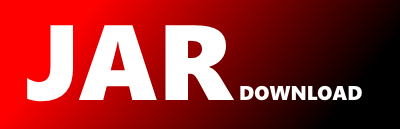
com.amazonaws.services.cloudformation.model.StackSetSummary Maven / Gradle / Ivy
/*
* Copyright 2017-2022 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudformation.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* The structures that contain summary information about the specified stack set.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StackSetSummary implements Serializable, Cloneable {
/**
*
* The name of the stack set.
*
*/
private String stackSetName;
/**
*
* The ID of the stack set.
*
*/
private String stackSetId;
/**
*
* A description of the stack set that you specify when the stack set is created or updated.
*
*/
private String description;
/**
*
* The status of the stack set.
*
*/
private String status;
/**
*
* [Service-managed permissions] Describes whether StackSets automatically deploys to Organizations accounts that
* are added to a target organizational unit (OU).
*
*/
private AutoDeployment autoDeployment;
/**
*
* Describes how the IAM roles required for stack set operations are created.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*/
private String permissionModel;
/**
*
* Status of the stack set's actual configuration compared to its expected template and parameter configuration. A
* stack set is considered to have drifted if one or more of its stack instances have drifted from their expected
* template and parameter configuration.
*
*
* -
*
* DRIFTED
: One or more of the stack instances belonging to the stack set stack differs from the
* expected template and parameter configuration. A stack instance is considered to have drifted if one or more of
* the resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation hasn't checked the stack set for drift.
*
*
* -
*
* IN_SYNC
: All the stack instances belonging to the stack set stack match from the expected template
* and parameter configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*/
private String driftStatus;
/**
*
* Most recent time when CloudFormation performed a drift detection operation on the stack set. This value will be
* NULL
for any stack set on which drift detection hasn't yet been performed.
*
*/
private java.util.Date lastDriftCheckTimestamp;
/**
*
* Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting operations.
*
*/
private ManagedExecution managedExecution;
/**
*
* The name of the stack set.
*
*
* @param stackSetName
* The name of the stack set.
*/
public void setStackSetName(String stackSetName) {
this.stackSetName = stackSetName;
}
/**
*
* The name of the stack set.
*
*
* @return The name of the stack set.
*/
public String getStackSetName() {
return this.stackSetName;
}
/**
*
* The name of the stack set.
*
*
* @param stackSetName
* The name of the stack set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetSummary withStackSetName(String stackSetName) {
setStackSetName(stackSetName);
return this;
}
/**
*
* The ID of the stack set.
*
*
* @param stackSetId
* The ID of the stack set.
*/
public void setStackSetId(String stackSetId) {
this.stackSetId = stackSetId;
}
/**
*
* The ID of the stack set.
*
*
* @return The ID of the stack set.
*/
public String getStackSetId() {
return this.stackSetId;
}
/**
*
* The ID of the stack set.
*
*
* @param stackSetId
* The ID of the stack set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetSummary withStackSetId(String stackSetId) {
setStackSetId(stackSetId);
return this;
}
/**
*
* A description of the stack set that you specify when the stack set is created or updated.
*
*
* @param description
* A description of the stack set that you specify when the stack set is created or updated.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the stack set that you specify when the stack set is created or updated.
*
*
* @return A description of the stack set that you specify when the stack set is created or updated.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the stack set that you specify when the stack set is created or updated.
*
*
* @param description
* A description of the stack set that you specify when the stack set is created or updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetSummary withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The status of the stack set.
*
*
* @param status
* The status of the stack set.
* @see StackSetStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the stack set.
*
*
* @return The status of the stack set.
* @see StackSetStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the stack set.
*
*
* @param status
* The status of the stack set.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackSetStatus
*/
public StackSetSummary withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the stack set.
*
*
* @param status
* The status of the stack set.
* @see StackSetStatus
*/
public void setStatus(StackSetStatus status) {
withStatus(status);
}
/**
*
* The status of the stack set.
*
*
* @param status
* The status of the stack set.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackSetStatus
*/
public StackSetSummary withStatus(StackSetStatus status) {
this.status = status.toString();
return this;
}
/**
*
* [Service-managed permissions] Describes whether StackSets automatically deploys to Organizations accounts that
* are added to a target organizational unit (OU).
*
*
* @param autoDeployment
* [Service-managed permissions] Describes whether StackSets automatically deploys to Organizations accounts
* that are added to a target organizational unit (OU).
*/
public void setAutoDeployment(AutoDeployment autoDeployment) {
this.autoDeployment = autoDeployment;
}
/**
*
* [Service-managed permissions] Describes whether StackSets automatically deploys to Organizations accounts that
* are added to a target organizational unit (OU).
*
*
* @return [Service-managed permissions] Describes whether StackSets automatically deploys to Organizations accounts
* that are added to a target organizational unit (OU).
*/
public AutoDeployment getAutoDeployment() {
return this.autoDeployment;
}
/**
*
* [Service-managed permissions] Describes whether StackSets automatically deploys to Organizations accounts that
* are added to a target organizational unit (OU).
*
*
* @param autoDeployment
* [Service-managed permissions] Describes whether StackSets automatically deploys to Organizations accounts
* that are added to a target organizational unit (OU).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetSummary withAutoDeployment(AutoDeployment autoDeployment) {
setAutoDeployment(autoDeployment);
return this;
}
/**
*
* Describes how the IAM roles required for stack set operations are created.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* @param permissionModel
* Describes how the IAM roles required for stack set operations are created.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required
* to deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @see PermissionModels
*/
public void setPermissionModel(String permissionModel) {
this.permissionModel = permissionModel;
}
/**
*
* Describes how the IAM roles required for stack set operations are created.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* @return Describes how the IAM roles required for stack set operations are created.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles
* required to deploy to target accounts. For more information, see Grant Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @see PermissionModels
*/
public String getPermissionModel() {
return this.permissionModel;
}
/**
*
* Describes how the IAM roles required for stack set operations are created.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* @param permissionModel
* Describes how the IAM roles required for stack set operations are created.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required
* to deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PermissionModels
*/
public StackSetSummary withPermissionModel(String permissionModel) {
setPermissionModel(permissionModel);
return this;
}
/**
*
* Describes how the IAM roles required for stack set operations are created.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* @param permissionModel
* Describes how the IAM roles required for stack set operations are created.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required
* to deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @see PermissionModels
*/
public void setPermissionModel(PermissionModels permissionModel) {
withPermissionModel(permissionModel);
}
/**
*
* Describes how the IAM roles required for stack set operations are created.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* @param permissionModel
* Describes how the IAM roles required for stack set operations are created.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required
* to deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PermissionModels
*/
public StackSetSummary withPermissionModel(PermissionModels permissionModel) {
this.permissionModel = permissionModel.toString();
return this;
}
/**
*
* Status of the stack set's actual configuration compared to its expected template and parameter configuration. A
* stack set is considered to have drifted if one or more of its stack instances have drifted from their expected
* template and parameter configuration.
*
*
* -
*
* DRIFTED
: One or more of the stack instances belonging to the stack set stack differs from the
* expected template and parameter configuration. A stack instance is considered to have drifted if one or more of
* the resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation hasn't checked the stack set for drift.
*
*
* -
*
* IN_SYNC
: All the stack instances belonging to the stack set stack match from the expected template
* and parameter configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*
* @param driftStatus
* Status of the stack set's actual configuration compared to its expected template and parameter
* configuration. A stack set is considered to have drifted if one or more of its stack instances have
* drifted from their expected template and parameter configuration.
*
* -
*
* DRIFTED
: One or more of the stack instances belonging to the stack set stack differs from the
* expected template and parameter configuration. A stack instance is considered to have drifted if one or
* more of the resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation hasn't checked the stack set for drift.
*
*
* -
*
* IN_SYNC
: All the stack instances belonging to the stack set stack match from the expected
* template and parameter configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
* @see StackDriftStatus
*/
public void setDriftStatus(String driftStatus) {
this.driftStatus = driftStatus;
}
/**
*
* Status of the stack set's actual configuration compared to its expected template and parameter configuration. A
* stack set is considered to have drifted if one or more of its stack instances have drifted from their expected
* template and parameter configuration.
*
*
* -
*
* DRIFTED
: One or more of the stack instances belonging to the stack set stack differs from the
* expected template and parameter configuration. A stack instance is considered to have drifted if one or more of
* the resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation hasn't checked the stack set for drift.
*
*
* -
*
* IN_SYNC
: All the stack instances belonging to the stack set stack match from the expected template
* and parameter configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*
* @return Status of the stack set's actual configuration compared to its expected template and parameter
* configuration. A stack set is considered to have drifted if one or more of its stack instances have
* drifted from their expected template and parameter configuration.
*
* -
*
* DRIFTED
: One or more of the stack instances belonging to the stack set stack differs from
* the expected template and parameter configuration. A stack instance is considered to have drifted if one
* or more of the resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation hasn't checked the stack set for drift.
*
*
* -
*
* IN_SYNC
: All the stack instances belonging to the stack set stack match from the expected
* template and parameter configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
* @see StackDriftStatus
*/
public String getDriftStatus() {
return this.driftStatus;
}
/**
*
* Status of the stack set's actual configuration compared to its expected template and parameter configuration. A
* stack set is considered to have drifted if one or more of its stack instances have drifted from their expected
* template and parameter configuration.
*
*
* -
*
* DRIFTED
: One or more of the stack instances belonging to the stack set stack differs from the
* expected template and parameter configuration. A stack instance is considered to have drifted if one or more of
* the resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation hasn't checked the stack set for drift.
*
*
* -
*
* IN_SYNC
: All the stack instances belonging to the stack set stack match from the expected template
* and parameter configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*
* @param driftStatus
* Status of the stack set's actual configuration compared to its expected template and parameter
* configuration. A stack set is considered to have drifted if one or more of its stack instances have
* drifted from their expected template and parameter configuration.
*
* -
*
* DRIFTED
: One or more of the stack instances belonging to the stack set stack differs from the
* expected template and parameter configuration. A stack instance is considered to have drifted if one or
* more of the resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation hasn't checked the stack set for drift.
*
*
* -
*
* IN_SYNC
: All the stack instances belonging to the stack set stack match from the expected
* template and parameter configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackDriftStatus
*/
public StackSetSummary withDriftStatus(String driftStatus) {
setDriftStatus(driftStatus);
return this;
}
/**
*
* Status of the stack set's actual configuration compared to its expected template and parameter configuration. A
* stack set is considered to have drifted if one or more of its stack instances have drifted from their expected
* template and parameter configuration.
*
*
* -
*
* DRIFTED
: One or more of the stack instances belonging to the stack set stack differs from the
* expected template and parameter configuration. A stack instance is considered to have drifted if one or more of
* the resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation hasn't checked the stack set for drift.
*
*
* -
*
* IN_SYNC
: All the stack instances belonging to the stack set stack match from the expected template
* and parameter configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*
* @param driftStatus
* Status of the stack set's actual configuration compared to its expected template and parameter
* configuration. A stack set is considered to have drifted if one or more of its stack instances have
* drifted from their expected template and parameter configuration.
*
* -
*
* DRIFTED
: One or more of the stack instances belonging to the stack set stack differs from the
* expected template and parameter configuration. A stack instance is considered to have drifted if one or
* more of the resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation hasn't checked the stack set for drift.
*
*
* -
*
* IN_SYNC
: All the stack instances belonging to the stack set stack match from the expected
* template and parameter configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
* @see StackDriftStatus
*/
public void setDriftStatus(StackDriftStatus driftStatus) {
withDriftStatus(driftStatus);
}
/**
*
* Status of the stack set's actual configuration compared to its expected template and parameter configuration. A
* stack set is considered to have drifted if one or more of its stack instances have drifted from their expected
* template and parameter configuration.
*
*
* -
*
* DRIFTED
: One or more of the stack instances belonging to the stack set stack differs from the
* expected template and parameter configuration. A stack instance is considered to have drifted if one or more of
* the resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation hasn't checked the stack set for drift.
*
*
* -
*
* IN_SYNC
: All the stack instances belonging to the stack set stack match from the expected template
* and parameter configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
*
*
* @param driftStatus
* Status of the stack set's actual configuration compared to its expected template and parameter
* configuration. A stack set is considered to have drifted if one or more of its stack instances have
* drifted from their expected template and parameter configuration.
*
* -
*
* DRIFTED
: One or more of the stack instances belonging to the stack set stack differs from the
* expected template and parameter configuration. A stack instance is considered to have drifted if one or
* more of the resources in the associated stack have drifted.
*
*
* -
*
* NOT_CHECKED
: CloudFormation hasn't checked the stack set for drift.
*
*
* -
*
* IN_SYNC
: All the stack instances belonging to the stack set stack match from the expected
* template and parameter configuration.
*
*
* -
*
* UNKNOWN
: This value is reserved for future use.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackDriftStatus
*/
public StackSetSummary withDriftStatus(StackDriftStatus driftStatus) {
this.driftStatus = driftStatus.toString();
return this;
}
/**
*
* Most recent time when CloudFormation performed a drift detection operation on the stack set. This value will be
* NULL
for any stack set on which drift detection hasn't yet been performed.
*
*
* @param lastDriftCheckTimestamp
* Most recent time when CloudFormation performed a drift detection operation on the stack set. This value
* will be NULL
for any stack set on which drift detection hasn't yet been performed.
*/
public void setLastDriftCheckTimestamp(java.util.Date lastDriftCheckTimestamp) {
this.lastDriftCheckTimestamp = lastDriftCheckTimestamp;
}
/**
*
* Most recent time when CloudFormation performed a drift detection operation on the stack set. This value will be
* NULL
for any stack set on which drift detection hasn't yet been performed.
*
*
* @return Most recent time when CloudFormation performed a drift detection operation on the stack set. This value
* will be NULL
for any stack set on which drift detection hasn't yet been performed.
*/
public java.util.Date getLastDriftCheckTimestamp() {
return this.lastDriftCheckTimestamp;
}
/**
*
* Most recent time when CloudFormation performed a drift detection operation on the stack set. This value will be
* NULL
for any stack set on which drift detection hasn't yet been performed.
*
*
* @param lastDriftCheckTimestamp
* Most recent time when CloudFormation performed a drift detection operation on the stack set. This value
* will be NULL
for any stack set on which drift detection hasn't yet been performed.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetSummary withLastDriftCheckTimestamp(java.util.Date lastDriftCheckTimestamp) {
setLastDriftCheckTimestamp(lastDriftCheckTimestamp);
return this;
}
/**
*
* Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting operations.
*
*
* @param managedExecution
* Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting
* operations.
*/
public void setManagedExecution(ManagedExecution managedExecution) {
this.managedExecution = managedExecution;
}
/**
*
* Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting operations.
*
*
* @return Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting
* operations.
*/
public ManagedExecution getManagedExecution() {
return this.managedExecution;
}
/**
*
* Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting operations.
*
*
* @param managedExecution
* Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting
* operations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetSummary withManagedExecution(ManagedExecution managedExecution) {
setManagedExecution(managedExecution);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStackSetName() != null)
sb.append("StackSetName: ").append(getStackSetName()).append(",");
if (getStackSetId() != null)
sb.append("StackSetId: ").append(getStackSetId()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getAutoDeployment() != null)
sb.append("AutoDeployment: ").append(getAutoDeployment()).append(",");
if (getPermissionModel() != null)
sb.append("PermissionModel: ").append(getPermissionModel()).append(",");
if (getDriftStatus() != null)
sb.append("DriftStatus: ").append(getDriftStatus()).append(",");
if (getLastDriftCheckTimestamp() != null)
sb.append("LastDriftCheckTimestamp: ").append(getLastDriftCheckTimestamp()).append(",");
if (getManagedExecution() != null)
sb.append("ManagedExecution: ").append(getManagedExecution());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StackSetSummary == false)
return false;
StackSetSummary other = (StackSetSummary) obj;
if (other.getStackSetName() == null ^ this.getStackSetName() == null)
return false;
if (other.getStackSetName() != null && other.getStackSetName().equals(this.getStackSetName()) == false)
return false;
if (other.getStackSetId() == null ^ this.getStackSetId() == null)
return false;
if (other.getStackSetId() != null && other.getStackSetId().equals(this.getStackSetId()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getAutoDeployment() == null ^ this.getAutoDeployment() == null)
return false;
if (other.getAutoDeployment() != null && other.getAutoDeployment().equals(this.getAutoDeployment()) == false)
return false;
if (other.getPermissionModel() == null ^ this.getPermissionModel() == null)
return false;
if (other.getPermissionModel() != null && other.getPermissionModel().equals(this.getPermissionModel()) == false)
return false;
if (other.getDriftStatus() == null ^ this.getDriftStatus() == null)
return false;
if (other.getDriftStatus() != null && other.getDriftStatus().equals(this.getDriftStatus()) == false)
return false;
if (other.getLastDriftCheckTimestamp() == null ^ this.getLastDriftCheckTimestamp() == null)
return false;
if (other.getLastDriftCheckTimestamp() != null && other.getLastDriftCheckTimestamp().equals(this.getLastDriftCheckTimestamp()) == false)
return false;
if (other.getManagedExecution() == null ^ this.getManagedExecution() == null)
return false;
if (other.getManagedExecution() != null && other.getManagedExecution().equals(this.getManagedExecution()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStackSetName() == null) ? 0 : getStackSetName().hashCode());
hashCode = prime * hashCode + ((getStackSetId() == null) ? 0 : getStackSetId().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getAutoDeployment() == null) ? 0 : getAutoDeployment().hashCode());
hashCode = prime * hashCode + ((getPermissionModel() == null) ? 0 : getPermissionModel().hashCode());
hashCode = prime * hashCode + ((getDriftStatus() == null) ? 0 : getDriftStatus().hashCode());
hashCode = prime * hashCode + ((getLastDriftCheckTimestamp() == null) ? 0 : getLastDriftCheckTimestamp().hashCode());
hashCode = prime * hashCode + ((getManagedExecution() == null) ? 0 : getManagedExecution().hashCode());
return hashCode;
}
@Override
public StackSetSummary clone() {
try {
return (StackSetSummary) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}