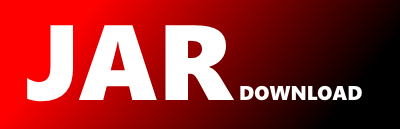
com.amazonaws.services.cloudformation.model.ResourceChange Maven / Gradle / Ivy
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudformation.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* The ResourceChange
structure describes the resource and the action that CloudFormation will perform on
* it if you execute this change set.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class ResourceChange implements Serializable, Cloneable {
/**
*
* The action that CloudFormation takes on the resource, such as Add
(adds a new resource),
* Modify
(changes a resource), Remove
(deletes a resource), Import
(imports
* a resource), or Dynamic
(exact action for the resource can't be determined).
*
*/
private String action;
/**
*
* The resource's logical ID, which is defined in the stack's template.
*
*/
private String logicalResourceId;
/**
*
* The resource's physical ID (resource name). Resources that you are adding don't have physical IDs because they
* haven't been created.
*
*/
private String physicalResourceId;
/**
*
* The type of CloudFormation resource, such as AWS::S3::Bucket
.
*
*/
private String resourceType;
/**
*
* For the Modify
action, indicates whether CloudFormation will replace the resource by creating a new
* one and deleting the old one. This value depends on the value of the RequiresRecreation
property in
* the ResourceTargetDefinition
structure. For example, if the RequiresRecreation
field is
* Always
and the Evaluation
field is Static
, Replacement
is
* True
. If the RequiresRecreation
field is Always
and the
* Evaluation
field is Dynamic
, Replacement
is Conditionally
.
*
*
* If you have multiple changes with different RequiresRecreation
values, the Replacement
* value depends on the change with the most impact. A RequiresRecreation
value of Always
* has the most impact, followed by Conditionally
, and then Never
.
*
*/
private String replacement;
/**
*
* For the Modify
action, indicates which resource attribute is triggering this update, such as a
* change in the resource attribute's Metadata
, Properties
, or Tags
.
*
*/
private com.amazonaws.internal.SdkInternalList scope;
/**
*
* For the Modify
action, a list of ResourceChangeDetail
structures that describes the
* changes that CloudFormation will make to the resource.
*
*/
private com.amazonaws.internal.SdkInternalList details;
/**
*
* The change set ID of the nested change set.
*
*/
private String changeSetId;
/**
*
* Contains information about the module from which the resource was created, if the resource was created from a
* module included in the stack template.
*
*/
private ModuleInfo moduleInfo;
/**
*
* The action that CloudFormation takes on the resource, such as Add
(adds a new resource),
* Modify
(changes a resource), Remove
(deletes a resource), Import
(imports
* a resource), or Dynamic
(exact action for the resource can't be determined).
*
*
* @param action
* The action that CloudFormation takes on the resource, such as Add
(adds a new resource),
* Modify
(changes a resource), Remove
(deletes a resource), Import
* (imports a resource), or Dynamic
(exact action for the resource can't be determined).
* @see ChangeAction
*/
public void setAction(String action) {
this.action = action;
}
/**
*
* The action that CloudFormation takes on the resource, such as Add
(adds a new resource),
* Modify
(changes a resource), Remove
(deletes a resource), Import
(imports
* a resource), or Dynamic
(exact action for the resource can't be determined).
*
*
* @return The action that CloudFormation takes on the resource, such as Add
(adds a new resource),
* Modify
(changes a resource), Remove
(deletes a resource), Import
* (imports a resource), or Dynamic
(exact action for the resource can't be determined).
* @see ChangeAction
*/
public String getAction() {
return this.action;
}
/**
*
* The action that CloudFormation takes on the resource, such as Add
(adds a new resource),
* Modify
(changes a resource), Remove
(deletes a resource), Import
(imports
* a resource), or Dynamic
(exact action for the resource can't be determined).
*
*
* @param action
* The action that CloudFormation takes on the resource, such as Add
(adds a new resource),
* Modify
(changes a resource), Remove
(deletes a resource), Import
* (imports a resource), or Dynamic
(exact action for the resource can't be determined).
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChangeAction
*/
public ResourceChange withAction(String action) {
setAction(action);
return this;
}
/**
*
* The action that CloudFormation takes on the resource, such as Add
(adds a new resource),
* Modify
(changes a resource), Remove
(deletes a resource), Import
(imports
* a resource), or Dynamic
(exact action for the resource can't be determined).
*
*
* @param action
* The action that CloudFormation takes on the resource, such as Add
(adds a new resource),
* Modify
(changes a resource), Remove
(deletes a resource), Import
* (imports a resource), or Dynamic
(exact action for the resource can't be determined).
* @see ChangeAction
*/
public void setAction(ChangeAction action) {
withAction(action);
}
/**
*
* The action that CloudFormation takes on the resource, such as Add
(adds a new resource),
* Modify
(changes a resource), Remove
(deletes a resource), Import
(imports
* a resource), or Dynamic
(exact action for the resource can't be determined).
*
*
* @param action
* The action that CloudFormation takes on the resource, such as Add
(adds a new resource),
* Modify
(changes a resource), Remove
(deletes a resource), Import
* (imports a resource), or Dynamic
(exact action for the resource can't be determined).
* @return Returns a reference to this object so that method calls can be chained together.
* @see ChangeAction
*/
public ResourceChange withAction(ChangeAction action) {
this.action = action.toString();
return this;
}
/**
*
* The resource's logical ID, which is defined in the stack's template.
*
*
* @param logicalResourceId
* The resource's logical ID, which is defined in the stack's template.
*/
public void setLogicalResourceId(String logicalResourceId) {
this.logicalResourceId = logicalResourceId;
}
/**
*
* The resource's logical ID, which is defined in the stack's template.
*
*
* @return The resource's logical ID, which is defined in the stack's template.
*/
public String getLogicalResourceId() {
return this.logicalResourceId;
}
/**
*
* The resource's logical ID, which is defined in the stack's template.
*
*
* @param logicalResourceId
* The resource's logical ID, which is defined in the stack's template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceChange withLogicalResourceId(String logicalResourceId) {
setLogicalResourceId(logicalResourceId);
return this;
}
/**
*
* The resource's physical ID (resource name). Resources that you are adding don't have physical IDs because they
* haven't been created.
*
*
* @param physicalResourceId
* The resource's physical ID (resource name). Resources that you are adding don't have physical IDs because
* they haven't been created.
*/
public void setPhysicalResourceId(String physicalResourceId) {
this.physicalResourceId = physicalResourceId;
}
/**
*
* The resource's physical ID (resource name). Resources that you are adding don't have physical IDs because they
* haven't been created.
*
*
* @return The resource's physical ID (resource name). Resources that you are adding don't have physical IDs because
* they haven't been created.
*/
public String getPhysicalResourceId() {
return this.physicalResourceId;
}
/**
*
* The resource's physical ID (resource name). Resources that you are adding don't have physical IDs because they
* haven't been created.
*
*
* @param physicalResourceId
* The resource's physical ID (resource name). Resources that you are adding don't have physical IDs because
* they haven't been created.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceChange withPhysicalResourceId(String physicalResourceId) {
setPhysicalResourceId(physicalResourceId);
return this;
}
/**
*
* The type of CloudFormation resource, such as AWS::S3::Bucket
.
*
*
* @param resourceType
* The type of CloudFormation resource, such as AWS::S3::Bucket
.
*/
public void setResourceType(String resourceType) {
this.resourceType = resourceType;
}
/**
*
* The type of CloudFormation resource, such as AWS::S3::Bucket
.
*
*
* @return The type of CloudFormation resource, such as AWS::S3::Bucket
.
*/
public String getResourceType() {
return this.resourceType;
}
/**
*
* The type of CloudFormation resource, such as AWS::S3::Bucket
.
*
*
* @param resourceType
* The type of CloudFormation resource, such as AWS::S3::Bucket
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceChange withResourceType(String resourceType) {
setResourceType(resourceType);
return this;
}
/**
*
* For the Modify
action, indicates whether CloudFormation will replace the resource by creating a new
* one and deleting the old one. This value depends on the value of the RequiresRecreation
property in
* the ResourceTargetDefinition
structure. For example, if the RequiresRecreation
field is
* Always
and the Evaluation
field is Static
, Replacement
is
* True
. If the RequiresRecreation
field is Always
and the
* Evaluation
field is Dynamic
, Replacement
is Conditionally
.
*
*
* If you have multiple changes with different RequiresRecreation
values, the Replacement
* value depends on the change with the most impact. A RequiresRecreation
value of Always
* has the most impact, followed by Conditionally
, and then Never
.
*
*
* @param replacement
* For the Modify
action, indicates whether CloudFormation will replace the resource by creating
* a new one and deleting the old one. This value depends on the value of the RequiresRecreation
* property in the ResourceTargetDefinition
structure. For example, if the
* RequiresRecreation
field is Always
and the Evaluation
field is
* Static
, Replacement
is True
. If the RequiresRecreation
* field is Always
and the Evaluation
field is Dynamic
,
* Replacement
is Conditionally
.
*
* If you have multiple changes with different RequiresRecreation
values, the
* Replacement
value depends on the change with the most impact. A
* RequiresRecreation
value of Always
has the most impact, followed by
* Conditionally
, and then Never
.
* @see Replacement
*/
public void setReplacement(String replacement) {
this.replacement = replacement;
}
/**
*
* For the Modify
action, indicates whether CloudFormation will replace the resource by creating a new
* one and deleting the old one. This value depends on the value of the RequiresRecreation
property in
* the ResourceTargetDefinition
structure. For example, if the RequiresRecreation
field is
* Always
and the Evaluation
field is Static
, Replacement
is
* True
. If the RequiresRecreation
field is Always
and the
* Evaluation
field is Dynamic
, Replacement
is Conditionally
.
*
*
* If you have multiple changes with different RequiresRecreation
values, the Replacement
* value depends on the change with the most impact. A RequiresRecreation
value of Always
* has the most impact, followed by Conditionally
, and then Never
.
*
*
* @return For the Modify
action, indicates whether CloudFormation will replace the resource by
* creating a new one and deleting the old one. This value depends on the value of the
* RequiresRecreation
property in the ResourceTargetDefinition
structure. For
* example, if the RequiresRecreation
field is Always
and the
* Evaluation
field is Static
, Replacement
is True
. If
* the RequiresRecreation
field is Always
and the Evaluation
field is
* Dynamic
, Replacement
is Conditionally
.
*
* If you have multiple changes with different RequiresRecreation
values, the
* Replacement
value depends on the change with the most impact. A
* RequiresRecreation
value of Always
has the most impact, followed by
* Conditionally
, and then Never
.
* @see Replacement
*/
public String getReplacement() {
return this.replacement;
}
/**
*
* For the Modify
action, indicates whether CloudFormation will replace the resource by creating a new
* one and deleting the old one. This value depends on the value of the RequiresRecreation
property in
* the ResourceTargetDefinition
structure. For example, if the RequiresRecreation
field is
* Always
and the Evaluation
field is Static
, Replacement
is
* True
. If the RequiresRecreation
field is Always
and the
* Evaluation
field is Dynamic
, Replacement
is Conditionally
.
*
*
* If you have multiple changes with different RequiresRecreation
values, the Replacement
* value depends on the change with the most impact. A RequiresRecreation
value of Always
* has the most impact, followed by Conditionally
, and then Never
.
*
*
* @param replacement
* For the Modify
action, indicates whether CloudFormation will replace the resource by creating
* a new one and deleting the old one. This value depends on the value of the RequiresRecreation
* property in the ResourceTargetDefinition
structure. For example, if the
* RequiresRecreation
field is Always
and the Evaluation
field is
* Static
, Replacement
is True
. If the RequiresRecreation
* field is Always
and the Evaluation
field is Dynamic
,
* Replacement
is Conditionally
.
*
* If you have multiple changes with different RequiresRecreation
values, the
* Replacement
value depends on the change with the most impact. A
* RequiresRecreation
value of Always
has the most impact, followed by
* Conditionally
, and then Never
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Replacement
*/
public ResourceChange withReplacement(String replacement) {
setReplacement(replacement);
return this;
}
/**
*
* For the Modify
action, indicates whether CloudFormation will replace the resource by creating a new
* one and deleting the old one. This value depends on the value of the RequiresRecreation
property in
* the ResourceTargetDefinition
structure. For example, if the RequiresRecreation
field is
* Always
and the Evaluation
field is Static
, Replacement
is
* True
. If the RequiresRecreation
field is Always
and the
* Evaluation
field is Dynamic
, Replacement
is Conditionally
.
*
*
* If you have multiple changes with different RequiresRecreation
values, the Replacement
* value depends on the change with the most impact. A RequiresRecreation
value of Always
* has the most impact, followed by Conditionally
, and then Never
.
*
*
* @param replacement
* For the Modify
action, indicates whether CloudFormation will replace the resource by creating
* a new one and deleting the old one. This value depends on the value of the RequiresRecreation
* property in the ResourceTargetDefinition
structure. For example, if the
* RequiresRecreation
field is Always
and the Evaluation
field is
* Static
, Replacement
is True
. If the RequiresRecreation
* field is Always
and the Evaluation
field is Dynamic
,
* Replacement
is Conditionally
.
*
* If you have multiple changes with different RequiresRecreation
values, the
* Replacement
value depends on the change with the most impact. A
* RequiresRecreation
value of Always
has the most impact, followed by
* Conditionally
, and then Never
.
* @see Replacement
*/
public void setReplacement(Replacement replacement) {
withReplacement(replacement);
}
/**
*
* For the Modify
action, indicates whether CloudFormation will replace the resource by creating a new
* one and deleting the old one. This value depends on the value of the RequiresRecreation
property in
* the ResourceTargetDefinition
structure. For example, if the RequiresRecreation
field is
* Always
and the Evaluation
field is Static
, Replacement
is
* True
. If the RequiresRecreation
field is Always
and the
* Evaluation
field is Dynamic
, Replacement
is Conditionally
.
*
*
* If you have multiple changes with different RequiresRecreation
values, the Replacement
* value depends on the change with the most impact. A RequiresRecreation
value of Always
* has the most impact, followed by Conditionally
, and then Never
.
*
*
* @param replacement
* For the Modify
action, indicates whether CloudFormation will replace the resource by creating
* a new one and deleting the old one. This value depends on the value of the RequiresRecreation
* property in the ResourceTargetDefinition
structure. For example, if the
* RequiresRecreation
field is Always
and the Evaluation
field is
* Static
, Replacement
is True
. If the RequiresRecreation
* field is Always
and the Evaluation
field is Dynamic
,
* Replacement
is Conditionally
.
*
* If you have multiple changes with different RequiresRecreation
values, the
* Replacement
value depends on the change with the most impact. A
* RequiresRecreation
value of Always
has the most impact, followed by
* Conditionally
, and then Never
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Replacement
*/
public ResourceChange withReplacement(Replacement replacement) {
this.replacement = replacement.toString();
return this;
}
/**
*
* For the Modify
action, indicates which resource attribute is triggering this update, such as a
* change in the resource attribute's Metadata
, Properties
, or Tags
.
*
*
* @return For the Modify
action, indicates which resource attribute is triggering this update, such as
* a change in the resource attribute's Metadata
, Properties
, or Tags
* .
* @see ResourceAttribute
*/
public java.util.List getScope() {
if (scope == null) {
scope = new com.amazonaws.internal.SdkInternalList();
}
return scope;
}
/**
*
* For the Modify
action, indicates which resource attribute is triggering this update, such as a
* change in the resource attribute's Metadata
, Properties
, or Tags
.
*
*
* @param scope
* For the Modify
action, indicates which resource attribute is triggering this update, such as
* a change in the resource attribute's Metadata
, Properties
, or Tags
.
* @see ResourceAttribute
*/
public void setScope(java.util.Collection scope) {
if (scope == null) {
this.scope = null;
return;
}
this.scope = new com.amazonaws.internal.SdkInternalList(scope);
}
/**
*
* For the Modify
action, indicates which resource attribute is triggering this update, such as a
* change in the resource attribute's Metadata
, Properties
, or Tags
.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setScope(java.util.Collection)} or {@link #withScope(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param scope
* For the Modify
action, indicates which resource attribute is triggering this update, such as
* a change in the resource attribute's Metadata
, Properties
, or Tags
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceAttribute
*/
public ResourceChange withScope(String... scope) {
if (this.scope == null) {
setScope(new com.amazonaws.internal.SdkInternalList(scope.length));
}
for (String ele : scope) {
this.scope.add(ele);
}
return this;
}
/**
*
* For the Modify
action, indicates which resource attribute is triggering this update, such as a
* change in the resource attribute's Metadata
, Properties
, or Tags
.
*
*
* @param scope
* For the Modify
action, indicates which resource attribute is triggering this update, such as
* a change in the resource attribute's Metadata
, Properties
, or Tags
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceAttribute
*/
public ResourceChange withScope(java.util.Collection scope) {
setScope(scope);
return this;
}
/**
*
* For the Modify
action, indicates which resource attribute is triggering this update, such as a
* change in the resource attribute's Metadata
, Properties
, or Tags
.
*
*
* @param scope
* For the Modify
action, indicates which resource attribute is triggering this update, such as
* a change in the resource attribute's Metadata
, Properties
, or Tags
.
* @return Returns a reference to this object so that method calls can be chained together.
* @see ResourceAttribute
*/
public ResourceChange withScope(ResourceAttribute... scope) {
com.amazonaws.internal.SdkInternalList scopeCopy = new com.amazonaws.internal.SdkInternalList(scope.length);
for (ResourceAttribute value : scope) {
scopeCopy.add(value.toString());
}
if (getScope() == null) {
setScope(scopeCopy);
} else {
getScope().addAll(scopeCopy);
}
return this;
}
/**
*
* For the Modify
action, a list of ResourceChangeDetail
structures that describes the
* changes that CloudFormation will make to the resource.
*
*
* @return For the Modify
action, a list of ResourceChangeDetail
structures that describes
* the changes that CloudFormation will make to the resource.
*/
public java.util.List getDetails() {
if (details == null) {
details = new com.amazonaws.internal.SdkInternalList();
}
return details;
}
/**
*
* For the Modify
action, a list of ResourceChangeDetail
structures that describes the
* changes that CloudFormation will make to the resource.
*
*
* @param details
* For the Modify
action, a list of ResourceChangeDetail
structures that describes
* the changes that CloudFormation will make to the resource.
*/
public void setDetails(java.util.Collection details) {
if (details == null) {
this.details = null;
return;
}
this.details = new com.amazonaws.internal.SdkInternalList(details);
}
/**
*
* For the Modify
action, a list of ResourceChangeDetail
structures that describes the
* changes that CloudFormation will make to the resource.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setDetails(java.util.Collection)} or {@link #withDetails(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param details
* For the Modify
action, a list of ResourceChangeDetail
structures that describes
* the changes that CloudFormation will make to the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceChange withDetails(ResourceChangeDetail... details) {
if (this.details == null) {
setDetails(new com.amazonaws.internal.SdkInternalList(details.length));
}
for (ResourceChangeDetail ele : details) {
this.details.add(ele);
}
return this;
}
/**
*
* For the Modify
action, a list of ResourceChangeDetail
structures that describes the
* changes that CloudFormation will make to the resource.
*
*
* @param details
* For the Modify
action, a list of ResourceChangeDetail
structures that describes
* the changes that CloudFormation will make to the resource.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceChange withDetails(java.util.Collection details) {
setDetails(details);
return this;
}
/**
*
* The change set ID of the nested change set.
*
*
* @param changeSetId
* The change set ID of the nested change set.
*/
public void setChangeSetId(String changeSetId) {
this.changeSetId = changeSetId;
}
/**
*
* The change set ID of the nested change set.
*
*
* @return The change set ID of the nested change set.
*/
public String getChangeSetId() {
return this.changeSetId;
}
/**
*
* The change set ID of the nested change set.
*
*
* @param changeSetId
* The change set ID of the nested change set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceChange withChangeSetId(String changeSetId) {
setChangeSetId(changeSetId);
return this;
}
/**
*
* Contains information about the module from which the resource was created, if the resource was created from a
* module included in the stack template.
*
*
* @param moduleInfo
* Contains information about the module from which the resource was created, if the resource was created
* from a module included in the stack template.
*/
public void setModuleInfo(ModuleInfo moduleInfo) {
this.moduleInfo = moduleInfo;
}
/**
*
* Contains information about the module from which the resource was created, if the resource was created from a
* module included in the stack template.
*
*
* @return Contains information about the module from which the resource was created, if the resource was created
* from a module included in the stack template.
*/
public ModuleInfo getModuleInfo() {
return this.moduleInfo;
}
/**
*
* Contains information about the module from which the resource was created, if the resource was created from a
* module included in the stack template.
*
*
* @param moduleInfo
* Contains information about the module from which the resource was created, if the resource was created
* from a module included in the stack template.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public ResourceChange withModuleInfo(ModuleInfo moduleInfo) {
setModuleInfo(moduleInfo);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getAction() != null)
sb.append("Action: ").append(getAction()).append(",");
if (getLogicalResourceId() != null)
sb.append("LogicalResourceId: ").append(getLogicalResourceId()).append(",");
if (getPhysicalResourceId() != null)
sb.append("PhysicalResourceId: ").append(getPhysicalResourceId()).append(",");
if (getResourceType() != null)
sb.append("ResourceType: ").append(getResourceType()).append(",");
if (getReplacement() != null)
sb.append("Replacement: ").append(getReplacement()).append(",");
if (getScope() != null)
sb.append("Scope: ").append(getScope()).append(",");
if (getDetails() != null)
sb.append("Details: ").append(getDetails()).append(",");
if (getChangeSetId() != null)
sb.append("ChangeSetId: ").append(getChangeSetId()).append(",");
if (getModuleInfo() != null)
sb.append("ModuleInfo: ").append(getModuleInfo());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof ResourceChange == false)
return false;
ResourceChange other = (ResourceChange) obj;
if (other.getAction() == null ^ this.getAction() == null)
return false;
if (other.getAction() != null && other.getAction().equals(this.getAction()) == false)
return false;
if (other.getLogicalResourceId() == null ^ this.getLogicalResourceId() == null)
return false;
if (other.getLogicalResourceId() != null && other.getLogicalResourceId().equals(this.getLogicalResourceId()) == false)
return false;
if (other.getPhysicalResourceId() == null ^ this.getPhysicalResourceId() == null)
return false;
if (other.getPhysicalResourceId() != null && other.getPhysicalResourceId().equals(this.getPhysicalResourceId()) == false)
return false;
if (other.getResourceType() == null ^ this.getResourceType() == null)
return false;
if (other.getResourceType() != null && other.getResourceType().equals(this.getResourceType()) == false)
return false;
if (other.getReplacement() == null ^ this.getReplacement() == null)
return false;
if (other.getReplacement() != null && other.getReplacement().equals(this.getReplacement()) == false)
return false;
if (other.getScope() == null ^ this.getScope() == null)
return false;
if (other.getScope() != null && other.getScope().equals(this.getScope()) == false)
return false;
if (other.getDetails() == null ^ this.getDetails() == null)
return false;
if (other.getDetails() != null && other.getDetails().equals(this.getDetails()) == false)
return false;
if (other.getChangeSetId() == null ^ this.getChangeSetId() == null)
return false;
if (other.getChangeSetId() != null && other.getChangeSetId().equals(this.getChangeSetId()) == false)
return false;
if (other.getModuleInfo() == null ^ this.getModuleInfo() == null)
return false;
if (other.getModuleInfo() != null && other.getModuleInfo().equals(this.getModuleInfo()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getAction() == null) ? 0 : getAction().hashCode());
hashCode = prime * hashCode + ((getLogicalResourceId() == null) ? 0 : getLogicalResourceId().hashCode());
hashCode = prime * hashCode + ((getPhysicalResourceId() == null) ? 0 : getPhysicalResourceId().hashCode());
hashCode = prime * hashCode + ((getResourceType() == null) ? 0 : getResourceType().hashCode());
hashCode = prime * hashCode + ((getReplacement() == null) ? 0 : getReplacement().hashCode());
hashCode = prime * hashCode + ((getScope() == null) ? 0 : getScope().hashCode());
hashCode = prime * hashCode + ((getDetails() == null) ? 0 : getDetails().hashCode());
hashCode = prime * hashCode + ((getChangeSetId() == null) ? 0 : getChangeSetId().hashCode());
hashCode = prime * hashCode + ((getModuleInfo() == null) ? 0 : getModuleInfo().hashCode());
return hashCode;
}
@Override
public ResourceChange clone() {
try {
return (ResourceChange) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}