IN_SYNC
: The stack instance's actual configuration matches its expected stack set
* configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
* @see StackDriftStatus
*/
public void setDriftStatus(String driftStatus) {
this.driftStatus = driftStatus;
}
/**
*
* Status of the stack instance's actual configuration compared to the expected template and parameter configuration
* of the stack set to which it belongs.
*
*
* -
*
* DRIFTED
: The stack differs from the expected template and parameter configuration of the stack set
* to which it belongs. A stack instance is considered to have drifted if one or more of the resources in the
* associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation has not checked if the stack instance differs from its expected stack
* set configuration.
*
*
* -
*
* IN_SYNC
: The stack instance's actual configuration matches its expected stack set configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*
* @return Status of the stack instance's actual configuration compared to the expected template and parameter
* configuration of the stack set to which it belongs.
*
* -
*
* DRIFTED
: The stack differs from the expected template and parameter configuration of the
* stack set to which it belongs. A stack instance is considered to have drifted if one or more of the
* resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation has not checked if the stack instance differs from its expected
* stack set configuration.
*
*
* -
*
* IN_SYNC
: The stack instance's actual configuration matches its expected stack set
* configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
* @see StackDriftStatus
*/
public String getDriftStatus() {
return this.driftStatus;
}
/**
*
* Status of the stack instance's actual configuration compared to the expected template and parameter configuration
* of the stack set to which it belongs.
*
*
* -
*
* DRIFTED
: The stack differs from the expected template and parameter configuration of the stack set
* to which it belongs. A stack instance is considered to have drifted if one or more of the resources in the
* associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation has not checked if the stack instance differs from its expected stack
* set configuration.
*
*
* -
*
* IN_SYNC
: The stack instance's actual configuration matches its expected stack set configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*
* @param driftStatus
* Status of the stack instance's actual configuration compared to the expected template and parameter
* configuration of the stack set to which it belongs.
*
* -
*
* DRIFTED
: The stack differs from the expected template and parameter configuration of the
* stack set to which it belongs. A stack instance is considered to have drifted if one or more of the
* resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation has not checked if the stack instance differs from its expected
* stack set configuration.
*
*
* -
*
* IN_SYNC
: The stack instance's actual configuration matches its expected stack set
* configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackDriftStatus
*/
public StackInstanceSummary withDriftStatus(String driftStatus) {
setDriftStatus(driftStatus);
return this;
}
/**
*
* Status of the stack instance's actual configuration compared to the expected template and parameter configuration
* of the stack set to which it belongs.
*
*
* -
*
* DRIFTED
: The stack differs from the expected template and parameter configuration of the stack set
* to which it belongs. A stack instance is considered to have drifted if one or more of the resources in the
* associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation has not checked if the stack instance differs from its expected stack
* set configuration.
*
*
* -
*
* IN_SYNC
: The stack instance's actual configuration matches its expected stack set configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*
* @param driftStatus
* Status of the stack instance's actual configuration compared to the expected template and parameter
* configuration of the stack set to which it belongs.
*
* -
*
* DRIFTED
: The stack differs from the expected template and parameter configuration of the
* stack set to which it belongs. A stack instance is considered to have drifted if one or more of the
* resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation has not checked if the stack instance differs from its expected
* stack set configuration.
*
*
* -
*
* IN_SYNC
: The stack instance's actual configuration matches its expected stack set
* configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
* @see StackDriftStatus
*/
public void setDriftStatus(StackDriftStatus driftStatus) {
withDriftStatus(driftStatus);
}
/**
*
* Status of the stack instance's actual configuration compared to the expected template and parameter configuration
* of the stack set to which it belongs.
*
*
* -
*
* DRIFTED
: The stack differs from the expected template and parameter configuration of the stack set
* to which it belongs. A stack instance is considered to have drifted if one or more of the resources in the
* associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation has not checked if the stack instance differs from its expected stack
* set configuration.
*
*
* -
*
* IN_SYNC
: The stack instance's actual configuration matches its expected stack set configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*
* @param driftStatus
* Status of the stack instance's actual configuration compared to the expected template and parameter
* configuration of the stack set to which it belongs.
*
* -
*
* DRIFTED
: The stack differs from the expected template and parameter configuration of the
* stack set to which it belongs. A stack instance is considered to have drifted if one or more of the
* resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation has not checked if the stack instance differs from its expected
* stack set configuration.
*
*
* -
*
* IN_SYNC
: The stack instance's actual configuration matches its expected stack set
* configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackDriftStatus
*/
public StackInstanceSummary withDriftStatus(StackDriftStatus driftStatus) {
this.driftStatus = driftStatus.toString();
return this;
}
/**
*
* Most recent time when CloudFormation performed a drift detection operation on the stack instance. This value will
* be NULL
for any stack instance on which drift detection has not yet been performed.
*
*
* @param lastDriftCheckTimestamp
* Most recent time when CloudFormation performed a drift detection operation on the stack instance. This
* value will be NULL
for any stack instance on which drift detection has not yet been
* performed.
*/
public void setLastDriftCheckTimestamp(java.util.Date lastDriftCheckTimestamp) {
this.lastDriftCheckTimestamp = lastDriftCheckTimestamp;
}
/**
*
* Most recent time when CloudFormation performed a drift detection operation on the stack instance. This value will
* be NULL
for any stack instance on which drift detection has not yet been performed.
*
*
* @return Most recent time when CloudFormation performed a drift detection operation on the stack instance. This
* value will be NULL
for any stack instance on which drift detection has not yet been
* performed.
*/
public java.util.Date getLastDriftCheckTimestamp() {
return this.lastDriftCheckTimestamp;
}
/**
*
* Most recent time when CloudFormation performed a drift detection operation on the stack instance. This value will
* be NULL
for any stack instance on which drift detection has not yet been performed.
*
*
* @param lastDriftCheckTimestamp
* Most recent time when CloudFormation performed a drift detection operation on the stack instance. This
* value will be NULL
for any stack instance on which drift detection has not yet been
* performed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackInstanceSummary withLastDriftCheckTimestamp(java.util.Date lastDriftCheckTimestamp) {
setLastDriftCheckTimestamp(lastDriftCheckTimestamp);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStackSetId() != null)
sb.append("StackSetId: ").append(getStackSetId()).append(",");
if (getRegion() != null)
sb.append("Region: ").append(getRegion()).append(",");
if (getAccount() != null)
sb.append("Account: ").append(getAccount()).append(",");
if (getStackId() != null)
sb.append("StackId: ").append(getStackId()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getStatusReason() != null)
sb.append("StatusReason: ").append(getStatusReason()).append(",");
if (getStackInstanceStatus() != null)
sb.append("StackInstanceStatus: ").append(getStackInstanceStatus()).append(",");
if (getOrganizationalUnitId() != null)
sb.append("OrganizationalUnitId: ").append(getOrganizationalUnitId()).append(",");
if (getDriftStatus() != null)
sb.append("DriftStatus: ").append(getDriftStatus()).append(",");
if (getLastDriftCheckTimestamp() != null)
sb.append("LastDriftCheckTimestamp: ").append(getLastDriftCheckTimestamp());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StackInstanceSummary == false)
return false;
StackInstanceSummary other = (StackInstanceSummary) obj;
if (other.getStackSetId() == null ^ this.getStackSetId() == null)
return false;
if (other.getStackSetId() != null && other.getStackSetId().equals(this.getStackSetId()) == false)
return false;
if (other.getRegion() == null ^ this.getRegion() == null)
return false;
if (other.getRegion() != null && other.getRegion().equals(this.getRegion()) == false)
return false;
if (other.getAccount() == null ^ this.getAccount() == null)
return false;
if (other.getAccount() != null && other.getAccount().equals(this.getAccount()) == false)
return false;
if (other.getStackId() == null ^ this.getStackId() == null)
return false;
if (other.getStackId() != null && other.getStackId().equals(this.getStackId()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getStatusReason() == null ^ this.getStatusReason() == null)
return false;
if (other.getStatusReason() != null && other.getStatusReason().equals(this.getStatusReason()) == false)
return false;
if (other.getStackInstanceStatus() == null ^ this.getStackInstanceStatus() == null)
return false;
if (other.getStackInstanceStatus() != null && other.getStackInstanceStatus().equals(this.getStackInstanceStatus()) == false)
return false;
if (other.getOrganizationalUnitId() == null ^ this.getOrganizationalUnitId() == null)
return false;
if (other.getOrganizationalUnitId() != null && other.getOrganizationalUnitId().equals(this.getOrganizationalUnitId()) == false)
return false;
if (other.getDriftStatus() == null ^ this.getDriftStatus() == null)
return false;
if (other.getDriftStatus() != null && other.getDriftStatus().equals(this.getDriftStatus()) == false)
return false;
if (other.getLastDriftCheckTimestamp() == null ^ this.getLastDriftCheckTimestamp() == null)
return false;
if (other.getLastDriftCheckTimestamp() != null && other.getLastDriftCheckTimestamp().equals(this.getLastDriftCheckTimestamp()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStackSetId() == null) ? 0 : getStackSetId().hashCode());
hashCode = prime * hashCode + ((getRegion() == null) ? 0 : getRegion().hashCode());
hashCode = prime * hashCode + ((getAccount() == null) ? 0 : getAccount().hashCode());
hashCode = prime * hashCode + ((getStackId() == null) ? 0 : getStackId().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getStatusReason() == null) ? 0 : getStatusReason().hashCode());
hashCode = prime * hashCode + ((getStackInstanceStatus() == null) ? 0 : getStackInstanceStatus().hashCode());
hashCode = prime * hashCode + ((getOrganizationalUnitId() == null) ? 0 : getOrganizationalUnitId().hashCode());
hashCode = prime * hashCode + ((getDriftStatus() == null) ? 0 : getDriftStatus().hashCode());
hashCode = prime * hashCode + ((getLastDriftCheckTimestamp() == null) ? 0 : getLastDriftCheckTimestamp().hashCode());
return hashCode;
}
@Override
public StackInstanceSummary clone() {
try {
return (StackInstanceSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}