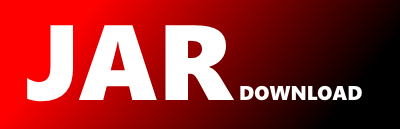
com.amazonaws.services.cloudformation.model.DeleteStackRequest Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudformation.model;
import java.io.Serializable;
import javax.annotation.Generated;
import com.amazonaws.AmazonWebServiceRequest;
/**
*
* The input for DeleteStack action.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class DeleteStackRequest extends com.amazonaws.AmazonWebServiceRequest implements Serializable, Cloneable {
/**
*
* The name or the unique stack ID that's associated with the stack.
*
*/
private String stackName;
/**
*
* For stacks in the DELETE_FAILED
state, a list of resource logical IDs that are associated with the
* resources you want to retain. During deletion, CloudFormation deletes the stack but doesn't delete the retained
* resources.
*
*
* Retaining resources is useful when you can't delete a resource, such as a non-empty S3 bucket, but you want to
* delete the stack.
*
*/
private com.amazonaws.internal.SdkInternalList retainResources;
/**
*
* The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation assumes to
* delete the stack. CloudFormation uses the role's credentials to make calls on your behalf.
*
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the stack. If no
* role is available, CloudFormation uses a temporary session that's generated from your user credentials.
*
*/
private String roleARN;
/**
*
* A unique identifier for this DeleteStack
request. Specify this token if you plan to retry requests
* so that CloudFormation knows that you're not attempting to delete a stack with the same name. You might retry
* DeleteStack
requests to ensure that CloudFormation successfully received them.
*
*
* All events initiated by a given stack operation are assigned the same client request token, which you can use to
* track operations. For example, if you execute a CreateStack
operation with the token
* token1
, then all the StackEvents
generated by that operation will have
* ClientRequestToken
set as token1
.
*
*
* In the console, stack operations display the client request token on the Events tab. Stack operations that are
* initiated from the console use the token format Console-StackOperation-ID, which helps you easily identify
* the stack operation . For example, if you create a stack using the console, each stack event would be assigned
* the same token in the following format: Console-CreateStack-7f59c3cf-00d2-40c7-b2ff-e75db0987002
.
*
*/
private String clientRequestToken;
/**
*
* Specifies the deletion mode for the stack. Possible values are:
*
*
* -
*
* STANDARD
- Use the standard behavior. Specifying this value is the same as not specifying this
* parameter.
*
*
* -
*
* FORCE_DELETE_STACK
- Delete the stack if it's stuck in a DELETE_FAILED
state due to
* resource deletion failure.
*
*
*
*/
private String deletionMode;
/**
*
* The name or the unique stack ID that's associated with the stack.
*
*
* @param stackName
* The name or the unique stack ID that's associated with the stack.
*/
public void setStackName(String stackName) {
this.stackName = stackName;
}
/**
*
* The name or the unique stack ID that's associated with the stack.
*
*
* @return The name or the unique stack ID that's associated with the stack.
*/
public String getStackName() {
return this.stackName;
}
/**
*
* The name or the unique stack ID that's associated with the stack.
*
*
* @param stackName
* The name or the unique stack ID that's associated with the stack.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteStackRequest withStackName(String stackName) {
setStackName(stackName);
return this;
}
/**
*
* For stacks in the DELETE_FAILED
state, a list of resource logical IDs that are associated with the
* resources you want to retain. During deletion, CloudFormation deletes the stack but doesn't delete the retained
* resources.
*
*
* Retaining resources is useful when you can't delete a resource, such as a non-empty S3 bucket, but you want to
* delete the stack.
*
*
* @return For stacks in the DELETE_FAILED
state, a list of resource logical IDs that are associated
* with the resources you want to retain. During deletion, CloudFormation deletes the stack but doesn't
* delete the retained resources.
*
* Retaining resources is useful when you can't delete a resource, such as a non-empty S3 bucket, but you
* want to delete the stack.
*/
public java.util.List getRetainResources() {
if (retainResources == null) {
retainResources = new com.amazonaws.internal.SdkInternalList();
}
return retainResources;
}
/**
*
* For stacks in the DELETE_FAILED
state, a list of resource logical IDs that are associated with the
* resources you want to retain. During deletion, CloudFormation deletes the stack but doesn't delete the retained
* resources.
*
*
* Retaining resources is useful when you can't delete a resource, such as a non-empty S3 bucket, but you want to
* delete the stack.
*
*
* @param retainResources
* For stacks in the DELETE_FAILED
state, a list of resource logical IDs that are associated
* with the resources you want to retain. During deletion, CloudFormation deletes the stack but doesn't
* delete the retained resources.
*
* Retaining resources is useful when you can't delete a resource, such as a non-empty S3 bucket, but you
* want to delete the stack.
*/
public void setRetainResources(java.util.Collection retainResources) {
if (retainResources == null) {
this.retainResources = null;
return;
}
this.retainResources = new com.amazonaws.internal.SdkInternalList(retainResources);
}
/**
*
* For stacks in the DELETE_FAILED
state, a list of resource logical IDs that are associated with the
* resources you want to retain. During deletion, CloudFormation deletes the stack but doesn't delete the retained
* resources.
*
*
* Retaining resources is useful when you can't delete a resource, such as a non-empty S3 bucket, but you want to
* delete the stack.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRetainResources(java.util.Collection)} or {@link #withRetainResources(java.util.Collection)} if you
* want to override the existing values.
*
*
* @param retainResources
* For stacks in the DELETE_FAILED
state, a list of resource logical IDs that are associated
* with the resources you want to retain. During deletion, CloudFormation deletes the stack but doesn't
* delete the retained resources.
*
* Retaining resources is useful when you can't delete a resource, such as a non-empty S3 bucket, but you
* want to delete the stack.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteStackRequest withRetainResources(String... retainResources) {
if (this.retainResources == null) {
setRetainResources(new com.amazonaws.internal.SdkInternalList(retainResources.length));
}
for (String ele : retainResources) {
this.retainResources.add(ele);
}
return this;
}
/**
*
* For stacks in the DELETE_FAILED
state, a list of resource logical IDs that are associated with the
* resources you want to retain. During deletion, CloudFormation deletes the stack but doesn't delete the retained
* resources.
*
*
* Retaining resources is useful when you can't delete a resource, such as a non-empty S3 bucket, but you want to
* delete the stack.
*
*
* @param retainResources
* For stacks in the DELETE_FAILED
state, a list of resource logical IDs that are associated
* with the resources you want to retain. During deletion, CloudFormation deletes the stack but doesn't
* delete the retained resources.
*
* Retaining resources is useful when you can't delete a resource, such as a non-empty S3 bucket, but you
* want to delete the stack.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteStackRequest withRetainResources(java.util.Collection retainResources) {
setRetainResources(retainResources);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation assumes to
* delete the stack. CloudFormation uses the role's credentials to make calls on your behalf.
*
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the stack. If no
* role is available, CloudFormation uses a temporary session that's generated from your user credentials.
*
*
* @param roleARN
* The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation assumes
* to delete the stack. CloudFormation uses the role's credentials to make calls on your behalf.
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the stack.
* If no role is available, CloudFormation uses a temporary session that's generated from your user
* credentials.
*/
public void setRoleARN(String roleARN) {
this.roleARN = roleARN;
}
/**
*
* The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation assumes to
* delete the stack. CloudFormation uses the role's credentials to make calls on your behalf.
*
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the stack. If no
* role is available, CloudFormation uses a temporary session that's generated from your user credentials.
*
*
* @return The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation
* assumes to delete the stack. CloudFormation uses the role's credentials to make calls on your behalf.
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the stack.
* If no role is available, CloudFormation uses a temporary session that's generated from your user
* credentials.
*/
public String getRoleARN() {
return this.roleARN;
}
/**
*
* The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation assumes to
* delete the stack. CloudFormation uses the role's credentials to make calls on your behalf.
*
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the stack. If no
* role is available, CloudFormation uses a temporary session that's generated from your user credentials.
*
*
* @param roleARN
* The Amazon Resource Name (ARN) of an Identity and Access Management (IAM) role that CloudFormation assumes
* to delete the stack. CloudFormation uses the role's credentials to make calls on your behalf.
*
* If you don't specify a value, CloudFormation uses the role that was previously associated with the stack.
* If no role is available, CloudFormation uses a temporary session that's generated from your user
* credentials.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteStackRequest withRoleARN(String roleARN) {
setRoleARN(roleARN);
return this;
}
/**
*
* A unique identifier for this DeleteStack
request. Specify this token if you plan to retry requests
* so that CloudFormation knows that you're not attempting to delete a stack with the same name. You might retry
* DeleteStack
requests to ensure that CloudFormation successfully received them.
*
*
* All events initiated by a given stack operation are assigned the same client request token, which you can use to
* track operations. For example, if you execute a CreateStack
operation with the token
* token1
, then all the StackEvents
generated by that operation will have
* ClientRequestToken
set as token1
.
*
*
* In the console, stack operations display the client request token on the Events tab. Stack operations that are
* initiated from the console use the token format Console-StackOperation-ID, which helps you easily identify
* the stack operation . For example, if you create a stack using the console, each stack event would be assigned
* the same token in the following format: Console-CreateStack-7f59c3cf-00d2-40c7-b2ff-e75db0987002
.
*
*
* @param clientRequestToken
* A unique identifier for this DeleteStack
request. Specify this token if you plan to retry
* requests so that CloudFormation knows that you're not attempting to delete a stack with the same name. You
* might retry DeleteStack
requests to ensure that CloudFormation successfully received
* them.
*
* All events initiated by a given stack operation are assigned the same client request token, which you can
* use to track operations. For example, if you execute a CreateStack
operation with the token
* token1
, then all the StackEvents
generated by that operation will have
* ClientRequestToken
set as token1
.
*
*
* In the console, stack operations display the client request token on the Events tab. Stack operations that
* are initiated from the console use the token format Console-StackOperation-ID, which helps you
* easily identify the stack operation . For example, if you create a stack using the console, each stack
* event would be assigned the same token in the following format:
* Console-CreateStack-7f59c3cf-00d2-40c7-b2ff-e75db0987002
.
*/
public void setClientRequestToken(String clientRequestToken) {
this.clientRequestToken = clientRequestToken;
}
/**
*
* A unique identifier for this DeleteStack
request. Specify this token if you plan to retry requests
* so that CloudFormation knows that you're not attempting to delete a stack with the same name. You might retry
* DeleteStack
requests to ensure that CloudFormation successfully received them.
*
*
* All events initiated by a given stack operation are assigned the same client request token, which you can use to
* track operations. For example, if you execute a CreateStack
operation with the token
* token1
, then all the StackEvents
generated by that operation will have
* ClientRequestToken
set as token1
.
*
*
* In the console, stack operations display the client request token on the Events tab. Stack operations that are
* initiated from the console use the token format Console-StackOperation-ID, which helps you easily identify
* the stack operation . For example, if you create a stack using the console, each stack event would be assigned
* the same token in the following format: Console-CreateStack-7f59c3cf-00d2-40c7-b2ff-e75db0987002
.
*
*
* @return A unique identifier for this DeleteStack
request. Specify this token if you plan to retry
* requests so that CloudFormation knows that you're not attempting to delete a stack with the same name.
* You might retry DeleteStack
requests to ensure that CloudFormation successfully received
* them.
*
* All events initiated by a given stack operation are assigned the same client request token, which you can
* use to track operations. For example, if you execute a CreateStack
operation with the token
* token1
, then all the StackEvents
generated by that operation will have
* ClientRequestToken
set as token1
.
*
*
* In the console, stack operations display the client request token on the Events tab. Stack operations
* that are initiated from the console use the token format Console-StackOperation-ID, which helps
* you easily identify the stack operation . For example, if you create a stack using the console, each
* stack event would be assigned the same token in the following format:
* Console-CreateStack-7f59c3cf-00d2-40c7-b2ff-e75db0987002
.
*/
public String getClientRequestToken() {
return this.clientRequestToken;
}
/**
*
* A unique identifier for this DeleteStack
request. Specify this token if you plan to retry requests
* so that CloudFormation knows that you're not attempting to delete a stack with the same name. You might retry
* DeleteStack
requests to ensure that CloudFormation successfully received them.
*
*
* All events initiated by a given stack operation are assigned the same client request token, which you can use to
* track operations. For example, if you execute a CreateStack
operation with the token
* token1
, then all the StackEvents
generated by that operation will have
* ClientRequestToken
set as token1
.
*
*
* In the console, stack operations display the client request token on the Events tab. Stack operations that are
* initiated from the console use the token format Console-StackOperation-ID, which helps you easily identify
* the stack operation . For example, if you create a stack using the console, each stack event would be assigned
* the same token in the following format: Console-CreateStack-7f59c3cf-00d2-40c7-b2ff-e75db0987002
.
*
*
* @param clientRequestToken
* A unique identifier for this DeleteStack
request. Specify this token if you plan to retry
* requests so that CloudFormation knows that you're not attempting to delete a stack with the same name. You
* might retry DeleteStack
requests to ensure that CloudFormation successfully received
* them.
*
* All events initiated by a given stack operation are assigned the same client request token, which you can
* use to track operations. For example, if you execute a CreateStack
operation with the token
* token1
, then all the StackEvents
generated by that operation will have
* ClientRequestToken
set as token1
.
*
*
* In the console, stack operations display the client request token on the Events tab. Stack operations that
* are initiated from the console use the token format Console-StackOperation-ID, which helps you
* easily identify the stack operation . For example, if you create a stack using the console, each stack
* event would be assigned the same token in the following format:
* Console-CreateStack-7f59c3cf-00d2-40c7-b2ff-e75db0987002
.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public DeleteStackRequest withClientRequestToken(String clientRequestToken) {
setClientRequestToken(clientRequestToken);
return this;
}
/**
*
* Specifies the deletion mode for the stack. Possible values are:
*
*
* -
*
* STANDARD
- Use the standard behavior. Specifying this value is the same as not specifying this
* parameter.
*
*
* -
*
* FORCE_DELETE_STACK
- Delete the stack if it's stuck in a DELETE_FAILED
state due to
* resource deletion failure.
*
*
*
*
* @param deletionMode
* Specifies the deletion mode for the stack. Possible values are:
*
* -
*
* STANDARD
- Use the standard behavior. Specifying this value is the same as not specifying
* this parameter.
*
*
* -
*
* FORCE_DELETE_STACK
- Delete the stack if it's stuck in a DELETE_FAILED
state due
* to resource deletion failure.
*
*
* @see DeletionMode
*/
public void setDeletionMode(String deletionMode) {
this.deletionMode = deletionMode;
}
/**
*
* Specifies the deletion mode for the stack. Possible values are:
*
*
* -
*
* STANDARD
- Use the standard behavior. Specifying this value is the same as not specifying this
* parameter.
*
*
* -
*
* FORCE_DELETE_STACK
- Delete the stack if it's stuck in a DELETE_FAILED
state due to
* resource deletion failure.
*
*
*
*
* @return Specifies the deletion mode for the stack. Possible values are:
*
* -
*
* STANDARD
- Use the standard behavior. Specifying this value is the same as not specifying
* this parameter.
*
*
* -
*
* FORCE_DELETE_STACK
- Delete the stack if it's stuck in a DELETE_FAILED
state
* due to resource deletion failure.
*
*
* @see DeletionMode
*/
public String getDeletionMode() {
return this.deletionMode;
}
/**
*
* Specifies the deletion mode for the stack. Possible values are:
*
*
* -
*
* STANDARD
- Use the standard behavior. Specifying this value is the same as not specifying this
* parameter.
*
*
* -
*
* FORCE_DELETE_STACK
- Delete the stack if it's stuck in a DELETE_FAILED
state due to
* resource deletion failure.
*
*
*
*
* @param deletionMode
* Specifies the deletion mode for the stack. Possible values are:
*
* -
*
* STANDARD
- Use the standard behavior. Specifying this value is the same as not specifying
* this parameter.
*
*
* -
*
* FORCE_DELETE_STACK
- Delete the stack if it's stuck in a DELETE_FAILED
state due
* to resource deletion failure.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeletionMode
*/
public DeleteStackRequest withDeletionMode(String deletionMode) {
setDeletionMode(deletionMode);
return this;
}
/**
*
* Specifies the deletion mode for the stack. Possible values are:
*
*
* -
*
* STANDARD
- Use the standard behavior. Specifying this value is the same as not specifying this
* parameter.
*
*
* -
*
* FORCE_DELETE_STACK
- Delete the stack if it's stuck in a DELETE_FAILED
state due to
* resource deletion failure.
*
*
*
*
* @param deletionMode
* Specifies the deletion mode for the stack. Possible values are:
*
* -
*
* STANDARD
- Use the standard behavior. Specifying this value is the same as not specifying
* this parameter.
*
*
* -
*
* FORCE_DELETE_STACK
- Delete the stack if it's stuck in a DELETE_FAILED
state due
* to resource deletion failure.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see DeletionMode
*/
public DeleteStackRequest withDeletionMode(DeletionMode deletionMode) {
this.deletionMode = deletionMode.toString();
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStackName() != null)
sb.append("StackName: ").append(getStackName()).append(",");
if (getRetainResources() != null)
sb.append("RetainResources: ").append(getRetainResources()).append(",");
if (getRoleARN() != null)
sb.append("RoleARN: ").append(getRoleARN()).append(",");
if (getClientRequestToken() != null)
sb.append("ClientRequestToken: ").append(getClientRequestToken()).append(",");
if (getDeletionMode() != null)
sb.append("DeletionMode: ").append(getDeletionMode());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof DeleteStackRequest == false)
return false;
DeleteStackRequest other = (DeleteStackRequest) obj;
if (other.getStackName() == null ^ this.getStackName() == null)
return false;
if (other.getStackName() != null && other.getStackName().equals(this.getStackName()) == false)
return false;
if (other.getRetainResources() == null ^ this.getRetainResources() == null)
return false;
if (other.getRetainResources() != null && other.getRetainResources().equals(this.getRetainResources()) == false)
return false;
if (other.getRoleARN() == null ^ this.getRoleARN() == null)
return false;
if (other.getRoleARN() != null && other.getRoleARN().equals(this.getRoleARN()) == false)
return false;
if (other.getClientRequestToken() == null ^ this.getClientRequestToken() == null)
return false;
if (other.getClientRequestToken() != null && other.getClientRequestToken().equals(this.getClientRequestToken()) == false)
return false;
if (other.getDeletionMode() == null ^ this.getDeletionMode() == null)
return false;
if (other.getDeletionMode() != null && other.getDeletionMode().equals(this.getDeletionMode()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStackName() == null) ? 0 : getStackName().hashCode());
hashCode = prime * hashCode + ((getRetainResources() == null) ? 0 : getRetainResources().hashCode());
hashCode = prime * hashCode + ((getRoleARN() == null) ? 0 : getRoleARN().hashCode());
hashCode = prime * hashCode + ((getClientRequestToken() == null) ? 0 : getClientRequestToken().hashCode());
hashCode = prime * hashCode + ((getDeletionMode() == null) ? 0 : getDeletionMode().hashCode());
return hashCode;
}
@Override
public DeleteStackRequest clone() {
return (DeleteStackRequest) super.clone();
}
}