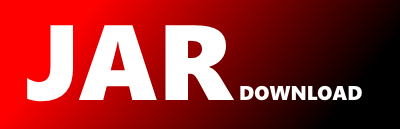
com.amazonaws.services.cloudformation.model.StackSetOperationPreferences Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2018-2023 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudformation.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* The user-specified preferences for how CloudFormation performs a stack set operation.
*
*
* For more information about maximum concurrent accounts and failure tolerance, see Stack set operation options.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StackSetOperationPreferences implements Serializable, Cloneable {
/**
*
* The concurrency type of deploying StackSets operations in Regions, could be in parallel or one Region at a time.
*
*/
private String regionConcurrencyType;
/**
*
* The order of the Regions where you want to perform the stack operation.
*
*/
private com.amazonaws.internal.SdkInternalList regionOrder;
/**
*
* The number of accounts, per Region, for which this operation can fail before CloudFormation stops the operation
* in that Region. If the operation is stopped in a Region, CloudFormation doesn't attempt the operation in any
* subsequent Regions.
*
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
(but not both).
*
*
* By default, 0
is specified.
*
*/
private Integer failureToleranceCount;
/**
*
* The percentage of accounts, per Region, for which this stack operation can fail before CloudFormation stops the
* operation in that Region. If the operation is stopped in a Region, CloudFormation doesn't attempt the operation
* in any subsequent Regions.
*
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds down to
* the next whole number.
*
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
, but not both.
*
*
* By default, 0
is specified.
*
*/
private Integer failureTolerancePercentage;
/**
*
* The maximum number of accounts in which to perform this operation at one time. This can depend on the value of
* FailureToleranceCount
depending on your ConcurrencyMode
.
* MaxConcurrentCount
is at most one more than the FailureToleranceCount
if you're using
* STRICT_FAILURE_TOLERANCE
.
*
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under certain
* circumstances the actual number of accounts acted upon concurrently may be lower due to service throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or MaxConcurrentPercentage
, but
* not both.
*
*
* By default, 1
is specified.
*
*/
private Integer maxConcurrentCount;
/**
*
* The maximum percentage of accounts in which to perform this operation at one time.
*
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds down to the next
* whole number. This is true except in cases where rounding down would result is zero. In this case, CloudFormation
* sets the number as one instead.
*
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under certain
* circumstances the actual number of accounts acted upon concurrently may be lower due to service throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or MaxConcurrentPercentage
, but
* not both.
*
*
* By default, 1
is specified.
*
*/
private Integer maxConcurrentPercentage;
/**
*
* Specifies how the concurrency level behaves during the operation execution.
*
*
* -
*
* STRICT_FAILURE_TOLERANCE
: This option dynamically lowers the concurrency level to ensure the number
* of failed accounts never exceeds the value of FailureToleranceCount
+1. The initial actual
* concurrency is set to the lower of either the value of the MaxConcurrentCount
, or the value of
* MaxConcurrentCount
+1. The actual concurrency is then reduced proportionally by the number of
* failures. This is the default behavior.
*
*
* If failure tolerance or Maximum concurrent accounts are set to percentages, the behavior is similar.
*
*
* -
*
* SOFT_FAILURE_TOLERANCE
: This option decouples FailureToleranceCount
from the actual
* concurrency. This allows stack set operations to run at the concurrency level set by the
* MaxConcurrentCount
value, or MaxConcurrentPercentage
, regardless of the number of
* failures.
*
*
*
*/
private String concurrencyMode;
/**
*
* The concurrency type of deploying StackSets operations in Regions, could be in parallel or one Region at a time.
*
*
* @param regionConcurrencyType
* The concurrency type of deploying StackSets operations in Regions, could be in parallel or one Region at a
* time.
* @see RegionConcurrencyType
*/
public void setRegionConcurrencyType(String regionConcurrencyType) {
this.regionConcurrencyType = regionConcurrencyType;
}
/**
*
* The concurrency type of deploying StackSets operations in Regions, could be in parallel or one Region at a time.
*
*
* @return The concurrency type of deploying StackSets operations in Regions, could be in parallel or one Region at
* a time.
* @see RegionConcurrencyType
*/
public String getRegionConcurrencyType() {
return this.regionConcurrencyType;
}
/**
*
* The concurrency type of deploying StackSets operations in Regions, could be in parallel or one Region at a time.
*
*
* @param regionConcurrencyType
* The concurrency type of deploying StackSets operations in Regions, could be in parallel or one Region at a
* time.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RegionConcurrencyType
*/
public StackSetOperationPreferences withRegionConcurrencyType(String regionConcurrencyType) {
setRegionConcurrencyType(regionConcurrencyType);
return this;
}
/**
*
* The concurrency type of deploying StackSets operations in Regions, could be in parallel or one Region at a time.
*
*
* @param regionConcurrencyType
* The concurrency type of deploying StackSets operations in Regions, could be in parallel or one Region at a
* time.
* @return Returns a reference to this object so that method calls can be chained together.
* @see RegionConcurrencyType
*/
public StackSetOperationPreferences withRegionConcurrencyType(RegionConcurrencyType regionConcurrencyType) {
this.regionConcurrencyType = regionConcurrencyType.toString();
return this;
}
/**
*
* The order of the Regions where you want to perform the stack operation.
*
*
* @return The order of the Regions where you want to perform the stack operation.
*/
public java.util.List getRegionOrder() {
if (regionOrder == null) {
regionOrder = new com.amazonaws.internal.SdkInternalList();
}
return regionOrder;
}
/**
*
* The order of the Regions where you want to perform the stack operation.
*
*
* @param regionOrder
* The order of the Regions where you want to perform the stack operation.
*/
public void setRegionOrder(java.util.Collection regionOrder) {
if (regionOrder == null) {
this.regionOrder = null;
return;
}
this.regionOrder = new com.amazonaws.internal.SdkInternalList(regionOrder);
}
/**
*
* The order of the Regions where you want to perform the stack operation.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRegionOrder(java.util.Collection)} or {@link #withRegionOrder(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param regionOrder
* The order of the Regions where you want to perform the stack operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetOperationPreferences withRegionOrder(String... regionOrder) {
if (this.regionOrder == null) {
setRegionOrder(new com.amazonaws.internal.SdkInternalList(regionOrder.length));
}
for (String ele : regionOrder) {
this.regionOrder.add(ele);
}
return this;
}
/**
*
* The order of the Regions where you want to perform the stack operation.
*
*
* @param regionOrder
* The order of the Regions where you want to perform the stack operation.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetOperationPreferences withRegionOrder(java.util.Collection regionOrder) {
setRegionOrder(regionOrder);
return this;
}
/**
*
* The number of accounts, per Region, for which this operation can fail before CloudFormation stops the operation
* in that Region. If the operation is stopped in a Region, CloudFormation doesn't attempt the operation in any
* subsequent Regions.
*
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
(but not both).
*
*
* By default, 0
is specified.
*
*
* @param failureToleranceCount
* The number of accounts, per Region, for which this operation can fail before CloudFormation stops the
* operation in that Region. If the operation is stopped in a Region, CloudFormation doesn't attempt the
* operation in any subsequent Regions.
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
(but not both).
*
*
* By default, 0
is specified.
*/
public void setFailureToleranceCount(Integer failureToleranceCount) {
this.failureToleranceCount = failureToleranceCount;
}
/**
*
* The number of accounts, per Region, for which this operation can fail before CloudFormation stops the operation
* in that Region. If the operation is stopped in a Region, CloudFormation doesn't attempt the operation in any
* subsequent Regions.
*
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
(but not both).
*
*
* By default, 0
is specified.
*
*
* @return The number of accounts, per Region, for which this operation can fail before CloudFormation stops the
* operation in that Region. If the operation is stopped in a Region, CloudFormation doesn't attempt the
* operation in any subsequent Regions.
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
(but not both).
*
*
* By default, 0
is specified.
*/
public Integer getFailureToleranceCount() {
return this.failureToleranceCount;
}
/**
*
* The number of accounts, per Region, for which this operation can fail before CloudFormation stops the operation
* in that Region. If the operation is stopped in a Region, CloudFormation doesn't attempt the operation in any
* subsequent Regions.
*
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
(but not both).
*
*
* By default, 0
is specified.
*
*
* @param failureToleranceCount
* The number of accounts, per Region, for which this operation can fail before CloudFormation stops the
* operation in that Region. If the operation is stopped in a Region, CloudFormation doesn't attempt the
* operation in any subsequent Regions.
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
(but not both).
*
*
* By default, 0
is specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetOperationPreferences withFailureToleranceCount(Integer failureToleranceCount) {
setFailureToleranceCount(failureToleranceCount);
return this;
}
/**
*
* The percentage of accounts, per Region, for which this stack operation can fail before CloudFormation stops the
* operation in that Region. If the operation is stopped in a Region, CloudFormation doesn't attempt the operation
* in any subsequent Regions.
*
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds down to
* the next whole number.
*
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
, but not both.
*
*
* By default, 0
is specified.
*
*
* @param failureTolerancePercentage
* The percentage of accounts, per Region, for which this stack operation can fail before CloudFormation
* stops the operation in that Region. If the operation is stopped in a Region, CloudFormation doesn't
* attempt the operation in any subsequent Regions.
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds
* down to the next whole number.
*
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
, but not both.
*
*
* By default, 0
is specified.
*/
public void setFailureTolerancePercentage(Integer failureTolerancePercentage) {
this.failureTolerancePercentage = failureTolerancePercentage;
}
/**
*
* The percentage of accounts, per Region, for which this stack operation can fail before CloudFormation stops the
* operation in that Region. If the operation is stopped in a Region, CloudFormation doesn't attempt the operation
* in any subsequent Regions.
*
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds down to
* the next whole number.
*
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
, but not both.
*
*
* By default, 0
is specified.
*
*
* @return The percentage of accounts, per Region, for which this stack operation can fail before CloudFormation
* stops the operation in that Region. If the operation is stopped in a Region, CloudFormation doesn't
* attempt the operation in any subsequent Regions.
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds
* down to the next whole number.
*
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
, but not both.
*
*
* By default, 0
is specified.
*/
public Integer getFailureTolerancePercentage() {
return this.failureTolerancePercentage;
}
/**
*
* The percentage of accounts, per Region, for which this stack operation can fail before CloudFormation stops the
* operation in that Region. If the operation is stopped in a Region, CloudFormation doesn't attempt the operation
* in any subsequent Regions.
*
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds down to
* the next whole number.
*
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
, but not both.
*
*
* By default, 0
is specified.
*
*
* @param failureTolerancePercentage
* The percentage of accounts, per Region, for which this stack operation can fail before CloudFormation
* stops the operation in that Region. If the operation is stopped in a Region, CloudFormation doesn't
* attempt the operation in any subsequent Regions.
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds
* down to the next whole number.
*
*
* Conditional: You must specify either FailureToleranceCount
or
* FailureTolerancePercentage
, but not both.
*
*
* By default, 0
is specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetOperationPreferences withFailureTolerancePercentage(Integer failureTolerancePercentage) {
setFailureTolerancePercentage(failureTolerancePercentage);
return this;
}
/**
*
* The maximum number of accounts in which to perform this operation at one time. This can depend on the value of
* FailureToleranceCount
depending on your ConcurrencyMode
.
* MaxConcurrentCount
is at most one more than the FailureToleranceCount
if you're using
* STRICT_FAILURE_TOLERANCE
.
*
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under certain
* circumstances the actual number of accounts acted upon concurrently may be lower due to service throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or MaxConcurrentPercentage
, but
* not both.
*
*
* By default, 1
is specified.
*
*
* @param maxConcurrentCount
* The maximum number of accounts in which to perform this operation at one time. This can depend on the
* value of FailureToleranceCount
depending on your ConcurrencyMode
.
* MaxConcurrentCount
is at most one more than the FailureToleranceCount
if you're
* using STRICT_FAILURE_TOLERANCE
.
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under
* certain circumstances the actual number of accounts acted upon concurrently may be lower due to service
* throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or
* MaxConcurrentPercentage
, but not both.
*
*
* By default, 1
is specified.
*/
public void setMaxConcurrentCount(Integer maxConcurrentCount) {
this.maxConcurrentCount = maxConcurrentCount;
}
/**
*
* The maximum number of accounts in which to perform this operation at one time. This can depend on the value of
* FailureToleranceCount
depending on your ConcurrencyMode
.
* MaxConcurrentCount
is at most one more than the FailureToleranceCount
if you're using
* STRICT_FAILURE_TOLERANCE
.
*
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under certain
* circumstances the actual number of accounts acted upon concurrently may be lower due to service throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or MaxConcurrentPercentage
, but
* not both.
*
*
* By default, 1
is specified.
*
*
* @return The maximum number of accounts in which to perform this operation at one time. This can depend on the
* value of FailureToleranceCount
depending on your ConcurrencyMode
.
* MaxConcurrentCount
is at most one more than the FailureToleranceCount
if you're
* using STRICT_FAILURE_TOLERANCE
.
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under
* certain circumstances the actual number of accounts acted upon concurrently may be lower due to service
* throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or
* MaxConcurrentPercentage
, but not both.
*
*
* By default, 1
is specified.
*/
public Integer getMaxConcurrentCount() {
return this.maxConcurrentCount;
}
/**
*
* The maximum number of accounts in which to perform this operation at one time. This can depend on the value of
* FailureToleranceCount
depending on your ConcurrencyMode
.
* MaxConcurrentCount
is at most one more than the FailureToleranceCount
if you're using
* STRICT_FAILURE_TOLERANCE
.
*
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under certain
* circumstances the actual number of accounts acted upon concurrently may be lower due to service throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or MaxConcurrentPercentage
, but
* not both.
*
*
* By default, 1
is specified.
*
*
* @param maxConcurrentCount
* The maximum number of accounts in which to perform this operation at one time. This can depend on the
* value of FailureToleranceCount
depending on your ConcurrencyMode
.
* MaxConcurrentCount
is at most one more than the FailureToleranceCount
if you're
* using STRICT_FAILURE_TOLERANCE
.
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under
* certain circumstances the actual number of accounts acted upon concurrently may be lower due to service
* throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or
* MaxConcurrentPercentage
, but not both.
*
*
* By default, 1
is specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetOperationPreferences withMaxConcurrentCount(Integer maxConcurrentCount) {
setMaxConcurrentCount(maxConcurrentCount);
return this;
}
/**
*
* The maximum percentage of accounts in which to perform this operation at one time.
*
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds down to the next
* whole number. This is true except in cases where rounding down would result is zero. In this case, CloudFormation
* sets the number as one instead.
*
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under certain
* circumstances the actual number of accounts acted upon concurrently may be lower due to service throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or MaxConcurrentPercentage
, but
* not both.
*
*
* By default, 1
is specified.
*
*
* @param maxConcurrentPercentage
* The maximum percentage of accounts in which to perform this operation at one time.
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds down to
* the next whole number. This is true except in cases where rounding down would result is zero. In this
* case, CloudFormation sets the number as one instead.
*
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under
* certain circumstances the actual number of accounts acted upon concurrently may be lower due to service
* throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or
* MaxConcurrentPercentage
, but not both.
*
*
* By default, 1
is specified.
*/
public void setMaxConcurrentPercentage(Integer maxConcurrentPercentage) {
this.maxConcurrentPercentage = maxConcurrentPercentage;
}
/**
*
* The maximum percentage of accounts in which to perform this operation at one time.
*
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds down to the next
* whole number. This is true except in cases where rounding down would result is zero. In this case, CloudFormation
* sets the number as one instead.
*
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under certain
* circumstances the actual number of accounts acted upon concurrently may be lower due to service throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or MaxConcurrentPercentage
, but
* not both.
*
*
* By default, 1
is specified.
*
*
* @return The maximum percentage of accounts in which to perform this operation at one time.
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds down to
* the next whole number. This is true except in cases where rounding down would result is zero. In this
* case, CloudFormation sets the number as one instead.
*
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under
* certain circumstances the actual number of accounts acted upon concurrently may be lower due to service
* throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or
* MaxConcurrentPercentage
, but not both.
*
*
* By default, 1
is specified.
*/
public Integer getMaxConcurrentPercentage() {
return this.maxConcurrentPercentage;
}
/**
*
* The maximum percentage of accounts in which to perform this operation at one time.
*
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds down to the next
* whole number. This is true except in cases where rounding down would result is zero. In this case, CloudFormation
* sets the number as one instead.
*
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under certain
* circumstances the actual number of accounts acted upon concurrently may be lower due to service throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or MaxConcurrentPercentage
, but
* not both.
*
*
* By default, 1
is specified.
*
*
* @param maxConcurrentPercentage
* The maximum percentage of accounts in which to perform this operation at one time.
*
* When calculating the number of accounts based on the specified percentage, CloudFormation rounds down to
* the next whole number. This is true except in cases where rounding down would result is zero. In this
* case, CloudFormation sets the number as one instead.
*
*
* Note that this setting lets you specify the maximum for operations. For large deployments, under
* certain circumstances the actual number of accounts acted upon concurrently may be lower due to service
* throttling.
*
*
* Conditional: You must specify either MaxConcurrentCount
or
* MaxConcurrentPercentage
, but not both.
*
*
* By default, 1
is specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSetOperationPreferences withMaxConcurrentPercentage(Integer maxConcurrentPercentage) {
setMaxConcurrentPercentage(maxConcurrentPercentage);
return this;
}
/**
*
* Specifies how the concurrency level behaves during the operation execution.
*
*
* -
*
* STRICT_FAILURE_TOLERANCE
: This option dynamically lowers the concurrency level to ensure the number
* of failed accounts never exceeds the value of FailureToleranceCount
+1. The initial actual
* concurrency is set to the lower of either the value of the MaxConcurrentCount
, or the value of
* MaxConcurrentCount
+1. The actual concurrency is then reduced proportionally by the number of
* failures. This is the default behavior.
*
*
* If failure tolerance or Maximum concurrent accounts are set to percentages, the behavior is similar.
*
*
* -
*
* SOFT_FAILURE_TOLERANCE
: This option decouples FailureToleranceCount
from the actual
* concurrency. This allows stack set operations to run at the concurrency level set by the
* MaxConcurrentCount
value, or MaxConcurrentPercentage
, regardless of the number of
* failures.
*
*
*
*
* @param concurrencyMode
* Specifies how the concurrency level behaves during the operation execution.
*
* -
*
* STRICT_FAILURE_TOLERANCE
: This option dynamically lowers the concurrency level to ensure the
* number of failed accounts never exceeds the value of FailureToleranceCount
+1. The initial
* actual concurrency is set to the lower of either the value of the MaxConcurrentCount
, or the
* value of MaxConcurrentCount
+1. The actual concurrency is then reduced proportionally by the
* number of failures. This is the default behavior.
*
*
* If failure tolerance or Maximum concurrent accounts are set to percentages, the behavior is similar.
*
*
* -
*
* SOFT_FAILURE_TOLERANCE
: This option decouples FailureToleranceCount
from the
* actual concurrency. This allows stack set operations to run at the concurrency level set by the
* MaxConcurrentCount
value, or MaxConcurrentPercentage
, regardless of the number
* of failures.
*
*
* @see ConcurrencyMode
*/
public void setConcurrencyMode(String concurrencyMode) {
this.concurrencyMode = concurrencyMode;
}
/**
*
* Specifies how the concurrency level behaves during the operation execution.
*
*
* -
*
* STRICT_FAILURE_TOLERANCE
: This option dynamically lowers the concurrency level to ensure the number
* of failed accounts never exceeds the value of FailureToleranceCount
+1. The initial actual
* concurrency is set to the lower of either the value of the MaxConcurrentCount
, or the value of
* MaxConcurrentCount
+1. The actual concurrency is then reduced proportionally by the number of
* failures. This is the default behavior.
*
*
* If failure tolerance or Maximum concurrent accounts are set to percentages, the behavior is similar.
*
*
* -
*
* SOFT_FAILURE_TOLERANCE
: This option decouples FailureToleranceCount
from the actual
* concurrency. This allows stack set operations to run at the concurrency level set by the
* MaxConcurrentCount
value, or MaxConcurrentPercentage
, regardless of the number of
* failures.
*
*
*
*
* @return Specifies how the concurrency level behaves during the operation execution.
*
* -
*
* STRICT_FAILURE_TOLERANCE
: This option dynamically lowers the concurrency level to ensure the
* number of failed accounts never exceeds the value of FailureToleranceCount
+1. The initial
* actual concurrency is set to the lower of either the value of the MaxConcurrentCount
, or the
* value of MaxConcurrentCount
+1. The actual concurrency is then reduced proportionally by the
* number of failures. This is the default behavior.
*
*
* If failure tolerance or Maximum concurrent accounts are set to percentages, the behavior is similar.
*
*
* -
*
* SOFT_FAILURE_TOLERANCE
: This option decouples FailureToleranceCount
from the
* actual concurrency. This allows stack set operations to run at the concurrency level set by the
* MaxConcurrentCount
value, or MaxConcurrentPercentage
, regardless of the number
* of failures.
*
*
* @see ConcurrencyMode
*/
public String getConcurrencyMode() {
return this.concurrencyMode;
}
/**
*
* Specifies how the concurrency level behaves during the operation execution.
*
*
* -
*
* STRICT_FAILURE_TOLERANCE
: This option dynamically lowers the concurrency level to ensure the number
* of failed accounts never exceeds the value of FailureToleranceCount
+1. The initial actual
* concurrency is set to the lower of either the value of the MaxConcurrentCount
, or the value of
* MaxConcurrentCount
+1. The actual concurrency is then reduced proportionally by the number of
* failures. This is the default behavior.
*
*
* If failure tolerance or Maximum concurrent accounts are set to percentages, the behavior is similar.
*
*
* -
*
* SOFT_FAILURE_TOLERANCE
: This option decouples FailureToleranceCount
from the actual
* concurrency. This allows stack set operations to run at the concurrency level set by the
* MaxConcurrentCount
value, or MaxConcurrentPercentage
, regardless of the number of
* failures.
*
*
*
*
* @param concurrencyMode
* Specifies how the concurrency level behaves during the operation execution.
*
* -
*
* STRICT_FAILURE_TOLERANCE
: This option dynamically lowers the concurrency level to ensure the
* number of failed accounts never exceeds the value of FailureToleranceCount
+1. The initial
* actual concurrency is set to the lower of either the value of the MaxConcurrentCount
, or the
* value of MaxConcurrentCount
+1. The actual concurrency is then reduced proportionally by the
* number of failures. This is the default behavior.
*
*
* If failure tolerance or Maximum concurrent accounts are set to percentages, the behavior is similar.
*
*
* -
*
* SOFT_FAILURE_TOLERANCE
: This option decouples FailureToleranceCount
from the
* actual concurrency. This allows stack set operations to run at the concurrency level set by the
* MaxConcurrentCount
value, or MaxConcurrentPercentage
, regardless of the number
* of failures.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ConcurrencyMode
*/
public StackSetOperationPreferences withConcurrencyMode(String concurrencyMode) {
setConcurrencyMode(concurrencyMode);
return this;
}
/**
*
* Specifies how the concurrency level behaves during the operation execution.
*
*
* -
*
* STRICT_FAILURE_TOLERANCE
: This option dynamically lowers the concurrency level to ensure the number
* of failed accounts never exceeds the value of FailureToleranceCount
+1. The initial actual
* concurrency is set to the lower of either the value of the MaxConcurrentCount
, or the value of
* MaxConcurrentCount
+1. The actual concurrency is then reduced proportionally by the number of
* failures. This is the default behavior.
*
*
* If failure tolerance or Maximum concurrent accounts are set to percentages, the behavior is similar.
*
*
* -
*
* SOFT_FAILURE_TOLERANCE
: This option decouples FailureToleranceCount
from the actual
* concurrency. This allows stack set operations to run at the concurrency level set by the
* MaxConcurrentCount
value, or MaxConcurrentPercentage
, regardless of the number of
* failures.
*
*
*
*
* @param concurrencyMode
* Specifies how the concurrency level behaves during the operation execution.
*
* -
*
* STRICT_FAILURE_TOLERANCE
: This option dynamically lowers the concurrency level to ensure the
* number of failed accounts never exceeds the value of FailureToleranceCount
+1. The initial
* actual concurrency is set to the lower of either the value of the MaxConcurrentCount
, or the
* value of MaxConcurrentCount
+1. The actual concurrency is then reduced proportionally by the
* number of failures. This is the default behavior.
*
*
* If failure tolerance or Maximum concurrent accounts are set to percentages, the behavior is similar.
*
*
* -
*
* SOFT_FAILURE_TOLERANCE
: This option decouples FailureToleranceCount
from the
* actual concurrency. This allows stack set operations to run at the concurrency level set by the
* MaxConcurrentCount
value, or MaxConcurrentPercentage
, regardless of the number
* of failures.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see ConcurrencyMode
*/
public StackSetOperationPreferences withConcurrencyMode(ConcurrencyMode concurrencyMode) {
this.concurrencyMode = concurrencyMode.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getRegionConcurrencyType() != null)
sb.append("RegionConcurrencyType: ").append(getRegionConcurrencyType()).append(",");
if (getRegionOrder() != null)
sb.append("RegionOrder: ").append(getRegionOrder()).append(",");
if (getFailureToleranceCount() != null)
sb.append("FailureToleranceCount: ").append(getFailureToleranceCount()).append(",");
if (getFailureTolerancePercentage() != null)
sb.append("FailureTolerancePercentage: ").append(getFailureTolerancePercentage()).append(",");
if (getMaxConcurrentCount() != null)
sb.append("MaxConcurrentCount: ").append(getMaxConcurrentCount()).append(",");
if (getMaxConcurrentPercentage() != null)
sb.append("MaxConcurrentPercentage: ").append(getMaxConcurrentPercentage()).append(",");
if (getConcurrencyMode() != null)
sb.append("ConcurrencyMode: ").append(getConcurrencyMode());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StackSetOperationPreferences == false)
return false;
StackSetOperationPreferences other = (StackSetOperationPreferences) obj;
if (other.getRegionConcurrencyType() == null ^ this.getRegionConcurrencyType() == null)
return false;
if (other.getRegionConcurrencyType() != null && other.getRegionConcurrencyType().equals(this.getRegionConcurrencyType()) == false)
return false;
if (other.getRegionOrder() == null ^ this.getRegionOrder() == null)
return false;
if (other.getRegionOrder() != null && other.getRegionOrder().equals(this.getRegionOrder()) == false)
return false;
if (other.getFailureToleranceCount() == null ^ this.getFailureToleranceCount() == null)
return false;
if (other.getFailureToleranceCount() != null && other.getFailureToleranceCount().equals(this.getFailureToleranceCount()) == false)
return false;
if (other.getFailureTolerancePercentage() == null ^ this.getFailureTolerancePercentage() == null)
return false;
if (other.getFailureTolerancePercentage() != null && other.getFailureTolerancePercentage().equals(this.getFailureTolerancePercentage()) == false)
return false;
if (other.getMaxConcurrentCount() == null ^ this.getMaxConcurrentCount() == null)
return false;
if (other.getMaxConcurrentCount() != null && other.getMaxConcurrentCount().equals(this.getMaxConcurrentCount()) == false)
return false;
if (other.getMaxConcurrentPercentage() == null ^ this.getMaxConcurrentPercentage() == null)
return false;
if (other.getMaxConcurrentPercentage() != null && other.getMaxConcurrentPercentage().equals(this.getMaxConcurrentPercentage()) == false)
return false;
if (other.getConcurrencyMode() == null ^ this.getConcurrencyMode() == null)
return false;
if (other.getConcurrencyMode() != null && other.getConcurrencyMode().equals(this.getConcurrencyMode()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getRegionConcurrencyType() == null) ? 0 : getRegionConcurrencyType().hashCode());
hashCode = prime * hashCode + ((getRegionOrder() == null) ? 0 : getRegionOrder().hashCode());
hashCode = prime * hashCode + ((getFailureToleranceCount() == null) ? 0 : getFailureToleranceCount().hashCode());
hashCode = prime * hashCode + ((getFailureTolerancePercentage() == null) ? 0 : getFailureTolerancePercentage().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrentCount() == null) ? 0 : getMaxConcurrentCount().hashCode());
hashCode = prime * hashCode + ((getMaxConcurrentPercentage() == null) ? 0 : getMaxConcurrentPercentage().hashCode());
hashCode = prime * hashCode + ((getConcurrencyMode() == null) ? 0 : getConcurrencyMode().hashCode());
return hashCode;
}
@Override
public StackSetOperationPreferences clone() {
try {
return (StackSetOperationPreferences) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}