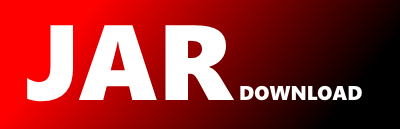
com.amazonaws.services.cloudformation.model.StackSet Maven / Gradle / Ivy
Show all versions of aws-java-sdk-cloudformation Show documentation
/*
* Copyright 2019-2024 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudformation.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* A structure that contains information about a stack set. A stack set enables you to provision stacks into Amazon Web
* Services accounts and across Regions by using a single CloudFormation template. In the stack set, you specify the
* template to use, in addition to any parameters and capabilities that the template requires.
*
*
* @see AWS API
* Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class StackSet implements Serializable, Cloneable {
/**
*
* The name that's associated with the stack set.
*
*/
private String stackSetName;
/**
*
* The ID of the stack set.
*
*/
private String stackSetId;
/**
*
* A description of the stack set that you specify when the stack set is created or updated.
*
*/
private String description;
/**
*
* The status of the stack set.
*
*/
private String status;
/**
*
* The structure that contains the body of the template that was used to create or update the stack set.
*
*/
private String templateBody;
/**
*
* A list of input parameters for a stack set.
*
*/
private com.amazonaws.internal.SdkInternalList parameters;
/**
*
* The capabilities that are allowed in the stack set. Some stack set templates might include resources that can
* affect permissions in your Amazon Web Services account—for example, by creating new Identity and Access
* Management (IAM) users. For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*/
private com.amazonaws.internal.SdkInternalList capabilities;
/**
*
* A list of tags that specify information about the stack set. A maximum number of 50 tags can be specified.
*
*/
private com.amazonaws.internal.SdkInternalList tags;
/**
*
* The Amazon Resource Name (ARN) of the stack set.
*
*/
private String stackSetARN;
/**
*
* The Amazon Resource Name (ARN) of the IAM role used to create or update the stack set.
*
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within the
* same administrator account. For more information, see Prerequisites:
* Granting Permissions for Stack Set Operations in the CloudFormation User Guide.
*
*/
private String administrationRoleARN;
/**
*
* The name of the IAM execution role used to create or update the stack set.
*
*
* Use customized execution roles to control which stack resources users and groups can include in their stack sets.
*
*/
private String executionRoleName;
/**
*
* Detailed information about the drift status of the stack set.
*
*
* For stack sets, contains information about the last completed drift operation performed on the stack set.
* Information about drift operations currently in progress isn't included.
*
*/
private StackSetDriftDetectionDetails stackSetDriftDetectionDetails;
/**
*
* [Service-managed permissions] Describes whether StackSets automatically deploys to Organizations accounts that
* are added to a target organization or organizational unit (OU).
*
*/
private AutoDeployment autoDeployment;
/**
*
* Describes how the IAM roles required for stack set operations are created.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*/
private String permissionModel;
/**
*
* [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified for
* DeploymentTargets.
*
*/
private com.amazonaws.internal.SdkInternalList organizationalUnitIds;
/**
*
* Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting operations.
*
*/
private ManagedExecution managedExecution;
/**
*
* Returns a list of all Amazon Web Services Regions the given StackSet has stack instances deployed in. The Amazon
* Web Services Regions list output is in no particular order.
*
*/
private com.amazonaws.internal.SdkInternalList regions;
/**
*
* The name that's associated with the stack set.
*
*
* @param stackSetName
* The name that's associated with the stack set.
*/
public void setStackSetName(String stackSetName) {
this.stackSetName = stackSetName;
}
/**
*
* The name that's associated with the stack set.
*
*
* @return The name that's associated with the stack set.
*/
public String getStackSetName() {
return this.stackSetName;
}
/**
*
* The name that's associated with the stack set.
*
*
* @param stackSetName
* The name that's associated with the stack set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withStackSetName(String stackSetName) {
setStackSetName(stackSetName);
return this;
}
/**
*
* The ID of the stack set.
*
*
* @param stackSetId
* The ID of the stack set.
*/
public void setStackSetId(String stackSetId) {
this.stackSetId = stackSetId;
}
/**
*
* The ID of the stack set.
*
*
* @return The ID of the stack set.
*/
public String getStackSetId() {
return this.stackSetId;
}
/**
*
* The ID of the stack set.
*
*
* @param stackSetId
* The ID of the stack set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withStackSetId(String stackSetId) {
setStackSetId(stackSetId);
return this;
}
/**
*
* A description of the stack set that you specify when the stack set is created or updated.
*
*
* @param description
* A description of the stack set that you specify when the stack set is created or updated.
*/
public void setDescription(String description) {
this.description = description;
}
/**
*
* A description of the stack set that you specify when the stack set is created or updated.
*
*
* @return A description of the stack set that you specify when the stack set is created or updated.
*/
public String getDescription() {
return this.description;
}
/**
*
* A description of the stack set that you specify when the stack set is created or updated.
*
*
* @param description
* A description of the stack set that you specify when the stack set is created or updated.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withDescription(String description) {
setDescription(description);
return this;
}
/**
*
* The status of the stack set.
*
*
* @param status
* The status of the stack set.
* @see StackSetStatus
*/
public void setStatus(String status) {
this.status = status;
}
/**
*
* The status of the stack set.
*
*
* @return The status of the stack set.
* @see StackSetStatus
*/
public String getStatus() {
return this.status;
}
/**
*
* The status of the stack set.
*
*
* @param status
* The status of the stack set.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackSetStatus
*/
public StackSet withStatus(String status) {
setStatus(status);
return this;
}
/**
*
* The status of the stack set.
*
*
* @param status
* The status of the stack set.
* @see StackSetStatus
*/
public void setStatus(StackSetStatus status) {
withStatus(status);
}
/**
*
* The status of the stack set.
*
*
* @param status
* The status of the stack set.
* @return Returns a reference to this object so that method calls can be chained together.
* @see StackSetStatus
*/
public StackSet withStatus(StackSetStatus status) {
this.status = status.toString();
return this;
}
/**
*
* The structure that contains the body of the template that was used to create or update the stack set.
*
*
* @param templateBody
* The structure that contains the body of the template that was used to create or update the stack set.
*/
public void setTemplateBody(String templateBody) {
this.templateBody = templateBody;
}
/**
*
* The structure that contains the body of the template that was used to create or update the stack set.
*
*
* @return The structure that contains the body of the template that was used to create or update the stack set.
*/
public String getTemplateBody() {
return this.templateBody;
}
/**
*
* The structure that contains the body of the template that was used to create or update the stack set.
*
*
* @param templateBody
* The structure that contains the body of the template that was used to create or update the stack set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withTemplateBody(String templateBody) {
setTemplateBody(templateBody);
return this;
}
/**
*
* A list of input parameters for a stack set.
*
*
* @return A list of input parameters for a stack set.
*/
public java.util.List getParameters() {
if (parameters == null) {
parameters = new com.amazonaws.internal.SdkInternalList();
}
return parameters;
}
/**
*
* A list of input parameters for a stack set.
*
*
* @param parameters
* A list of input parameters for a stack set.
*/
public void setParameters(java.util.Collection parameters) {
if (parameters == null) {
this.parameters = null;
return;
}
this.parameters = new com.amazonaws.internal.SdkInternalList(parameters);
}
/**
*
* A list of input parameters for a stack set.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setParameters(java.util.Collection)} or {@link #withParameters(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param parameters
* A list of input parameters for a stack set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withParameters(Parameter... parameters) {
if (this.parameters == null) {
setParameters(new com.amazonaws.internal.SdkInternalList(parameters.length));
}
for (Parameter ele : parameters) {
this.parameters.add(ele);
}
return this;
}
/**
*
* A list of input parameters for a stack set.
*
*
* @param parameters
* A list of input parameters for a stack set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withParameters(java.util.Collection parameters) {
setParameters(parameters);
return this;
}
/**
*
* The capabilities that are allowed in the stack set. Some stack set templates might include resources that can
* affect permissions in your Amazon Web Services account—for example, by creating new Identity and Access
* Management (IAM) users. For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* @return The capabilities that are allowed in the stack set. Some stack set templates might include resources that
* can affect permissions in your Amazon Web Services account—for example, by creating new Identity and
* Access Management (IAM) users. For more information, see Acknowledging IAM Resources in CloudFormation Templates.
* @see Capability
*/
public java.util.List getCapabilities() {
if (capabilities == null) {
capabilities = new com.amazonaws.internal.SdkInternalList();
}
return capabilities;
}
/**
*
* The capabilities that are allowed in the stack set. Some stack set templates might include resources that can
* affect permissions in your Amazon Web Services account—for example, by creating new Identity and Access
* Management (IAM) users. For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* @param capabilities
* The capabilities that are allowed in the stack set. Some stack set templates might include resources that
* can affect permissions in your Amazon Web Services account—for example, by creating new Identity and
* Access Management (IAM) users. For more information, see Acknowledging IAM Resources in CloudFormation Templates.
* @see Capability
*/
public void setCapabilities(java.util.Collection capabilities) {
if (capabilities == null) {
this.capabilities = null;
return;
}
this.capabilities = new com.amazonaws.internal.SdkInternalList(capabilities);
}
/**
*
* The capabilities that are allowed in the stack set. Some stack set templates might include resources that can
* affect permissions in your Amazon Web Services account—for example, by creating new Identity and Access
* Management (IAM) users. For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setCapabilities(java.util.Collection)} or {@link #withCapabilities(java.util.Collection)} if you want to
* override the existing values.
*
*
* @param capabilities
* The capabilities that are allowed in the stack set. Some stack set templates might include resources that
* can affect permissions in your Amazon Web Services account—for example, by creating new Identity and
* Access Management (IAM) users. For more information, see Acknowledging IAM Resources in CloudFormation Templates.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Capability
*/
public StackSet withCapabilities(String... capabilities) {
if (this.capabilities == null) {
setCapabilities(new com.amazonaws.internal.SdkInternalList(capabilities.length));
}
for (String ele : capabilities) {
this.capabilities.add(ele);
}
return this;
}
/**
*
* The capabilities that are allowed in the stack set. Some stack set templates might include resources that can
* affect permissions in your Amazon Web Services account—for example, by creating new Identity and Access
* Management (IAM) users. For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* @param capabilities
* The capabilities that are allowed in the stack set. Some stack set templates might include resources that
* can affect permissions in your Amazon Web Services account—for example, by creating new Identity and
* Access Management (IAM) users. For more information, see Acknowledging IAM Resources in CloudFormation Templates.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Capability
*/
public StackSet withCapabilities(java.util.Collection capabilities) {
setCapabilities(capabilities);
return this;
}
/**
*
* The capabilities that are allowed in the stack set. Some stack set templates might include resources that can
* affect permissions in your Amazon Web Services account—for example, by creating new Identity and Access
* Management (IAM) users. For more information, see Acknowledging IAM Resources in CloudFormation Templates.
*
*
* @param capabilities
* The capabilities that are allowed in the stack set. Some stack set templates might include resources that
* can affect permissions in your Amazon Web Services account—for example, by creating new Identity and
* Access Management (IAM) users. For more information, see Acknowledging IAM Resources in CloudFormation Templates.
* @return Returns a reference to this object so that method calls can be chained together.
* @see Capability
*/
public StackSet withCapabilities(Capability... capabilities) {
com.amazonaws.internal.SdkInternalList capabilitiesCopy = new com.amazonaws.internal.SdkInternalList(capabilities.length);
for (Capability value : capabilities) {
capabilitiesCopy.add(value.toString());
}
if (getCapabilities() == null) {
setCapabilities(capabilitiesCopy);
} else {
getCapabilities().addAll(capabilitiesCopy);
}
return this;
}
/**
*
* A list of tags that specify information about the stack set. A maximum number of 50 tags can be specified.
*
*
* @return A list of tags that specify information about the stack set. A maximum number of 50 tags can be
* specified.
*/
public java.util.List getTags() {
if (tags == null) {
tags = new com.amazonaws.internal.SdkInternalList();
}
return tags;
}
/**
*
* A list of tags that specify information about the stack set. A maximum number of 50 tags can be specified.
*
*
* @param tags
* A list of tags that specify information about the stack set. A maximum number of 50 tags can be specified.
*/
public void setTags(java.util.Collection tags) {
if (tags == null) {
this.tags = null;
return;
}
this.tags = new com.amazonaws.internal.SdkInternalList(tags);
}
/**
*
* A list of tags that specify information about the stack set. A maximum number of 50 tags can be specified.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setTags(java.util.Collection)} or {@link #withTags(java.util.Collection)} if you want to override the
* existing values.
*
*
* @param tags
* A list of tags that specify information about the stack set. A maximum number of 50 tags can be specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withTags(Tag... tags) {
if (this.tags == null) {
setTags(new com.amazonaws.internal.SdkInternalList(tags.length));
}
for (Tag ele : tags) {
this.tags.add(ele);
}
return this;
}
/**
*
* A list of tags that specify information about the stack set. A maximum number of 50 tags can be specified.
*
*
* @param tags
* A list of tags that specify information about the stack set. A maximum number of 50 tags can be specified.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withTags(java.util.Collection tags) {
setTags(tags);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the stack set.
*
*
* @param stackSetARN
* The Amazon Resource Name (ARN) of the stack set.
*/
public void setStackSetARN(String stackSetARN) {
this.stackSetARN = stackSetARN;
}
/**
*
* The Amazon Resource Name (ARN) of the stack set.
*
*
* @return The Amazon Resource Name (ARN) of the stack set.
*/
public String getStackSetARN() {
return this.stackSetARN;
}
/**
*
* The Amazon Resource Name (ARN) of the stack set.
*
*
* @param stackSetARN
* The Amazon Resource Name (ARN) of the stack set.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withStackSetARN(String stackSetARN) {
setStackSetARN(stackSetARN);
return this;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role used to create or update the stack set.
*
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within the
* same administrator account. For more information, see Prerequisites:
* Granting Permissions for Stack Set Operations in the CloudFormation User Guide.
*
*
* @param administrationRoleARN
* The Amazon Resource Name (ARN) of the IAM role used to create or update the stack set.
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within
* the same administrator account. For more information, see Prerequisites: Granting Permissions for Stack Set Operations in the CloudFormation User Guide.
*/
public void setAdministrationRoleARN(String administrationRoleARN) {
this.administrationRoleARN = administrationRoleARN;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role used to create or update the stack set.
*
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within the
* same administrator account. For more information, see Prerequisites:
* Granting Permissions for Stack Set Operations in the CloudFormation User Guide.
*
*
* @return The Amazon Resource Name (ARN) of the IAM role used to create or update the stack set.
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within
* the same administrator account. For more information, see Prerequisites: Granting Permissions for Stack Set Operations in the CloudFormation User
* Guide.
*/
public String getAdministrationRoleARN() {
return this.administrationRoleARN;
}
/**
*
* The Amazon Resource Name (ARN) of the IAM role used to create or update the stack set.
*
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within the
* same administrator account. For more information, see Prerequisites:
* Granting Permissions for Stack Set Operations in the CloudFormation User Guide.
*
*
* @param administrationRoleARN
* The Amazon Resource Name (ARN) of the IAM role used to create or update the stack set.
*
* Use customized administrator roles to control which users or groups can manage specific stack sets within
* the same administrator account. For more information, see Prerequisites: Granting Permissions for Stack Set Operations in the CloudFormation User Guide.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withAdministrationRoleARN(String administrationRoleARN) {
setAdministrationRoleARN(administrationRoleARN);
return this;
}
/**
*
* The name of the IAM execution role used to create or update the stack set.
*
*
* Use customized execution roles to control which stack resources users and groups can include in their stack sets.
*
*
* @param executionRoleName
* The name of the IAM execution role used to create or update the stack set.
*
* Use customized execution roles to control which stack resources users and groups can include in their
* stack sets.
*/
public void setExecutionRoleName(String executionRoleName) {
this.executionRoleName = executionRoleName;
}
/**
*
* The name of the IAM execution role used to create or update the stack set.
*
*
* Use customized execution roles to control which stack resources users and groups can include in their stack sets.
*
*
* @return The name of the IAM execution role used to create or update the stack set.
*
* Use customized execution roles to control which stack resources users and groups can include in their
* stack sets.
*/
public String getExecutionRoleName() {
return this.executionRoleName;
}
/**
*
* The name of the IAM execution role used to create or update the stack set.
*
*
* Use customized execution roles to control which stack resources users and groups can include in their stack sets.
*
*
* @param executionRoleName
* The name of the IAM execution role used to create or update the stack set.
*
* Use customized execution roles to control which stack resources users and groups can include in their
* stack sets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withExecutionRoleName(String executionRoleName) {
setExecutionRoleName(executionRoleName);
return this;
}
/**
*
* Detailed information about the drift status of the stack set.
*
*
* For stack sets, contains information about the last completed drift operation performed on the stack set.
* Information about drift operations currently in progress isn't included.
*
*
* @param stackSetDriftDetectionDetails
* Detailed information about the drift status of the stack set.
*
* For stack sets, contains information about the last completed drift operation performed on the
* stack set. Information about drift operations currently in progress isn't included.
*/
public void setStackSetDriftDetectionDetails(StackSetDriftDetectionDetails stackSetDriftDetectionDetails) {
this.stackSetDriftDetectionDetails = stackSetDriftDetectionDetails;
}
/**
*
* Detailed information about the drift status of the stack set.
*
*
* For stack sets, contains information about the last completed drift operation performed on the stack set.
* Information about drift operations currently in progress isn't included.
*
*
* @return Detailed information about the drift status of the stack set.
*
* For stack sets, contains information about the last completed drift operation performed on the
* stack set. Information about drift operations currently in progress isn't included.
*/
public StackSetDriftDetectionDetails getStackSetDriftDetectionDetails() {
return this.stackSetDriftDetectionDetails;
}
/**
*
* Detailed information about the drift status of the stack set.
*
*
* For stack sets, contains information about the last completed drift operation performed on the stack set.
* Information about drift operations currently in progress isn't included.
*
*
* @param stackSetDriftDetectionDetails
* Detailed information about the drift status of the stack set.
*
* For stack sets, contains information about the last completed drift operation performed on the
* stack set. Information about drift operations currently in progress isn't included.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withStackSetDriftDetectionDetails(StackSetDriftDetectionDetails stackSetDriftDetectionDetails) {
setStackSetDriftDetectionDetails(stackSetDriftDetectionDetails);
return this;
}
/**
*
* [Service-managed permissions] Describes whether StackSets automatically deploys to Organizations accounts that
* are added to a target organization or organizational unit (OU).
*
*
* @param autoDeployment
* [Service-managed permissions] Describes whether StackSets automatically deploys to Organizations accounts
* that are added to a target organization or organizational unit (OU).
*/
public void setAutoDeployment(AutoDeployment autoDeployment) {
this.autoDeployment = autoDeployment;
}
/**
*
* [Service-managed permissions] Describes whether StackSets automatically deploys to Organizations accounts that
* are added to a target organization or organizational unit (OU).
*
*
* @return [Service-managed permissions] Describes whether StackSets automatically deploys to Organizations accounts
* that are added to a target organization or organizational unit (OU).
*/
public AutoDeployment getAutoDeployment() {
return this.autoDeployment;
}
/**
*
* [Service-managed permissions] Describes whether StackSets automatically deploys to Organizations accounts that
* are added to a target organization or organizational unit (OU).
*
*
* @param autoDeployment
* [Service-managed permissions] Describes whether StackSets automatically deploys to Organizations accounts
* that are added to a target organization or organizational unit (OU).
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withAutoDeployment(AutoDeployment autoDeployment) {
setAutoDeployment(autoDeployment);
return this;
}
/**
*
* Describes how the IAM roles required for stack set operations are created.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* @param permissionModel
* Describes how the IAM roles required for stack set operations are created.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required
* to deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @see PermissionModels
*/
public void setPermissionModel(String permissionModel) {
this.permissionModel = permissionModel;
}
/**
*
* Describes how the IAM roles required for stack set operations are created.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* @return Describes how the IAM roles required for stack set operations are created.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles
* required to deploy to target accounts. For more information, see Grant Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @see PermissionModels
*/
public String getPermissionModel() {
return this.permissionModel;
}
/**
*
* Describes how the IAM roles required for stack set operations are created.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* @param permissionModel
* Describes how the IAM roles required for stack set operations are created.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required
* to deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PermissionModels
*/
public StackSet withPermissionModel(String permissionModel) {
setPermissionModel(permissionModel);
return this;
}
/**
*
* Describes how the IAM roles required for stack set operations are created.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* @param permissionModel
* Describes how the IAM roles required for stack set operations are created.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required
* to deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @see PermissionModels
*/
public void setPermissionModel(PermissionModels permissionModel) {
withPermissionModel(permissionModel);
}
/**
*
* Describes how the IAM roles required for stack set operations are created.
*
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required to
* deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to deploy
* to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
*
*
* @param permissionModel
* Describes how the IAM roles required for stack set operations are created.
*
* -
*
* With self-managed
permissions, you must create the administrator and execution roles required
* to deploy to target accounts. For more information, see Grant
* Self-Managed Stack Set Permissions.
*
*
* -
*
* With service-managed
permissions, StackSets automatically creates the IAM roles required to
* deploy to accounts managed by Organizations. For more information, see Grant Service-Managed Stack Set Permissions.
*
*
* @return Returns a reference to this object so that method calls can be chained together.
* @see PermissionModels
*/
public StackSet withPermissionModel(PermissionModels permissionModel) {
this.permissionModel = permissionModel.toString();
return this;
}
/**
*
* [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified for
* DeploymentTargets.
*
*
* @return [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified
* for DeploymentTargets.
*/
public java.util.List getOrganizationalUnitIds() {
if (organizationalUnitIds == null) {
organizationalUnitIds = new com.amazonaws.internal.SdkInternalList();
}
return organizationalUnitIds;
}
/**
*
* [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified for
* DeploymentTargets.
*
*
* @param organizationalUnitIds
* [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified
* for
* DeploymentTargets.
*/
public void setOrganizationalUnitIds(java.util.Collection organizationalUnitIds) {
if (organizationalUnitIds == null) {
this.organizationalUnitIds = null;
return;
}
this.organizationalUnitIds = new com.amazonaws.internal.SdkInternalList(organizationalUnitIds);
}
/**
*
* [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified for
* DeploymentTargets.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setOrganizationalUnitIds(java.util.Collection)} or
* {@link #withOrganizationalUnitIds(java.util.Collection)} if you want to override the existing values.
*
*
* @param organizationalUnitIds
* [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified
* for
* DeploymentTargets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withOrganizationalUnitIds(String... organizationalUnitIds) {
if (this.organizationalUnitIds == null) {
setOrganizationalUnitIds(new com.amazonaws.internal.SdkInternalList(organizationalUnitIds.length));
}
for (String ele : organizationalUnitIds) {
this.organizationalUnitIds.add(ele);
}
return this;
}
/**
*
* [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified for
* DeploymentTargets.
*
*
* @param organizationalUnitIds
* [Service-managed permissions] The organization root ID or organizational unit (OU) IDs that you specified
* for
* DeploymentTargets.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withOrganizationalUnitIds(java.util.Collection organizationalUnitIds) {
setOrganizationalUnitIds(organizationalUnitIds);
return this;
}
/**
*
* Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting operations.
*
*
* @param managedExecution
* Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting
* operations.
*/
public void setManagedExecution(ManagedExecution managedExecution) {
this.managedExecution = managedExecution;
}
/**
*
* Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting operations.
*
*
* @return Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting
* operations.
*/
public ManagedExecution getManagedExecution() {
return this.managedExecution;
}
/**
*
* Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting operations.
*
*
* @param managedExecution
* Describes whether StackSets performs non-conflicting operations concurrently and queues conflicting
* operations.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withManagedExecution(ManagedExecution managedExecution) {
setManagedExecution(managedExecution);
return this;
}
/**
*
* Returns a list of all Amazon Web Services Regions the given StackSet has stack instances deployed in. The Amazon
* Web Services Regions list output is in no particular order.
*
*
* @return Returns a list of all Amazon Web Services Regions the given StackSet has stack instances deployed in. The
* Amazon Web Services Regions list output is in no particular order.
*/
public java.util.List getRegions() {
if (regions == null) {
regions = new com.amazonaws.internal.SdkInternalList();
}
return regions;
}
/**
*
* Returns a list of all Amazon Web Services Regions the given StackSet has stack instances deployed in. The Amazon
* Web Services Regions list output is in no particular order.
*
*
* @param regions
* Returns a list of all Amazon Web Services Regions the given StackSet has stack instances deployed in. The
* Amazon Web Services Regions list output is in no particular order.
*/
public void setRegions(java.util.Collection regions) {
if (regions == null) {
this.regions = null;
return;
}
this.regions = new com.amazonaws.internal.SdkInternalList(regions);
}
/**
*
* Returns a list of all Amazon Web Services Regions the given StackSet has stack instances deployed in. The Amazon
* Web Services Regions list output is in no particular order.
*
*
* NOTE: This method appends the values to the existing list (if any). Use
* {@link #setRegions(java.util.Collection)} or {@link #withRegions(java.util.Collection)} if you want to override
* the existing values.
*
*
* @param regions
* Returns a list of all Amazon Web Services Regions the given StackSet has stack instances deployed in. The
* Amazon Web Services Regions list output is in no particular order.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withRegions(String... regions) {
if (this.regions == null) {
setRegions(new com.amazonaws.internal.SdkInternalList(regions.length));
}
for (String ele : regions) {
this.regions.add(ele);
}
return this;
}
/**
*
* Returns a list of all Amazon Web Services Regions the given StackSet has stack instances deployed in. The Amazon
* Web Services Regions list output is in no particular order.
*
*
* @param regions
* Returns a list of all Amazon Web Services Regions the given StackSet has stack instances deployed in. The
* Amazon Web Services Regions list output is in no particular order.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public StackSet withRegions(java.util.Collection regions) {
setRegions(regions);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getStackSetName() != null)
sb.append("StackSetName: ").append(getStackSetName()).append(",");
if (getStackSetId() != null)
sb.append("StackSetId: ").append(getStackSetId()).append(",");
if (getDescription() != null)
sb.append("Description: ").append(getDescription()).append(",");
if (getStatus() != null)
sb.append("Status: ").append(getStatus()).append(",");
if (getTemplateBody() != null)
sb.append("TemplateBody: ").append(getTemplateBody()).append(",");
if (getParameters() != null)
sb.append("Parameters: ").append(getParameters()).append(",");
if (getCapabilities() != null)
sb.append("Capabilities: ").append(getCapabilities()).append(",");
if (getTags() != null)
sb.append("Tags: ").append(getTags()).append(",");
if (getStackSetARN() != null)
sb.append("StackSetARN: ").append(getStackSetARN()).append(",");
if (getAdministrationRoleARN() != null)
sb.append("AdministrationRoleARN: ").append(getAdministrationRoleARN()).append(",");
if (getExecutionRoleName() != null)
sb.append("ExecutionRoleName: ").append(getExecutionRoleName()).append(",");
if (getStackSetDriftDetectionDetails() != null)
sb.append("StackSetDriftDetectionDetails: ").append(getStackSetDriftDetectionDetails()).append(",");
if (getAutoDeployment() != null)
sb.append("AutoDeployment: ").append(getAutoDeployment()).append(",");
if (getPermissionModel() != null)
sb.append("PermissionModel: ").append(getPermissionModel()).append(",");
if (getOrganizationalUnitIds() != null)
sb.append("OrganizationalUnitIds: ").append(getOrganizationalUnitIds()).append(",");
if (getManagedExecution() != null)
sb.append("ManagedExecution: ").append(getManagedExecution()).append(",");
if (getRegions() != null)
sb.append("Regions: ").append(getRegions());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof StackSet == false)
return false;
StackSet other = (StackSet) obj;
if (other.getStackSetName() == null ^ this.getStackSetName() == null)
return false;
if (other.getStackSetName() != null && other.getStackSetName().equals(this.getStackSetName()) == false)
return false;
if (other.getStackSetId() == null ^ this.getStackSetId() == null)
return false;
if (other.getStackSetId() != null && other.getStackSetId().equals(this.getStackSetId()) == false)
return false;
if (other.getDescription() == null ^ this.getDescription() == null)
return false;
if (other.getDescription() != null && other.getDescription().equals(this.getDescription()) == false)
return false;
if (other.getStatus() == null ^ this.getStatus() == null)
return false;
if (other.getStatus() != null && other.getStatus().equals(this.getStatus()) == false)
return false;
if (other.getTemplateBody() == null ^ this.getTemplateBody() == null)
return false;
if (other.getTemplateBody() != null && other.getTemplateBody().equals(this.getTemplateBody()) == false)
return false;
if (other.getParameters() == null ^ this.getParameters() == null)
return false;
if (other.getParameters() != null && other.getParameters().equals(this.getParameters()) == false)
return false;
if (other.getCapabilities() == null ^ this.getCapabilities() == null)
return false;
if (other.getCapabilities() != null && other.getCapabilities().equals(this.getCapabilities()) == false)
return false;
if (other.getTags() == null ^ this.getTags() == null)
return false;
if (other.getTags() != null && other.getTags().equals(this.getTags()) == false)
return false;
if (other.getStackSetARN() == null ^ this.getStackSetARN() == null)
return false;
if (other.getStackSetARN() != null && other.getStackSetARN().equals(this.getStackSetARN()) == false)
return false;
if (other.getAdministrationRoleARN() == null ^ this.getAdministrationRoleARN() == null)
return false;
if (other.getAdministrationRoleARN() != null && other.getAdministrationRoleARN().equals(this.getAdministrationRoleARN()) == false)
return false;
if (other.getExecutionRoleName() == null ^ this.getExecutionRoleName() == null)
return false;
if (other.getExecutionRoleName() != null && other.getExecutionRoleName().equals(this.getExecutionRoleName()) == false)
return false;
if (other.getStackSetDriftDetectionDetails() == null ^ this.getStackSetDriftDetectionDetails() == null)
return false;
if (other.getStackSetDriftDetectionDetails() != null
&& other.getStackSetDriftDetectionDetails().equals(this.getStackSetDriftDetectionDetails()) == false)
return false;
if (other.getAutoDeployment() == null ^ this.getAutoDeployment() == null)
return false;
if (other.getAutoDeployment() != null && other.getAutoDeployment().equals(this.getAutoDeployment()) == false)
return false;
if (other.getPermissionModel() == null ^ this.getPermissionModel() == null)
return false;
if (other.getPermissionModel() != null && other.getPermissionModel().equals(this.getPermissionModel()) == false)
return false;
if (other.getOrganizationalUnitIds() == null ^ this.getOrganizationalUnitIds() == null)
return false;
if (other.getOrganizationalUnitIds() != null && other.getOrganizationalUnitIds().equals(this.getOrganizationalUnitIds()) == false)
return false;
if (other.getManagedExecution() == null ^ this.getManagedExecution() == null)
return false;
if (other.getManagedExecution() != null && other.getManagedExecution().equals(this.getManagedExecution()) == false)
return false;
if (other.getRegions() == null ^ this.getRegions() == null)
return false;
if (other.getRegions() != null && other.getRegions().equals(this.getRegions()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getStackSetName() == null) ? 0 : getStackSetName().hashCode());
hashCode = prime * hashCode + ((getStackSetId() == null) ? 0 : getStackSetId().hashCode());
hashCode = prime * hashCode + ((getDescription() == null) ? 0 : getDescription().hashCode());
hashCode = prime * hashCode + ((getStatus() == null) ? 0 : getStatus().hashCode());
hashCode = prime * hashCode + ((getTemplateBody() == null) ? 0 : getTemplateBody().hashCode());
hashCode = prime * hashCode + ((getParameters() == null) ? 0 : getParameters().hashCode());
hashCode = prime * hashCode + ((getCapabilities() == null) ? 0 : getCapabilities().hashCode());
hashCode = prime * hashCode + ((getTags() == null) ? 0 : getTags().hashCode());
hashCode = prime * hashCode + ((getStackSetARN() == null) ? 0 : getStackSetARN().hashCode());
hashCode = prime * hashCode + ((getAdministrationRoleARN() == null) ? 0 : getAdministrationRoleARN().hashCode());
hashCode = prime * hashCode + ((getExecutionRoleName() == null) ? 0 : getExecutionRoleName().hashCode());
hashCode = prime * hashCode + ((getStackSetDriftDetectionDetails() == null) ? 0 : getStackSetDriftDetectionDetails().hashCode());
hashCode = prime * hashCode + ((getAutoDeployment() == null) ? 0 : getAutoDeployment().hashCode());
hashCode = prime * hashCode + ((getPermissionModel() == null) ? 0 : getPermissionModel().hashCode());
hashCode = prime * hashCode + ((getOrganizationalUnitIds() == null) ? 0 : getOrganizationalUnitIds().hashCode());
hashCode = prime * hashCode + ((getManagedExecution() == null) ? 0 : getManagedExecution().hashCode());
hashCode = prime * hashCode + ((getRegions() == null) ? 0 : getRegions().hashCode());
return hashCode;
}
@Override
public StackSet clone() {
try {
return (StackSet) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}