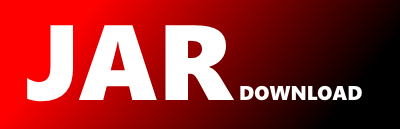
com.amazonaws.services.cloudformation.model.CreateStackInstancesRequest Maven / Gradle / Ivy
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudformation.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class CreateStackInstancesRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name or unique ID of the stack set that you want to create stack instances from.
*
*/
private String stackSetName;
/**
*
* [Self-managed permissions] The names of one or more Amazon Web Services accounts that you want to create stack
* instances in the specified Region(s) for.
*
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*
*/
private com.amazonaws.internal.SdkInternalList accounts;
/**
*
* [Service-managed permissions] The Organizations accounts for which to create stack instances in the specified
* Amazon Web Services Regions.
*
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*
*/
private DeploymentTargets deploymentTargets;
/**
*
* The names of one or more Amazon Web Services Regions where you want to create stack instances using the specified
* Amazon Web Services accounts.
*
*/
private com.amazonaws.internal.SdkInternalList regions;
/**
*
* A list of stack set parameters whose values you want to override in the selected stack instances.
*
*
* Any overridden parameter values will be applied to all stack instances in the specified accounts and Amazon Web
* Services Regions. When specifying parameters and their values, be aware of how CloudFormation sets parameter
* values during stack instance operations:
*
*
* -
*
* To override the current value for a parameter, include the parameter and specify its value.
*
*
* -
*
* To leave an overridden parameter set to its present value, include the parameter and specify
* UsePreviousValue
as true
. (You can't specify both a value and set
* UsePreviousValue
to true
.)
*
*
* -
*
* To set an overridden parameter back to the value specified in the stack set, specify a parameter list but don't
* include the parameter in the list.
*
*
* -
*
* To leave all parameters set to their present values, don't specify this property at all.
*
*
*
*
* During stack set updates, any parameter values overridden for a stack instance aren't updated, but retain their
* overridden value.
*
*
* You can only override the parameter values that are specified in the stack set; to add or delete a
* parameter itself, use UpdateStackSet to update the stack set template.
*
*/
private com.amazonaws.internal.SdkInternalList parameterOverrides;
/**
*
* Preferences for how CloudFormation performs this stack set operation.
*
*/
private StackSetOperationPreferences operationPreferences;
/**
*
* The unique identifier for this stack set operation.
*
*
* The operation ID also functions as an idempotency token, to ensure that CloudFormation performs the stack set
* operation only once, even if you retry the request multiple times. You might retry stack set operation requests
* to ensure that CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*
*/
private String operationId;
/**
*
* [Service-managed permissions] Specifies whether you are acting as an account administrator in the organization's
* management account or as a delegated administrator in a member account.
*
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed permissions.
*
*
* -
*
* If you are signed in to the management account, specify SELF
.
*
*
* -
*
* If you are signed in to a delegated administrator account, specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated administrator in the management account. For
* more information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
*
*/
private String callAs;
/**
*
* The name or unique ID of the stack set that you want to create stack instances from.
*
*
* @param stackSetName
* The name or unique ID of the stack set that you want to create stack instances from.
*/
public void setStackSetName(String stackSetName) {
this.stackSetName = stackSetName;
}
/**
*
* The name or unique ID of the stack set that you want to create stack instances from.
*
*
* @return The name or unique ID of the stack set that you want to create stack instances from.
*/
public String getStackSetName() {
return this.stackSetName;
}
/**
*
* The name or unique ID of the stack set that you want to create stack instances from.
*
*
* @param stackSetName
* The name or unique ID of the stack set that you want to create stack instances from.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackInstancesRequest withStackSetName(String stackSetName) {
setStackSetName(stackSetName);
return this;
}
/**
*
* [Self-managed permissions] The names of one or more Amazon Web Services accounts that you want to create stack
* instances in the specified Region(s) for.
*
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*
*
* @return [Self-managed permissions] The names of one or more Amazon Web Services accounts that you want to create
* stack instances in the specified Region(s) for.
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*/
public java.util.List getAccounts() {
if (accounts == null) {
accounts = new com.amazonaws.internal.SdkInternalList();
}
return accounts;
}
/**
*
* [Self-managed permissions] The names of one or more Amazon Web Services accounts that you want to create stack
* instances in the specified Region(s) for.
*
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*
*
* @param accounts
* [Self-managed permissions] The names of one or more Amazon Web Services accounts that you want to create
* stack instances in the specified Region(s) for.
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*/
public void setAccounts(java.util.Collection accounts) {
if (accounts == null) {
this.accounts = null;
return;
}
this.accounts = new com.amazonaws.internal.SdkInternalList(accounts);
}
/**
*
* [Self-managed permissions] The names of one or more Amazon Web Services accounts that you want to create stack
* instances in the specified Region(s) for.
*
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setAccounts(java.util.Collection)} or {@link #withAccounts(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param accounts
* [Self-managed permissions] The names of one or more Amazon Web Services accounts that you want to create
* stack instances in the specified Region(s) for.
*
* You can specify Accounts
or DeploymentTargets
, but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackInstancesRequest withAccounts(String... accounts) {
if (this.accounts == null) {
setAccounts(new com.amazonaws.internal.SdkInternalList(accounts.length));
}
for (String ele : accounts) {
this.accounts.add(ele);
}
return this;
}
/**
*
* [Self-managed permissions] The names of one or more Amazon Web Services accounts that you want to create stack
* instances in the specified Region(s) for.
*
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*
*
* @param accounts
* [Self-managed permissions] The names of one or more Amazon Web Services accounts that you want to create
* stack instances in the specified Region(s) for.
*
* You can specify Accounts
or DeploymentTargets
, but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackInstancesRequest withAccounts(java.util.Collection accounts) {
setAccounts(accounts);
return this;
}
/**
*
* [Service-managed permissions] The Organizations accounts for which to create stack instances in the specified
* Amazon Web Services Regions.
*
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*
*
* @param deploymentTargets
* [Service-managed permissions] The Organizations accounts for which to create stack instances in the
* specified Amazon Web Services Regions.
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*/
public void setDeploymentTargets(DeploymentTargets deploymentTargets) {
this.deploymentTargets = deploymentTargets;
}
/**
*
* [Service-managed permissions] The Organizations accounts for which to create stack instances in the specified
* Amazon Web Services Regions.
*
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*
*
* @return [Service-managed permissions] The Organizations accounts for which to create stack instances in the
* specified Amazon Web Services Regions.
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*/
public DeploymentTargets getDeploymentTargets() {
return this.deploymentTargets;
}
/**
*
* [Service-managed permissions] The Organizations accounts for which to create stack instances in the specified
* Amazon Web Services Regions.
*
*
* You can specify Accounts
or DeploymentTargets
, but not both.
*
*
* @param deploymentTargets
* [Service-managed permissions] The Organizations accounts for which to create stack instances in the
* specified Amazon Web Services Regions.
*
* You can specify Accounts
or DeploymentTargets
, but not both.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackInstancesRequest withDeploymentTargets(DeploymentTargets deploymentTargets) {
setDeploymentTargets(deploymentTargets);
return this;
}
/**
*
* The names of one or more Amazon Web Services Regions where you want to create stack instances using the specified
* Amazon Web Services accounts.
*
*
* @return The names of one or more Amazon Web Services Regions where you want to create stack instances using the
* specified Amazon Web Services accounts.
*/
public java.util.List getRegions() {
if (regions == null) {
regions = new com.amazonaws.internal.SdkInternalList();
}
return regions;
}
/**
*
* The names of one or more Amazon Web Services Regions where you want to create stack instances using the specified
* Amazon Web Services accounts.
*
*
* @param regions
* The names of one or more Amazon Web Services Regions where you want to create stack instances using the
* specified Amazon Web Services accounts.
*/
public void setRegions(java.util.Collection regions) {
if (regions == null) {
this.regions = null;
return;
}
this.regions = new com.amazonaws.internal.SdkInternalList(regions);
}
/**
*
* The names of one or more Amazon Web Services Regions where you want to create stack instances using the specified
* Amazon Web Services accounts.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRegions(java.util.Collection)} or {@link #withRegions(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param regions
* The names of one or more Amazon Web Services Regions where you want to create stack instances using the
* specified Amazon Web Services accounts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackInstancesRequest withRegions(String... regions) {
if (this.regions == null) {
setRegions(new com.amazonaws.internal.SdkInternalList(regions.length));
}
for (String ele : regions) {
this.regions.add(ele);
}
return this;
}
/**
*
* The names of one or more Amazon Web Services Regions where you want to create stack instances using the specified
* Amazon Web Services accounts.
*
*
* @param regions
* The names of one or more Amazon Web Services Regions where you want to create stack instances using the
* specified Amazon Web Services accounts.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackInstancesRequest withRegions(java.util.Collection regions) {
setRegions(regions);
return this;
}
/**
*
* A list of stack set parameters whose values you want to override in the selected stack instances.
*
*
* Any overridden parameter values will be applied to all stack instances in the specified accounts and Amazon Web
* Services Regions. When specifying parameters and their values, be aware of how CloudFormation sets parameter
* values during stack instance operations:
*
*
* -
*
* To override the current value for a parameter, include the parameter and specify its value.
*
*
* -
*
* To leave an overridden parameter set to its present value, include the parameter and specify
* UsePreviousValue
as true
. (You can't specify both a value and set
* UsePreviousValue
to true
.)
*
*
* -
*
* To set an overridden parameter back to the value specified in the stack set, specify a parameter list but don't
* include the parameter in the list.
*
*
* -
*
* To leave all parameters set to their present values, don't specify this property at all.
*
*
*
*
* During stack set updates, any parameter values overridden for a stack instance aren't updated, but retain their
* overridden value.
*
*
* You can only override the parameter values that are specified in the stack set; to add or delete a
* parameter itself, use UpdateStackSet to update the stack set template.
*
*
* @return A list of stack set parameters whose values you want to override in the selected stack instances.
*
* Any overridden parameter values will be applied to all stack instances in the specified accounts and
* Amazon Web Services Regions. When specifying parameters and their values, be aware of how CloudFormation
* sets parameter values during stack instance operations:
*
*
* -
*
* To override the current value for a parameter, include the parameter and specify its value.
*
*
* -
*
* To leave an overridden parameter set to its present value, include the parameter and specify
* UsePreviousValue
as true
. (You can't specify both a value and set
* UsePreviousValue
to true
.)
*
*
* -
*
* To set an overridden parameter back to the value specified in the stack set, specify a parameter list but
* don't include the parameter in the list.
*
*
* -
*
* To leave all parameters set to their present values, don't specify this property at all.
*
*
*
*
* During stack set updates, any parameter values overridden for a stack instance aren't updated, but retain
* their overridden value.
*
*
* You can only override the parameter values that are specified in the stack set; to add or delete a
* parameter itself, use UpdateStackSet to update the stack set template.
*/
public java.util.List getParameterOverrides() {
if (parameterOverrides == null) {
parameterOverrides = new com.amazonaws.internal.SdkInternalList();
}
return parameterOverrides;
}
/**
*
* A list of stack set parameters whose values you want to override in the selected stack instances.
*
*
* Any overridden parameter values will be applied to all stack instances in the specified accounts and Amazon Web
* Services Regions. When specifying parameters and their values, be aware of how CloudFormation sets parameter
* values during stack instance operations:
*
*
* -
*
* To override the current value for a parameter, include the parameter and specify its value.
*
*
* -
*
* To leave an overridden parameter set to its present value, include the parameter and specify
* UsePreviousValue
as true
. (You can't specify both a value and set
* UsePreviousValue
to true
.)
*
*
* -
*
* To set an overridden parameter back to the value specified in the stack set, specify a parameter list but don't
* include the parameter in the list.
*
*
* -
*
* To leave all parameters set to their present values, don't specify this property at all.
*
*
*
*
* During stack set updates, any parameter values overridden for a stack instance aren't updated, but retain their
* overridden value.
*
*
* You can only override the parameter values that are specified in the stack set; to add or delete a
* parameter itself, use UpdateStackSet to update the stack set template.
*
*
* @param parameterOverrides
* A list of stack set parameters whose values you want to override in the selected stack instances.
*
* Any overridden parameter values will be applied to all stack instances in the specified accounts and
* Amazon Web Services Regions. When specifying parameters and their values, be aware of how CloudFormation
* sets parameter values during stack instance operations:
*
*
* -
*
* To override the current value for a parameter, include the parameter and specify its value.
*
*
* -
*
* To leave an overridden parameter set to its present value, include the parameter and specify
* UsePreviousValue
as true
. (You can't specify both a value and set
* UsePreviousValue
to true
.)
*
*
* -
*
* To set an overridden parameter back to the value specified in the stack set, specify a parameter list but
* don't include the parameter in the list.
*
*
* -
*
* To leave all parameters set to their present values, don't specify this property at all.
*
*
*
*
* During stack set updates, any parameter values overridden for a stack instance aren't updated, but retain
* their overridden value.
*
*
* You can only override the parameter values that are specified in the stack set; to add or delete a
* parameter itself, use UpdateStackSet to update the stack set template.
*/
public void setParameterOverrides(java.util.Collection parameterOverrides) {
if (parameterOverrides == null) {
this.parameterOverrides = null;
return;
}
this.parameterOverrides = new com.amazonaws.internal.SdkInternalList(parameterOverrides);
}
/**
*
* A list of stack set parameters whose values you want to override in the selected stack instances.
*
*
* Any overridden parameter values will be applied to all stack instances in the specified accounts and Amazon Web
* Services Regions. When specifying parameters and their values, be aware of how CloudFormation sets parameter
* values during stack instance operations:
*
*
* -
*
* To override the current value for a parameter, include the parameter and specify its value.
*
*
* -
*
* To leave an overridden parameter set to its present value, include the parameter and specify
* UsePreviousValue
as true
. (You can't specify both a value and set
* UsePreviousValue
to true
.)
*
*
* -
*
* To set an overridden parameter back to the value specified in the stack set, specify a parameter list but don't
* include the parameter in the list.
*
*
* -
*
* To leave all parameters set to their present values, don't specify this property at all.
*
*
*
*
* During stack set updates, any parameter values overridden for a stack instance aren't updated, but retain their
* overridden value.
*
*
* You can only override the parameter values that are specified in the stack set; to add or delete a
* parameter itself, use UpdateStackSet to update the stack set template.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setParameterOverrides(java.util.Collection)} or {@link #withParameterOverrides(java.util.Collection)} if
* you want to override the existing values.
*
*
* @param parameterOverrides
* A list of stack set parameters whose values you want to override in the selected stack instances.
*
* Any overridden parameter values will be applied to all stack instances in the specified accounts and
* Amazon Web Services Regions. When specifying parameters and their values, be aware of how CloudFormation
* sets parameter values during stack instance operations:
*
*
* -
*
* To override the current value for a parameter, include the parameter and specify its value.
*
*
* -
*
* To leave an overridden parameter set to its present value, include the parameter and specify
* UsePreviousValue
as true
. (You can't specify both a value and set
* UsePreviousValue
to true
.)
*
*
* -
*
* To set an overridden parameter back to the value specified in the stack set, specify a parameter list but
* don't include the parameter in the list.
*
*
* -
*
* To leave all parameters set to their present values, don't specify this property at all.
*
*
*
*
* During stack set updates, any parameter values overridden for a stack instance aren't updated, but retain
* their overridden value.
*
*
* You can only override the parameter values that are specified in the stack set; to add or delete a
* parameter itself, use UpdateStackSet to update the stack set template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackInstancesRequest withParameterOverrides(Parameter... parameterOverrides) {
if (this.parameterOverrides == null) {
setParameterOverrides(new com.amazonaws.internal.SdkInternalList(parameterOverrides.length));
}
for (Parameter ele : parameterOverrides) {
this.parameterOverrides.add(ele);
}
return this;
}
/**
*
* A list of stack set parameters whose values you want to override in the selected stack instances.
*
*
* Any overridden parameter values will be applied to all stack instances in the specified accounts and Amazon Web
* Services Regions. When specifying parameters and their values, be aware of how CloudFormation sets parameter
* values during stack instance operations:
*
*
* -
*
* To override the current value for a parameter, include the parameter and specify its value.
*
*
* -
*
* To leave an overridden parameter set to its present value, include the parameter and specify
* UsePreviousValue
as true
. (You can't specify both a value and set
* UsePreviousValue
to true
.)
*
*
* -
*
* To set an overridden parameter back to the value specified in the stack set, specify a parameter list but don't
* include the parameter in the list.
*
*
* -
*
* To leave all parameters set to their present values, don't specify this property at all.
*
*
*
*
* During stack set updates, any parameter values overridden for a stack instance aren't updated, but retain their
* overridden value.
*
*
* You can only override the parameter values that are specified in the stack set; to add or delete a
* parameter itself, use UpdateStackSet to update the stack set template.
*
*
* @param parameterOverrides
* A list of stack set parameters whose values you want to override in the selected stack instances.
*
* Any overridden parameter values will be applied to all stack instances in the specified accounts and
* Amazon Web Services Regions. When specifying parameters and their values, be aware of how CloudFormation
* sets parameter values during stack instance operations:
*
*
* -
*
* To override the current value for a parameter, include the parameter and specify its value.
*
*
* -
*
* To leave an overridden parameter set to its present value, include the parameter and specify
* UsePreviousValue
as true
. (You can't specify both a value and set
* UsePreviousValue
to true
.)
*
*
* -
*
* To set an overridden parameter back to the value specified in the stack set, specify a parameter list but
* don't include the parameter in the list.
*
*
* -
*
* To leave all parameters set to their present values, don't specify this property at all.
*
*
*
*
* During stack set updates, any parameter values overridden for a stack instance aren't updated, but retain
* their overridden value.
*
*
* You can only override the parameter values that are specified in the stack set; to add or delete a
* parameter itself, use UpdateStackSet to update the stack set template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackInstancesRequest withParameterOverrides(java.util.Collection parameterOverrides) {
setParameterOverrides(parameterOverrides);
return this;
}
/**
*
* Preferences for how CloudFormation performs this stack set operation.
*
*
* @param operationPreferences
* Preferences for how CloudFormation performs this stack set operation.
*/
public void setOperationPreferences(StackSetOperationPreferences operationPreferences) {
this.operationPreferences = operationPreferences;
}
/**
*
* Preferences for how CloudFormation performs this stack set operation.
*
*
* @return Preferences for how CloudFormation performs this stack set operation.
*/
public StackSetOperationPreferences getOperationPreferences() {
return this.operationPreferences;
}
/**
*
* Preferences for how CloudFormation performs this stack set operation.
*
*
* @param operationPreferences
* Preferences for how CloudFormation performs this stack set operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackInstancesRequest withOperationPreferences(StackSetOperationPreferences operationPreferences) {
setOperationPreferences(operationPreferences);
return this;
}
/**
*
* The unique identifier for this stack set operation.
*
*
* The operation ID also functions as an idempotency token, to ensure that CloudFormation performs the stack set
* operation only once, even if you retry the request multiple times. You might retry stack set operation requests
* to ensure that CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*
*
* @param operationId
* The unique identifier for this stack set operation.
*
* The operation ID also functions as an idempotency token, to ensure that CloudFormation performs the stack
* set operation only once, even if you retry the request multiple times. You might retry stack set operation
* requests to ensure that CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*/
public void setOperationId(String operationId) {
this.operationId = operationId;
}
/**
*
* The unique identifier for this stack set operation.
*
*
* The operation ID also functions as an idempotency token, to ensure that CloudFormation performs the stack set
* operation only once, even if you retry the request multiple times. You might retry stack set operation requests
* to ensure that CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*
*
* @return The unique identifier for this stack set operation.
*
* The operation ID also functions as an idempotency token, to ensure that CloudFormation performs the stack
* set operation only once, even if you retry the request multiple times. You might retry stack set
* operation requests to ensure that CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*/
public String getOperationId() {
return this.operationId;
}
/**
*
* The unique identifier for this stack set operation.
*
*
* The operation ID also functions as an idempotency token, to ensure that CloudFormation performs the stack set
* operation only once, even if you retry the request multiple times. You might retry stack set operation requests
* to ensure that CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
*
*
* @param operationId
* The unique identifier for this stack set operation.
*
* The operation ID also functions as an idempotency token, to ensure that CloudFormation performs the stack
* set operation only once, even if you retry the request multiple times. You might retry stack set operation
* requests to ensure that CloudFormation successfully received them.
*
*
* If you don't specify an operation ID, the SDK generates one automatically.
*
*
* Repeating this stack set operation with a new operation ID retries all stack instances whose status is
* OUTDATED
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public CreateStackInstancesRequest withOperationId(String operationId) {
setOperationId(operationId);
return this;
}
/**
*
* [Service-managed permissions] Specifies whether you are acting as an account administrator in the organization's
* management account or as a delegated administrator in a member account.
*
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed permissions.
*
*
* -
*
* If you are signed in to the management account, specify SELF
.
*
*
* -
*
* If you are signed in to a delegated administrator account, specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated administrator in the management account. For
* more information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
*
*
* @param callAs
* [Service-managed permissions] Specifies whether you are acting as an account administrator in the
* organization's management account or as a delegated administrator in a member account.
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed
* permissions.
*
*
* -
*
* If you are signed in to the management account, specify SELF
.
*
*
* -
*
* If you are signed in to a delegated administrator account, specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated administrator in the management
* account. For more information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
* @see CallAs
*/
public void setCallAs(String callAs) {
this.callAs = callAs;
}
/**
*
* [Service-managed permissions] Specifies whether you are acting as an account administrator in the organization's
* management account or as a delegated administrator in a member account.
*
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed permissions.
*
*
* -
*
* If you are signed in to the management account, specify SELF
.
*
*
* -
*
* If you are signed in to a delegated administrator account, specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated administrator in the management account. For
* more information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
*
*
* @return [Service-managed permissions] Specifies whether you are acting as an account administrator in the
* organization's management account or as a delegated administrator in a member account.
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed
* permissions.
*
*
* -
*
* If you are signed in to the management account, specify SELF
.
*
*
* -
*
* If you are signed in to a delegated administrator account, specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated administrator in the management
* account. For more information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
* @see CallAs
*/
public String getCallAs() {
return this.callAs;
}
/**
*
* [Service-managed permissions] Specifies whether you are acting as an account administrator in the organization's
* management account or as a delegated administrator in a member account.
*
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed permissions.
*
*
* -
*
* If you are signed in to the management account, specify SELF
.
*
*
* -
*
* If you are signed in to a delegated administrator account, specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated administrator in the management account. For
* more information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
*
*
* @param callAs
* [Service-managed permissions] Specifies whether you are acting as an account administrator in the
* organization's management account or as a delegated administrator in a member account.
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed
* permissions.
*
*
* -
*
* If you are signed in to the management account, specify SELF
.
*
*
* -
*
* If you are signed in to a delegated administrator account, specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated administrator in the management
* account. For more information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see CallAs
*/
public CreateStackInstancesRequest withCallAs(String callAs) {
setCallAs(callAs);
return this;
}
/**
*
* [Service-managed permissions] Specifies whether you are acting as an account administrator in the organization's
* management account or as a delegated administrator in a member account.
*
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed permissions.
*
*
* -
*
* If you are signed in to the management account, specify SELF
.
*
*
* -
*
* If you are signed in to a delegated administrator account, specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated administrator in the management account. For
* more information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
*
*
* @param callAs
* [Service-managed permissions] Specifies whether you are acting as an account administrator in the
* organization's management account or as a delegated administrator in a member account.
*
* By default, SELF
is specified. Use SELF
for stack sets with self-managed
* permissions.
*
*
* -
*
* If you are signed in to the management account, specify SELF
.
*
*
* -
*
* If you are signed in to a delegated administrator account, specify DELEGATED_ADMIN
.
*
*
* Your Amazon Web Services account must be registered as a delegated administrator in the management
* account. For more information, see Register a delegated administrator in the CloudFormation User Guide.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see CallAs
*/
public CreateStackInstancesRequest withCallAs(CallAs callAs) {
this.callAs = callAs.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStackSetName() != null)
sb.append("StackSetName: ").append(getStackSetName()).append(",");
if (getAccounts() != null)
sb.append("Accounts: ").append(getAccounts()).append(",");
if (getDeploymentTargets() != null)
sb.append("DeploymentTargets: ").append(getDeploymentTargets()).append(",");
if (getRegions() != null)
sb.append("Regions: ").append(getRegions()).append(",");
if (getParameterOverrides() != null)
sb.append("ParameterOverrides: ").append(getParameterOverrides()).append(",");
if (getOperationPreferences() != null)
sb.append("OperationPreferences: ").append(getOperationPreferences()).append(",");
if (getOperationId() != null)
sb.append("OperationId: ").append(getOperationId()).append(",");
if (getCallAs() != null)
sb.append("CallAs: ").append(getCallAs());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof CreateStackInstancesRequest == false)
return false;
CreateStackInstancesRequest other = (CreateStackInstancesRequest) obj;
if (other.getStackSetName() == null ^ this.getStackSetName() == null)
return false;
if (other.getStackSetName() != null && other.getStackSetName().equals(this.getStackSetName()) == false)
return false;
if (other.getAccounts() == null ^ this.getAccounts() == null)
return false;
if (other.getAccounts() != null && other.getAccounts().equals(this.getAccounts()) == false)
return false;
if (other.getDeploymentTargets() == null ^ this.getDeploymentTargets() == null)
return false;
if (other.getDeploymentTargets() != null && other.getDeploymentTargets().equals(this.getDeploymentTargets()) == false)
return false;
if (other.getRegions() == null ^ this.getRegions() == null)
return false;
if (other.getRegions() != null && other.getRegions().equals(this.getRegions()) == false)
return false;
if (other.getParameterOverrides() == null ^ this.getParameterOverrides() == null)
return false;
if (other.getParameterOverrides() != null && other.getParameterOverrides().equals(this.getParameterOverrides()) == false)
return false;
if (other.getOperationPreferences() == null ^ this.getOperationPreferences() == null)
return false;
if (other.getOperationPreferences() != null && other.getOperationPreferences().equals(this.getOperationPreferences()) == false)
return false;
if (other.getOperationId() == null ^ this.getOperationId() == null)
return false;
if (other.getOperationId() != null && other.getOperationId().equals(this.getOperationId()) == false)
return false;
if (other.getCallAs() == null ^ this.getCallAs() == null)
return false;
if (other.getCallAs() != null && other.getCallAs().equals(this.getCallAs()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStackSetName() == null) ? 0 : getStackSetName().hashCode());
hashCode = prime * hashCode + ((getAccounts() == null) ? 0 : getAccounts().hashCode());
hashCode = prime * hashCode + ((getDeploymentTargets() == null) ? 0 : getDeploymentTargets().hashCode());
hashCode = prime * hashCode + ((getRegions() == null) ? 0 : getRegions().hashCode());
hashCode = prime * hashCode + ((getParameterOverrides() == null) ? 0 : getParameterOverrides().hashCode());
hashCode = prime * hashCode + ((getOperationPreferences() == null) ? 0 : getOperationPreferences().hashCode());
hashCode = prime * hashCode + ((getOperationId() == null) ? 0 : getOperationId().hashCode());
hashCode = prime * hashCode + ((getCallAs() == null) ? 0 : getCallAs().hashCode());
return hashCode;
}
@Override
public CreateStackInstancesRequest clone() {
return (CreateStackInstancesRequest) super.clone();
}
}