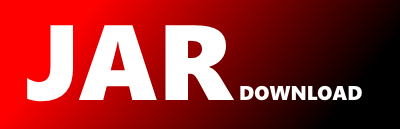
com.amazonaws.services.cloudfront.model.OriginRequestPolicyConfig Maven / Gradle / Ivy
/*
* Copyright 2016-2021 Amazon.com, Inc. or its affiliates. All Rights Reserved.
*
* Licensed under the Apache License, Version 2.0 (the "License"). You may not use this file except in compliance with
* the License. A copy of the License is located at
*
* http://aws.amazon.com/apache2.0
*
* or in the "license" file accompanying this file. This file is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR
* CONDITIONS OF ANY KIND, either express or implied. See the License for the specific language governing permissions
* and limitations under the License.
*/
package com.amazonaws.services.cloudfront.model;
import java.io.Serializable;
import javax.annotation.Generated;
/**
*
* An origin request policy configuration.
*
*
* This configuration determines the values that CloudFront includes in requests that it sends to the origin. Each
* request that CloudFront sends to the origin includes the following:
*
*
* -
*
* The request body and the URL path (without the domain name) from the viewer request.
*
*
* -
*
* The headers that CloudFront automatically includes in every origin request, including Host
,
* User-Agent
, and X-Amz-Cf-Id
.
*
*
* -
*
* All HTTP headers, cookies, and URL query strings that are specified in the cache policy or the origin request policy.
* These can include items from the viewer request and, in the case of headers, additional ones that are added by
* CloudFront.
*
*
*
*
* CloudFront sends a request when it can’t find an object in its cache that matches the request. If you want to send
* values to the origin and also include them in the cache key, use CachePolicy
.
*
*
* @see AWS API Documentation
*/
@Generated("com.amazonaws:aws-java-sdk-code-generator")
public class OriginRequestPolicyConfig implements Serializable, Cloneable {
/**
*
* A comment to describe the origin request policy. The comment cannot be longer than 128 characters.
*
*/
private String comment;
/**
*
* A unique name to identify the origin request policy.
*
*/
private String name;
/**
*
* The HTTP headers to include in origin requests. These can include headers from viewer requests and additional
* headers added by CloudFront.
*
*/
private OriginRequestPolicyHeadersConfig headersConfig;
/**
*
* The cookies from viewer requests to include in origin requests.
*
*/
private OriginRequestPolicyCookiesConfig cookiesConfig;
/**
*
* The URL query strings from viewer requests to include in origin requests.
*
*/
private OriginRequestPolicyQueryStringsConfig queryStringsConfig;
/**
*
* A comment to describe the origin request policy. The comment cannot be longer than 128 characters.
*
*
* @param comment
* A comment to describe the origin request policy. The comment cannot be longer than 128 characters.
*/
public void setComment(String comment) {
this.comment = comment;
}
/**
*
* A comment to describe the origin request policy. The comment cannot be longer than 128 characters.
*
*
* @return A comment to describe the origin request policy. The comment cannot be longer than 128 characters.
*/
public String getComment() {
return this.comment;
}
/**
*
* A comment to describe the origin request policy. The comment cannot be longer than 128 characters.
*
*
* @param comment
* A comment to describe the origin request policy. The comment cannot be longer than 128 characters.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OriginRequestPolicyConfig withComment(String comment) {
setComment(comment);
return this;
}
/**
*
* A unique name to identify the origin request policy.
*
*
* @param name
* A unique name to identify the origin request policy.
*/
public void setName(String name) {
this.name = name;
}
/**
*
* A unique name to identify the origin request policy.
*
*
* @return A unique name to identify the origin request policy.
*/
public String getName() {
return this.name;
}
/**
*
* A unique name to identify the origin request policy.
*
*
* @param name
* A unique name to identify the origin request policy.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OriginRequestPolicyConfig withName(String name) {
setName(name);
return this;
}
/**
*
* The HTTP headers to include in origin requests. These can include headers from viewer requests and additional
* headers added by CloudFront.
*
*
* @param headersConfig
* The HTTP headers to include in origin requests. These can include headers from viewer requests and
* additional headers added by CloudFront.
*/
public void setHeadersConfig(OriginRequestPolicyHeadersConfig headersConfig) {
this.headersConfig = headersConfig;
}
/**
*
* The HTTP headers to include in origin requests. These can include headers from viewer requests and additional
* headers added by CloudFront.
*
*
* @return The HTTP headers to include in origin requests. These can include headers from viewer requests and
* additional headers added by CloudFront.
*/
public OriginRequestPolicyHeadersConfig getHeadersConfig() {
return this.headersConfig;
}
/**
*
* The HTTP headers to include in origin requests. These can include headers from viewer requests and additional
* headers added by CloudFront.
*
*
* @param headersConfig
* The HTTP headers to include in origin requests. These can include headers from viewer requests and
* additional headers added by CloudFront.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OriginRequestPolicyConfig withHeadersConfig(OriginRequestPolicyHeadersConfig headersConfig) {
setHeadersConfig(headersConfig);
return this;
}
/**
*
* The cookies from viewer requests to include in origin requests.
*
*
* @param cookiesConfig
* The cookies from viewer requests to include in origin requests.
*/
public void setCookiesConfig(OriginRequestPolicyCookiesConfig cookiesConfig) {
this.cookiesConfig = cookiesConfig;
}
/**
*
* The cookies from viewer requests to include in origin requests.
*
*
* @return The cookies from viewer requests to include in origin requests.
*/
public OriginRequestPolicyCookiesConfig getCookiesConfig() {
return this.cookiesConfig;
}
/**
*
* The cookies from viewer requests to include in origin requests.
*
*
* @param cookiesConfig
* The cookies from viewer requests to include in origin requests.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OriginRequestPolicyConfig withCookiesConfig(OriginRequestPolicyCookiesConfig cookiesConfig) {
setCookiesConfig(cookiesConfig);
return this;
}
/**
*
* The URL query strings from viewer requests to include in origin requests.
*
*
* @param queryStringsConfig
* The URL query strings from viewer requests to include in origin requests.
*/
public void setQueryStringsConfig(OriginRequestPolicyQueryStringsConfig queryStringsConfig) {
this.queryStringsConfig = queryStringsConfig;
}
/**
*
* The URL query strings from viewer requests to include in origin requests.
*
*
* @return The URL query strings from viewer requests to include in origin requests.
*/
public OriginRequestPolicyQueryStringsConfig getQueryStringsConfig() {
return this.queryStringsConfig;
}
/**
*
* The URL query strings from viewer requests to include in origin requests.
*
*
* @param queryStringsConfig
* The URL query strings from viewer requests to include in origin requests.
* @return Returns a reference to this object so that method calls can be chained together.
*/
public OriginRequestPolicyConfig withQueryStringsConfig(OriginRequestPolicyQueryStringsConfig queryStringsConfig) {
setQueryStringsConfig(queryStringsConfig);
return this;
}
/**
* Returns a string representation of this object. This is useful for testing and debugging. Sensitive data will be
* redacted from this string using a placeholder value.
*
* @return A string representation of this object.
*
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("{");
if (getComment() != null)
sb.append("Comment: ").append(getComment()).append(",");
if (getName() != null)
sb.append("Name: ").append(getName()).append(",");
if (getHeadersConfig() != null)
sb.append("HeadersConfig: ").append(getHeadersConfig()).append(",");
if (getCookiesConfig() != null)
sb.append("CookiesConfig: ").append(getCookiesConfig()).append(",");
if (getQueryStringsConfig() != null)
sb.append("QueryStringsConfig: ").append(getQueryStringsConfig());
sb.append("}");
return sb.toString();
}
@Override
public boolean equals(Object obj) {
if (this == obj)
return true;
if (obj == null)
return false;
if (obj instanceof OriginRequestPolicyConfig == false)
return false;
OriginRequestPolicyConfig other = (OriginRequestPolicyConfig) obj;
if (other.getComment() == null ^ this.getComment() == null)
return false;
if (other.getComment() != null && other.getComment().equals(this.getComment()) == false)
return false;
if (other.getName() == null ^ this.getName() == null)
return false;
if (other.getName() != null && other.getName().equals(this.getName()) == false)
return false;
if (other.getHeadersConfig() == null ^ this.getHeadersConfig() == null)
return false;
if (other.getHeadersConfig() != null && other.getHeadersConfig().equals(this.getHeadersConfig()) == false)
return false;
if (other.getCookiesConfig() == null ^ this.getCookiesConfig() == null)
return false;
if (other.getCookiesConfig() != null && other.getCookiesConfig().equals(this.getCookiesConfig()) == false)
return false;
if (other.getQueryStringsConfig() == null ^ this.getQueryStringsConfig() == null)
return false;
if (other.getQueryStringsConfig() != null && other.getQueryStringsConfig().equals(this.getQueryStringsConfig()) == false)
return false;
return true;
}
@Override
public int hashCode() {
final int prime = 31;
int hashCode = 1;
hashCode = prime * hashCode + ((getComment() == null) ? 0 : getComment().hashCode());
hashCode = prime * hashCode + ((getName() == null) ? 0 : getName().hashCode());
hashCode = prime * hashCode + ((getHeadersConfig() == null) ? 0 : getHeadersConfig().hashCode());
hashCode = prime * hashCode + ((getCookiesConfig() == null) ? 0 : getCookiesConfig().hashCode());
hashCode = prime * hashCode + ((getQueryStringsConfig() == null) ? 0 : getQueryStringsConfig().hashCode());
return hashCode;
}
@Override
public OriginRequestPolicyConfig clone() {
try {
return (OriginRequestPolicyConfig) super.clone();
} catch (CloneNotSupportedException e) {
throw new IllegalStateException("Got a CloneNotSupportedException from Object.clone() " + "even though we're Cloneable!", e);
}
}
}