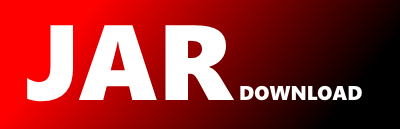
com.amazonaws.services.cloudformation.AmazonCloudFormationClient Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudformation;
import org.w3c.dom.*;
import java.net.*;
import java.util.*;
import javax.annotation.Generated;
import org.apache.commons.logging.*;
import com.amazonaws.*;
import com.amazonaws.annotation.SdkInternalApi;
import com.amazonaws.auth.*;
import com.amazonaws.handlers.*;
import com.amazonaws.http.*;
import com.amazonaws.internal.*;
import com.amazonaws.internal.auth.*;
import com.amazonaws.metrics.*;
import com.amazonaws.regions.*;
import com.amazonaws.transform.*;
import com.amazonaws.util.*;
import com.amazonaws.protocol.json.*;
import com.amazonaws.util.AWSRequestMetrics.Field;
import com.amazonaws.annotation.ThreadSafe;
import com.amazonaws.client.AwsSyncClientParams;
import com.amazonaws.client.builder.AdvancedConfig;
import com.amazonaws.services.cloudformation.AmazonCloudFormationClientBuilder;
import com.amazonaws.services.cloudformation.waiters.AmazonCloudFormationWaiters;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.services.cloudformation.model.*;
import com.amazonaws.services.cloudformation.model.transform.*;
/**
* Client for accessing AWS CloudFormation. All service calls made using this client are blocking, and will not return
* until the service call completes.
*
* CloudFormation
*
* CloudFormation allows you to create and manage Amazon Web Services infrastructure deployments predictably and
* repeatedly. You can use CloudFormation to leverage Amazon Web Services products, such as Amazon Elastic Compute
* Cloud, Amazon Elastic Block Store, Amazon Simple Notification Service, Elastic Load Balancing, and Auto Scaling to
* build highly reliable, highly scalable, cost-effective applications without creating or configuring the underlying
* Amazon Web Services infrastructure.
*
*
* With CloudFormation, you declare all your resources and dependencies in a template file. The template defines a
* collection of resources as a single unit called a stack. CloudFormation creates and deletes all member resources of
* the stack together and manages all dependencies between the resources for you.
*
*
* For more information about CloudFormation, see the CloudFormation
* product page.
*
*
* CloudFormation makes use of other Amazon Web Services products. If you need additional technical information about a
* specific Amazon Web Services product, you can find the product's technical documentation at docs.aws.amazon.com.
*
*/
@ThreadSafe
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class AmazonCloudFormationClient extends AmazonWebServiceClient implements AmazonCloudFormation {
/** Provider for AWS credentials. */
private final AWSCredentialsProvider awsCredentialsProvider;
private static final Log log = LogFactory.getLog(AmazonCloudFormation.class);
/** Default signing name for the service. */
private static final String DEFAULT_SIGNING_NAME = "cloudformation";
private volatile AmazonCloudFormationWaiters waiters;
/** Client configuration factory providing ClientConfigurations tailored to this client */
protected static final ClientConfigurationFactory configFactory = new ClientConfigurationFactory();
private final AdvancedConfig advancedConfig;
/**
* Map of exception unmarshallers for all modeled exceptions
*/
private final Map> exceptionUnmarshallersMap = new HashMap>();
/**
* List of exception unmarshallers for all modeled exceptions Even though this exceptionUnmarshallers is not used in
* Clients, this is not removed since this was directly used by Client extended classes. Using this list can cause
* performance impact.
*/
protected final List> exceptionUnmarshallers = new ArrayList>();
protected Unmarshaller defaultUnmarshaller;
/**
* Constructs a new client to invoke service methods on AWS CloudFormation. A credentials provider chain will be
* used that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonCloudFormationClientBuilder#defaultClient()}
*/
@Deprecated
public AmazonCloudFormationClient() {
this(DefaultAWSCredentialsProviderChain.getInstance(), configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWS CloudFormation. A credentials provider chain will be
* used that searches for credentials in this order:
*
* - Environment Variables - AWS_ACCESS_KEY_ID and AWS_SECRET_KEY
* - Java System Properties - aws.accessKeyId and aws.secretKey
* - Instance profile credentials delivered through the Amazon EC2 metadata service
*
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWS CloudFormation (ex: proxy
* settings, retry counts, etc.).
*
* @see DefaultAWSCredentialsProviderChain
* @deprecated use {@link AmazonCloudFormationClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonCloudFormationClient(ClientConfiguration clientConfiguration) {
this(DefaultAWSCredentialsProviderChain.getInstance(), clientConfiguration);
}
/**
* Constructs a new client to invoke service methods on AWS CloudFormation using the specified AWS account
* credentials.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @deprecated use {@link AmazonCloudFormationClientBuilder#withCredentials(AWSCredentialsProvider)} for example:
* {@code AmazonCloudFormationClientBuilder.standard().withCredentials(new AWSStaticCredentialsProvider(awsCredentials)).build();}
*/
@Deprecated
public AmazonCloudFormationClient(AWSCredentials awsCredentials) {
this(awsCredentials, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWS CloudFormation using the specified AWS account
* credentials and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentials
* The AWS credentials (access key ID and secret key) to use when authenticating with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWS CloudFormation (ex: proxy
* settings, retry counts, etc.).
* @deprecated use {@link AmazonCloudFormationClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonCloudFormationClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonCloudFormationClient(AWSCredentials awsCredentials, ClientConfiguration clientConfiguration) {
super(clientConfiguration);
this.awsCredentialsProvider = new StaticCredentialsProvider(awsCredentials);
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
/**
* Constructs a new client to invoke service methods on AWS CloudFormation using the specified AWS account
* credentials provider.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @deprecated use {@link AmazonCloudFormationClientBuilder#withCredentials(AWSCredentialsProvider)}
*/
@Deprecated
public AmazonCloudFormationClient(AWSCredentialsProvider awsCredentialsProvider) {
this(awsCredentialsProvider, configFactory.getConfig());
}
/**
* Constructs a new client to invoke service methods on AWS CloudFormation using the specified AWS account
* credentials provider and client configuration options.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWS CloudFormation (ex: proxy
* settings, retry counts, etc.).
* @deprecated use {@link AmazonCloudFormationClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonCloudFormationClientBuilder#withClientConfiguration(ClientConfiguration)}
*/
@Deprecated
public AmazonCloudFormationClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration) {
this(awsCredentialsProvider, clientConfiguration, null);
}
/**
* Constructs a new client to invoke service methods on AWS CloudFormation using the specified AWS account
* credentials provider, client configuration options, and request metric collector.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param awsCredentialsProvider
* The AWS credentials provider which will provide credentials to authenticate requests with AWS services.
* @param clientConfiguration
* The client configuration options controlling how this client connects to AWS CloudFormation (ex: proxy
* settings, retry counts, etc.).
* @param requestMetricCollector
* optional request metric collector
* @deprecated use {@link AmazonCloudFormationClientBuilder#withCredentials(AWSCredentialsProvider)} and
* {@link AmazonCloudFormationClientBuilder#withClientConfiguration(ClientConfiguration)} and
* {@link AmazonCloudFormationClientBuilder#withMetricsCollector(RequestMetricCollector)}
*/
@Deprecated
public AmazonCloudFormationClient(AWSCredentialsProvider awsCredentialsProvider, ClientConfiguration clientConfiguration,
RequestMetricCollector requestMetricCollector) {
super(clientConfiguration, requestMetricCollector);
this.awsCredentialsProvider = awsCredentialsProvider;
this.advancedConfig = AdvancedConfig.EMPTY;
init();
}
public static AmazonCloudFormationClientBuilder builder() {
return AmazonCloudFormationClientBuilder.standard();
}
/**
* Constructs a new client to invoke service methods on AWS CloudFormation using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonCloudFormationClient(AwsSyncClientParams clientParams) {
this(clientParams, false);
}
/**
* Constructs a new client to invoke service methods on AWS CloudFormation using the specified parameters.
*
*
* All service calls made using this new client object are blocking, and will not return until the service call
* completes.
*
* @param clientParams
* Object providing client parameters.
*/
AmazonCloudFormationClient(AwsSyncClientParams clientParams, boolean endpointDiscoveryEnabled) {
super(clientParams);
this.awsCredentialsProvider = clientParams.getCredentialsProvider();
this.advancedConfig = clientParams.getAdvancedConfig();
init();
}
private void init() {
if (exceptionUnmarshallersMap.get("InvalidOperationException") == null) {
exceptionUnmarshallersMap.put("InvalidOperationException", new InvalidOperationExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidOperationExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("TypeConfigurationNotFoundException") == null) {
exceptionUnmarshallersMap.put("TypeConfigurationNotFoundException", new TypeConfigurationNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new TypeConfigurationNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("TokenAlreadyExistsException") == null) {
exceptionUnmarshallersMap.put("TokenAlreadyExistsException", new TokenAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new TokenAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ConditionalCheckFailed") == null) {
exceptionUnmarshallersMap.put("ConditionalCheckFailed", new OperationStatusCheckFailedExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new OperationStatusCheckFailedExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("NameAlreadyExistsException") == null) {
exceptionUnmarshallersMap.put("NameAlreadyExistsException", new NameAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new NameAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("LimitExceededException") == null) {
exceptionUnmarshallersMap.put("LimitExceededException", new LimitExceededExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new LimitExceededExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("OperationNotFoundException") == null) {
exceptionUnmarshallersMap.put("OperationNotFoundException", new OperationNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new OperationNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("StackSetNotFoundException") == null) {
exceptionUnmarshallersMap.put("StackSetNotFoundException", new StackSetNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new StackSetNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InsufficientCapabilitiesException") == null) {
exceptionUnmarshallersMap.put("InsufficientCapabilitiesException", new InsufficientCapabilitiesExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InsufficientCapabilitiesExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("AlreadyExistsException") == null) {
exceptionUnmarshallersMap.put("AlreadyExistsException", new AlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new AlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("OperationInProgressException") == null) {
exceptionUnmarshallersMap.put("OperationInProgressException", new OperationInProgressExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new OperationInProgressExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("StaleRequestException") == null) {
exceptionUnmarshallersMap.put("StaleRequestException", new StaleRequestExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new StaleRequestExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidChangeSetStatus") == null) {
exceptionUnmarshallersMap.put("InvalidChangeSetStatus", new InvalidChangeSetStatusExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidChangeSetStatusExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("StackSetNotEmptyException") == null) {
exceptionUnmarshallersMap.put("StackSetNotEmptyException", new StackSetNotEmptyExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new StackSetNotEmptyExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("ChangeSetNotFound") == null) {
exceptionUnmarshallersMap.put("ChangeSetNotFound", new ChangeSetNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new ChangeSetNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("StackInstanceNotFoundException") == null) {
exceptionUnmarshallersMap.put("StackInstanceNotFoundException", new StackInstanceNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new StackInstanceNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("StackNotFoundException") == null) {
exceptionUnmarshallersMap.put("StackNotFoundException", new StackNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new StackNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("CFNRegistryException") == null) {
exceptionUnmarshallersMap.put("CFNRegistryException", new CFNRegistryExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new CFNRegistryExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("InvalidStateTransition") == null) {
exceptionUnmarshallersMap.put("InvalidStateTransition", new InvalidStateTransitionExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new InvalidStateTransitionExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("OperationIdAlreadyExistsException") == null) {
exceptionUnmarshallersMap.put("OperationIdAlreadyExistsException", new OperationIdAlreadyExistsExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new OperationIdAlreadyExistsExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("TypeNotFoundException") == null) {
exceptionUnmarshallersMap.put("TypeNotFoundException", new TypeNotFoundExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new TypeNotFoundExceptionUnmarshaller());
if (exceptionUnmarshallersMap.get("CreatedButModifiedException") == null) {
exceptionUnmarshallersMap.put("CreatedButModifiedException", new CreatedButModifiedExceptionUnmarshaller());
}
exceptionUnmarshallers.add(new CreatedButModifiedExceptionUnmarshaller());
defaultUnmarshaller = new StandardErrorUnmarshaller(com.amazonaws.services.cloudformation.model.AmazonCloudFormationException.class);
exceptionUnmarshallers.add(new StandardErrorUnmarshaller(com.amazonaws.services.cloudformation.model.AmazonCloudFormationException.class));
setServiceNameIntern(DEFAULT_SIGNING_NAME);
setEndpointPrefix(ENDPOINT_PREFIX);
// calling this.setEndPoint(...) will also modify the signer accordingly
this.setEndpoint("https://cloudformation.us-east-1.amazonaws.com");
HandlerChainFactory chainFactory = new HandlerChainFactory();
requestHandler2s.addAll(chainFactory.newRequestHandlerChain("/com/amazonaws/services/cloudformation/request.handlers"));
requestHandler2s.addAll(chainFactory.newRequestHandler2Chain("/com/amazonaws/services/cloudformation/request.handler2s"));
requestHandler2s.addAll(chainFactory.getGlobalHandlers());
}
/**
*
* Activate trusted access with Organizations. With trusted access between StackSets and Organizations activated,
* the management account has permissions to create and manage StackSets for your organization.
*
*
* @param activateOrganizationsAccessRequest
* @return Result of the ActivateOrganizationsAccess operation returned by the service.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @sample AmazonCloudFormation.ActivateOrganizationsAccess
* @see AWS API Documentation
*/
@Override
public ActivateOrganizationsAccessResult activateOrganizationsAccess(ActivateOrganizationsAccessRequest request) {
request = beforeClientExecution(request);
return executeActivateOrganizationsAccess(request);
}
@SdkInternalApi
final ActivateOrganizationsAccessResult executeActivateOrganizationsAccess(ActivateOrganizationsAccessRequest activateOrganizationsAccessRequest) {
ExecutionContext executionContext = createExecutionContext(activateOrganizationsAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ActivateOrganizationsAccessRequestMarshaller().marshall(super.beforeMarshalling(activateOrganizationsAccessRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ActivateOrganizationsAccess");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ActivateOrganizationsAccessResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Activates a public third-party extension, making it available for use in stack templates. For more information,
* see Using public
* extensions in the CloudFormation User Guide.
*
*
* Once you have activated a public third-party extension in your account and Region, use SetTypeConfiguration to specify configuration properties for the extension. For more information, see Configuring extensions at the account level in the CloudFormation User Guide.
*
*
* @param activateTypeRequest
* @return Result of the ActivateType operation returned by the service.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws TypeNotFoundException
* The specified extension doesn't exist in the CloudFormation registry.
* @sample AmazonCloudFormation.ActivateType
* @see AWS
* API Documentation
*/
@Override
public ActivateTypeResult activateType(ActivateTypeRequest request) {
request = beforeClientExecution(request);
return executeActivateType(request);
}
@SdkInternalApi
final ActivateTypeResult executeActivateType(ActivateTypeRequest activateTypeRequest) {
ExecutionContext executionContext = createExecutionContext(activateTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ActivateTypeRequestMarshaller().marshall(super.beforeMarshalling(activateTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ActivateType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new ActivateTypeResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns configuration data for the specified CloudFormation extensions, from the CloudFormation registry for the
* account and Region.
*
*
* For more information, see Configuring extensions at the account level in the CloudFormation User Guide.
*
*
* @param batchDescribeTypeConfigurationsRequest
* @return Result of the BatchDescribeTypeConfigurations operation returned by the service.
* @throws TypeConfigurationNotFoundException
* The specified extension configuration can't be found.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @sample AmazonCloudFormation.BatchDescribeTypeConfigurations
* @see AWS API Documentation
*/
@Override
public BatchDescribeTypeConfigurationsResult batchDescribeTypeConfigurations(BatchDescribeTypeConfigurationsRequest request) {
request = beforeClientExecution(request);
return executeBatchDescribeTypeConfigurations(request);
}
@SdkInternalApi
final BatchDescribeTypeConfigurationsResult executeBatchDescribeTypeConfigurations(
BatchDescribeTypeConfigurationsRequest batchDescribeTypeConfigurationsRequest) {
ExecutionContext executionContext = createExecutionContext(batchDescribeTypeConfigurationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new BatchDescribeTypeConfigurationsRequestMarshaller().marshall(super.beforeMarshalling(batchDescribeTypeConfigurationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "BatchDescribeTypeConfigurations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new BatchDescribeTypeConfigurationsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Cancels an update on the specified stack. If the call completes successfully, the stack rolls back the update and
* reverts to the previous stack configuration.
*
*
*
* You can cancel only stacks that are in the UPDATE_IN_PROGRESS
state.
*
*
*
* @param cancelUpdateStackRequest
* The input for the CancelUpdateStack action.
* @return Result of the CancelUpdateStack operation returned by the service.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @sample AmazonCloudFormation.CancelUpdateStack
* @see AWS API Documentation
*/
@Override
public CancelUpdateStackResult cancelUpdateStack(CancelUpdateStackRequest request) {
request = beforeClientExecution(request);
return executeCancelUpdateStack(request);
}
@SdkInternalApi
final CancelUpdateStackResult executeCancelUpdateStack(CancelUpdateStackRequest cancelUpdateStackRequest) {
ExecutionContext executionContext = createExecutionContext(cancelUpdateStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CancelUpdateStackRequestMarshaller().marshall(super.beforeMarshalling(cancelUpdateStackRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CancelUpdateStack");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CancelUpdateStackResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* For a specified stack that's in the UPDATE_ROLLBACK_FAILED
state, continues rolling it back to the
* UPDATE_ROLLBACK_COMPLETE
state. Depending on the cause of the failure, you can manually fix the error and continue the rollback. By continuing the rollback, you can return your stack to a working
* state (the UPDATE_ROLLBACK_COMPLETE
state), and then try to update the stack again.
*
*
* A stack goes into the UPDATE_ROLLBACK_FAILED
state when CloudFormation can't roll back all changes
* after a failed stack update. For example, you might have a stack that's rolling back to an old database instance
* that was deleted outside of CloudFormation. Because CloudFormation doesn't know the database was deleted, it
* assumes that the database instance still exists and attempts to roll back to it, causing the update rollback to
* fail.
*
*
* @param continueUpdateRollbackRequest
* The input for the ContinueUpdateRollback action.
* @return Result of the ContinueUpdateRollback operation returned by the service.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @sample AmazonCloudFormation.ContinueUpdateRollback
* @see AWS API Documentation
*/
@Override
public ContinueUpdateRollbackResult continueUpdateRollback(ContinueUpdateRollbackRequest request) {
request = beforeClientExecution(request);
return executeContinueUpdateRollback(request);
}
@SdkInternalApi
final ContinueUpdateRollbackResult executeContinueUpdateRollback(ContinueUpdateRollbackRequest continueUpdateRollbackRequest) {
ExecutionContext executionContext = createExecutionContext(continueUpdateRollbackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ContinueUpdateRollbackRequestMarshaller().marshall(super.beforeMarshalling(continueUpdateRollbackRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ContinueUpdateRollback");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ContinueUpdateRollbackResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a list of changes that will be applied to a stack so that you can review the changes before executing
* them. You can create a change set for a stack that doesn't exist or an existing stack. If you create a change set
* for a stack that doesn't exist, the change set shows all of the resources that CloudFormation will create. If you
* create a change set for an existing stack, CloudFormation compares the stack's information with the information
* that you submit in the change set and lists the differences. Use change sets to understand which resources
* CloudFormation will create or change, and how it will change resources in an existing stack, before you create or
* update a stack.
*
*
* To create a change set for a stack that doesn't exist, for the ChangeSetType
parameter, specify
* CREATE
. To create a change set for an existing stack, specify UPDATE
for the
* ChangeSetType
parameter. To create a change set for an import operation, specify IMPORT
* for the ChangeSetType
parameter. After the CreateChangeSet
call successfully completes,
* CloudFormation starts creating the change set. To check the status of the change set or to review it, use the
* DescribeChangeSet action.
*
*
* When you are satisfied with the changes the change set will make, execute the change set by using the
* ExecuteChangeSet action. CloudFormation doesn't make changes until you execute the change set.
*
*
* To create a change set for the entire stack hierarchy, set IncludeNestedStacks
to True
.
*
*
* @param createChangeSetRequest
* The input for the CreateChangeSet action.
* @return Result of the CreateChangeSet operation returned by the service.
* @throws AlreadyExistsException
* The resource with the name requested already exists.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @throws LimitExceededException
* The quota for the resource has already been reached.
*
* For information about resource and stack limitations, see CloudFormation quotas in the CloudFormation User Guide.
* @sample AmazonCloudFormation.CreateChangeSet
* @see AWS
* API Documentation
*/
@Override
public CreateChangeSetResult createChangeSet(CreateChangeSetRequest request) {
request = beforeClientExecution(request);
return executeCreateChangeSet(request);
}
@SdkInternalApi
final CreateChangeSetResult executeCreateChangeSet(CreateChangeSetRequest createChangeSetRequest) {
ExecutionContext executionContext = createExecutionContext(createChangeSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateChangeSetRequestMarshaller().marshall(super.beforeMarshalling(createChangeSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateChangeSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateChangeSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a stack as specified in the template. After the call completes successfully, the stack creation starts.
* You can check the status of the stack through the DescribeStacks operation.
*
*
* @param createStackRequest
* The input for CreateStack action.
* @return Result of the CreateStack operation returned by the service.
* @throws LimitExceededException
* The quota for the resource has already been reached.
*
* For information about resource and stack limitations, see CloudFormation quotas in the CloudFormation User Guide.
* @throws AlreadyExistsException
* The resource with the name requested already exists.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @sample AmazonCloudFormation.CreateStack
* @see AWS API
* Documentation
*/
@Override
public CreateStackResult createStack(CreateStackRequest request) {
request = beforeClientExecution(request);
return executeCreateStack(request);
}
@SdkInternalApi
final CreateStackResult executeCreateStack(CreateStackRequest createStackRequest) {
ExecutionContext executionContext = createExecutionContext(createStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateStackRequestMarshaller().marshall(super.beforeMarshalling(createStackRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateStack");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new CreateStackResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates stack instances for the specified accounts, within the specified Amazon Web Services Regions. A stack
* instance refers to a stack in a specific account and Region. You must specify at least one value for either
* Accounts
or DeploymentTargets
, and you must specify at least one value for
* Regions
.
*
*
* @param createStackInstancesRequest
* @return Result of the CreateStackInstances operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws LimitExceededException
* The quota for the resource has already been reached.
*
* For information about resource and stack limitations, see CloudFormation quotas in the CloudFormation User Guide.
* @sample AmazonCloudFormation.CreateStackInstances
* @see AWS API Documentation
*/
@Override
public CreateStackInstancesResult createStackInstances(CreateStackInstancesRequest request) {
request = beforeClientExecution(request);
return executeCreateStackInstances(request);
}
@SdkInternalApi
final CreateStackInstancesResult executeCreateStackInstances(CreateStackInstancesRequest createStackInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(createStackInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateStackInstancesRequestMarshaller().marshall(super.beforeMarshalling(createStackInstancesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateStackInstances");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateStackInstancesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Creates a stack set.
*
*
* @param createStackSetRequest
* @return Result of the CreateStackSet operation returned by the service.
* @throws NameAlreadyExistsException
* The specified name is already in use.
* @throws CreatedButModifiedException
* The specified resource exists, but has been changed.
* @throws LimitExceededException
* The quota for the resource has already been reached.
*
* For information about resource and stack limitations, see CloudFormation quotas in the CloudFormation User Guide.
* @sample AmazonCloudFormation.CreateStackSet
* @see AWS
* API Documentation
*/
@Override
public CreateStackSetResult createStackSet(CreateStackSetRequest request) {
request = beforeClientExecution(request);
return executeCreateStackSet(request);
}
@SdkInternalApi
final CreateStackSetResult executeCreateStackSet(CreateStackSetRequest createStackSetRequest) {
ExecutionContext executionContext = createExecutionContext(createStackSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new CreateStackSetRequestMarshaller().marshall(super.beforeMarshalling(createStackSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "CreateStackSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new CreateStackSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deactivates trusted access with Organizations. If trusted access is deactivated, the management account does not
* have permissions to create and manage service-managed StackSets for your organization.
*
*
* @param deactivateOrganizationsAccessRequest
* @return Result of the DeactivateOrganizationsAccess operation returned by the service.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @sample AmazonCloudFormation.DeactivateOrganizationsAccess
* @see AWS API Documentation
*/
@Override
public DeactivateOrganizationsAccessResult deactivateOrganizationsAccess(DeactivateOrganizationsAccessRequest request) {
request = beforeClientExecution(request);
return executeDeactivateOrganizationsAccess(request);
}
@SdkInternalApi
final DeactivateOrganizationsAccessResult executeDeactivateOrganizationsAccess(DeactivateOrganizationsAccessRequest deactivateOrganizationsAccessRequest) {
ExecutionContext executionContext = createExecutionContext(deactivateOrganizationsAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeactivateOrganizationsAccessRequestMarshaller().marshall(super.beforeMarshalling(deactivateOrganizationsAccessRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeactivateOrganizationsAccess");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeactivateOrganizationsAccessResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deactivates a public extension that was previously activated in this account and Region.
*
*
* Once deactivated, an extension can't be used in any CloudFormation operation. This includes stack update
* operations where the stack template includes the extension, even if no updates are being made to the extension.
* In addition, deactivated extensions aren't automatically updated if a new version of the extension is released.
*
*
* @param deactivateTypeRequest
* @return Result of the DeactivateType operation returned by the service.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws TypeNotFoundException
* The specified extension doesn't exist in the CloudFormation registry.
* @sample AmazonCloudFormation.DeactivateType
* @see AWS
* API Documentation
*/
@Override
public DeactivateTypeResult deactivateType(DeactivateTypeRequest request) {
request = beforeClientExecution(request);
return executeDeactivateType(request);
}
@SdkInternalApi
final DeactivateTypeResult executeDeactivateType(DeactivateTypeRequest deactivateTypeRequest) {
ExecutionContext executionContext = createExecutionContext(deactivateTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeactivateTypeRequestMarshaller().marshall(super.beforeMarshalling(deactivateTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeactivateType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeactivateTypeResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes the specified change set. Deleting change sets ensures that no one executes the wrong change set.
*
*
* If the call successfully completes, CloudFormation successfully deleted the change set.
*
*
* If IncludeNestedStacks
specifies True
during the creation of the nested change set,
* then DeleteChangeSet
will delete all change sets that belong to the stacks hierarchy and will also
* delete all change sets for nested stacks with the status of REVIEW_IN_PROGRESS
.
*
*
* @param deleteChangeSetRequest
* The input for the DeleteChangeSet action.
* @return Result of the DeleteChangeSet operation returned by the service.
* @throws InvalidChangeSetStatusException
* The specified change set can't be used to update the stack. For example, the change set status might be
* CREATE_IN_PROGRESS
, or the stack status might be UPDATE_IN_PROGRESS
.
* @sample AmazonCloudFormation.DeleteChangeSet
* @see AWS
* API Documentation
*/
@Override
public DeleteChangeSetResult deleteChangeSet(DeleteChangeSetRequest request) {
request = beforeClientExecution(request);
return executeDeleteChangeSet(request);
}
@SdkInternalApi
final DeleteChangeSetResult executeDeleteChangeSet(DeleteChangeSetRequest deleteChangeSetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteChangeSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteChangeSetRequestMarshaller().marshall(super.beforeMarshalling(deleteChangeSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteChangeSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteChangeSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a specified stack. Once the call completes successfully, stack deletion starts. Deleted stacks don't show
* up in the DescribeStacks operation if the deletion has been completed successfully.
*
*
* @param deleteStackRequest
* The input for DeleteStack action.
* @return Result of the DeleteStack operation returned by the service.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @sample AmazonCloudFormation.DeleteStack
* @see AWS API
* Documentation
*/
@Override
public DeleteStackResult deleteStack(DeleteStackRequest request) {
request = beforeClientExecution(request);
return executeDeleteStack(request);
}
@SdkInternalApi
final DeleteStackResult executeDeleteStack(DeleteStackRequest deleteStackRequest) {
ExecutionContext executionContext = createExecutionContext(deleteStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteStackRequestMarshaller().marshall(super.beforeMarshalling(deleteStackRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteStack");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DeleteStackResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes stack instances for the specified accounts, in the specified Amazon Web Services Regions.
*
*
* @param deleteStackInstancesRequest
* @return Result of the DeleteStackInstances operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @sample AmazonCloudFormation.DeleteStackInstances
* @see AWS API Documentation
*/
@Override
public DeleteStackInstancesResult deleteStackInstances(DeleteStackInstancesRequest request) {
request = beforeClientExecution(request);
return executeDeleteStackInstances(request);
}
@SdkInternalApi
final DeleteStackInstancesResult executeDeleteStackInstances(DeleteStackInstancesRequest deleteStackInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(deleteStackInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteStackInstancesRequestMarshaller().marshall(super.beforeMarshalling(deleteStackInstancesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteStackInstances");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteStackInstancesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Deletes a stack set. Before you can delete a stack set, all its member stack instances must be deleted. For more
* information about how to complete this, see DeleteStackInstances.
*
*
* @param deleteStackSetRequest
* @return Result of the DeleteStackSet operation returned by the service.
* @throws StackSetNotEmptyException
* You can't yet delete this stack set, because it still contains one or more stack instances. Delete all
* stack instances from the stack set before deleting the stack set.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @sample AmazonCloudFormation.DeleteStackSet
* @see AWS
* API Documentation
*/
@Override
public DeleteStackSetResult deleteStackSet(DeleteStackSetRequest request) {
request = beforeClientExecution(request);
return executeDeleteStackSet(request);
}
@SdkInternalApi
final DeleteStackSetResult executeDeleteStackSet(DeleteStackSetRequest deleteStackSetRequest) {
ExecutionContext executionContext = createExecutionContext(deleteStackSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeleteStackSetRequestMarshaller().marshall(super.beforeMarshalling(deleteStackSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeleteStackSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeleteStackSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Marks an extension or extension version as DEPRECATED
in the CloudFormation registry, removing it
* from active use. Deprecated extensions or extension versions cannot be used in CloudFormation operations.
*
*
* To deregister an entire extension, you must individually deregister all active versions of that extension. If an
* extension has only a single active version, deregistering that version results in the extension itself being
* deregistered and marked as deprecated in the registry.
*
*
* You can't deregister the default version of an extension if there are other active version of that extension. If
* you do deregister the default version of an extension, the extension type itself is deregistered as well and
* marked as deprecated.
*
*
* To view the deprecation status of an extension or extension version, use DescribeType.
*
*
* @param deregisterTypeRequest
* @return Result of the DeregisterType operation returned by the service.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws TypeNotFoundException
* The specified extension doesn't exist in the CloudFormation registry.
* @sample AmazonCloudFormation.DeregisterType
* @see AWS
* API Documentation
*/
@Override
public DeregisterTypeResult deregisterType(DeregisterTypeRequest request) {
request = beforeClientExecution(request);
return executeDeregisterType(request);
}
@SdkInternalApi
final DeregisterTypeResult executeDeregisterType(DeregisterTypeRequest deregisterTypeRequest) {
ExecutionContext executionContext = createExecutionContext(deregisterTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DeregisterTypeRequestMarshaller().marshall(super.beforeMarshalling(deregisterTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DeregisterType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DeregisterTypeResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves your account's CloudFormation limits, such as the maximum number of stacks that you can create in your
* account. For more information about account limits, see CloudFormation
* Quotas in the CloudFormation User Guide.
*
*
* @param describeAccountLimitsRequest
* The input for the DescribeAccountLimits action.
* @return Result of the DescribeAccountLimits operation returned by the service.
* @sample AmazonCloudFormation.DescribeAccountLimits
* @see AWS API Documentation
*/
@Override
public DescribeAccountLimitsResult describeAccountLimits(DescribeAccountLimitsRequest request) {
request = beforeClientExecution(request);
return executeDescribeAccountLimits(request);
}
@SdkInternalApi
final DescribeAccountLimitsResult executeDescribeAccountLimits(DescribeAccountLimitsRequest describeAccountLimitsRequest) {
ExecutionContext executionContext = createExecutionContext(describeAccountLimitsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeAccountLimitsRequestMarshaller().marshall(super.beforeMarshalling(describeAccountLimitsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeAccountLimits");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeAccountLimitsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the inputs for the change set and a list of changes that CloudFormation will make if you execute the
* change set. For more information, see Updating Stacks Using Change Sets in the CloudFormation User Guide.
*
*
* @param describeChangeSetRequest
* The input for the DescribeChangeSet action.
* @return Result of the DescribeChangeSet operation returned by the service.
* @throws ChangeSetNotFoundException
* The specified change set name or ID doesn't exit. To view valid change sets for a stack, use the
* ListChangeSets
operation.
* @sample AmazonCloudFormation.DescribeChangeSet
* @see AWS API Documentation
*/
@Override
public DescribeChangeSetResult describeChangeSet(DescribeChangeSetRequest request) {
request = beforeClientExecution(request);
return executeDescribeChangeSet(request);
}
@SdkInternalApi
final DescribeChangeSetResult executeDescribeChangeSet(DescribeChangeSetRequest describeChangeSetRequest) {
ExecutionContext executionContext = createExecutionContext(describeChangeSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeChangeSetRequestMarshaller().marshall(super.beforeMarshalling(describeChangeSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeChangeSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeChangeSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns hook-related information for the change set and a list of changes that CloudFormation makes when you run
* the change set.
*
*
* @param describeChangeSetHooksRequest
* @return Result of the DescribeChangeSetHooks operation returned by the service.
* @throws ChangeSetNotFoundException
* The specified change set name or ID doesn't exit. To view valid change sets for a stack, use the
* ListChangeSets
operation.
* @sample AmazonCloudFormation.DescribeChangeSetHooks
* @see AWS API Documentation
*/
@Override
public DescribeChangeSetHooksResult describeChangeSetHooks(DescribeChangeSetHooksRequest request) {
request = beforeClientExecution(request);
return executeDescribeChangeSetHooks(request);
}
@SdkInternalApi
final DescribeChangeSetHooksResult executeDescribeChangeSetHooks(DescribeChangeSetHooksRequest describeChangeSetHooksRequest) {
ExecutionContext executionContext = createExecutionContext(describeChangeSetHooksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeChangeSetHooksRequestMarshaller().marshall(super.beforeMarshalling(describeChangeSetHooksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeChangeSetHooks");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeChangeSetHooksResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Retrieves information about the account's OrganizationAccess
status. This API can be called either
* by the management account or the delegated administrator by using the CallAs
parameter. This API can
* also be called without the CallAs
parameter by the management account.
*
*
* @param describeOrganizationsAccessRequest
* @return Result of the DescribeOrganizationsAccess operation returned by the service.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @sample AmazonCloudFormation.DescribeOrganizationsAccess
* @see AWS API Documentation
*/
@Override
public DescribeOrganizationsAccessResult describeOrganizationsAccess(DescribeOrganizationsAccessRequest request) {
request = beforeClientExecution(request);
return executeDescribeOrganizationsAccess(request);
}
@SdkInternalApi
final DescribeOrganizationsAccessResult executeDescribeOrganizationsAccess(DescribeOrganizationsAccessRequest describeOrganizationsAccessRequest) {
ExecutionContext executionContext = createExecutionContext(describeOrganizationsAccessRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeOrganizationsAccessRequestMarshaller().marshall(super.beforeMarshalling(describeOrganizationsAccessRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeOrganizationsAccess");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeOrganizationsAccessResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a CloudFormation extension publisher.
*
*
* If you don't supply a PublisherId
, and you have registered as an extension publisher,
* DescribePublisher
returns information about your own publisher account.
*
*
* For more information about registering as a publisher, see:
*
*
* -
*
*
* -
*
* Publishing
* extensions to make them available for public use in the CloudFormation CLI User Guide
*
*
*
*
* @param describePublisherRequest
* @return Result of the DescribePublisher operation returned by the service.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @sample AmazonCloudFormation.DescribePublisher
* @see AWS API Documentation
*/
@Override
public DescribePublisherResult describePublisher(DescribePublisherRequest request) {
request = beforeClientExecution(request);
return executeDescribePublisher(request);
}
@SdkInternalApi
final DescribePublisherResult executeDescribePublisher(DescribePublisherRequest describePublisherRequest) {
ExecutionContext executionContext = createExecutionContext(describePublisherRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribePublisherRequestMarshaller().marshall(super.beforeMarshalling(describePublisherRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribePublisher");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribePublisherResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a stack drift detection operation. A stack drift detection operation detects whether a
* stack's actual configuration differs, or has drifted, from its expected configuration, as defined in the
* stack template and any values specified as template parameters. A stack is considered to have drifted if one or
* more of its resources have drifted. For more information about stack and resource drift, see Detecting
* Unregulated Configuration Changes to Stacks and Resources.
*
*
* Use DetectStackDrift to initiate a stack drift detection operation. DetectStackDrift
returns
* a StackDriftDetectionId
you can use to monitor the progress of the operation using
* DescribeStackDriftDetectionStatus
. Once the drift detection operation has completed, use
* DescribeStackResourceDrifts to return drift information about the stack and its resources.
*
*
* @param describeStackDriftDetectionStatusRequest
* @return Result of the DescribeStackDriftDetectionStatus operation returned by the service.
* @sample AmazonCloudFormation.DescribeStackDriftDetectionStatus
* @see AWS API Documentation
*/
@Override
public DescribeStackDriftDetectionStatusResult describeStackDriftDetectionStatus(DescribeStackDriftDetectionStatusRequest request) {
request = beforeClientExecution(request);
return executeDescribeStackDriftDetectionStatus(request);
}
@SdkInternalApi
final DescribeStackDriftDetectionStatusResult executeDescribeStackDriftDetectionStatus(
DescribeStackDriftDetectionStatusRequest describeStackDriftDetectionStatusRequest) {
ExecutionContext executionContext = createExecutionContext(describeStackDriftDetectionStatusRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStackDriftDetectionStatusRequestMarshaller().marshall(super.beforeMarshalling(describeStackDriftDetectionStatusRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeStackDriftDetectionStatus");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeStackDriftDetectionStatusResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns all stack related events for a specified stack in reverse chronological order. For more information about
* a stack's event history, go to Stacks in the
* CloudFormation User Guide.
*
*
*
* You can list events for stacks that have failed to create or have been deleted by specifying the unique stack
* identifier (stack ID).
*
*
*
* @param describeStackEventsRequest
* The input for DescribeStackEvents action.
* @return Result of the DescribeStackEvents operation returned by the service.
* @sample AmazonCloudFormation.DescribeStackEvents
* @see AWS API Documentation
*/
@Override
public DescribeStackEventsResult describeStackEvents(DescribeStackEventsRequest request) {
request = beforeClientExecution(request);
return executeDescribeStackEvents(request);
}
@SdkInternalApi
final DescribeStackEventsResult executeDescribeStackEvents(DescribeStackEventsRequest describeStackEventsRequest) {
ExecutionContext executionContext = createExecutionContext(describeStackEventsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStackEventsRequestMarshaller().marshall(super.beforeMarshalling(describeStackEventsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeStackEvents");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeStackEventsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the stack instance that's associated with the specified StackSet, Amazon Web Services account, and Amazon
* Web Services Region.
*
*
* For a list of stack instances that are associated with a specific StackSet, use ListStackInstances.
*
*
* @param describeStackInstanceRequest
* @return Result of the DescribeStackInstance operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws StackInstanceNotFoundException
* The specified stack instance doesn't exist.
* @sample AmazonCloudFormation.DescribeStackInstance
* @see AWS API Documentation
*/
@Override
public DescribeStackInstanceResult describeStackInstance(DescribeStackInstanceRequest request) {
request = beforeClientExecution(request);
return executeDescribeStackInstance(request);
}
@SdkInternalApi
final DescribeStackInstanceResult executeDescribeStackInstance(DescribeStackInstanceRequest describeStackInstanceRequest) {
ExecutionContext executionContext = createExecutionContext(describeStackInstanceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStackInstanceRequestMarshaller().marshall(super.beforeMarshalling(describeStackInstanceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeStackInstance");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeStackInstanceResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns a description of the specified resource in the specified stack.
*
*
* For deleted stacks, DescribeStackResource returns resource information for up to 90 days after the stack has been
* deleted.
*
*
* @param describeStackResourceRequest
* The input for DescribeStackResource action.
* @return Result of the DescribeStackResource operation returned by the service.
* @sample AmazonCloudFormation.DescribeStackResource
* @see AWS API Documentation
*/
@Override
public DescribeStackResourceResult describeStackResource(DescribeStackResourceRequest request) {
request = beforeClientExecution(request);
return executeDescribeStackResource(request);
}
@SdkInternalApi
final DescribeStackResourceResult executeDescribeStackResource(DescribeStackResourceRequest describeStackResourceRequest) {
ExecutionContext executionContext = createExecutionContext(describeStackResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStackResourceRequestMarshaller().marshall(super.beforeMarshalling(describeStackResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeStackResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeStackResourceResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns drift information for the resources that have been checked for drift in the specified stack. This
* includes actual and expected configuration values for resources where CloudFormation detects configuration drift.
*
*
* For a given stack, there will be one StackResourceDrift
for each stack resource that has been
* checked for drift. Resources that haven't yet been checked for drift aren't included. Resources that don't
* currently support drift detection aren't checked, and so not included. For a list of resources that support drift
* detection, see Resources that Support Drift Detection.
*
*
* Use DetectStackResourceDrift to detect drift on individual resources, or DetectStackDrift to detect
* drift on all supported resources for a given stack.
*
*
* @param describeStackResourceDriftsRequest
* @return Result of the DescribeStackResourceDrifts operation returned by the service.
* @sample AmazonCloudFormation.DescribeStackResourceDrifts
* @see AWS API Documentation
*/
@Override
public DescribeStackResourceDriftsResult describeStackResourceDrifts(DescribeStackResourceDriftsRequest request) {
request = beforeClientExecution(request);
return executeDescribeStackResourceDrifts(request);
}
@SdkInternalApi
final DescribeStackResourceDriftsResult executeDescribeStackResourceDrifts(DescribeStackResourceDriftsRequest describeStackResourceDriftsRequest) {
ExecutionContext executionContext = createExecutionContext(describeStackResourceDriftsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStackResourceDriftsRequestMarshaller().marshall(super.beforeMarshalling(describeStackResourceDriftsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeStackResourceDrifts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeStackResourceDriftsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns Amazon Web Services resource descriptions for running and deleted stacks. If StackName
is
* specified, all the associated resources that are part of the stack are returned. If
* PhysicalResourceId
is specified, the associated resources of the stack that the resource belongs to
* are returned.
*
*
*
* Only the first 100 resources will be returned. If your stack has more resources than this, you should use
* ListStackResources
instead.
*
*
*
* For deleted stacks, DescribeStackResources
returns resource information for up to 90 days after the
* stack has been deleted.
*
*
* You must specify either StackName
or PhysicalResourceId
, but not both. In addition, you
* can specify LogicalResourceId
to filter the returned result. For more information about resources,
* the LogicalResourceId
and PhysicalResourceId
, go to the CloudFormation User Guide.
*
*
*
* A ValidationError
is returned if you specify both StackName
and
* PhysicalResourceId
in the same request.
*
*
*
* @param describeStackResourcesRequest
* The input for DescribeStackResources action.
* @return Result of the DescribeStackResources operation returned by the service.
* @sample AmazonCloudFormation.DescribeStackResources
* @see AWS API Documentation
*/
@Override
public DescribeStackResourcesResult describeStackResources(DescribeStackResourcesRequest request) {
request = beforeClientExecution(request);
return executeDescribeStackResources(request);
}
@SdkInternalApi
final DescribeStackResourcesResult executeDescribeStackResources(DescribeStackResourcesRequest describeStackResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(describeStackResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStackResourcesRequestMarshaller().marshall(super.beforeMarshalling(describeStackResourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeStackResources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeStackResourcesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the description of the specified StackSet.
*
*
* @param describeStackSetRequest
* @return Result of the DescribeStackSet operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @sample AmazonCloudFormation.DescribeStackSet
* @see AWS API Documentation
*/
@Override
public DescribeStackSetResult describeStackSet(DescribeStackSetRequest request) {
request = beforeClientExecution(request);
return executeDescribeStackSet(request);
}
@SdkInternalApi
final DescribeStackSetResult executeDescribeStackSet(DescribeStackSetRequest describeStackSetRequest) {
ExecutionContext executionContext = createExecutionContext(describeStackSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStackSetRequestMarshaller().marshall(super.beforeMarshalling(describeStackSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeStackSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeStackSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the description of the specified StackSet operation.
*
*
* @param describeStackSetOperationRequest
* @return Result of the DescribeStackSetOperation operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @sample AmazonCloudFormation.DescribeStackSetOperation
* @see AWS API Documentation
*/
@Override
public DescribeStackSetOperationResult describeStackSetOperation(DescribeStackSetOperationRequest request) {
request = beforeClientExecution(request);
return executeDescribeStackSetOperation(request);
}
@SdkInternalApi
final DescribeStackSetOperationResult executeDescribeStackSetOperation(DescribeStackSetOperationRequest describeStackSetOperationRequest) {
ExecutionContext executionContext = createExecutionContext(describeStackSetOperationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStackSetOperationRequestMarshaller().marshall(super.beforeMarshalling(describeStackSetOperationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeStackSetOperation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeStackSetOperationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the description for the specified stack; if no stack name was specified, then it returns the description
* for all the stacks created.
*
*
*
* If the stack doesn't exist, a ValidationError
is returned.
*
*
*
* @param describeStacksRequest
* The input for DescribeStacks action.
* @return Result of the DescribeStacks operation returned by the service.
* @sample AmazonCloudFormation.DescribeStacks
* @see AWS
* API Documentation
*/
@Override
public DescribeStacksResult describeStacks(DescribeStacksRequest request) {
request = beforeClientExecution(request);
return executeDescribeStacks(request);
}
@SdkInternalApi
final DescribeStacksResult executeDescribeStacks(DescribeStacksRequest describeStacksRequest) {
ExecutionContext executionContext = createExecutionContext(describeStacksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeStacksRequestMarshaller().marshall(super.beforeMarshalling(describeStacksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeStacks");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeStacksResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public DescribeStacksResult describeStacks() {
return describeStacks(new DescribeStacksRequest());
}
/**
*
* Returns detailed information about an extension that has been registered.
*
*
* If you specify a VersionId
, DescribeType
returns information about that specific
* extension version. Otherwise, it returns information about the default extension version.
*
*
* @param describeTypeRequest
* @return Result of the DescribeType operation returned by the service.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws TypeNotFoundException
* The specified extension doesn't exist in the CloudFormation registry.
* @sample AmazonCloudFormation.DescribeType
* @see AWS
* API Documentation
*/
@Override
public DescribeTypeResult describeType(DescribeTypeRequest request) {
request = beforeClientExecution(request);
return executeDescribeType(request);
}
@SdkInternalApi
final DescribeTypeResult executeDescribeType(DescribeTypeRequest describeTypeRequest) {
ExecutionContext executionContext = createExecutionContext(describeTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTypeRequestMarshaller().marshall(super.beforeMarshalling(describeTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new DescribeTypeResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about an extension's registration, including its current status and type and version
* identifiers.
*
*
* When you initiate a registration request using RegisterType, you can then use
* DescribeTypeRegistration to monitor the progress of that registration request.
*
*
* Once the registration request has completed, use DescribeType to return detailed information about an
* extension.
*
*
* @param describeTypeRegistrationRequest
* @return Result of the DescribeTypeRegistration operation returned by the service.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @sample AmazonCloudFormation.DescribeTypeRegistration
* @see AWS API Documentation
*/
@Override
public DescribeTypeRegistrationResult describeTypeRegistration(DescribeTypeRegistrationRequest request) {
request = beforeClientExecution(request);
return executeDescribeTypeRegistration(request);
}
@SdkInternalApi
final DescribeTypeRegistrationResult executeDescribeTypeRegistration(DescribeTypeRegistrationRequest describeTypeRegistrationRequest) {
ExecutionContext executionContext = createExecutionContext(describeTypeRegistrationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DescribeTypeRegistrationRequestMarshaller().marshall(super.beforeMarshalling(describeTypeRegistrationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DescribeTypeRegistration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DescribeTypeRegistrationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Detects whether a stack's actual configuration differs, or has drifted, from its expected configuration,
* as defined in the stack template and any values specified as template parameters. For each resource in the stack
* that supports drift detection, CloudFormation compares the actual configuration of the resource with its expected
* template configuration. Only resource properties explicitly defined in the stack template are checked for drift.
* A stack is considered to have drifted if one or more of its resources differ from their expected template
* configurations. For more information, see Detecting
* Unregulated Configuration Changes to Stacks and Resources.
*
*
* Use DetectStackDrift
to detect drift on all supported resources for a given stack, or
* DetectStackResourceDrift to detect drift on individual resources.
*
*
* For a list of stack resources that currently support drift detection, see Resources that Support Drift Detection.
*
*
* DetectStackDrift
can take up to several minutes, depending on the number of resources contained
* within the stack. Use DescribeStackDriftDetectionStatus to monitor the progress of a detect stack drift
* operation. Once the drift detection operation has completed, use DescribeStackResourceDrifts to return
* drift information about the stack and its resources.
*
*
* When detecting drift on a stack, CloudFormation doesn't detect drift on any nested stacks belonging to that
* stack. Perform DetectStackDrift
directly on the nested stack itself.
*
*
* @param detectStackDriftRequest
* @return Result of the DetectStackDrift operation returned by the service.
* @sample AmazonCloudFormation.DetectStackDrift
* @see AWS API Documentation
*/
@Override
public DetectStackDriftResult detectStackDrift(DetectStackDriftRequest request) {
request = beforeClientExecution(request);
return executeDetectStackDrift(request);
}
@SdkInternalApi
final DetectStackDriftResult executeDetectStackDrift(DetectStackDriftRequest detectStackDriftRequest) {
ExecutionContext executionContext = createExecutionContext(detectStackDriftRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DetectStackDriftRequestMarshaller().marshall(super.beforeMarshalling(detectStackDriftRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DetectStackDrift");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DetectStackDriftResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about whether a resource's actual configuration differs, or has drifted, from its
* expected configuration, as defined in the stack template and any values specified as template parameters. This
* information includes actual and expected property values for resources in which CloudFormation detects drift.
* Only resource properties explicitly defined in the stack template are checked for drift. For more information
* about stack and resource drift, see Detecting
* Unregulated Configuration Changes to Stacks and Resources.
*
*
* Use DetectStackResourceDrift
to detect drift on individual resources, or DetectStackDrift to
* detect drift on all resources in a given stack that support drift detection.
*
*
* Resources that don't currently support drift detection can't be checked. For a list of resources that support
* drift detection, see Resources that Support Drift Detection.
*
*
* @param detectStackResourceDriftRequest
* @return Result of the DetectStackResourceDrift operation returned by the service.
* @sample AmazonCloudFormation.DetectStackResourceDrift
* @see AWS API Documentation
*/
@Override
public DetectStackResourceDriftResult detectStackResourceDrift(DetectStackResourceDriftRequest request) {
request = beforeClientExecution(request);
return executeDetectStackResourceDrift(request);
}
@SdkInternalApi
final DetectStackResourceDriftResult executeDetectStackResourceDrift(DetectStackResourceDriftRequest detectStackResourceDriftRequest) {
ExecutionContext executionContext = createExecutionContext(detectStackResourceDriftRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DetectStackResourceDriftRequestMarshaller().marshall(super.beforeMarshalling(detectStackResourceDriftRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DetectStackResourceDrift");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DetectStackResourceDriftResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Detect drift on a stack set. When CloudFormation performs drift detection on a stack set, it performs drift
* detection on the stack associated with each stack instance in the stack set. For more information, see How CloudFormation
* performs drift detection on a stack set.
*
*
* DetectStackSetDrift
returns the OperationId
of the stack set drift detection operation.
* Use this operation id with DescribeStackSetOperation to monitor the progress of the drift detection
* operation. The drift detection operation may take some time, depending on the number of stack instances included
* in the stack set, in addition to the number of resources included in each stack.
*
*
* Once the operation has completed, use the following actions to return drift information:
*
*
* -
*
* Use DescribeStackSet to return detailed information about the stack set, including detailed information
* about the last completed drift operation performed on the stack set. (Information about drift operations
* that are in progress isn't included.)
*
*
* -
*
* Use ListStackInstances to return a list of stack instances belonging to the stack set, including the drift
* status and last drift time checked of each instance.
*
*
* -
*
* Use DescribeStackInstance to return detailed information about a specific stack instance, including its
* drift status and last drift time checked.
*
*
*
*
* For more information about performing a drift detection operation on a stack set, see Detecting unmanaged
* changes in stack sets.
*
*
* You can only run a single drift detection operation on a given stack set at one time.
*
*
* To stop a drift detection stack set operation, use StopStackSetOperation.
*
*
* @param detectStackSetDriftRequest
* @return Result of the DetectStackSetDrift operation returned by the service.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @sample AmazonCloudFormation.DetectStackSetDrift
* @see AWS API Documentation
*/
@Override
public DetectStackSetDriftResult detectStackSetDrift(DetectStackSetDriftRequest request) {
request = beforeClientExecution(request);
return executeDetectStackSetDrift(request);
}
@SdkInternalApi
final DetectStackSetDriftResult executeDetectStackSetDrift(DetectStackSetDriftRequest detectStackSetDriftRequest) {
ExecutionContext executionContext = createExecutionContext(detectStackSetDriftRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new DetectStackSetDriftRequestMarshaller().marshall(super.beforeMarshalling(detectStackSetDriftRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "DetectStackSetDrift");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new DetectStackSetDriftResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the estimated monthly cost of a template. The return value is an Amazon Web Services Simple Monthly
* Calculator URL with a query string that describes the resources required to run the template.
*
*
* @param estimateTemplateCostRequest
* The input for an EstimateTemplateCost action.
* @return Result of the EstimateTemplateCost operation returned by the service.
* @sample AmazonCloudFormation.EstimateTemplateCost
* @see AWS API Documentation
*/
@Override
public EstimateTemplateCostResult estimateTemplateCost(EstimateTemplateCostRequest request) {
request = beforeClientExecution(request);
return executeEstimateTemplateCost(request);
}
@SdkInternalApi
final EstimateTemplateCostResult executeEstimateTemplateCost(EstimateTemplateCostRequest estimateTemplateCostRequest) {
ExecutionContext executionContext = createExecutionContext(estimateTemplateCostRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new EstimateTemplateCostRequestMarshaller().marshall(super.beforeMarshalling(estimateTemplateCostRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "EstimateTemplateCost");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new EstimateTemplateCostResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public EstimateTemplateCostResult estimateTemplateCost() {
return estimateTemplateCost(new EstimateTemplateCostRequest());
}
/**
*
* Updates a stack using the input information that was provided when the specified change set was created. After
* the call successfully completes, CloudFormation starts updating the stack. Use the DescribeStacks action
* to view the status of the update.
*
*
* When you execute a change set, CloudFormation deletes all other change sets associated with the stack because
* they aren't valid for the updated stack.
*
*
* If a stack policy is associated with the stack, CloudFormation enforces the policy during the update. You can't
* specify a temporary stack policy that overrides the current policy.
*
*
* To create a change set for the entire stack hierarchy, IncludeNestedStacks
must have been set to
* True
.
*
*
* @param executeChangeSetRequest
* The input for the ExecuteChangeSet action.
* @return Result of the ExecuteChangeSet operation returned by the service.
* @throws InvalidChangeSetStatusException
* The specified change set can't be used to update the stack. For example, the change set status might be
* CREATE_IN_PROGRESS
, or the stack status might be UPDATE_IN_PROGRESS
.
* @throws ChangeSetNotFoundException
* The specified change set name or ID doesn't exit. To view valid change sets for a stack, use the
* ListChangeSets
operation.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @sample AmazonCloudFormation.ExecuteChangeSet
* @see AWS API Documentation
*/
@Override
public ExecuteChangeSetResult executeChangeSet(ExecuteChangeSetRequest request) {
request = beforeClientExecution(request);
return executeExecuteChangeSet(request);
}
@SdkInternalApi
final ExecuteChangeSetResult executeExecuteChangeSet(ExecuteChangeSetRequest executeChangeSetRequest) {
ExecutionContext executionContext = createExecutionContext(executeChangeSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ExecuteChangeSetRequestMarshaller().marshall(super.beforeMarshalling(executeChangeSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ExecuteChangeSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ExecuteChangeSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the stack policy for a specified stack. If a stack doesn't have a policy, a null value is returned.
*
*
* @param getStackPolicyRequest
* The input for the GetStackPolicy action.
* @return Result of the GetStackPolicy operation returned by the service.
* @sample AmazonCloudFormation.GetStackPolicy
* @see AWS
* API Documentation
*/
@Override
public GetStackPolicyResult getStackPolicy(GetStackPolicyRequest request) {
request = beforeClientExecution(request);
return executeGetStackPolicy(request);
}
@SdkInternalApi
final GetStackPolicyResult executeGetStackPolicy(GetStackPolicyRequest getStackPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(getStackPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetStackPolicyRequestMarshaller().marshall(super.beforeMarshalling(getStackPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetStackPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetStackPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the template body for a specified stack. You can get the template for running or deleted stacks.
*
*
* For deleted stacks, GetTemplate
returns the template for up to 90 days after the stack has been
* deleted.
*
*
*
* If the template doesn't exist, a ValidationError
is returned.
*
*
*
* @param getTemplateRequest
* The input for a GetTemplate action.
* @return Result of the GetTemplate operation returned by the service.
* @throws ChangeSetNotFoundException
* The specified change set name or ID doesn't exit. To view valid change sets for a stack, use the
* ListChangeSets
operation.
* @sample AmazonCloudFormation.GetTemplate
* @see AWS API
* Documentation
*/
@Override
public GetTemplateResult getTemplate(GetTemplateRequest request) {
request = beforeClientExecution(request);
return executeGetTemplate(request);
}
@SdkInternalApi
final GetTemplateResult executeGetTemplate(GetTemplateRequest getTemplateRequest) {
ExecutionContext executionContext = createExecutionContext(getTemplateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetTemplateRequestMarshaller().marshall(super.beforeMarshalling(getTemplateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetTemplate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new GetTemplateResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns information about a new or existing template. The GetTemplateSummary
action is useful for
* viewing parameter information, such as default parameter values and parameter types, before you create or update
* a stack or stack set.
*
*
* You can use the GetTemplateSummary
action when you submit a template, or you can get template
* information for a stack set, or a running or deleted stack.
*
*
* For deleted stacks, GetTemplateSummary
returns the template information for up to 90 days after the
* stack has been deleted. If the template doesn't exist, a ValidationError
is returned.
*
*
* @param getTemplateSummaryRequest
* The input for the GetTemplateSummary action.
* @return Result of the GetTemplateSummary operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @sample AmazonCloudFormation.GetTemplateSummary
* @see AWS API Documentation
*/
@Override
public GetTemplateSummaryResult getTemplateSummary(GetTemplateSummaryRequest request) {
request = beforeClientExecution(request);
return executeGetTemplateSummary(request);
}
@SdkInternalApi
final GetTemplateSummaryResult executeGetTemplateSummary(GetTemplateSummaryRequest getTemplateSummaryRequest) {
ExecutionContext executionContext = createExecutionContext(getTemplateSummaryRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new GetTemplateSummaryRequestMarshaller().marshall(super.beforeMarshalling(getTemplateSummaryRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "GetTemplateSummary");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new GetTemplateSummaryResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public GetTemplateSummaryResult getTemplateSummary() {
return getTemplateSummary(new GetTemplateSummaryRequest());
}
/**
*
* Import existing stacks into a new stack sets. Use the stack import operation to import up to 10 stacks into a new
* stack set in the same account as the source stack or in a different administrator account and Region, by
* specifying the stack ID of the stack you intend to import.
*
*
* @param importStacksToStackSetRequest
* @return Result of the ImportStacksToStackSet operation returned by the service.
* @throws LimitExceededException
* The quota for the resource has already been reached.
*
* For information about resource and stack limitations, see CloudFormation quotas in the CloudFormation User Guide.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StackNotFoundException
* The specified stack ARN doesn't exist or stack doesn't exist corresponding to the ARN in input.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @sample AmazonCloudFormation.ImportStacksToStackSet
* @see AWS API Documentation
*/
@Override
public ImportStacksToStackSetResult importStacksToStackSet(ImportStacksToStackSetRequest request) {
request = beforeClientExecution(request);
return executeImportStacksToStackSet(request);
}
@SdkInternalApi
final ImportStacksToStackSetResult executeImportStacksToStackSet(ImportStacksToStackSetRequest importStacksToStackSetRequest) {
ExecutionContext executionContext = createExecutionContext(importStacksToStackSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ImportStacksToStackSetRequestMarshaller().marshall(super.beforeMarshalling(importStacksToStackSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ImportStacksToStackSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ImportStacksToStackSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the ID and status of each active change set for a stack. For example, CloudFormation lists change sets
* that are in the CREATE_IN_PROGRESS
or CREATE_PENDING
state.
*
*
* @param listChangeSetsRequest
* The input for the ListChangeSets action.
* @return Result of the ListChangeSets operation returned by the service.
* @sample AmazonCloudFormation.ListChangeSets
* @see AWS
* API Documentation
*/
@Override
public ListChangeSetsResult listChangeSets(ListChangeSetsRequest request) {
request = beforeClientExecution(request);
return executeListChangeSets(request);
}
@SdkInternalApi
final ListChangeSetsResult executeListChangeSets(ListChangeSetsRequest listChangeSetsRequest) {
ExecutionContext executionContext = createExecutionContext(listChangeSetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListChangeSetsRequestMarshaller().marshall(super.beforeMarshalling(listChangeSetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListChangeSets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListChangeSetsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all exported output values in the account and Region in which you call this action. Use this action to see
* the exported output values that you can import into other stacks. To import values, use the
* Fn::ImportValue function.
*
*
* For more information, see
* CloudFormation export stack output values.
*
*
* @param listExportsRequest
* @return Result of the ListExports operation returned by the service.
* @sample AmazonCloudFormation.ListExports
* @see AWS API
* Documentation
*/
@Override
public ListExportsResult listExports(ListExportsRequest request) {
request = beforeClientExecution(request);
return executeListExports(request);
}
@SdkInternalApi
final ListExportsResult executeListExports(ListExportsRequest listExportsRequest) {
ExecutionContext executionContext = createExecutionContext(listExportsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListExportsRequestMarshaller().marshall(super.beforeMarshalling(listExportsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListExports");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new ListExportsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Lists all stacks that are importing an exported output value. To modify or remove an exported output value, first
* use this action to see which stacks are using it. To see the exported output values in your account, see
* ListExports.
*
*
* For more information about importing an exported output value, see the Fn::ImportValue function.
*
*
* @param listImportsRequest
* @return Result of the ListImports operation returned by the service.
* @sample AmazonCloudFormation.ListImports
* @see AWS API
* Documentation
*/
@Override
public ListImportsResult listImports(ListImportsRequest request) {
request = beforeClientExecution(request);
return executeListImports(request);
}
@SdkInternalApi
final ListImportsResult executeListImports(ListImportsRequest listImportsRequest) {
ExecutionContext executionContext = createExecutionContext(listImportsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListImportsRequestMarshaller().marshall(super.beforeMarshalling(listImportsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListImports");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new ListImportsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns drift information for resources in a stack instance.
*
*
*
* ListStackInstanceResourceDrifts
returns drift information for the most recent drift detection
* operation. If an operation is in progress, it may only return partial results.
*
*
*
* @param listStackInstanceResourceDriftsRequest
* @return Result of the ListStackInstanceResourceDrifts operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws StackInstanceNotFoundException
* The specified stack instance doesn't exist.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @sample AmazonCloudFormation.ListStackInstanceResourceDrifts
* @see AWS API Documentation
*/
@Override
public ListStackInstanceResourceDriftsResult listStackInstanceResourceDrifts(ListStackInstanceResourceDriftsRequest request) {
request = beforeClientExecution(request);
return executeListStackInstanceResourceDrifts(request);
}
@SdkInternalApi
final ListStackInstanceResourceDriftsResult executeListStackInstanceResourceDrifts(
ListStackInstanceResourceDriftsRequest listStackInstanceResourceDriftsRequest) {
ExecutionContext executionContext = createExecutionContext(listStackInstanceResourceDriftsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListStackInstanceResourceDriftsRequestMarshaller().marshall(super.beforeMarshalling(listStackInstanceResourceDriftsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListStackInstanceResourceDrifts");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListStackInstanceResourceDriftsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns summary information about stack instances that are associated with the specified stack set. You can
* filter for stack instances that are associated with a specific Amazon Web Services account name or Region, or
* that have a specific status.
*
*
* @param listStackInstancesRequest
* @return Result of the ListStackInstances operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @sample AmazonCloudFormation.ListStackInstances
* @see AWS API Documentation
*/
@Override
public ListStackInstancesResult listStackInstances(ListStackInstancesRequest request) {
request = beforeClientExecution(request);
return executeListStackInstances(request);
}
@SdkInternalApi
final ListStackInstancesResult executeListStackInstances(ListStackInstancesRequest listStackInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(listStackInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListStackInstancesRequestMarshaller().marshall(super.beforeMarshalling(listStackInstancesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListStackInstances");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListStackInstancesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns descriptions of all resources of the specified stack.
*
*
* For deleted stacks, ListStackResources returns resource information for up to 90 days after the stack has been
* deleted.
*
*
* @param listStackResourcesRequest
* The input for the ListStackResource action.
* @return Result of the ListStackResources operation returned by the service.
* @sample AmazonCloudFormation.ListStackResources
* @see AWS API Documentation
*/
@Override
public ListStackResourcesResult listStackResources(ListStackResourcesRequest request) {
request = beforeClientExecution(request);
return executeListStackResources(request);
}
@SdkInternalApi
final ListStackResourcesResult executeListStackResources(ListStackResourcesRequest listStackResourcesRequest) {
ExecutionContext executionContext = createExecutionContext(listStackResourcesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListStackResourcesRequestMarshaller().marshall(super.beforeMarshalling(listStackResourcesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListStackResources");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListStackResourcesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns summary information about the results of a stack set operation.
*
*
* @param listStackSetOperationResultsRequest
* @return Result of the ListStackSetOperationResults operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @sample AmazonCloudFormation.ListStackSetOperationResults
* @see AWS API Documentation
*/
@Override
public ListStackSetOperationResultsResult listStackSetOperationResults(ListStackSetOperationResultsRequest request) {
request = beforeClientExecution(request);
return executeListStackSetOperationResults(request);
}
@SdkInternalApi
final ListStackSetOperationResultsResult executeListStackSetOperationResults(ListStackSetOperationResultsRequest listStackSetOperationResultsRequest) {
ExecutionContext executionContext = createExecutionContext(listStackSetOperationResultsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListStackSetOperationResultsRequestMarshaller().marshall(super.beforeMarshalling(listStackSetOperationResultsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListStackSetOperationResults");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListStackSetOperationResultsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns summary information about operations performed on a stack set.
*
*
* @param listStackSetOperationsRequest
* @return Result of the ListStackSetOperations operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @sample AmazonCloudFormation.ListStackSetOperations
* @see AWS API Documentation
*/
@Override
public ListStackSetOperationsResult listStackSetOperations(ListStackSetOperationsRequest request) {
request = beforeClientExecution(request);
return executeListStackSetOperations(request);
}
@SdkInternalApi
final ListStackSetOperationsResult executeListStackSetOperations(ListStackSetOperationsRequest listStackSetOperationsRequest) {
ExecutionContext executionContext = createExecutionContext(listStackSetOperationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListStackSetOperationsRequestMarshaller().marshall(super.beforeMarshalling(listStackSetOperationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListStackSetOperations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListStackSetOperationsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns summary information about stack sets that are associated with the user.
*
*
* -
*
* [Self-managed permissions] If you set the CallAs
parameter to SELF
while signed in to
* your Amazon Web Services account, ListStackSets
returns all self-managed stack sets in your Amazon
* Web Services account.
*
*
* -
*
* [Service-managed permissions] If you set the CallAs
parameter to SELF
while signed in
* to the organization's management account, ListStackSets
returns all stack sets in the management
* account.
*
*
* -
*
* [Service-managed permissions] If you set the CallAs
parameter to DELEGATED_ADMIN
while
* signed in to your member account, ListStackSets
returns all stack sets with service-managed
* permissions in the management account.
*
*
*
*
* @param listStackSetsRequest
* @return Result of the ListStackSets operation returned by the service.
* @sample AmazonCloudFormation.ListStackSets
* @see AWS
* API Documentation
*/
@Override
public ListStackSetsResult listStackSets(ListStackSetsRequest request) {
request = beforeClientExecution(request);
return executeListStackSets(request);
}
@SdkInternalApi
final ListStackSetsResult executeListStackSets(ListStackSetsRequest listStackSetsRequest) {
ExecutionContext executionContext = createExecutionContext(listStackSetsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListStackSetsRequestMarshaller().marshall(super.beforeMarshalling(listStackSetsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListStackSets");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new ListStackSetsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns the summary information for stacks whose status matches the specified StackStatusFilter. Summary
* information for stacks that have been deleted is kept for 90 days after the stack is deleted. If no
* StackStatusFilter is specified, summary information for all stacks is returned (including existing stacks and
* stacks that have been deleted).
*
*
* @param listStacksRequest
* The input for ListStacks action.
* @return Result of the ListStacks operation returned by the service.
* @sample AmazonCloudFormation.ListStacks
* @see AWS API
* Documentation
*/
@Override
public ListStacksResult listStacks(ListStacksRequest request) {
request = beforeClientExecution(request);
return executeListStacks(request);
}
@SdkInternalApi
final ListStacksResult executeListStacks(ListStacksRequest listStacksRequest) {
ExecutionContext executionContext = createExecutionContext(listStacksRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListStacksRequestMarshaller().marshall(super.beforeMarshalling(listStacksRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListStacks");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new ListStacksResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
@Override
public ListStacksResult listStacks() {
return listStacks(new ListStacksRequest());
}
/**
*
* Returns a list of registration tokens for the specified extension(s).
*
*
* @param listTypeRegistrationsRequest
* @return Result of the ListTypeRegistrations operation returned by the service.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @sample AmazonCloudFormation.ListTypeRegistrations
* @see AWS API Documentation
*/
@Override
public ListTypeRegistrationsResult listTypeRegistrations(ListTypeRegistrationsRequest request) {
request = beforeClientExecution(request);
return executeListTypeRegistrations(request);
}
@SdkInternalApi
final ListTypeRegistrationsResult executeListTypeRegistrations(ListTypeRegistrationsRequest listTypeRegistrationsRequest) {
ExecutionContext executionContext = createExecutionContext(listTypeRegistrationsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTypeRegistrationsRequestMarshaller().marshall(super.beforeMarshalling(listTypeRegistrationsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTypeRegistrations");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListTypeRegistrationsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns summary information about the versions of an extension.
*
*
* @param listTypeVersionsRequest
* @return Result of the ListTypeVersions operation returned by the service.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @sample AmazonCloudFormation.ListTypeVersions
* @see AWS API Documentation
*/
@Override
public ListTypeVersionsResult listTypeVersions(ListTypeVersionsRequest request) {
request = beforeClientExecution(request);
return executeListTypeVersions(request);
}
@SdkInternalApi
final ListTypeVersionsResult executeListTypeVersions(ListTypeVersionsRequest listTypeVersionsRequest) {
ExecutionContext executionContext = createExecutionContext(listTypeVersionsRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTypeVersionsRequestMarshaller().marshall(super.beforeMarshalling(listTypeVersionsRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTypeVersions");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ListTypeVersionsResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Returns summary information about extension that have been registered with CloudFormation.
*
*
* @param listTypesRequest
* @return Result of the ListTypes operation returned by the service.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @sample AmazonCloudFormation.ListTypes
* @see AWS API
* Documentation
*/
@Override
public ListTypesResult listTypes(ListTypesRequest request) {
request = beforeClientExecution(request);
return executeListTypes(request);
}
@SdkInternalApi
final ListTypesResult executeListTypes(ListTypesRequest listTypesRequest) {
ExecutionContext executionContext = createExecutionContext(listTypesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ListTypesRequestMarshaller().marshall(super.beforeMarshalling(listTypesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ListTypes");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new ListTypesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Publishes the specified extension to the CloudFormation registry as a public extension in this Region. Public
* extensions are available for use by all CloudFormation users. For more information about publishing extensions,
* see Publishing
* extensions to make them available for public use in the CloudFormation CLI User Guide.
*
*
* To publish an extension, you must be registered as a publisher with CloudFormation. For more information, see
* RegisterPublisher.
*
*
* @param publishTypeRequest
* @return Result of the PublishType operation returned by the service.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws TypeNotFoundException
* The specified extension doesn't exist in the CloudFormation registry.
* @sample AmazonCloudFormation.PublishType
* @see AWS API
* Documentation
*/
@Override
public PublishTypeResult publishType(PublishTypeRequest request) {
request = beforeClientExecution(request);
return executePublishType(request);
}
@SdkInternalApi
final PublishTypeResult executePublishType(PublishTypeRequest publishTypeRequest) {
ExecutionContext executionContext = createExecutionContext(publishTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new PublishTypeRequestMarshaller().marshall(super.beforeMarshalling(publishTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "PublishType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new PublishTypeResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Reports progress of a resource handler to CloudFormation.
*
*
* Reserved for use by the CloudFormation CLI. Don't use this API in your code.
*
*
* @param recordHandlerProgressRequest
* @return Result of the RecordHandlerProgress operation returned by the service.
* @throws InvalidStateTransitionException
* Error reserved for use by the CloudFormation CLI. CloudFormation doesn't return this error to users.
* @throws OperationStatusCheckFailedException
* Error reserved for use by the CloudFormation CLI. CloudFormation doesn't return this error to users.
* @sample AmazonCloudFormation.RecordHandlerProgress
* @see AWS API Documentation
*/
@Override
public RecordHandlerProgressResult recordHandlerProgress(RecordHandlerProgressRequest request) {
request = beforeClientExecution(request);
return executeRecordHandlerProgress(request);
}
@SdkInternalApi
final RecordHandlerProgressResult executeRecordHandlerProgress(RecordHandlerProgressRequest recordHandlerProgressRequest) {
ExecutionContext executionContext = createExecutionContext(recordHandlerProgressRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RecordHandlerProgressRequestMarshaller().marshall(super.beforeMarshalling(recordHandlerProgressRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RecordHandlerProgress");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new RecordHandlerProgressResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Registers your account as a publisher of public extensions in the CloudFormation registry. Public extensions are
* available for use by all CloudFormation users. This publisher ID applies to your account in all Amazon Web
* Services Regions.
*
*
* For information about requirements for registering as a public extension publisher, see Registering your account to publish CloudFormation extensions in the CloudFormation CLI User Guide.
*
*
*
* @param registerPublisherRequest
* @return Result of the RegisterPublisher operation returned by the service.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @sample AmazonCloudFormation.RegisterPublisher
* @see AWS API Documentation
*/
@Override
public RegisterPublisherResult registerPublisher(RegisterPublisherRequest request) {
request = beforeClientExecution(request);
return executeRegisterPublisher(request);
}
@SdkInternalApi
final RegisterPublisherResult executeRegisterPublisher(RegisterPublisherRequest registerPublisherRequest) {
ExecutionContext executionContext = createExecutionContext(registerPublisherRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RegisterPublisherRequestMarshaller().marshall(super.beforeMarshalling(registerPublisherRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RegisterPublisher");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new RegisterPublisherResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Registers an extension with the CloudFormation service. Registering an extension makes it available for use in
* CloudFormation templates in your Amazon Web Services account, and includes:
*
*
* -
*
* Validating the extension schema.
*
*
* -
*
* Determining which handlers, if any, have been specified for the extension.
*
*
* -
*
* Making the extension available for use in your account.
*
*
*
*
* For more information about how to develop extensions and ready them for registration, see Creating Resource
* Providers in the CloudFormation CLI User Guide.
*
*
* You can have a maximum of 50 resource extension versions registered at a time. This maximum is per account and
* per Region. Use DeregisterType to deregister specific extension versions if necessary.
*
*
* Once you have initiated a registration request using RegisterType, you can use
* DescribeTypeRegistration to monitor the progress of the registration request.
*
*
* Once you have registered a private extension in your account and Region, use SetTypeConfiguration to specify configuration properties for the extension. For more information, see Configuring extensions at the account level in the CloudFormation User Guide.
*
*
* @param registerTypeRequest
* @return Result of the RegisterType operation returned by the service.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @sample AmazonCloudFormation.RegisterType
* @see AWS
* API Documentation
*/
@Override
public RegisterTypeResult registerType(RegisterTypeRequest request) {
request = beforeClientExecution(request);
return executeRegisterType(request);
}
@SdkInternalApi
final RegisterTypeResult executeRegisterType(RegisterTypeRequest registerTypeRequest) {
ExecutionContext executionContext = createExecutionContext(registerTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RegisterTypeRequestMarshaller().marshall(super.beforeMarshalling(registerTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RegisterType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new RegisterTypeResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* When specifying RollbackStack
, you preserve the state of previously provisioned resources when an
* operation fails. You can check the status of the stack through the DescribeStacks operation.
*
*
* Rolls back the specified stack to the last known stable state from CREATE_FAILED
or
* UPDATE_FAILED
stack statuses.
*
*
* This operation will delete a stack if it doesn't contain a last known stable state. A last known stable state
* includes any status in a *_COMPLETE
. This includes the following stack statuses.
*
*
* -
*
* CREATE_COMPLETE
*
*
* -
*
* UPDATE_COMPLETE
*
*
* -
*
* UPDATE_ROLLBACK_COMPLETE
*
*
* -
*
* IMPORT_COMPLETE
*
*
* -
*
* IMPORT_ROLLBACK_COMPLETE
*
*
*
*
* @param rollbackStackRequest
* @return Result of the RollbackStack operation returned by the service.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @sample AmazonCloudFormation.RollbackStack
* @see AWS
* API Documentation
*/
@Override
public RollbackStackResult rollbackStack(RollbackStackRequest request) {
request = beforeClientExecution(request);
return executeRollbackStack(request);
}
@SdkInternalApi
final RollbackStackResult executeRollbackStack(RollbackStackRequest rollbackStackRequest) {
ExecutionContext executionContext = createExecutionContext(rollbackStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new RollbackStackRequestMarshaller().marshall(super.beforeMarshalling(rollbackStackRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "RollbackStack");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new RollbackStackResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Sets a stack policy for a specified stack.
*
*
* @param setStackPolicyRequest
* The input for the SetStackPolicy action.
* @return Result of the SetStackPolicy operation returned by the service.
* @sample AmazonCloudFormation.SetStackPolicy
* @see AWS
* API Documentation
*/
@Override
public SetStackPolicyResult setStackPolicy(SetStackPolicyRequest request) {
request = beforeClientExecution(request);
return executeSetStackPolicy(request);
}
@SdkInternalApi
final SetStackPolicyResult executeSetStackPolicy(SetStackPolicyRequest setStackPolicyRequest) {
ExecutionContext executionContext = createExecutionContext(setStackPolicyRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetStackPolicyRequestMarshaller().marshall(super.beforeMarshalling(setStackPolicyRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SetStackPolicy");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new SetStackPolicyResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Specifies the configuration data for a registered CloudFormation extension, in the given account and Region.
*
*
* To view the current configuration data for an extension, refer to the ConfigurationSchema
element of
* DescribeType.
* For more information, see Configuring extensions at the account level in the CloudFormation User Guide.
*
*
*
* It's strongly recommended that you use dynamic references to restrict sensitive configuration definitions, such
* as third-party credentials. For more details on dynamic references, see Using dynamic references to specify template values in the
* CloudFormation User Guide.
*
*
*
* @param setTypeConfigurationRequest
* @return Result of the SetTypeConfiguration operation returned by the service.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws TypeNotFoundException
* The specified extension doesn't exist in the CloudFormation registry.
* @sample AmazonCloudFormation.SetTypeConfiguration
* @see AWS API Documentation
*/
@Override
public SetTypeConfigurationResult setTypeConfiguration(SetTypeConfigurationRequest request) {
request = beforeClientExecution(request);
return executeSetTypeConfiguration(request);
}
@SdkInternalApi
final SetTypeConfigurationResult executeSetTypeConfiguration(SetTypeConfigurationRequest setTypeConfigurationRequest) {
ExecutionContext executionContext = createExecutionContext(setTypeConfigurationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetTypeConfigurationRequestMarshaller().marshall(super.beforeMarshalling(setTypeConfigurationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SetTypeConfiguration");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new SetTypeConfigurationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Specify the default version of an extension. The default version of an extension will be used in CloudFormation
* operations.
*
*
* @param setTypeDefaultVersionRequest
* @return Result of the SetTypeDefaultVersion operation returned by the service.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws TypeNotFoundException
* The specified extension doesn't exist in the CloudFormation registry.
* @sample AmazonCloudFormation.SetTypeDefaultVersion
* @see AWS API Documentation
*/
@Override
public SetTypeDefaultVersionResult setTypeDefaultVersion(SetTypeDefaultVersionRequest request) {
request = beforeClientExecution(request);
return executeSetTypeDefaultVersion(request);
}
@SdkInternalApi
final SetTypeDefaultVersionResult executeSetTypeDefaultVersion(SetTypeDefaultVersionRequest setTypeDefaultVersionRequest) {
ExecutionContext executionContext = createExecutionContext(setTypeDefaultVersionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SetTypeDefaultVersionRequestMarshaller().marshall(super.beforeMarshalling(setTypeDefaultVersionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SetTypeDefaultVersion");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new SetTypeDefaultVersionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Sends a signal to the specified resource with a success or failure status. You can use the
* SignalResource
operation in conjunction with a creation policy or update policy. CloudFormation
* doesn't proceed with a stack creation or update until resources receive the required number of signals or the
* timeout period is exceeded. The SignalResource
operation is useful in cases where you want to send
* signals from anywhere other than an Amazon EC2 instance.
*
*
* @param signalResourceRequest
* The input for the SignalResource action.
* @return Result of the SignalResource operation returned by the service.
* @sample AmazonCloudFormation.SignalResource
* @see AWS
* API Documentation
*/
@Override
public SignalResourceResult signalResource(SignalResourceRequest request) {
request = beforeClientExecution(request);
return executeSignalResource(request);
}
@SdkInternalApi
final SignalResourceResult executeSignalResource(SignalResourceRequest signalResourceRequest) {
ExecutionContext executionContext = createExecutionContext(signalResourceRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new SignalResourceRequestMarshaller().marshall(super.beforeMarshalling(signalResourceRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "SignalResource");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new SignalResourceResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Stops an in-progress operation on a stack set and its associated stack instances. StackSets will cancel all the
* unstarted stack instance deployments and wait for those are in-progress to complete.
*
*
* @param stopStackSetOperationRequest
* @return Result of the StopStackSetOperation operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationNotFoundException
* The specified ID refers to an operation that doesn't exist.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @sample AmazonCloudFormation.StopStackSetOperation
* @see AWS API Documentation
*/
@Override
public StopStackSetOperationResult stopStackSetOperation(StopStackSetOperationRequest request) {
request = beforeClientExecution(request);
return executeStopStackSetOperation(request);
}
@SdkInternalApi
final StopStackSetOperationResult executeStopStackSetOperation(StopStackSetOperationRequest stopStackSetOperationRequest) {
ExecutionContext executionContext = createExecutionContext(stopStackSetOperationRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new StopStackSetOperationRequestMarshaller().marshall(super.beforeMarshalling(stopStackSetOperationRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "StopStackSetOperation");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new StopStackSetOperationResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Tests a registered extension to make sure it meets all necessary requirements for being published in the
* CloudFormation registry.
*
*
* -
*
* For resource types, this includes passing all contracts tests defined for the type.
*
*
* -
*
* For modules, this includes determining if the module's model meets all necessary requirements.
*
*
*
*
* For more information, see Testing your public extension prior to publishing in the CloudFormation CLI User Guide.
*
*
* If you don't specify a version, CloudFormation uses the default version of the extension in your account and
* Region for testing.
*
*
* To perform testing, CloudFormation assumes the execution role specified when the type was registered. For more
* information, see RegisterType.
*
*
* Once you've initiated testing on an extension using TestType
, you can pass the returned
* TypeVersionArn
into DescribeType
* to monitor the current test status and test status description for the extension.
*
*
* An extension must have a test status of PASSED
before it can be published. For more information, see
* Publishing
* extensions to make them available for public use in the CloudFormation CLI User Guide.
*
*
* @param testTypeRequest
* @return Result of the TestType operation returned by the service.
* @throws CFNRegistryException
* An error occurred during a CloudFormation registry operation.
* @throws TypeNotFoundException
* The specified extension doesn't exist in the CloudFormation registry.
* @sample AmazonCloudFormation.TestType
* @see AWS API
* Documentation
*/
@Override
public TestTypeResult testType(TestTypeRequest request) {
request = beforeClientExecution(request);
return executeTestType(request);
}
@SdkInternalApi
final TestTypeResult executeTestType(TestTypeRequest testTypeRequest) {
ExecutionContext executionContext = createExecutionContext(testTypeRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new TestTypeRequestMarshaller().marshall(super.beforeMarshalling(testTypeRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "TestType");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new TestTypeResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates a stack as specified in the template. After the call completes successfully, the stack update starts. You
* can check the status of the stack through the DescribeStacks action.
*
*
* To get a copy of the template for an existing stack, you can use the GetTemplate action.
*
*
* For more information about creating an update template, updating a stack, and monitoring the progress of the
* update, see Updating a
* Stack.
*
*
* @param updateStackRequest
* The input for an UpdateStack action.
* @return Result of the UpdateStack operation returned by the service.
* @throws InsufficientCapabilitiesException
* The template contains resources with capabilities that weren't specified in the Capabilities parameter.
* @throws TokenAlreadyExistsException
* A client request token already exists.
* @sample AmazonCloudFormation.UpdateStack
* @see AWS API
* Documentation
*/
@Override
public UpdateStackResult updateStack(UpdateStackRequest request) {
request = beforeClientExecution(request);
return executeUpdateStack(request);
}
@SdkInternalApi
final UpdateStackResult executeUpdateStack(UpdateStackRequest updateStackRequest) {
ExecutionContext executionContext = createExecutionContext(updateStackRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateStackRequestMarshaller().marshall(super.beforeMarshalling(updateStackRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateStack");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(new UpdateStackResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the parameter values for stack instances for the specified accounts, within the specified Amazon Web
* Services Regions. A stack instance refers to a stack in a specific account and Region.
*
*
* You can only update stack instances in Amazon Web Services Regions and accounts where they already exist; to
* create additional stack instances, use CreateStackInstances.
*
*
* During stack set updates, any parameters overridden for a stack instance aren't updated, but retain their
* overridden value.
*
*
* You can only update the parameter values that are specified in the stack set; to add or delete a parameter
* itself, use UpdateStackSet
* to update the stack set template. If you add a parameter to a template, before you can override the
* parameter value specified in the stack set you must first use UpdateStackSet to update all stack instances with the updated template and parameter value specified in the
* stack set. Once a stack instance has been updated with the new parameter, you can then override the parameter
* value using UpdateStackInstances
.
*
*
* @param updateStackInstancesRequest
* @return Result of the UpdateStackInstances operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws StackInstanceNotFoundException
* The specified stack instance doesn't exist.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @sample AmazonCloudFormation.UpdateStackInstances
* @see AWS API Documentation
*/
@Override
public UpdateStackInstancesResult updateStackInstances(UpdateStackInstancesRequest request) {
request = beforeClientExecution(request);
return executeUpdateStackInstances(request);
}
@SdkInternalApi
final UpdateStackInstancesResult executeUpdateStackInstances(UpdateStackInstancesRequest updateStackInstancesRequest) {
ExecutionContext executionContext = createExecutionContext(updateStackInstancesRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateStackInstancesRequestMarshaller().marshall(super.beforeMarshalling(updateStackInstancesRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateStackInstances");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new UpdateStackInstancesResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates the stack set, and associated stack instances in the specified accounts and Amazon Web Services Regions.
*
*
* Even if the stack set operation created by updating the stack set fails (completely or partially, below or above
* a specified failure tolerance), the stack set is updated with your changes. Subsequent
* CreateStackInstances calls on the specified stack set use the updated stack set.
*
*
* @param updateStackSetRequest
* @return Result of the UpdateStackSet operation returned by the service.
* @throws StackSetNotFoundException
* The specified stack set doesn't exist.
* @throws OperationInProgressException
* Another operation is currently in progress for this stack set. Only one operation can be performed for a
* stack set at a given time.
* @throws OperationIdAlreadyExistsException
* The specified operation ID already exists.
* @throws StaleRequestException
* Another operation has been performed on this stack set since the specified operation was performed.
* @throws InvalidOperationException
* The specified operation isn't valid.
* @throws StackInstanceNotFoundException
* The specified stack instance doesn't exist.
* @sample AmazonCloudFormation.UpdateStackSet
* @see AWS
* API Documentation
*/
@Override
public UpdateStackSetResult updateStackSet(UpdateStackSetRequest request) {
request = beforeClientExecution(request);
return executeUpdateStackSet(request);
}
@SdkInternalApi
final UpdateStackSetResult executeUpdateStackSet(UpdateStackSetRequest updateStackSetRequest) {
ExecutionContext executionContext = createExecutionContext(updateStackSetRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateStackSetRequestMarshaller().marshall(super.beforeMarshalling(updateStackSetRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateStackSet");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new UpdateStackSetResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Updates termination protection for the specified stack. If a user attempts to delete a stack with termination
* protection enabled, the operation fails and the stack remains unchanged. For more information, see Protecting a
* Stack From Being Deleted in the CloudFormation User Guide.
*
*
* For nested
* stacks, termination protection is set on the root stack and can't be changed directly on the nested stack.
*
*
* @param updateTerminationProtectionRequest
* @return Result of the UpdateTerminationProtection operation returned by the service.
* @sample AmazonCloudFormation.UpdateTerminationProtection
* @see AWS API Documentation
*/
@Override
public UpdateTerminationProtectionResult updateTerminationProtection(UpdateTerminationProtectionRequest request) {
request = beforeClientExecution(request);
return executeUpdateTerminationProtection(request);
}
@SdkInternalApi
final UpdateTerminationProtectionResult executeUpdateTerminationProtection(UpdateTerminationProtectionRequest updateTerminationProtectionRequest) {
ExecutionContext executionContext = createExecutionContext(updateTerminationProtectionRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new UpdateTerminationProtectionRequestMarshaller().marshall(super.beforeMarshalling(updateTerminationProtectionRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "UpdateTerminationProtection");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new UpdateTerminationProtectionResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
*
* Validates a specified template. CloudFormation first checks if the template is valid JSON. If it isn't,
* CloudFormation checks if the template is valid YAML. If both these checks fail, CloudFormation returns a template
* validation error.
*
*
* @param validateTemplateRequest
* The input for ValidateTemplate action.
* @return Result of the ValidateTemplate operation returned by the service.
* @sample AmazonCloudFormation.ValidateTemplate
* @see AWS API Documentation
*/
@Override
public ValidateTemplateResult validateTemplate(ValidateTemplateRequest request) {
request = beforeClientExecution(request);
return executeValidateTemplate(request);
}
@SdkInternalApi
final ValidateTemplateResult executeValidateTemplate(ValidateTemplateRequest validateTemplateRequest) {
ExecutionContext executionContext = createExecutionContext(validateTemplateRequest);
AWSRequestMetrics awsRequestMetrics = executionContext.getAwsRequestMetrics();
awsRequestMetrics.startEvent(Field.ClientExecuteTime);
Request request = null;
Response response = null;
try {
awsRequestMetrics.startEvent(Field.RequestMarshallTime);
try {
request = new ValidateTemplateRequestMarshaller().marshall(super.beforeMarshalling(validateTemplateRequest));
// Binds the request metrics to the current request.
request.setAWSRequestMetrics(awsRequestMetrics);
request.addHandlerContext(HandlerContextKey.CLIENT_ENDPOINT, endpoint);
request.addHandlerContext(HandlerContextKey.ENDPOINT_OVERRIDDEN, isEndpointOverridden());
request.addHandlerContext(HandlerContextKey.SIGNING_REGION, getSigningRegion());
request.addHandlerContext(HandlerContextKey.SERVICE_ID, "CloudFormation");
request.addHandlerContext(HandlerContextKey.OPERATION_NAME, "ValidateTemplate");
request.addHandlerContext(HandlerContextKey.ADVANCED_CONFIG, advancedConfig);
} finally {
awsRequestMetrics.endEvent(Field.RequestMarshallTime);
}
StaxResponseHandler responseHandler = new StaxResponseHandler(
new ValidateTemplateResultStaxUnmarshaller());
response = invoke(request, responseHandler, executionContext);
return response.getAwsResponse();
} finally {
endClientExecution(awsRequestMetrics, request, response);
}
}
/**
* Returns additional metadata for a previously executed successful, request, typically used for debugging issues
* where a service isn't acting as expected. This data isn't considered part of the result data returned by an
* operation, so it's available through this separate, diagnostic interface.
*
* Response metadata is only cached for a limited period of time, so if you need to access this extra diagnostic
* information for an executed request, you should use this method to retrieve it as soon as possible after
* executing the request.
*
* @param request
* The originally executed request
*
* @return The response metadata for the specified request, or null if none is available.
*/
public ResponseMetadata getCachedResponseMetadata(AmazonWebServiceRequest request) {
return client.getResponseMetadataForRequest(request);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext) {
return invoke(request, responseHandler, executionContext, null, null);
}
/**
* Normal invoke with authentication. Credentials are required and may be overriden at the request level.
**/
private Response invoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI cachedEndpoint, URI uriFromEndpointTrait) {
executionContext.setCredentialsProvider(CredentialUtils.getCredentialsProvider(request.getOriginalRequest(), awsCredentialsProvider));
return doInvoke(request, responseHandler, executionContext, cachedEndpoint, uriFromEndpointTrait);
}
/**
* Invoke with no authentication. Credentials are not required and any credentials set on the client or request will
* be ignored for this operation.
**/
private Response anonymousInvoke(Request request,
HttpResponseHandler> responseHandler, ExecutionContext executionContext) {
return doInvoke(request, responseHandler, executionContext, null, null);
}
/**
* Invoke the request using the http client. Assumes credentials (or lack thereof) have been configured in the
* ExecutionContext beforehand.
**/
private Response doInvoke(Request request, HttpResponseHandler> responseHandler,
ExecutionContext executionContext, URI discoveredEndpoint, URI uriFromEndpointTrait) {
if (discoveredEndpoint != null) {
request.setEndpoint(discoveredEndpoint);
request.getOriginalRequest().getRequestClientOptions().appendUserAgent("endpoint-discovery");
} else if (uriFromEndpointTrait != null) {
request.setEndpoint(uriFromEndpointTrait);
} else {
request.setEndpoint(endpoint);
}
request.setTimeOffset(timeOffset);
DefaultErrorResponseHandler errorResponseHandler = new DefaultErrorResponseHandler(exceptionUnmarshallersMap, defaultUnmarshaller);
return client.execute(request, responseHandler, errorResponseHandler, executionContext);
}
@Override
public AmazonCloudFormationWaiters waiters() {
if (waiters == null) {
synchronized (this) {
if (waiters == null) {
waiters = new AmazonCloudFormationWaiters(this);
}
}
}
return waiters;
}
@Override
public void shutdown() {
super.shutdown();
if (waiters != null) {
waiters.shutdown();
}
}
}